图片旋转和图片上添加中文汉字
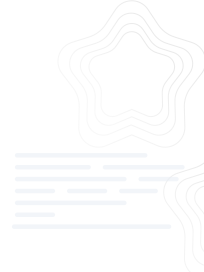

在图像处理领域,OpenCV是一个强大的库,广泛用于各种任务,包括图像旋转和在图片上添加文字。在本文中,我们将深入探讨如何利用OpenCV来实现这些功能,并结合Windows库函数来添加中文汉字。 让我们关注图片旋转。OpenCV中的`cv2.rotate()`函数可以用来旋转图像。这个函数提供了三种旋转选项:`ROTATE_90_CLOCKWISE`、`ROTATE_90_COUNTERCLOCKWISE`、`ROTATE_180`,以及一个自定义旋转角度的`cv2.getRotationMatrix2D()`函数。`getRotationMatrix2D()`需要输入三个参数:中心点坐标(通常是图像的中心),旋转角度,以及缩放因子(保持原图比例时设为1)。得到旋转矩阵后,可以使用`cv2.warpAffine()`进行图像变换。 代码示例: ```python import cv2 # 读取图片 img = cv2.imread('image.jpg') # 计算旋转矩阵 center = (img.shape[1] // 2, img.shape[0] // 2) angle = 45 scale = 1 M = cv2.getRotationMatrix2D(center, angle, scale) # 旋转图片 rotated_img = cv2.warpAffine(img, M, (img.shape[1], img.shape[0])) # 保存结果 cv2.imwrite('rotated_image.jpg', rotated_img) ``` 接下来,我们讨论如何在图片上添加中文汉字。OpenCV本身并不直接支持文本渲染,但可以借助PIL(Python Imaging Library)或者Windows GDI(Graphics Device Interface)库来实现。在Windows环境中,我们可以使用`cv2.putText()`配合`ctypes`模块调用GDI函数来添加中文汉字。 以下是一个示例,演示如何在图像上添加中文汉字: ```python import cv2 import numpy as np from ctypes import windll, Structure, c_int, c_float, byref # 定义结构体 class TEXTMETRIC(Structure): _fields_ = [("tmHeight", c_int), ("tmAscent", c_int), ("tmDescent", c_int), ("tmInternalLeading", c_int), ("tmExternalLeading", c_int), ("tmAveCharWidth", c_int), ("tmMaxCharWidth", c_int), ("tmWeight", c_int), ("tmOverhang", c_int), ("tmDigitizedAspectX", c_int), ("tmDigitizedAspectY", c_int), ("tmFirstChar", c_int), ("tmLastChar", c_int), ("tmDefaultChar", c_int), ("tmBreakChar", c_int), ("tmItalic", c_int), ("tmUnderlined", c_int), ("tmStruckOut", c_int), ("tmPitchAndFamily", c_int), ("tmCharSet", c_int)] # 创建字体对象 font_name = 'SimHei' # 使用SimHei作为中文字体 font_size = 30 hdc = windll.user32.GetDC(0) font = windll.gdi32.CreateFontIndirectW(byref( TEXTMETRIC( tmHeight=font_size, tmAscent=font_size, tmDescent=font_size//4, tmWeight=700, tmCharSet=134 # 134代表GB2312编码,适用于简体中文 ) )) # 设置字体 windll.gdi32.SelectObject(hdc, font) # 在图片上添加文字 img = cv2.imread('image.jpg') text = '你好,世界!' text_pos = (50, 50) text_color = (255, 255, 255) # 白色 text_bg_color = (0, 0, 0) # 黑色 text_width, text_height = windll.gdi32.GetTextExtent(hdc, text.encode('GBK')) # 创建一个背景矩形填充黑色 text_bg = np.ones((text_height, text_width, 3), dtype=np.uint8) * 255 text_bg[:, :] = text_bg_color # 使用GDI函数绘制文本 hmemdc = windll.gdi32.CreateCompatibleDC(hdc) hbitmap = windll.gdi32.CreateCompatibleBitmap(hdc, text_width, text_height) windll.gdi32.SelectObject(hmemdc, hbitmap) windll.gdi32.TextOutW(hdc, 0, 0, text.encode('GBK'), len(text)) windll.gdi32.BitBlt(hdc, text_pos[0], text_pos[1], text_width, text_height, hmemdc, 0, 0, 13369344) # SRCINVERT用于画出反色 # 将文字与图片合并 img[text_pos[1]:text_pos[1]+text_height, text_pos[0]:text_pos[0]+text_width] = cv2.bitwise_and(img[text_pos[1]:text_pos[1]+text_height, text_pos[0]:text_pos[0]+text_width], text_bg) # 释放资源 windll.gdi32.DeleteObject(font) windll.gdi32.DeleteObject(hbitmap) windll.gdi32.DeleteDC(hdc) windll.user32.ReleaseDC(0, hdc) # 保存结果 cv2.imwrite('text_image.jpg', img) ``` 在这个例子中,我们首先定义了一个TEXTMETRIC结构体来创建一个字体,然后使用GDI的`TextOutW()`函数在内存设备上下文(hdc)中绘制中文文本。通过`BitBlt()`函数将文本反色部分与原图像进行位运算合并,从而在图片上添加了中文汉字。 OpenCV结合其他库如PIL或Windows GDI,可以方便地实现图片的旋转和在图片上添加中文汉字的功能。无论是简单的图像操作还是复杂的图像处理任务,OpenCV都能提供强大的支持。
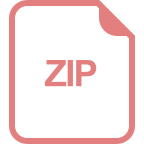
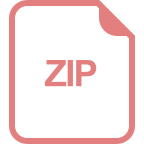
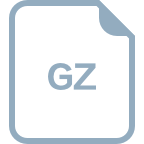
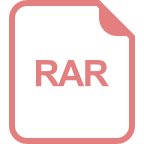
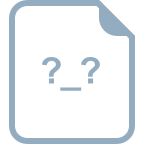
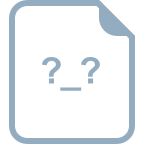
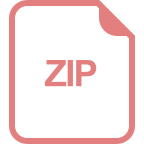
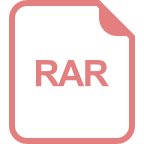
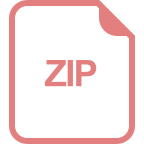
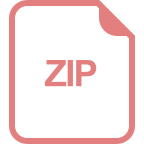
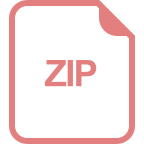
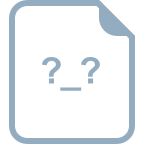
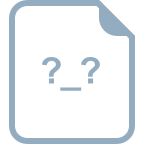
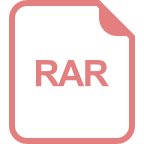
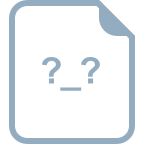
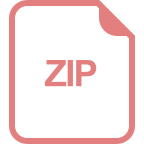
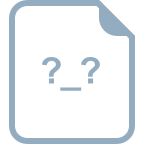
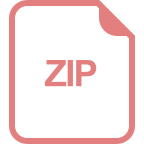
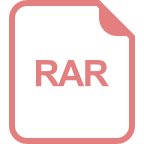
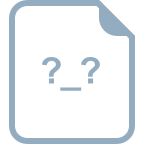
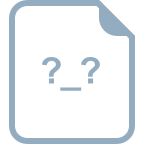
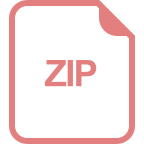


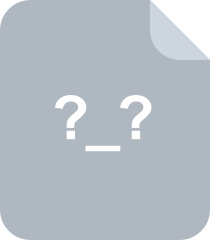
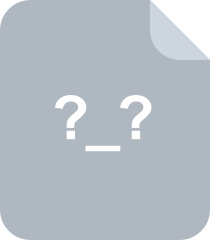
- 1
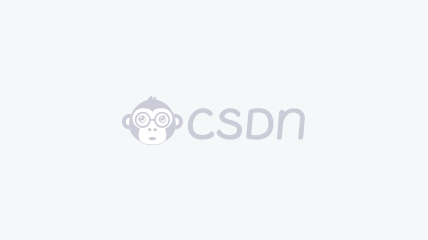

- 粉丝: 21
- 资源: 4
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

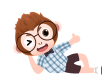
最新资源
- Gradle,Maven 插件将 Java 应用程序打包为原生 Windows、MacOS 或 Linux 可执行文件并为其创建安装程序 .zip
- Google Maps API Web 服务的 Java 客户端库.zip
- Google Java 核心库.zip
- GitBook 教授 Javascript 编程基础知识.zip
- Generation.org 开发的 JAVA 模块练习.zip
- FastDFS Java 客户端 SDK.zip
- etcd java 客户端.zip
- Esercizi di informatica!执行计划,metti alla prova!.zip
- Eloquent JavaScript 翻译 - 2ª edição .zip
- Eclipse Paho Java MQTT 客户端库 Paho 是一个 Eclipse IoT 项目 .zip

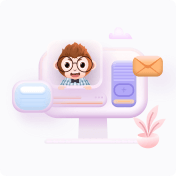
