/****************************************************************************
**
** Copyright (C) 2016 The Qt Company Ltd.
** Contact: https://www.qt.io/licensing/
**
** This file is part of the QtWebEngine module of the Qt Toolkit.
**
** $QT_BEGIN_LICENSE:LGPL$
** Commercial License Usage
** Licensees holding valid commercial Qt licenses may use this file in
** accordance with the commercial license agreement provided with the
** Software or, alternatively, in accordance with the terms contained in
** a written agreement between you and The Qt Company. For licensing terms
** and conditions see https://www.qt.io/terms-conditions. For further
** information use the contact form at https://www.qt.io/contact-us.
**
** GNU Lesser General Public License Usage
** Alternatively, this file may be used under the terms of the GNU Lesser
** General Public License version 3 as published by the Free Software
** Foundation and appearing in the file LICENSE.LGPL3 included in the
** packaging of this file. Please review the following information to
** ensure the GNU Lesser General Public License version 3 requirements
** will be met: https://www.gnu.org/licenses/lgpl-3.0.html.
**
** GNU General Public License Usage
** Alternatively, this file may be used under the terms of the GNU
** General Public License version 2.0 or (at your option) the GNU General
** Public license version 3 or any later version approved by the KDE Free
** Qt Foundation. The licenses are as published by the Free Software
** Foundation and appearing in the file LICENSE.GPL2 and LICENSE.GPL3
** included in the packaging of this file. Please review the following
** information to ensure the GNU General Public License requirements will
** be met: https://www.gnu.org/licenses/gpl-2.0.html and
** https://www.gnu.org/licenses/gpl-3.0.html.
**
** $QT_END_LICENSE$
**
****************************************************************************/
#ifndef QQUICKWEBENGINEVIEW_P_H
#define QQUICKWEBENGINEVIEW_P_H
//
// W A R N I N G
// -------------
//
// This file is not part of the Qt API. It exists purely as an
// implementation detail. This header file may change from version to
// version without notice, or even be removed.
//
// We mean it.
//
#include <private/qtwebengineglobal_p.h>
#include "qquickwebenginescript.h"
#include <QQuickItem>
#include <QtGui/qcolor.h>
QT_BEGIN_NAMESPACE
class QQmlWebChannel;
class QQuickWebEngineAuthenticationDialogRequest;
class QQuickWebEngineCertificateError;
class QQuickWebEngineColorDialogRequest;
class QQuickWebEngineContextMenuRequest;
class QQuickWebEngineFaviconProvider;
class QQuickWebEngineFileDialogRequest;
class QQuickWebEngineHistory;
class QQuickWebEngineJavaScriptDialogRequest;
class QQuickWebEngineLoadRequest;
class QQuickWebEngineNavigationRequest;
class QQuickWebEngineNewViewRequest;
class QQuickWebEngineProfile;
class QQuickWebEngineSettings;
class QQuickWebEngineFormValidationMessageRequest;
class QQuickWebEngineViewPrivate;
#ifdef ENABLE_QML_TESTSUPPORT_API
class QQuickWebEngineTestSupport;
#endif
class Q_WEBENGINE_PRIVATE_EXPORT QQuickWebEngineFullScreenRequest {
Q_GADGET
Q_PROPERTY(QUrl origin READ origin CONSTANT FINAL)
Q_PROPERTY(bool toggleOn READ toggleOn CONSTANT FINAL)
public:
QQuickWebEngineFullScreenRequest();
QQuickWebEngineFullScreenRequest(QQuickWebEngineViewPrivate *viewPrivate, const QUrl &origin, bool toggleOn);
Q_INVOKABLE void accept();
Q_INVOKABLE void reject();
QUrl origin() const { return m_origin; }
bool toggleOn() const { return m_toggleOn; }
private:
QQuickWebEngineViewPrivate *m_viewPrivate;
const QUrl m_origin;
const bool m_toggleOn;
};
class Q_WEBENGINE_PRIVATE_EXPORT QQuickWebEngineView : public QQuickItem {
Q_OBJECT
Q_PROPERTY(QUrl url READ url WRITE setUrl NOTIFY urlChanged FINAL)
Q_PROPERTY(QUrl icon READ icon NOTIFY iconChanged FINAL)
Q_PROPERTY(bool loading READ isLoading NOTIFY loadingChanged FINAL)
Q_PROPERTY(int loadProgress READ loadProgress NOTIFY loadProgressChanged FINAL)
Q_PROPERTY(QString title READ title NOTIFY titleChanged FINAL)
Q_PROPERTY(bool canGoBack READ canGoBack NOTIFY urlChanged FINAL)
Q_PROPERTY(bool canGoForward READ canGoForward NOTIFY urlChanged FINAL)
Q_PROPERTY(bool isFullScreen READ isFullScreen NOTIFY isFullScreenChanged REVISION 1 FINAL)
Q_PROPERTY(qreal zoomFactor READ zoomFactor WRITE setZoomFactor NOTIFY zoomFactorChanged REVISION 1 FINAL)
Q_PROPERTY(QQuickWebEngineProfile *profile READ profile WRITE setProfile NOTIFY profileChanged FINAL REVISION 1)
Q_PROPERTY(QQuickWebEngineSettings *settings READ settings REVISION 1 CONSTANT FINAL)
Q_PROPERTY(QQuickWebEngineHistory *navigationHistory READ navigationHistory CONSTANT FINAL REVISION 1)
Q_PROPERTY(QQmlWebChannel *webChannel READ webChannel WRITE setWebChannel NOTIFY webChannelChanged REVISION 1 FINAL)
Q_PROPERTY(QQmlListProperty<QQuickWebEngineScript> userScripts READ userScripts FINAL REVISION 1)
Q_PROPERTY(bool activeFocusOnPress READ activeFocusOnPress WRITE setActiveFocusOnPress NOTIFY activeFocusOnPressChanged REVISION 2 FINAL)
Q_PROPERTY(QColor backgroundColor READ backgroundColor WRITE setBackgroundColor NOTIFY backgroundColorChanged REVISION 2 FINAL)
Q_PROPERTY(QSizeF contentsSize READ contentsSize NOTIFY contentsSizeChanged FINAL REVISION 3)
Q_PROPERTY(QPointF scrollPosition READ scrollPosition NOTIFY scrollPositionChanged FINAL REVISION 3)
Q_PROPERTY(bool audioMuted READ isAudioMuted WRITE setAudioMuted NOTIFY audioMutedChanged FINAL REVISION 3)
Q_PROPERTY(bool recentlyAudible READ recentlyAudible NOTIFY recentlyAudibleChanged FINAL REVISION 3)
Q_PROPERTY(uint webChannelWorld READ webChannelWorld WRITE setWebChannelWorld NOTIFY webChannelWorldChanged REVISION 3 FINAL)
#ifdef ENABLE_QML_TESTSUPPORT_API
Q_PROPERTY(QQuickWebEngineTestSupport *testSupport READ testSupport WRITE setTestSupport NOTIFY testSupportChanged FINAL)
#endif
Q_FLAGS(FindFlags);
public:
QQuickWebEngineView(QQuickItem *parent = 0);
~QQuickWebEngineView();
QUrl url() const;
void setUrl(const QUrl&);
QUrl icon() const;
bool isLoading() const;
int loadProgress() const;
QString title() const;
bool canGoBack() const;
bool canGoForward() const;
bool isFullScreen() const;
qreal zoomFactor() const;
void setZoomFactor(qreal arg);
QColor backgroundColor() const;
void setBackgroundColor(const QColor &color);
QSizeF contentsSize() const;
QPointF scrollPosition() const;
// must match WebContentsAdapterClient::NavigationRequestAction
enum NavigationRequestAction {
AcceptRequest,
// Make room in the valid range of the enum so
// we can expose extra actions.
IgnoreRequest = 0xFF
};
Q_ENUM(NavigationRequestAction)
// must match WebContentsAdapterClient::NavigationType
enum NavigationType {
LinkClickedNavigation,
TypedNavigation,
FormSubmittedNavigation,
BackForwardNavigation,
ReloadNavigation,
OtherNavigation
};
Q_ENUM(NavigationType)
enum LoadStatus {
LoadStartedStatus,
LoadStoppedStatus,
LoadSucceededStatus,
LoadFailedStatus
};
Q_ENUM(LoadStatus)
enum ErrorDomain {
NoErrorDomain,
InternalErrorDomain,
ConnectionErrorDomain,
CertificateErrorDomain,
HttpErrorDomain,
FtpErrorDomain,
DnsErrorDomain
};
Q_ENUM(ErrorDomain)
enum NewViewDestination {
NewViewInWindow,
NewViewInTab,
NewViewInDialog,
NewViewInBackgroundTab
};
Q_ENUM(NewViewDestination)
enum Feature {
MediaAudioCapture,
MediaVideoCapture,
MediaAudioVideoCapture,
Geolocation
};
Q_ENUM(Feature)
enum WebAction {
NoWebAction = - 1,
Back,
Forward,
没有合适的资源?快使用搜索试试~ 我知道了~
Mac Qt5.9.8编译QWebEngine支持MP4的web相关库
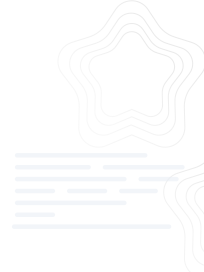
共269个文件
h:99个
pak:57个
prl:12个

需积分: 44 12 下载量 144 浏览量
2019-10-23
22:55:14
上传
评论
收藏 138.35MB ZIP 举报
温馨提示
Qt的QWebEngine默认是不支持MP4播放的,需要手动编译,比较耗时(在配置没有问题的情况下我的i7 6核 16G内存大概用了6个小时),为了方便有同样问题的小伙伴,所以上传了已经编译好的资源,需要下载后替换clang_64/lib下的相关文件
资源推荐
资源详情
资源评论
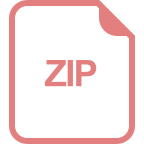
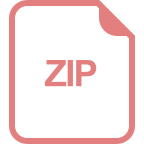
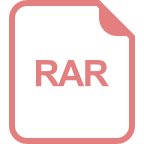
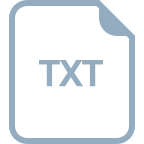
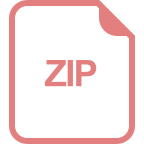
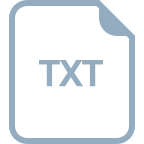
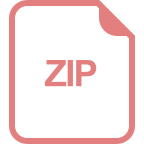
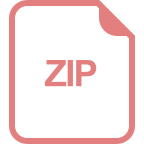
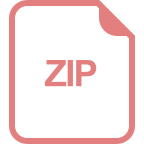
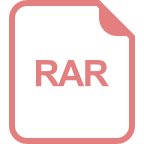
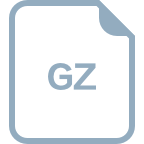
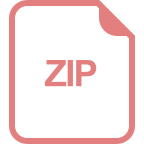
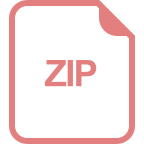
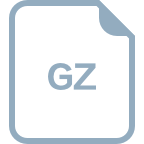
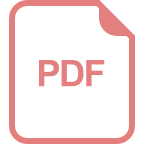
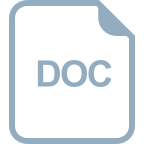
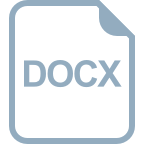
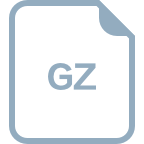
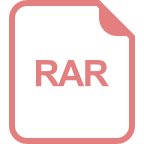
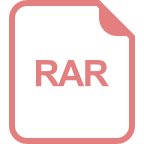
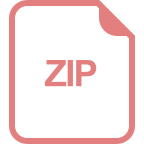
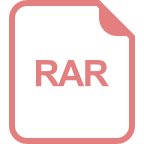
收起资源包目录

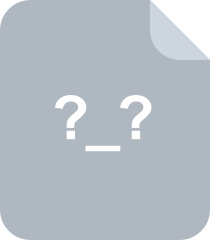
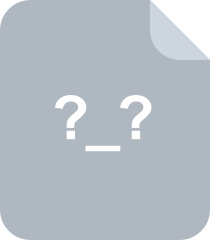
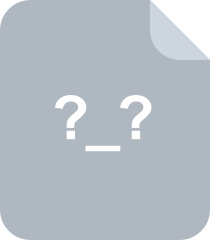
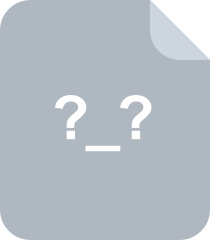
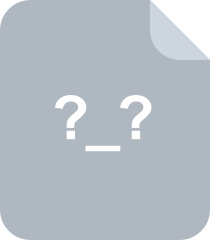
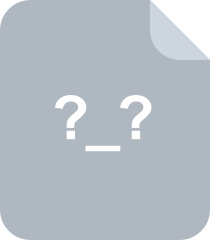
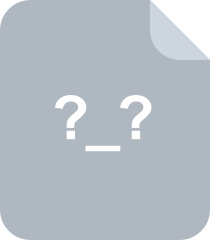
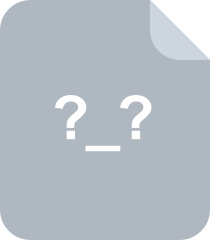
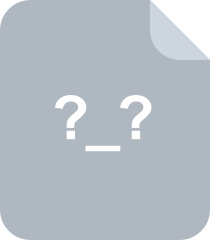
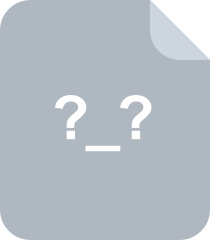
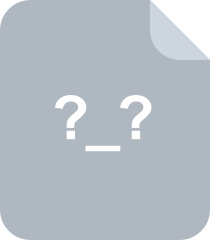
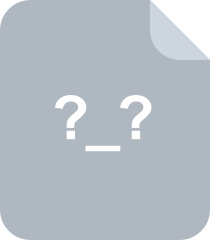
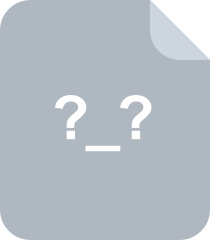
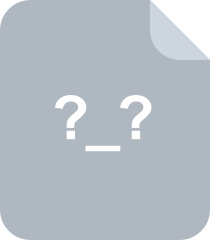
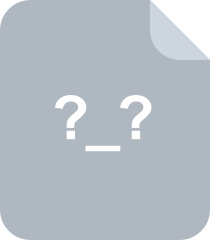
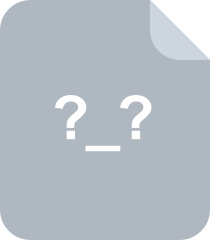
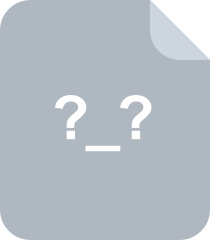
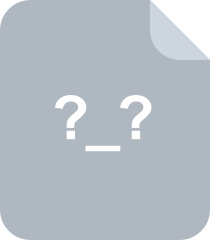
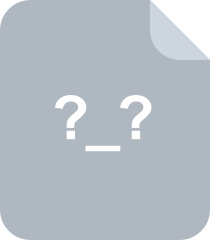
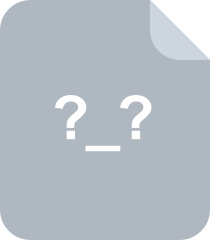
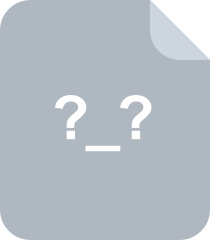
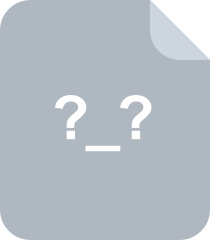
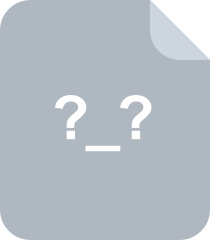
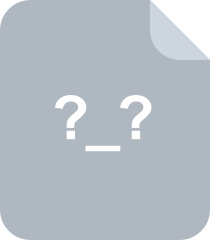
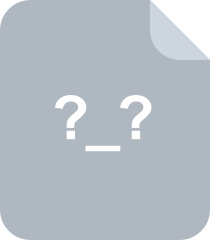
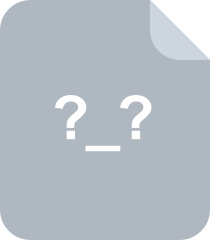
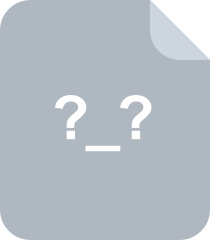
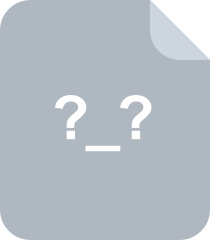
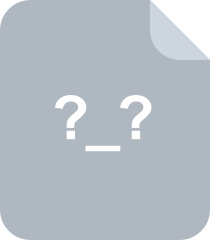
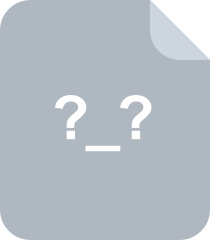
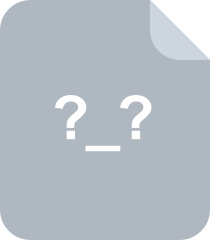
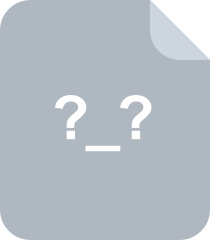
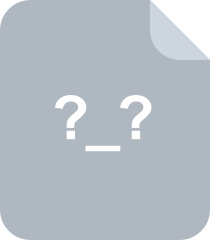
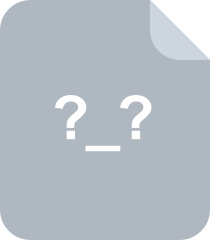
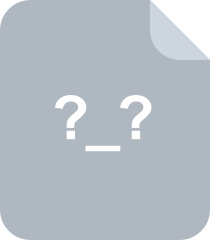
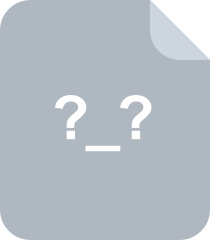
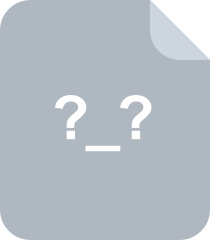
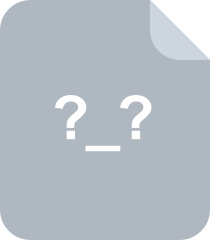
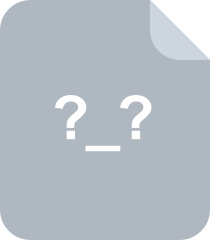
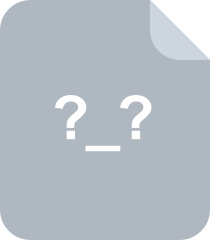
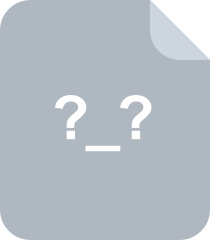
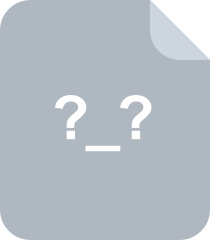
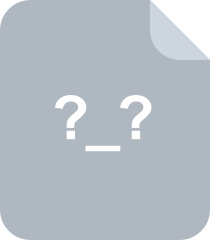
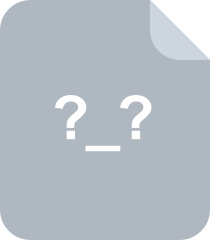
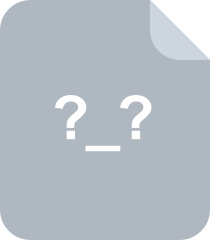
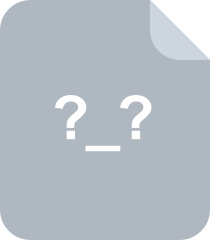
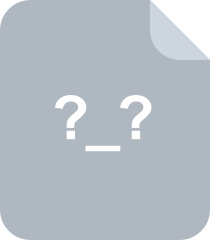
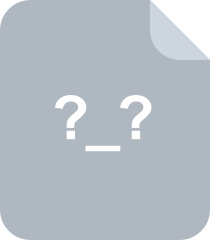
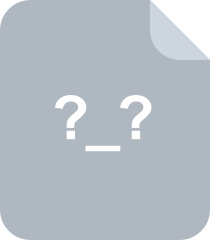
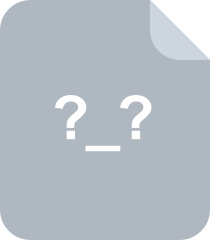
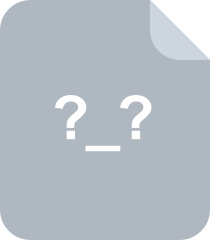
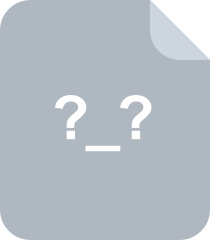
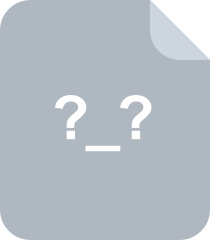
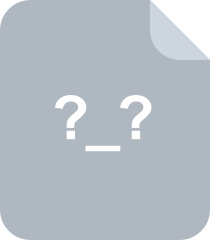
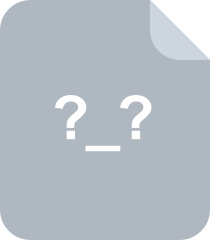
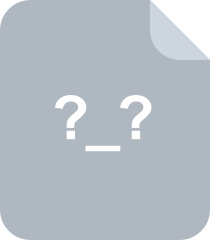
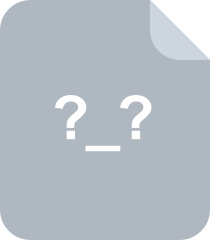
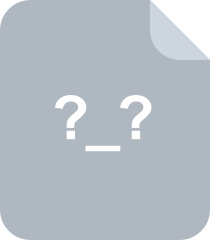
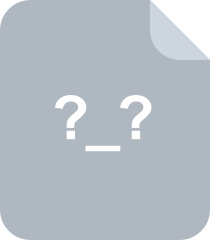
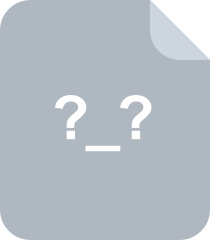
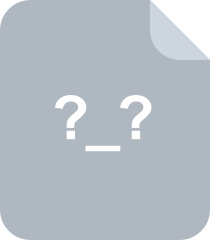
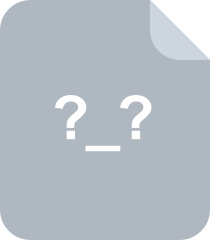
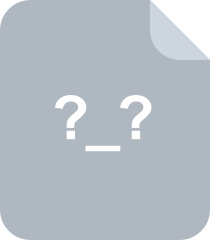
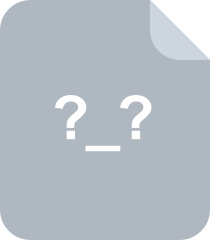
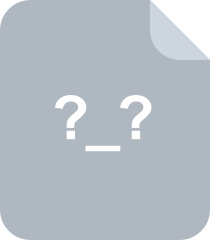
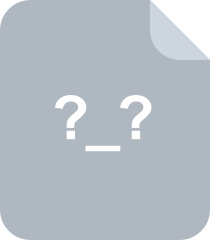
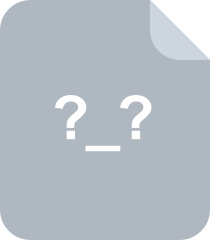
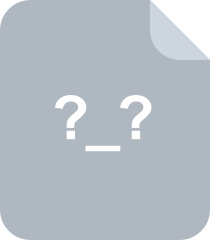
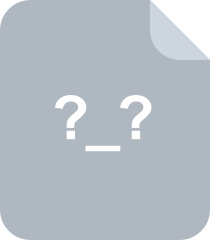
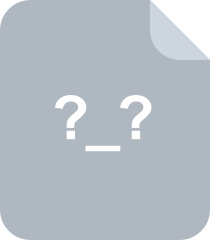
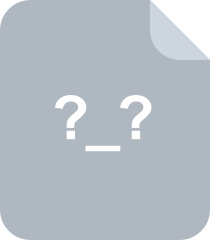
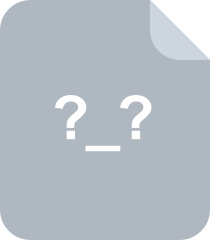
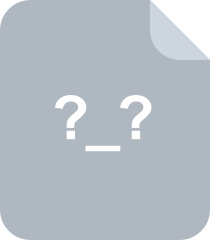
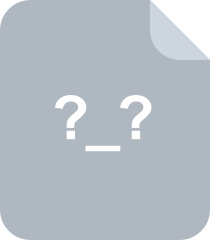
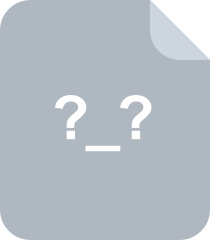
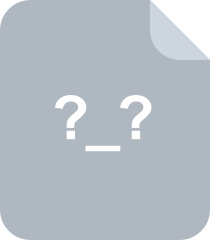
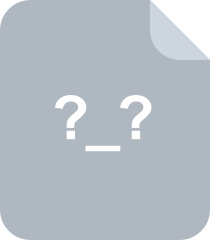
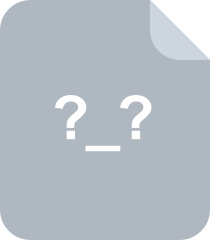
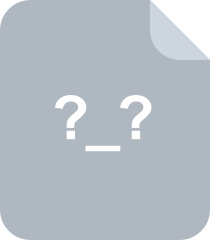
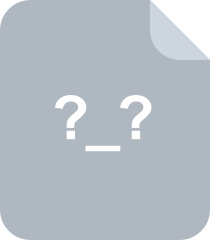
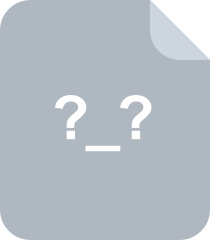
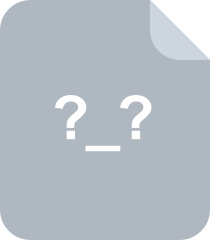
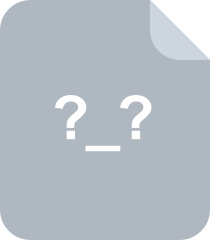
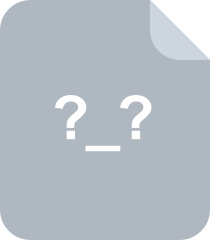
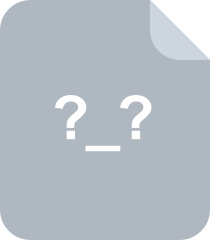
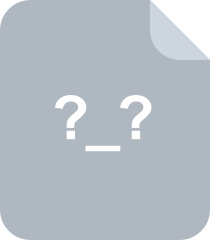
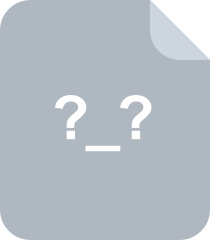
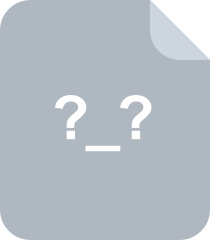
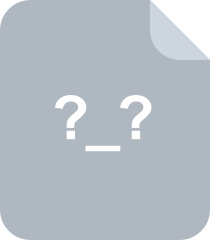
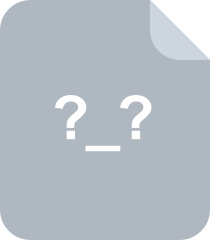
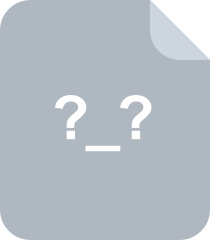
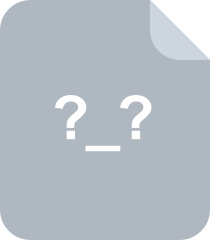
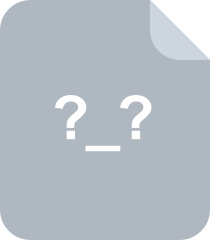
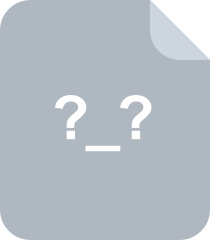
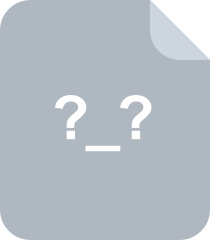
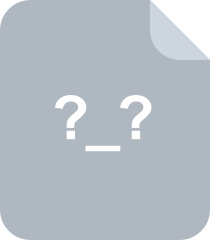
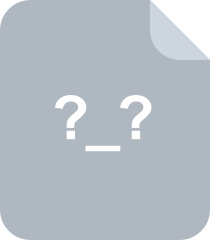
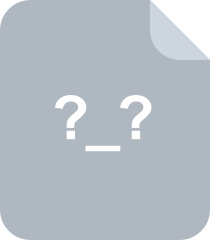
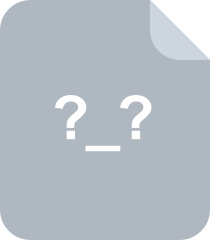
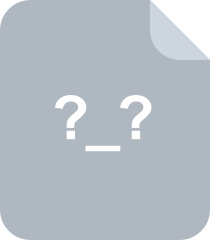
共 269 条
- 1
- 2
- 3
资源评论
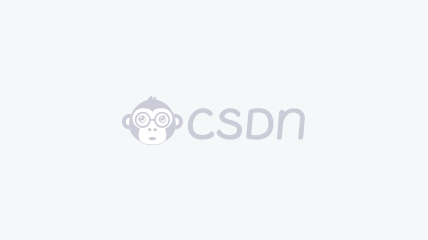

wanlong1215
- 粉丝: 10
- 资源: 4
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

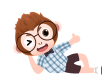
最新资源
- 深海采矿车路径规划MATLAB仿真源代码全套技术资料.zip
- Python基础学习第一天(思维导图)
- (176602634)粒子群算法(PSO)优化双向长短期记忆神经网络的数据回归预测,PSO-BiLSTM回归预测,多输入单输出模型 评价指标包括:
- 华强北商城二手手机-JAVA-基于springBoot华强北商城二手手机管理系统(毕业论文)
- 水中物体检测1-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma数据集合集.rar
- 夕阳红公寓管理-JAVA-基于springBoot夕阳红公寓管理系统的设计与实现(毕业论文)
- (178784654)java基于ssm(spring+springmvc+mybatis)框架的图书借阅管理系统
- 新冠病毒密接者跟踪-JAVA-基于springBoot新冠病毒密接者跟踪系统(毕业论文)
- (179744002)2防御性驾驶安全常识.mp4.zip
- 办公室行政事务-JAVA-基于springBoot的高校办公室行政事务管理系统设计与实现(毕业论文+开题)
- IMG_1343.PNG
- 15 unit 11.mp3
- 保险合同-JAVA-基于Spring Boot的可盈保险合同管理系统的设计与实现(毕业论文+任务书)
- 智慧图书管理-JAVA-基于springBoot智慧图书管理系统设计与实现(毕业论文)
- 水杯和笔检测16-YOLOv5数据集合集.rar
- ECharts散点图-各国人均寿命与GDP关系演变.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


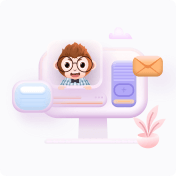
安全验证
文档复制为VIP权益,开通VIP直接复制
