没有合适的资源?快使用搜索试试~ 我知道了~
salesforce_visualforce_developer_cheatsheet
需积分: 5 2 下载量 17 浏览量
2012-09-10
15:49:34
上传
评论
收藏 852KB PDF 举报
温馨提示
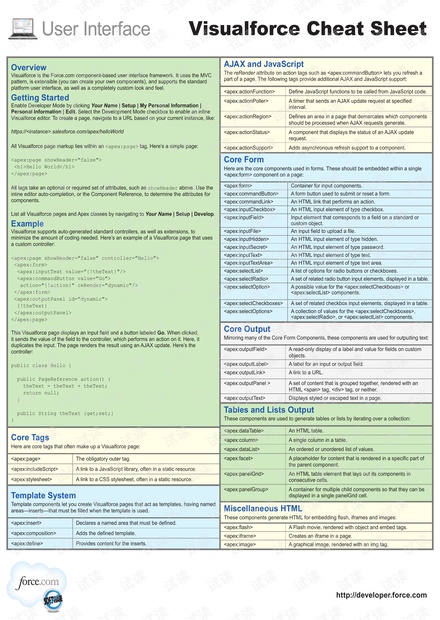

试读
2页
Salesforce官方的visualforce cheat sheet,对于salesforce的开发人员很有用.
资源推荐
资源详情
资源评论
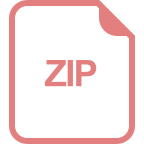
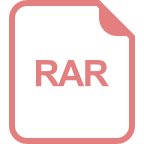
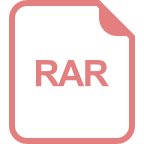
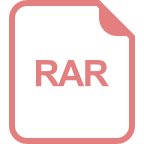
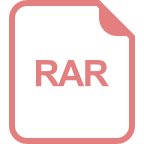
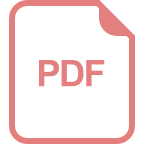
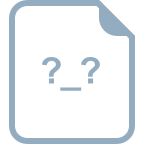
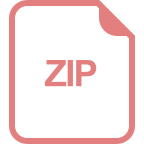
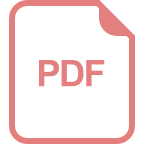
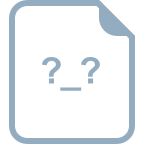
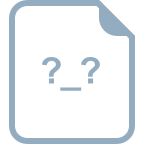
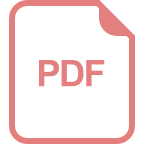
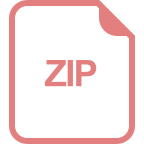
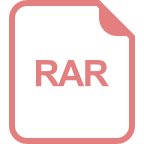
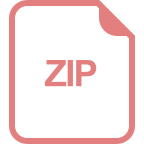
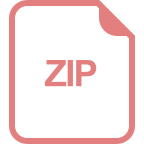
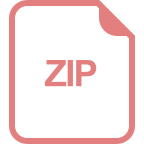
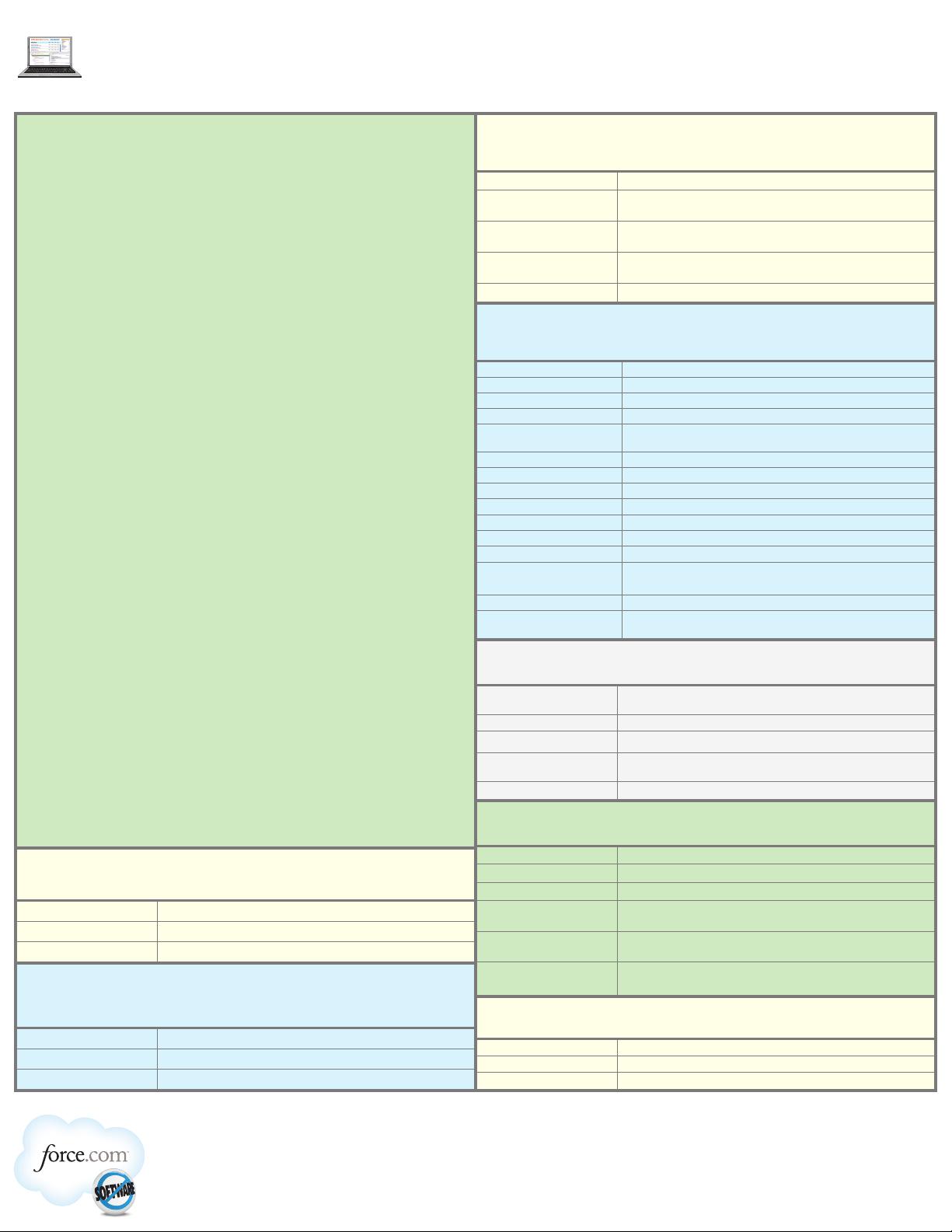
Visualforce Cheat Sheet
User Interface
http://developer.force.com
Overview
Visualforce is the Force.com component-based user interface framework. It uses the MVC
pattern, is extensible (you can create your own components), and supports the standard
platform user interface, as well as a completely custom look and feel.
Getting Started
Enable Developer Mode by clicking Your Name | Setup | My Personal Information |
Personal Information | Edit. Select the Development Mode checkbox to enable an inline
Visualforce editor. To create a page, navigate to a URL based on your current instance, like:
https://<instance>.salesforce.com/apex/helloWorld
All Visualforce page markup lies within an <apex:page> tag. Here’s a simple page:
<apex:page showHeader="false">
<h1>Hello World</h1>
</apex:page>
All tags take an optional or required set of attributes, such as showHeader above. Use the
inline editor auto-completion, or the Component Reference, to determine the attributes for
components.
List all Visualforce pages and Apex classes by navigating to Your Name | Setup | Develop.
Example
Visualforce supports auto-generated standard controllers, as well as extensions, to
minimize the amount of coding needed. Here’s an example of a Visualforce page that uses
a custom controller:
<apex:page showHeader="false" controller="Hello">
<apex:form>
<apex:inputText value="{!theText}"/>
<apex:commandButton value="Go">
action="{!action}" reRender="dynamic"/>
</apex:form>
<apex:outputPanel id="dynamic">
{!theText}
</apex:outputPanel>
</apex:page>
This Visualforce page displays an input field and a button labeled Go. When clicked,
it sends the value of the field to the controller, which performs an action on it. Here, it
duplicates the input. The page renders the result using an AJAX update. Here’s the
controller:
public class Hello {
public PageReference action() {
theText = theText + theText;
return null;
}
public String theText {get;set;}
}
Core Tags
Here are core tags that often make up a Visualforce page:
<apex:page> The obligatory outer tag.
<apex:includeScript> A link to a JavaScript library, often in a static resource.
<apex:stylesheet> A link to a CSS stylesheet, often in a static resource.
Template System
Template components let you create Visualforce pages that act as templates, having named
areas—inserts—that must be filled when the template is used.
<apex:insert> Declares a named area that must be defined.
<apex:composition> Adds the defined template.
<apex:define> Provides content for the inserts.
AJAX and JavaScript
The reRender attribute on action tags such as <apex:commandButton> lets you refresh a
part of a page. The following tags provide additional AJAX and JavaScript support:
<apex:actionFunction> Define JavaScript functions to be called from JavaScript code.
<apex:actionPoller> A timer that sends an AJAX update request at specified
interval.
<apex:actionRegion> Defines an area in a page that demarcates which components
should be processed when AJAX requests generate.
<apex:actionStatus> A component that displays the status of an AJAX update
request.
<apex:actionSupport> Adds asynchronous refresh support to a component.
Core Form
Here are the core components used in forms. These should be embedded within a single
<apex:form> component on a page:
<apex:form> Container for input components.
<apex:commandButton> A form button used to submit or reset a form.
<apex:commandLink> An HTML link that performs an action.
<apex:inputCheckbox> An HTML input element of type checkbox.
<apex:inputField> Input element that corresponds to a field on a standard or
custom object.
<apex:inputFile> An input field to upload a file.
<apex:inputHidden> An HTML input element of type hidden.
<apex:inputSecret> An HTML input element of type password.
<apex:inputText> An HTML input element of type text.
<apex:inputTextArea> An HTML input element of type text area.
<apex:selectList> A list of options for radio buttons or checkboxes.
<apex:selectRadio> A set of related radio button input elements, displayed in a table.
<apex:selectOption> A possible value for the <apex:selectCheckboxes> or
<apex:selectList> components.
<apex:selectCheckboxes> A set of related checkbox input elements, displayed in a table.
<apex:selectOptions> A collection of values for the <apex:selectCheckboxes>,
<apex:selectRadio>, or <apex:selectList> components.
Core Output
Mirroring many of the Core Form Components, these components are used for outputting text:
<apex:outputField> A read-only display of a label and value for fields on custom
objects.
<apex:outputLabel> A label for an input or output field.
<apex:outputLink> A link to a URL.
<apex:outputPanel > A set of content that is grouped together, rendered with an
HTML <span> tag, <div> tag, or neither.
<apex:outputText> Displays styled or escaped text in a page.
Tables and Lists Output
These components are used to generate tables or lists by iterating over a collection:
<apex:dataTable> An HTML table.
<apex:column> A single column in a table.
<apex:dataList> An ordered or unordered list of values.
<apex:facet> A placeholder for content that is rendered in a specific part of
the parent component.
<apex:panelGrid> An HTML table element that lays out its components in
consecutive cells.
<apex:panelGroup> A container for multiple child components so that they can be
displayed in a single panelGrid cell.
Miscellaneous HTML
These components generate HTML for embedding flash, iframes and images:
<apex:flash> A Flash movie, rendered with object and embed tags.
<apex:iframe> Creates an iframe in a page.
<apex:image> A graphical image, rendered with an img tag.
资源评论
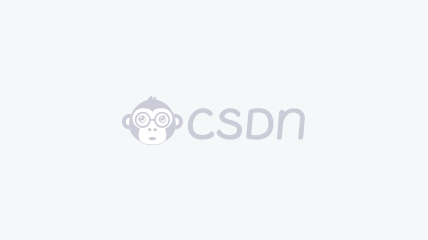

swonizuka
- 粉丝: 6
- 资源: 8
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

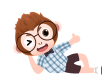
最新资源
- 农村信用社联合社计算机信息系统投产与变更管理办.docx
- 农村信用社联合社计算机信息系统数据管理办法.docx
- 利用SPSS作临床效度分析线上计算网站介绍-医学研究部统计谘.(医学PPT课件).ppt
- 利用Zabbix监控mysqldump定时备份数据库状态.docx
- 利用计算机解决问题的基本过程.doc
- 化工铁路通信工程总结.doc
- 北京大学网络教育软件工程作业.docx
- 医药公司(连锁店)计算机操作规程未新系统的自行按照旧制修改-新系统过制的编号加修模版.doc
- 医药公司(连锁店)计算机系统操作规程模版.doc
- 医药连锁门店计算机系统的操作和管理程序未新系统的自行按照旧制修改-新系统过制的编号加修模版.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


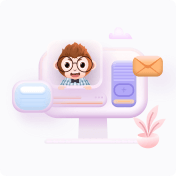
安全验证
文档复制为VIP权益,开通VIP直接复制
