在Windows Forms(WinForm)应用开发中,Listbox控件是一种常见的用户界面元素,用于显示一系列可选择的项目。在某些交互设计中,允许用户通过拖放操作将Listbox中的条目从一个列表移动到另一个列表是提高用户体验的有效方式。本篇文章将详细探讨如何在C#环境下实现这一功能。 我们需要创建两个Listbox控件,分别命名为ListBox1和ListBox2,然后开启它们的AllowDrop属性,使得用户可以将一项拖放到另一项上。在C#代码中,这可以通过以下设置实现: ```csharp ListBox1.AllowDrop = true; ListBox2.AllowDrop = true; ``` 接下来,我们需要监听DragEnter和DragDrop事件,这两个事件在用户开始拖动和实际放置项目时触发。在DragEnter事件处理程序中,我们需要检查拖动的数据是否是文本类型,并设置Effect为Copy或Move,表示拖放操作的类型。在DragDrop事件处理程序中,我们执行实际的移动或复制操作。 ```csharp private void ListBox1_DragEnter(object sender, DragEventArgs e) { if (e.Data.GetDataPresent(DataFormats.Text)) e.Effect = DragDropEffects.Copy; // 或者使用 DragDropEffects.Move } private void ListBox1_DragDrop(object sender, DragEventArgs e) { string item = e.Data.GetData(DataFormats.Text) as string; ListBox1.Items.Remove(item); ListBox2.Items.Add(item); } ``` 这里,我们假设Listbox中的每一项都是字符串类型。如果Listbox中存储的是自定义对象,我们需要在DragDrop事件中处理对象的序列化和反序列化。同时,DragDropEffects.Copy表示复制操作,而DragDropEffects.Move表示移动操作。如果希望在拖放后从源Listbox中移除项目,应使用Move。 为了使从ListBox2拖放到ListBox1也生效,我们需要为ListBox2添加相同的事件处理程序: ```csharp private void ListBox2_DragEnter(object sender, DragEventArgs e) { if (e.Data.GetDataPresent(DataFormats.Text)) e.Effect = DragDropEffects.Copy; // 或者使用 DragDropEffects.Move } private void ListBox2_DragDrop(object sender, DragEventArgs e) { string item = e.Data.GetData(DataFormats.Text) as string; ListBox2.Items.Remove(item); ListBox1.Items.Add(item); } ``` 除此之外,还可以增加选中项的拖放支持。在ListBox的MouseDown和MouseUp事件中,我们可以检测是否有鼠标按键被按下和释放,并在按下时开始拖动操作。例如: ```csharp private void ListBox1_MouseDown(object sender, MouseEventArgs e) { if (e.Button == MouseButtons.Left && ListBox1.SelectedItem != null) { string selectedText = ListBox1.SelectedItem.ToString(); DataObject data = new DataObject(DataFormats.Text, selectedText); ListBox1.DoDragDrop(data, DragDropEffects.Copy | DragDropEffects.Move); } } private void ListBox2_MouseDown(object sender, MouseEventArgs e) { if (e.Button == MouseButtons.Left && ListBox2.SelectedItem != null) { string selectedText = ListBox2.SelectedItem.ToString(); DataObject data = new DataObject(DataFormats.Text, selectedText); ListBox2.DoDragDrop(data, DragDropEffects.Copy | DragDropEffects.Move); } } ``` 不要忘记在程序启动时初始化控件和事件处理程序,确保所有功能正常运行。 通过C# WinForm,我们可以利用Listbox的AllowDrop属性、DragEnter、DragDrop、MouseDown和MouseUp事件,实现Listbox控件之间的拖放功能。这不仅提高了用户交互性,还增强了应用的功能性和灵活性。在实际应用中,开发者可以根据具体需求进行调整,例如添加对多选的支持、处理自定义对象等。
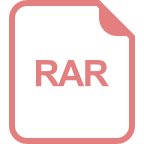
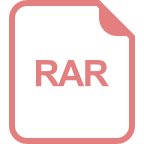
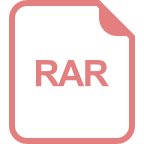
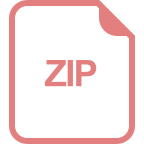
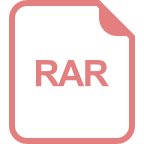
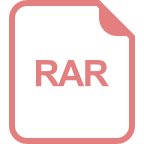
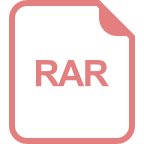
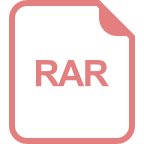
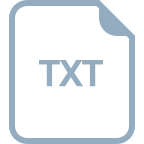
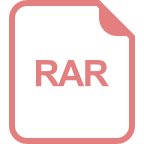
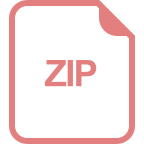
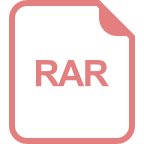
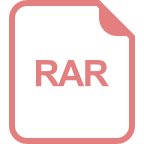
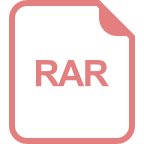
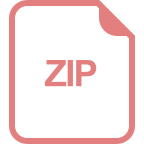
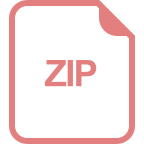


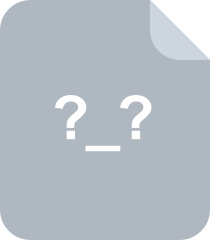
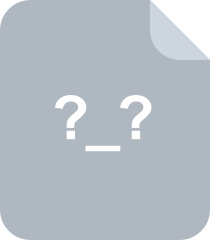

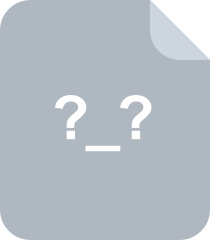
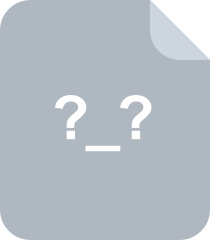


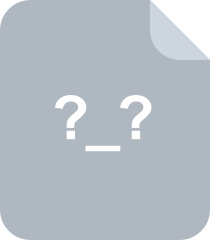
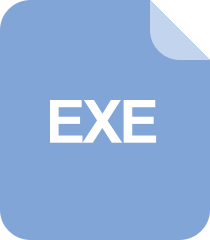

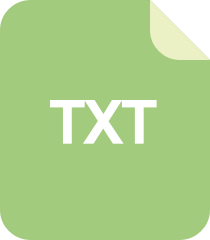
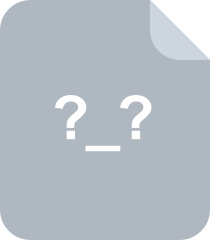
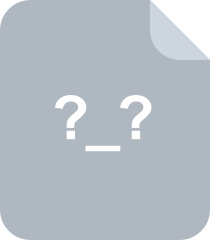
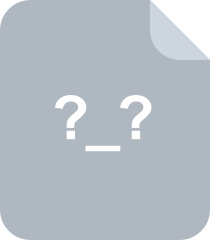


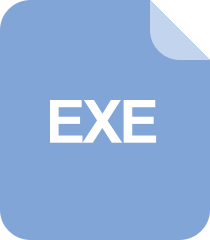
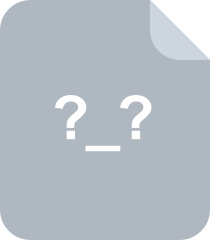
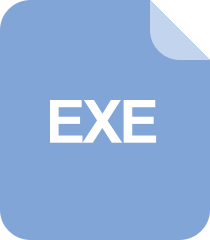
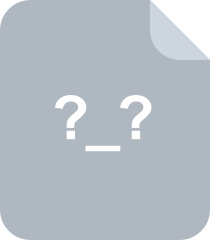
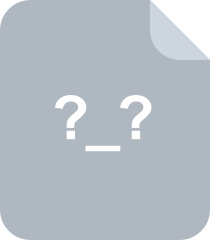
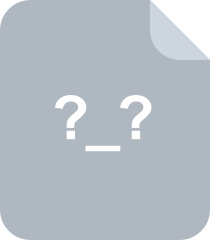
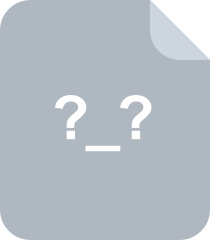

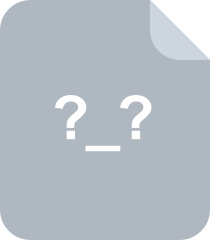
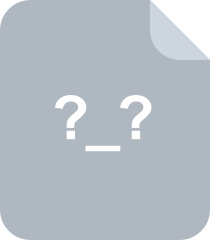
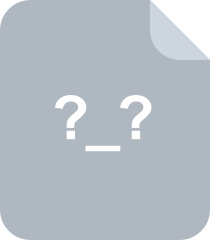
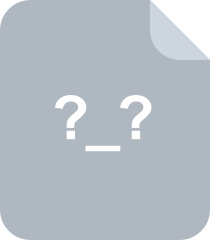
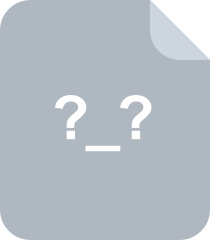
- 1

- 粉丝: 0
- 资源: 23
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

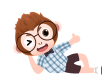
最新资源

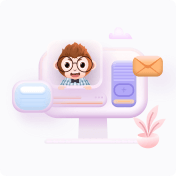

- 1
- 2
前往页