/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
(function (global, factory) {
typeof exports === 'object' && typeof module !== 'undefined' ? factory(exports) :
typeof define === 'function' && define.amd ? define(['exports'], factory) :
(global = typeof globalThis !== 'undefined' ? globalThis : global || self, factory(global.echarts = {}));
}(this, (function (exports) { 'use strict';
/*! *****************************************************************************
Copyright (c) Microsoft Corporation.
Permission to use, copy, modify, and/or distribute this software for any
purpose with or without fee is hereby granted.
THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES WITH
REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY
AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY SPECIAL, DIRECT,
INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES WHATSOEVER RESULTING FROM
LOSS OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF CONTRACT, NEGLIGENCE OR
OTHER TORTIOUS ACTION, ARISING OUT OF OR IN CONNECTION WITH THE USE OR
PERFORMANCE OF THIS SOFTWARE.
***************************************************************************** */
/* global Reflect, Promise */
var extendStatics = function(d, b) {
extendStatics = Object.setPrototypeOf ||
({ __proto__: [] } instanceof Array && function (d, b) { d.__proto__ = b; }) ||
function (d, b) { for (var p in b) if (Object.prototype.hasOwnProperty.call(b, p)) d[p] = b[p]; };
return extendStatics(d, b);
};
function __extends(d, b) {
if (typeof b !== "function" && b !== null)
throw new TypeError("Class extends value " + String(b) + " is not a constructor or null");
extendStatics(d, b);
function __() { this.constructor = d; }
d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __());
}
var __assign = function() {
__assign = Object.assign || function __assign(t) {
for (var s, i = 1, n = arguments.length; i < n; i++) {
s = arguments[i];
for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p)) t[p] = s[p];
}
return t;
};
return __assign.apply(this, arguments);
};
function __spreadArray(to, from, pack) {
if (pack || arguments.length === 2) for (var i = 0, l = from.length, ar; i < l; i++) {
if (ar || !(i in from)) {
if (!ar) ar = Array.prototype.slice.call(from, 0, i);
ar[i] = from[i];
}
}
return to.concat(ar || from);
}
var Browser = (function () {
function Browser() {
this.firefox = false;
this.ie = false;
this.edge = false;
this.newEdge = false;
this.weChat = false;
}
return Browser;
}());
var Env = (function () {
function Env() {
this.browser = new Browser();
this.node = false;
this.wxa = false;
this.worker = false;
this.canvasSupported = false;
this.svgSupported = false;
this.touchEventsSupported = false;
this.pointerEventsSupported = false;
this.domSupported = false;
this.transformSupported = false;
this.transform3dSupported = false;
}
return Env;
}());
var env = new Env();
if (typeof wx === 'object' && typeof wx.getSystemInfoSync === 'function') {
env.wxa = true;
env.canvasSupported = true;
env.touchEventsSupported = true;
}
else if (typeof document === 'undefined' && typeof self !== 'undefined') {
env.worker = true;
env.canvasSupported = true;
}
else if (typeof navigator === 'undefined') {
env.node = true;
env.canvasSupported = true;
env.svgSupported = true;
}
else {
detect(navigator.userAgent, env);
}
function detect(ua, env) {
var browser = env.browser;
var firefox = ua.match(/Firefox\/([\d.]+)/);
var ie = ua.match(/MSIE\s([\d.]+)/)
|| ua.match(/Trident\/.+?rv:(([\d.]+))/);
var edge = ua.match(/Edge?\/([\d.]+)/);
var weChat = (/micromessenger/i).test(ua);
if (firefox) {
browser.firefox = true;
browser.version = firefox[1];
}
if (ie) {
browser.ie = true;
browser.version = ie[1];
}
if (edge) {
browser.edge = true;
browser.version = edge[1];
browser.newEdge = +edge[1].split('.')[0] > 18;
}
if (weChat) {
browser.weChat = true;
}
env.canvasSupported = !!document.createElement('canvas').getContext;
env.svgSupported = typeof SVGRect !== 'undefined';
env.touchEventsSupported = 'ontouchstart' in window && !browser.ie && !browser.edge;
env.pointerEventsSupported = 'onpointerdown' in window
&& (browser.edge || (browser.ie && +browser.version >= 11));
env.domSupported = typeof document !== 'undefined';
var style = document.documentElement.style;
env.transform3dSupported = ((browser.ie && 'transition' in style)
|| browser.edge
|| (('WebKitCSSMatrix' in window) && ('m11' in new WebKitCSSMatrix()))
|| 'MozPerspective' in style)
&& !('OTransition' in style);
env.transformSupported = env.transform3dSupported
|| (browser.ie && +browser.version >= 9);
}
var BUILTIN_OBJECT = {
'[object Function]': true,
'[object RegExp]': true,
'[object Date]': true,
'[object Error]': true,
'[object CanvasGradient]': true,
'[object CanvasPattern]': true,
'[object Image]': true,
'[object Canvas]': true
};
var TYPED_ARRAY = {
'[object Int8Array]': true,
'[object Uint8Array]': true,
'[object Uint8ClampedArray]': true,
'[object Int16Array]': true,
'[object Uint16Array]': true,
'[object Int32Array]': true,
'[object Uint32Array]': true,
'[object Float32Array]': true,
'[object Float64Array]': true
};
var objToString = Object.prototype.toString;
var arrayProto = Array.prototype;
var nativeForEach = arrayProto.forEach;
var nativeFilter = arrayProto.filter;
var nativeSlice = arrayProto.slice;
var nativeMap = arrayProto.map;
var ctorFunction = function () { }.constructor;
var protoFunction = ctorFunction ? ctorFunction.prototype : null;
var protoKey = '__proto__';
var methods = {};
function $override(name, fn) {
methods[name] = fn;
}
var idStart = 0x0907;
function guid() {
return idStart++;
}
function logError() {
var args = [];
for (var _i = 0; _i < arguments.length; _i++) {
args[_i] = arguments[_i];
}
if (typeof console !== 'undefined') {
console.error.apply(console, args);
}
}
function clo
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
该项目为Java语言的Age客栈项目设计源码,集成了丰富的前端资源,包含10242个文件。具体文件类型分布如下:JavaScript(3694个),TypeScript(2721个),映射文件(2063个),MJS(1256个),SCSS(114个),CSS(108个),Flow(72个),JSON(53个),Markdown(47个),Java源文件(14个)。
资源推荐
资源详情
资源评论
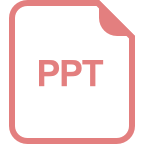
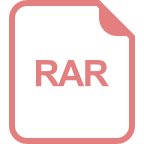
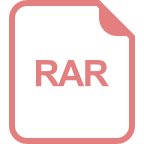
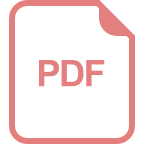
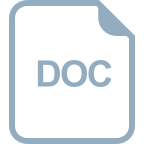
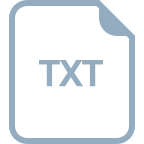
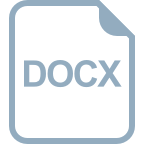
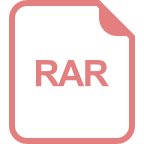
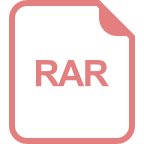
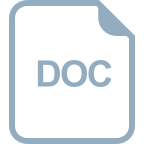
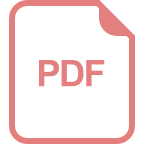
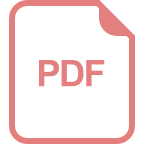
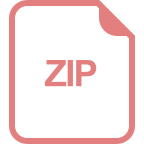
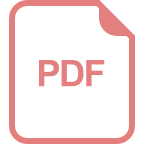
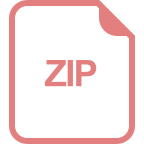
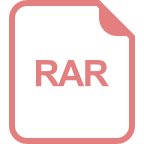
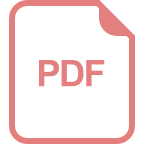
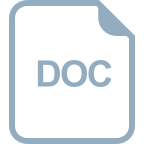
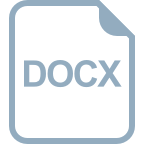
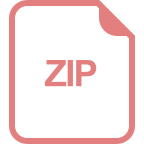
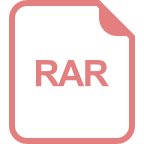
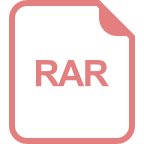
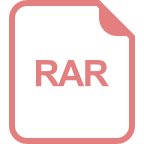
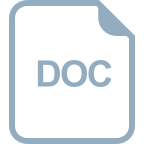
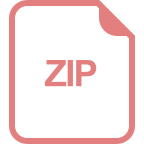
收起资源包目录

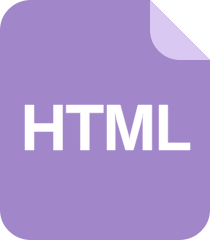
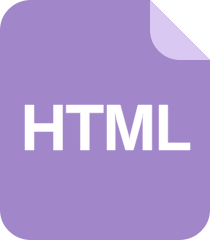
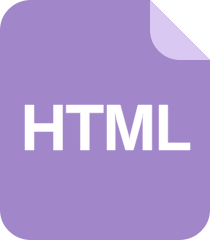
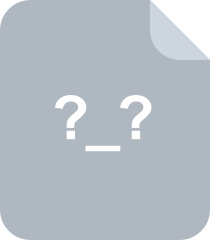
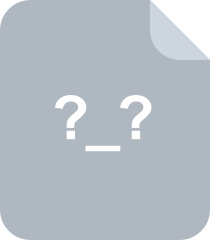
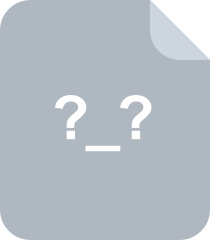
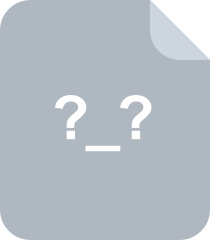
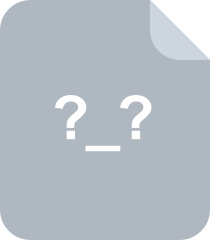
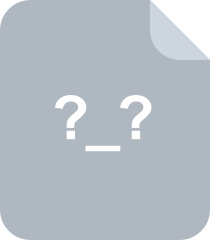
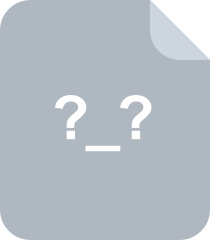
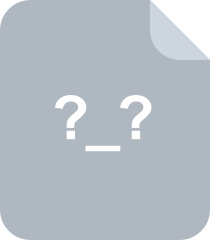
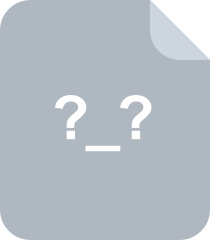
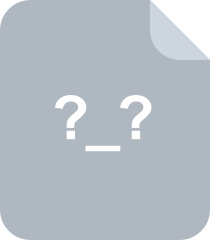
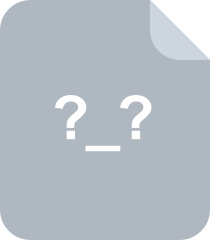
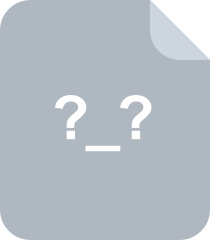
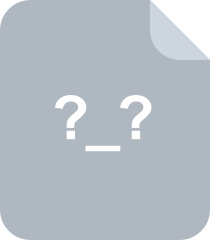
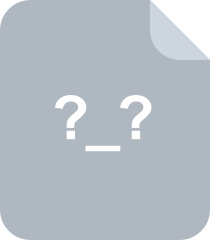
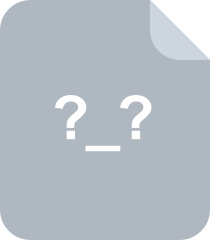
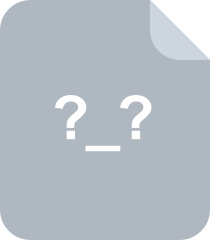
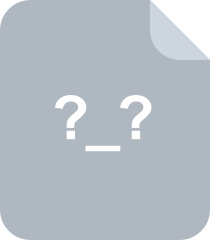
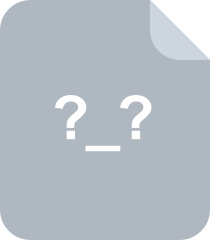
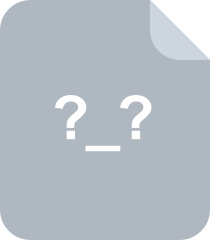
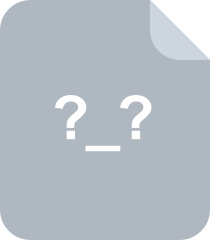
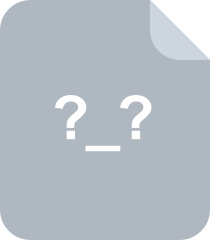
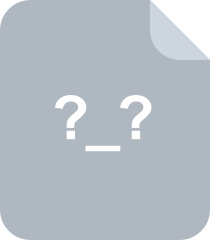
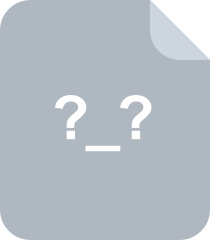
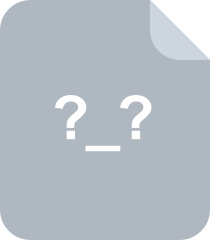
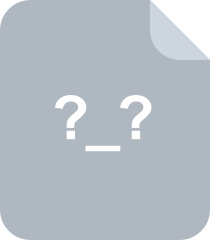
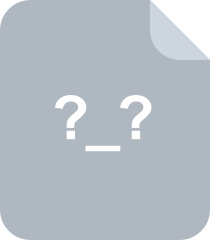
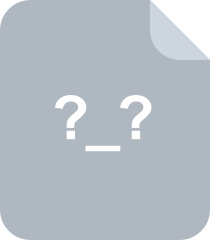
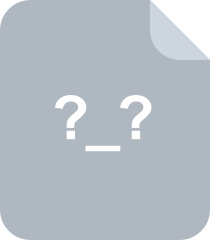
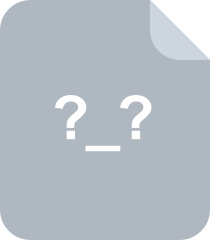
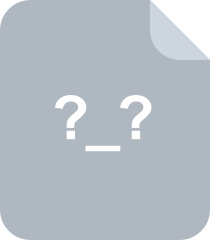
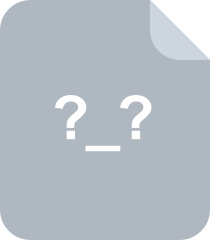
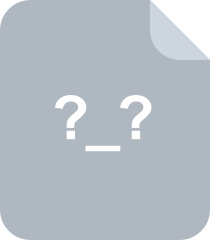
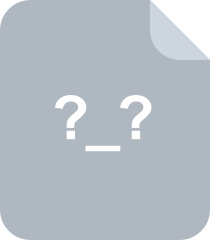
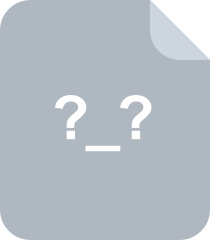
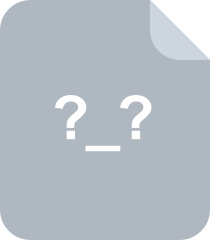
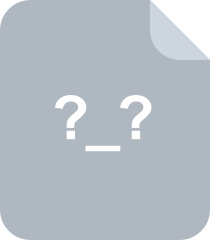
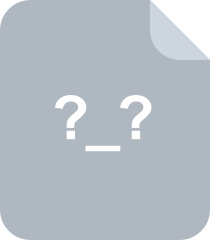
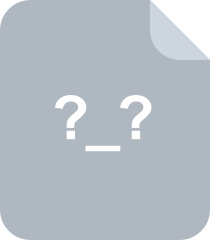
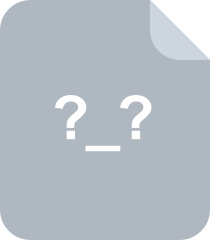
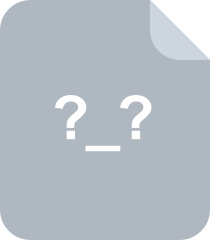
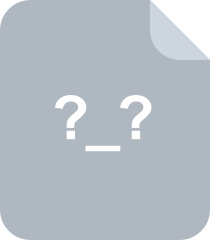
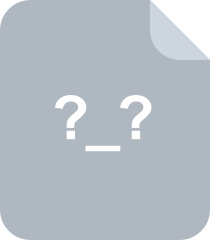
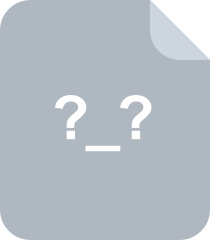
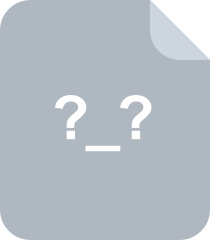
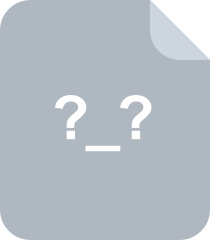
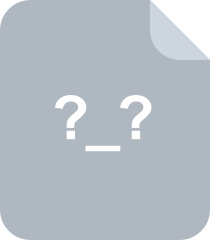
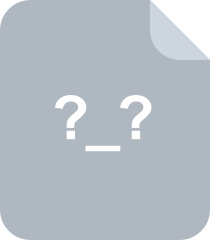
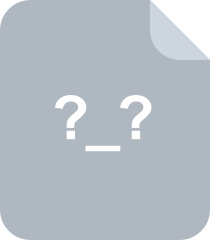
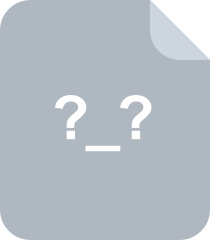
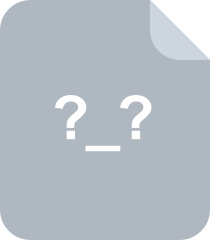
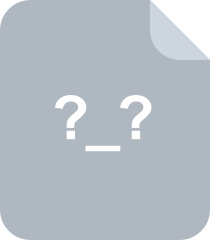
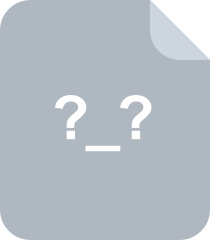
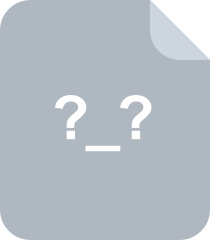
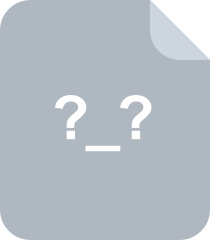
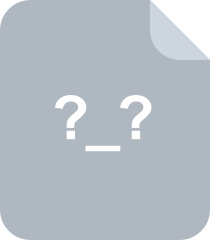
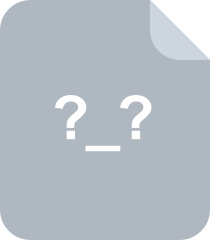
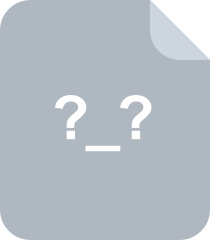
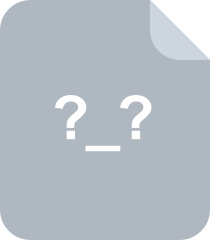
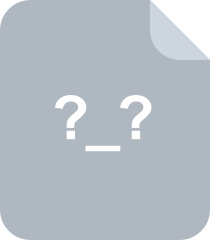
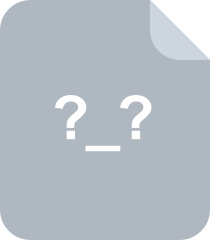
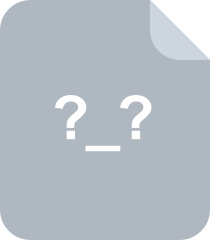
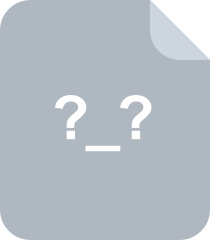
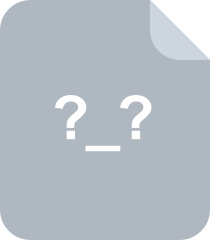
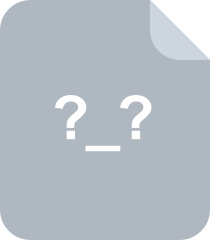
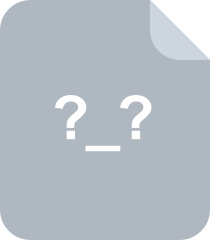
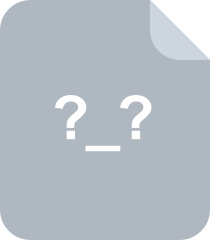
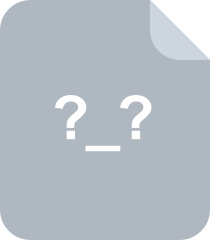
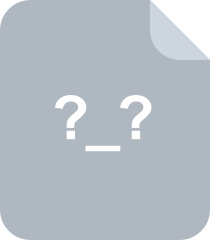
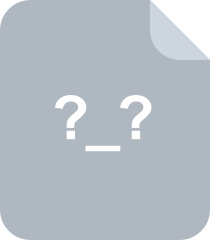
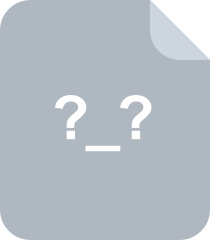
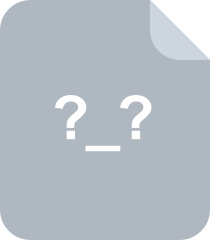
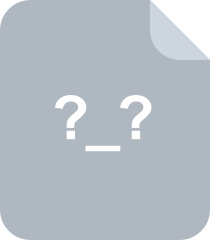
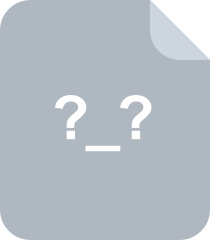
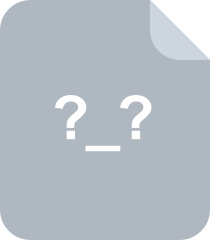
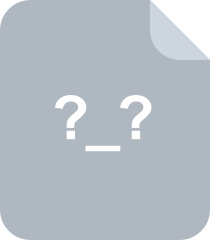
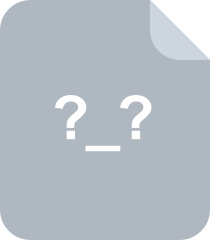
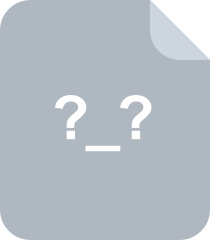
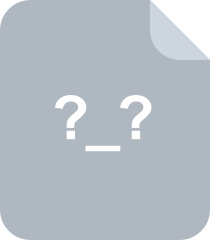
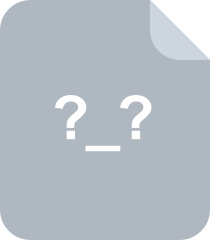
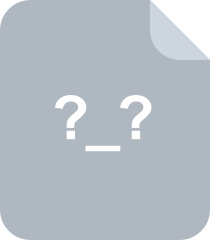
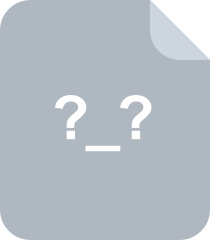
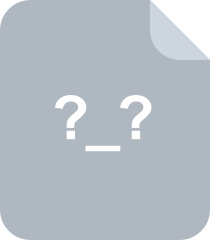
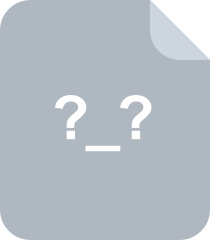
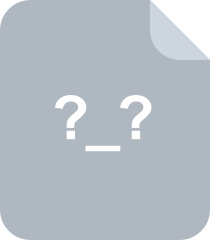
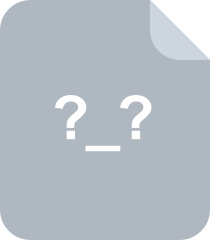
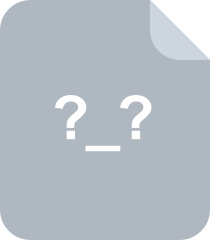
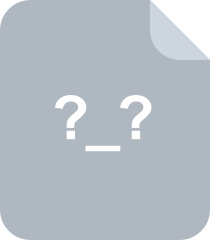
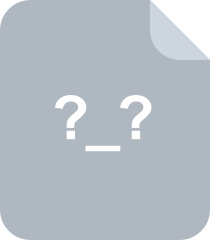
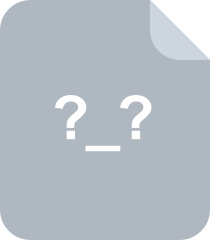
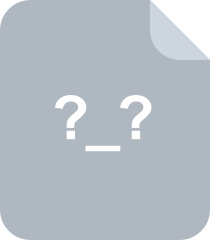
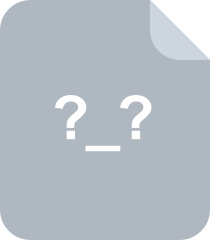
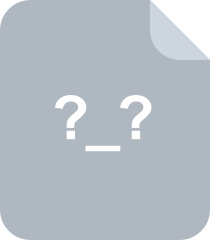
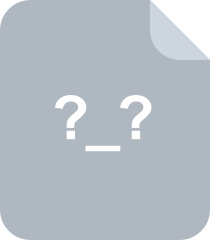
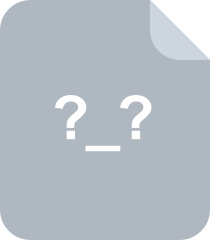
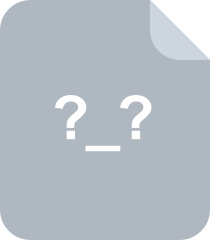
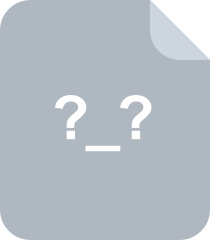
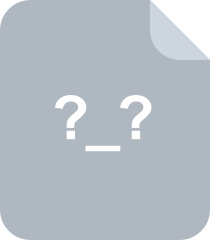
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
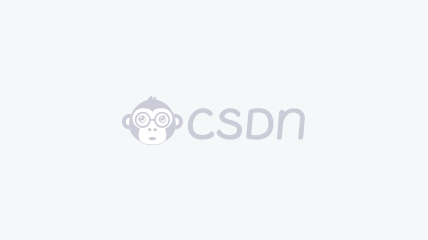

lsx202406
- 粉丝: 2354
- 资源: 5562
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

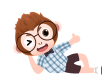
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


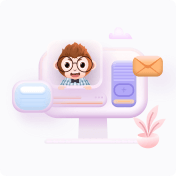
安全验证
文档复制为VIP权益,开通VIP直接复制
