#⚠️ Looking for a maintainer ⚠️
Please contact me if you are interested in keeping our community alive:
https://github.com/stevenwanderski/bxslider-4/issues/1095
---
#bxSlider 4.2.12
##The fully-loaded, responsive jQuery content slider
###Why should I use this slider?
* Fully responsive - will adapt to any device
* Horizontal, vertical, and fade modes
* Slides can contain images, video, or HTML content
* Full callback API and public methods
* Small file size, fully themed, simple to implement
* Browser support: Firefox, Chrome, Safari, iOS, Android, IE7+
* Tons of configuration options
For complete documentation, tons of examples, and a good time, visit:
[http://bxslider.com](http://bxslider.com)
Written by: Steven Wanderski - [http://stevenwanderski.com](http://stevenwanderski.com)
###License
Released under the MIT license - http://opensource.org/licenses/MIT
Let's get on with it!
##Installation
###Step 1: Link required files
First and most important, the jQuery library needs to be included (no need to download - link directly from Google). Next, download the package from this site and link the bxSlider CSS file (for the theme) and the bxSlider Javascript file.
```html
<!-- jQuery library (served from Google) -->
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.3/jquery.min.js"></script>
<!-- bxSlider Javascript file -->
<script src="/js/jquery.bxslider.min.js"></script>
<!-- bxSlider CSS file -->
<link href="/lib/jquery.bxslider.css" rel="stylesheet" />
```
###Step 2: Create HTML markup
Create a `<ul class="bxslider">` element, with a `<li>` for each slide. Slides can contain images, video, or any other HTML content!
```html
<ul class="bxslider">
<li><img src="/images/pic1.jpg" /></li>
<li><img src="/images/pic2.jpg" /></li>
<li><img src="/images/pic3.jpg" /></li>
<li><img src="/images/pic4.jpg" /></li>
</ul>
```
###Step 3: Call the bxSlider
Call .bxSlider() on `<ul class="bxslider">`. Note that the call must be made inside of a $(document).ready() call, or the plugin will not work!
```javascript
$(document).ready(function(){
$('.bxslider').bxSlider();
});
```
##Configuration options
###General
**mode**
Type of transition between slides
```
default: 'horizontal'
options: 'horizontal', 'vertical', 'fade'
```
**speed**
Slide transition duration (in ms)
```
default: 500
options: integer
```
**slideMargin**
Margin between each slide
```
default: 0
options: integer
```
**startSlide**
Starting slide index (zero-based)
```
default: 0
options: integer
```
**randomStart**
Start slider on a random slide
```
default: false
options: boolean (true / false)
```
**slideSelector**
Element to use as slides (ex. <code>'div.slide'</code>).<br />Note: by default, bxSlider will use all immediate children of the slider element
```
default: ''
options: jQuery selector
```
**infiniteLoop**
If <code>true</code>, clicking "Next" while on the last slide will transition to the first slide and vice-versa
```
default: true
options: boolean (true / false)
```
**hideControlOnEnd**
If <code>true</code>, "Prev" and "Next" controls will receive a class <code>disabled</code> when slide is the first or the last<br/>Note: Only used when <code>infiniteLoop: false</code>
```
default: false
options: boolean (true / false)
```
**easing**
The type of "easing" to use during transitions. If using CSS transitions, include a value for the <code>transition-timing-function</code> property. If not using CSS transitions, you may include <code>plugins/jquery.easing.1.3.js</code> for many options.<br />See <a href="http://gsgd.co.uk/sandbox/jquery/easing/" target="_blank">http://gsgd.co.uk/sandbox/jquery/easing/</a> for more info.
```
default: null
options: if using CSS: 'linear', 'ease', 'ease-in', 'ease-out', 'ease-in-out', 'cubic-bezier(n,n,n,n)'. If not using CSS: 'swing', 'linear' (see the above file for more options)
```
**captions**
Include image captions. Captions are derived from the image's <code>title</code> attribute
```
default: false
options: boolean (true / false)
```
**ticker**
Use slider in ticker mode (similar to a news ticker)
```
default: false
options: boolean (true / false)
```
**tickerHover**
Ticker will pause when mouse hovers over slider. Note: this functionality does NOT work if using CSS transitions!
```
default: false
options: boolean (true / false)
```
**adaptiveHeight**
Dynamically adjust slider height based on each slide's height
```
default: false
options: boolean (true / false)
```
**adaptiveHeightSpeed**
Slide height transition duration (in ms). Note: only used if <code>adaptiveHeight: true</code>
```
default: 500
options: integer
```
**video**
If any slides contain video, set this to <code>true</code>. Also, include <code>plugins/jquery.fitvids.js</code><br />See <a href="http://fitvidsjs.com/" target="_blank">http://fitvidsjs.com/</a> for more info
```
default: false
options: boolean (true / false)
```
**responsive**
Enable or disable auto resize of the slider. Useful if you need to use fixed width sliders.
```
default: true
options: boolean (true /false)
```
**useCSS**
If true, CSS transitions will be used for horizontal and vertical slide animations (this uses native hardware acceleration). If false, jQuery animate() will be used.
```
default: true
options: boolean (true / false)
```
**preloadImages**
If 'all', preloads all images before starting the slider. If 'visible', preloads only images in the initially visible slides before starting the slider (tip: use 'visible' if all slides are identical dimensions)
```
default: 'visible'
options: 'all', 'visible'
```
**touchEnabled**
If <code>true</code>, slider will allow touch swipe transitions
```
default: true
options: boolean (true / false)
```
**swipeThreshold**
Amount of pixels a touch swipe needs to exceed in order to execute a slide transition. Note: only used if <code>touchEnabled: true</code>
```
default: 50
options: integer
```
**oneToOneTouch**
If <code>true</code>, non-fade slides follow the finger as it swipes
```
default: true
options: boolean (true / false)
```
**preventDefaultSwipeX**
If <code>true</code>, touch screen will not move along the x-axis as the finger swipes
```
default: true
options: boolean (true / false)
```
**preventDefaultSwipeY**
If <code>true</code>, touch screen will not move along the y-axis as the finger swipes
```
default: false
options: boolean (true / false)
```
**wrapperClass**
Class to wrap the slider in. Change to prevent from using default bxSlider styles.
```
default: 'bx-wrapper'
options: string
```
###Pager
**pager**
If <code>true</code>, a pager will be added
```
default: true
options: boolean (true / false)
```
**pagerType**
If <code>'full'</code>, a pager link will be generated for each slide. If <code>'short'</code>, a x / y pager will be used (ex. 1 / 5)
```
default: 'full'
options: 'full', 'short'
```
**pagerShortSeparator**
If <code>pagerType: 'short'</code>, pager will use this value as the separating character
```
default: ' / '
options: string
```
**pagerSelector**
Element used to populate the populate the pager. By default, the pager is appended to the bx-viewport
```
default: ''
options: jQuery selector
```
**pagerCustom**
Parent element to be used as the pager. Parent element must contain a <code><a data-slide-index="x"></code> element for each slide. See example <a href="/examples/thumbnail-method-1">here</a>. Not for use with dynamic carousels.
```
default: null
options: jQuery selector
```
**buildPager**
If supplied, function is called on every slide element, and the returned value is used as the pager item markup.<br />See <a href="http://bxslider.com/examples">examples</a> for detailed implementation
```
default: null
options: function(slideIndex)
```
###Controls
**controls**
If <code>true</code>, "Next" / "Prev" controls will be added
```
default: true
options: boolean (true / false)
```
**nextText**
Text to be used for the "Next" control
```
default: 'Next'

Lee达森
- 粉丝: 1572
- 资源: 1万+
最新资源
- 机器学习-bert模型
- 【毕业设计】基于Python的Django-html基于语音识别的智能垃圾分类系统源码(完整前后端+mysql+说明文档+LW+PPT).zip
- ERA5再分析数据根区土壤水分下载_era5土壤湿度-CSDN博客.html
- 【毕业设计】基于Python的Django-html基于小波变换的数字水印研究系统源码(完整前后端+mysql+说明文档+LW+PPT).zip
- 【毕业设计】基于Python的Django-html基于知识图谱的百科知识问答平台源码(完整前后端+mysql+说明文档+LW+PPT).zip
- 【毕业设计】基于Python的Django-html开放领域事件抽取系统源码(完整前后端+mysql+说明文档+LW+PPT).zip
- 【毕业设计】基于Python的Django-html旅游城市关键词分析系统源码(完整前后端+mysql+说明文档+LW+PPT).zip
- 【毕业设计】基于Python的Django-html基于知识图谱的医疗问答系统源码(完整前后端+mysql+说明文档+LW+PPT).zip
- 【毕业设计】基于Python的Django-html某大学学生影响力分析系统源码(完整前后端+mysql+说明文档+LW+PPT).zip
- Unity RPG地图场景资源
- 【毕业设计】基于Python的Django-html棉花数据平台建设与可视化系统源码(完整前后端+mysql+说明文档+LW+PPT).zip
- hymenoptera-data 数据集
- Java I/O流及其应用详解:字节流、字符流及相关工具类全面解析
- 机器学习-apriori算法-超市关联分析
- 【毕业设计】基于Python的Django-html企业物流管理系统源码(完整前后端+mysql+说明文档+LW+PPT).zip
- 【毕业设计】基于Python的Django-html某医院体检挂号系统源码(完整前后端+mysql+说明文档+LW+PPT).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


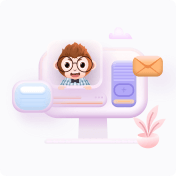