<?php
/**
* PHPMailer - PHP email creation and transport class.
* PHP Version 5.5.
*
* @see https://github.com/PHPMailer/PHPMailer/ The PHPMailer GitHub project
*
* @author Marcus Bointon (Synchro/coolbru) <phpmailer@synchromedia.co.uk>
* @author Jim Jagielski (jimjag) <jimjag@gmail.com>
* @author Andy Prevost (codeworxtech) <codeworxtech@users.sourceforge.net>
* @author Brent R. Matzelle (original founder)
* @copyright 2012 - 2020 Marcus Bointon
* @copyright 2010 - 2012 Jim Jagielski
* @copyright 2004 - 2009 Andy Prevost
* @license http://www.gnu.org/copyleft/lesser.html GNU Lesser General Public License
* @note This program is distributed in the hope that it will be useful - WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE.
*/
namespace PHPMailer\PHPMailer;
/**
* PHPMailer - PHP email creation and transport class.
*
* @author Marcus Bointon (Synchro/coolbru) <phpmailer@synchromedia.co.uk>
* @author Jim Jagielski (jimjag) <jimjag@gmail.com>
* @author Andy Prevost (codeworxtech) <codeworxtech@users.sourceforge.net>
* @author Brent R. Matzelle (original founder)
*/
class PHPMailer
{
const CHARSET_ASCII = 'us-ascii';
const CHARSET_ISO88591 = 'iso-8859-1';
const CHARSET_UTF8 = 'utf-8';
const CONTENT_TYPE_PLAINTEXT = 'text/plain';
const CONTENT_TYPE_TEXT_CALENDAR = 'text/calendar';
const CONTENT_TYPE_TEXT_HTML = 'text/html';
const CONTENT_TYPE_MULTIPART_ALTERNATIVE = 'multipart/alternative';
const CONTENT_TYPE_MULTIPART_MIXED = 'multipart/mixed';
const CONTENT_TYPE_MULTIPART_RELATED = 'multipart/related';
const ENCODING_7BIT = '7bit';
const ENCODING_8BIT = '8bit';
const ENCODING_BASE64 = 'base64';
const ENCODING_BINARY = 'binary';
const ENCODING_QUOTED_PRINTABLE = 'quoted-printable';
const ENCRYPTION_STARTTLS = 'tls';
const ENCRYPTION_SMTPS = 'ssl';
const ICAL_METHOD_REQUEST = 'REQUEST';
const ICAL_METHOD_PUBLISH = 'PUBLISH';
const ICAL_METHOD_REPLY = 'REPLY';
const ICAL_METHOD_ADD = 'ADD';
const ICAL_METHOD_CANCEL = 'CANCEL';
const ICAL_METHOD_REFRESH = 'REFRESH';
const ICAL_METHOD_COUNTER = 'COUNTER';
const ICAL_METHOD_DECLINECOUNTER = 'DECLINECOUNTER';
/**
* Email priority.
* Options: null (default), 1 = High, 3 = Normal, 5 = low.
* When null, the header is not set at all.
*
* @var int|null
*/
public $Priority;
/**
* The character set of the message.
*
* @var string
*/
public $CharSet = self::CHARSET_ISO88591;
/**
* The MIME Content-type of the message.
*
* @var string
*/
public $ContentType = self::CONTENT_TYPE_PLAINTEXT;
/**
* The message encoding.
* Options: "8bit", "7bit", "binary", "base64", and "quoted-printable".
*
* @var string
*/
public $Encoding = self::ENCODING_8BIT;
/**
* Holds the most recent mailer error message.
*
* @var string
*/
public $ErrorInfo = '';
/**
* The From email address for the message.
*
* @var string
*/
public $From = 'root@localhost';
/**
* The From name of the message.
*
* @var string
*/
public $FromName = 'Root User';
/**
* The envelope sender of the message.
* This will usually be turned into a Return-Path header by the receiver,
* and is the address that bounces will be sent to.
* If not empty, will be passed via `-f` to sendmail or as the 'MAIL FROM' value over SMTP.
*
* @var string
*/
public $Sender = '';
/**
* The Subject of the message.
*
* @var string
*/
public $Subject = '';
/**
* An HTML or plain text message body.
* If HTML then call isHTML(true).
*
* @var string
*/
public $Body = '';
/**
* The plain-text message body.
* This body can be read by mail clients that do not have HTML email
* capability such as mutt & Eudora.
* Clients that can read HTML will view the normal Body.
*
* @var string
*/
public $AltBody = '';
/**
* An iCal message part body.
* Only supported in simple alt or alt_inline message types
* To generate iCal event structures, use classes like EasyPeasyICS or iCalcreator.
*
* @see http://sprain.ch/blog/downloads/php-class-easypeasyics-create-ical-files-with-php/
* @see http://kigkonsult.se/iCalcreator/
*
* @var string
*/
public $Ical = '';
/**
* Value-array of "method" in Contenttype header "text/calendar"
*
* @var string[]
*/
protected static $IcalMethods = [
self::ICAL_METHOD_REQUEST,
self::ICAL_METHOD_PUBLISH,
self::ICAL_METHOD_REPLY,
self::ICAL_METHOD_ADD,
self::ICAL_METHOD_CANCEL,
self::ICAL_METHOD_REFRESH,
self::ICAL_METHOD_COUNTER,
self::ICAL_METHOD_DECLINECOUNTER,
];
/**
* The complete compiled MIME message body.
*
* @var string
*/
protected $MIMEBody = '';
/**
* The complete compiled MIME message headers.
*
* @var string
*/
protected $MIMEHeader = '';
/**
* Extra headers that createHeader() doesn't fold in.
*
* @var string
*/
protected $mailHeader = '';
/**
* Word-wrap the message body to this number of chars.
* Set to 0 to not wrap. A useful value here is 78, for RFC2822 section 2.1.1 compliance.
*
* @see static::STD_LINE_LENGTH
*
* @var int
*/
public $WordWrap = 0;
/**
* Which method to use to send mail.
* Options: "mail", "sendmail", or "smtp".
*
* @var string
*/
public $Mailer = 'mail';
/**
* The path to the sendmail program.
*
* @var string
*/
public $Sendmail = '/usr/sbin/sendmail';
/**
* Whether mail() uses a fully sendmail-compatible MTA.
* One which supports sendmail's "-oi -f" options.
*
* @var bool
*/
public $UseSendmailOptions = true;
/**
* The email address that a reading confirmation should be sent to, also known as read receipt.
*
* @var string
*/
public $ConfirmReadingTo = '';
/**
* The hostname to use in the Message-ID header and as default HELO string.
* If empty, PHPMailer attempts to find one with, in order,
* $_SERVER['SERVER_NAME'], gethostname(), php_uname('n'), or the value
* 'localhost.localdomain'.
*
* @see PHPMailer::$Helo
*
* @var string
*/
public $Hostname = '';
/**
* An ID to be used in the Message-ID header.
* If empty, a unique id will be generated.
* You can set your own, but it must be in the format "<id@domain>",
* as defined in RFC5322 section 3.6.4 or it will be ignored.
*
* @see https://tools.ietf.org/html/rfc5322#section-3.6.4
*
* @var string
*/
public $MessageID = '';
/**
* The message Date to be used in the Date header.
* If empty, the current date will be added.
*
* @var string
*/
public $MessageDate = '';
/**
* SMTP hosts.
* Either a single hostname or multiple semicolon-delimited hostnames.
* You can also specify a different port
* for each host by using this format: [hostname:port]
* (e.g. "smtp1.example.com:25;smtp2.example.com").
* You can also specify encryption type, for example:
* (e.g. "tls://smtp1.example.com:587;ssl://smtp2.example.com:465").
* Hosts will be tried in order.
*
* @var string
*/
public $Host = 'localhost';
/**
* The default SMTP server port.
*
* @var int
*/
public $Port = 25;
/**
* The SMTP HELO/EHLO name used for the SMTP connection.
* Default is $Hostname. If $Hostname is empty, PHPMailer attempts to f
没有合适的资源?快使用搜索试试~ 我知道了~
孜然导航系统 免授权多模板的导航网源码.zip
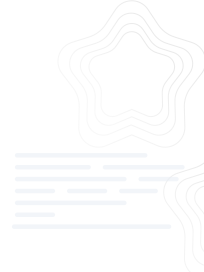
共1096个文件
js:358个
php:220个
gif:166个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 177 浏览量
2023-02-01
10:04:08
上传
评论
收藏 11.14MB ZIP 举报
温馨提示
孜然导航系统 是一个以PHP+MYSQL进行开发的网址导航系统,无需授权, 需要安装SG11插件,站内使用阿帕奇伪静态的配置好cofin.php文件然后直接访问即可,Nginx的话看文件里的文件。 安装比较简单,企业猫就没有写教程,php安装sg11。上传源码,访问域名进行安装即可。
资源推荐
资源详情
资源评论
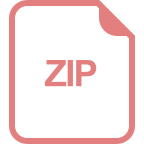
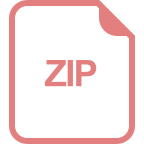
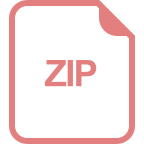
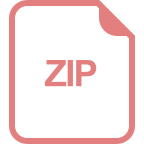
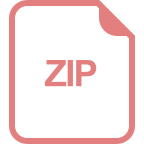
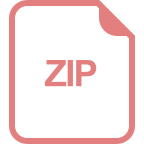
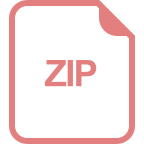
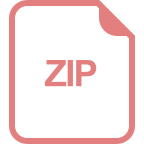
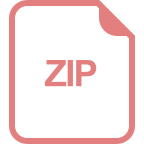
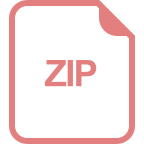
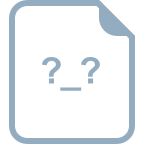
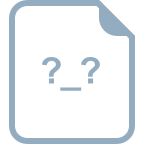
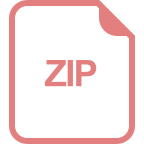
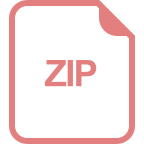
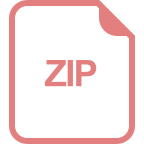
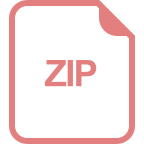
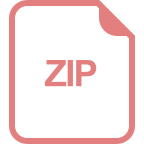
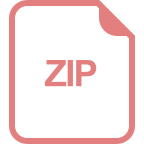
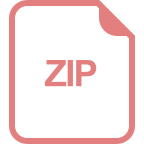
收起资源包目录

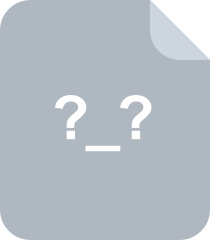
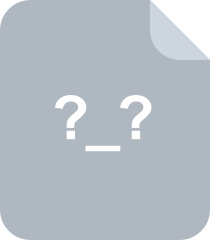
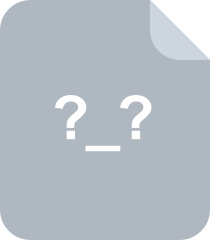
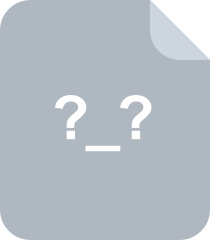
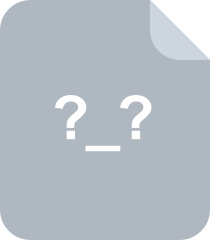
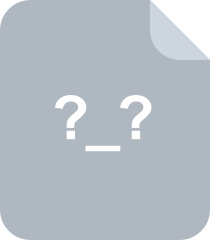
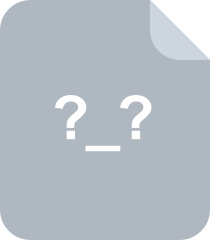
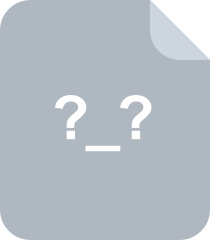
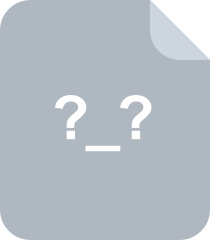
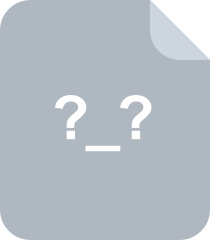
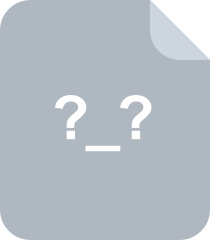
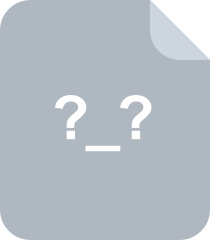
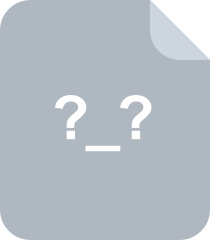
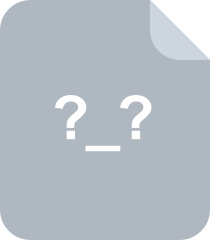
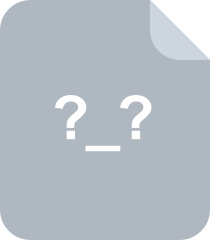
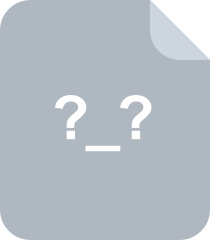
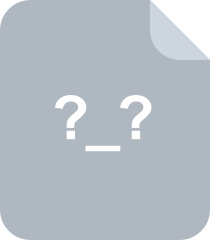
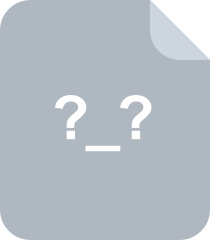
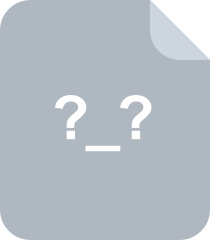
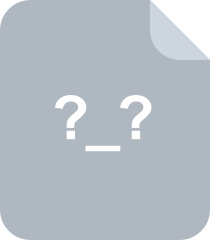
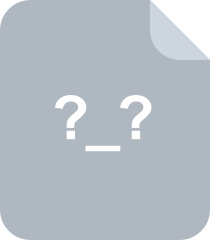
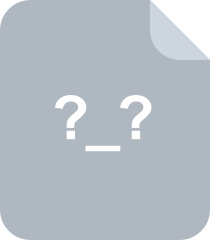
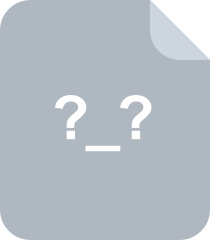
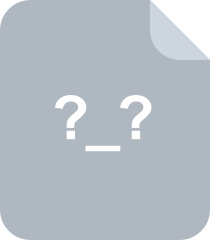
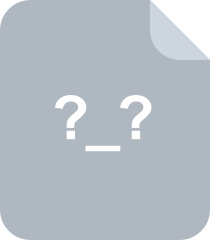
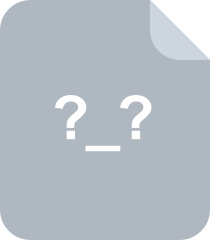
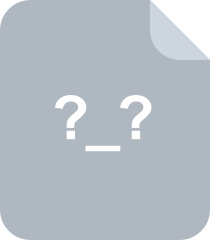
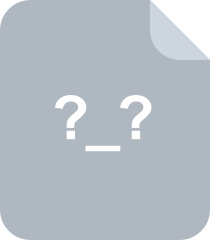
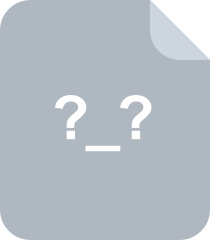
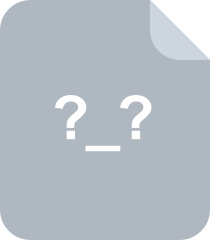
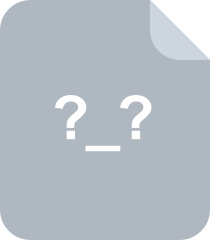
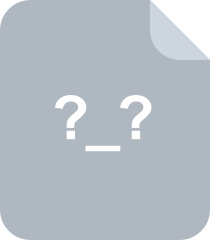
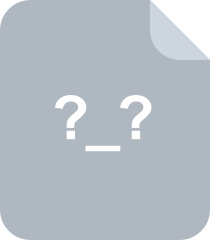
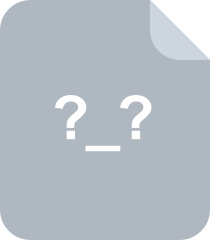
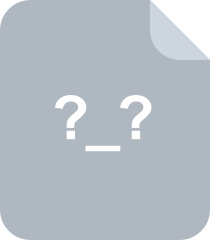
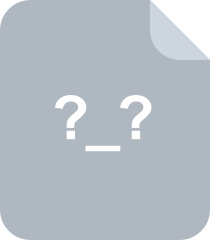
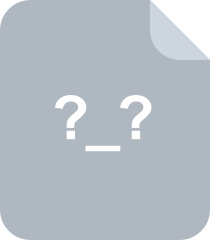
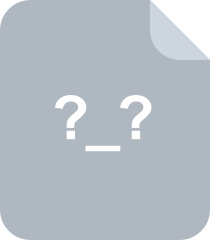
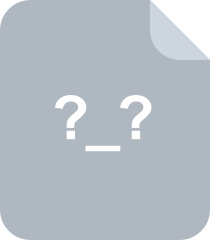
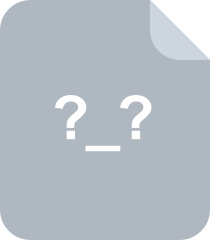
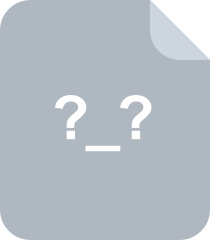
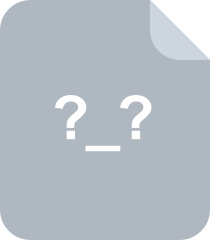
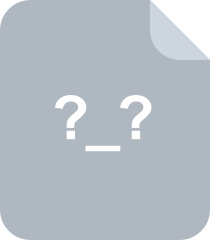
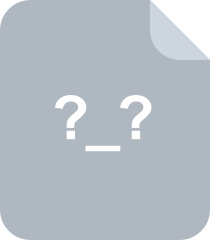
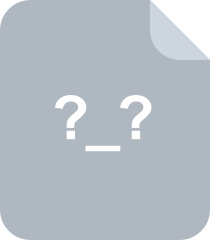
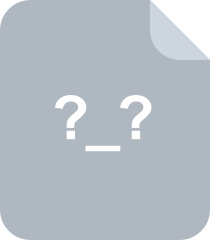
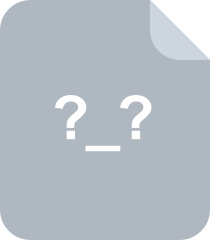
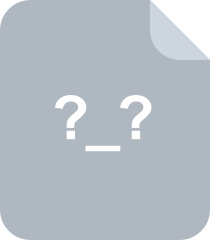
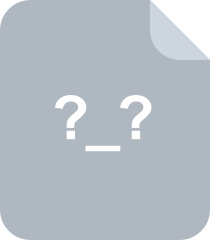
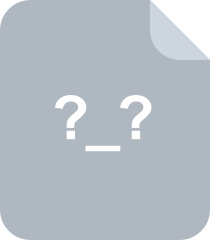
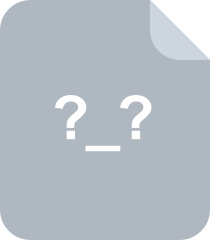
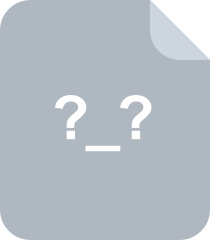
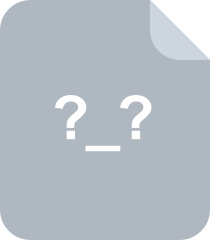
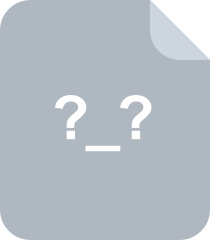
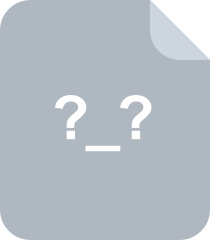
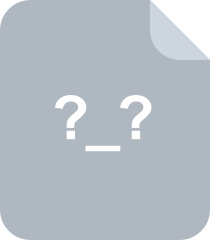
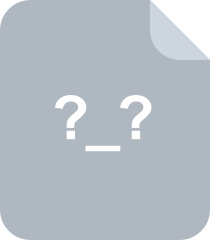
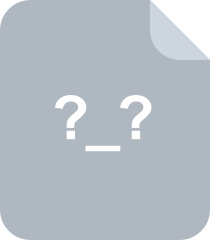
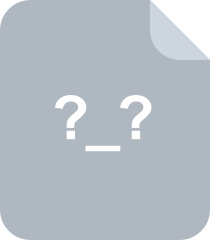
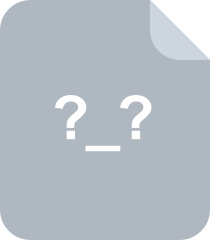
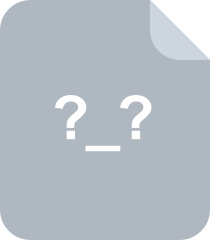
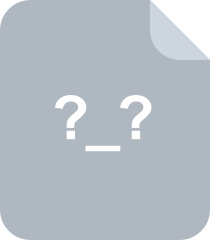
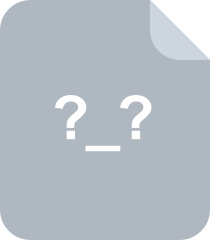
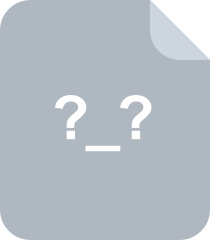
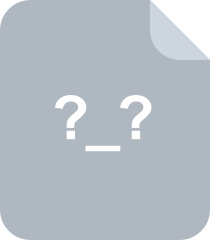
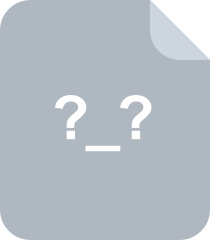
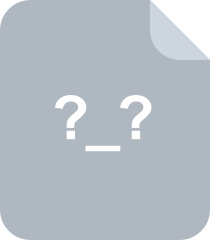
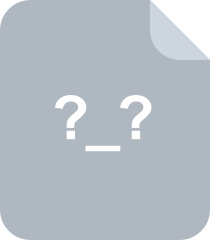
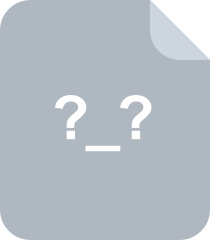
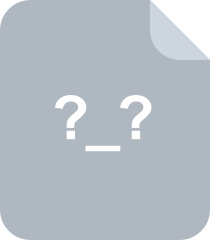
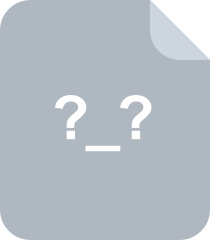
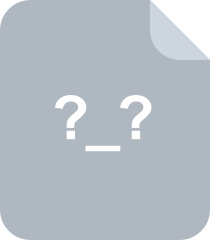
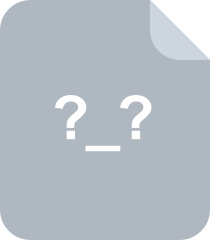
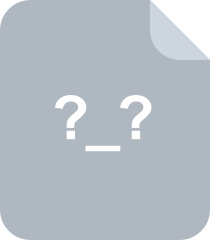
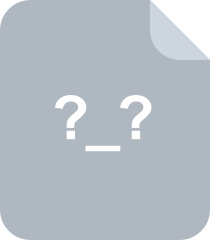
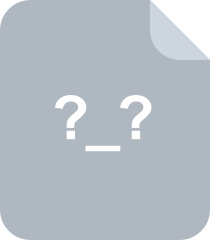
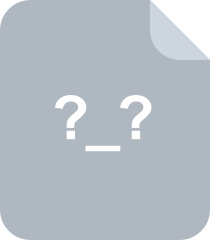
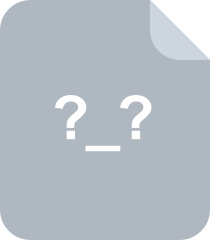
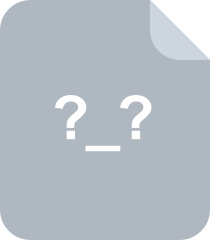
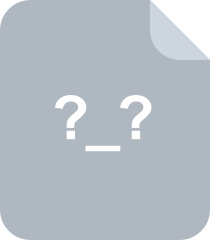
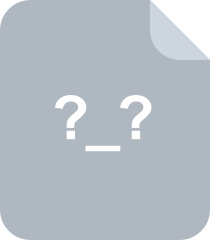
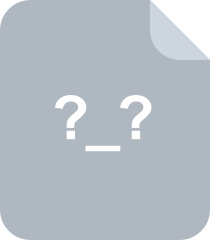
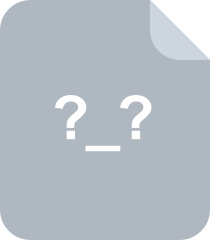
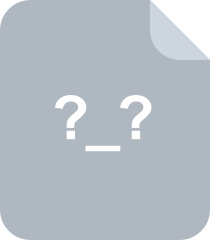
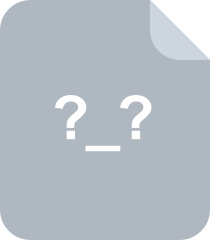
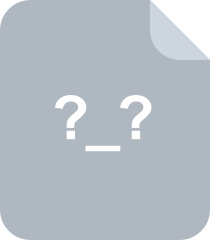
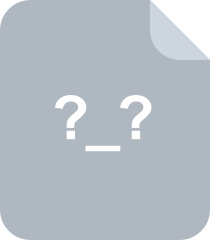
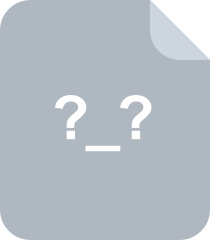
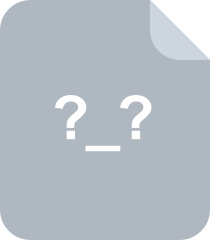
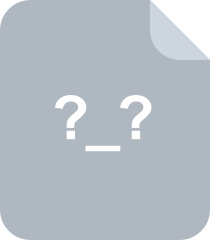
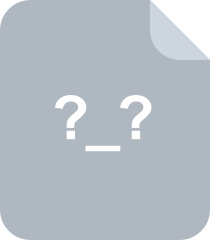
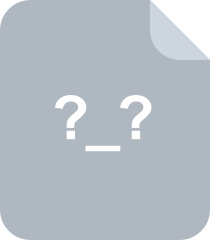
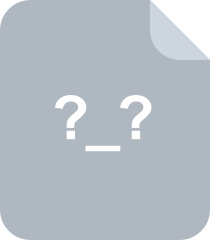
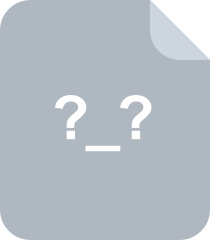
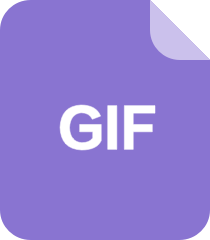
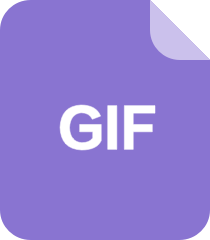
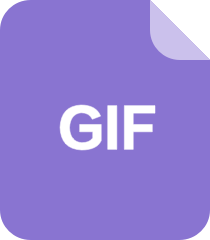
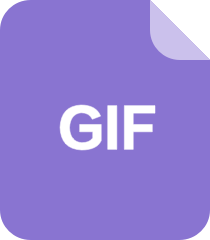
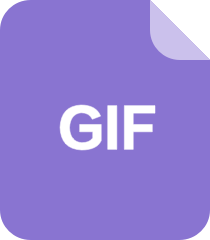
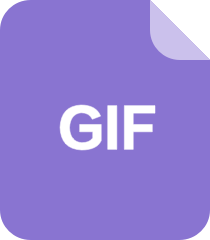
共 1096 条
- 1
- 2
- 3
- 4
- 5
- 6
- 11
资源评论
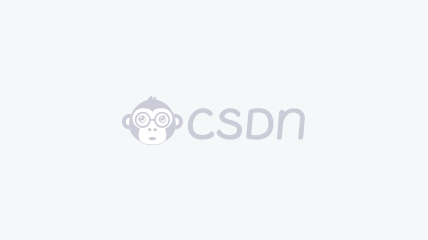

智慧浩海
- 粉丝: 1w+
- 资源: 5439

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

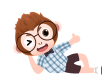
最新资源
- Chrome代理 switchyOmega
- GVC-全球价值链参与地位指数,基于ICIO表,(Wang等 2017a)计算方法
- 易语言ADS指纹浏览器管理工具
- 易语言奇易模块5.3.6
- cad定制家具平面图工具-(FG)门板覆盖柜体
- asp.net 原生js代码及HTML实现多文件分片上传功能(自定义上传文件大小、文件上传类型)
- whl@pip install pyaudio ERROR: Failed building wheel for pyaudio
- Constantsfd密钥和权限集合.kt
- 基于Java的财务报销管理系统后端开发源码
- 基于Python核心技术的cola项目设计源码介绍
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


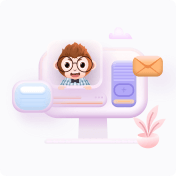
安全验证
文档复制为VIP权益,开通VIP直接复制
