package s2jsp.bysj.dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.List;
import s2jsp.bysj.entity.Product;
public class ProductDao extends BaseDao {
private Connection conn = null; // 保存数据库连接
private PreparedStatement pstmt = null; // 用于执行SQL语句
private ResultSet rs = null; // 用户保存查询结果集
public static final int PAGE_NUM = 5;// 定义产品列表页面显示产品个数
/**
* 查询所有产品信息
*
* @return 产品信息列表
*/
public List findAllProduct() {
List list = new ArrayList();
String sql = "select * from product ";
try {
conn = this.getConn();
pstmt = conn.prepareStatement(sql);
rs = pstmt.executeQuery();
while (rs.next()) {
Product product = new Product();
product.setProductID(rs.getInt("productID"));
product.setSerialNumber(rs.getString("serialNumber"));
product.setName(rs.getString("name"));
product.setPrice(rs.getDouble("price"));
product.setBrand(rs.getString("brand"));
product.setModel(rs.getString("model"));
product.setPicture(rs.getString("picture"));
product.setDescription(rs.getString("description"));
list.add(product);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
this.closeAll(conn, pstmt, rs);
}
return list;
}
/**
* 根据产品ID查找相关产品信息
*
* @return 产品对象
*/
public Product findProductByID(String productID) {
Product product = null;
String sql = "select * from product where productID = ? ";
try {
conn = this.getConn();
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, productID);
rs = pstmt.executeQuery();
if (rs.next()) {
product = new Product();
product.setProductID(rs.getInt("productID"));
product.setSerialNumber(rs.getString("serialNumber"));
product.setName(rs.getString("name"));
product.setPrice(rs.getDouble("price"));
product.setBrand(rs.getString("brand"));
product.setModel(rs.getString("model"));
product.setPicture(rs.getString("picture"));
product.setDescription(rs.getString("description"));
}
} catch (Exception e) {
e.printStackTrace();
} finally {
this.closeAll(conn, pstmt, rs);
}
return product;
}
/**
* 实现产品分页显示
*
* @param page
* 当前页数
* @return 显示产品的列表
*/
public List showProductForPage(int page) {
List list = new ArrayList();
int number = 0;
if (page > 1) {
number = PAGE_NUM * (page - 1);
}
String sql = "select top "
+ PAGE_NUM
+ " * from product where productID not in ( select top "
+ number
+ " productID from product order by productID desc ) order by productID desc";
try {
conn = this.getConn();
pstmt = conn.prepareStatement(sql);
rs = pstmt.executeQuery();
while (rs.next()) {
Product product = new Product();
product.setProductID(rs.getInt("productID"));
product.setSerialNumber(rs.getString("serialNumber"));
product.setName(rs.getString("name"));
product.setBrand(rs.getString("brand"));
product.setModel(rs.getString("model"));
product.setPrice(rs.getDouble("price"));
product.setPicture(rs.getString("picture"));
product.setDescription(rs.getString("description"));
list.add(product);
}
} catch (Exception e) {
e.printStackTrace();
} finally {
this.closeAll(conn, pstmt, rs);
}
return list;
}
/**
* 根据产品ID删除相关产品信息
*
* @param productID
* 产品ID
* @return 执行SQL语句影响数据库的行数
*/
public int deleteProductByID(String productID) {
String sql = "delete from product where productID = ? ";
String[] param = new String[] { productID };
int count = this.executeSQL(sql, param);
return count;
}
/**
* 添加产品信息
*
* @param product
* 封装了信息的产品对象
* @return 执行SQL语句所影响数据库的行数
*/
public int insertProduct(Product product) {
String sql = "insert into product values(?,?,?,?," + product.getPrice()
+ ",?,?)";
String[] param = new String[] { product.getSerialNumber(),
product.getName(), product.getBrand(), product.getModel(),
product.getPicture(), product.getDescription() };
return this.executeSQL(sql, param);
}
/**
* 根据商品ID和对象参数修改商品信息
* @param product封装了数据的商品对象
* @return 返回执行SQL语句,数据库影响行数
*/
public int updateProduct(Product product) {
String sql = "update product set serialnumber=?,name=?,brand=?,model=?,price="
+ product.getPrice()
+ ",picture=?,description=? where productID="
+ product.getProductID();
String[] param = new String[] { product.getSerialNumber(),
product.getName(), product.getBrand(), product.getModel(),
product.getPicture(), product.getDescription() };
return this.executeSQL(sql, param);
}
}

wangwei753
- 粉丝: 4
- 资源: 6
最新资源
- go-zero和gorm构建的分布式微服务后端权限管理系统(含前端+后端完整 源码).zip
- GA优化BP神经网络项目-基于Python(含源码+项目说明+设计报告).zip
- Golang开发的微服务博客系统+设计文档资料等(包括博文、评论、用户服务等模块).zip
- Go语言编写的区块链可信对账系统(含源码及设计报告).zip
- Go语言构建的简易区块链demo实验(包含完整代码和指导手册).zip
- Go语言搭建多节点区块链网络实战指南(含源码及设计文档).zip
- Go语言开发微信视频号自动回复打招呼服务端项目源码+设计文档.zip
- GPT-SoVITS推理-中文情绪分析自动切换参考音含源码.zip
- Hyperledger Fabric支持的分布式身份可信认证区块链解决方案(含链码、启动脚本及SDK).zip
- IPCC2020初赛题目-Stencil代码实现+项目说明.zip
- Java开发基于SpringCloud-Kubernetes的微服务架构实例(含源码).zip
- JavaWeb前后端搜索服务系统-基于SpringBoot+Elasticsearch+SparkMLib开发(含源码+项目说明+实验报告).zip
- Jenkins+Kubernetes搭建自动化部署平台指南(附源码).zip
- JPEG图像压缩算法在STM32平台的实现(含源码+项目说明+主要算法介绍).zip
- k8s和Argo工作流结合构建的安全扫描器系统设计源码+设计文档.zip
- Kaggle猫狗分类竞赛-基于PyTorch(准确率超过99%,含源码+项目说明+设计报告).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


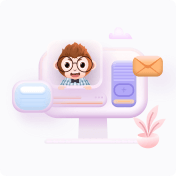