<?php
/**
* PHP SDK for weibo.com (using OAuth2)
*
* @author Elmer Zhang <freeboy6716@gmail.com>
*/
/**
* @ignore
*/
class OAuthException extends Exception {
// pass
}
/**
* 新浪微博 OAuth 认证类(OAuth2)
*
* 授权机制说明请大家参考微博开放平台文档:{@link http://open.weibo.com/wiki/Oauth2}
*
* @package sae
* @author Elmer Zhang
* @version 1.0
*/
class SaeTOAuthV2 {
/**
* @ignore
*/
public $client_id;
/**
* @ignore
*/
public $client_secret;
/**
* @ignore
*/
public $access_token;
/**
* @ignore
*/
public $refresh_token;
/**
* Contains the last HTTP status code returned.
*
* @ignore
*/
public $http_code;
/**
* Contains the last API call.
*
* @ignore
*/
public $url;
/**
* Set up the API root URL.
*
* @ignore
*/
public $host = "https://api.weibo.com/2/";
/**
* Set timeout default.
*
* @ignore
*/
public $timeout = 30;
/**
* Set connect timeout.
*
* @ignore
*/
public $connecttimeout = 30;
/**
* Verify SSL Cert.
*
* @ignore
*/
public $ssl_verifypeer = FALSE;
/**
* Respons format.
*
* @ignore
*/
public $format = 'json';
/**
* Decode returned json data.
*
* @ignore
*/
public $decode_json = TRUE;
/**
* Contains the last HTTP headers returned.
*
* @ignore
*/
public $http_info;
/**
* Set the useragnet.
*
* @ignore
*/
public $useragent = 'Sae T OAuth2 v0.1';
/**
* print the debug info
*
* @ignore
*/
public $debug = FALSE;
/**
* boundary of multipart
* @ignore
*/
public static $boundary = '';
/**
* Set API URLS
*/
/**
* @ignore
*/
function accessTokenURL() { return 'https://api.weibo.com/oauth2/access_token'; }
/**
* @ignore
*/
function authorizeURL() { return 'https://api.weibo.com/oauth2/authorize'; }
/**
* construct WeiboOAuth object
*/
function __construct($client_id, $client_secret, $access_token = NULL, $refresh_token = NULL) {
$this->client_id = $client_id;
$this->client_secret = $client_secret;
$this->access_token = $access_token;
$this->refresh_token = $refresh_token;
}
/**
* authorize接口
*
* 对应API:{@link http://open.weibo.com/wiki/Oauth2/authorize Oauth2/authorize}
*
* @param string $url 授权后的回调地址,站外应用需与回调地址一致,站内应用需要填写canvas page的地址
* @param string $response_type 支持的值包括 code 和token 默认值为code
* @param string $state 用于保持请求和回调的状态。在回调时,会在Query Parameter中回传该参数
* @param string $display 授权页面类型 可选范围:
* - default 默认授权页面
* - mobile 支持html5的手机
* - popup 弹窗授权页
* - wap1.2 wap1.2页面
* - wap2.0 wap2.0页面
* - js js-sdk 专用 授权页面是弹窗,返回结果为js-sdk回掉函数
* - apponweibo 站内应用专用,站内应用不传display参数,并且response_type为token时,默认使用改display.授权后不会返回access_token,只是输出js刷新站内应用父框架
* @return array
*/
function getAuthorizeURL( $url, $response_type = 'code', $state = NULL, $display = NULL ) {
$params = array();
$params['client_id'] = $this->client_id;
$params['redirect_uri'] = $url;
$params['response_type'] = $response_type;
$params['state'] = $state;
$params['display'] = $display;
return $this->authorizeURL() . "?" . http_build_query($params);
}
/**
* access_token接口
*
* 对应API:{@link http://open.weibo.com/wiki/OAuth2/access_token OAuth2/access_token}
*
* @param string $type 请求的类型,可以为:code, password, token
* @param array $keys 其他参数:
* - 当$type为code时: array('code'=>..., 'redirect_uri'=>...)
* - 当$type为password时: array('username'=>..., 'password'=>...)
* - 当$type为token时: array('refresh_token'=>...)
* @return array
*/
function getAccessToken( $type = 'code', $keys ) {
$params = array();
$params['client_id'] = $this->client_id;
$params['client_secret'] = $this->client_secret;
if ( $type === 'token' ) {
$params['grant_type'] = 'refresh_token';
$params['refresh_token'] = $keys['refresh_token'];
} elseif ( $type === 'code' ) {
$params['grant_type'] = 'authorization_code';
$params['code'] = $keys['code'];
$params['redirect_uri'] = $keys['redirect_uri'];
} elseif ( $type === 'password' ) {
$params['grant_type'] = 'password';
$params['username'] = $keys['username'];
$params['password'] = $keys['password'];
} else {
throw new OAuthException("wrong auth type");
}
$response = $this->oAuthRequest($this->accessTokenURL(), 'POST', $params);
$token = json_decode($response, true);
if ( is_array($token) && !isset($token['error']) ) {
$this->access_token = $token['access_token'];
$this->refresh_token = $token['refresh_token'];
} else {
throw new OAuthException("get access token failed." . $token['error']);
}
return $token;
}
/**
* 解析 signed_request
*
* @param string $signed_request 应用框架在加载iframe时会通过向Canvas URL post的参数signed_request
*
* @return array
*/
function parseSignedRequest($signed_request) {
list($encoded_sig, $payload) = explode('.', $signed_request, 2);
$sig = self::base64decode($encoded_sig) ;
$data = json_decode(self::base64decode($payload), true);
if (strtoupper($data['algorithm']) !== 'HMAC-SHA256') return '-1';
$expected_sig = hash_hmac('sha256', $payload, $this->client_secret, true);
return ($sig !== $expected_sig)? '-2':$data;
}
/**
* @ignore
*/
function base64decode($str) {
return base64_decode(strtr($str.str_repeat('=', (4 - strlen($str) % 4)), '-_', '+/'));
}
/**
* 读取jssdk授权信息,用于和jssdk的同步登录
*
* @return array 成功返回array('access_token'=>'value', 'refresh_token'=>'value'); 失败返回false
*/
function getTokenFromJSSDK() {
$key = "weibojs_" . $this->client_id;
if ( isset($_COOKIE[$key]) && $cookie = $_COOKIE[$key] ) {
parse_str($cookie, $token);
if ( isset($token['access_token']) && isset($token['refresh_token']) ) {
$this->access_token = $token['access_token'];
$this->refresh_token = $token['refresh_token'];
return $token;
} else {
return false;
}
} else {
return false;
}
}
/**
* 从数组中读取access_token和refresh_token
* 常用于从Session或Cookie中读取token,或通过Session/Cookie中是否存有token判断登录状态。
*
* @param array $arr 存有access_token和secret_token的数组
* @return array 成功返回array('access_token'=>'value', 'refresh_token'=>'value'); 失败返回false
*/
function getTokenFromArray( $arr ) {
if (isset($arr['access_token']) && $arr['access_token']) {
$token = array();
$this->access_token = $token['access_token'] = $arr['access_token'];
if (isset($arr['refresh_token']) && $arr['refresh_token']) {
$this->refresh_token = $token['refresh_token'] = $arr['refresh_token'];
}
return $token;
} else {
return false;
}
}
/**
* GET wrappwer for oAuthRequest.
*
* @return mixed
*/
function get($url, $parameters = array()) {
$response = $this->oAuthRequest($url, 'GET', $parameters);
if ($this->format === 'json' && $this->decode_json) {
return json_decode($response, true);
}
return $response;
}
/**
* POST wreapper for oAuthRequest.
*
* @return mixed
*/
function post($url, $parameters = array(), $multi = false) {
$response = $this->oAuthRequest($url, 'POST', $parameters, $multi );
if ($this->format === 'json' && $this->decode_json) {
return json_decode($response, true);
}
return $response;
}
/**
* DELTE wrapper for oAuthReqeust.
*
* @return mixed
*/
function delete($url, $parameters = array()) {
$response = $this->oAuthRequest($url, 'DELETE', $parameters);
if ($this->format === 'json' && $this->decode_json) {
return json_decode($response, true);
}
没有合适的资源?快使用搜索试试~ 我知道了~
非常时尚大气的淘宝客源码网站-时尚php源码网站
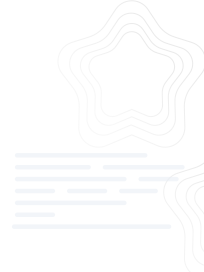
共2000个文件
jpg:1050个
php:442个
html:210个

0 下载量 76 浏览量
2023-08-15
21:32:12
上传
评论 1
收藏 25.3MB ZIP 举报
温馨提示
php源码 非常时尚大气的淘宝客源码网站_时尚php源码网站 源码类别: 淘宝客源码 运行环境: PHP+MySQL 语言编码: utf-8版 源码大小: 24 M(压缩包) 使用范围: 淘宝客网站,购物导航网站 安装使用: 安装的方法也很简单,直接运行 http://你的域名/install.php就可以正常安装。如果安装的时候出现空白,请删除data下面的install.lock。然后在输http://你的域名/install.php 其他说明: 最近淘宝客搞的比较好的,个人觉得就是什么值得买这个网站,这个网站首先是页面设计的很好,感觉不像在浏览一个购物网站,而是一个收藏好东西的网站。用户体验做的非常不错,介绍什么的写的比较好。我想这个会成为以后做淘宝客网站的一个主流趋势,以前的那种API调用模式已经被做死掉了。
资源推荐
资源详情
资源评论
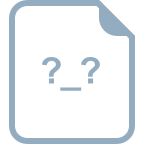
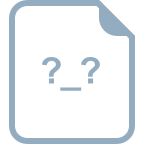
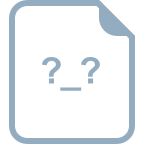
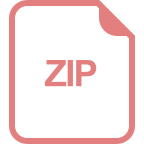
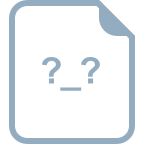
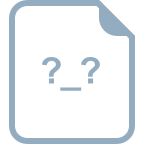
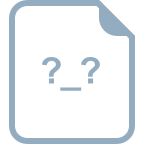
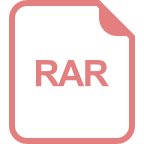
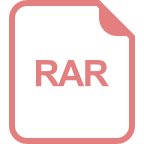
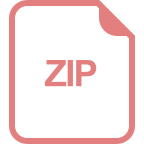
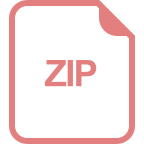
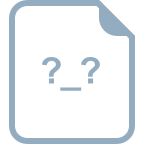
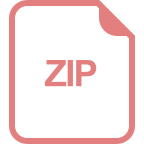
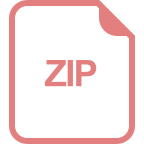
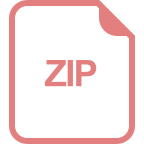
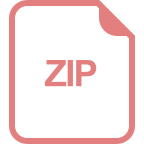
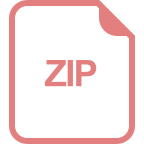
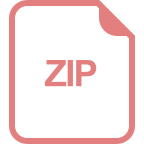
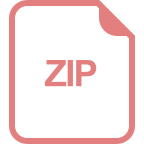
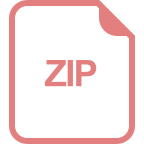
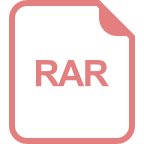
收起资源包目录

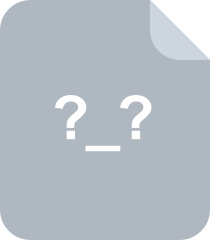
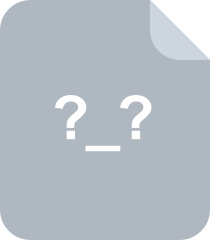
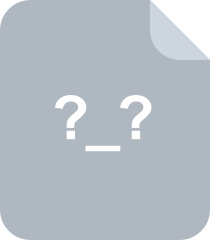
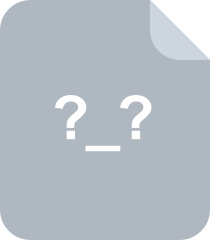
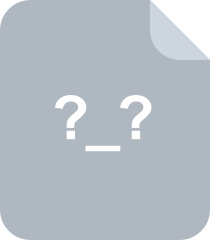
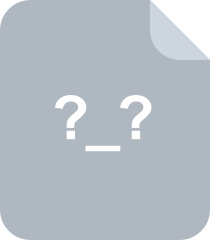
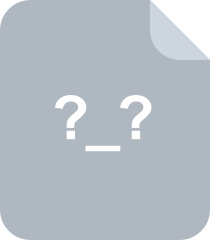
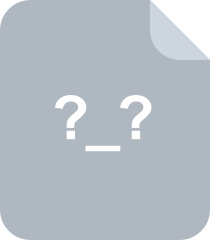
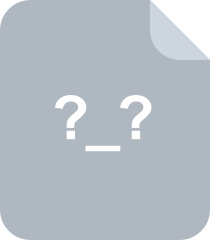
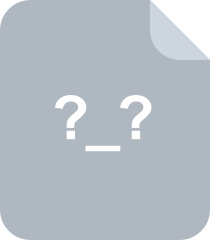
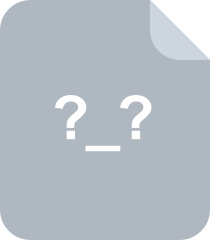
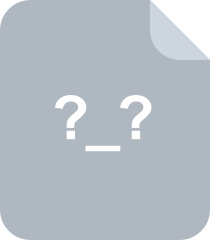
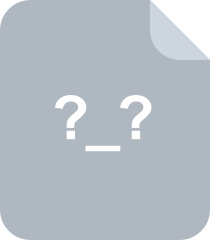
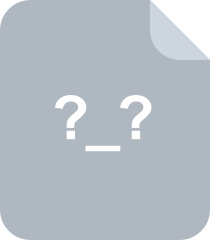
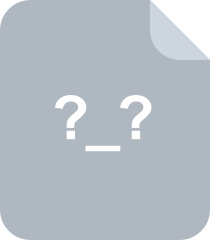
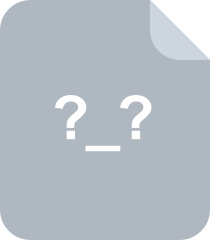
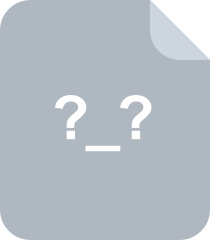
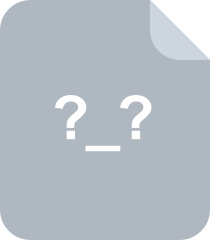
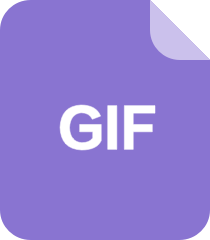
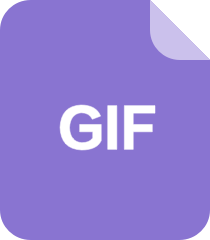
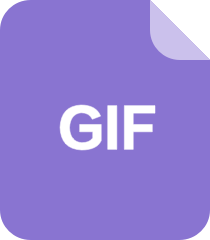
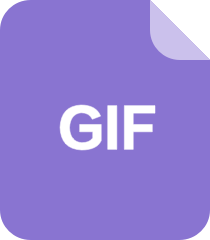
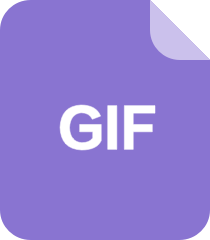
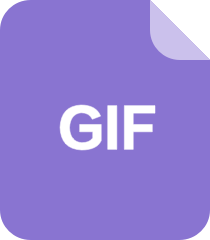
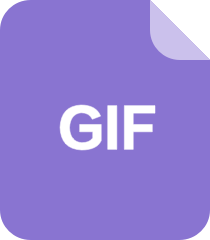
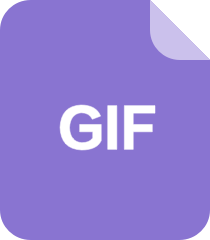
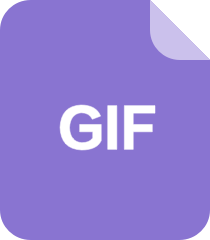
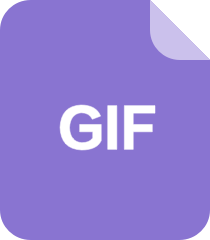
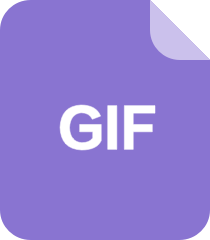
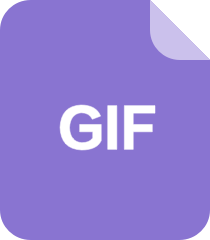
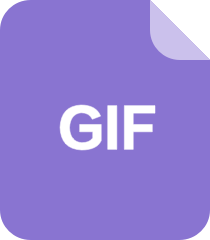
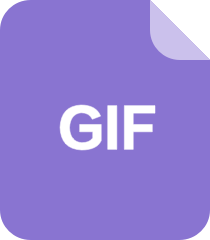
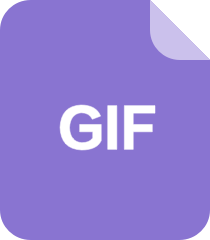
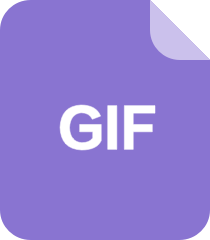
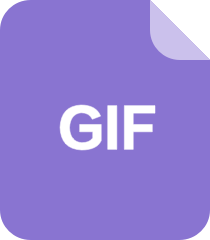
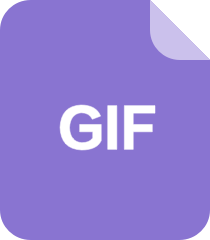
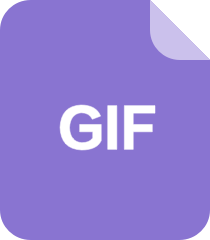
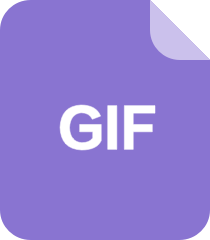
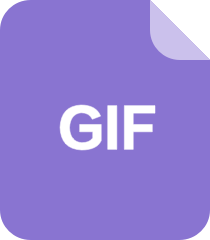
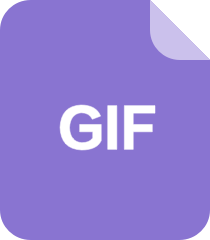
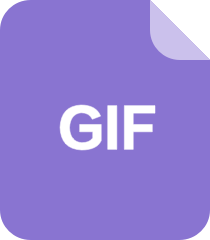
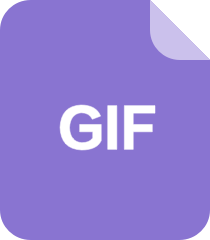
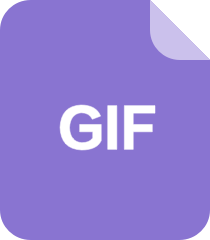
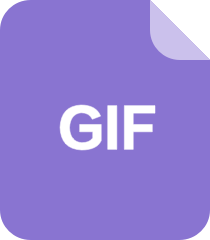
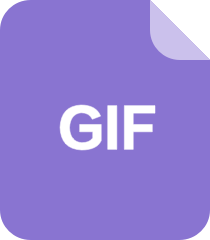
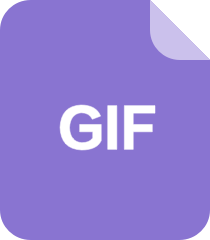
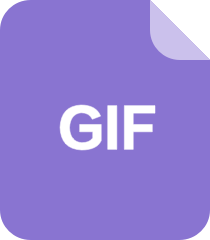
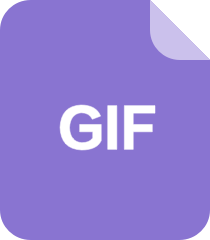
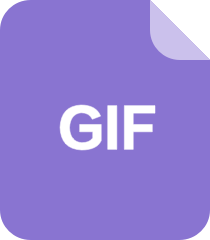
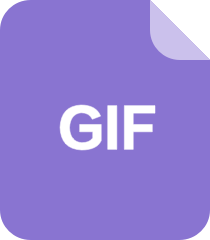
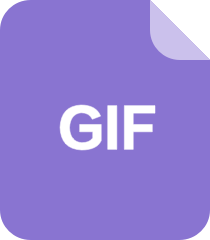
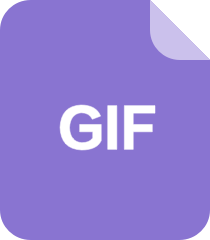
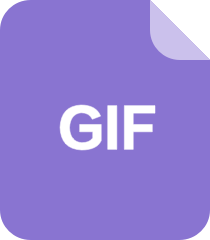
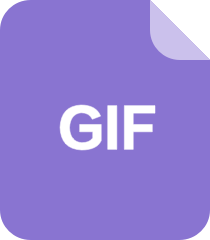
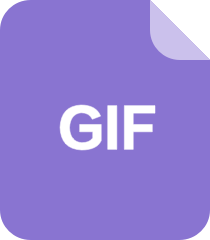
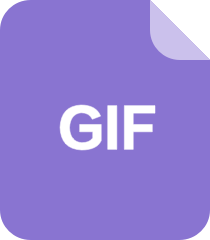
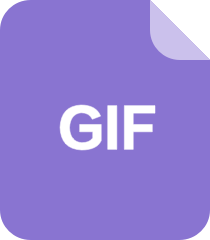
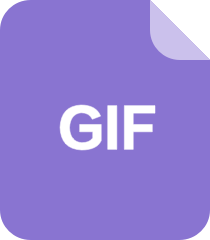
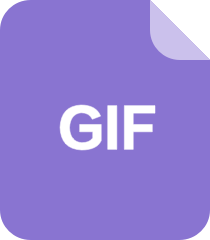
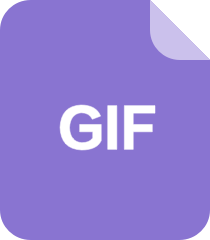
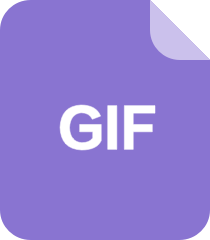
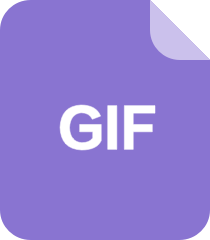
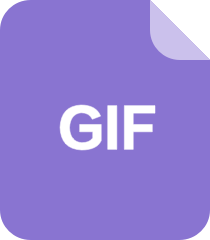
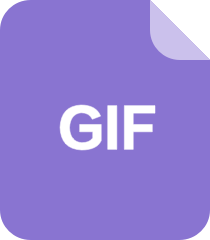
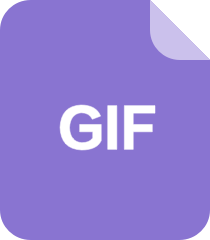
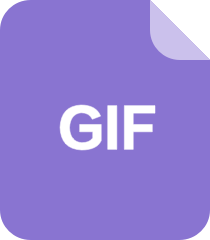
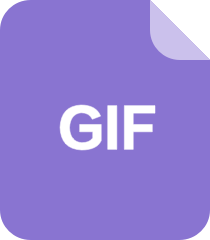
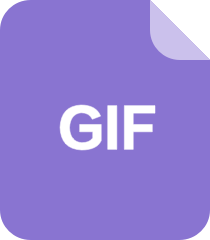
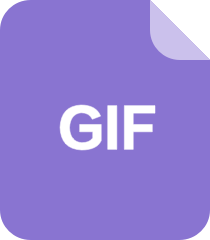
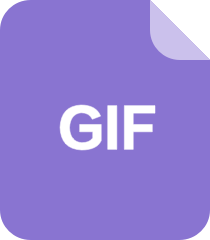
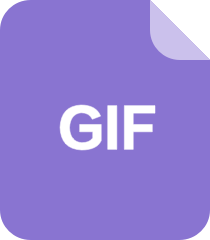
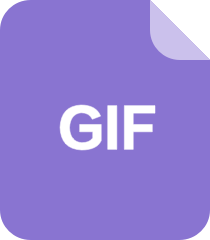
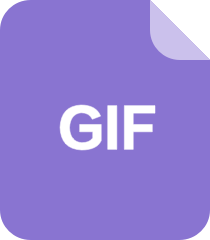
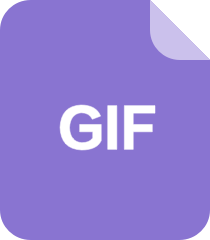
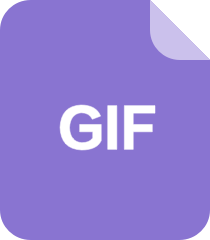
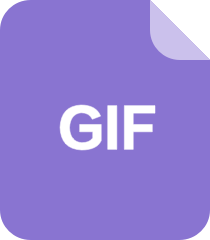
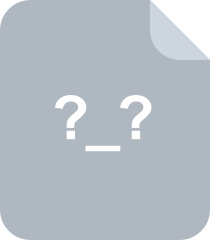
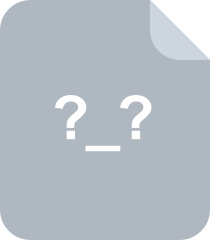
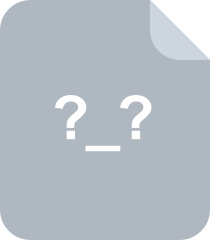
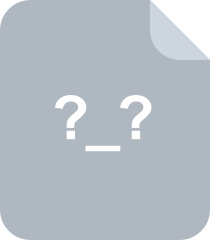
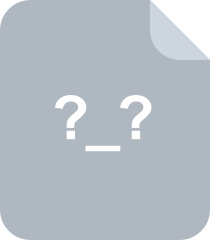
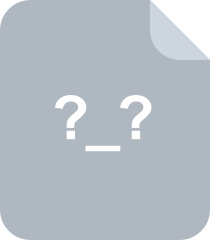
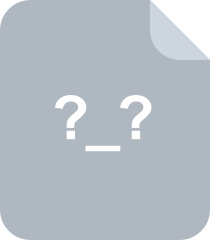
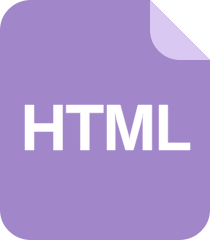
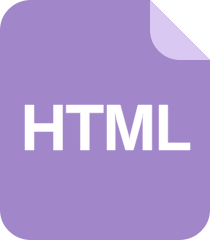
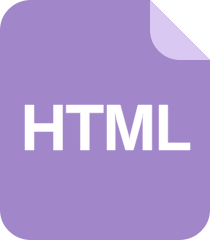
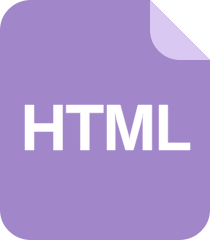
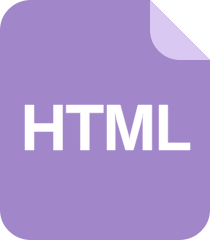
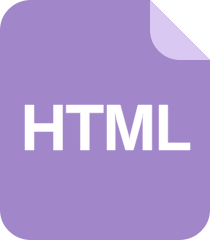
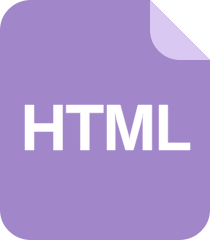
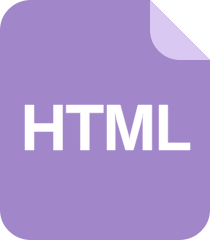
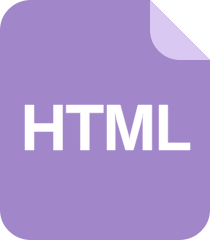
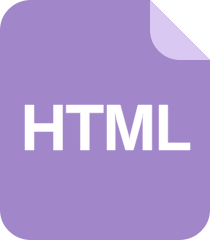
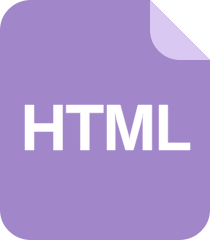
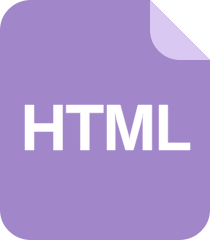
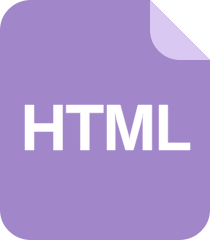
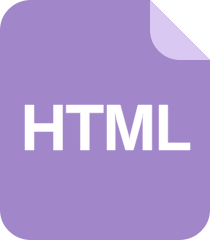
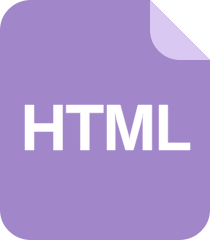
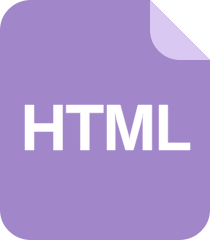
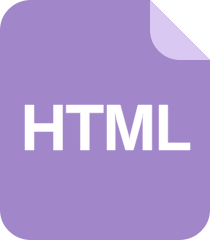
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
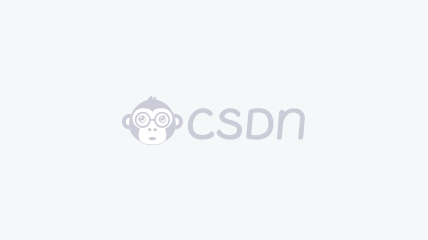


行动之上
- 粉丝: 2268
- 资源: 927
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

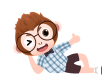
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


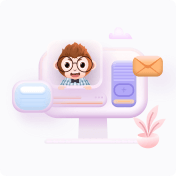
安全验证
文档复制为VIP权益,开通VIP直接复制
