<?php
// Disable Gutenberg
add_filter( 'gutenberg_can_edit_post_type', '__return_false' );
add_filter( 'use_block_editor_for_post_type', '__return_false' );
// Disable "Try Gutenberg" panel
remove_action( 'try_gutenberg_panel', 'wp_try_gutenberg_panel' );
function ppb_text_func($atts, $content) {
//extract short code attr
extract(shortcode_atts(array(
'size' => 'one',
'layout' => 'fixedwidth',
'background' => '',
'background_parallax' => 'none',
'custom_css' => '',
), $atts));
$return_html = '<div class="'.$size.' withsmallpadding ';
if(!empty($background))
{
$return_html.= 'withbg ';
}
if(!empty($layout) && $layout == 'fullwidth')
{
$return_html.= 'fullwidth ';
}
if(!empty($background_parallax) && $background_parallax!='none')
{
$return_html.= 'parallax';
}
$return_html.= '" ';
$parallax_data = '';
//Get image width and height
$background = esc_url($background);
$pp_background_image_id = pp_get_image_id($background);
if(!empty($pp_background_image_id))
{
$background_image_arr = wp_get_attachment_image_src($pp_background_image_id, 'original');
$background_image = $background_image_arr[0];
$background_image_width = $background_image_arr[1];
$background_image_height = $background_image_arr[2];
}
else
{
$background_image = $background;
$background_image_width = '';
$background_image_height = '';
}
//Check parallax background
switch($background_parallax)
{
case 'scroll_pos':
case 'mouse_pos':
case 'scroll_pos':
case 'mouse_scroll_pos':
$parallax_data = ' data-image="'.esc_attr($background_image).'" data-width="'.esc_attr($background_image_width).'" data-height="'.esc_attr($background_image_height).'"';
break;
}
if((empty($background_parallax) OR $background_parallax=='none') && !empty($background))
{
$return_html.= 'style="background-image:url('.esc_attr($background_image).');background-size:cover;'.urldecode(esc_attr($custom_css)).'" ';
}
elseif(!empty($custom_css))
{
$return_html.= 'style="'.urldecode(esc_attr($custom_css)).'" ';
}
$return_html.= $parallax_data;
$return_html.= '><div class="page_content_wrapper">'.do_shortcode(tg_apply_content($content)).'</div>';
$return_html.= '</div>';
return $return_html;
}
add_shortcode('ppb_text', 'ppb_text_func');
function ppb_divider_func($atts, $content) {
//extract short code attr
extract(shortcode_atts(array(
'size' => 'one'
), $atts));
$return_html = '<div class="divider '.$size.'"> </div>';
return $return_html;
}
add_shortcode('ppb_divider', 'ppb_divider_func');
function ppb_tour_search_func($atts, $content) {
//extract short code attr
extract(shortcode_atts(array(
'size' => 'one',
'action' => '',
'custom_css' => '',
), $atts));
wp_enqueue_script("jquery-ui-core");
wp_enqueue_script("jquery-ui-datepicker");
wp_enqueue_script("custom_date", get_template_directory_uri()."/js/custom-date.js", false, THEMEVERSION, "all");
$return_html = '<div class="one pp_tour_search" ';
if(!empty($custom_css))
{
$return_html.= 'style="'.urldecode($custom_css).'" ';
}
$return_html.= '><div class="page_content_wrapper">';
//Begin search form
$return_html.= '<form id="tour_search_form" name="tour_search_form" method="get" action="'.get_the_permalink($action).'">
<div class="tour_search_wrapper">';
$return_html.= '<div class="one_fourth">
<label for="keyword">'.__( 'Destination', THEMEDOMAIN ).'</label>
<input id="keyword" name="keyword" type="text" placeholder="'.__( 'City, region or keywords', THEMEDOMAIN ).'"/>
</div>';
$return_html.= '<div class="one_fourth">
<label for="start_date">'.__( 'Date', THEMEDOMAIN ).'</label>
<div class="start_date_input">
<input id="start_date" name="start_date" type="text" placeholder="'.__( 'Departure', THEMEDOMAIN ).'" />
<input id="start_date_raw" name="start_date_raw" type="hidden"/>
<i class="fa fa-calendar"></i>
</div>
<div class="end_date_input">
<input id="end_date" name="end_date" type="text" placeholder="'.__( 'Arrival', THEMEDOMAIN ).'"/>
<input id="end_date_raw" name="end_date_raw" type="hidden"/>
<i class="fa fa-calendar"></i>
</div>
</div>';
$return_html.= '<div class="one_fourth">
<label for="budget">'.__( 'Max Budgets', THEMEDOMAIN ).'</label>
<input id="budget" name="budget" type="text" placeholder="'.__( 'USD EX. 100', THEMEDOMAIN ).'"/>
</div>';
$return_html.= '<div class="one_fourth last">
<input id="tour_search_btn" type="submit" value="'.__( 'Search', THEMEDOMAIN ).'"/>
</div>';
$return_html.= '</div>';
$return_html.= '</form>';
$return_html.= '</div>';
$return_html.= '</div>';
return $return_html;
}
add_shortcode('ppb_tour_search', 'ppb_tour_search_func');
function ppb_tour_func($atts, $content) {
//extract short code attr
extract(shortcode_atts(array(
'size' => 'one',
'title' => '',
'items' => 4,
'tourcat' => '',
'order' => 'default',
'custom_css' => '',
'layout' => 'fullwidth',
), $atts));
if(!is_numeric($items))
{
$items = 4;
}
$return_html = '<div class="ppb_tour '.$size.' withpadding ';
$columns_class = 'three_cols';
if($layout=='fullwidth')
{
$columns_class.= ' fullwidth';
}
$element_class = 'one_third gallery3';
$tour_h = 'h5';
if(empty($content) && empty($title))
{
$return_html.='nopadding ';
}
$return_html.= '" ';
if(!empty($custom_css))
{
$return_html.= 'style="'.urldecode($custom_css).'" ';
}
$return_html.= '>';
$return_html.='<div class="page_content_wrapper ';
if($layout == 'fullwidth')
{
$return_html.='full_width';
}
$return_html.= '" style="text-align:center">';
//Display Title
if(!empty($title))
{
$return_html.= '<h2 class="ppb_title">'.$title.'</h2>';
}
//Display Content
if(!empty($content) && !empty($title))
{
$return_html.= '<div class="page_caption_desc">'.$content.'</div>';
}
//Display Horizontal Line
if(empty($content) && !empty($title))
{
$return_html.= '<br/>';
}
$tour_order = 'ASC';
$tour_order_by = 'menu_order';
switch($order)
{
case 'default':
$tour_order = 'ASC';
$tour_order_by = 'menu_order';
break;
case 'newest':
$tour_order = 'DESC';
$tour_order_by = 'post_date';
break;
case 'oldest':
$tour_order = 'ASC';
$tour_order_by = 'post_date';
break;
case 'title':
$tour_order = 'ASC';
$tour_order_by = 'title';
break;
case 'random':
$tour_order = 'ASC';
$tour_order_by = 'rand';
break;
}
//Get tour items
$args = array(
'numberposts' => $items,
'order' => $tour_order,
'orderby' => $tour_order_by,
'post_type' => array('tours'),
'suppress_filters' => 0,
);
if(!empty($tourcat))
{
$args['tourcats'] = $tourcat;
}
$tours_arr = get_posts($args);
if(!empty($tours_arr) && is_array($tours_arr))
{
$return_html.= '<div class="portfolio_filter_wrapper '.$columns_class.' shortcode gallery section content clearfix">';
foreach($tours_arr as $key => $tour)
{
$image_url = '';
$tour_ID = $tour->ID;
if(has_post_thumbnail($tour_ID, 'large'))
{
$image_id = get_post_thumbnail_id($tour_ID);
$image_url = wp_get_attachment_image_src($image_id, 'full', true);
$small_image_url = wp_get_attachment_image_src($image_id, 'gallery_grid', true);
}
//Get Tour Meta
$tour_permalink_url = get_permalink($tour_ID);
$tour_title = $tour->post_title;
$tour_country= get_post_meta($tour_ID, 'tour_country', true);
$tour_price= get_post_meta($tour_ID, 'tour_price', true);
$tour_price_discount= get_post_meta($tour_ID, 'tour_price_discount', true);
$tour_price_currency= get_post_meta($tour_ID, 'tour_price_currency', true);
$tour_discount_percentage = 0;
$tour_price_display = '';
if(!empty($tour_price))
{
if(!empty($tour_price_discount))
{
if($tour_pr
没有合适的资源?快使用搜索试试~ 我知道了~
【WordPress主题】2022年最新版完整功能demo+插件V5.2.zip
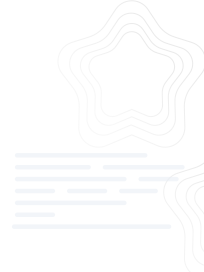
共419个文件
png:177个
php:90个
js:57个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 117 浏览量
2022-03-31
16:36:02
上传
评论
收藏 2.47MB ZIP 举报
温馨提示
"【WordPress主题】2022年最新版完整功能demo+插件V5.2 Altair - Travel Agency WordPress Theme Altair - 旅行社Wordpress主题" ---------- 泰森云每天更新发布最新WordPress主题、HTML主题、WordPress插件、shopify主题、opencart主题、PHP项目源码、安卓项目源码、ios项目源码,更有超10000个资源可供选择,如有需要请站内联系。
资源推荐
资源详情
资源评论
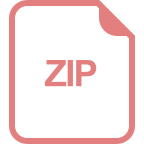
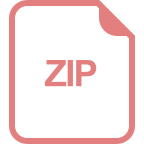
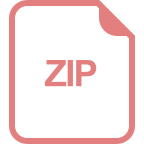
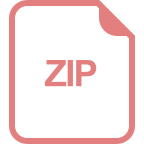
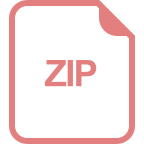
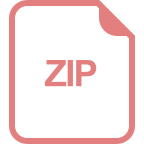
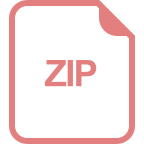
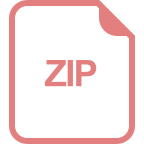
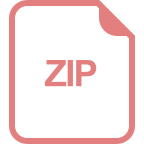
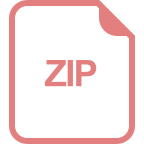
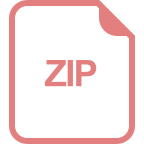
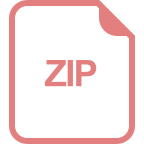
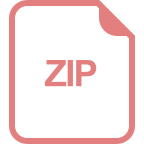
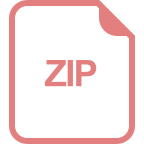
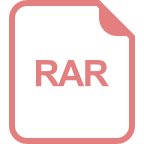
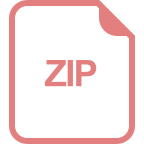
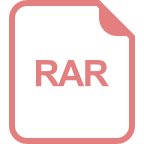
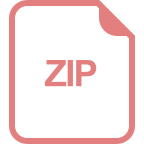
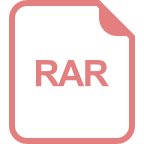
收起资源包目录

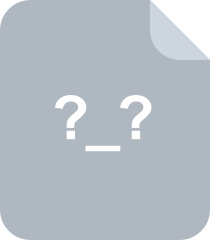
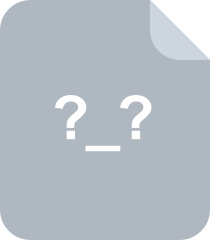
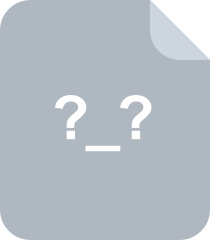
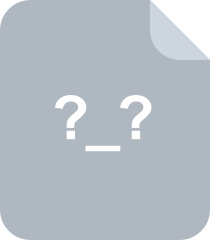
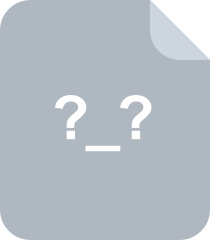
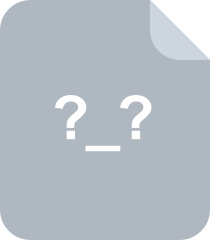
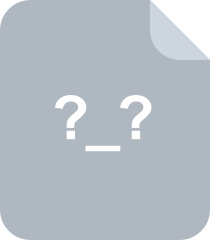
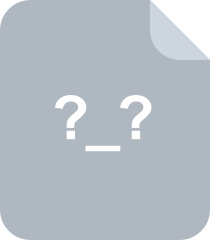
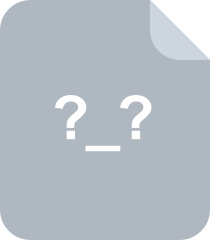
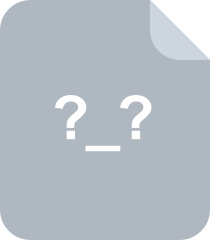
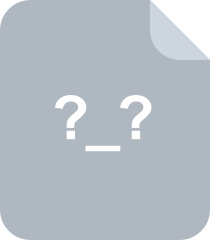
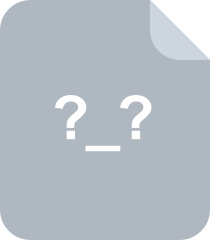
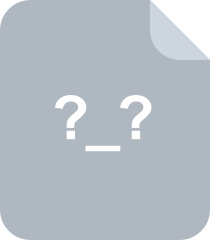
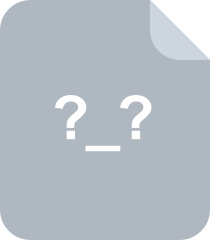
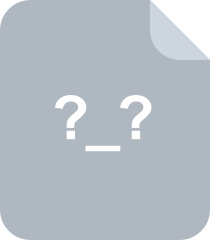
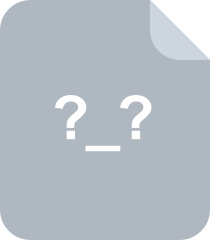
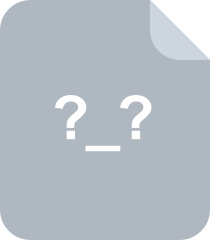
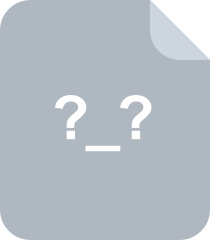
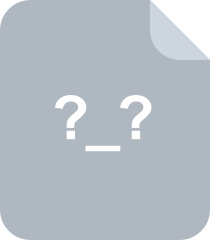
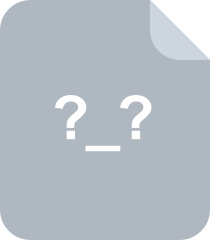
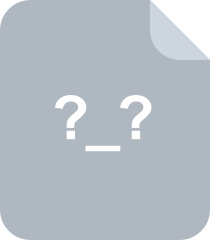
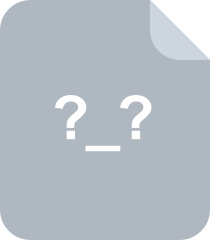
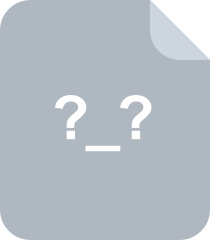
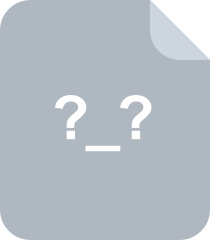
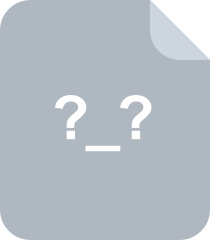
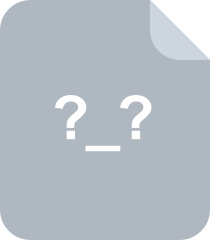
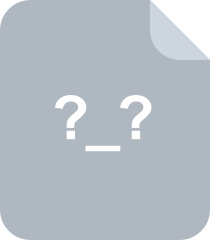
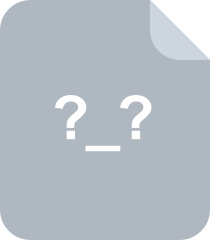
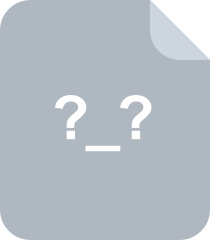
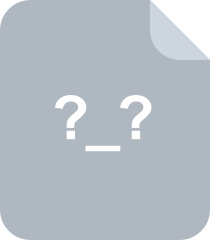
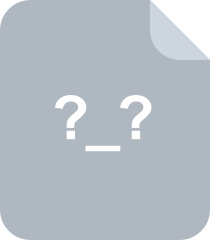
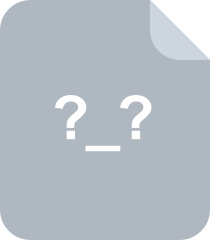
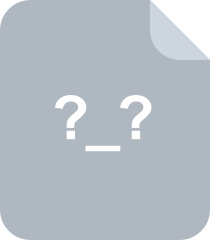
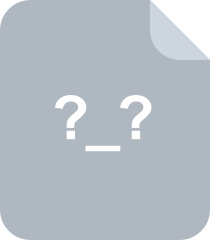
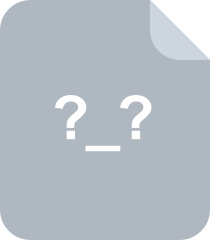
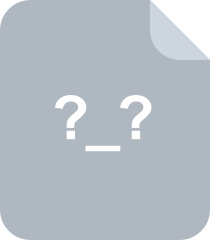
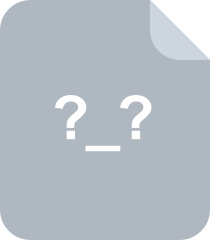
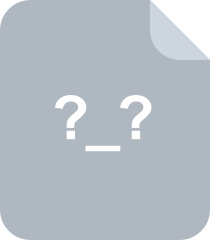
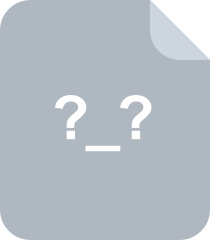
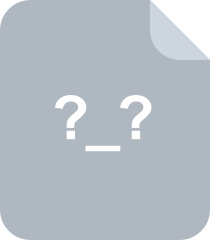
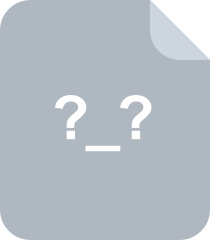
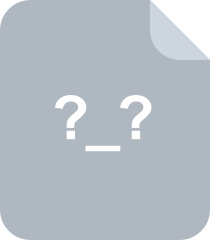
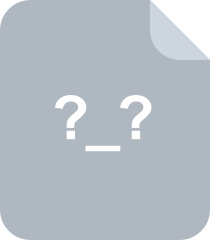
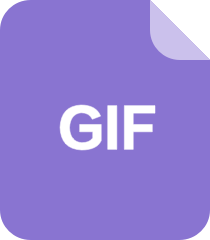
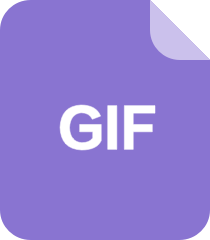
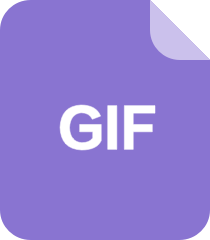
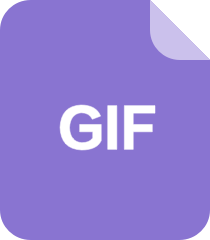
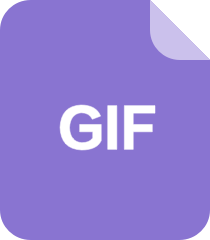
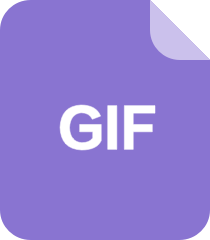
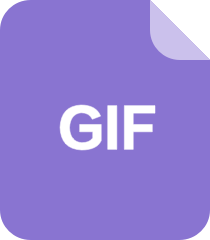
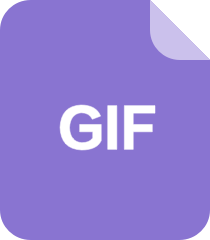
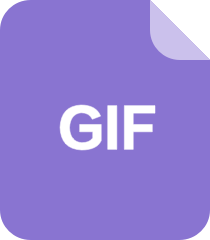
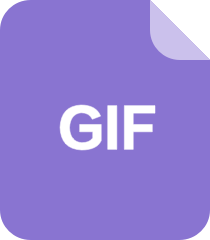
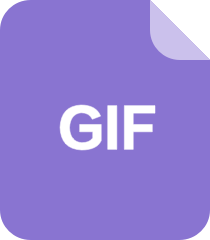
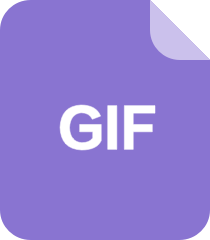
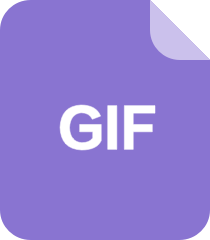
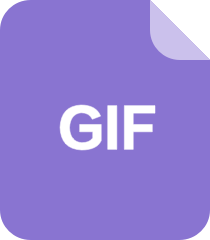
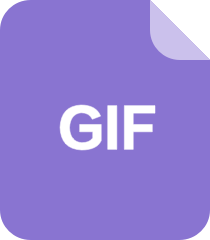
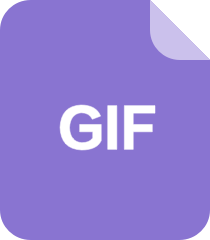
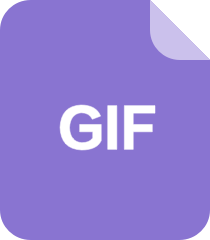
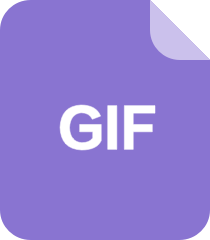
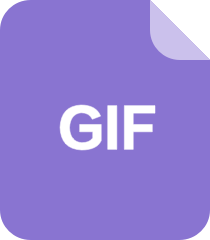
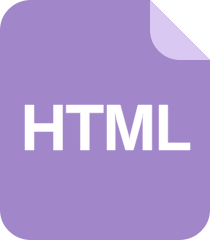
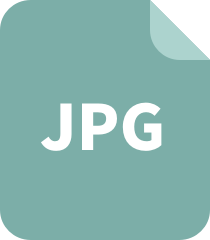
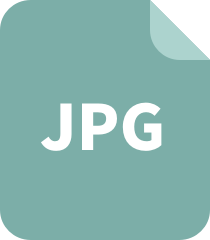
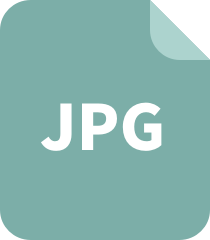
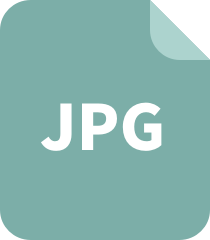
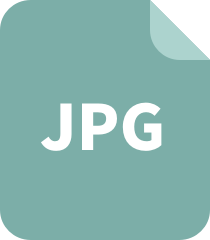
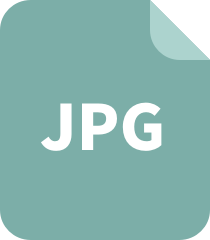
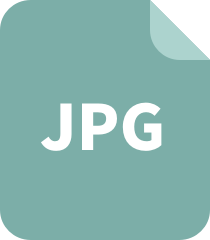
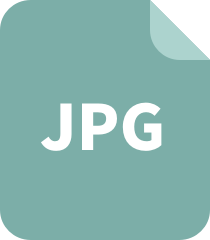
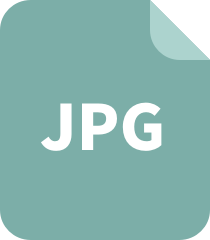
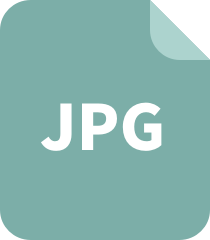
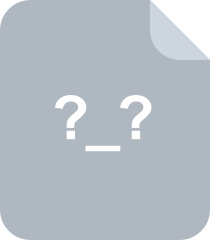
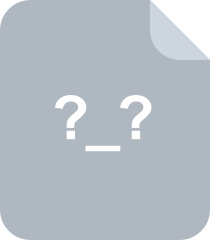
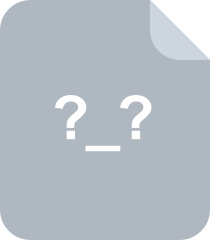
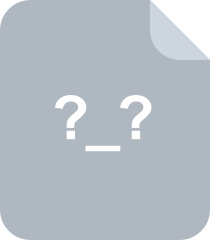
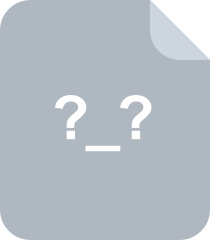
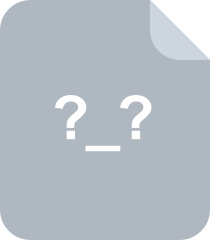
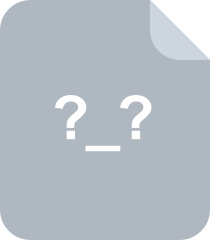
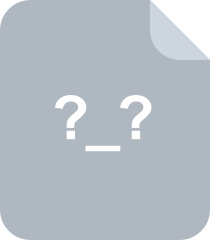
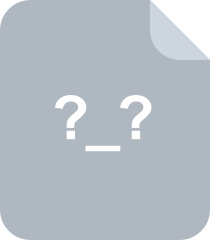
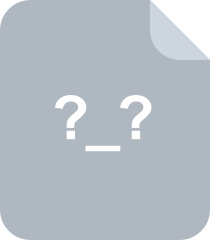
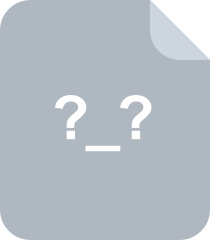
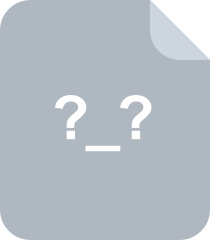
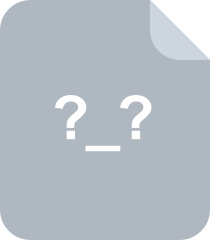
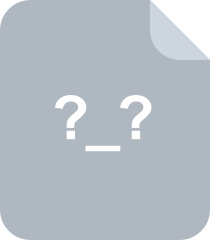
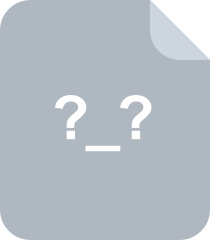
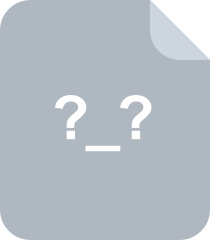
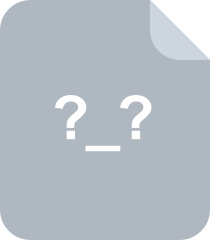
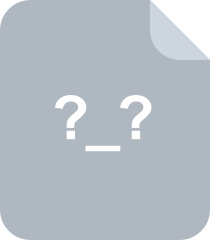
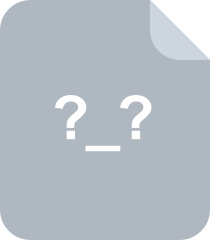
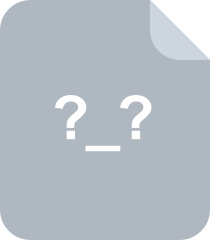
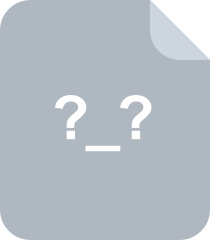
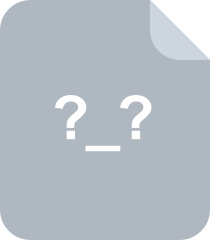
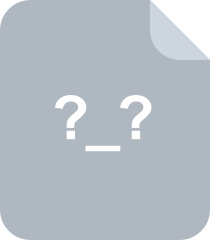
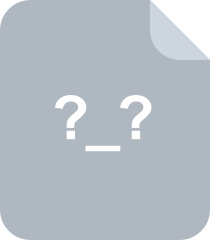
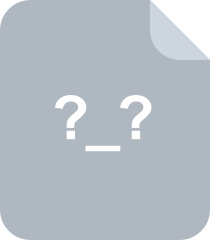
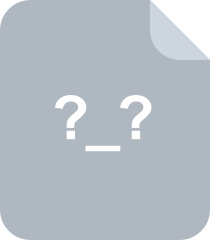
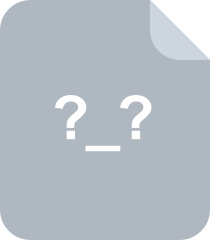
共 419 条
- 1
- 2
- 3
- 4
- 5
资源评论
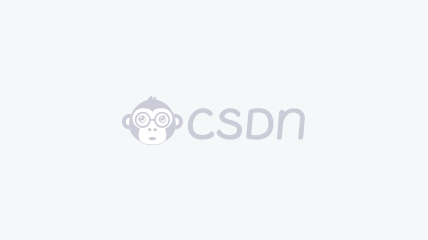

Lee达森
- 粉丝: 1519
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

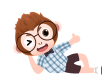
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


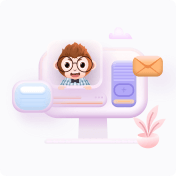
安全验证
文档复制为VIP权益,开通VIP直接复制
