package cn.lqgx.www;
import entity.Student;
import service.StudentService;
import service.impl.StudentServiceImpl;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.PrintWriter;
//注解方式替换web.xml中的配置
@WebServlet("/update")
public class StudentUpdate extends HttpServlet {
StudentService studentService=new StudentServiceImpl();
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
req.setCharacterEncoding("UTF-8");
resp.setCharacterEncoding("UTF-8");
int stuId= Integer.parseInt(req.getParameter("stuId"));
String stuName = req.getParameter("stuName");
int stuAge = Integer.parseInt(req.getParameter("stuAge"));
String stuSex=req.getParameter("stuSex");
String stuAddress=req.getParameter("stuAddress");
String stuPhone=req.getParameter("stuPhone");
PrintWriter printWriter=resp.getWriter();
Student student=new Student(stuId,stuName,stuAge,stuSex,stuAddress,stuPhone);
System.out.println(student);
Student student1=studentService.selectStudent(stuId,stuName);
if(student1!=null){
int num=studentService.updateStudent(student);
if(num>0){
// resp.getWriter().println("学生信息添加成功!");
resp.setContentType("text/html;charset=UTF-8");
printWriter.write("<html lang=\"en\">");
printWriter.write("<head>");
printWriter.write("<meta charset=\"UTF-8\">");
printWriter.write("<title>Title</title>");
printWriter.write("<style>");
printWriter.write("td{width:200px;height:45px;line-height:45px;text-align:center;}");
printWriter.write("table tr:nth-child(odd){background:green;}");
printWriter.write("table tr:nth-child(even){background:#FE5F97;}");
printWriter.write("th{height:40px;line-height:40px;text-align:center;background:white;}");
printWriter.write("caption{height:45px;line-height:45px;text-align:center;}");
printWriter.write("</style>");
printWriter.write("</head>");
printWriter.write("<body>");
printWriter.write("<table border=\"1\" width=\"100%\" cellspacing=\"0\">");
printWriter.write("<caption align=\"top\">修改后的学生信息</caption>");
printWriter.write("<thead>");
printWriter.write("<tr>");
printWriter.write("<th>学号</th>");
printWriter.write("<th>姓名</th>");
printWriter.write("<th>年龄</th>");
printWriter.write("<th>性别</th>");
printWriter.write("<th>地址</th>");
printWriter.write("<th>电话号码</th>");
printWriter.write("</tr>");
printWriter.write("</thead>");
printWriter.write("<tbody>");
printWriter.write("<tr>");
printWriter.write("<td>"+student.getSid()+"</td>");
printWriter.write("<td>"+student.getSname()+"</td>");
printWriter.write("<td>"+student.getSage()+"</td>");
printWriter.write("<td>"+student.getSsex()+"</td>");
printWriter.write("<td>"+student.getSaddress()+"</td>");
printWriter.write("<td>"+student.getSphoneNum()+"</td>");
printWriter.write("</tr>");
printWriter.write("</tbody>");
printWriter.write("</table>");
printWriter.write("</body>");
printWriter.write("</html>");
// resp.getWriter().println("【学生信息为:】"+student);
}else {
resp.getWriter().println("学生信息修改失败!");
}
}else{
resp.getWriter().println("学生信息不存在,不能修改!");
}
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doGet(req, resp);
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
Java+Servlet+HTML+CSS+数据库,实现的学生信息管理系统,实现了 新增和查询功能。 覆盖知识 java部分:程序基本概念、数据类型、流程控制、顺序、选择 、循环、跳转语句、变量、类、方法、实用类、JDBC、三层架构Druid连接池、Apache的DBUtils使用、Servlet等。 数据库部分:创建表、增删改查语句的书写等。 前端部分:HTML、CSS、jQuery、JavaScript等。 编码顺序 添加项目需要使用的各种jar包 HTML 页面表单的编写 Apache方法的DBUtils编写 实体类的数据初始化(添加私有变量、有参/无参构造、get/set方法、toString() 方法重写) 完成数据表创建 三层架构的搭建(dao层接口和实现类的编写、service层接口和实现类的编写、view层的Servlet类的编写)
资源推荐
资源详情
资源评论
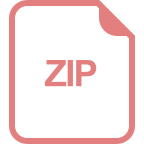
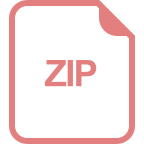
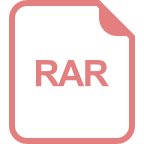
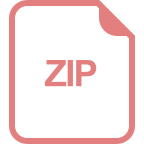
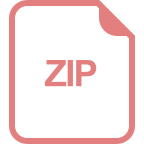
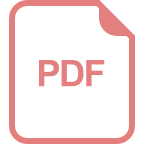
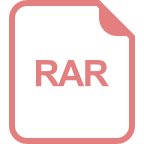
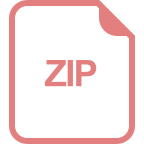
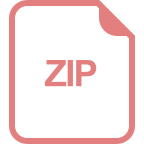
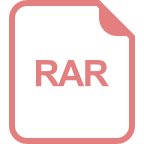
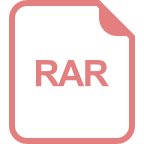
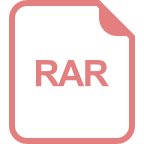
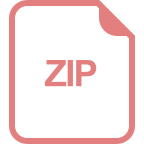
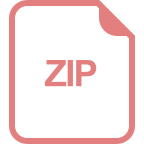
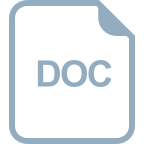
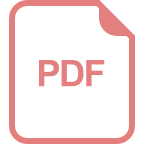
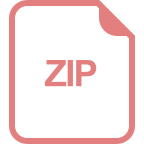
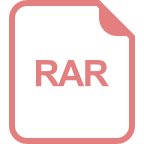
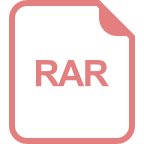
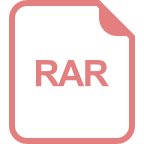
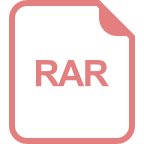
收起资源包目录




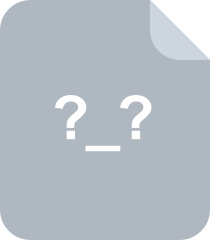

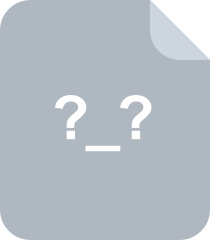

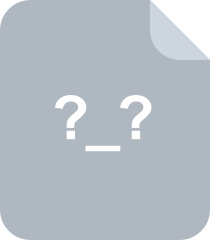



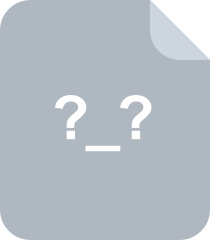
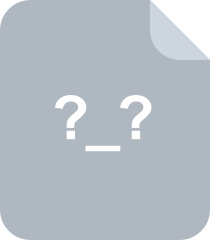
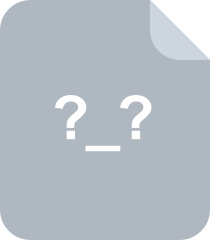
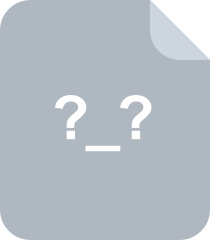
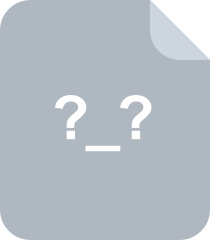


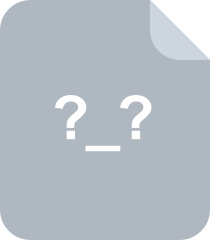
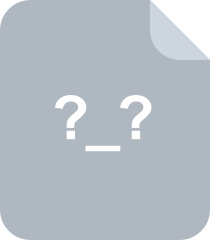

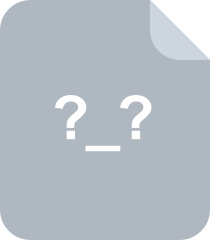

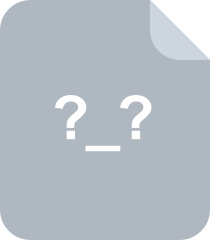
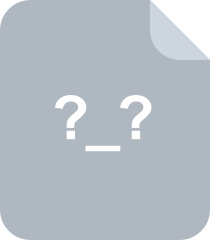
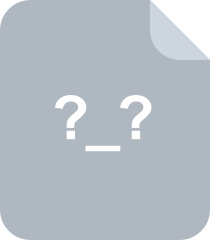

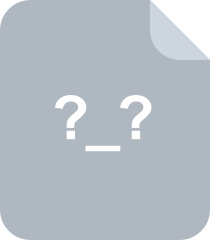
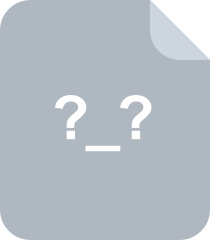
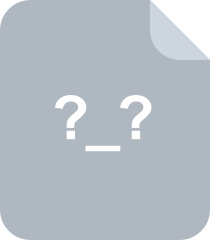
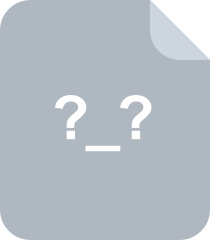
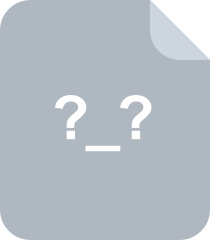

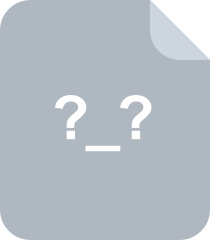
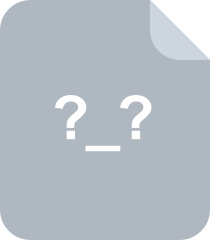
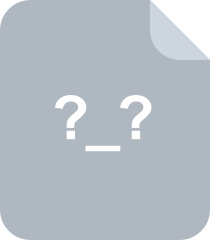
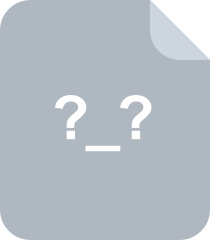
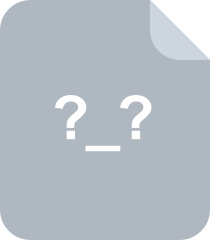
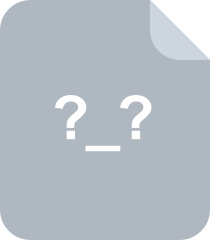
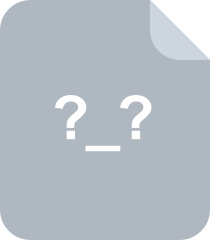
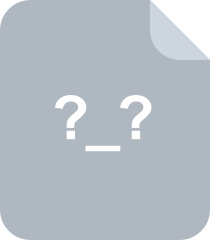

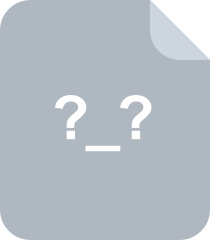



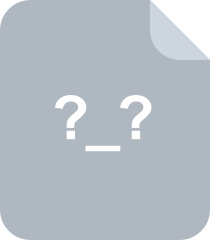

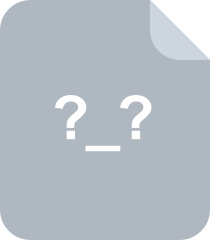

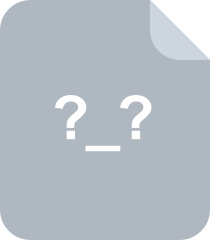



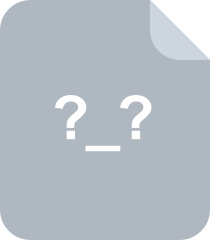
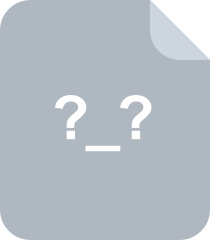
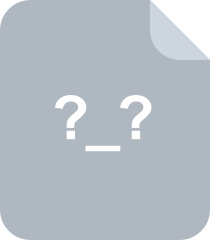
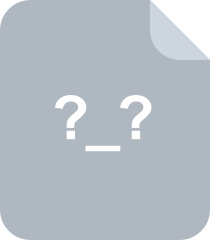
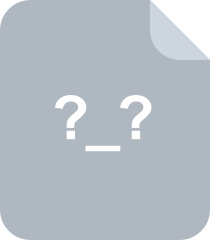
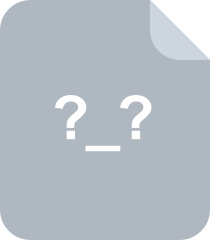

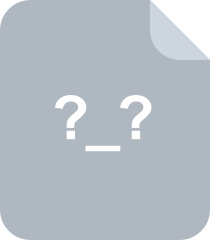

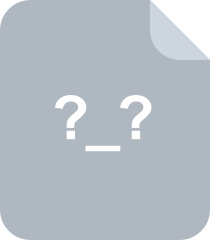

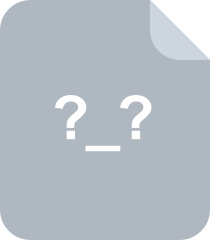

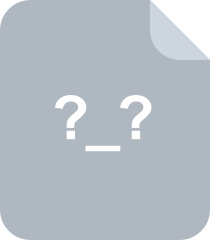
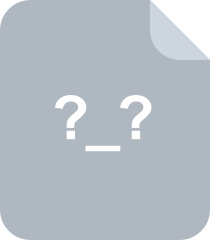



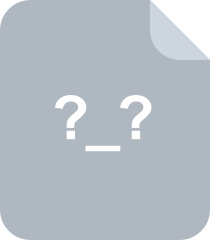
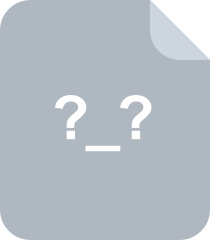
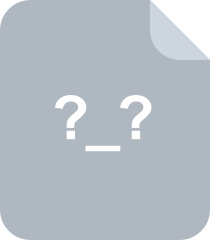
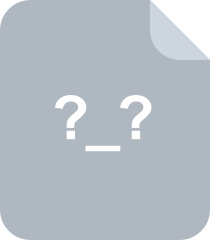
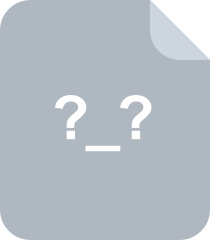

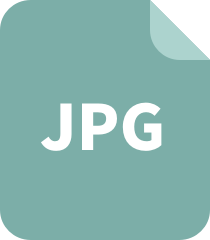
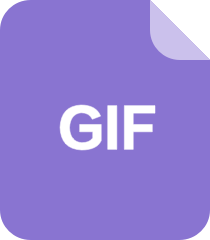
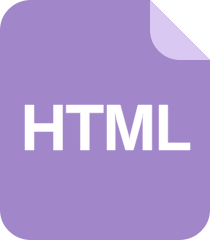
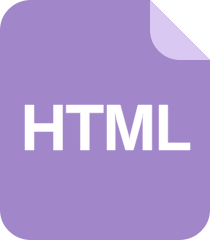
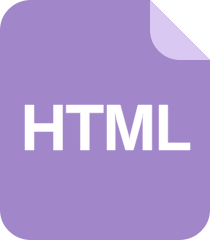

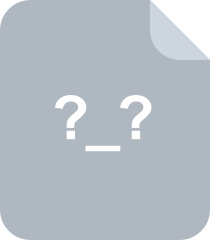

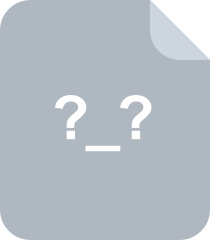

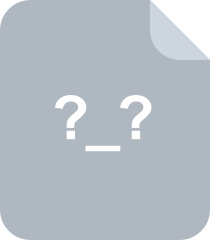

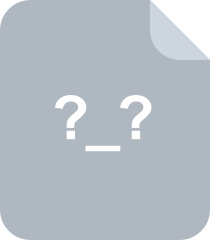



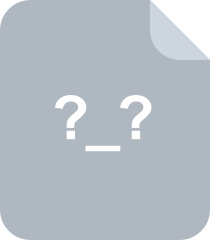
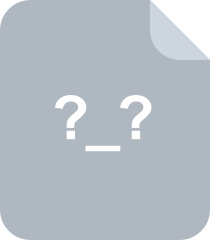
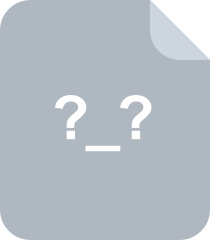
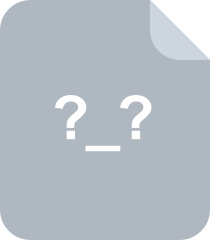
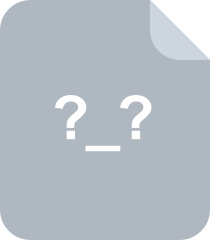
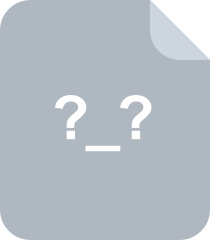

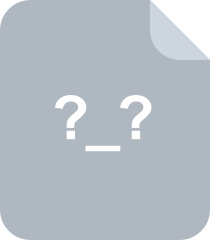

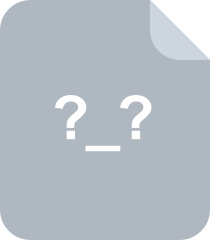

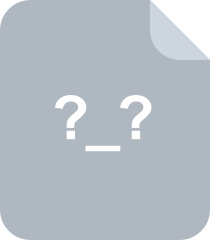

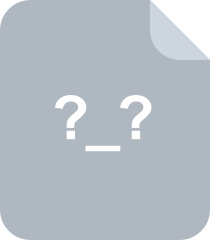
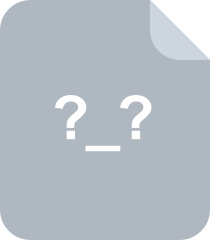

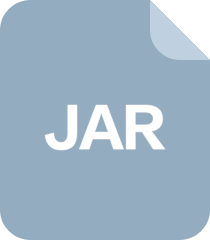
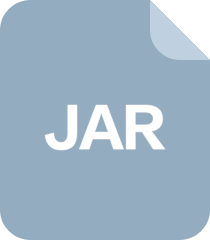
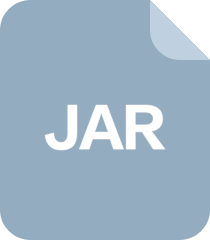
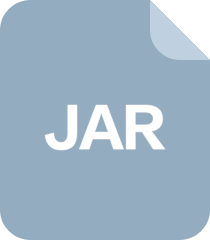
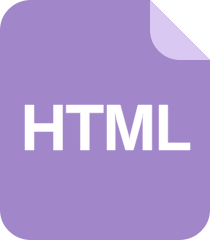

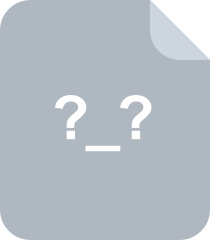
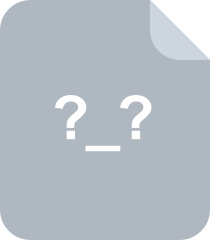
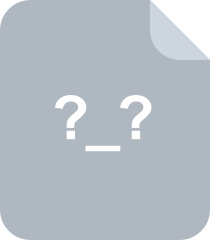
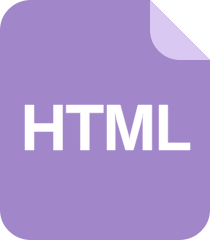


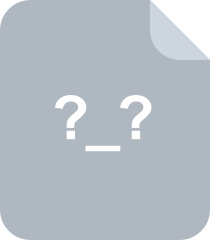
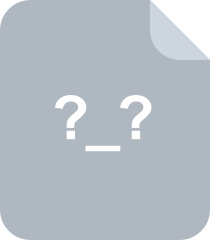
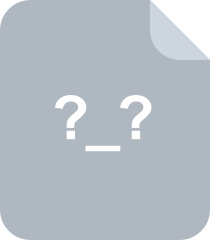
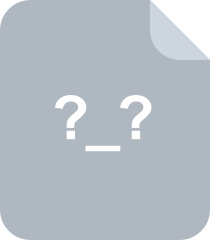
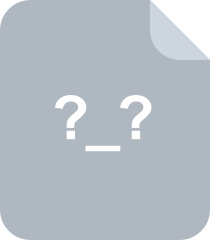

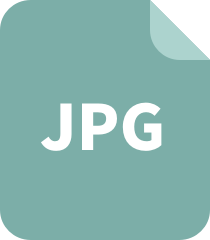
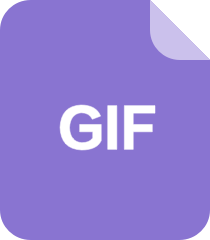
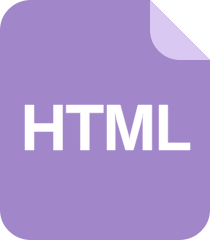
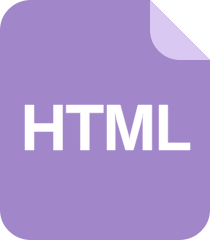
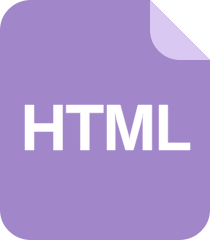

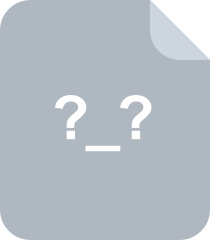

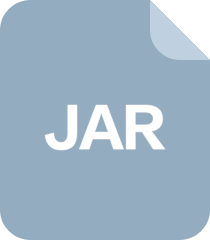
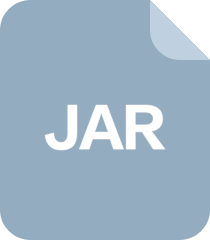
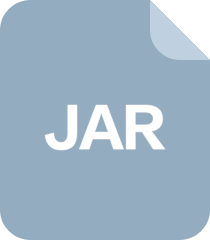
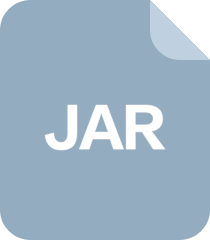
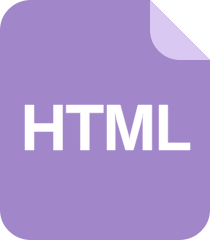

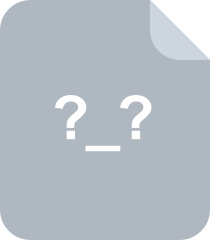
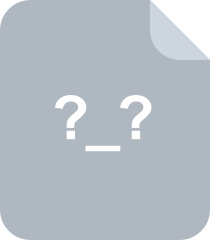
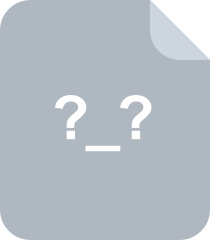
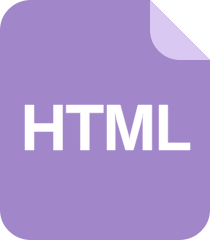
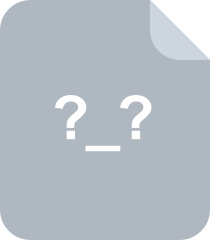
共 97 条
- 1
资源评论
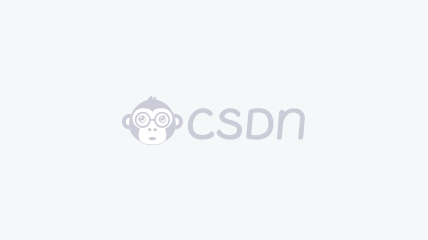
- 丶锅开了就进来啊2023-04-21大哥,我怎么运行不了呢,可以出来界面,但是输好信息后,页面跳转失败啊

JavaFans
- 粉丝: 5w+
- 资源: 31
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

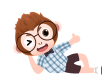
最新资源
- (源码)基于ESP8266和Arduino的HomeMatic水表读数系统.zip
- (源码)基于Django和OpenCV的智能车视频处理系统.zip
- (源码)基于ESP8266的WebDAV服务器与3D打印机管理系统.zip
- (源码)基于Nio实现的Mycat 2.0数据库代理系统.zip
- (源码)基于Java的高校学生就业管理系统.zip
- (源码)基于Spring Boot框架的博客系统.zip
- (源码)基于Spring Boot框架的博客管理系统.zip
- (源码)基于ESP8266和Blynk的IR设备控制系统.zip
- (源码)基于Java和JSP的校园论坛系统.zip
- (源码)基于ROS Kinetic框架的AGV激光雷达导航与SLAM系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


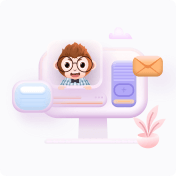
安全验证
文档复制为VIP权益,开通VIP直接复制
