<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
body{
background-color: rgb(235, 235, 235);
overflow: hidden;
width: 100%;
height: 100%;
}
#cj{
position: absolute;
top: 2%;
left: 3%;
color: blueviolet;
background-color: rgb(99, 178, 247);
}
#cj1{
position: absolute;
top: 2%;
left: 8%;
color: blueviolet;
background-color: rgb(99, 178, 247);
}
#cj2{
position: absolute;
top: 2%;
left: 13%;
color: blueviolet;
background-color: rgb(99, 178, 247);
}
</style>
</head>
<body>
<div id="cj">场景1</div>
<div id="cj1">场景2</div>
<div id="cj2">场景3</div>
<script src="../build/jquery-3.6.0.js"></script>
<script src="../build/Tween.js"></script>
<script type="module">
import * as THREE from '../build/three.module1.js';
import {OrbitControls} from './jsm/controls/OrbitControls.js';
let scene , camera , ambientLight , renderer , controls , axesHelper , geometry , material , color1 , color2 , color3 , Mesh , vertices
let faces , plane , planegeometry , planematerial , planegeometry2 , planematerial2 , plane2 , planegeometry1 , planematerial1 , plane1
let Geometry3 , texture3 , materials3 , mesh3 , s = 0 , p = 1
let scene2 , camera2 , SceneShow = 1
let texture1 , texture2 , texture4 , scene4 , planegeometry4 , planematerial4 , plane4
function initScene(){
scene = new THREE.Scene();
texture1 = new THREE.TextureLoader().load("textures/kandao3.jpg")
scene.background = texture1
axesHelper = new THREE.AxesHelper( 1 );
scene.add( axesHelper );
}
function initCamera(){
camera = new THREE.PerspectiveCamera( 60 , window.innerWidth / window.innerHeight , 1 , 1000)
camera.position.set(0,0,10);
camera.lookAt(new THREE.Vector3(0,0,0));
camera.up.y = 1;
}
function initLight(){
ambientLight = new THREE.AmbientLight(0xffffff , 1.0)
scene.add(ambientLight);
}
function initRender(){
renderer = new THREE.WebGLRenderer({antialias:true, alpha:true, side:THREE.DoubleSide});
renderer.setSize(window.innerWidth , window.innerHeight);
document.body.appendChild(renderer.domElement);
}
function initControls(){
controls = new OrbitControls(camera, renderer.domElement)
}
function update(){
controls.update();
}
function onWindowResize(){
camera.aspect = window.innerWidth / window.innerHeight;
camera.updateProjectionMatrix();
renderer.setSize(window.innerWidth , window.innerHeight);
}
function initObject(){
geometry = new THREE.BufferGeometry();
vertices = new Float32Array([
0 , 2 , 0 ,
-2 , 0 , 0 ,
2 , 0 , 0 ,
0 , 2 , 0
])
color1 = new THREE.Color(0x334499);
color2 = new THREE.Color(0x224433);
color3 = new THREE.Color(0xff2288);
geometry.setAttribute('position', new THREE.BufferAttribute(vertices , 3));
material = new THREE.MeshBasicMaterial({color : 0x33ff99});
var Mesh = new THREE.Line(geometry , material );
scene.add(Mesh);
}
function CreatePlane(){
planegeometry = new THREE.BoxGeometry(1,1,1);
planematerial = new THREE.MeshBasicMaterial({
color : 0x335599,
side : THREE.DoubleSide
})
plane = new THREE.Mesh(planegeometry , planematerial);
scene.add(plane);
planegeometry1 = new THREE.BoxGeometry(1,1,1);
planematerial1 = new THREE.MeshBasicMaterial({
color : 0x33ff99,
side : THREE.DoubleSide
})
plane1 = new THREE.Mesh(planegeometry , planematerial);
plane1.position.set(2,2,0)
scene.add(plane1);
console.log(plane1)
console.log(plane.matrix);
//目前的矩阵数据 matrix--- Matrix4 [1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1]
// plane.position.set(15 , 8 ,-10);
// console.log(plane.matrix);
//平移之后的矩阵数据 matrix---Matrix4 [1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 15, 8, -10, 1]
// plane.rotation.x = THREE.Math.degToRad(30);
// console.log(plane.matrix); // [1, 0, 0, 0, 0, 0.8660254037844387, 0.49999999999999994, 0, 0, -0.49999999999999994, 0.8660254037844387, 0, 15, 8, -10, 1]
}
function CreatePlane2(){
scene2 = new THREE.Scene();
texture2 = new THREE.TextureLoader().load("textures/Up.jpg")
scene2.background = texture2
planegeometry2 = new THREE.BoxGeometry(1,1,1);
planematerial2 = new THREE.MeshBasicMaterial({
color : 0x99ff66,
side : THREE.DoubleSide
})
plane2 = new THREE.Mesh(planegeometry2 , planematerial2);
plane2.position.set(0,0,0)
scene2.add(plane2)
// controls2 = new OrbitControls(camera, renderer.domElement)
}
function CreatePlane4(){
scene4 = new THREE.Scene();
texture4 = new THREE.TextureLoader().load("textures/sprite.png")
scene4.background = texture4
planegeometry4 = new THREE.BoxGeometry(1,1,1);
planematerial4 = new THREE.MeshBasicMaterial({
color : 0x9988ff,
side : THREE.DoubleSide
})
plane4 = new THREE.Mesh(planegeometry4 , planematerial4);
plane4.position.set(0,0,0)
scene4.add(plane4)
// controls2 = new OrbitControls(camera, renderer.domElement)
}
function Switch(){
$('#cj').click(function(){
SceneShow = 1
// camera.position.set(1,1,1)
// if(SceneShow === false){
// var tween = new TWEEN.Tween(camera.position)
// .to({x:0,y:0,z:10},600)
// .start();
// }else{
// // plane2.rotation.y = Math.PI
// var tween = new TWEEN.Tween(camera.position)
// .to({x:1,y:1,z:1},2000)
// .start();
// // camera.position.set(1,1,1)
// }
})
$('#cj1').click(function(){
SceneShow = 2
})
$('#cj2').click(function(){
SceneShow = 3
})
}
function init(){
initScene();
initCamera();
initLight();
initRender();
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
threejs切换场景 实现图朴上的切换效果, 而不是相机的视角移动,不浪费性能,非常棒的效果,代码简单,实现思路简单,适合新手开发项目中,遇到当前视角切换到模型内部 等效果 , threejs scene的切换 , 视角的移动会导致页面不是很流畅,而且旋转也会有点小bug 直接切换场景,就不会存在这种的问题,值得收藏,学不会找博主 私信 看到了会回复
资源详情
资源评论
资源推荐
收起资源包目录

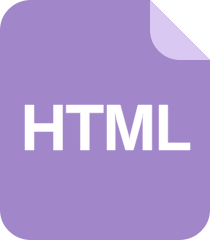
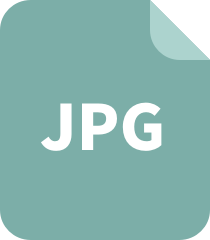
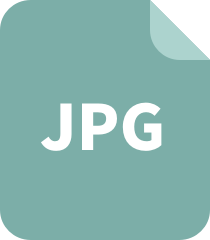
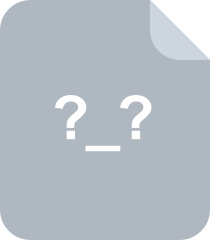
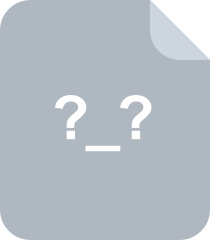
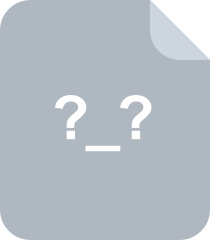
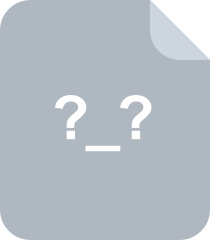
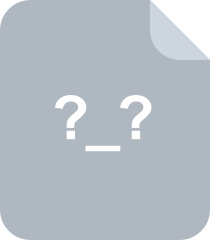
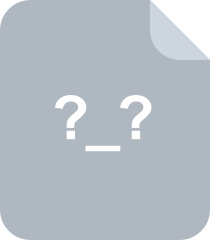
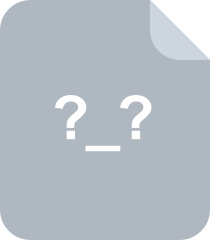
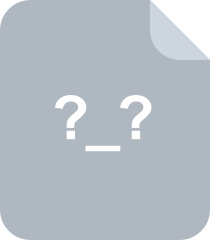
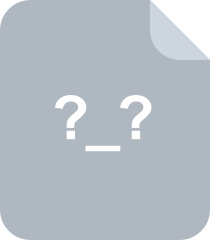
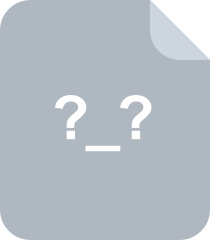
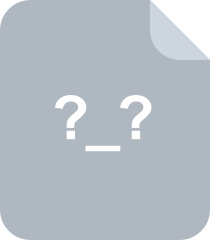
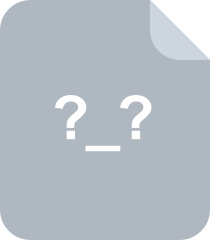
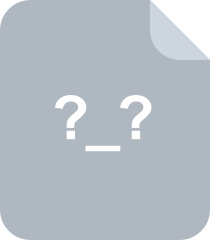
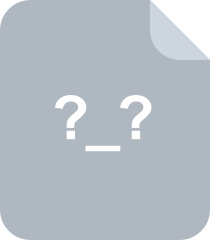
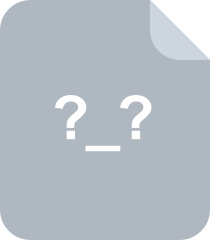
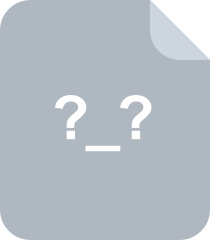
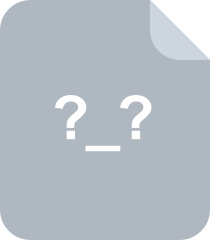
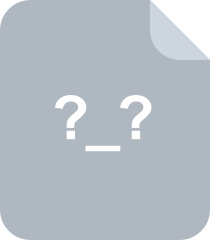
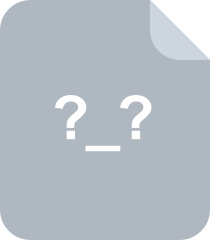
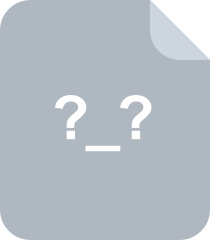
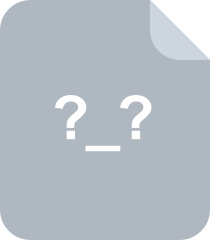
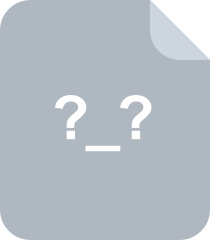
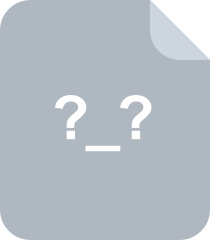
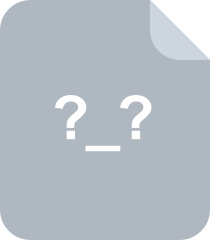
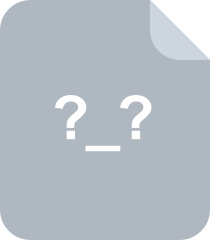
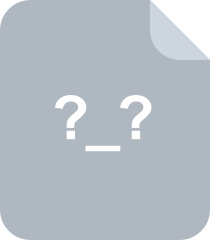
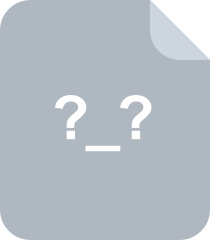
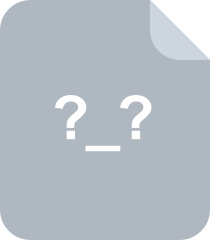
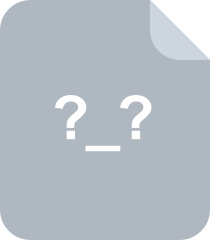
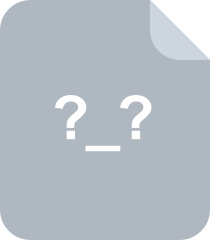
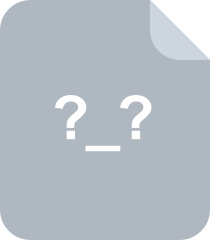
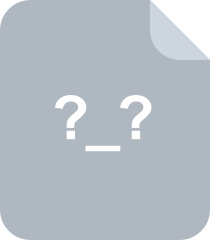
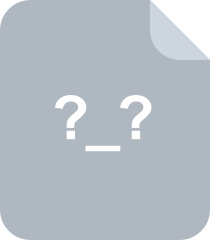
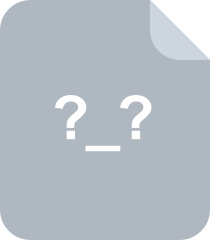
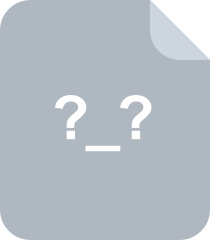
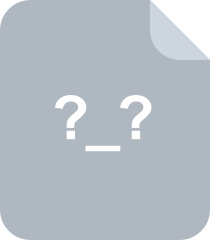
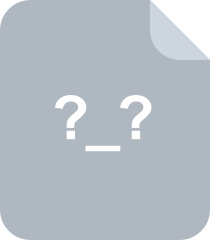
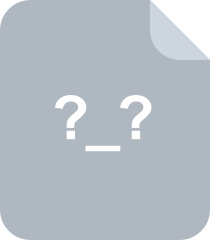
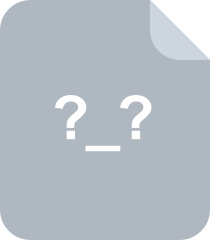
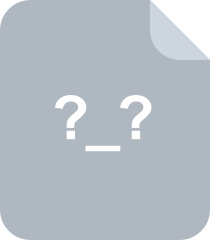
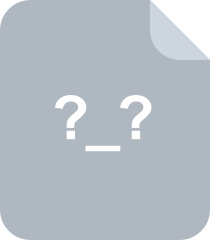
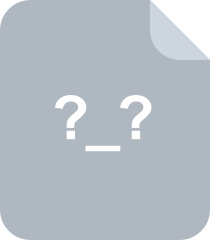
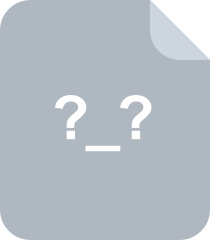
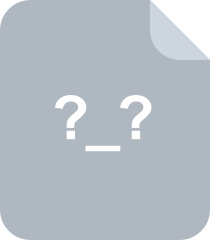
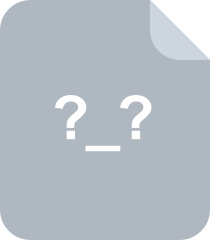
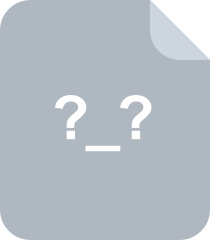
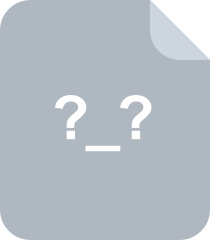
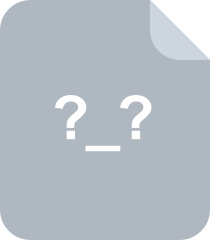
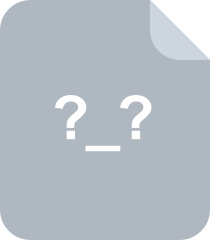
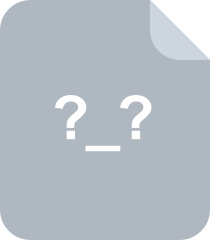
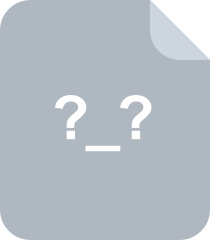
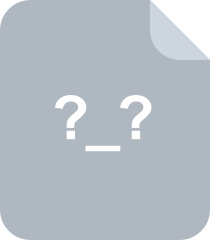
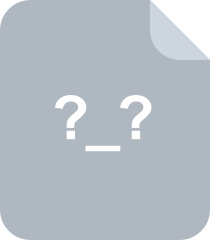
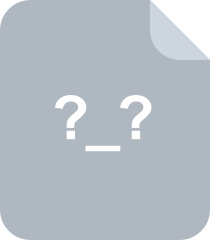
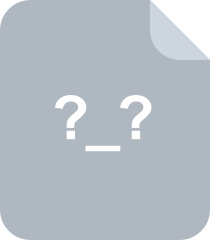
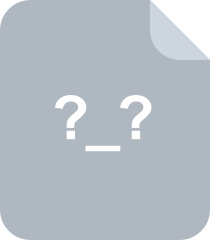
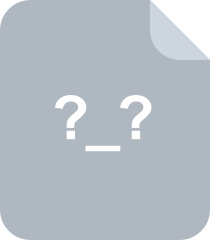
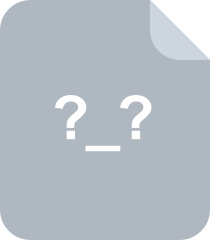
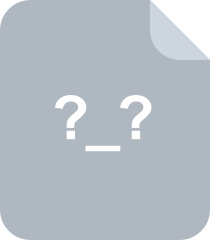
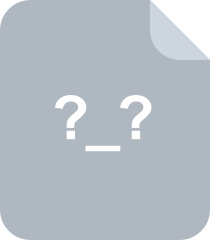
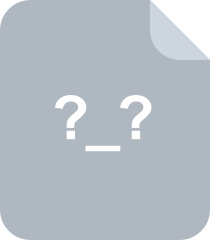
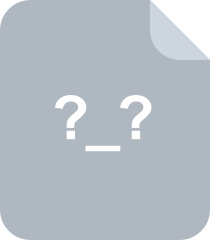
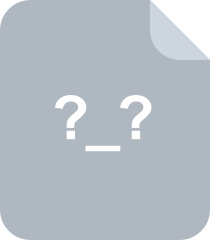
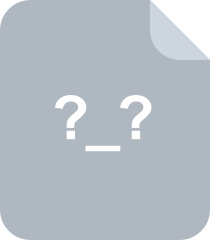
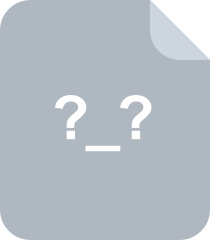
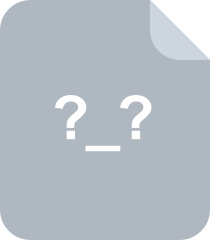
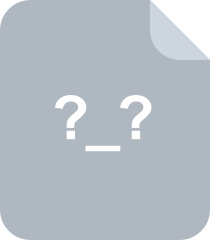
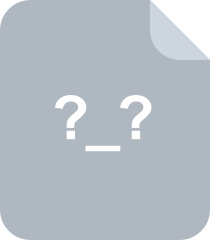
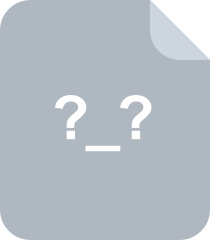
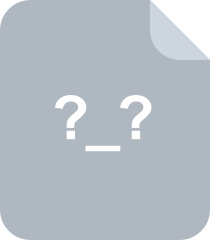
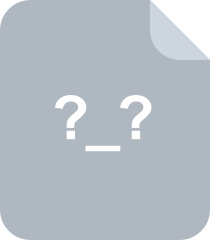
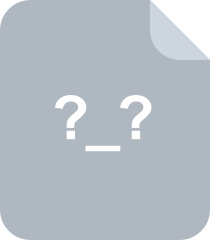
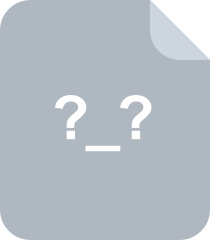
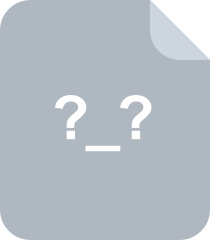
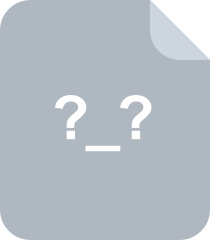
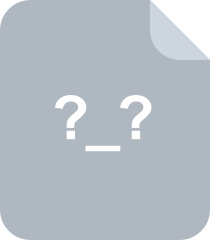
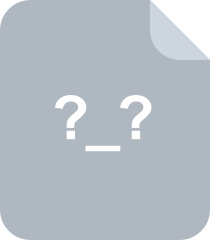
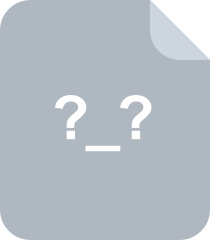
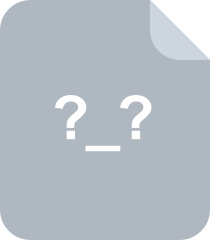
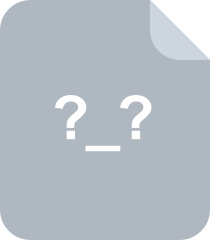
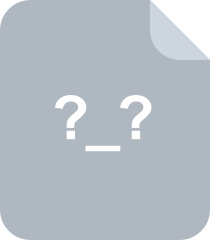
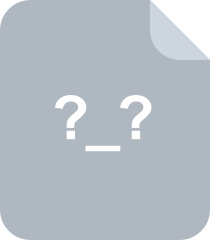
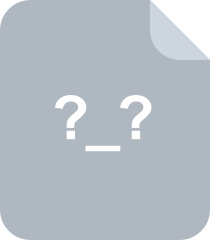
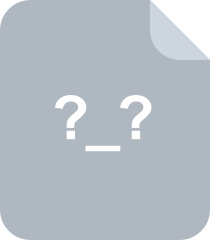
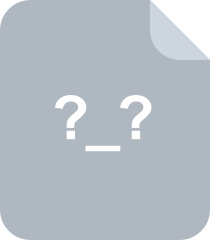
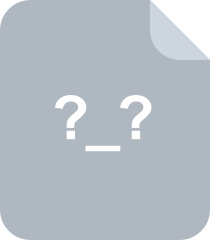
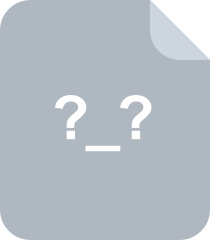
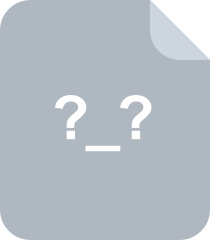
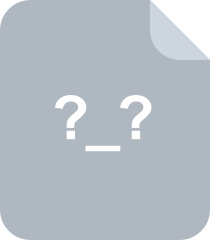
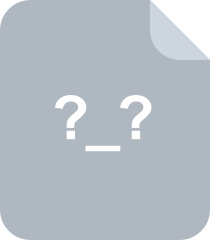
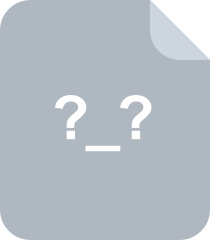
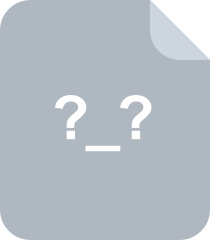
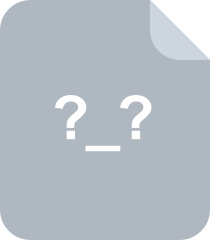
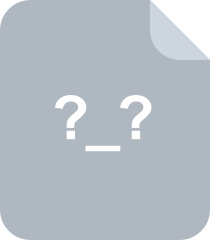
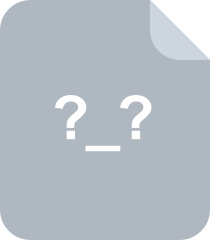
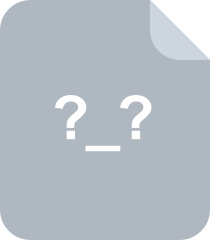
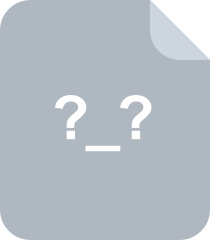
共 466 条
- 1
- 2
- 3
- 4
- 5
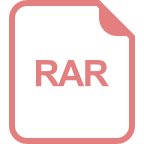
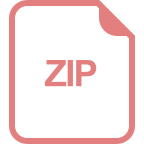
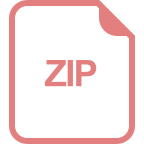
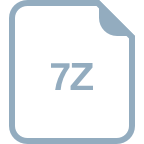
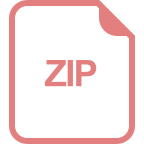
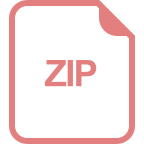
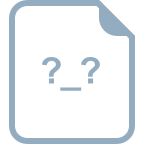
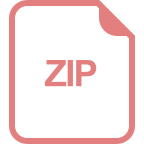
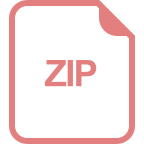
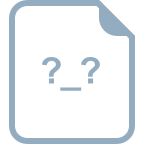
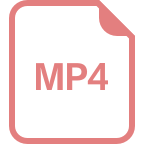
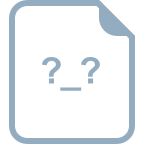

Zombie391
- 粉丝: 62
- 资源: 43
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

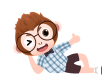
最新资源
- 打包和分发Rust工具.pdf
- SQL中的CREATE LOGFILE GROUP 语句.pdf
- C语言-leetcode题解之第172题阶乘后的零.zip
- C语言-leetcode题解之第171题Excel列表序号.zip
- C语言-leetcode题解之第169题多数元素.zip
- ocr-图像识别资源ocr-图像识别资源
- 图像识别:基于Resnet50 + VGG16模型融合的人体细胞癌症分类模型实现-图像识别资源
- C语言-leetcode题解之第168题Excel列表名称.zip
- C语言-leetcode题解之第167题两数之和II-输入有序数组.zip
- C语言-leetcode题解之第166题分数到小数.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


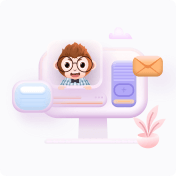
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0