package util;
import java.sql.*;
public class DBConn {
public static String driver;//定义驱动
public static String url;//定义URL
public static String user;//定义用户名
public static String password;//定义密码
public static Connection conn;//定义连接
public static Statement stmt;//定义STMT
public ResultSet rs;//定义结果集
//设置CONN
//连接第一种办法
public static Statement getStatment(){
try{
driver="com.microsoft.sqlserver.jdbc.SQLServerDriver";
url="jdbc:sqlserver://192.168.4.133:1433;databaseName=xc";
user="sa";
password="123.com123.com";
Class.forName(driver);
conn=DriverManager.getConnection(url, user, password);
System.out.println("-------连接成功------");
}catch(ClassNotFoundException e){
e.printStackTrace();
System.out.println("db: " + e.getMessage());
}catch(SQLException ex){
ex.printStackTrace();
System.err.println("db.getconn(): " + ex.getMessage());
}
return null;
}
//连接第二种办法
/*public static Statement getStatment(){
try{
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
conn=DriverManager.getConnection("jdbc:sqlserver://192.168.4.133:1433;databaseName=xc", "sa", "123.com123.com");
System.out.println("-------连接成功------");
}catch(ClassNotFoundException e){
e.printStackTrace();
System.out.println("db: " + e.getMessage());
}catch(SQLException ex){
ex.printStackTrace();
System.err.println("db.getconn(): " + ex.getMessage());
}
return null;
}*/
//构造函数,默认加裁配置文件为jdbc.driver
public DBConn(){
this.conn=this.getConn();
}
//返回Conn
public Connection getConn(){
return this.conn;
}
//执行插入
public void doInsert(String sql) {
try {
stmt = conn.createStatement();
int i = stmt.executeUpdate(sql);
} catch(SQLException sqlexception) {
System.err.println("db.executeInset:" + sqlexception.getMessage());
}finally{
}
}
//执行删除
public void doDelete(String sql) {
try {
stmt = conn.createStatement();
int i = stmt.executeUpdate(sql);
} catch(SQLException sqlexception) {
System.err.println("db.executeDelete:" + sqlexception.getMessage());
}
}
//执行更新
public void doUpdate(String sql) {
try {
stmt = conn.createStatement();
int i = stmt.executeUpdate(sql);
} catch(SQLException sqlexception) {
System.err.println("db.executeUpdate:" + sqlexception.getMessage());
}
}
//查询结果集
public ResultSet doSelect(String sql) {
try {
conn=DriverManager.getConnection(url,user,password);
stmt = conn.createStatement(java.sql.ResultSet.TYPE_SCROLL_INSENSITIVE,java.sql.ResultSet.CONCUR_READ_ONLY);
rs = stmt.executeQuery(sql);
System.out.println("取得结果集");
} catch(SQLException sqlexception) {
System.err.println("db.executeQuery: " + sqlexception.getMessage());
}
return rs;
}
/**
*关闭数据库结果集,数据库操作对象,数据库链接
@Function: Close all the statement and conn int this instance and close the parameter ResultSet
@Param: ResultSet
@Exception: SQLException,Exception
**/
public void close(ResultSet rs) throws SQLException, Exception {
if (rs != null) {
rs.close();
rs = null;
}
if (stmt != null) {
stmt.close();
stmt = null;
}
if (conn != null) {
conn.close();
conn = null;
}
}
/**
*关闭数据库操作对象,数据库连接对象
* Close all the statement and conn int this instance
* @throws SQLException
* @throws Exception
*/
public void close() throws SQLException, Exception {
if (stmt != null) {
stmt.close();
stmt = null;
}
if (conn != null) {
conn.close();
conn = null;
}
}
//测试类
/*public static void main(String []args){
DBConn db=new DBConn();
db.getConn();
ResultSet rs=db.doSelect("select * from db_user where userName='admin'");
try {
while(rs.next()){
System.out.println(rs.getInt(1));
System.out.println(rs.getString(3));
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
} */
}
没有合适的资源?快使用搜索试试~ 我知道了~
Jsp+Servlet+JavaBean+JDBC简单登录实例
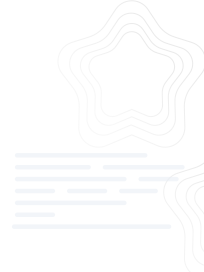
共23个文件
class:4个
java:4个
jsp:3个


温馨提示
基于Jsp+Servlet+JavaBean+JDBC实现登录功能,简单实例
资源推荐
资源详情
资源评论
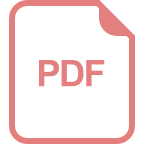
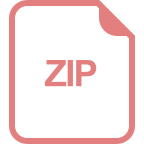
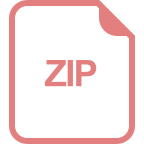
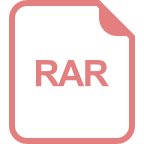
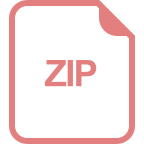
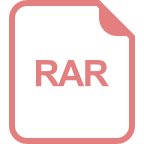
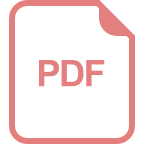
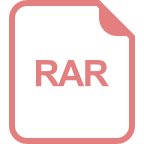
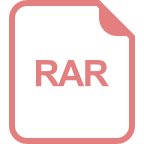
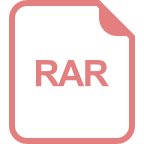
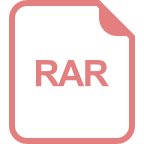
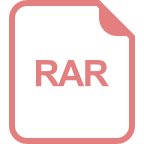
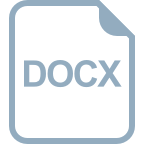
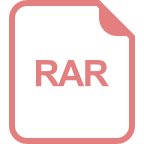
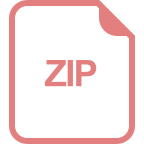
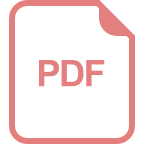
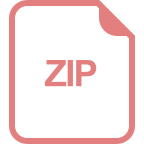
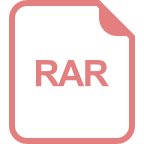
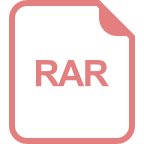
收起资源包目录


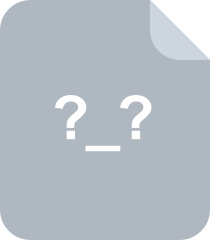


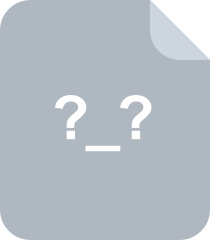

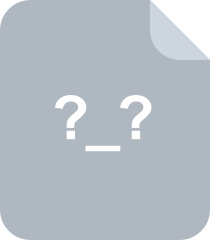

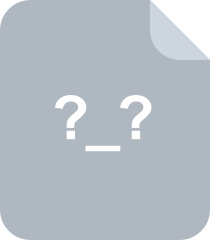

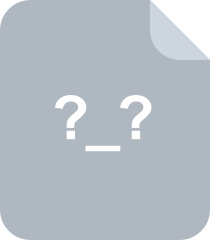



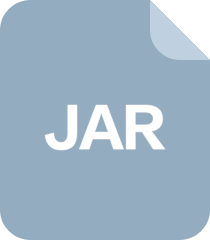
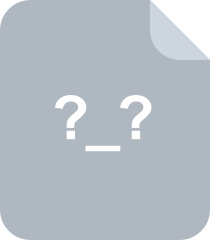
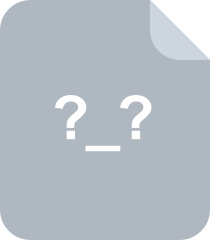
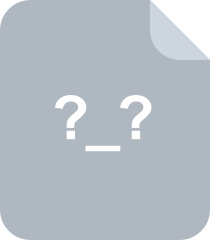

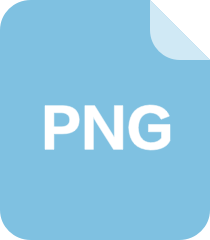

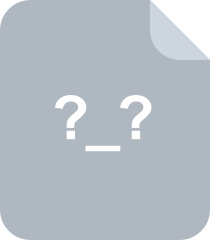
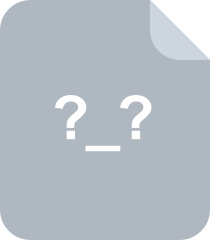

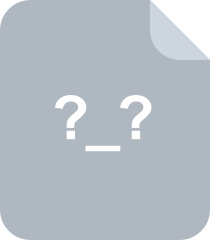
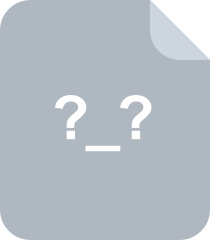
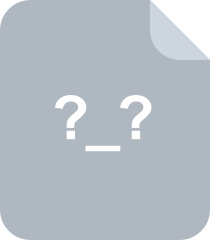
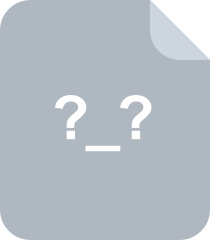
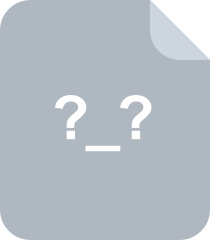
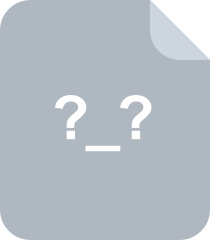



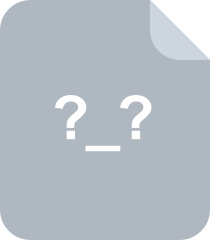

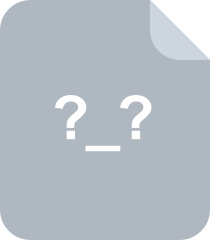

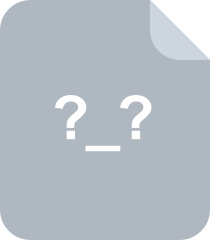

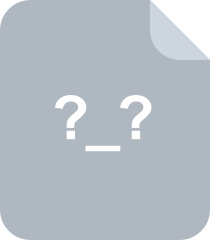
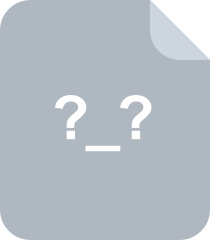
共 23 条
- 1

quwei1991
- 粉丝: 1
- 资源: 9
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

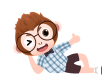
最新资源
- yolov5,SSD 可能使用到的一些代码
- 基于c51单片机+DS1302+DHT11温湿度模块+LCD1602显示的万年历硬件原理图+BOM+软件程源码序+仿真图.zip
- NSGA2的MATLAB代码
- Messagepassingtest_GCN_DGL.py
- Sh,Docker 运维好帮手,一招通过 sh 脚本批量快速启动和重启多个Docker 容器
- PCF2123.pdf
- 打开注册表操作.doc
- Windows 常见运行运行库32+64
- WMJUL8iC.html
- 基于3KW光伏并网单相逆变器设计(TMS320F28035控制板+显示板+STM32F103功率板)硬件(原理图+PCB)工程
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


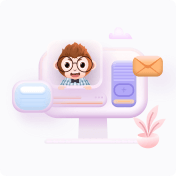
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
前往页