在C# WinForm开发中,用户界面的交互性是至关重要的。`ListBox`控件是常用的展示列表数据的组件,但默认情况下它不支持直接通过拖拽来改变条目的顺序。本示例将介绍如何为`ListBox`添加拖拽排序功能,使用户能够更直观、便捷地对列表进行排序。 我们需要在`Form1.cs`文件中定义`ListBox`控件,并为其设置一些基本属性,如`SelectionMode`和`AllowDrop`。`SelectionMode`应设置为`SelectionMode.MultiExtended`,这样用户可以选中多个项目;`AllowDrop`应设置为`true`,以便允许拖放操作。 ```csharp public partial class Form1 : Form { public Form1() { InitializeComponent(); listBox1.SelectionMode = SelectionMode.MultiExtended; listBox1.AllowDrop = true; } } ``` 接下来,我们需要处理几个关键的事件:`DragEnter`, `DragLeave`, `DragOver`, 和 `Drop`。这些事件会在用户拖动鼠标时触发,帮助我们实现拖拽排序的功能。 在`DragEnter`事件中,我们将检查数据是否可以被拖放到`ListBox`中。如果是,我们将设置`DragEffect`为`DragDropEffects.Move`,表示可以移动项目。 ```csharp private void listBox1_DragEnter(object sender, DragEventArgs e) { if (e.Data.GetDataPresent(typeof(string))) { e.Effect = DragDropEffects.Move; } else { e.Effect = DragDropEffects.None; } } ``` `DragOver`事件用于更新鼠标下方项的位置。我们需要计算出鼠标的相对位置,并调整`ListBox`的选中项。 ```csharp private void listBox1_DragOver(object sender, DragEventArgs e) { Point mousePosition = Control.MousePosition; Point controlPoint = listBox1.PointToClient(mousePosition); int index = listBox1.IndexFromPoint(controlPoint); // 防止越界 if (index < 0) index = 0; else if (index > listBox1.Items.Count - 1) index = listBox1.Items.Count - 1; // 如果当前选中的项和新位置不同,更新选中项 if (listBox1.SelectedIndex != index) { listBox1.SelectedIndex = index; } } ``` 在`Drop`事件中,我们实际上执行了项目的移动操作。我们获取到被拖放的数据,然后交换当前选中项和新位置的项。 ```csharp private void listBox1_Drop(object sender, DragEventArgs e) { if (e.Data.GetDataPresent(typeof(string))) { string[] data = (string[])e.Data.GetData(typeof(string)); int currentIndex = listBox1.SelectedIndex; listBox1.Items[currentIndex] = listBox1.Items[index]; listBox1.Items[index] = data[0]; listBox1.SelectedIndex = index; } } ``` 同时,为了启动拖放操作,我们还需要在`ListBox`的`MouseDown`事件中设置`DoDragDrop`,以便在用户点击并拖动时开始拖放。 ```csharp private void listBox1_MouseDown(object sender, MouseEventArgs e) { if (e.Button == MouseButtons.Left && listBox1.SelectedItem != null) { string[] selectedItems = new string[listBox1.SelectedItems.Count]; listBox1.SelectedItems.CopyTo(selectedItems, 0); listBox1.DoDragDrop(selectedItems, DragDropEffects.Move); } } ``` 以上代码实现了一个基本的`ListBox`拖拽排序功能。在`Form1.Designer.cs`中,你需要确保`listBox1`已经被正确初始化,并且在`InitializeComponent`方法中调用了上面的事件处理器。 `Program.cs`文件通常包含了应用程序的主入口点,`WindowsFormsApplication1.csproj`是项目文件,而`Form1.resx`和`Properties`文件夹则包含了窗体资源和其他配置信息,这些文件在实现拖拽排序功能中并不直接涉及。 通过处理`ListBox`控件的相关事件,我们可以为用户提供一种直观的交互方式,让他们通过拖拽来轻松调整列表的顺序。这种增强的用户体验使得C# WinForm应用更加符合现代软件设计的要求。
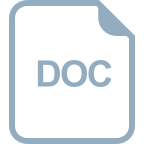
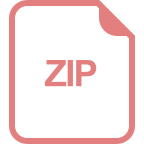
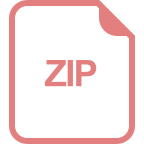
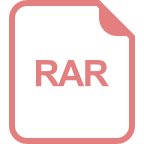
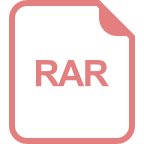
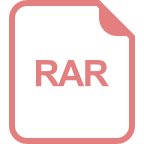
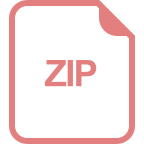
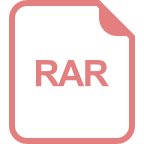
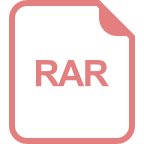
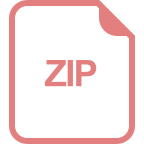
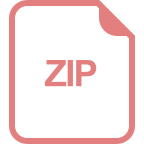
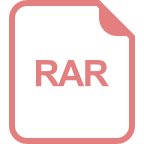
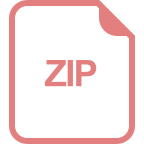

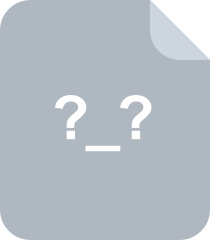
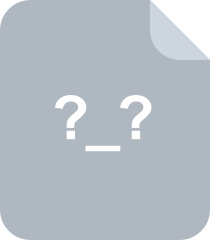
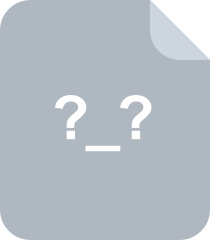
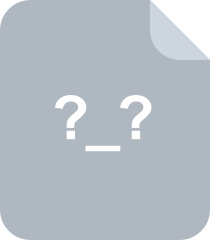

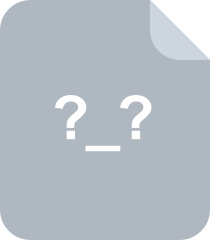
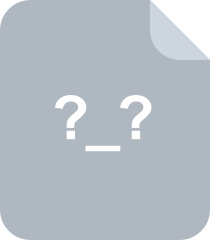
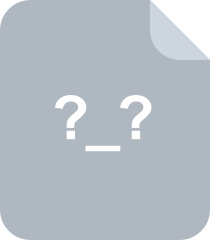
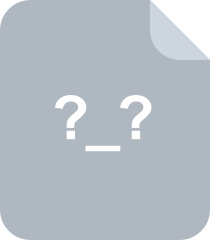
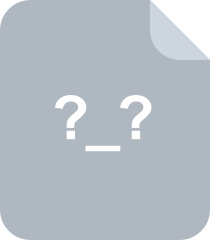
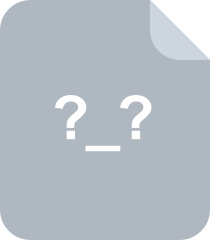
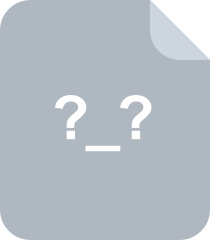
- 1
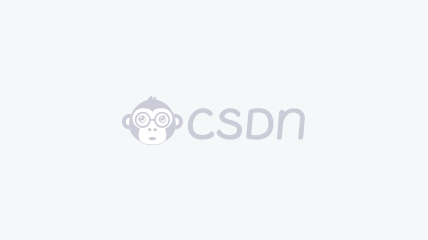

- 粉丝: 2
- 资源: 4
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

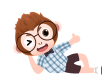
最新资源
- java全大撒大撒大苏打
- pca20241222
- LabVIEW实现LoRa通信【LabVIEW物联网实战】
- CS-TY4-4WCN-转-公版-XP1-8B4WF-wifi8188
- 计算机网络期末复习资料(课后题答案+往年考试题+复习提纲+知识点总结)
- 从零学习自动驾驶Lattice规划算法(下) 轨迹采样 轨迹评估 碰撞检测 包含matlab代码实现和cpp代码实现,方便对照学习 cpp代码用vs2019编译 依赖qt5.15做可视化 更新:
- 风光储、风光储并网直流微电网simulink仿真模型 系统由光伏发电系统、风力发电系统、混合储能系统(可单独储能系统)、逆变器VSR+大电网构成 光伏系统采用扰动观察法实现mppt控
- (180014016)pycairo-1.18.2-cp35-cp35m-win32.whl.rar
- (180014046)pycairo-1.21.0-cp311-cp311-win32.whl.rar
- DS-7808-HS-HF / DS-7808-HW-E1

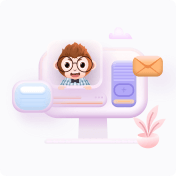
