% MFO-BP algorithm for predicting concrete strength
% Date: 2024-5-4
% User: Shuqian Wang
% --------------------------
function varargout = MFO_BP(varargin)
% Begin initialization code - DO NOT EDIT
gui_Singleton = 1;
gui_State = struct('gui_Name', mfilename, ...
'gui_Singleton', gui_Singleton, ...
'gui_OpeningFcn', @MFO_BP_OpeningFcn, ...
'gui_OutputFcn', @MFO_BP_OutputFcn, ...
'gui_LayoutFcn', [] , ...
'gui_Callback', []);
if nargin && ischar(varargin{1})
gui_State.gui_Callback = str2func(varargin{1});
end
if nargout
[varargout{1:nargout}] = gui_mainfcn(gui_State, varargin{:});
else
gui_mainfcn(gui_State, varargin{:});
end
% End initialization code - DO NOT EDIT
% --- Executes just before MFO_BP is made visible.
function MFO_BP_OpeningFcn(hObject, eventdata, handles, varargin)
% This function has no output args, see OutputFcn.
% hObject handle to figure
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% varargin command line arguments to MFO_BP (see VARARGIN)
% Choose default command line output for MFO_BP
handles.output = hObject;
% Update handles structure
guidata(hObject, handles);
% UIWAIT makes MFO_BP wait for user response (see UIRESUME)
% uiwait(handles.figure1);
% --- Outputs from this function are returned to the command line.
function varargout = MFO_BP_OutputFcn(hObject, eventdata, handles)
% varargout cell array for returning output args (see VARARGOUT);
% hObject handle to figure
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% Get default command line output from handles structure
varargout{1} = handles.output;
function edit1_Callback(hObject, eventdata, handles)
% --- Executes during object creation, after setting all properties.
function edit1_CreateFcn(hObject, eventdata, handles)
if ispc && isequal(get(hObject,'BackgroundColor'), get(0,'defaultUicontrolBackgroundColor'))
set(hObject,'BackgroundColor','white');
end
function edit2_Callback(hObject, eventdata, handles)
% --- Executes during object creation, after setting all properties.
function edit2_CreateFcn(hObject, eventdata, handles)
if ispc && isequal(get(hObject,'BackgroundColor'), get(0,'defaultUicontrolBackgroundColor'))
set(hObject,'BackgroundColor','white');
end
function edit3_Callback(hObject, eventdata, handles)
% --- Executes during object creation, after setting all properties.
function edit3_CreateFcn(hObject, eventdata, handles)
if ispc && isequal(get(hObject,'BackgroundColor'), get(0,'defaultUicontrolBackgroundColor'))
set(hObject,'BackgroundColor','white');
end
function edit4_Callback(hObject, eventdata, handles)
% --- Executes during object creation, after setting all properties.
function edit4_CreateFcn(hObject, eventdata, handles)
if ispc && isequal(get(hObject,'BackgroundColor'), get(0,'defaultUicontrolBackgroundColor'))
set(hObject,'BackgroundColor','white');
end
function edit5_Callback(hObject, eventdata, handles)
% --- Executes during object creation, after setting all properties.
function edit5_CreateFcn(hObject, eventdata, handles)
if ispc && isequal(get(hObject,'BackgroundColor'), get(0,'defaultUicontrolBackgroundColor'))
set(hObject,'BackgroundColor','white');
end
% --- Executes on button press in pushbutton1.
function pushbutton1_Callback(hObject, eventdata, handles)
%% Data preprocessing
predit_num = handles.predit;
train_num = handles.train_num;
test_num = handles.test_num;
filename = 'Data.xlsx'; % Excel filename
P = xlsread(filename); % Read data
save('Data.mat', 'P'); % Save as .mat file
P_train = P(1:train_num,1:6); % Rows 1-train_num are used as training data, and columns 1-6 of the input data dimension
T_train = P(1:train_num,predit_num); % Rows 1-train_num are used as training data, and columns predit_num as the final predicted indicator
P_test = P(1:test_num,1:6); % Rows 1-test_num are used as testing data, and columns 1-6 of the input data dimension
T_test = P(1:test_num,predit_num); % Rows 1-test_num are used as testing data, and columns predit_num as the final predicted indicator
data = P(:, 7:8);
handles.list.Data = P;
handles.list.ColumnName = {'MgO', 'HCSA', '水(t)', '水泥(t)', '沙子(t)', '水胶比', '7d抗压强度', '28d抗压强度'};
%% Get the number of nodes in the input and output layers
% Initialize the number of neurons in the hidden layer
inputnum = size(P_train,2); % Number of nodes in the input layer
hiddennum = 2 * inputnum + 1; % Number of nodes in the hidden layer
outputnum = size(T_train,2); % Number of nodes in the output layer
w1num = inputnum * hiddennum; % Number of weights from input to hidden layer
w2num = outputnum * hiddennum; % Number of weights from hidden to output layer
dim = w1num + hiddennum + w2num + outputnum; % Number of variables to be optimized
% warning('off')
%% Define algorithm parameters
N = 50; % Population size
Max_iteration = 50; % Maximum number of iterations
lb = -0.5; % Lower bound
ub = 0.5; % Upper bound
% Initialize moth positions
Moth_pos = initialization(N,dim,ub,lb);
Convergence_curve = zeros(1,Max_iteration);
Iteration = 1;
tic;
while Iteration < Max_iteration + 1
Flame_no = round(N - Iteration * ((N - 1)/Max_iteration)); % Calculate flame number based on iteration
for i = 1:size(Moth_pos,1)
% Check if moths are out of search space
Flag4ub = Moth_pos(i,:) > ub;
Flag4lb = Moth_pos(i,:) < lb;
Moth_pos(i,:) = (Moth_pos(i,:) .* (~(Flag4ub+Flag4lb))) + ub .* Flag4ub + lb .* Flag4lb;
% Calculate fitness function
X = Moth_pos(i,:);
Moth_fitness(1,i) = Objfun1(X, P_train, T_train, hiddennum, P_test, T_test);
% Moth_fitness(1,i)=fobj(Moth_pos(i,:));
end
if Iteration == 1
% Sort the first batch of moths
[fitness_sorted I] = sort(Moth_fitness);
sorted_population = Moth_pos(I,:);
% Update
best_flames = sorted_population;
best_flame_fitness = fitness_sorted;
else
% Sort
double_population = [previous_population;best_flames];
double_fitness = [previous_fitness best_flame_fitness];
[double_fitness_sorted I] = sort(double_fitness);
double_sorted_population = double_population(I,:);
fitness_sorted = double_fitness_sorted(1:N);
sorted_population = double_sorted_population(1:N,:);
% Update
best_flames = sorted_population;
best_flame_fitness = fitness_sorted;
end
% Update the best flame position obtained so far
Best_flame_score = fitness_sorted(1);
Best_flame_pos = sorted_population(1,:);
previous_population = Moth_pos;
previous_fitness = Moth_fitness;
% Update a linearly decreasing from -1 to -2
a = -1 + Iteration * ((-1)/Max_iteration);
for i = 1:size(Moth_pos,1)
for j = 1:size(Moth_pos,2)
if i <= Flame_no % Update moth position relative to respective flame
distance_to_flame = abs(sorted_population(i,j) - Moth_pos(i,j));
b = 1;
t = (a-1) * rand + 1;
Moth_pos(i,j) = distance_to_flame * exp(b.*t) .* cos(t.*2*pi) + sorted_population(i,j);
end
if i > Flame_no % Adjust moth position using a single flame
distance_to_flame = abs(sorted_population(i,j) - Moth_pos(i,j));
b = 1;
t = (a-1) * rand + 1;
Moth_pos(i,j) = distance_to_flame * exp(b.*t) .* cos(t.*2*pi) + sorted_population(Flame_no,j);
end
end
end
Convergence_curve(Iteration) = Best_flame_score;
% Display the iteration and best solution obtained so far
if m
没有合适的资源?快使用搜索试试~ 我知道了~
基于飞蛾扑火算法与反向传播算法的混凝土强度预测:MFO-BP
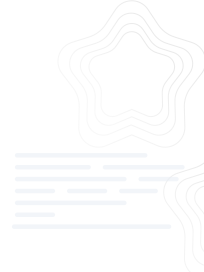
共7个文件
m:4个
xlsx:1个
mat:1个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 124 浏览量
2024-12-21
13:03:17
上传
评论
收藏 102KB RAR 举报
温馨提示
内容概要: 本资料介绍了一种基于飞蛾扑火算法(Moth Flame Optimization, MFO)与反向传播算法(Backpropagation, BP)的混凝土强度预测模型。飞蛾扑火算法是一种新兴的元启发式优化算法,它模拟了飞蛾在自然界中向光源飞行的行为,用于寻找最优解。结合反向传播算法,该模型能够优化神经网络的权重和偏置,以提高混凝土强度预测的准确性。这种集成方法特别适用于处理具有多个输入变量和复杂非线性关系的工程问题。 其他说明: 算法优势:飞蛾扑火算法以其简单的数学模型和强大的全局搜索能力,为神经网络的训练提供了有效的参数优化。 实现方式:该模型的实现通常需要编程技能,通过MATLAB编程语言进行开发。 性能评估:模型的预测性能可以通过决定系数(R²)、均方误差(MSE)等统计指标进行评估 实现:包含GUI界面,附赠案例数据可直接运行matlab程序。
资源推荐
资源详情
资源评论
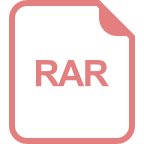
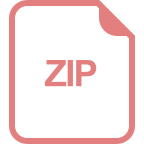
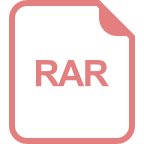
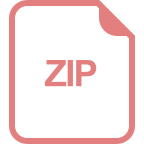
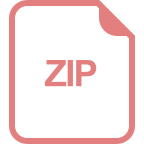
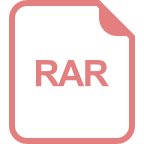
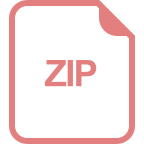
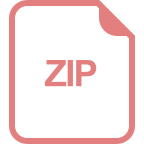
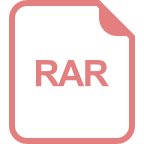
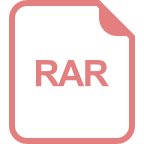
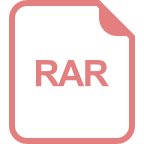
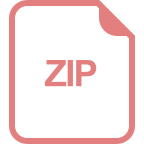
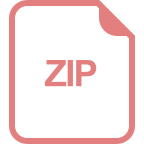
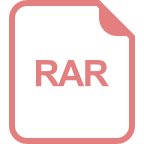
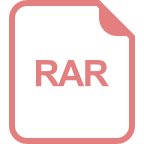
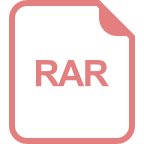
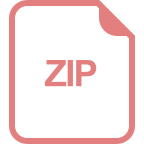
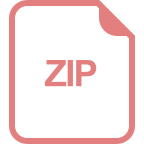
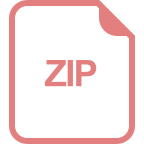
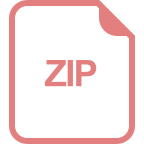
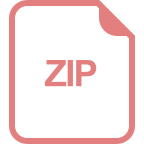
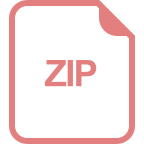
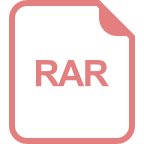
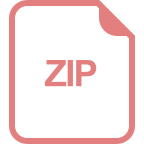
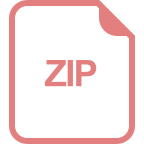
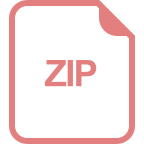
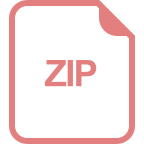
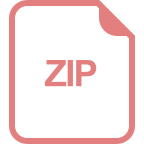
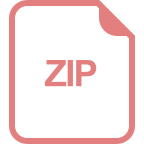
收起资源包目录



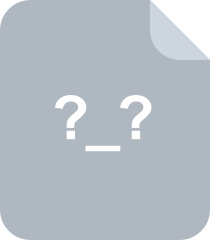
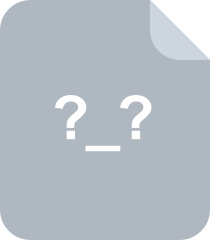
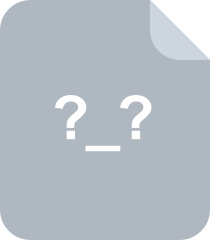
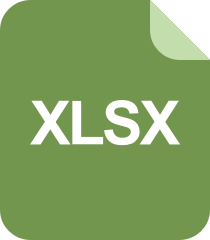
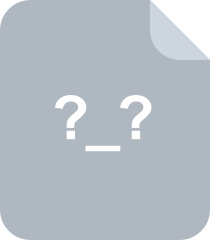
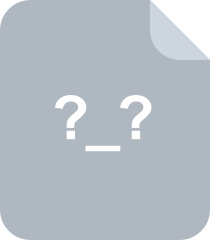
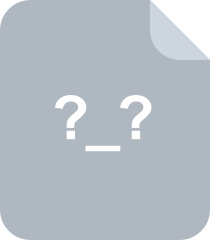
共 7 条
- 1
资源评论
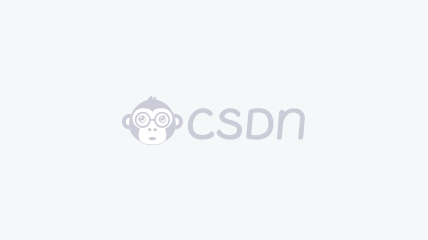

电科_银尘
- 粉丝: 1w+
- 资源: 170
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

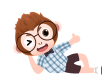
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


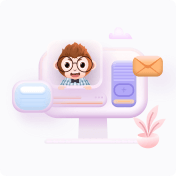
安全验证
文档复制为VIP权益,开通VIP直接复制
