Tensorflow卷积神经网络MNIST示例
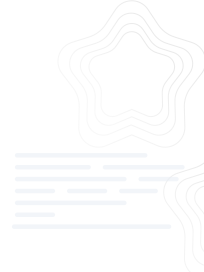

卷积神经网络(Convolutional Neural Networks,简称CNN)是一种深度学习模型,广泛应用于图像识别与处理领域。在本示例中,我们将深入探讨如何利用TensorFlow这一强大的开源机器学习库来实现对MNIST手写数字数据集的识别。MNIST数据集包含60,000个训练样本和10,000个测试样本,是机器学习初学者入门的常用数据集。 我们需要导入必要的库,包括TensorFlow和MNIST数据集: ```python import tensorflow as tf from tensorflow.examples.tutorials.mnist import input_data ``` 然后,我们加载MNIST数据集: ```python mnist = input_data.read_data_sets("MNIST_data/", one_hot=True) ``` 这里的`one_hot=True`表示将标签转化为独热编码形式,便于神经网络处理。 接下来,构建卷积神经网络模型。CNN通常由卷积层(Conv2D)、池化层(Pooling)、全连接层(Dense)和激活函数等组成。以下是一个简单示例: ```python # 定义超参数 learning_rate = 0.001 epochs = 10 batch_size = 128 # 输入层 x_input = tf.placeholder(tf.float32, [None, 28, 28, 1], name='x-input') # 第一个卷积层 conv1 = tf.layers.conv2d(x_input, filters=32, kernel_size=5, padding='same', activation=tf.nn.relu) # 池化层 pool1 = tf.layers.max_pooling2d(conv1, pool_size=2, strides=2) # 第二个卷积层 conv2 = tf.layers.conv2d(pool1, filters=64, kernel_size=5, padding='same', activation=tf.nn.relu) # 池化层 pool2 = tf.layers.max_pooling2d(conv2, pool_size=2, strides=2) # 全连接层 flatten = tf.reshape(pool2, [-1, 7 * 7 * 64]) fc1 = tf.layers.dense(flatten, units=128, activation=tf.nn.relu) # 输出层 output = tf.layers.dense(fc1, units=10, activation=tf.nn.softmax) # 定义损失函数和优化器 y_label = tf.placeholder(tf.float32, [None, 10]) cross_entropy = tf.reduce_mean(-tf.reduce_sum(y_label * tf.log(output), axis=1)) optimizer = tf.train.AdamOptimizer(learning_rate).minimize(cross_entropy) # 计算准确率 correct_pred = tf.equal(tf.argmax(output, 1), tf.argmax(y_label, 1)) accuracy = tf.reduce_mean(tf.cast(correct_pred, tf.float32)) ``` 在定义了模型结构后,我们需要初始化会话并开始训练: ```python with tf.Session() as sess: sess.run(tf.global_variables_initializer()) for epoch in range(epochs): for batch_idx in range(int(mnist.train.num_examples / batch_size)): batch_x, batch_y = mnist.train.next_batch(batch_size) sess.run(optimizer, feed_dict={x_input: batch_x, y_label: batch_y}) train_acc = sess.run(accuracy, feed_dict={x_input: mnist.train.images, y_label: mnist.train.labels}) test_acc = sess.run(accuracy, feed_dict={x_input: mnist.test.images, y_label: mnist.test.labels}) print('Epoch:', epoch+1, 'Training Accuracy:', train_acc, 'Test Accuracy:', test_acc) ``` 这个示例展示了如何在TensorFlow中构建一个基础的CNN模型,并应用到MNIST数据集上进行手写数字识别。在实际应用中,可以调整超参数(如学习率、卷积核大小、层数等)以优化模型性能。通过这个过程,你可以深入理解CNN的工作原理以及TensorFlow在构建和训练神经网络中的作用。
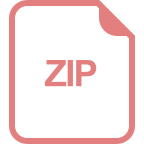
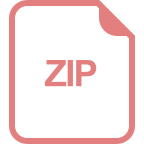
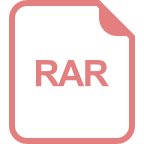
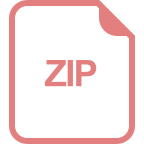
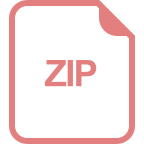
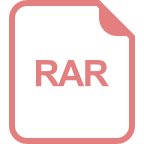
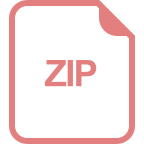
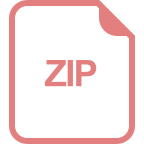
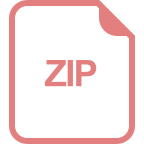
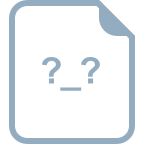
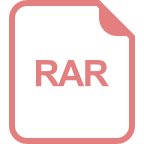
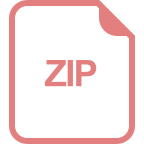
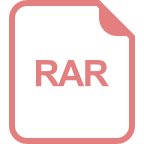
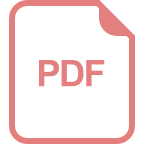
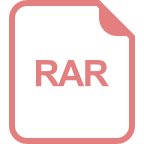
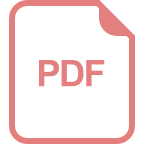
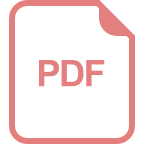
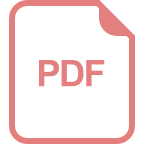
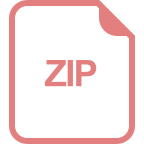
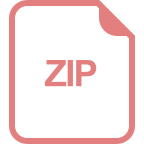
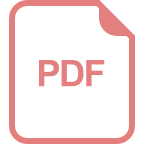
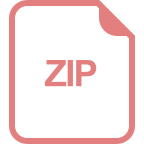


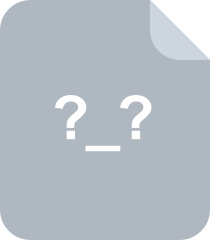
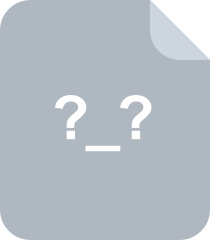
- 1
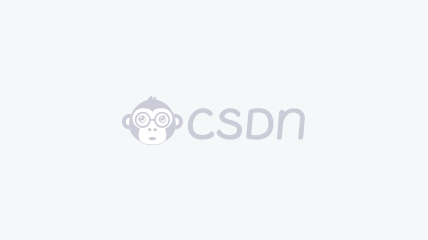

- 粉丝: 1
- 资源: 2
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

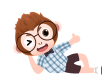
最新资源

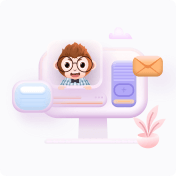
