package com.quicklyteam.easybuy.dao.impl;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import com.quicklyteam.easybuy.dao.BaseDao;
import com.quicklyteam.easybuy.dao.ProductDao;
import com.quicklyteam.easybuy.entity.Product;
/**
* 商品数据访问层实现类
* @author Yzw
* @version 2013-5-10
*/
public class ProductDaoImpl extends BaseDao implements ProductDao {
/**
* 通过商品状态获取部分商品
*/
public List<Product> getProductsByStatus(int status, int number) {
// TODO Auto-generated method stub
Connection conn = getConnection();
PreparedStatement ps = null;
ResultSet rs = null;
List<Product> productList = new ArrayList<Product>();
String sql = "select * from easybuy_product where ep_status = ? and rownum <= ?";
try {
ps = conn.prepareStatement(sql);
ps.setInt(1, status);
ps.setInt(2, number);
rs = ps.executeQuery();
while (rs.next()) {
Product product = new Product(rs.getInt("ep_id"), rs.getString("ep_name"), rs.getString("ep_description"),
rs.getDouble("ep_price"), rs.getInt("ep_stock"), rs.getInt("ep_status"), rs.getInt("epc_id"),
rs.getInt("epc_child_id"), rs.getString("ep_file_name"), 1);
productList.add(product);
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
closeAll(conn, ps, rs);
}
return productList;
}
/**
* 通过类别获取商品,并限制数据范围
*/
public List<Product> getProductsByCategory(int id, int level, int pageSize, int pageIndex) {
// TODO Auto-generated method stub
Connection conn = getConnection();
PreparedStatement ps = null;
ResultSet rs = null;
List<Product> productList = new ArrayList<Product>();
String sql = "select *\n" + " from (select rownum r, t.*\n"
+ " from (select * from easybuy_product where epc_id = ? and ep_status != -1) t\n"
+ " where rownum <= ? * ?)\n" + " where r > (? - 1) * ?";
if (level == 2) {
sql = "select *\n" + " from (select rownum r, t.*\n"
+ " from (select * from easybuy_product where epc_child_id = ? and ep_status != -1) t\n"
+ " where rownum <= ? * ?)\n" + " where r > (? - 1) * ?";
}
if (id == -1 && level == -1) {
sql = "select *\n" + " from (select rownum r, t.*\n"
+ " from (select * from easybuy_product where ep_status != -1) t\n" + " where rownum <= ? * ?)\n"
+ " where r > (? - 1) * ?";
}
try {
ps = conn.prepareStatement(sql);
if (id == -1 && level == -1) {
ps.setInt(1, pageIndex);
ps.setInt(2, pageSize);
ps.setInt(3, pageIndex);
ps.setInt(4, pageSize);
} else {
ps.setInt(1, id);
ps.setInt(2, pageIndex);
ps.setInt(3, pageSize);
ps.setInt(4, pageIndex);
ps.setInt(5, pageSize);
}
rs = ps.executeQuery();
while (rs.next()) {
Product product = new Product(rs.getInt("ep_id"), rs.getString("ep_name"), rs.getString("ep_description"),
rs.getDouble("ep_price"), rs.getInt("ep_stock"), rs.getInt("ep_status"), rs.getInt("epc_id"),
rs.getInt("epc_child_id"), rs.getString("ep_file_name"), 1);
productList.add(product);
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
closeAll(conn, ps, rs);
}
return productList;
}
/**
* 获取指定商品类别ID的商品数量
*/
public int getCountByCategory(int id, int level) {
// TODO Auto-generated method stub
Connection conn = getConnection();
PreparedStatement ps = null;
ResultSet rs = null;
String sql = "select count(*) from easybuy_product where epc_id = ? and ep_status != -1";
if (level == 2) {
sql = "select count(*) from easybuy_product where epc_child_id = ? and ep_status != -1";
}
if (id == -1 && level == -1) {
sql = "select count(*) from easybuy_product where ep_status != -1";
}
try {
ps = conn.prepareStatement(sql);
if (id != -1 && level != -1) {
ps.setInt(1, id);
}
rs = ps.executeQuery();
if (rs.next()) {
return rs.getInt(1);
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
closeAll(conn, ps, rs);
}
return 0;
}
/**
* 获取可以显示的总页数
*/
public int getTotalPages(int count, int pageSize) {
// TODO Auto-generated method stub
return count % pageSize == 0 ? count / pageSize : count / pageSize + 1;
}
/**
* 通过ID获取商品信息
*/
public Product getProductById(int id) {
// TODO Auto-generated method stub
Connection conn = getConnection();
PreparedStatement ps = null;
ResultSet rs = null;
String sql = "select * from easybuy_product where ep_id = ?";
try {
ps = conn.prepareStatement(sql);
ps.setInt(1, id);
rs = ps.executeQuery();
if (rs.next()) {
Product product = new Product(rs.getInt("ep_id"), rs.getString("ep_name"), rs.getString("ep_description"),
rs.getDouble("ep_price"), rs.getInt("ep_stock"), rs.getInt("ep_status"), rs.getInt("epc_id"),
rs.getInt("epc_child_id"), rs.getString("ep_file_name"), 1);
return product;
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
closeAll(conn, ps, rs);
}
return null;
}
/**
* 通过ID删除商品
*/
public int deleteById(int id, int columnType) {
// TODO Auto-generated method stub
Connection conn = getConnection();
PreparedStatement ps = null;
String sql = "delete easybuy_product where";
if (columnType == 1) {
sql += " ep_id = ?";
} else if (columnType == 2) {
sql += " epc_id = ?";
} else if (columnType == 3) {
sql += " epc_child_id = ?";
} else {
return 0;
}
try {
ps = conn.prepareStatement(sql);
ps.setInt(1, id);
return ps.executeUpdate();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
closeAll(conn, ps, null);
}
return 0;
}
/**
* 添加一条商品
*/
public int insert(Product product) {
// TODO Auto-generated method stub
Connection conn = getConnection();
PreparedStatement ps = null;
String sql = "insert into easybuy_product values(easybuy_product_seq.nextval,?,?,?,?,?,?,?,?)";
try {
ps = conn.prepareStatement(sql);
ps.setString(1, product.getName());
ps.setString(2, product.getDescription());
ps.setDouble(3, product.getPrice());
ps.setInt(4, product.getStock());
ps.setInt(5, product.getStatus());
ps.setInt(6, product.getEpcId());
ps.setInt(7, product.getEpcChildId());
ps.setString(8, product.getFileName());
return ps.executeUpdate();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
closeAll(conn, ps, null);
}
return 0;
}
/**
* 更新一条商品
*/
public int update(Product product) {
// TODO Auto-generated method stub
Connection conn = getConnection();
PreparedStatement ps = null;
String sql = "update easybuy_product set ep_name=?,ep_description=?,ep_price=?,ep_stock=?,ep_status=?,epc_id=?,epc_child_id=?,ep_file_name=? where ep_id=?";
try {
ps = conn.prepareStatement(sql);
ps.setString(1, product.getName());
ps.setString(2, product.getDescription());
ps.setDouble(3, product.getPrice());
ps.setInt(4, product.getStock());
ps.setInt(5, product.getStatus());
ps.setInt(6, product.getEpcId());
ps.setInt(7, product.getEpcChildId());
ps.setString(8, product.getFileName());
ps.setInt(9, product.getId());
return ps.executeUpdate();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
closeAll(conn, ps, null);
}
return 0;
}
/**
* 禁
没有合适的资源?快使用搜索试试~ 我知道了~
easybuy项目一个jsp+servlet+javabean的一个实例
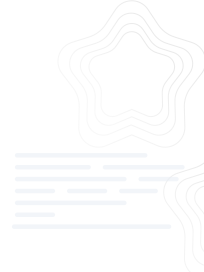
共288个文件
jpg:81个
java:75个
class:75个


温馨提示
使用java做的一个网上商城的开源项目-易买网。该项目使用jsp+servlet+javabean实现。其中用到了ajax技术。文件中包括了源代码和建表语句,数据库用的是oracle,源代码中没有上传所需的jar包,jar包需要jdbc驱动包和smartupload的jar包,可以自己到网上进行下载。该例子共学习jsp技术使用,不适合做开源的项目。
资源推荐
资源详情
资源评论
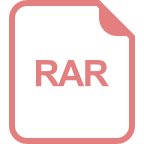
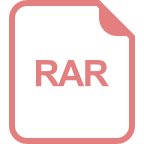
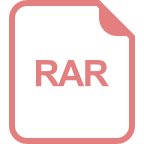
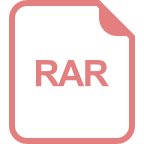
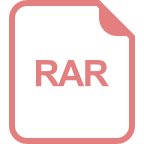
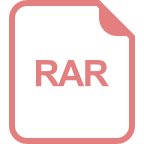
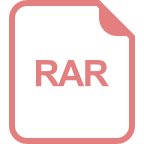
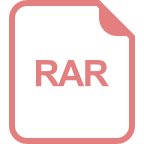
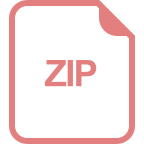
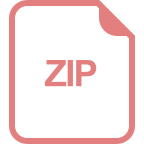
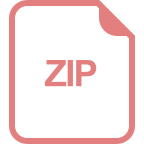
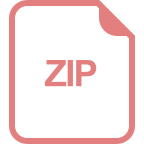
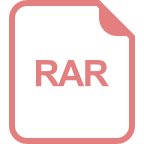
收起资源包目录

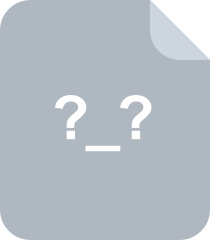
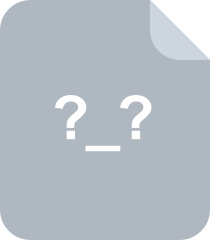
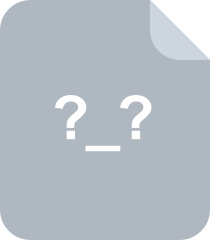
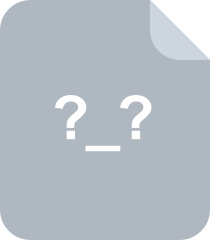
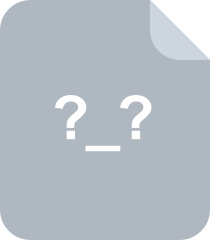
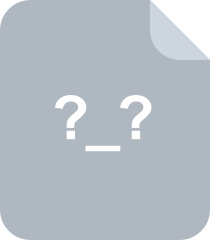
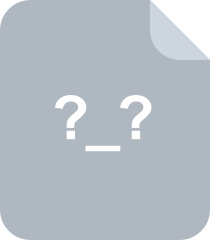
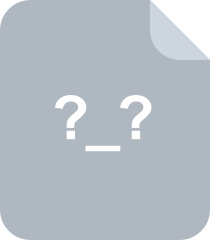
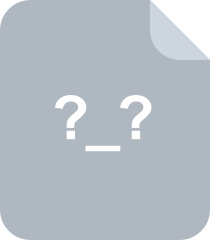
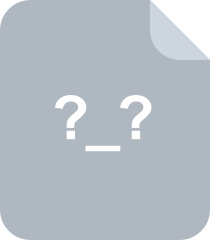
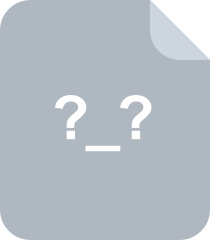
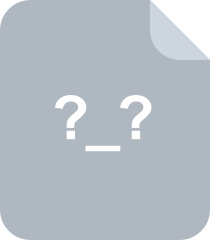
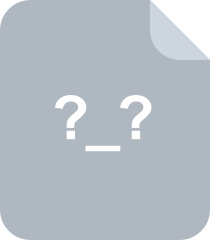
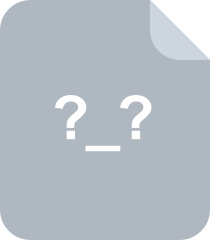
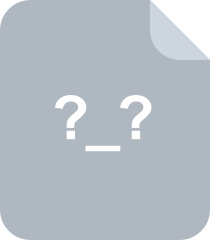
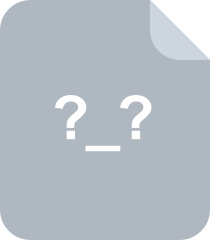
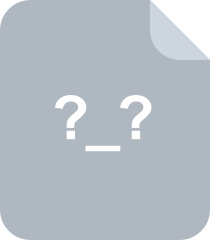
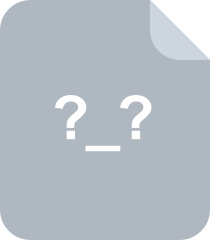
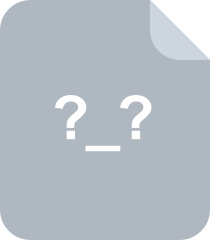
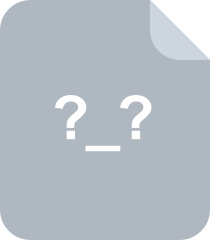
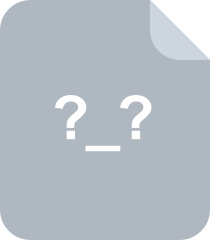
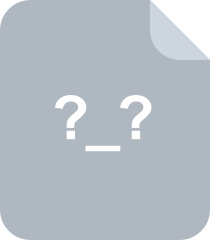
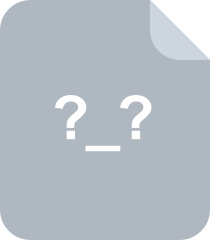
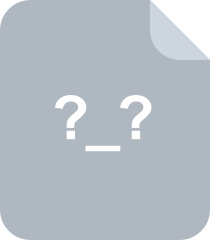
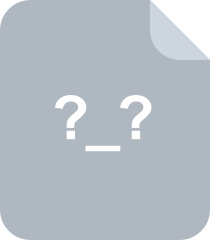
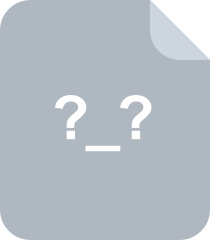
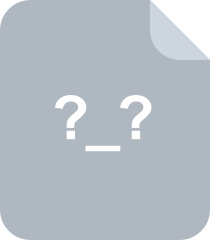
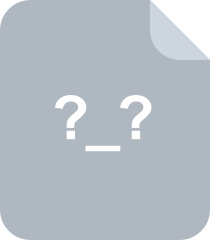
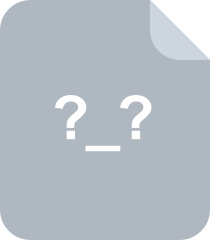
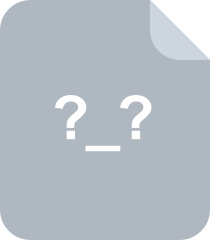
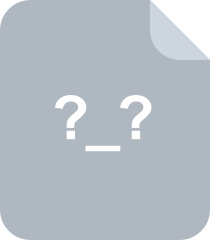
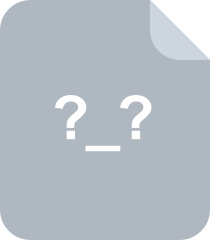
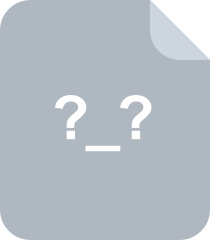
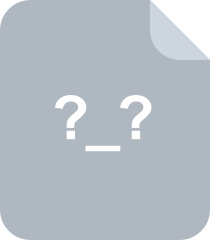
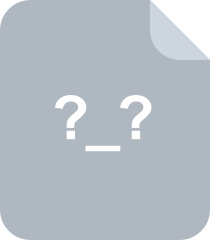
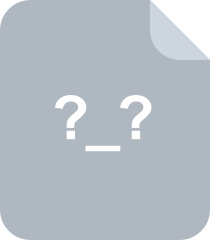
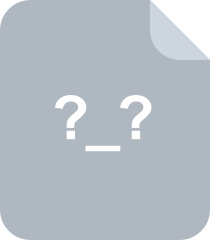
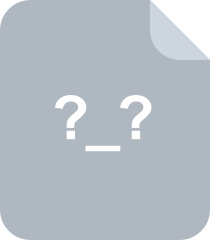
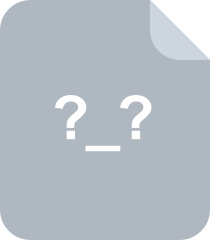
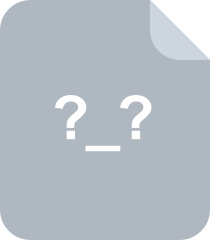
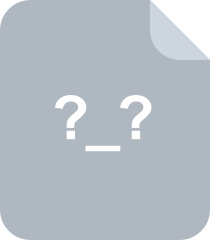
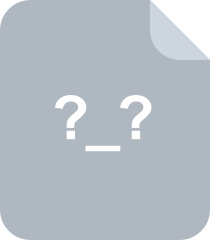
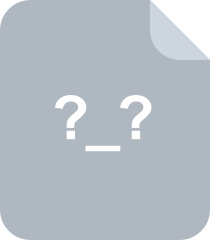
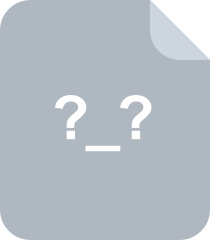
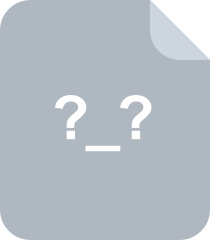
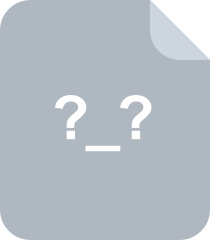
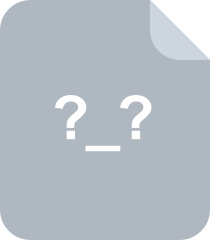
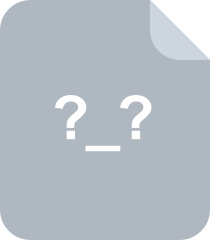
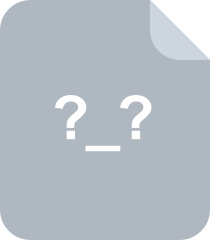
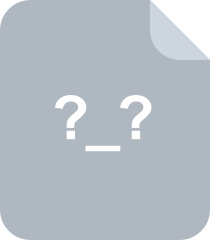
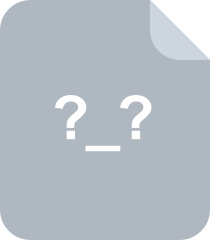
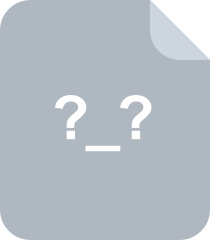
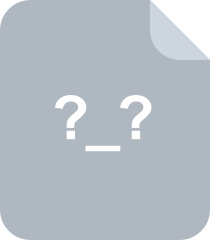
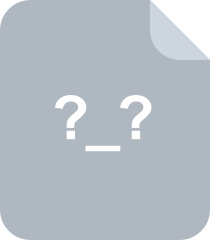
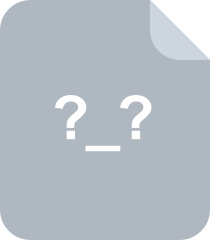
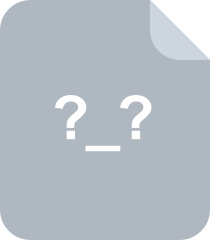
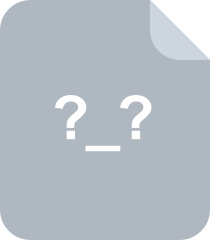
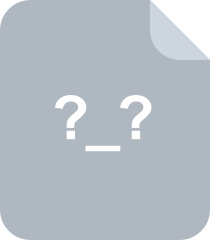
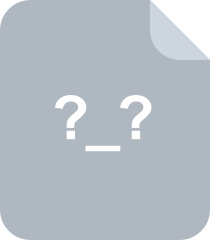
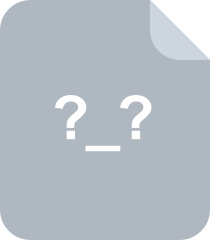
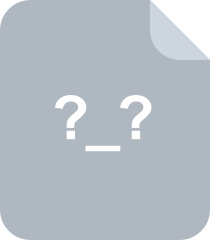
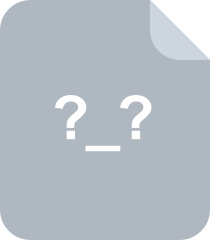
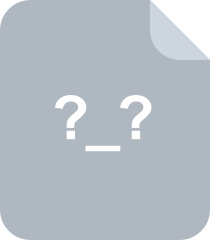
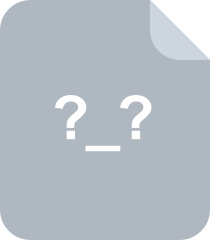
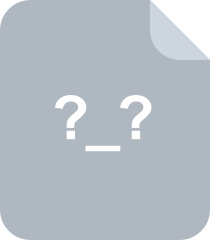
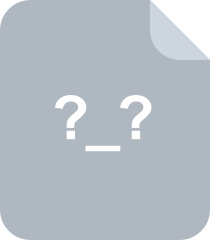
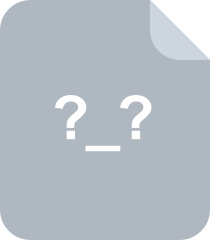
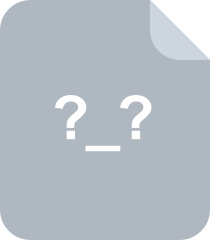
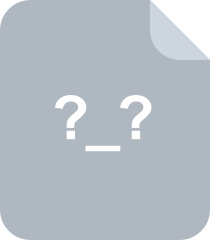
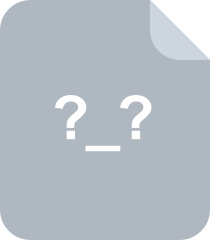
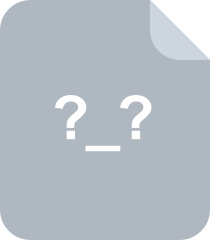
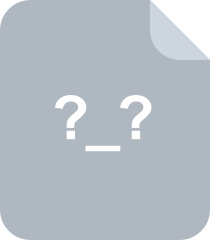
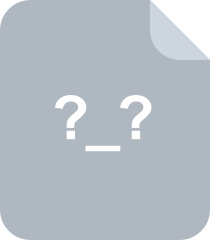
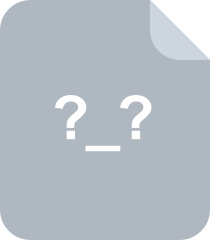
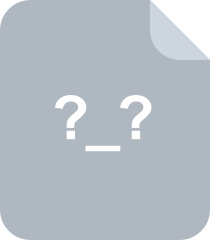
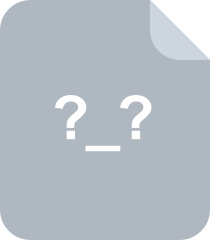
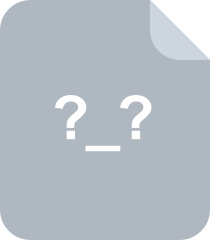
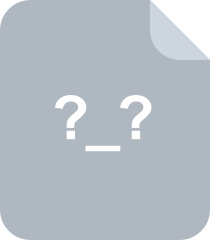
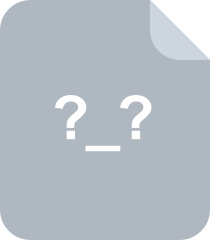
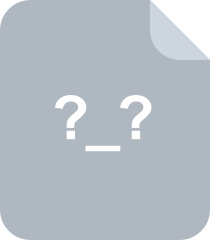
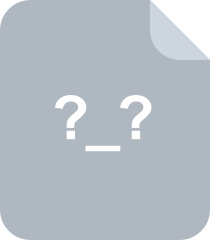
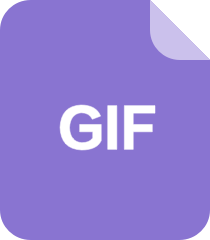
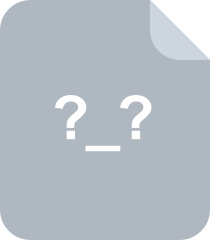
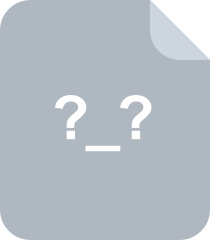
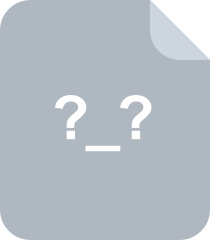
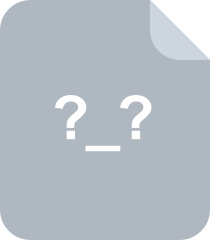
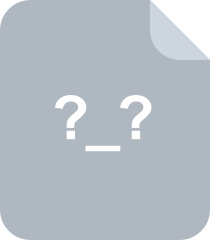
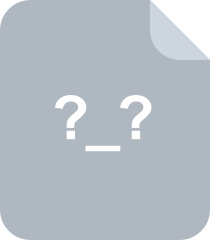
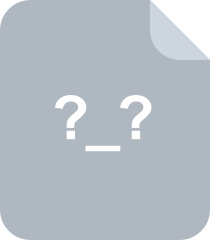
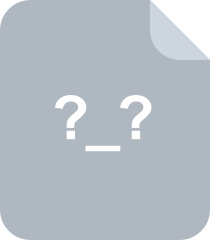
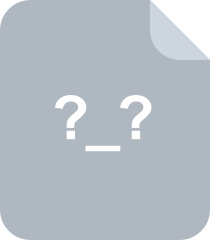
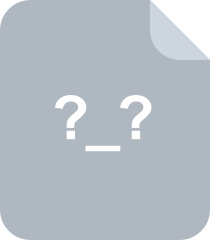
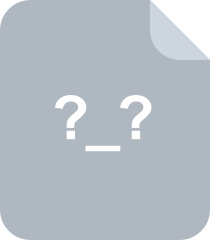
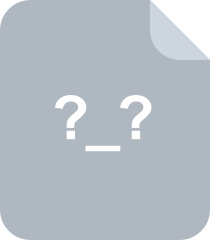
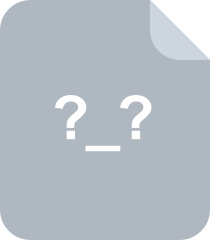
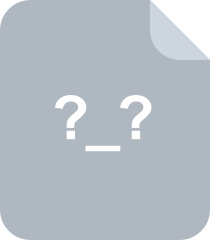
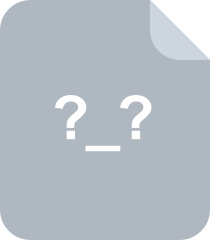
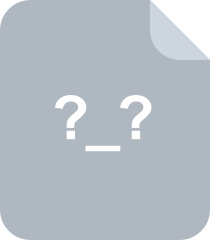
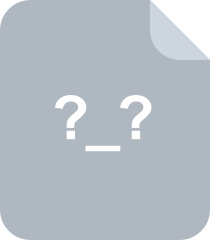
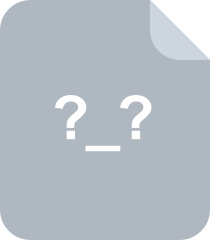
共 288 条
- 1
- 2
- 3

huaishuiyipen
- 粉丝: 2
- 资源: 14
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

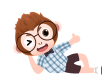
最新资源
- 机械手自动排列控制PLC与触摸屏程序设计
- uDDS源程序publisher
- 中国风格, 节日 主题, PPT模板
- 生菜生长记录数据集.zip
- 微环谐振腔的光学频率梳matlab仿真 微腔光频梳仿真 包括求解LLE方程(Lugiato-Lefever equation)实现微环中的光频梳,同时考虑了色散,克尔非线性,外部泵浦等因素,具有可延展
- 企业宣传PPT模板, 企业宣传PPT模板
- jetbra插件工具,方便开发者快速开发
- agv 1223.fbx
- 全国职业院校技能大赛网络建设与运维规程
- 混合动力汽车动态规划算法理论油耗计算与视频教学,使用matlab编写快速计算程序,整个工程结构模块化,可以快速改为串联,并联,混联等 控制量可以快速扩展为档位,转矩,转速等 状态量一般为SOC,目
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


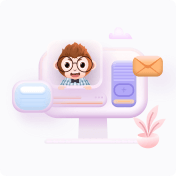
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
前往页