# pyright: reportPropertyTypeMismatch=false
from __future__ import annotations
import collections
from copy import deepcopy
import datetime as dt
from functools import partial
import gc
from json import loads
import operator
import pickle
import re
import sys
from typing import (
TYPE_CHECKING,
Any,
Callable,
ClassVar,
Literal,
NoReturn,
cast,
final,
overload,
)
import warnings
import weakref
import numpy as np
from pandas._config import (
config,
using_copy_on_write,
warn_copy_on_write,
)
from pandas._libs import lib
from pandas._libs.lib import is_range_indexer
from pandas._libs.tslibs import (
Period,
Tick,
Timestamp,
to_offset,
)
from pandas._libs.tslibs.dtypes import freq_to_period_freqstr
from pandas._typing import (
AlignJoin,
AnyArrayLike,
ArrayLike,
Axes,
Axis,
AxisInt,
CompressionOptions,
DtypeArg,
DtypeBackend,
DtypeObj,
FilePath,
FillnaOptions,
FloatFormatType,
FormattersType,
Frequency,
IgnoreRaise,
IndexKeyFunc,
IndexLabel,
InterpolateOptions,
IntervalClosedType,
JSONSerializable,
Level,
Manager,
NaPosition,
NDFrameT,
OpenFileErrors,
RandomState,
ReindexMethod,
Renamer,
Scalar,
Self,
SequenceNotStr,
SortKind,
StorageOptions,
Suffixes,
T,
TimeAmbiguous,
TimedeltaConvertibleTypes,
TimeNonexistent,
TimestampConvertibleTypes,
TimeUnit,
ValueKeyFunc,
WriteBuffer,
WriteExcelBuffer,
npt,
)
from pandas.compat import PYPY
from pandas.compat._constants import REF_COUNT
from pandas.compat._optional import import_optional_dependency
from pandas.compat.numpy import function as nv
from pandas.errors import (
AbstractMethodError,
ChainedAssignmentError,
InvalidIndexError,
SettingWithCopyError,
SettingWithCopyWarning,
_chained_assignment_method_msg,
_chained_assignment_warning_method_msg,
_check_cacher,
)
from pandas.util._decorators import (
deprecate_nonkeyword_arguments,
doc,
)
from pandas.util._exceptions import find_stack_level
from pandas.util._validators import (
check_dtype_backend,
validate_ascending,
validate_bool_kwarg,
validate_fillna_kwargs,
validate_inclusive,
)
from pandas.core.dtypes.astype import astype_is_view
from pandas.core.dtypes.common import (
ensure_object,
ensure_platform_int,
ensure_str,
is_bool,
is_bool_dtype,
is_dict_like,
is_extension_array_dtype,
is_list_like,
is_number,
is_numeric_dtype,
is_re_compilable,
is_scalar,
pandas_dtype,
)
from pandas.core.dtypes.dtypes import (
DatetimeTZDtype,
ExtensionDtype,
)
from pandas.core.dtypes.generic import (
ABCDataFrame,
ABCSeries,
)
from pandas.core.dtypes.inference import (
is_hashable,
is_nested_list_like,
)
from pandas.core.dtypes.missing import (
isna,
notna,
)
from pandas.core import (
algorithms as algos,
arraylike,
common,
indexing,
missing,
nanops,
sample,
)
from pandas.core.array_algos.replace import should_use_regex
from pandas.core.arrays import ExtensionArray
from pandas.core.base import PandasObject
from pandas.core.construction import extract_array
from pandas.core.flags import Flags
from pandas.core.indexes.api import (
DatetimeIndex,
Index,
MultiIndex,
PeriodIndex,
RangeIndex,
default_index,
ensure_index,
)
from pandas.core.internals import (
ArrayManager,
BlockManager,
SingleArrayManager,
)
from pandas.core.internals.construction import (
mgr_to_mgr,
ndarray_to_mgr,
)
from pandas.core.methods.describe import describe_ndframe
from pandas.core.missing import (
clean_fill_method,
clean_reindex_fill_method,
find_valid_index,
)
from pandas.core.reshape.concat import concat
from pandas.core.shared_docs import _shared_docs
from pandas.core.sorting import get_indexer_indexer
from pandas.core.window import (
Expanding,
ExponentialMovingWindow,
Rolling,
Window,
)
from pandas.io.formats.format import (
DataFrameFormatter,
DataFrameRenderer,
)
from pandas.io.formats.printing import pprint_thing
if TYPE_CHECKING:
from collections.abc import (
Hashable,
Iterator,
Mapping,
Sequence,
)
from pandas._libs.tslibs import BaseOffset
from pandas import (
DataFrame,
ExcelWriter,
HDFStore,
Series,
)
from pandas.core.indexers.objects import BaseIndexer
from pandas.core.resample import Resampler
# goal is to be able to define the docs close to function, while still being
# able to share
_shared_docs = {**_shared_docs}
_shared_doc_kwargs = {
"axes": "keywords for axes",
"klass": "Series/DataFrame",
"axes_single_arg": "{0 or 'index'} for Series, {0 or 'index', 1 or 'columns'} for DataFrame", # noqa: E501
"inplace": """
inplace : bool, default False
If True, performs operation inplace and returns None.""",
"optional_by": """
by : str or list of str
Name or list of names to sort by""",
}
bool_t = bool # Need alias because NDFrame has def bool:
class NDFrame(PandasObject, indexing.IndexingMixin):
"""
N-dimensional analogue of DataFrame. Store multi-dimensional in a
size-mutable, labeled data structure
Parameters
----------
data : BlockManager
axes : list
copy : bool, default False
"""
_internal_names: list[str] = [
"_mgr",
"_cacher",
"_item_cache",
"_cache",
"_is_copy",
"_name",
"_metadata",
"_flags",
]
_internal_names_set: set[str] = set(_internal_names)
_accessors: set[str] = set()
_hidden_attrs: frozenset[str] = frozenset([])
_metadata: list[str] = []
_is_copy: weakref.ReferenceType[NDFrame] | str | None = None
_mgr: Manager
_attrs: dict[Hashable, Any]
_typ: str
# ----------------------------------------------------------------------
# Constructors
def __init__(self, data: Manager) -> None:
object.__setattr__(self, "_is_copy", None)
object.__setattr__(self, "_mgr", data)
object.__setattr__(self, "_item_cache", {})
object.__setattr__(self, "_attrs", {})
object.__setattr__(self, "_flags", Flags(self, allows_duplicate_labels=True))
@final
@classmethod
def _init_mgr(
cls,
mgr: Manager,
axes: dict[Literal["index", "columns"], Axes | None],
dtype: DtypeObj | None = None,
copy: bool_t = False,
) -> Manager:
"""passed a manager and a axes dict"""
for a, axe in axes.items():
if axe is not None:
axe = ensure_index(axe)
bm_axis = cls._get_block_manager_axis(a)
mgr = mgr.reindex_axis(axe, axis=bm_axis)
# make a copy if explicitly requested
if copy:
mgr = mgr.copy()
if dtype is not None:
# avoid further copies if we can
if (
isinstance(mgr, BlockManager)
and len(mgr.blocks) == 1
and mgr.blocks[0].values.dtype == dtype
):
pass
else:
mgr = mgr.astype(dtype=dtype)
return mgr
@final
def _as_manager(self, typ: str, copy: bool_t = True) -> Self:
"""
Private helper function to create a DataFrame with specific manager.
Parameters
----------
typ : {"block", "array"}
copy : bool, default True
Only controls whether the conversion from Block->ArrayManager
copies the 1D arrays (to ensure proper/contiguous memory layout).
Returns
-------
DataFrame
New DataFrame using specified manager type. Is not guaranteed
to be a copy or not.
"""
没有合适的资源?快使用搜索试试~ 我知道了~
abaqus 调用外部模块pandas的方法, 这里是abaqus2024的方法,亲测好用
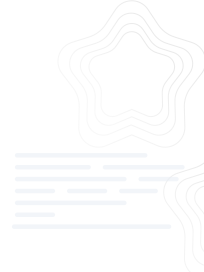
共2000个文件
py:1612个
json:14个
txt:11个

需积分: 5 0 下载量 21 浏览量
2024-05-19
23:04:43
上传
评论
收藏 19.51MB ZIP 举报
温馨提示
abaqus2024开始可以使用py3,支持了大部分都数学求解器numpy,但是性能方面还不是十分满意,有的时候会处理比较复杂的功能, 只有numpy远远不够的,因此,需要研究如何导入外部的模块,比如pandas。 但是默认是没有pandas。 资源里面有解决办法
资源推荐
资源详情
资源评论
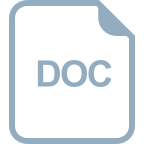
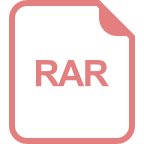
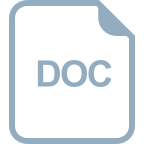
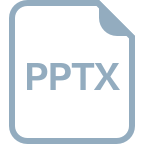
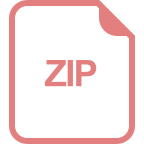
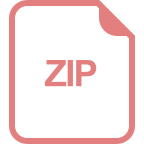
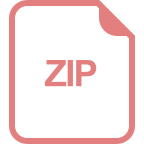
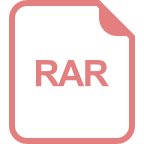
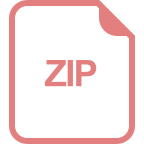
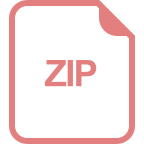
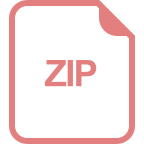
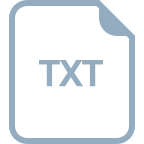
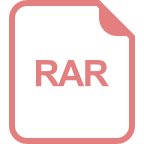
收起资源包目录

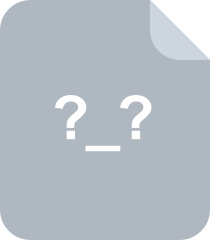
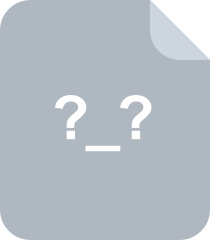
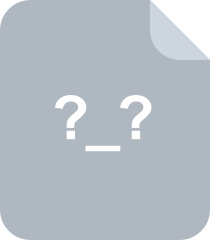
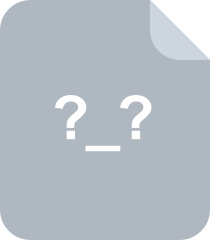
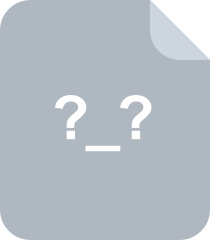
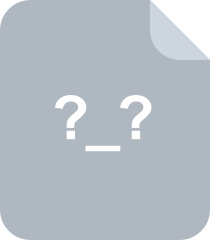
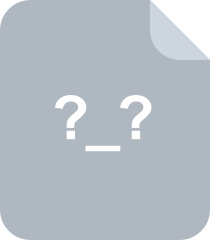
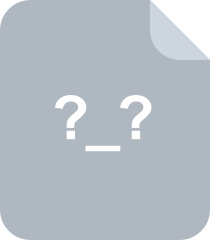
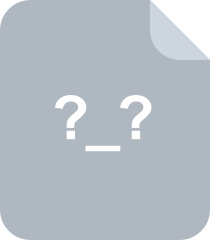
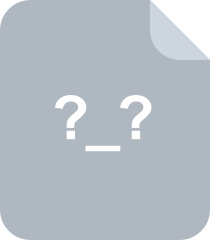
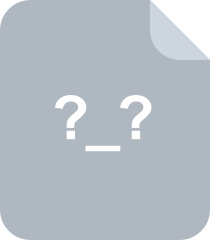
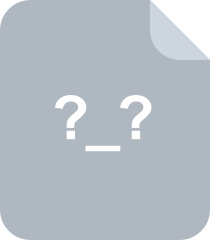
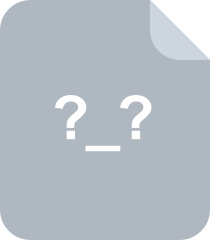
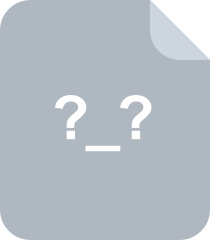
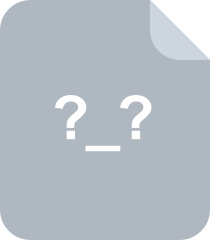
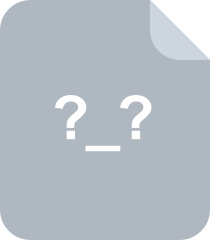
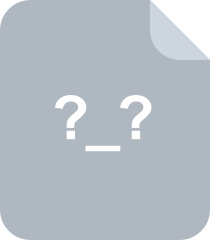
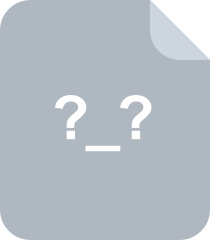
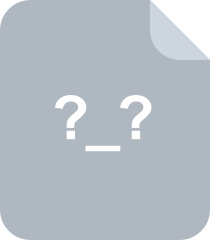
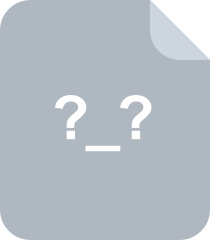
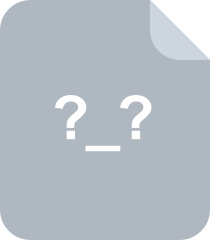
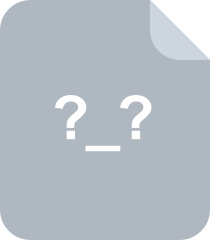
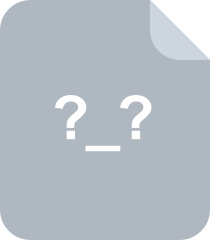
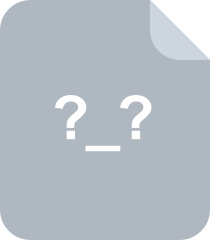
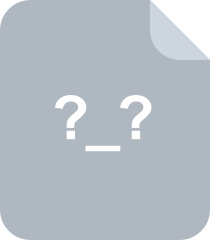
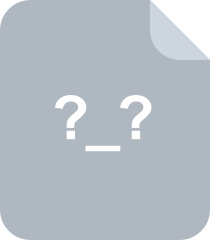
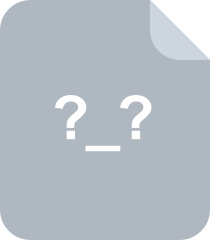
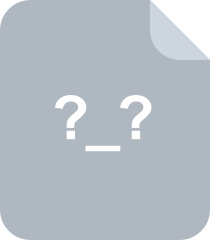
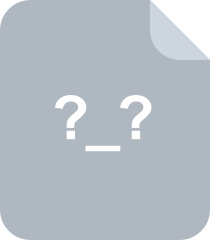
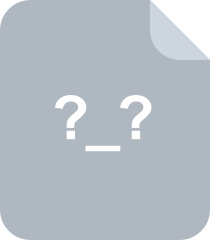
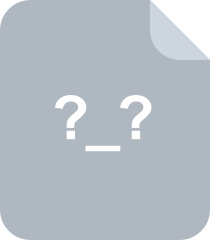
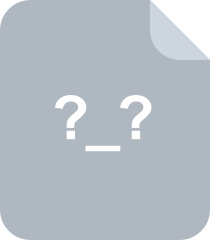
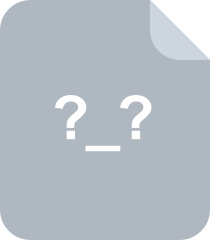
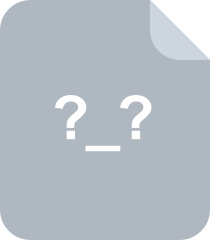
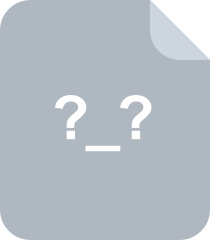
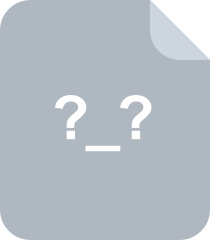
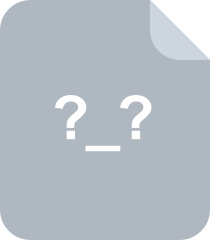
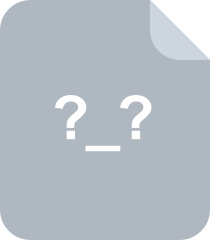
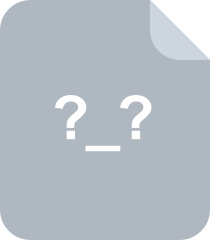
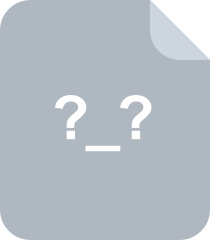
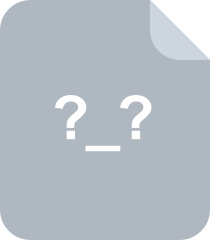
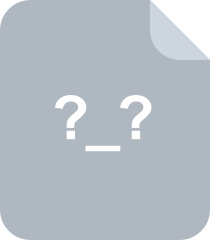
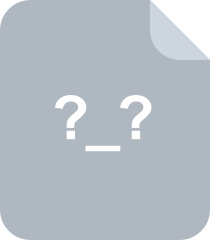
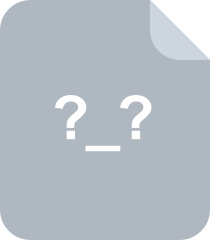
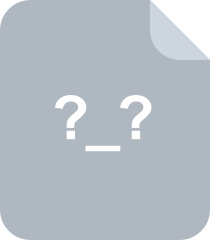
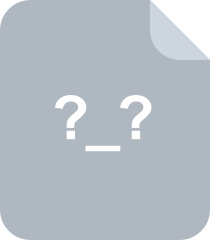
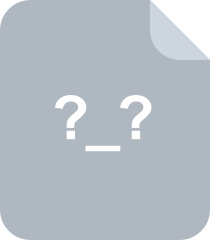
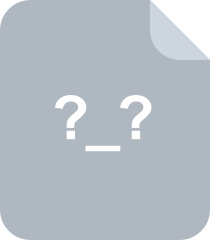
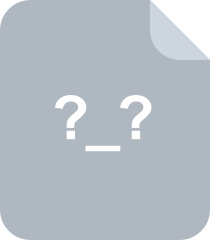
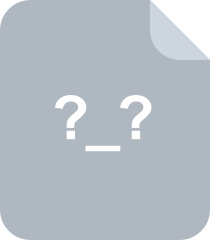
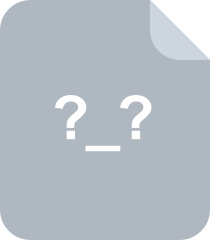
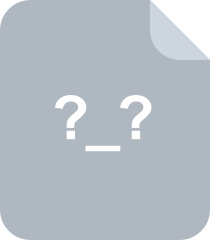
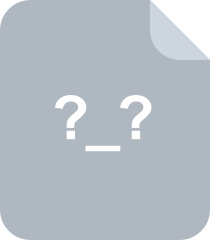
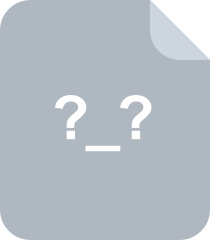
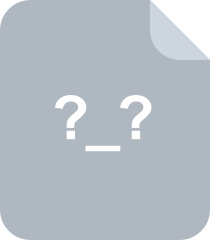
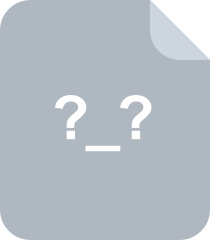
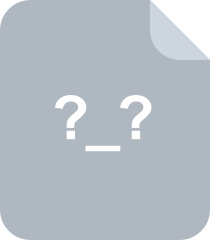
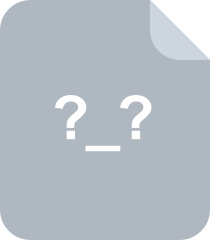
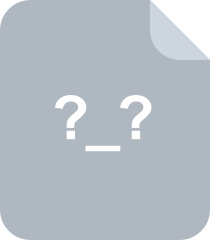
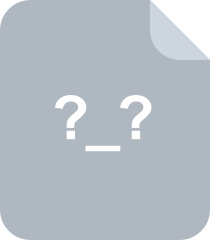
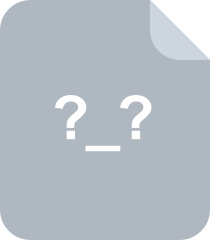
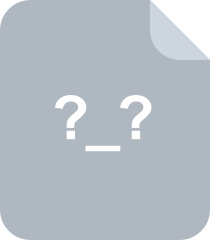
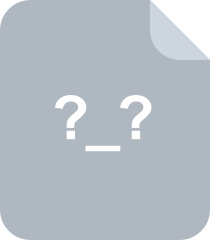
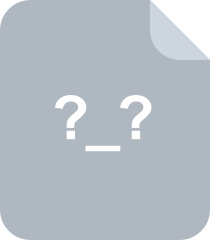
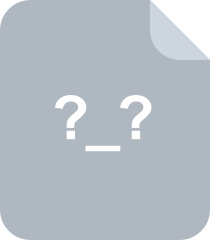
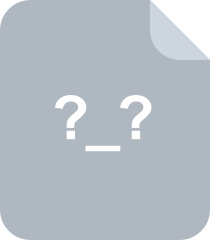
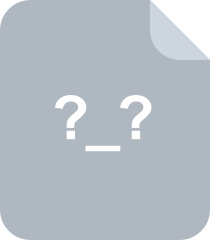
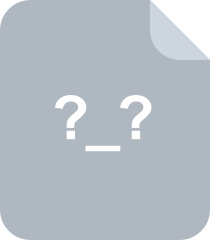
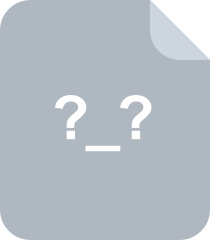
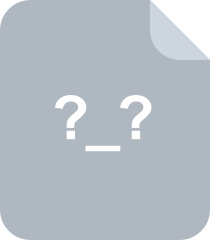
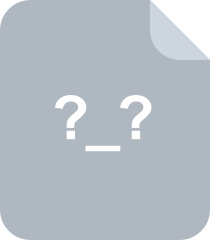
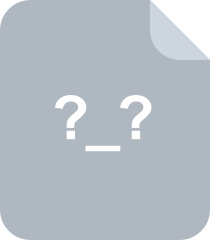
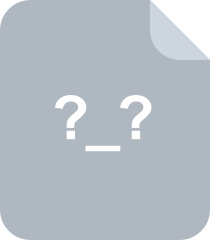
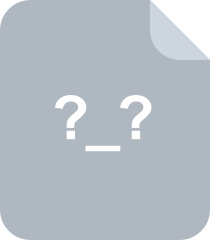
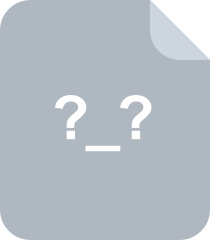
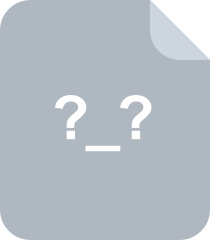
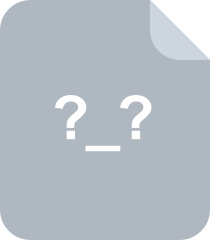
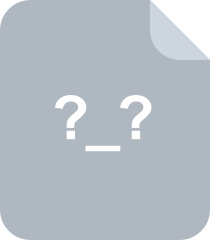
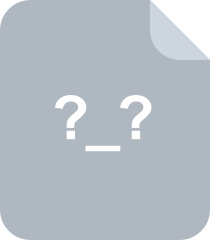
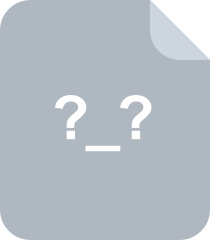
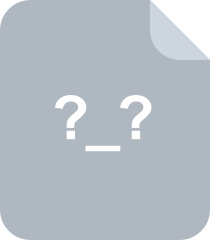
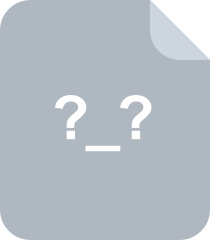
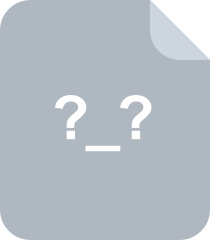
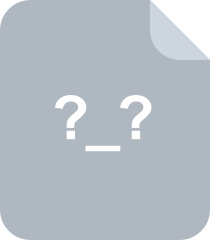
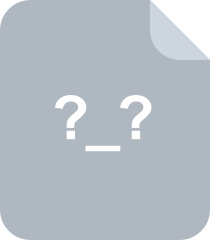
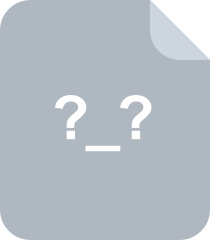
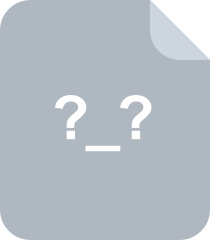
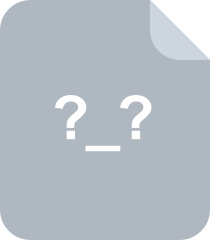
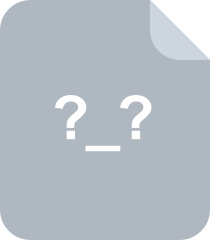
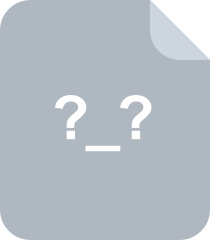
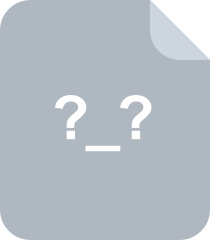
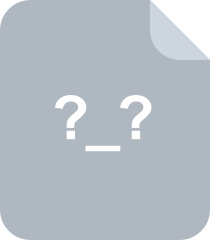
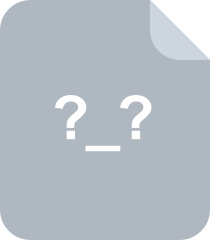
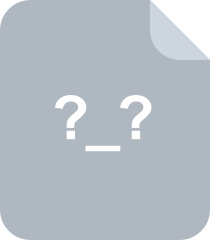
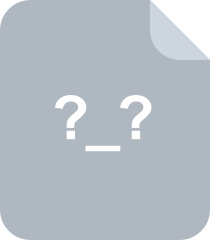
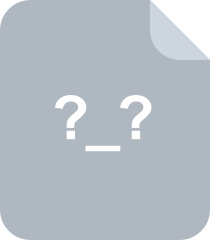
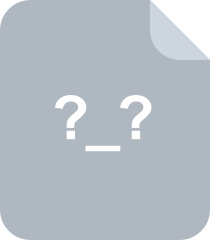
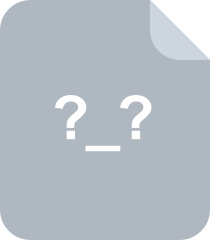
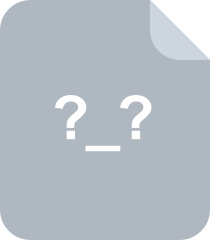
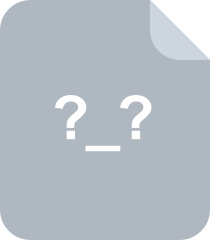
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
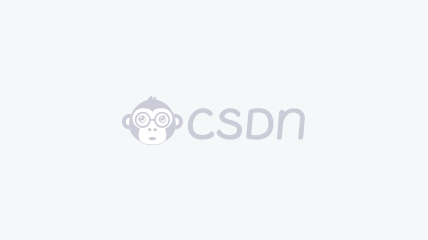

haifeng191919
- 粉丝: 0
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

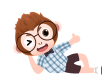
安全验证
文档复制为VIP权益,开通VIP直接复制
