在Java Web开发中,Servlet和JSP是两个关键的技术,常用于构建动态Web应用程序。本项目以"基于servlet和JSP的Java技术实现增删改查"为主题,旨在教你如何利用这两种技术来构建一个基本的数据操作应用。下面将详细阐述相关知识点。 1. **Servlet**:Servlet是Java编写的服务器端程序,主要功能是扩展服务器的功能,处理客户端的请求。在本项目中,Servlet用于接收HTTP请求,执行业务逻辑(增删改查操作),然后将结果返回给客户端。例如,你可以创建一个名为`CrudServlet`的类,里面包含处理GET和POST请求的方法,如`doGet()`和`doPost()`,分别对应用户的查询和提交操作。 2. **JSP(JavaServer Pages)**:JSP是HTML和Java代码的混合,主要用于生成动态网页内容。在本项目中,JSP页面主要负责展示数据并提供用户交互界面。例如,可以创建一个名为`crud.jsp`的页面,使用JSP的内置对象如`request`、`response`以及EL(Expression Language)和JSTL(JavaServer Pages Standard Tag Library)标签库来获取和显示数据。 3. **数据库连接与操作**:项目中提到需要在MySQL中创建相应的表,这表明你需要了解SQL语句来定义表结构,比如创建一个用户表可能包括`id`、`username`、`password`等字段。在Servlet中,你可以使用JDBC(Java Database Connectivity)来连接数据库,执行CRUD操作。例如,通过`Connection`对象执行`Statement`或`PreparedStatement`对象的`executeQuery()`和`executeUpdate()`方法。 4. **MVC(Model-View-Controller)模式**:虽然servlet和JSP没有强制要求使用MVC模式,但这个项目可以采用此模式进行设计。Model代表业务逻辑和数据,Servlet作为Controller处理请求并调用Model,JSP作为View负责渲染结果。 5. **请求转发与重定向**:在Servlet中,你可以使用`RequestDispatcher`的`forward()`方法进行请求转发,将请求传递给其他资源(如JSP)。而`response.sendRedirect()`方法用于重定向,使浏览器向新的URL发送请求。 6. **session和cookie管理**:如果项目涉及到用户登录,那么会用到session和cookie来跟踪用户状态。session在服务器端存储用户信息,cookie则在客户端保存,通常用于保持登录状态。 7. **异常处理**:在处理HTTP请求时,应考虑异常处理,比如使用try-catch-finally块来捕获和处理可能出现的异常,并向客户端返回适当的错误信息。 8. **安全性**:在实际开发中,应确保对输入数据进行验证,防止SQL注入攻击,并使用预编译的SQL语句(PreparedStatement)来提升安全性。 9. **测试与部署**:完成编码后,需要进行单元测试和集成测试以确保功能正确。项目部署时,需将应用打包成WAR文件,然后将其放入应用服务器(如Tomcat)的webapps目录下。 通过以上知识点的实践,你可以掌握Java Web开发的基本流程,为后续更复杂的应用开发打下坚实的基础。在这个项目中,你会了解到如何将用户在浏览器上的操作与后端数据库交互,从而实现数据的动态管理。
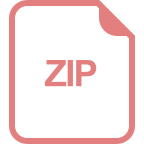
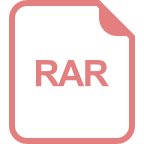
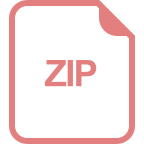
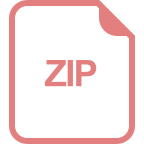
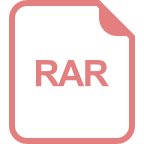
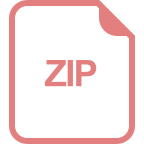
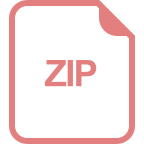
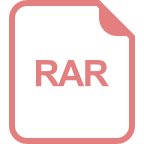
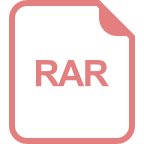
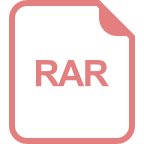
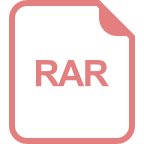
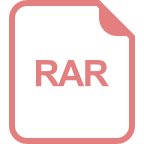
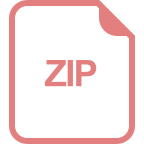


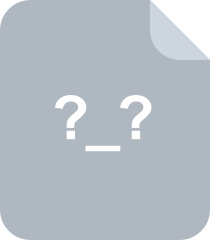

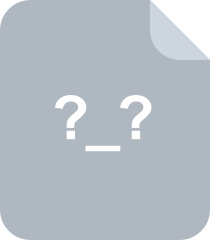
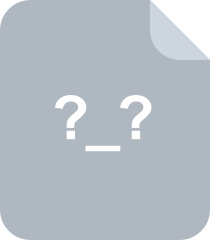
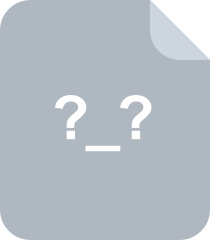
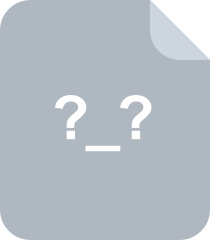
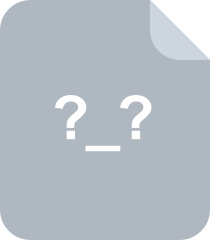
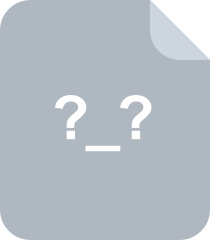


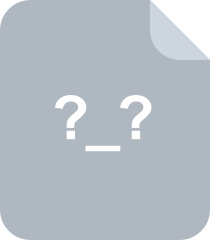

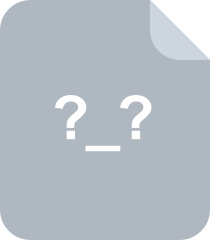

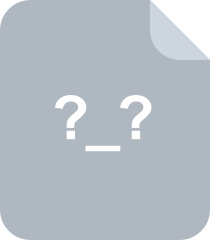
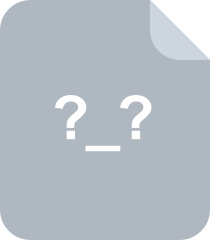
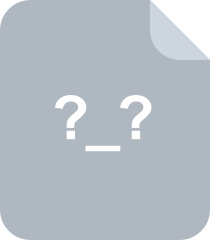
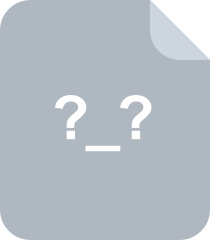
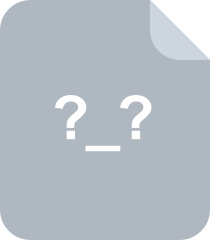

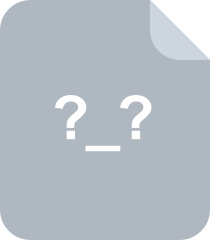



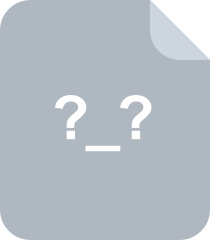

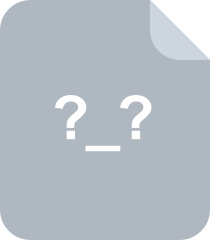

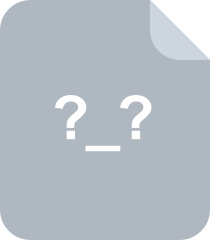
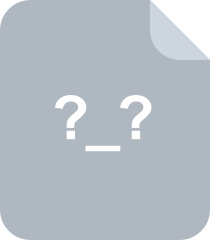
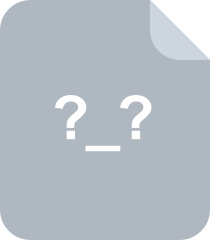
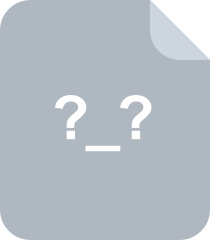
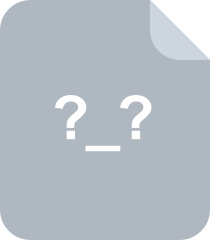

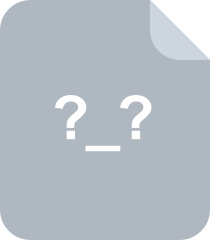


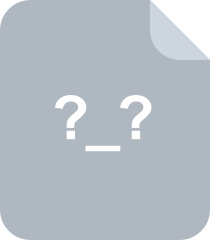

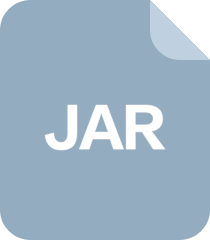
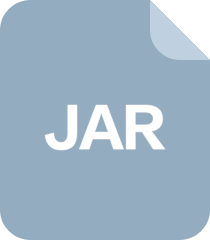
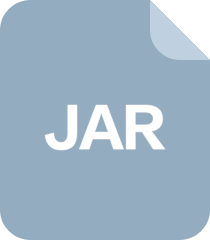
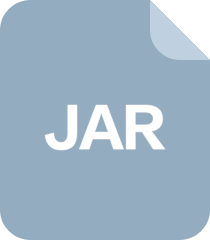
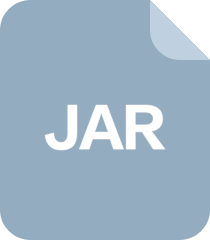
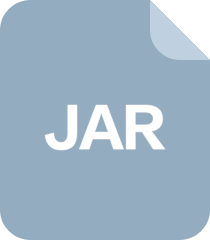
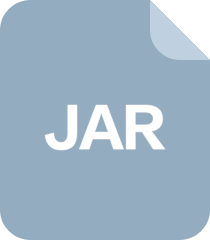
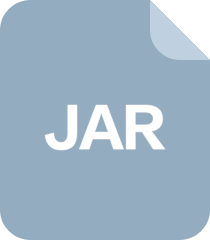
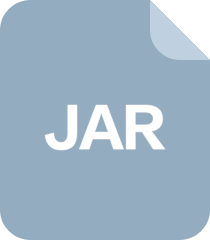
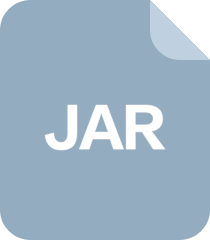

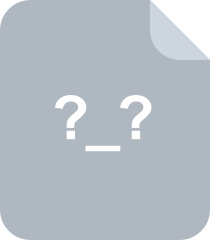
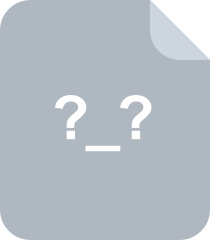
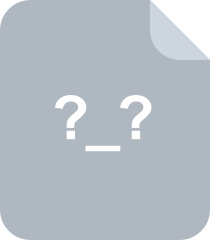
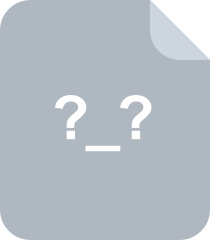
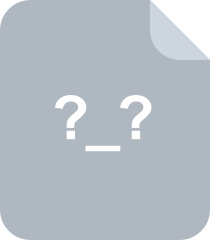
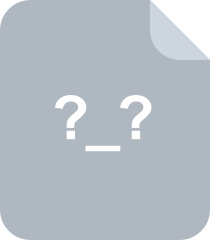
- 1
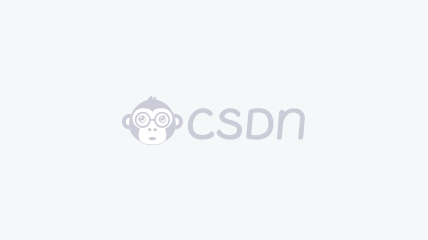

- 粉丝: 0
- 资源: 5
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

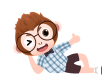
最新资源
- 基于Java和Vue的kopsoftKANBAN车间电子看板设计源码
- 影驰战将PS3111 东芝芯片TT18G23AIN开卡成功分享,图片里面画线的选项很重要
- 【C++初级程序设计·配套源码】第1期-语法基础
- 基于JavaScript、CSS、HTML的简易DOM版飞机游戏设计源码
- 基于Java开发的日程管理FlexTime应用设计源码
- SM2258XT-BGA144-4BGA180-6L-R1019 三星KLUCG4J1CB B0B1颗粒开盘工具 , EC, 3A, 94, 43, A4, CA 七彩虹SL300这个固件有用
- GJB 5236-2004 军用软件质量度量
- 30天开发操作系统 第 8 天 - 鼠标控制与切换32模式
- spice vd interface接口
- 安装Git时遇到找不到`/dev/null`的问题

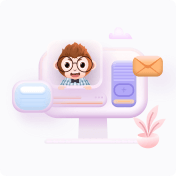
