<?php
/***** start session *****/
ob_start();
/***** translatable theme *****/
load_theme_textdomain( 'fundingpress', get_parent_theme_file_path('langs'));
/***** include files *****/
require_once (get_parent_theme_file_path('themeOptions/functions.php'));
require_once (get_parent_theme_file_path('themeOptions/rating.php'));
require_once (get_parent_theme_file_path('inc/lib/class-tgm-plugin-activation.php'));
require_once (get_parent_theme_file_path('vc.php'));
require_once ( ABSPATH . 'wp-admin/includes/plugin.php');
add_action( 'after_setup_theme', 'funding_theme_setup' );
if (!function_exists('funding_theme_setup')) {
function funding_theme_setup() {
/*****THEME-SUPPORTED FEATURES*****/
/*****ACTIONS*****/
/*init*/
add_action('init', 'funding_allowed_tags');
add_action('init', 'funding_do_output_buffer');
add_action( 'init', 'funding_register_my_menus' );
if ( current_user_can('contributor') && !current_user_can('upload_files') )
add_action('admin_init', 'funding_allow_contributor_uploads');
/*admin-init*/
add_action( 'admin_init', 'funding_update_caps');
add_action( 'admin_init', 'funding_restrict_admin_area_to_contributors');
/*project columns*/
add_action( 'manage_project_posts_custom_column', 'funding_my_manage_project_columns', 10, 2 );
/*theme scripts*/
add_action('wp_enqueue_scripts', 'funding_my_scripts');
add_action('wp_enqueue_scripts', 'funding_my_style' );
add_action('admin_enqueue_scripts', 'funding_admin_scripts');
add_action('admin_enqueue_scripts', 'funding_styles_admin');
/*ajax functions*/
add_action( 'wp_ajax_nopriv_funding_capture_card', 'funding_capture_card' );
add_action( 'wp_ajax_funding_capture_card', 'funding_capture_card' );
add_action( 'wp_ajax_nopriv_funding_unlink_stripe', 'funding_unlink_stripe' );
add_action( 'wp_ajax_funding_unlink_stripe', 'funding_unlink_stripe' );
add_action( 'wp_ajax_nopriv_load-filter-all', 'funding_prefix_load_cat_posts_all' );
add_action( 'wp_ajax_load-filter-all', 'funding_prefix_load_cat_posts_all' );
add_action( 'wp_ajax_nopriv_funding_delete_projects', 'funding_delete_projects' );
add_action( 'wp_ajax_funding_delete_projects', 'funding_delete_projects' );
add_action( 'wp_ajax_nopriv_funding_unlink_wepay', 'funding_unlink_wepay' );
add_action( 'wp_ajax_funding_unlink_wepay', 'funding_unlink_wepay' );
add_action( 'wp_ajax_nopriv_funding_get_wepay_token', 'funding_get_wepay_token' );
add_action( 'wp_ajax_funding_get_wepay_token', 'funding_get_wepay_token' );
add_action( 'wp_ajax_nopriv_delcomments', 'funding_delete_comments' );
add_action( 'wp_ajax_delcomments', 'funding_delete_comments' );
add_action( 'wp_ajax_nopriv_load-filter', 'funding_prefix_load_cat_posts' );
add_action( 'wp_ajax_load-filter', 'funding_prefix_load_cat_posts' );
add_action( 'wp_ajax_nopriv_return_currency', 'funding_return_currency' );
add_action( 'wp_ajax_return_currency', 'funding_return_currency' );
add_action( 'wp_ajax_nopriv_ajaxlogin', 'funding_ajax_login' );
add_action( 'wp_ajax_ajaxlogin', 'funding_ajax_login' );
add_action( 'wp_ajax_nopriv_charge_funder', 'funding_frontend_charge_funder' );
add_action( 'wp_ajax_charge_funder', 'funding_frontend_charge_funder' );
/*vc*/
add_action( 'vc_before_init', 'funding_vc_remove_be_pointers' );
add_action( 'vc_before_init', 'funding_vc_remove_fe_pointers' );
/*date var*/
add_action( 'admin_head', 'funding_dateformat_var' );
/*required plugins*/
add_action( 'tgmpa_register', 'funding_register_required_plugins' );
/*restrict media*/
add_action('pre_get_posts','funding_restrict_media_library');
/*admin class*/
add_action('admin_body_class', 'funding_adminclass');
/*menu*/
add_action( 'admin_menu', 'fundingpress_create_menu' );
/*notifications*/
add_action( 'transition_post_status', 'funding_notify_user_on_publish', 10, 3 );
/*project updates*/
add_action('comment_post', 'funding_notify_backers_update', 10, 3);
/*importer*/
add_action( 'radium_importer_after_content_import', 'gameaddict_import_additional_resources');
/*****FILTERS*****/
/***** add custom columns for projects in back end *****/
add_filter( 'manage_edit-project_columns', 'funding_my_edit_project_columns' ) ;
/*category pagination*/
add_filter('request', 'funding_fix_category_pagination');
/*comments*/
add_filter('comment_form_defaults', 'funding_comment_form_defaults');
add_filter( 'comment_text', 'funding_oembed_comments', 0 );
/*avatars*/
add_filter( 'get_avatar', 'funding_be_gravatar_filter', 1, 5 );
/*content*/
add_filter("the_content", "funding_the_content_filter");
/*meta filters*/
add_filter( 'postmeta_form_limit', 'funding_hide_meta_start' );
/*excerpt*/
add_filter( 'excerpt_length', 'funding_custom_excerpt_length', 999 );
add_filter('excerpt_more', 'funding_excerpt_more');
/*add custom menu support*/
add_theme_support( 'post-thumbnails' );
add_theme_support( 'woocommerce' );
add_theme_support( 'title-tag' );
add_theme_support( 'automatic-feed-links' );
add_theme_support( 'custom-header' );
add_theme_support( 'custom-background' );
if ( ! isset( $content_width ) ) $content_width = 900;
}
}
/***** get id by slug function *****/
if (!function_exists('funding_get_ID_by_slug')) {
function funding_get_ID_by_slug($page_slug) {
$page = get_page_by_path($page_slug);
if ($page) {
return $page->ID;
} else {
return null;
}
}
}
if (!function_exists('funding_allow_contributor_uploads')) {
function funding_allow_contributor_uploads() {
$role = get_role('contributor');
$role->add_cap('upload_files');
$role->add_cap('delete_others_posts');
$role->add_cap('delete_posts');
$role->add_cap('delete_published_posts');
}
}
if (!function_exists('funding_my_edit_project_columns')) {
function funding_my_edit_project_columns( $columns ) {
$columns = array(
"cb" => "<input type=\"checkbox\" />",
"title" => esc_html__( "Project Title", 'fundingpress' ),
"status" => esc_html__( 'Project status', 'fundingpress' ),
"funding-progress" => esc_html__( "Progress", 'fundingpress' ),
"funding-time" => esc_html__( "Time Remaining", 'fundingpress' ),
"ppal" => esc_html__( "Paypal", 'fundingpress' ),
"wepay" => esc_html__( "Wepay", 'fundingpress' ),
"stripe" => esc_html__( "Stripe", 'fundingpress' ),
"author" => esc_html__( "Creator", 'fundingpress' ),
"comments" => '<img src="'.site_url().'/wp-admin/images/comment-grey-bubble.png" alt="Comments" />',
'date' => esc_html__( 'Date', 'fundingpress' ),
);
return $columns;
}
}
if (!function_exists('funding_my_manage_project_columns')) {
function funding_my_manage_project_columns( $column, $post_id ) {
global $post;
switch( $column ) {
/* If displaying the 'duration' column. */
case 'funding-time' :
$project_settings = (array) get_post_meta($post_id, 'settings', true);
if(get_option('date_format') == 'm/d/Y' && strtotime($project_settings['date']) != false){
$array = explode('/', $project_settings['date']);
$tmp = $array[0];
$array[0] = $array[1];
$array[1] = $tmp;
unset($tmp);
if($array[0] == NULL){
$project_settings['date'] = $array[1];
}else{
$project_settings['date'] = implode('/', $array);
}
}
if(get_option('date_format') == 'd/m/Y' && strtotime($project_settings['date']) != false){
$array = explode('/', $project_settings['date']);
$tmp = $array[0];
$array[0] = $array[1];
$array[1] = $tmp;
unset($tmp);
if($array[0] == NULL){
$project_settings['date'] = $array[1];
}else{
$project_settings['date'] = implode('/', $array);
}
}
if(isset($project_settings['ta
没有合适的资源?快使用搜索试试~ 我知道了~
【WordPress主题】2022年最新版完整功能demo+插件5.2.zip
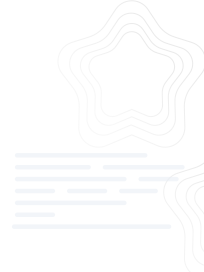
共252个文件
png:79个
php:55个
jpg:46个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 30 浏览量
2022-04-07
14:05:21
上传
评论
收藏 17.63MB ZIP 举报
温馨提示
"【WordPress主题】2022年最新版完整功能demo+插件5.2 Fundingpress - The Crowdfunding WordPress Theme FundingSpress - 众筹的WordPress主题" ---------- 泰森云每天更新发布最新WordPress主题、HTML主题、WordPress插件、shopify主题、opencart主题、PHP项目源码、安卓项目源码、ios项目源码,更有超10000个资源可供选择,如有需要请站内联系。
资源推荐
资源详情
资源评论
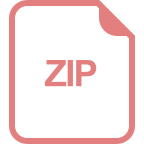
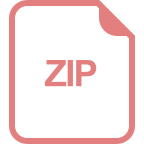
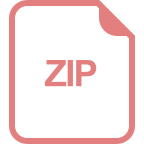
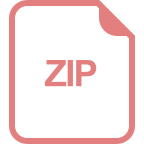
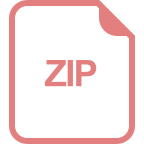
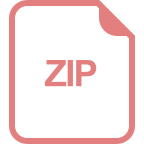
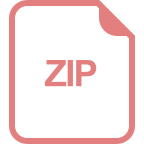
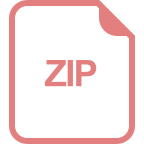
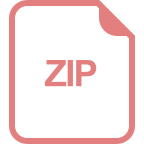
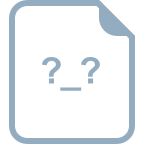
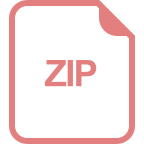
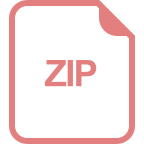
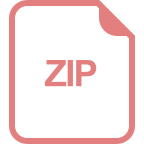
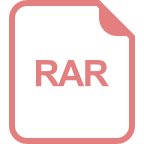
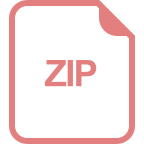
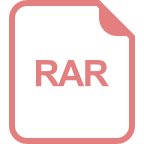
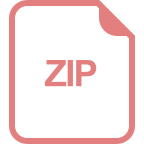
收起资源包目录

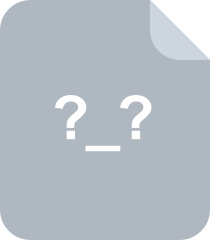
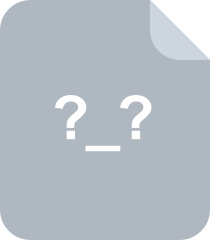
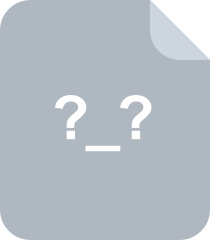
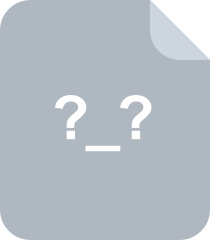
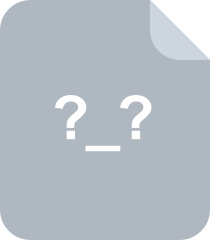
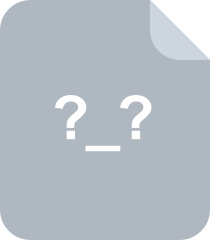
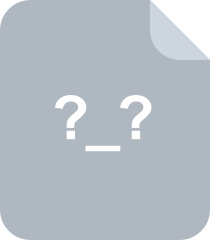
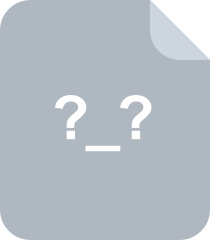
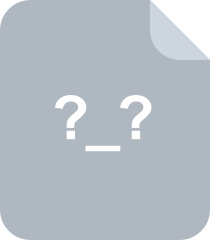
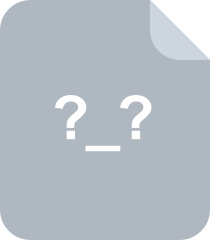
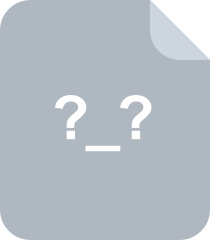
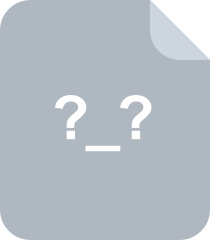
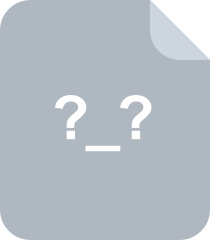
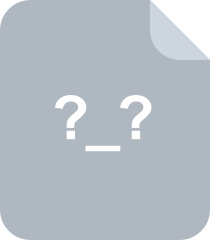
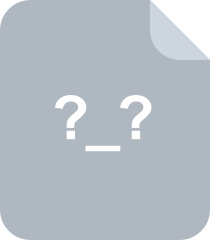
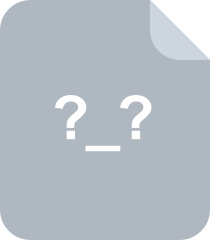
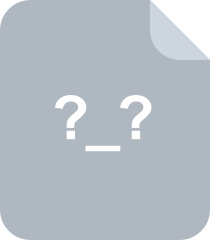
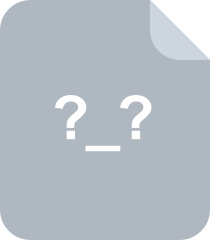
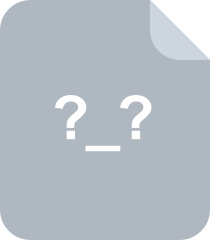
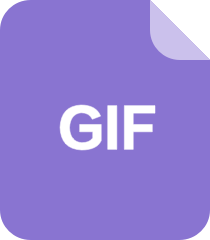
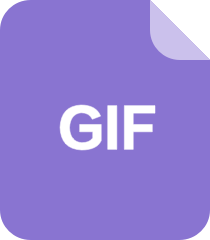
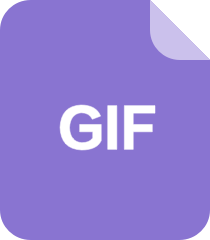
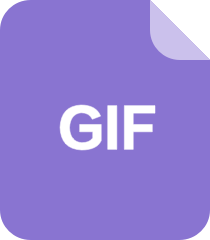
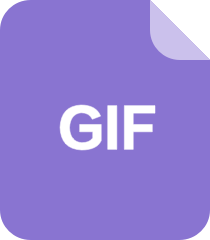
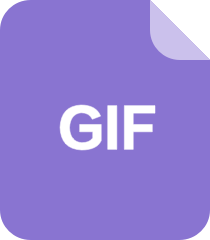
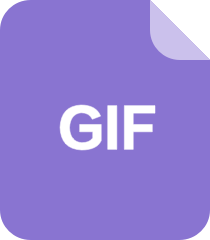
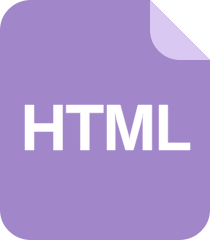
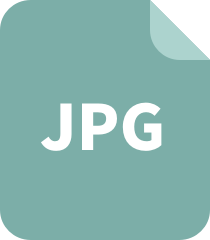
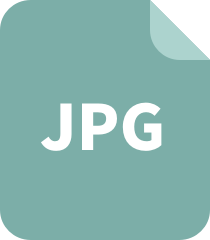
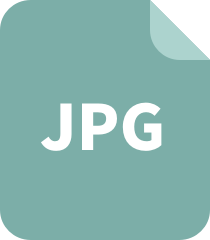
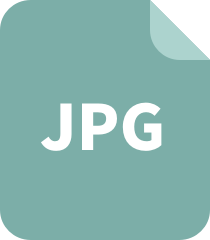
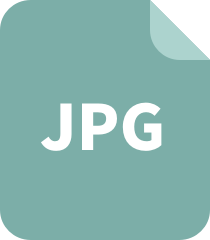
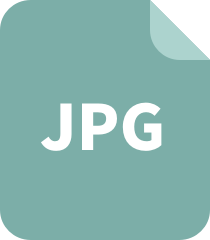
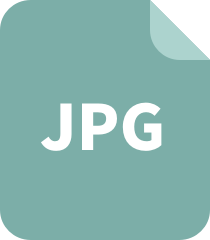
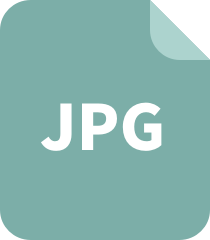
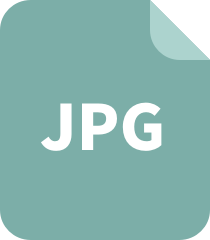
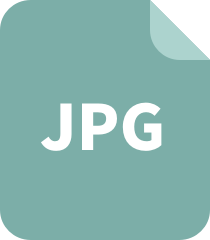
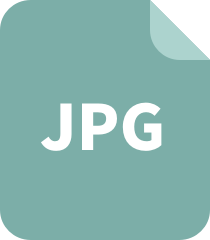
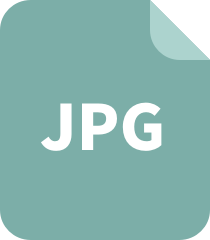
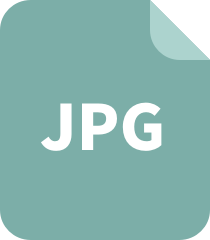
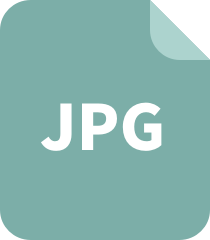
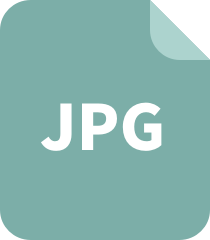
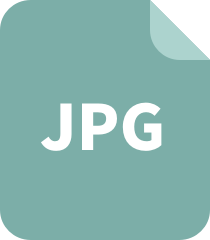
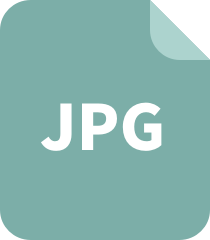
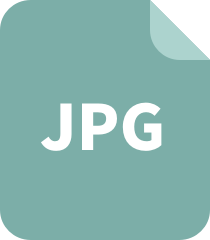
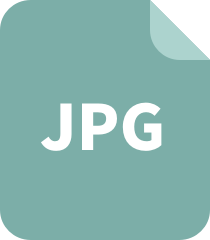
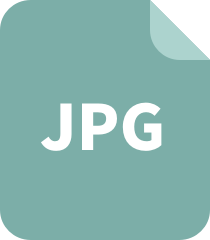
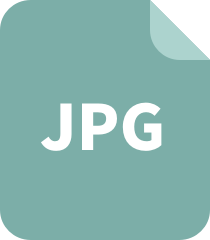
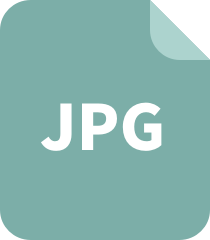
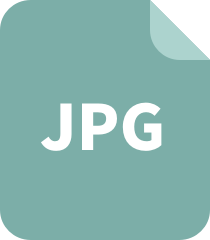
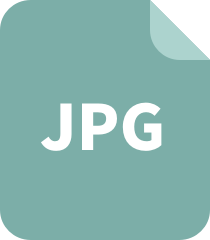
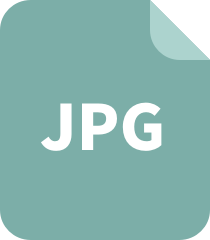
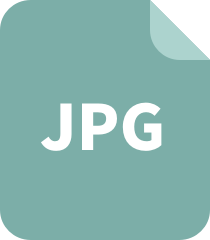
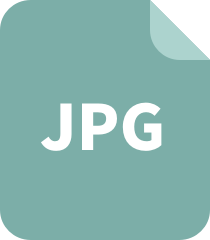
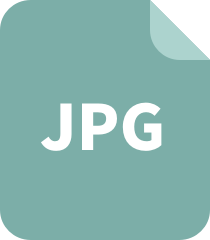
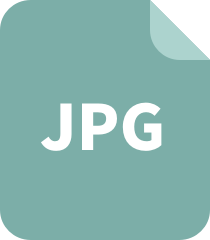
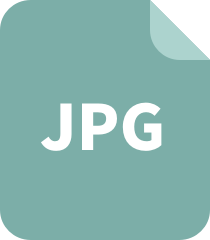
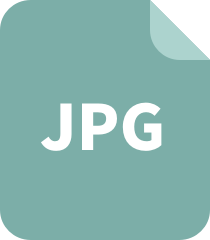
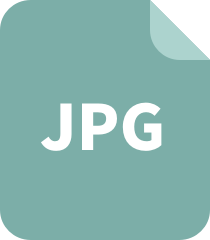
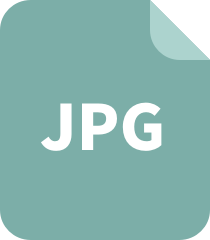
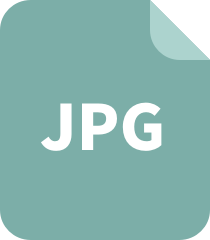
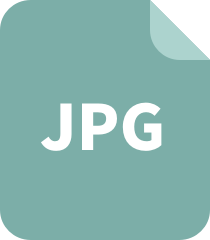
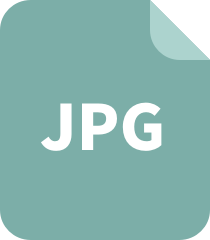
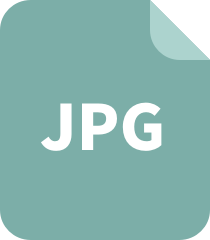
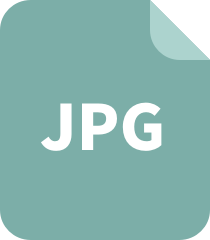
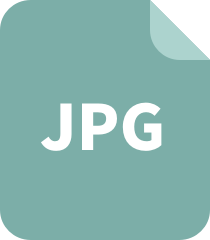
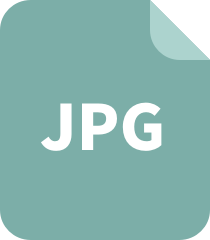
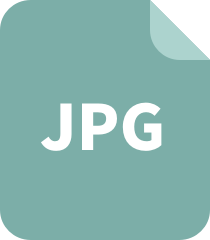
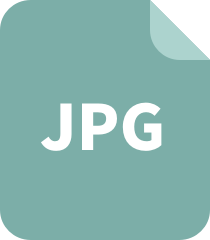
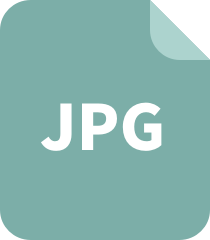
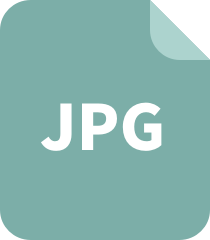
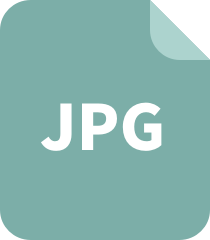
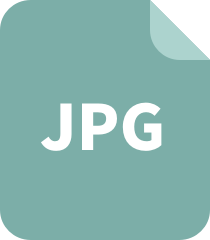
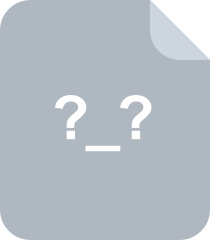
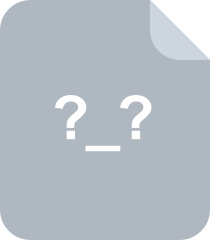
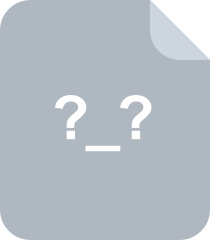
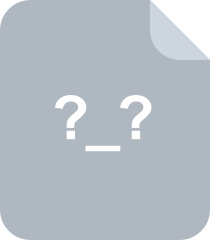
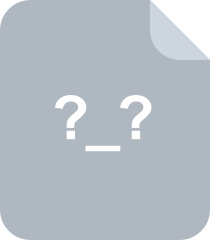
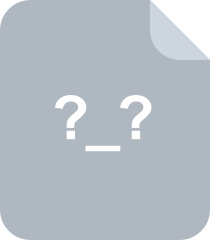
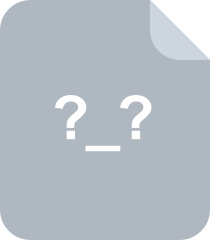
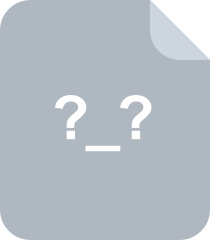
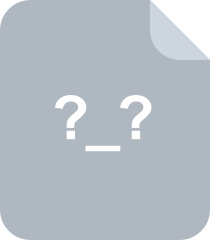
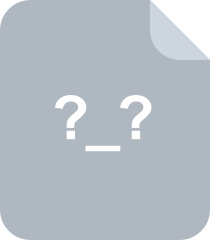
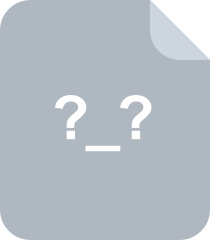
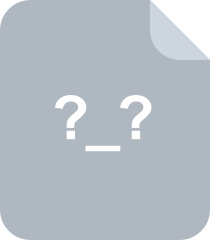
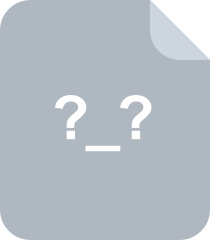
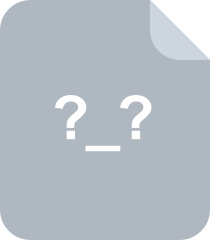
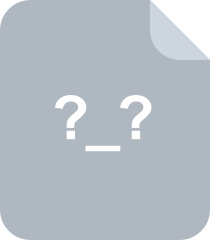
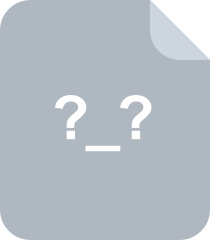
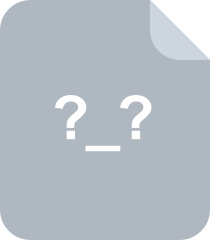
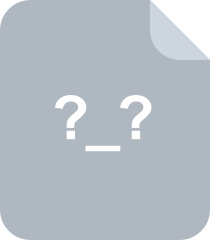
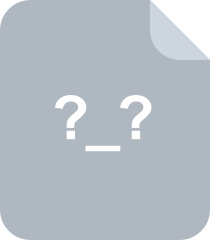
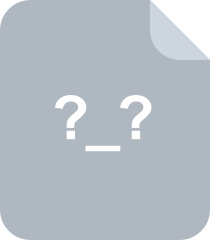
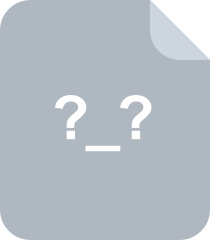
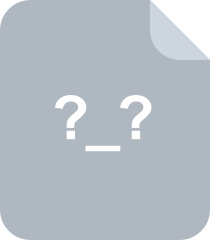
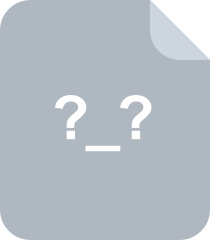
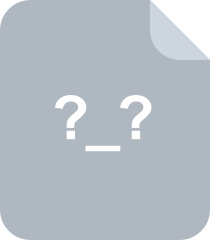
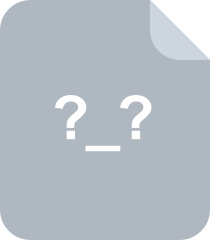
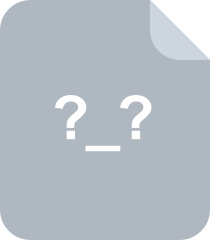
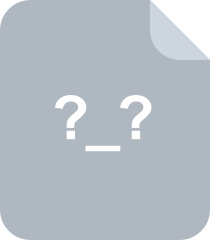
共 252 条
- 1
- 2
- 3
资源评论
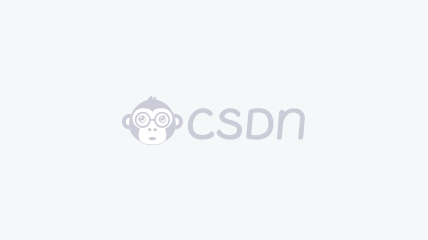

Lee达森
- 粉丝: 1519
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

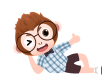
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


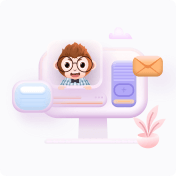
安全验证
文档复制为VIP权益,开通VIP直接复制
