/*
** The OpenGL Extension Wrangler Library
** Copyright (C) 2002-2008, Milan Ikits <milan ikits[]ieee org>
** Copyright (C) 2002-2008, Marcelo E. Magallon <mmagallo[]debian org>
** Copyright (C) 2002, Lev Povalahev
** All rights reserved.
**
** Redistribution and use in source and binary forms, with or without
** modification, are permitted provided that the following conditions are met:
**
** * Redistributions of source code must retain the above copyright notice,
** this list of conditions and the following disclaimer.
** * Redistributions in binary form must reproduce the above copyright notice,
** this list of conditions and the following disclaimer in the documentation
** and/or other materials provided with the distribution.
** * The name of the author may be used to endorse or promote products
** derived from this software without specific prior written permission.
**
** THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
** AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
** IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
** ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
** LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
** CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
** SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
** INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
** CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
** ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF
** THE POSSIBILITY OF SUCH DAMAGE.
*/
#include <GL/glew.h>
#if defined(_WIN32)
# include <GL/wglew.h>
#elif !defined(__APPLE__) || defined(GLEW_APPLE_GLX)
# include <GL/glxew.h>
#endif
/*
* Define glewGetContext and related helper macros.
*/
#ifdef GLEW_MX
# define glewGetContext() ctx
# ifdef _WIN32
# define GLEW_CONTEXT_ARG_DEF_INIT GLEWContext* ctx
# define GLEW_CONTEXT_ARG_VAR_INIT ctx
# define wglewGetContext() ctx
# define WGLEW_CONTEXT_ARG_DEF_INIT WGLEWContext* ctx
# define WGLEW_CONTEXT_ARG_DEF_LIST WGLEWContext* ctx
# else /* _WIN32 */
# define GLEW_CONTEXT_ARG_DEF_INIT void
# define GLEW_CONTEXT_ARG_VAR_INIT
# define glxewGetContext() ctx
# define GLXEW_CONTEXT_ARG_DEF_INIT void
# define GLXEW_CONTEXT_ARG_DEF_LIST GLXEWContext* ctx
# endif /* _WIN32 */
# define GLEW_CONTEXT_ARG_DEF_LIST GLEWContext* ctx
#else /* GLEW_MX */
# define GLEW_CONTEXT_ARG_DEF_INIT void
# define GLEW_CONTEXT_ARG_VAR_INIT
# define GLEW_CONTEXT_ARG_DEF_LIST void
# define WGLEW_CONTEXT_ARG_DEF_INIT void
# define WGLEW_CONTEXT_ARG_DEF_LIST void
# define GLXEW_CONTEXT_ARG_DEF_INIT void
# define GLXEW_CONTEXT_ARG_DEF_LIST void
#endif /* GLEW_MX */
#if defined(__APPLE__)
#include <stdlib.h>
#include <string.h>
#include <AvailabilityMacros.h>
#ifdef MAC_OS_X_VERSION_10_3
#include <dlfcn.h>
void* NSGLGetProcAddress (const GLubyte *name)
{
static void* image = NULL;
if (NULL == image)
{
image = dlopen("/System/Library/Frameworks/OpenGL.framework/Versions/Current/OpenGL", RTLD_LAZY);
}
return image ? dlsym(image, (const char*)name) : NULL;
}
#else
#include <mach-o/dyld.h>
void* NSGLGetProcAddress (const GLubyte *name)
{
static const struct mach_header* image = NULL;
NSSymbol symbol;
char* symbolName;
if (NULL == image)
{
image = NSAddImage("/System/Library/Frameworks/OpenGL.framework/Versions/Current/OpenGL", NSADDIMAGE_OPTION_RETURN_ON_ERROR);
}
/* prepend a '_' for the Unix C symbol mangling convention */
symbolName = malloc(strlen((const char*)name) + 2);
strcpy(symbolName+1, (const char*)name);
symbolName[0] = '_';
symbol = NULL;
/* if (NSIsSymbolNameDefined(symbolName))
symbol = NSLookupAndBindSymbol(symbolName); */
symbol = image ? NSLookupSymbolInImage(image, symbolName, NSLOOKUPSYMBOLINIMAGE_OPTION_BIND | NSLOOKUPSYMBOLINIMAGE_OPTION_RETURN_ON_ERROR) : NULL;
free(symbolName);
return symbol ? NSAddressOfSymbol(symbol) : NULL;
}
#endif /* MAC_OS_X_VERSION_10_3 */
#endif /* __APPLE__ */
#if defined(__sgi) || defined (__sun)
#include <dlfcn.h>
#include <stdio.h>
#include <stdlib.h>
void* dlGetProcAddress (const GLubyte* name)
{
static void* h = NULL;
static void* gpa;
if (h == NULL)
{
if ((h = dlopen(NULL, RTLD_LAZY | RTLD_LOCAL)) == NULL) return NULL;
gpa = dlsym(h, "glXGetProcAddress");
}
if (gpa != NULL)
return ((void*(*)(const GLubyte*))gpa)(name);
else
return dlsym(h, (const char*)name);
}
#endif /* __sgi || __sun */
/*
* Define glewGetProcAddress.
*/
#if defined(_WIN32)
# define glewGetProcAddress(name) wglGetProcAddress((LPCSTR)name)
#else
# if defined(__APPLE__)
# define glewGetProcAddress(name) NSGLGetProcAddress(name)
# else
# if defined(__sgi) || defined(__sun)
# define glewGetProcAddress(name) dlGetProcAddress(name)
# else /* __linux */
# define glewGetProcAddress(name) (*glXGetProcAddressARB)(name)
# endif
# endif
#endif
/*
* Define GLboolean const cast.
*/
#define CONST_CAST(x) (*(GLboolean*)&x)
/*
* GLEW, just like OpenGL or GLU, does not rely on the standard C library.
* These functions implement the functionality required in this file.
*/
static GLuint _glewStrLen (const GLubyte* s)
{
GLuint i=0;
if (s == NULL) return 0;
while (s[i] != '\0') i++;
return i;
}
static GLuint _glewStrCLen (const GLubyte* s, GLubyte c)
{
GLuint i=0;
if (s == NULL) return 0;
while (s[i] != '\0' && s[i] != c) i++;
return (s[i] == '\0' || s[i] == c) ? i : 0;
}
static GLboolean _glewStrSame (const GLubyte* a, const GLubyte* b, GLuint n)
{
GLuint i=0;
if(a == NULL || b == NULL)
return (a == NULL && b == NULL && n == 0) ? GL_TRUE : GL_FALSE;
while (i < n && a[i] != '\0' && b[i] != '\0' && a[i] == b[i]) i++;
return i == n ? GL_TRUE : GL_FALSE;
}
static GLboolean _glewStrSame1 (GLubyte** a, GLuint* na, const GLubyte* b, GLuint nb)
{
while (*na > 0 && (**a == ' ' || **a == '\n' || **a == '\r' || **a == '\t'))
{
(*a)++;
(*na)--;
}
if(*na >= nb)
{
GLuint i=0;
while (i < nb && (*a)+i != NULL && b+i != NULL && (*a)[i] == b[i]) i++;
if(i == nb)
{
*a = *a + nb;
*na = *na - nb;
return GL_TRUE;
}
}
return GL_FALSE;
}
static GLboolean _glewStrSame2 (GLubyte** a, GLuint* na, const GLubyte* b, GLuint nb)
{
if(*na >= nb)
{
GLuint i=0;
while (i < nb && (*a)+i != NULL && b+i != NULL && (*a)[i] == b[i]) i++;
if(i == nb)
{
*a = *a + nb;
*na = *na - nb;
return GL_TRUE;
}
}
return GL_FALSE;
}
static GLboolean _glewStrSame3 (GLubyte** a, GLuint* na, const GLubyte* b, GLuint nb)
{
if(*na >= nb)
{
GLuint i=0;
while (i < nb && (*a)+i != NULL && b+i != NULL && (*a)[i] == b[i]) i++;
if (i == nb && (*na == nb || (*a)[i] == ' ' || (*a)[i] == '\n' || (*a)[i] == '\r' || (*a)[i] == '\t'))
{
*a = *a + nb;
*na = *na - nb;
return GL_TRUE;
}
}
return GL_FALSE;
}
#if !defined(_WIN32) || !defined(GLEW_MX)
PFNGLCOPYTEXSUBIMAGE3DPROC __glewCopyTexSubImage3D = NULL;
PFNGLDRAWRANGEELEMENTSPROC __glewDrawRangeElements = NULL;
PFNGLTEXIMAGE3DPROC __glewTexImage3D = NULL;
PFNGLTEXSUBIMAGE3DPROC __glewTexSubImage3D = NULL;
PFNGLACTIVETEXTUREPROC __glewActiveTexture = NULL;
PFNGLCLIENTACTIVETEXTUREPROC __glewClientActiveTexture = NULL;
PFNGLCOMPRESSEDTEXIMAGE1DPROC __glewCompressedTexImage1D = NULL;
PFNGLCOMPRESSEDTEXIMAGE2DPROC __glewCompressedTexImage2D = NULL;
PFNGLCOMPRESSEDTEXIMAGE3DPROC __glewCompressedTexImage3D = NULL;
PFNGLCOMPRESSEDTEXSUBIMAGE1DPROC __glewCompressedTexSubImage1D = NULL;
PFNGLCOMPRESSEDTEXSUBIMAGE2DPROC __glew
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
OpenGL经典蓝宝书源代码,大概是应为文件大小的限制,CSDN里找到的都是去除了Xcode文件的版本,对于使用Mac的童鞋很不方便 这个是从svn下下来的,去除了VS相关文件夹,主要针对Mac童鞋,Windows的童鞋请自行搜索其他版本,或者直接去svn下载">OpenGL经典蓝宝书源代码,大概是应为文件大小的限制,CSDN里找到的都是去除了Xcode文件的版本,对于使用Mac的童鞋很不方便 这个是从svn下下来的,去除了VS相关文件夹,主要针对Mac童鞋,Windows的童鞋请自行搜索其他版本,或者? [更多]
资源推荐
资源详情
资源评论
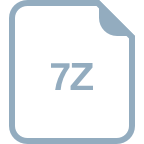
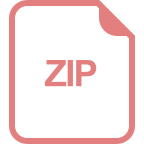
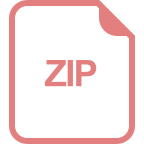
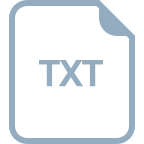
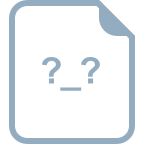
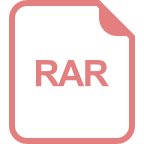
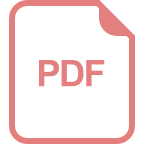
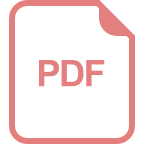
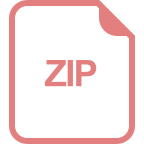
收起资源包目录

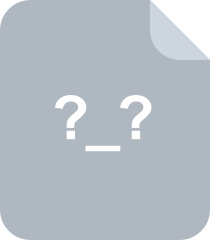
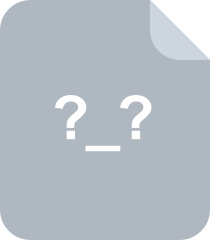
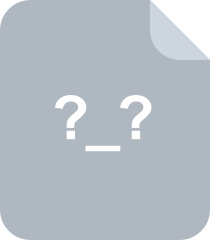
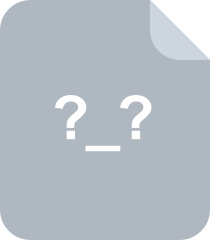
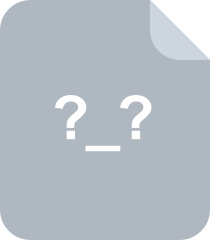
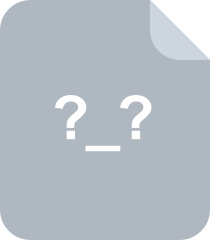
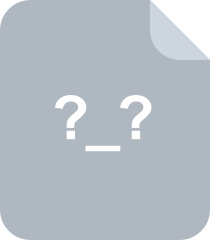
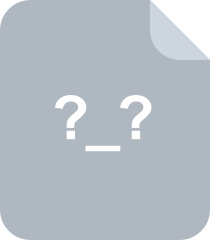
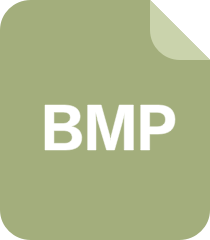
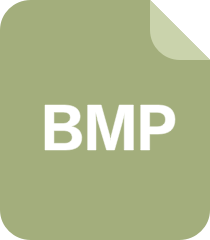
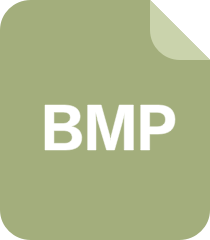
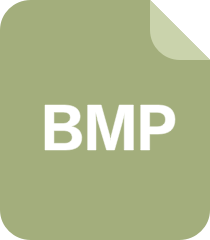
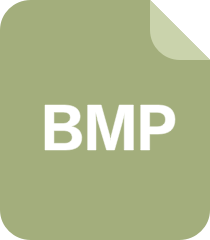
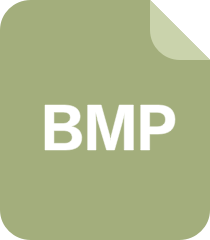
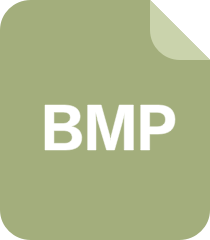
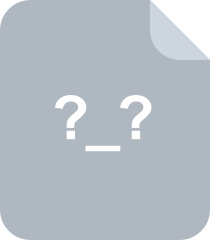
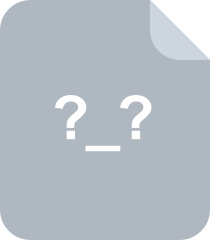
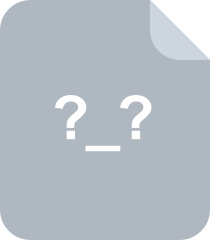
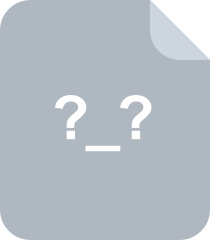
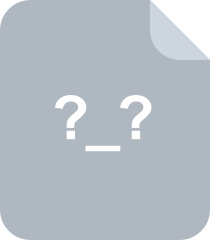
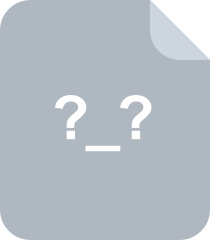
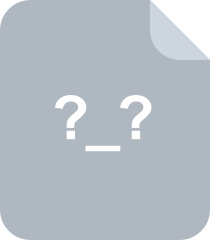
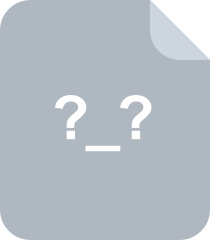
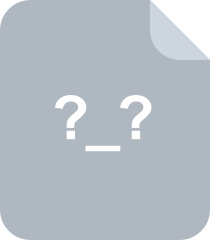
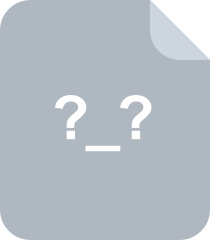
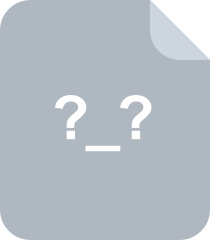
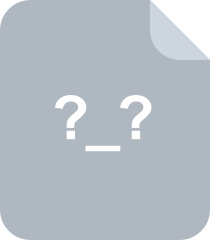
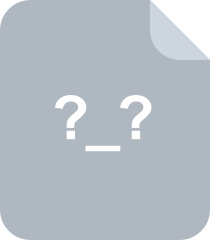
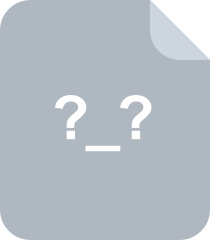
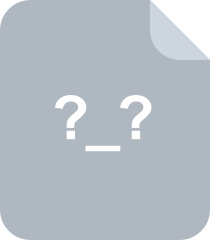
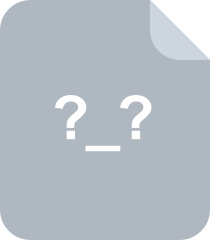
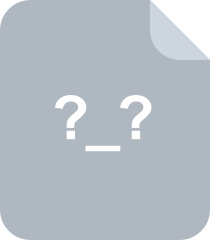
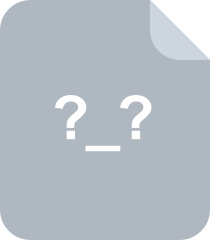
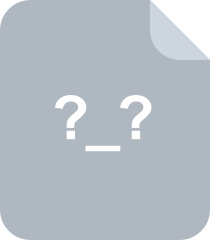
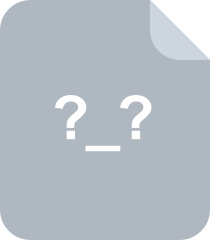
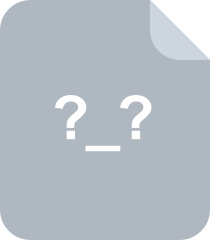
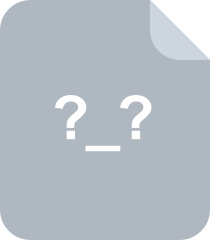
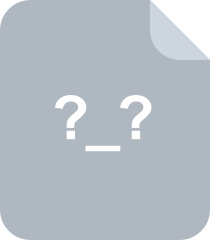
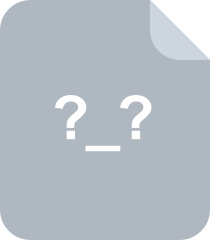
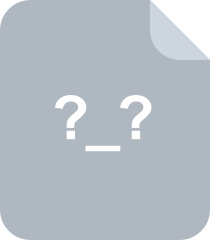
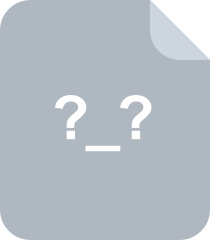
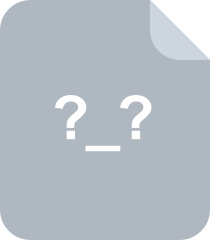
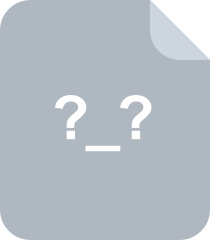
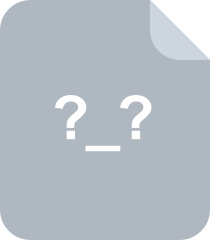
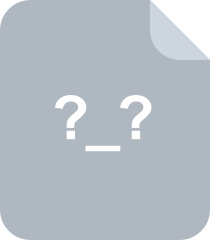
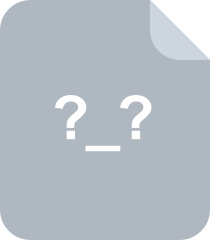
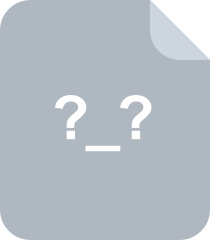
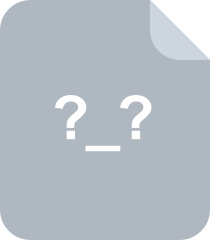
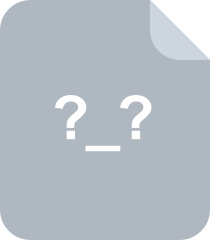
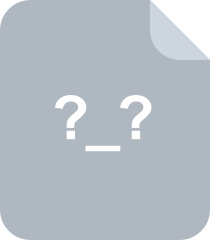
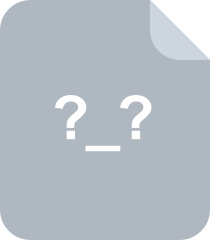
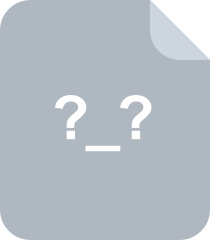
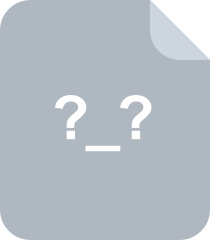
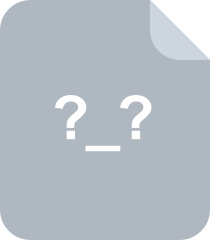
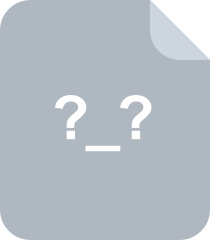
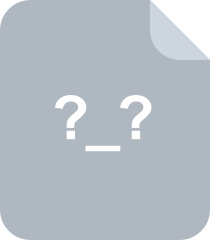
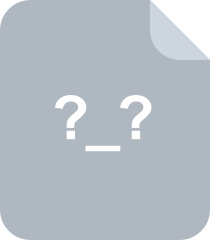
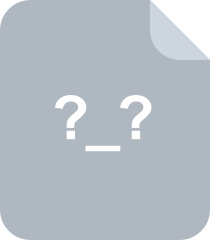
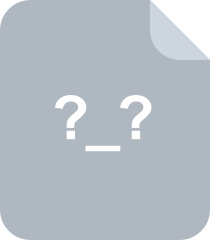
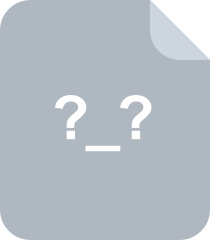
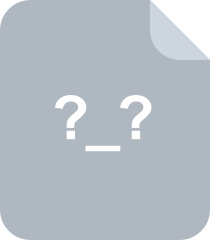
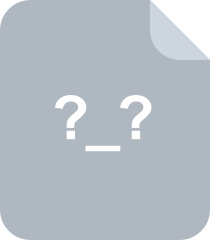
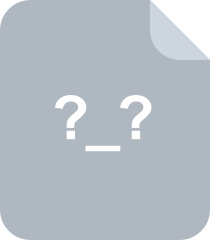
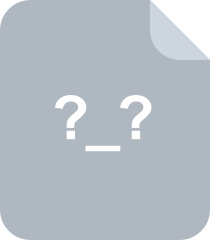
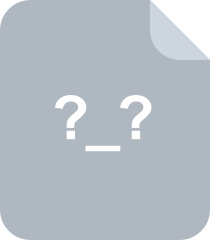
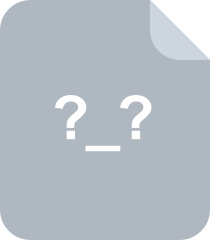
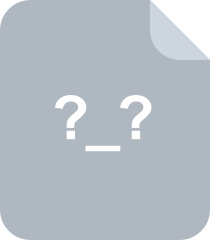
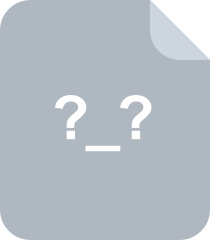
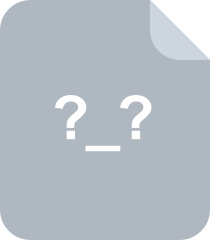
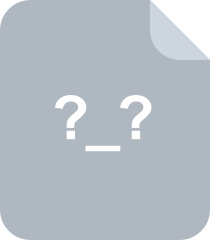
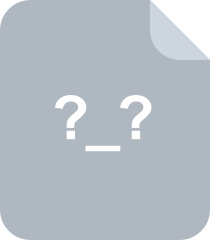
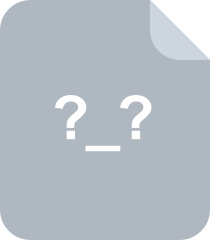
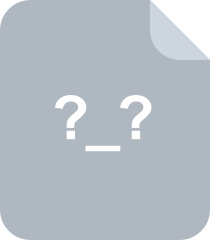
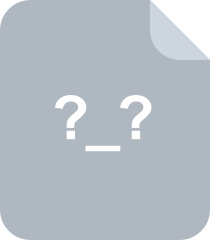
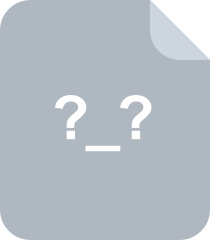
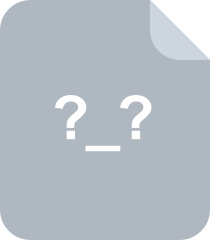
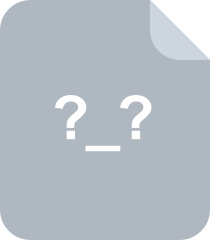
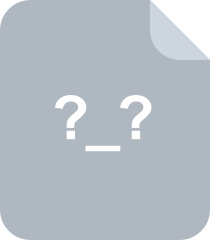
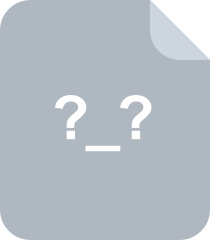
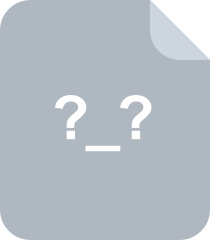
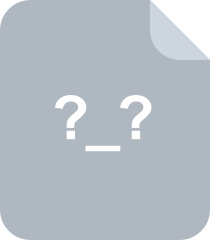
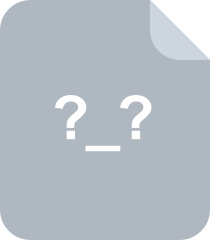
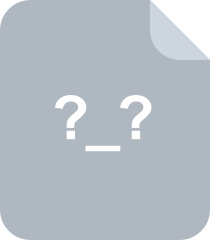
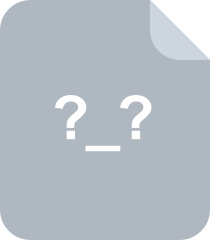
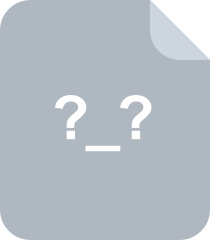
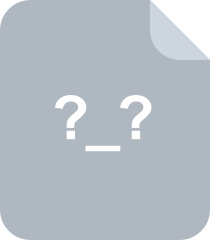
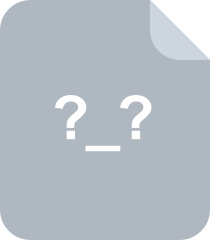
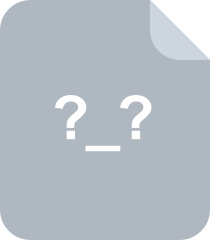
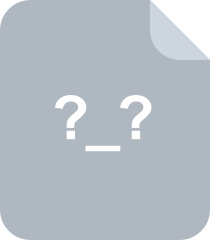
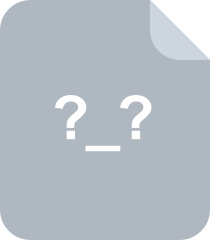
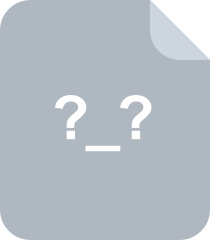
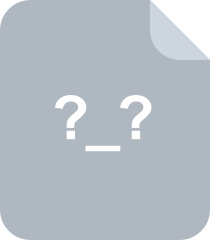
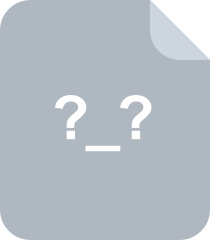
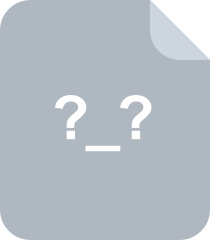
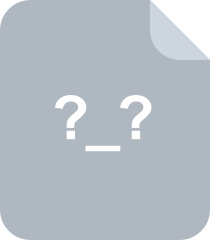
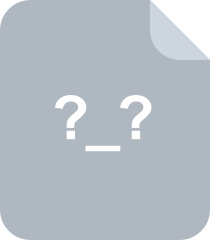
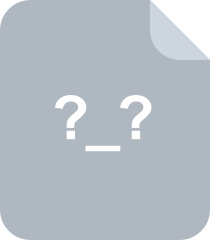
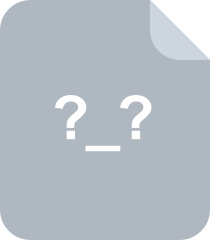
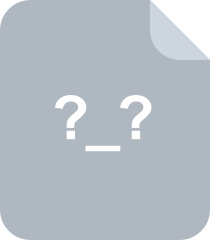
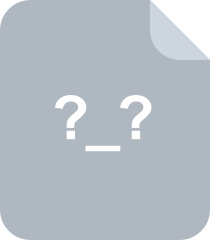
共 504 条
- 1
- 2
- 3
- 4
- 5
- 6

uolks
- 粉丝: 0
- 资源: 3
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

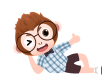
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
- 5
- 6
前往页