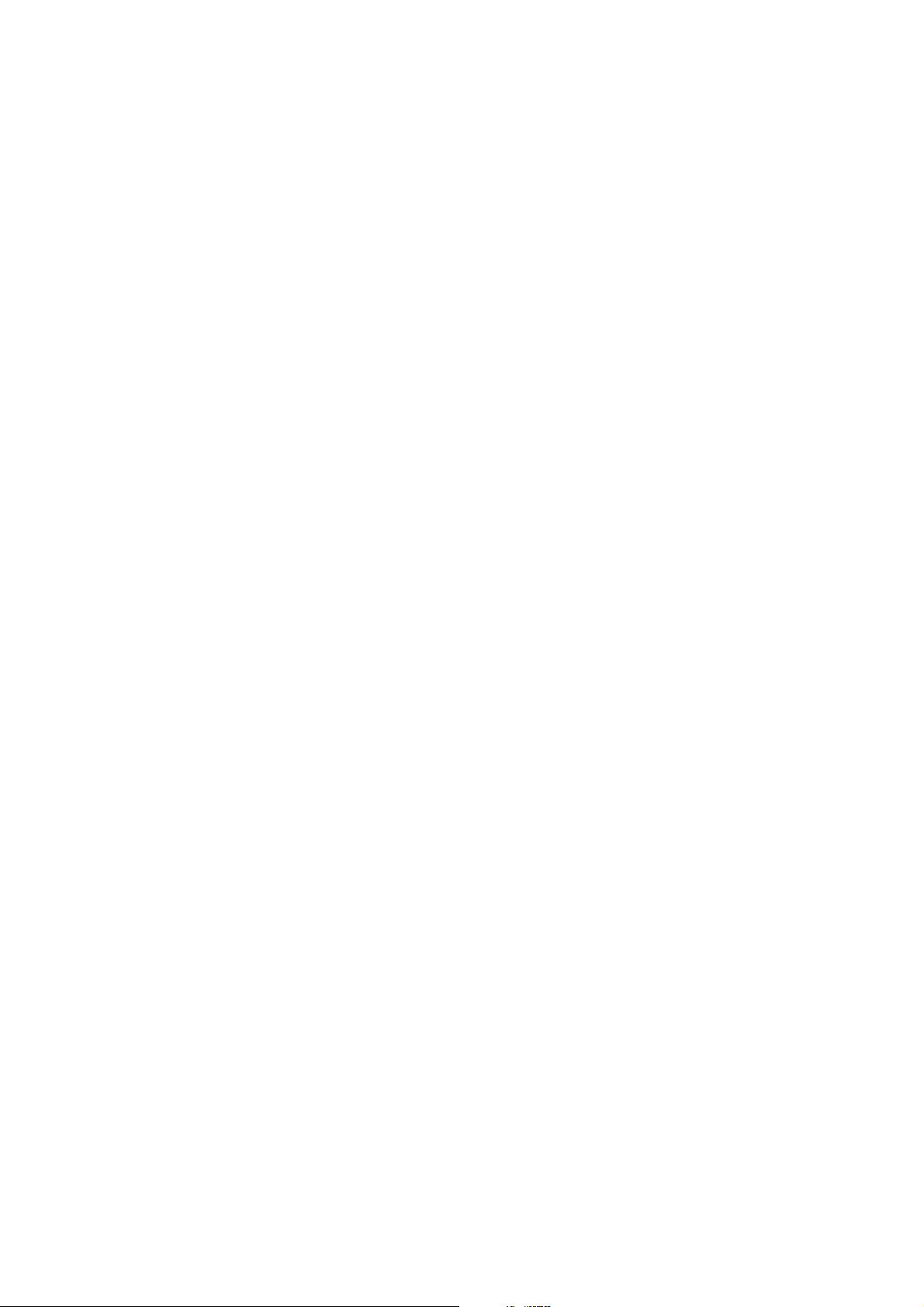
iv CONTENTS
3 Tools of the Trade 19
3.1 Scripting Languages . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 19
3.2 POSIX Multiprocessing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 19
3.2.1 POSIX Process Creation and Destruction . . . . . . . . . . . . . . . . . . . . . . . . . 20
3.2.2 POSIX Thread Creation and Destruction . . . . . . . . . . . . . . . . . . . . . . . . . 21
3.2.3 POSIX Locking . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 21
3.2.4 POSIX Reader-Writer Locking . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 23
3.3 Atomic Operations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 25
3.4 Linux-Kernel Equivalents to POSIX Operations . . . . . . . . . . . . . . . . . . . . . . . . . . 26
3.5 The Right Tool for the Job: How to Choose? . . . . . . . . . . . . . . . . . . . . . . . . . . . 26
4 Counting 29
4.1 Why Isn’t Concurrent Counting Trivial? . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 29
4.2 Statistical Counters . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 31
4.2.1 Design . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 31
4.2.2 Array-Based Imple mentation . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 31
4.2.3 Eventually Cons is te nt Implementation . . . . . . . . . . . . . . . . . . . . . . . . . . . 32
4.2.4 Per-Thread-Variable- Base d Implementation . . . . . . . . . . . . . . . . . . . . . . . . 33
4.2.5 Discussion . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 34
4.3 Approximate Limi t Counters . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 34
4.3.1 Design . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 34
4.3.2 Simple Limit Counter Implementation . . . . . . . . . . . . . . . . . . . . . . . . . . . 34
4.3.3 Simple Limit Counter Discussion . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 37
4.3.4 Approximate Limi t Counter Implementation . . . . . . . . . . . . . . . . . . . . . . . 37
4.3.5 Approximate Limi t Counter Discussion . . . . . . . . . . . . . . . . . . . . . . . . . . 38
4.4 Exact Limit Counters . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 38
4.4.1 Atomic Limit Counter Implementation . . . . . . . . . . . . . . . . . . . . . . . . . . . 38
4.4.2 Atomic Limit Counter Discussion . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 41
4.4.3 Signal-Theft Limit Counter Design . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 41
4.4.4 Signal-Theft Limit Counter Implementation . . . . . . . . . . . . . . . . . . . . . . . . 42
4.4.5 Signal-Theft Limit Counter Discussion . . . . . . . . . . . . . . . . . . . . . . . . . . . 44
4.5 Applying Specialized Parallel Counters . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 44
4.6 Parallel Counting Discussion . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 45
5 Partitioning and Synchronization Design 47
5.1 Partitioning Exercises . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 47
5.1.1 Dining Philosophers Problem . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 47
5.1.2 Double-Ended Queue . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 49
5.1.3 Partitioning Example Discu s s ion . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 53
5.2 Design Criteria . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 53
5.3 Synchronization Granularity . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 55
5.3.1 Sequential Program . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 55
5.3.2 Code Locking . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 56
5.3.3 Data Locking . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 56
5.3.4 Data Ownership . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 58
5.3.5 Locking Granularity and Performance . . . . . . . . . . . . . . . . . . . . . . . . . . . 59
5.4 Parallel Fastpath . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 60
5.4.1 Reader/Writer Locking . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 61
5.4.2 Read-Copy Update Introduction . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 61
5.4.3 Hierarchical Locking . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 62
5.4.4 Resource Allocator Caches . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 63
5.5 Performance Su mmary . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 66