Plugin Update Checker
=====================
This is a custom update checker library for WordPress plugins and themes. It lets you add automatic update notifications and one-click upgrades to your commercial plugins, private themes, and so on. All you need to do is put your plugin/theme details in a JSON file, place the file on your server, and pass the URL to the library. The library periodically checks the URL to see if there's a new version available and displays an update notification to the user if necessary.
From the users' perspective, it works just like with plugins and themes hosted on WordPress.org. The update checker uses the default upgrade UI that is familiar to most WordPress users.
<!-- START doctoc generated TOC please keep comment here to allow auto update -->
<!-- DON'T EDIT THIS SECTION, INSTEAD RE-RUN doctoc TO UPDATE -->
**Table of Contents**
- [Getting Started](#getting-started)
- [Self-hosted Plugins and Themes](#self-hosted-plugins-and-themes)
- [How to Release an Update](#how-to-release-an-update)
- [Notes](#notes)
- [GitHub Integration](#github-integration)
- [How to Release an Update](#how-to-release-an-update-1)
- [Notes](#notes-1)
- [BitBucket Integration](#bitbucket-integration)
- [How to Release an Update](#how-to-release-an-update-2)
- [GitLab Integration](#gitlab-integration)
- [How to Release an Update](#how-to-release-an-update-3)
- [License Management](#license-management)
- [Resources](#resources)
<!-- END doctoc generated TOC please keep comment here to allow auto update -->
Getting Started
---------------
### Self-hosted Plugins and Themes
1. Download [the latest release](https://github.com/YahnisElsts/plugin-update-checker/releases/latest) and copy the `plugin-update-checker` directory to your plugin or theme.
2. Go to the `examples` subdirectory and open the .json file that fits your project type. Replace the placeholder data with your plugin/theme details.
- Plugin example:
```json
{
"name" : "Plugin Name",
"version" : "2.0",
"download_url" : "http://example.com/plugin-name-2.0.zip",
"sections" : {
"description" : "Plugin description here. You can use HTML."
}
}
```
This is a minimal example that leaves out optional fields. See [this table](https://docs.google.com/spreadsheets/d/1eOBbW7Go2qEQXReOOCdidMTf_tDYRq4JfegcO1CBPIs/edit?usp=sharing) for a full list of supported fields and their descriptions.
- Theme example:
```json
{
"version": "2.0",
"details_url": "http://example.com/version-2.0-details.html",
"download_url": "http://example.com/example-theme-2.0.zip"
}
```
This is actually a complete example that shows all theme-related fields. `version` and `download_url` should be self-explanatory. The `details_url` key specifies the page that the user will see if they click the "View version 1.2.3 details" link in an update notification.
3. Upload the JSON file to a publicly accessible location.
4. Add the following code to the main plugin file or to the `functions.php` file:
```php
require 'path/to/plugin-update-checker/plugin-update-checker.php';
$myUpdateChecker = Puc_v4_Factory::buildUpdateChecker(
'http://example.com/path/to/details.json',
__FILE__, //Full path to the main plugin file or functions.php.
'unique-plugin-or-theme-slug'
);
```
Note: If you're using the Composer autoloader, you don't need to explicitly `require` the library.
#### How to Release an Update
Change the `version` number in the JSON file and make sure that `download_url` points to the latest version. Update the other fields if necessary. Tip: You can use [wp-update-server](https://github.com/YahnisElsts/wp-update-server) to automate this process.
By default, the library will check the specified URL for changes every 12 hours. You can force it to check immediately by clicking the "Check for updates" link on the "Plugins" page (it's next to the "Visit plugin site" link). Themes don't have that link, but you can also trigger an update check like this:
1. Install [Debug Bar](https://srd.wordpress.org/plugins/debug-bar/).
2. Click the "Debug" menu in the Admin Bar (a.k.a Toolbar).
3. Open the "PUC (your-slug)" panel.
4. Click the "Check Now" button.
#### Notes
- The second argument passed to `buildUpdateChecker` must be the absolute path to the main plugin file or any file in the theme directory. If you followed the "getting started" instructions, you can just use the `__FILE__` constant.
- The third argument - i.e. the slug - is optional but recommended. In most cases, the slug should be the same as the name of your plugin directory. For example, if your plugin lives in `/wp-content/plugins/my-plugin`, set the slug to `my-plugin`. If the slug is omitted, the update checker will use the name of the main plugin file as the slug (e.g. `my-cool-plugin.php` → `my-cool-plugin`). This can lead to conflicts if your plugin has a generic file name like `plugin.php`.
This doesn't affect themes because PUC uses the theme directory name as the default slug. Still, if you're planning to use the slug in your own code - e.g. to filter updates or override update checker behaviour - it can be a good idea to set it explicitly.
### GitHub Integration
1. Download [the latest release](https://github.com/YahnisElsts/plugin-update-checker/releases/latest) and copy the `plugin-update-checker` directory to your plugin or theme.
2. Add the following code to the main plugin file or `functions.php`:
```php
require 'plugin-update-checker/plugin-update-checker.php';
$myUpdateChecker = Puc_v4_Factory::buildUpdateChecker(
'https://github.com/user-name/repo-name/',
__FILE__,
'unique-plugin-or-theme-slug'
);
//Optional: If you're using a private repository, specify the access token like this:
$myUpdateChecker->setAuthentication('your-token-here');
//Optional: Set the branch that contains the stable release.
$myUpdateChecker->setBranch('stable-branch-name');
```
3. Plugins only: Add a `readme.txt` file formatted according to the [WordPress.org plugin readme standard](https://wordpress.org/plugins/readme.txt) to your repository. The contents of this file will be shown when the user clicks the "View version 1.2.3 details" link.
#### How to Release an Update
This library supports a couple of different ways to release updates on GitHub. Pick the one that best fits your workflow.
- **GitHub releases**
Create a new release using the "Releases" feature on GitHub. The tag name and release title don't matter. The description is optional, but if you do provide one, it will be displayed when the user clicks the "View version x.y.z details" link on the "Plugins" page. Note that PUC ignores releases marked as "This is a pre-release".
If you want to use release assets, call the `enableReleaseAssets()` method after creating the update checker instance:
```php
$myUpdateChecker->getVcsApi()->enableReleaseAssets();
```
- **Tags**
To release version 1.2.3, create a new Git tag named `v1.2.3` or `1.2.3`. That's it.
PUC doesn't require strict adherence to [SemVer](http://semver.org/). These are all valid tag names: `v1.2.3`, `v1.2-foo`, `1.2.3_rc1-ABC`, `1.2.3.4.5`. However, be warned that it's not smart enough to filter out alpha/beta/RC versions. If that's a problem, you might want to use GitHub releases or branches instead.
- **Stable branch**
Point the update checker at a stable, production-ready branch:
```php
$updateChecker->setBranch('branch-name');
```
PUC will periodically check the `Version` header in the main plugin file or `style.css` and display a notification if it's greater than the installed version.
Caveat: If you set the branch to `master` (the default), the update checker will look for recent releases and tags first. It'll only use the `master` branch if it doesn't find anything else suitable.
#### Notes
The library will pull update details from the following parts of a
没有合适的资源?快使用搜索试试~ 我知道了~
【WordPress主题】2022年最新版完整功能demo+插件v1.0.2.zip
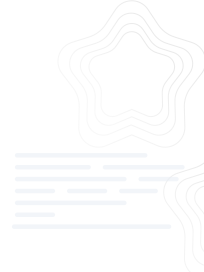
共270个文件
php:72个
js:44个
css:30个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 70 浏览量
2022-03-30
13:58:39
上传
评论
收藏 6.22MB ZIP 举报
温馨提示
"【WordPress主题】2022年最新版完整功能demo+插件v1.0.2 PAO - OnePage WordPress PAO - 单页" ---------- 泰森云每天更新发布最新WordPress主题、HTML主题、WordPress插件、shopify主题、opencart主题、PHP项目源码、安卓项目源码、ios项目源码,更有超10000个资源可供选择,如有需要请站内联系。
资源推荐
资源详情
资源评论
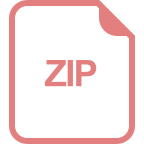
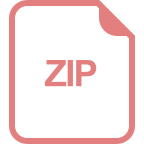
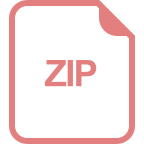
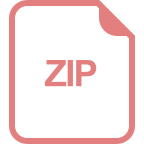
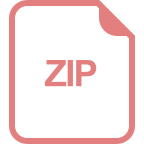
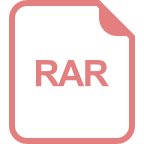
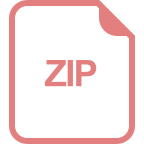
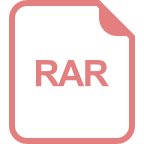
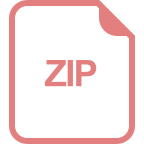
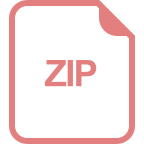
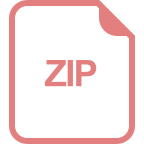
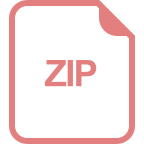
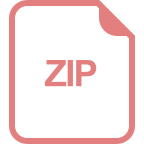
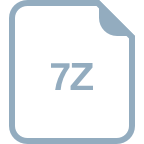
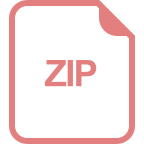
收起资源包目录

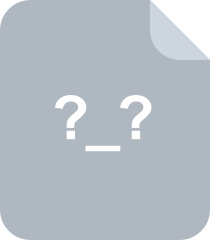
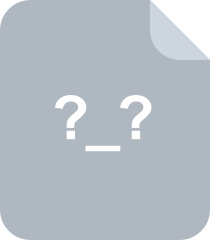
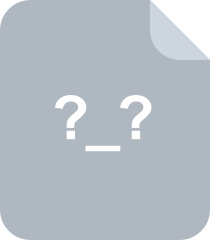
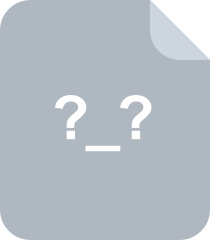
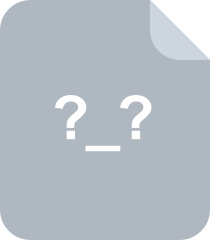
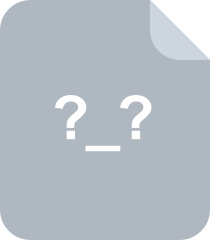
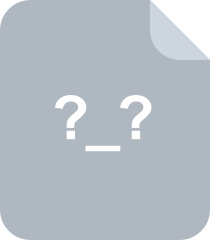
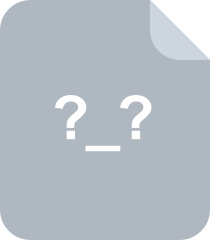
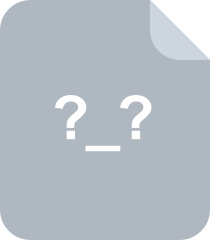
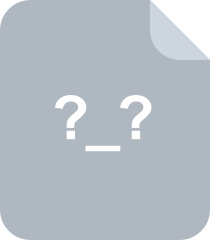
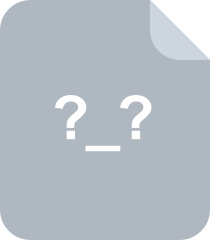
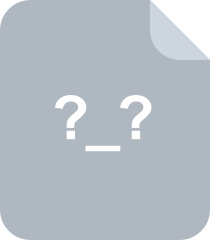
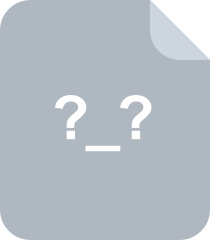
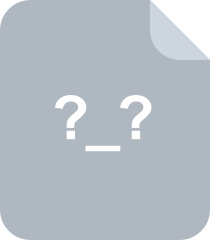
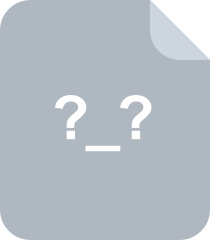
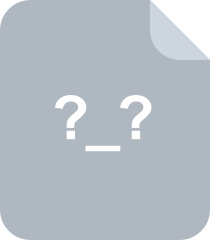
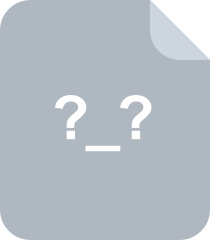
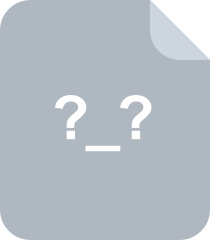
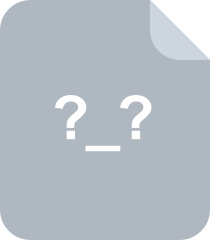
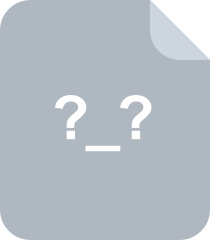
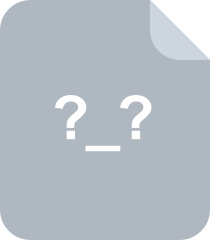
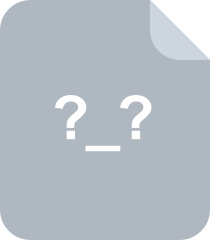
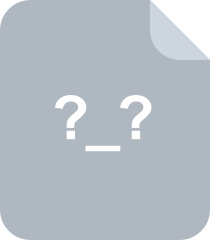
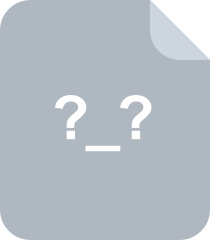
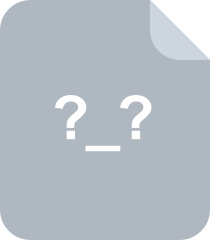
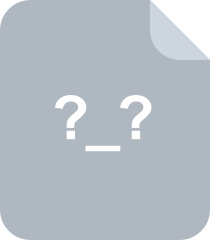
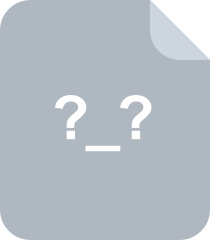
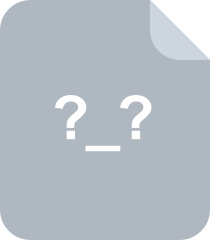
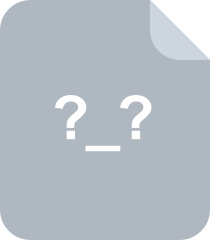
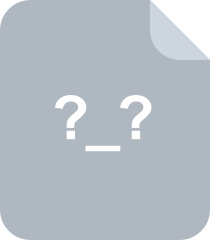
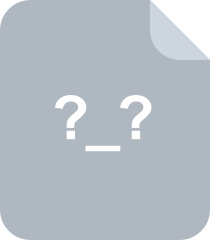
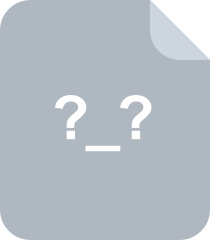
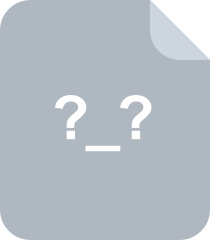
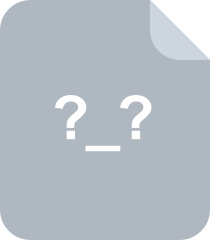
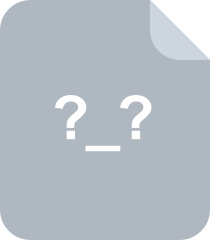
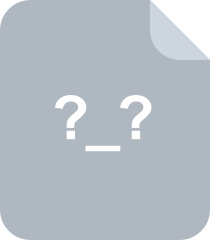
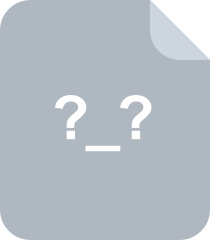
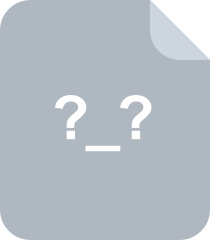
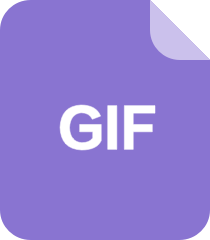
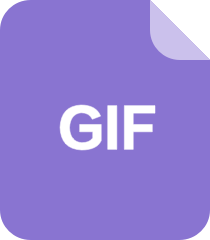
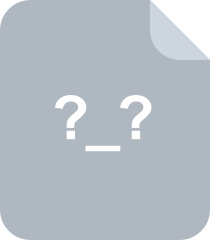
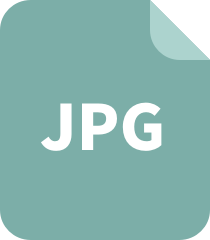
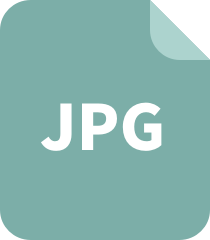
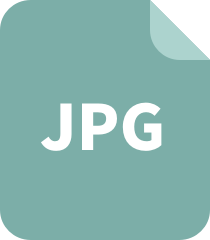
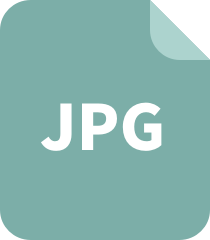
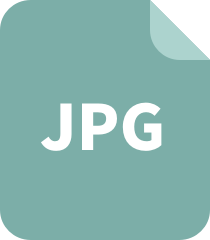
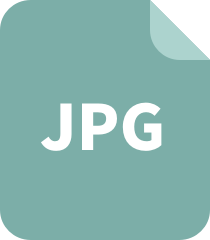
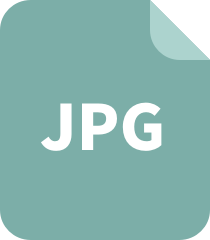
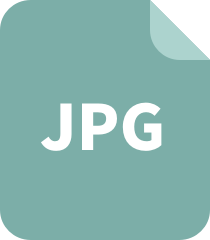
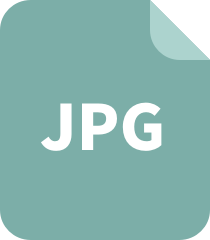
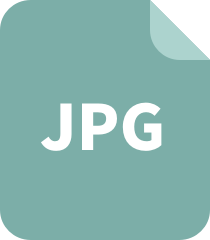
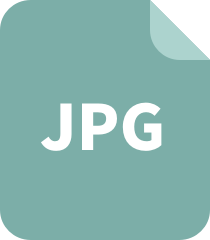
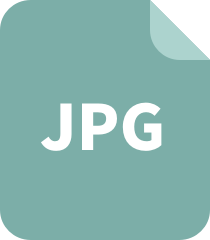
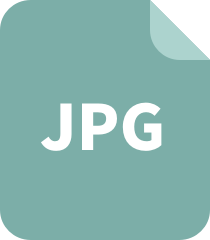
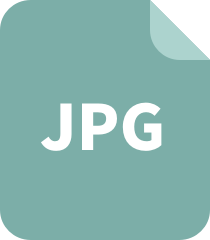
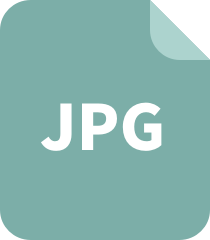
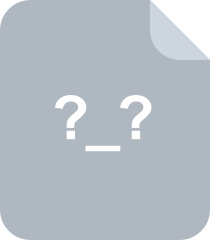
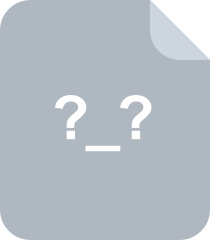
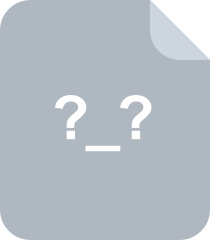
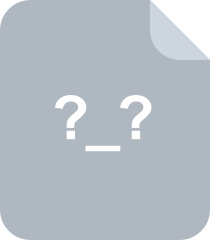
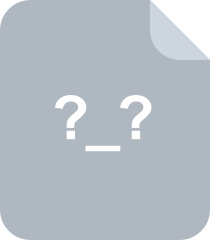
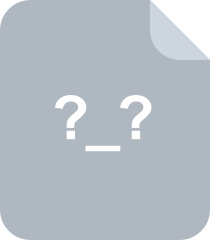
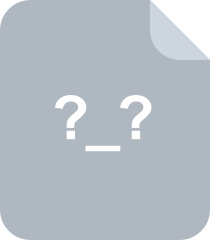
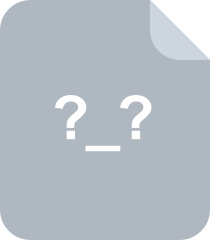
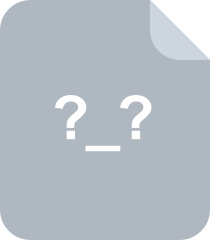
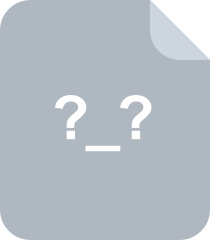
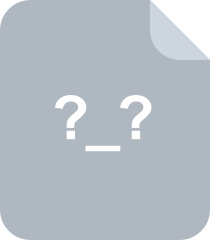
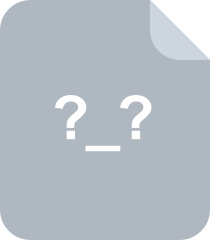
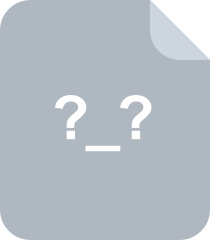
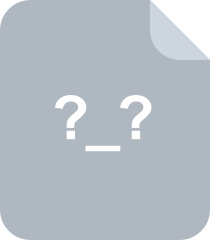
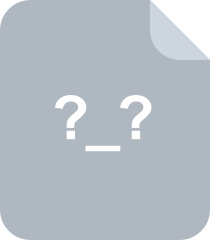
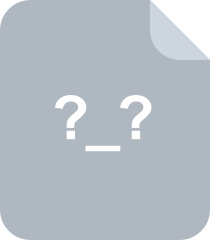
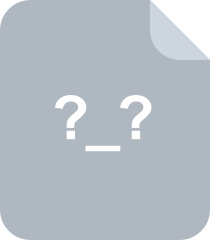
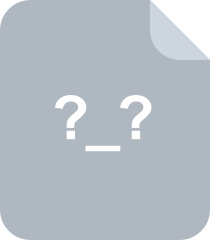
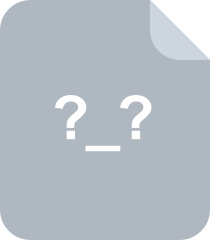
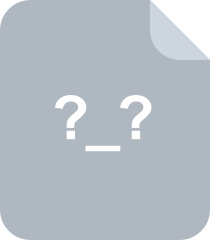
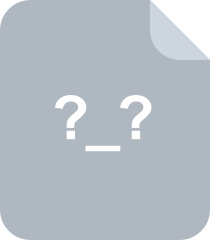
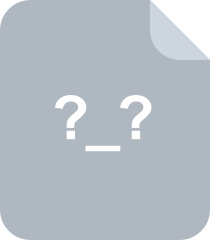
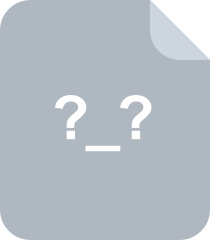
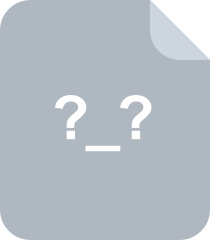
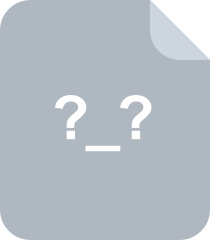
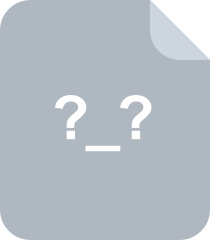
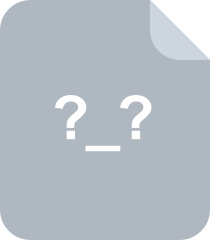
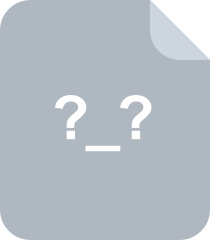
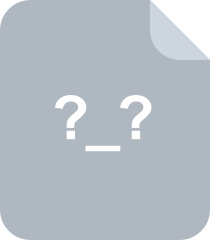
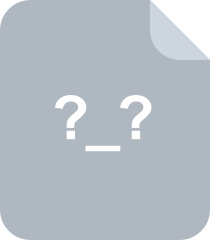
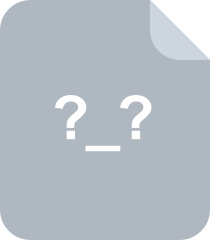
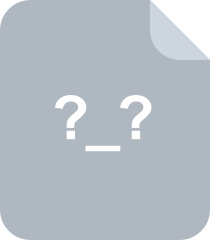
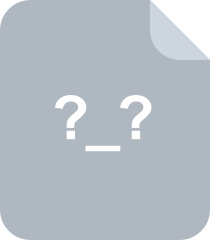
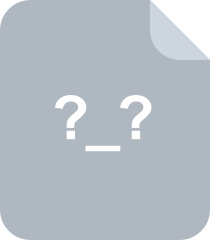
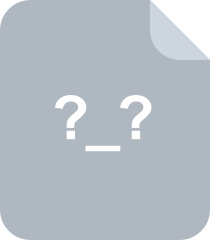
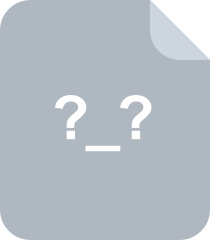
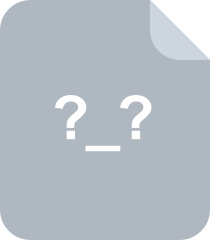
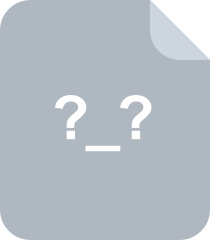
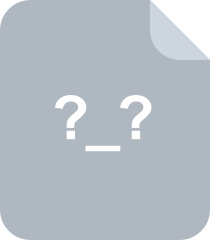
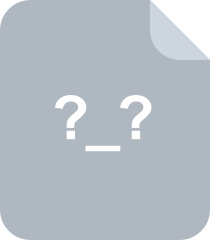
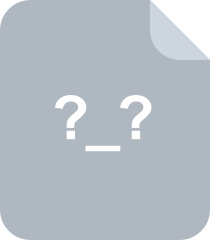
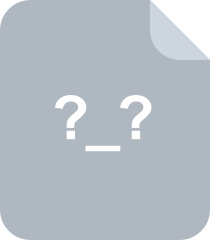
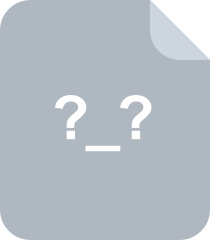
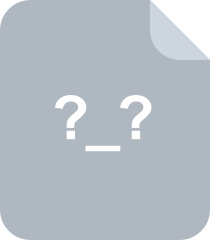
共 270 条
- 1
- 2
- 3
资源评论
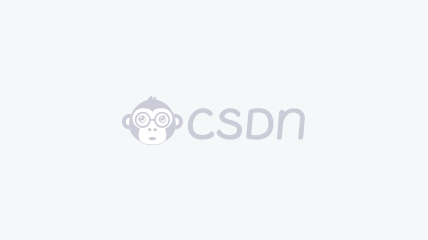

Lee达森
- 粉丝: 966
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

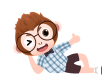
安全验证
文档复制为VIP权益,开通VIP直接复制
