没有合适的资源?快使用搜索试试~ 我知道了~
OMPL (Open Motion Planning Library) 是一个开源的运动规划库,专为解决机器人和其他系统在复杂环境中的路径规划问题而设计。这篇文档是OMPL的入门教程,旨在帮助用户了解如何使用这个强大的工具。 要使用OMPL,你需要具备一些基础知识,包括对机器人学、图论和概率理论的基本理解,因为这些是运动规划的基础。此外,熟悉C++编程语言也是必要的,因为OMPL主要用C++编写,并且许多示例代码和接口都是基于C++的。 运动规划的历史和采样基础是理解OMPL的关键。早期的运动规划方法如A*算法效率有限,当面临高维或连续空间时尤为困难。采样基
资源详情
资源评论
资源推荐
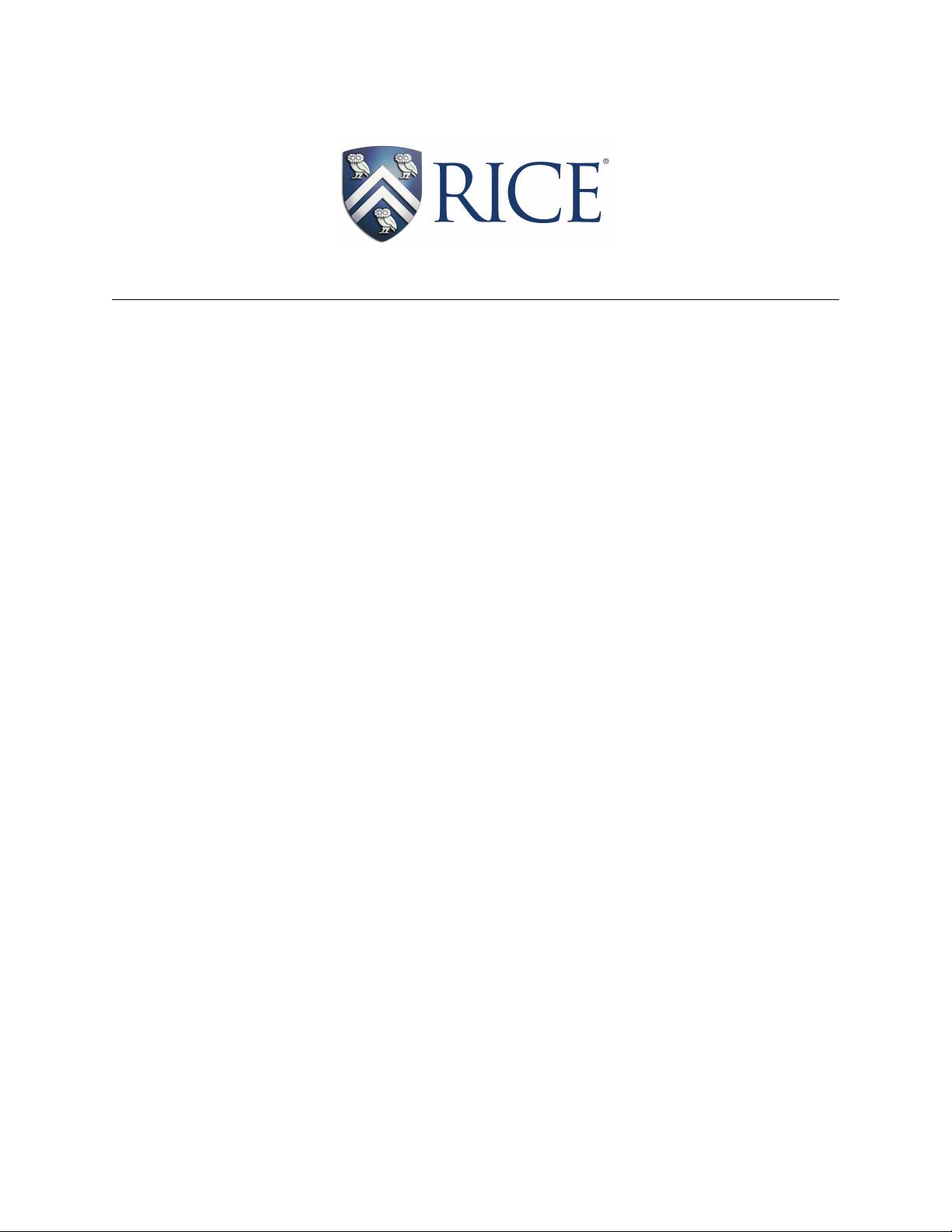
Open Motion Planning Library:
A Primer
Kavraki Lab
Rice University
ompl.kavrakilab.org
March 1, 2017
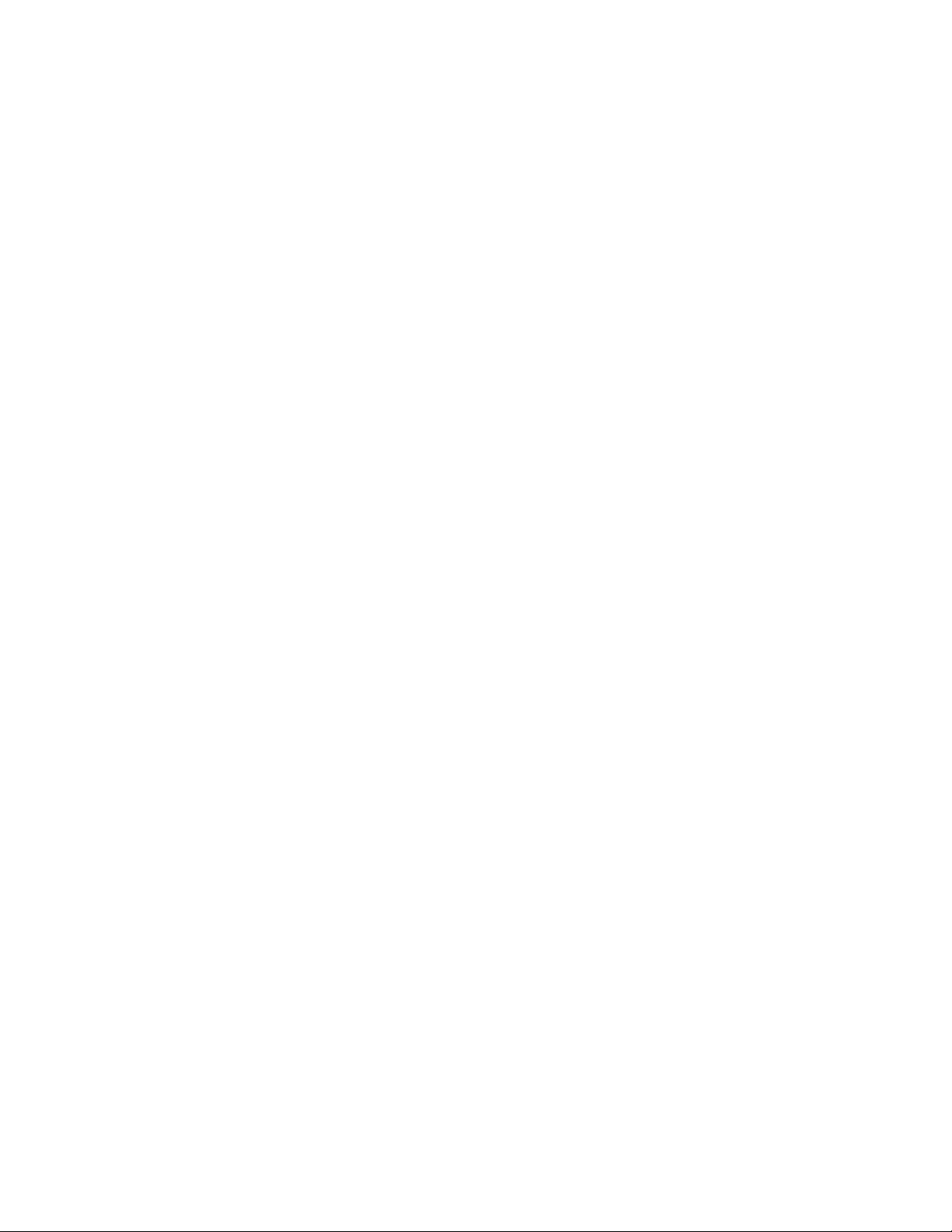
Contents
1 Introduction 1
1.1 Prerequisites for Using OMPL . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1
2 Introduction to Sampling-based Motion Planning 2
2.1 Historical Notes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 2
2.2 Sampling-based Motion Planning . . . . . . . . . . . . . . . . . . . . . . . . . . . 3
2.2.1 Problem Statement and Definitions . . . . . . . . . . . . . . . . . . . . . 4
2.2.2 Probabilistic Roadmap . . . . . . . . . . . . . . . . . . . . . . . . . . . . 4
2.2.3 Tree-based Planners . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
2.2.4 Primitives of Sampling-based Planning . . . . . . . . . . . . . . . . . . . 8
3 Getting Started with OMPL.app 9
3.1 Using OMPL.app . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 9
3.1.1 Selecting a Robot and an Environment . . . . . . . . . . . . . . . . . . . . 10
3.1.2 Choosing a Planner . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11
3.1.3 Bounding the Environment . . . . . . . . . . . . . . . . . . . . . . . . . . 12
3.1.4 Saving and Replaying Solution Paths . . . . . . . . . . . . . . . . . . . . 12
3.2 OMPL.app vs. OMPL . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 12
4 Planning with OMPL 13
4.1 Design Considerations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13
4.2 OMPL Foundations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 14
4.3 Solving a Query . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 15
4.4 Compiling Code with OMPL . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 16
4.5 Benchmarking . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 17
5 Advanced Topics in OMPL 19
5.1 State Space Construction . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 19
5.2 Planner Customization . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 19
5.3 Python Bindings . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 20
i
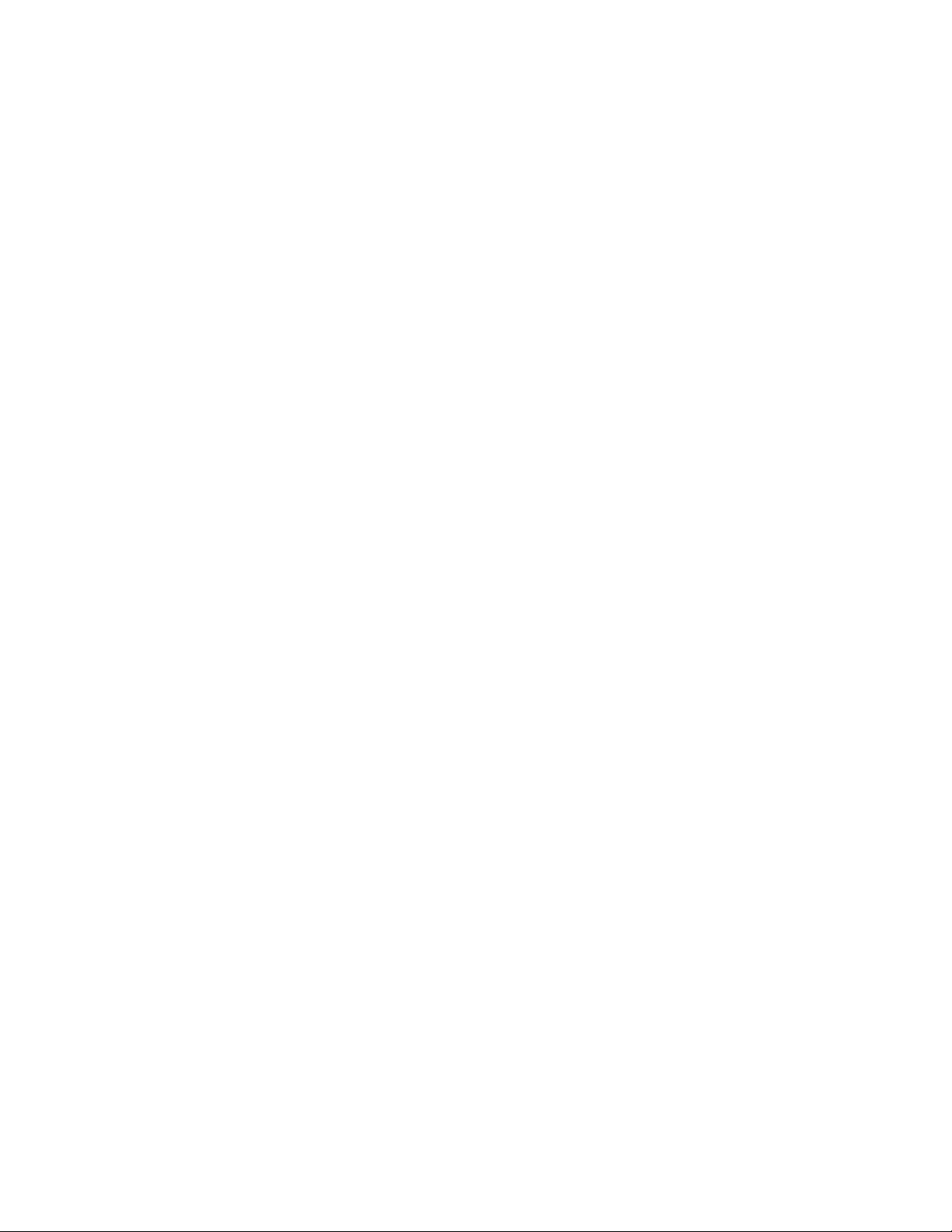
Chapter 1
Introduction
This document explains how the Open Motion Planning Library (OMPL) implements the basic
primitives of sampling-based motion planning, what planners are already available in OMPL, and
how to use the library to build new planners. This primer is segmented into the following sections:
An introduction to sampling-based motion planning, a guide to setup OMPL for solving motion
planning queries using OMPL.app, a description of the motion planning primitives in OMPL to
develop your own planner, and an explanation of some advanced OMPL topics.
At the end of this document, users should be able to use OMPL.app to solve motion planning
queries in 2D and 3D workspaces, and utilize the OMPL framework to develop their own algo-
rithms for state sampling, collision checking, nearest neighbor searching, and other components of
sampling-based methods to build a new planner.
1.1 Prerequisites for Using OMPL
This primer assumes that users are familiar with C++ programming and compiling code in a Unix
environment. Additionally, users should have basic knowledge of sampling-based motion planning.
OMPL and OMPL.app should also be installed. For information regarding the installation process,
please see ompl.kavrakilab.org.
1
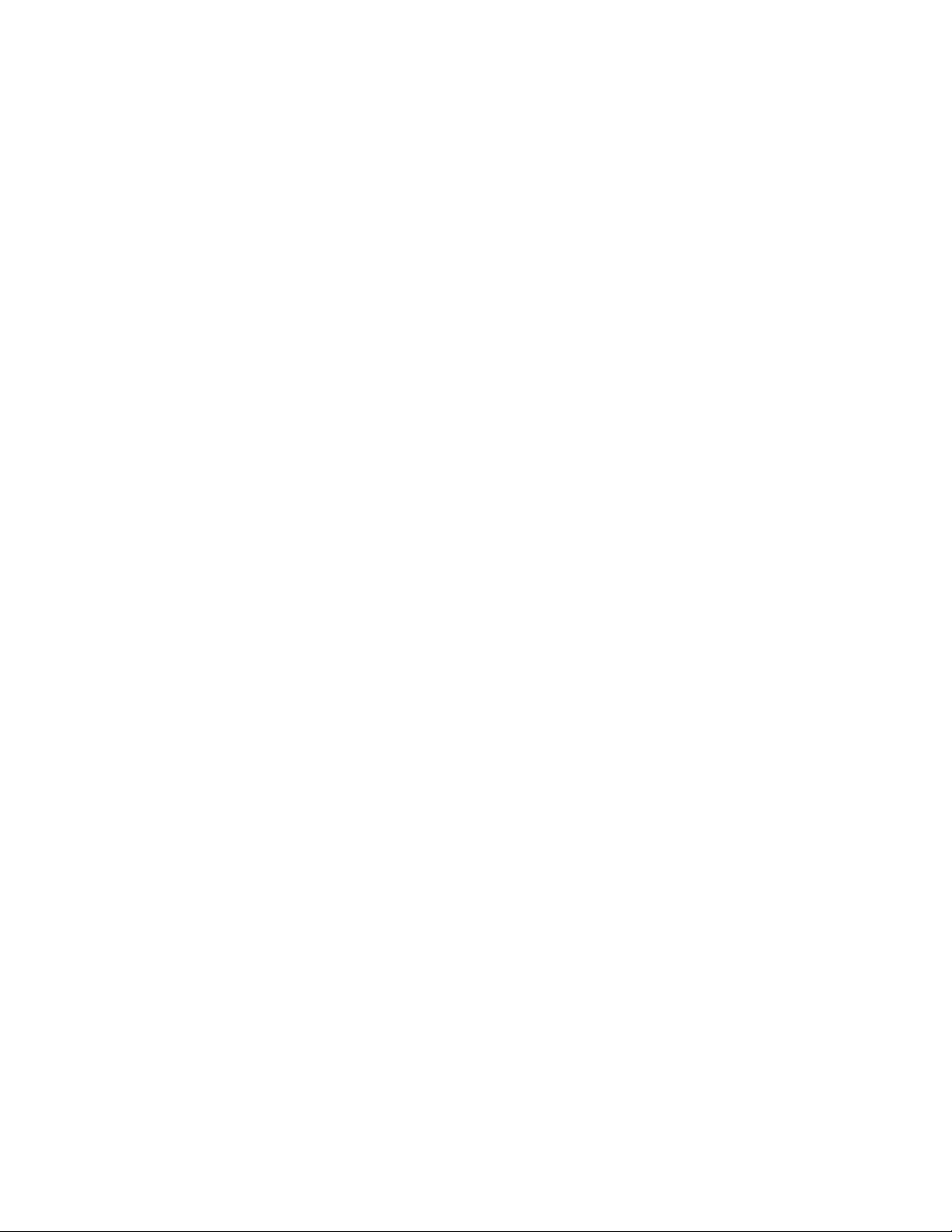
Chapter 2
Introduction to Sampling-based Motion
Planning
To put OMPL in context, a short introduction to the principles of sampling-based motion planning
is first given. Robotic motion planning seeks to find a solution to the problem of “Go from the
start to the goal while respecting all of the robot’s constraints." From a computational point of
view, however, such an inquiry can be very difficult when the robot has a large number of degrees
of freedom. For simplicity, consider the classical motion planning problem known as the piano
mover’s problem. In this formulation, there exists a rigid object in 3D (the piano), as well as a set
of known obstacles. The goal of the piano mover’s problem is to find a collision-free path for the
piano that begins at its starting position and ends at a prescribed goal configuration. Computing the
exact solution to this problem is very difficult. In this setup, the piano has six degrees of freedom:
three for movement in the coordinate planes (
x
,
y
,
z
), and three more to represent rotation along the
axes of these coordinate planes (roll, pitch, yaw). To solve the piano mover’s problem we must
compute a set of continuous changes in all six of these values in order to navigate the piano from
its starting configuration to the goal configuration while avoiding obstacles in the environment. It
has been shown that finding a solution for the piano mover’s problem is
PSPACE
-hard, indicating
computational intractability in the degrees of freedom of the robot [1, 2, 3].
2.1 Historical Notes
OMPL specializes in sampling-based motion planning, which is presented in Section 2.2. To
put sampling-based methods in context, a very brief historical overview to the methods that have
been proposed for motion planning is presented. Most of these methods were developed before
sampling-based planning, but are still applicable in many scenarios.
Exact and Approximate Cell Decomposition
In some instances, it is possible to partition the
workspace into discrete cells corresponding to the obstacle free portion of the environment. This
decomposition can then be modeled as a graph (roadmap), where the vertices represent the individual
2
剩余24页未读,继续阅读
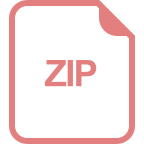
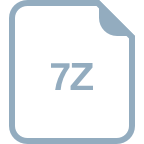
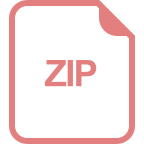
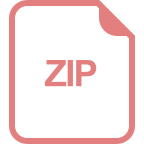
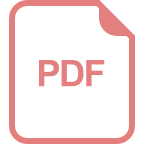
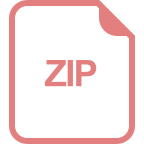
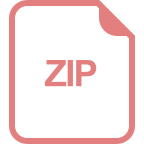
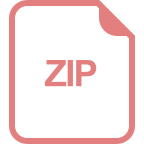
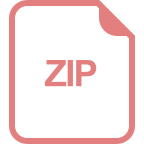
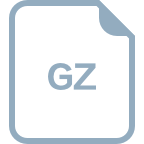
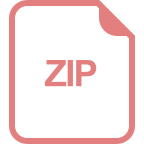
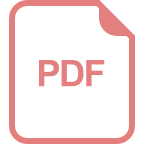
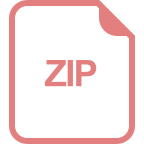
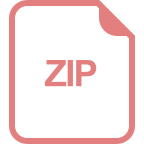
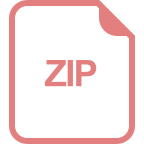
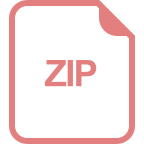
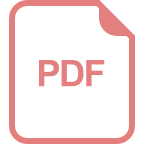
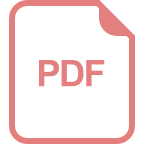

glowlaw
- 粉丝: 27
- 资源: 274
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

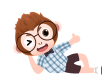
最新资源
- 基于Springboot的小区物业管理系统设计新版源码+数据库+说明
- 【Python】基于话题相似度的夸夸机器人_pgj.zip
- 【机器人】将ChatGPT飞书机器人钉钉机器人企业微信机器人公众号部署到vercel及docker_pgj.zip
- 基于STM32的电阻炉炉温控制系统设计20241229
- 【爬虫】vue2聊天室,图灵机器人,node爬虫_pgj.zip
- 【Python】基于Python爬虫爬取牛津三千词并导入到Anki方便背诵_pgj.zip
- 【Python】基于Python的美篇高清图片爬虫_pgj.zip
- 【机器人】基于code hijack和code injection极简微信机器人_pgj.zip
- 【机器人】语义地图构建、定位导航、三维重构、重定位、动态物体识别、移动避障、手势识别、人脸识别、语音合成与识别等功能_pgj.zip
- 【Python爬虫】基于Python实现基本的网页爬虫_pgj.zip
- 【Python】简明饭否机器人教程(使用Python)_pgj.zip
- 【java】用mirai机器人搜索音乐并以卡片的形式分享_pgj.zip
- MATLAB仿真 基于toa foa的无源定位方法,二次等式约束求解 有 参考文档 无源定位技术:二次等式约束最小二乘估计理论与方法 第八章
- 无人机仿真无人机四旋翼uav轨迹跟踪PID控制matlab,simulink仿真,包括位置三维图像,三个姿态角度图像,位置图像,以及参考位置实际位置对比图像 四旋翼无人机轨迹跟踪自适应滑模控制,ma
- 一款可以实现串口与mqtt客户端之间数据互相转发的工具
- Ego-planner/src
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


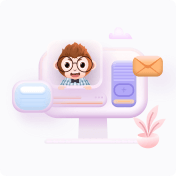
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0