**DWR级联菜单下拉框** DWR(Direct Web Remoting)是一个开源JavaScript库,它允许Web应用程序在客户端和服务器之间进行实时通信,而无需使用传统的页面刷新。在这个项目中,我们关注的是如何利用DWR实现一个三级联动的下拉菜单。这种交互式菜单在用户界面设计中常见,用于在多个选项之间建立关联,如省份-城市-区县的选择,以提供更加精细化的筛选。 我们需要理解DWR的核心概念:通过AJAX(异步JavaScript和XML)技术,DWR使Java方法可以直接在客户端调用,从而实现了前后端的无缝通信。在创建级联下拉框时,通常需要以下步骤: 1. **服务器端准备**: - 配置DWR:在项目中引入DWR库,配置dwr.xml文件,声明允许客户端调用的Java方法。 - 编写Java服务:根据数据库结构(例如Ms SQL 2005中的表和字段),编写获取数据的服务,如获取省份、城市和区县的列表。 2. **数据库设计**: - 设计关联表:为了实现级联效果,数据库中的相关表需要有合适的外键关联,比如省份表、城市表和区县表之间的层级关系。 3. **前端页面**: - HTML布局:创建包含三个下拉框的HTML页面,初始状态下只显示最顶层的下拉框。 - JavaScript/DWR调用:使用DWR的JavaScript API,为每个下拉框设置事件监听器,当用户在上一级选择一个选项时,触发DWR调用服务器获取下一级的选项并填充到对应的下拉框中。 4. **DWR配置与使用**: - 在dwr.xml中,定义一个或多个`<allow>`标签,指定可以被JavaScript调用的Java方法。 - 在JavaScript中,使用`DWRUtil`或`DWREngine`对象来调用Java方法,获取数据后更新DOM以改变下拉框的选项。 5. **事件处理与异步通信**: - 使用`onChange`事件监听下拉框的改变,当用户选择一个选项时,触发DWR调用服务器。 - DWR通过异步请求,不会阻塞用户界面,提高了用户体验。 6. **错误处理与优化**: - 实现错误处理机制,如网络中断或服务器异常时的提示。 - 对大量数据进行分页或缓存,提高响应速度。 在项目文件`dwr_test`中,可能包含了相关的HTML、JavaScript、CSS和Java代码,这些文件展示了如何将上述步骤具体实现。通过对这些文件的分析和学习,我们可以深入理解DWR如何与数据库交互,以及如何构建动态的前端界面。 DWR级联菜单下拉框是利用DWR和AJAX技术实现的动态交互功能,它简化了前后端通信,提供了更流畅的用户体验。通过学习和实践这个案例,开发者可以提升在Web应用开发中对DWR、AJAX以及级联菜单设计的理解和应用能力。
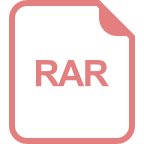
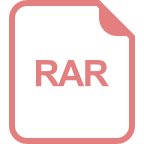
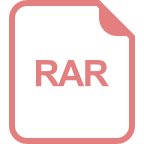
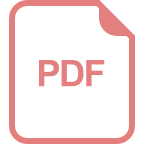
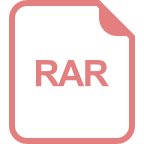
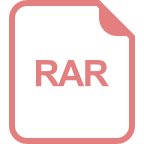
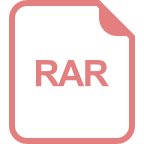
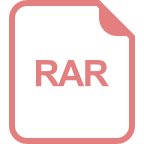
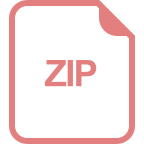
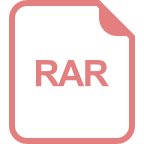
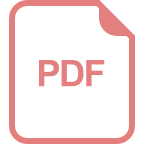
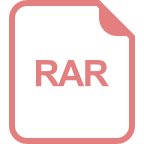
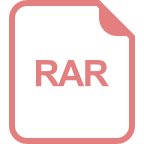
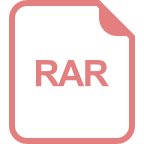
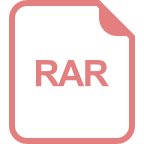
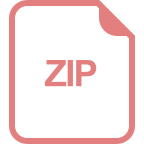
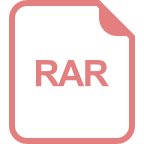
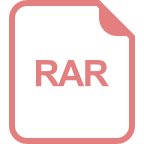
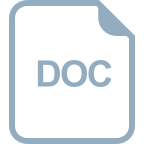
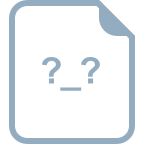
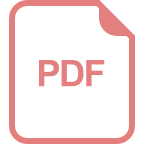
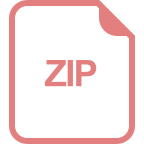
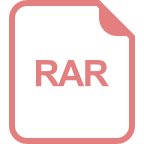
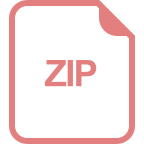




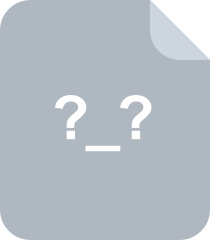
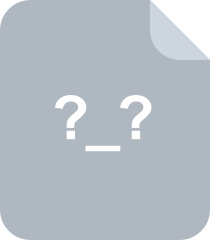
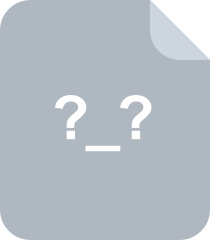
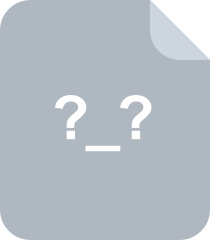
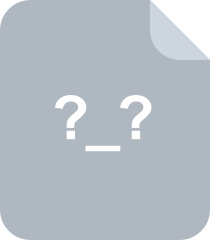
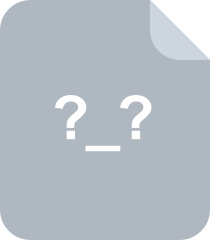
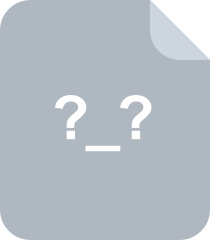
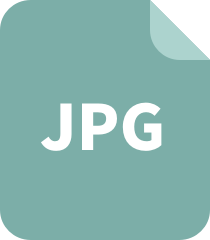
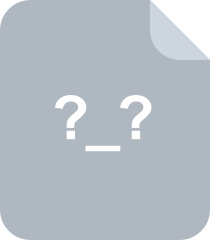

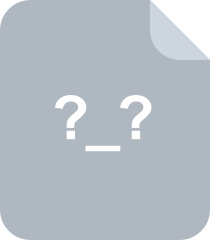
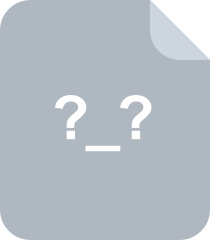
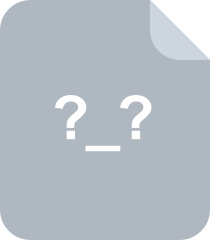
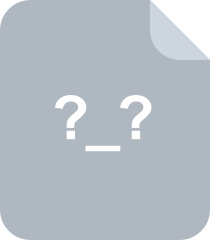

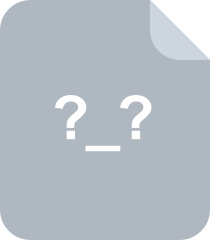


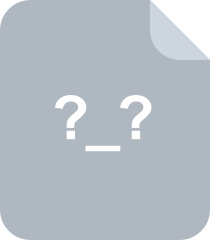


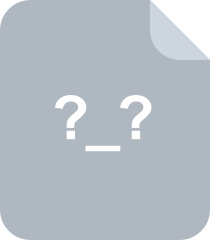
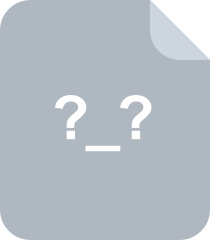
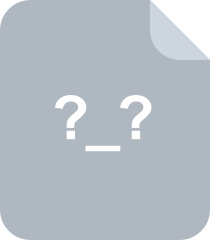
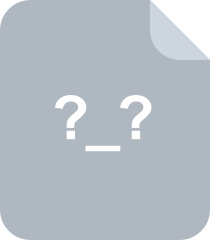
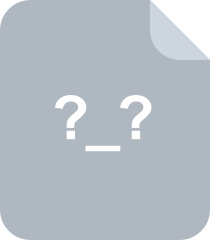



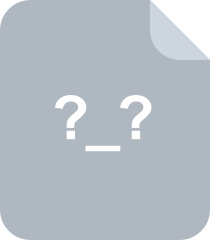
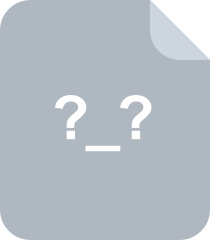
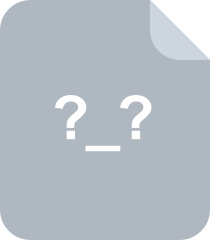
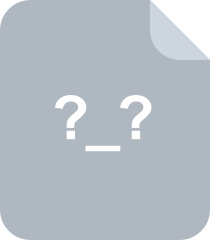
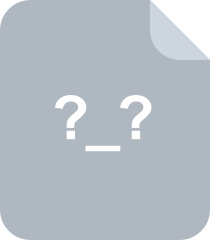
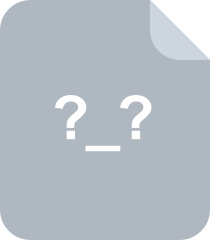

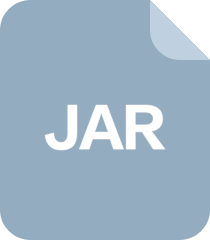
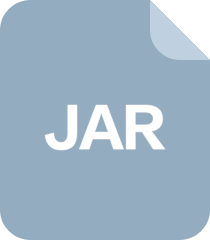
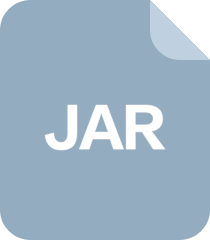
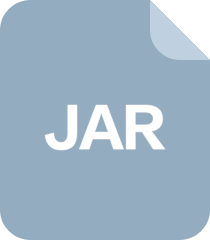
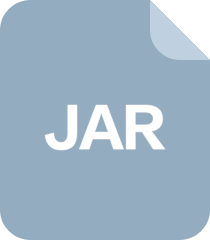
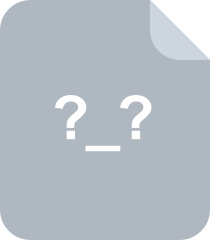
- 1
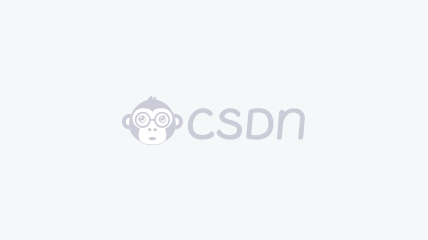
- fyJixiang2012-08-17代码好像有问题,不过思路挺好的。

- 粉丝: 13
- 资源: 3
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

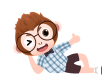
最新资源
- 使用NetBeans连接SQLserver2008数据库教程中文WORD版最新版本
- XPath实例中文WORD版最新版本
- XPath语法规则中文WORD版最新版本
- XPath入门教程中文WORD版最新版本
- ORACLE数据库管理系统体系结构中文WORD版最新版本
- Sybase数据库安装以及新建数据库中文WORD版最新版本
- tomcat6.0配置oracle数据库连接池中文WORD版最新版本
- hibernate连接oracle数据库中文WORD版最新版本
- MyEclipse连接MySQL的方法中文WORD版最新版本
- MyEclipse中配置Hibernate连接Oracle中文WORD版最新版本

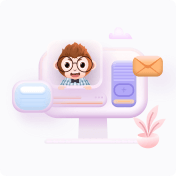
