//=============================================================
// 文件名称: gps.c
// 功能描述: GPS数据解析
// 维护记录: 2010-01-31 V1.0
//=============================================================
#include <string.h>
#include <stdlib.h>
#include "2440addr.h"
#include "2440lib.h"
#include "def.h"
#include "gps.h"
#include "uart.h"
#include "algorithm.h"
#define UART2_CH0 0
#define UART2_CH1 1
#define LEDOFF rGPFDAT = 0xFF
#define LED1ON rGPFDAT &= ~(0x01)
#define LED2ON rGPFDAT &= ~(0x02)
#define LED3ON rGPFDAT &= ~(0x04)
#define LED1OFF rGPFDAT |= 0x01
#define LED2OFF rGPFDAT |= 0x02
#define LED3OFF rGPFDAT |= 0x04
#define REV_YES LED1ON
#define REV_NO LED1OFF
#define RMC_YES LED2ON
#define RMC_NO LED2OFF
#define GGA_YES LED3ON
#define GGA_NO LED3OFF
extern unsigned int PCLK;
GPS_INFO *GPS; //GPS结构体
volatile U32 isRxInt; //串口接收标志
volatile U32 gps_rGPHCON,gps_rGPHDAT,gps_rGPHUP;
volatile U32 gps_ULCON2,gps_UCON2,gps_UFCON2,gps_UMCON2,gps_UBRDIV2;
char rev_buf[80]; //接收缓存
U8 rev_start,rev_stop,gps_flag; //开始接收,停止接收,gps开始处理
U8 num = 0;
void __sub_Uart2_RxInt(void);
void __sub_Uart2_RxErrInt(void);
void __irq Uart2_RxIntOrErr(void);
void UTC2BTC(DATE_TIME *);
void Select_Device(char Device)
{
rGPHCON |= 1<<(UART2_CH1<<1);
rGPHCON &= ~(1<<(UART2_CH1<<1)+1);
rGPHCON |= 1<<(UART2_CH0<<1);
rGPHCON &= ~(1<<(UART2_CH0<<1)+1); //设置为输出口
rGPHUP &=(~(0x03));
rGPHDAT =(rGPHDAT&0xfffffffc)|(Device);
}
void GPS_Port_Set(void)
{
gps_rGPHCON=rGPHCON;
gps_rGPHDAT=rGPHDAT;
gps_rGPHUP=rGPHUP;
rGPHCON&=0x3c0fff;
rGPHCON|=0x2a000;
rGPHUP|=0x1c0;
//Push Uart control registers
gps_ULCON2=rULCON2;
gps_UCON2 =rUCON2;
gps_UFCON2=rUFCON2;
gps_UMCON2=rUMCON2;
gps_UBRDIV2=rUBRDIV2;
}
void GPS_Port_Return(void)
{
rGPHCON=gps_rGPHCON;
rGPHDAT=gps_rGPHDAT;
rGPHUP =gps_rGPHUP;
//Pop Uart control registers
rULCON2=gps_ULCON2;
rUCON2 =gps_UCON2;
rUFCON2=gps_UFCON2;
rUMCON2=gps_UMCON2;
rUBRDIV2=gps_UBRDIV2;
}
void __irq Uart2_RxIntOrErr(void)
{
rINTSUBMSK|=(BIT_SUB_RXD2|BIT_SUB_TXD2|BIT_SUB_ERR2);
if(rSUBSRCPND&BIT_SUB_RXD2) __sub_Uart2_RxInt();
else __sub_Uart2_RxErrInt();
ClearPending(BIT_UART2);
rSUBSRCPND=(BIT_SUB_RXD2|BIT_SUB_ERR2); // Clear Sub int pending
rINTSUBMSK&=~(BIT_SUB_RXD2|BIT_SUB_ERR2);
}
void __sub_Uart2_RxInt(void) //串口接收中断
{
U8 ch;
ch = RdURXH2();
if ((ch == '$') && (gps_flag == 0)) //如果收到字符'$',便开始接收
{
rev_start = 1;
rev_stop = 0;
}
if (rev_start == 1) //标志位为1,开始接收
{
rev_buf[num++] = ch; //字符存到数组中
if (ch == '\n') //如果接收到换行
{
rev_buf[num] = '\0';
rev_start = 0;
rev_stop = 1;
gps_flag = 1;
num = 0;
}
}
isRxInt = 0;
}
void __sub_Uart2_RxErrInt(void)
{
switch(rUERSTAT2)//to clear and check the status of register bits
{
case 1:
Uart_Printf("Overrun error\n");
break;
case 2:
Uart_Printf("Parity error\n");
break;
case 4:
Uart_Printf("Frame error\n");
break;
case 8:
Uart_Printf("Breake detect\n");
break;
default :
break;
}
isRxInt=0;
}
void Uart2_Init(int pclk, int baud)
{
if(pclk == 0)
pclk = PCLK;
rUFCON2=0x0;
rUMCON2=0x0;
rULCON2=0x3;
// rUCON2=0x245;
rUCON2 |= (TX_INTTYPE<<9)|(RX_INTTYPE<<8)|(0<<7)|(0<<6)|(0<<5)|(0<<4)|(1<<2)|(1);
rUBRDIV2=((int)(pclk/(baud*16))-1);
pISR_UART2 =(unsigned)Uart2_RxIntOrErr;
ClearPending(BIT_UART2);
rINTMSK=~(BIT_UART2);
rSUBSRCPND=(BIT_SUB_RXD2|BIT_SUB_ERR2);
rINTSUBMSK=~(BIT_SUB_RXD2|BIT_SUB_ERR2);
// rSUBSRCPND=(BIT_SUB_RXD2);
// rINTSUBMSK=~(BIT_SUB_RXD2);
}
void GPS_Init(void)
{
GPS_Port_Set();
rGPFCON = (rGPFCON | 0xFFFF) & 0xFFFFFF55; //GPF0--GPF3设置为output
rGPFUP = rGPFUP & 0xFFF0; //使能GPF上拉电阻
rGPFDAT = 0x0F; //GPF低4位初始化为1
// Uart_Printf("baud=9600\n");
Uart_Printf("[GPS For Arm 测试程序]\n");
Uart_Printf("GPS模块初化中,正在搜索定位卫星.....\n");
Uart2_Init(0, 9600);
Delay(200);
if (GPS_GSV_Parse(rev_buf))
{
Uart_Printf("可用卫星数量: %2d\r", GPS->satellite);
}
}
//====================================================================//
// 语法格式:int GPS_RMC_Parse(char *line, GPS_INFO *GPS)
// 实现功能:把gps模块的GPRMC信息解析为可识别的数据
// 参 数:存放原始信息字符数组、存储可识别数据的结构体
// 返 回 值:
// 1: 解析GPRMC完毕
// 0: 没有进行解析,或数据无效
//====================================================================//
int GPS_RMC_Parse(char *line)
{
U8 ch, status, tmp;
float lati_cent_tmp, lati_second_tmp;
float long_cent_tmp, long_second_tmp;
float speed_tmp;
char *buf = line;
ch = buf[5];
status = buf[GetComma(2, buf)];
if (ch == 'C') //如果第五个字符是C,($GPRMC)
{
if (status == 'A') //如果数据有效,则分析
{
GPS -> NS = buf[GetComma(4, buf)];
GPS -> EW = buf[GetComma(6, buf)];
GPS->latitude = Get_Double_Number(&buf[GetComma(3, buf)]);
GPS->longitude = Get_Double_Number(&buf[GetComma( 5, buf)]);
GPS->latitude_Degree = (int)GPS->latitude / 100; //分离纬度
lati_cent_tmp = (GPS->latitude - GPS->latitude_Degree * 100);
GPS->latitude_Cent = (int)lati_cent_tmp;
lati_second_tmp = (lati_cent_tmp - GPS->latitude_Cent) * 60;
GPS->latitude_Second = (int)lati_second_tmp;
GPS->longitude_Degree = (int)GPS->longitude / 100; //分离经度
long_cent_tmp = (GPS->longitude - GPS->longitude_Degree * 100);
GPS->longitude_Cent = (int)long_cent_tmp;
long_second_tmp = (long_cent_tmp - GPS->longitude_Cent) * 60;
GPS->longitude_Second = (int)long_second_tmp;
speed_tmp = Get_Float_Number(&buf[GetComma(7, buf)]); //速度(单位:海里/时)
GPS->speed = speed_tmp * 1.85; //1海里=1.85公里
GPS->direction = Get_Float_Number(&buf[GetComma(8, buf)]); //角度
GPS->D.hour = (buf[7] - '0') * 10 + (buf[8] - '0'); //时间
GPS->D.minute = (buf[9] - '0') * 10 + (buf[10] - '0');
GPS->D.second = (buf[11] - '0') * 10 + (buf[12] - '0');
tmp = GetComma(9, buf);
GPS->D.day = (buf[tmp + 0] - '0') * 10 + (buf[tmp + 1] - '0'); //日期
GPS->D.month = (buf[tmp + 2] - '0') * 10 + (buf[tmp + 3] - '0');
GPS->D.year = (buf[tmp + 4] - '0') * 10 + (buf[tmp + 5] - '0')+2000;
UTC2BTC(&GPS->D);
return 1;
}
}
return 0;
}
//====================================================================//
// 语法格式:int GPS_GGA_Parse(char *line, GPS_INFO *GPS)
// 实现功能:把gps模块的GPGGA信息解析为可识别的数据
// 参 数:存放原始信息字符数组、存储可识别数据的结构体
// 返 回 值:
// 1: 解析GPGGA完毕
// 0: 没有进行解析,或数据无效
//====================================================================//
int GPS_GGA_Parse(char *line)
{
U8 ch, status;
char *buf = line;
ch = buf[4];
status = buf[GetComma(2, buf)];
if (ch == 'G') //$GPGGA
{
if (status != ',')
{
GPS->height_sea = Get_Float_Number(&buf[GetComma(9, buf)]);
GPS->height_ground = Get_Float_Number(&buf[GetComma(11, buf)]);
return 1;
}
}
return 0;
}
//====================================================================//
// 语法格式:int GPS_GSV_Parse(char *line, GPS_INFO *GPS)
// 实现功能:把gps模块的GPGSV信息解析为可识别的数据
// 参 数:存放原始信息字符数组、存储可识别数据的结构体
// 返 回 值:
// 1: 解析GPGGA完毕
// 0: 没有�
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
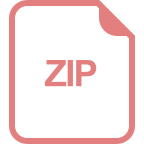
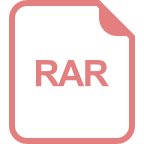
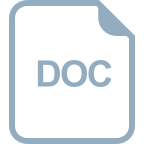
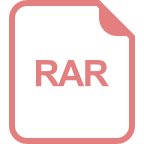
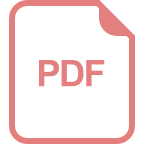
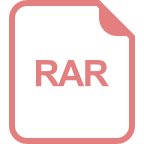
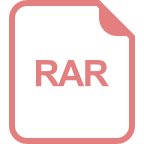
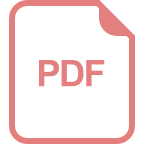
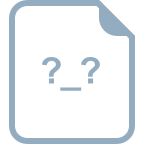
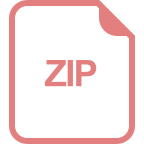
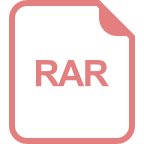
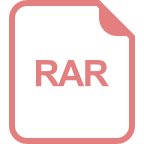
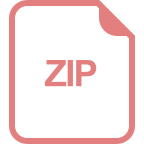
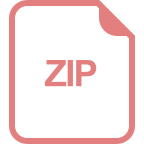
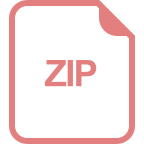
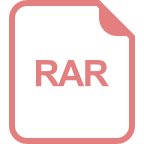
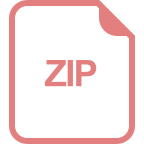
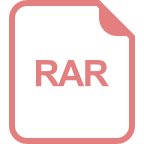
收起资源包目录


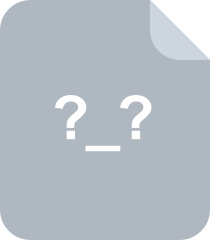
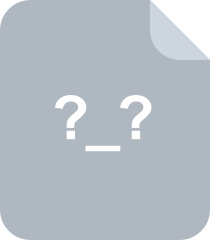
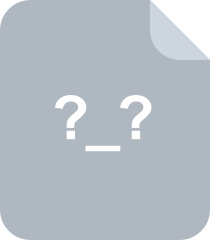
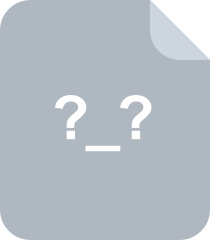
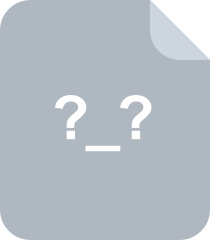
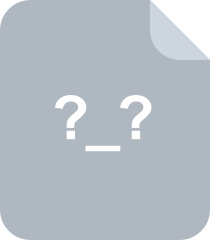
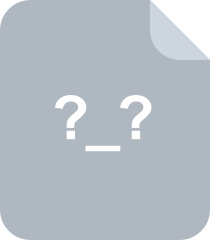
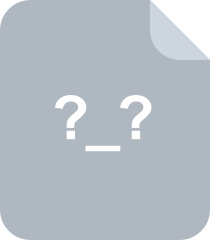
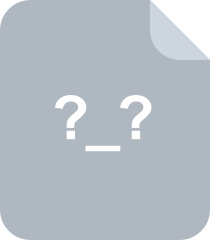
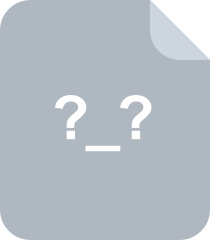
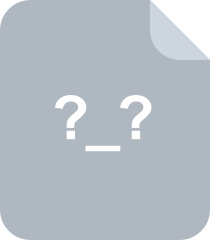

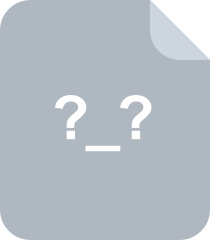


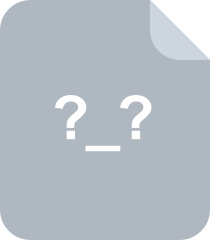
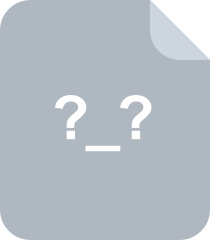
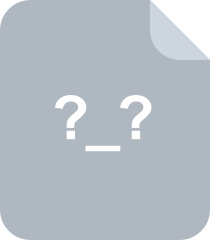
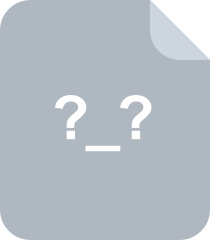
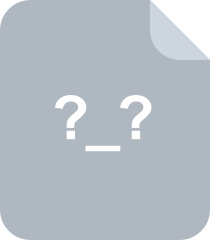
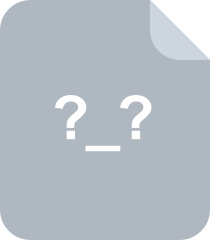
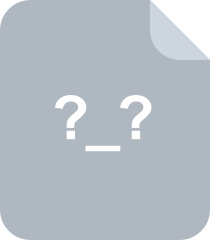
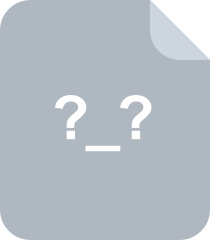
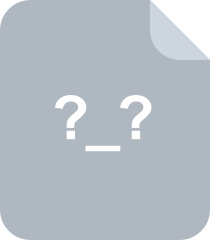

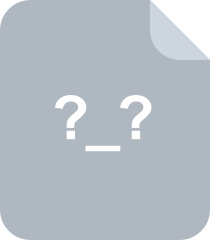
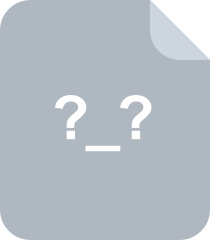
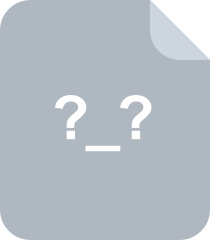
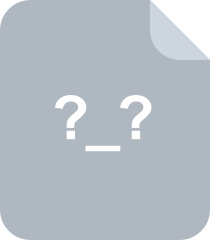
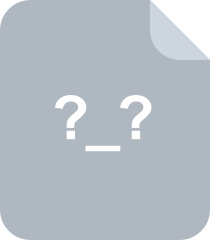
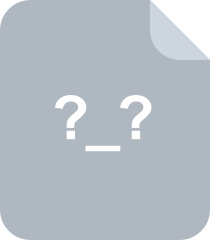

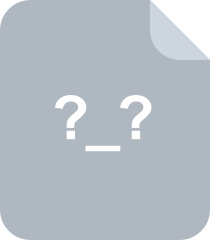
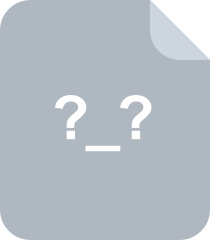
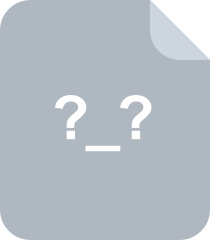
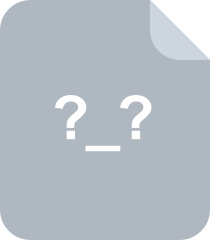


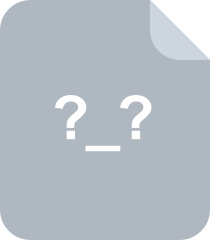

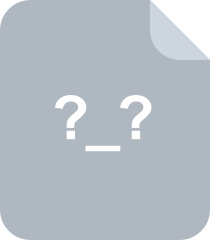
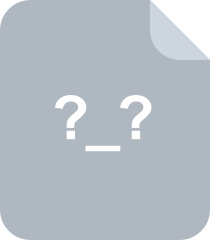
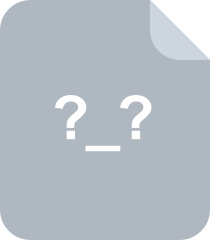
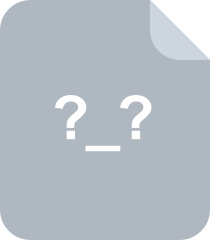
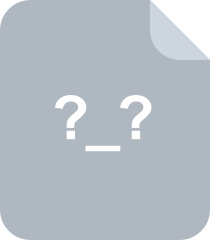
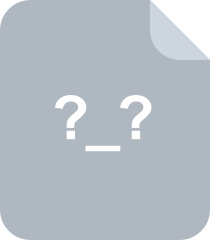
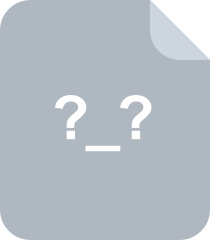
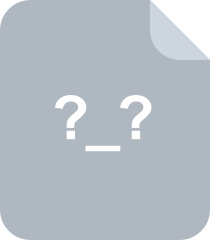
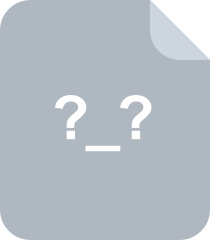
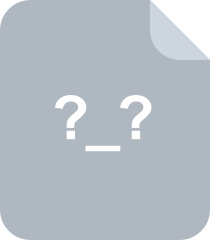

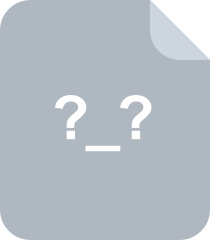

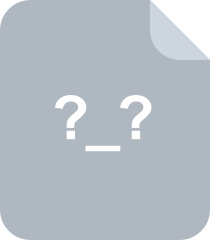
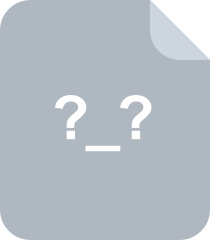
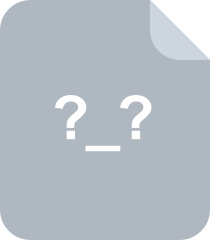
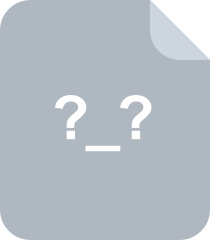
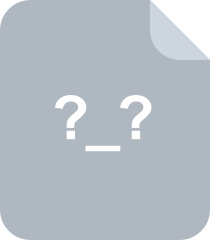
共 48 条
- 1
资源评论
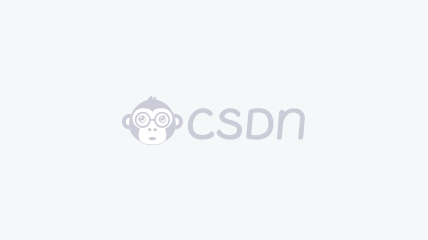
- 程行天下2020-10-26怎么是C写的啊,大兄弟啊要注明语言啊
- ba_wang_mao2022-09-28一般般啦,只解析了GPRMC报文

搬砖小王子
- 粉丝: 38
- 资源: 37
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

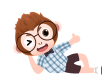
安全验证
文档复制为VIP权益,开通VIP直接复制
