Python基础训练100题(带答案).docx

Python3 100例实例001:数字组合实例002:“个税计算”实例003:完全平方数实例004:这天第几天实例005:三数排序实例006:斐波那契数列实例007:copy实例008:九九乘法表实例009:暂停一秒输出实例010:给人看的时间实例011:养兔子实例012:100到200的素数实例013:所有水仙花数 ### Python基础训练100题知识点解析 #### 实例001:数字组合 - **题目**:有四个数字:1、2、3、4,能组成多少个互不相同且无重复数字的三位数?各是多少? - **程序分析**: - 遍历1到4的所有可能性,确保三个数互不相同。 - 使用嵌套循环来生成不同的组合,并检查是否有重复的数字。 - 使用`itertools.permutations`函数可以更简洁地实现。 - **代码示例**: ```python import itertools total = 0 for i in range(1, 5): for j in range(1, 5): for k in range(1, 5): if (i != j) and (j != k) and (k != i): print(i, j, k) total += 1 print("Total:", total) # 或者使用itertools.permutations sum2 = 0 a = [1, 2, 3, 4] for i in itertools.permutations(a, 3): print(i) sum2 += 1 print("Total using permutations:", sum2) ``` #### 实例002:“个税计算” - **题目**:企业发放的奖金根据利润提成。利润(I)低于或等于10万元时,奖金可提10%;利润高于10万元,低于20万元时,低于10万元的部分按10%提成,高于10万元的部分,可提成7.5%;20万到40万之间时,高于20万元的部分,可提成5%;40万到60万之间时高于40万元的部分,可提成3%;60万到100万之间时,高于60万元的部分,可提成1.5%,高于100万元时,超过100万元的部分按1%提成,从键盘输入当月利润I,求应发放奖金总数? - **程序分析**: - 分区间计算奖金提成比例。 - 使用条件判断语句来确定奖金比例。 - 计算不同区间的奖金总额。 - **代码示例**: ```python def calculate_bonus(profit): bonus = 0 thresholds = [100000, 200000, 400000, 600000, 1000000] rates = [0.1, 0.075, 0.05, 0.03, 0.015, 0.01] if profit <= thresholds[0]: bonus = profit * rates[0] elif profit <= thresholds[1]: bonus = (thresholds[0] * rates[0]) + ((profit - thresholds[0]) * rates[1]) elif profit <= thresholds[2]: bonus = (thresholds[0] * rates[0]) + ((thresholds[1] - thresholds[0]) * rates[1]) + ((profit - thresholds[1]) * rates[2]) elif profit <= thresholds[3]: bonus = (thresholds[0] * rates[0]) + ((thresholds[1] - thresholds[0]) * rates[1]) + ((thresholds[2] - thresholds[1]) * rates[2]) + ((profit - thresholds[2]) * rates[3]) elif profit <= thresholds[4]: bonus = (thresholds[0] * rates[0]) + ((thresholds[1] - thresholds[0]) * rates[1]) + ((thresholds[2] - thresholds[1]) * rates[2]) + ((thresholds[3] - thresholds[2]) * rates[3]) + ((profit - thresholds[3]) * rates[4]) else: bonus = (thresholds[0] * rates[0]) + ((thresholds[1] - thresholds[0]) * rates[1]) + ((thresholds[2] - thresholds[1]) * rates[2]) + ((thresholds[3] - thresholds[2]) * rates[3]) + ((thresholds[4] - thresholds[3]) * rates[4]) + ((profit - thresholds[4]) * rates[5]) return bonus profit = int(input('请输入当月利润(单位:元): ')) bonus = calculate_bonus(profit) print(f'应发放奖金总额为:{bonus}元') ``` #### 实例003:完全平方数 - **题目**:判断一个数是否是完全平方数。 - **程序分析**: - 使用数学库中的sqrt函数来获取数的平方根。 - 检查平方根是否为整数。 - **代码示例**: ```python import math def is_perfect_square(n): root = math.sqrt(n) return root.is_integer() number = int(input('请输入一个数: ')) if is_perfect_square(number): print(f'{number} 是完全平方数') else: print(f'{number} 不是完全平方数') ``` #### 实例004:这天第几天 - **题目**:给定一个年份和月份,计算这一天是一年的第几天。 - **程序分析**: - 根据年份判断是否为闰年。 - 计算前几个月的总天数。 - 加上当前月的天数。 - **代码示例**: ```python def is_leap_year(year): return year % 4 == 0 and (year % 100 != 0 or year % 400 == 0) def day_of_year(year, month, day): days_in_month = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31] if is_leap_year(year): days_in_month[1] = 29 total_days = sum(days_in_month[:month - 1]) + day return total_days year = int(input('请输入年份: ')) month = int(input('请输入月份: ')) day = int(input('请输入日期: ')) print(f'这是第{day_of_year(year, month, day)}天') ``` #### 实例005:三数排序 - **题目**:输入三个整数,按照从小到大的顺序输出。 - **程序分析**: - 使用内置的排序函数。 - 或者使用条件语句比较每个数,调整顺序。 - **代码示例**: ```python def sort_three_numbers(a, b, c): return sorted([a, b, c]) numbers = input('请输入三个整数,用空格隔开: ') a, b, c = map(int, numbers.split()) sorted_numbers = sort_three_numbers(a, b, c) print('从小到大排序:', sorted_numbers) ``` #### 实例006:斐波那契数列 - **题目**:输出斐波那契数列的前N项。 - **程序分析**: - 使用循环或递归来生成斐波那契数列。 - 记录前两个数并不断更新。 - **代码示例**: ```python def fibonacci(n): if n <= 0: return [] elif n == 1: return [0] elif n == 2: return [0, 1] fib = [0, 1] for i in range(2, n): fib.append(fib[-1] + fib[-2]) return fib n = int(input('请输入项数: ')) print(f'斐波那契数列的前{n}项: {fibonacci(n)}') ``` #### 实例007:copy - **题目**:复制列表和字典。 - **程序分析**: - 使用`copy`模块的`deepcopy`函数来复制列表和字典。 - **代码示例**: ```python import copy def copy_data(data): return copy.deepcopy(data) original_list = [1, 2, [3, 4]] original_dict = {'a': 1, 'b': 2} copied_list = copy_data(original_list) copied_dict = copy_data(original_dict) print('Original list:', original_list) print('Copied list:', copied_list) print('Original dict:', original_dict) print('Copied dict:', copied_dict) ``` #### 实例008:九九乘法表 - **题目**:打印出九九乘法表。 - **程序分析**: - 使用嵌套循环来生成乘法表。 - **代码示例**: ```python def print_multiplication_table(): for i in range(1, 10): for j in range(1, i + 1): print(f'{j}×{i}={i * j}', end='\t') print() print_multiplication_table() ``` 以上几个实例展示了Python在处理基本算法问题、数据结构操作以及数学计算方面的应用。通过这些实例的学习,可以加深对Python语言的理解,并提高解决问题的能力。
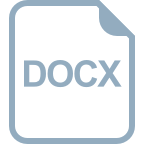
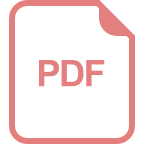
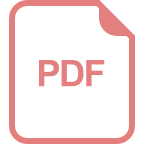
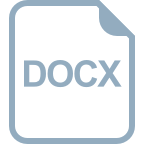
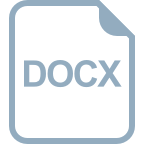
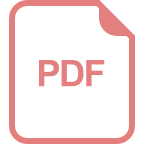
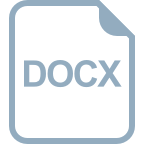
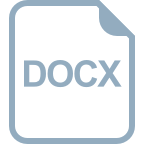
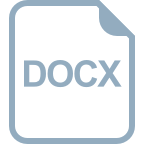
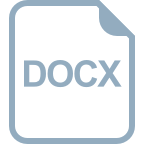
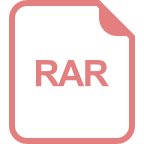
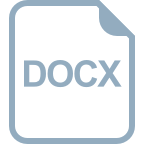
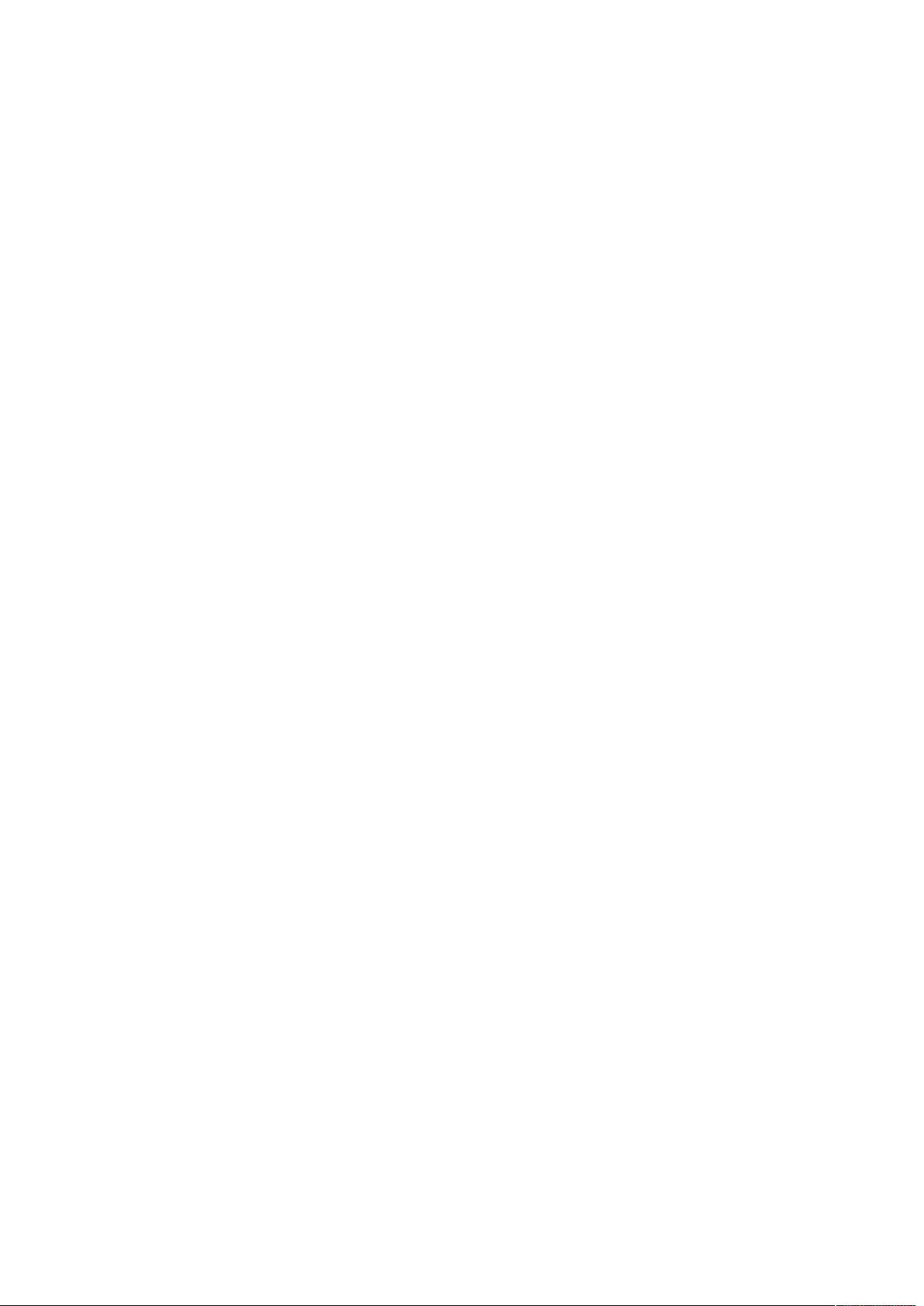
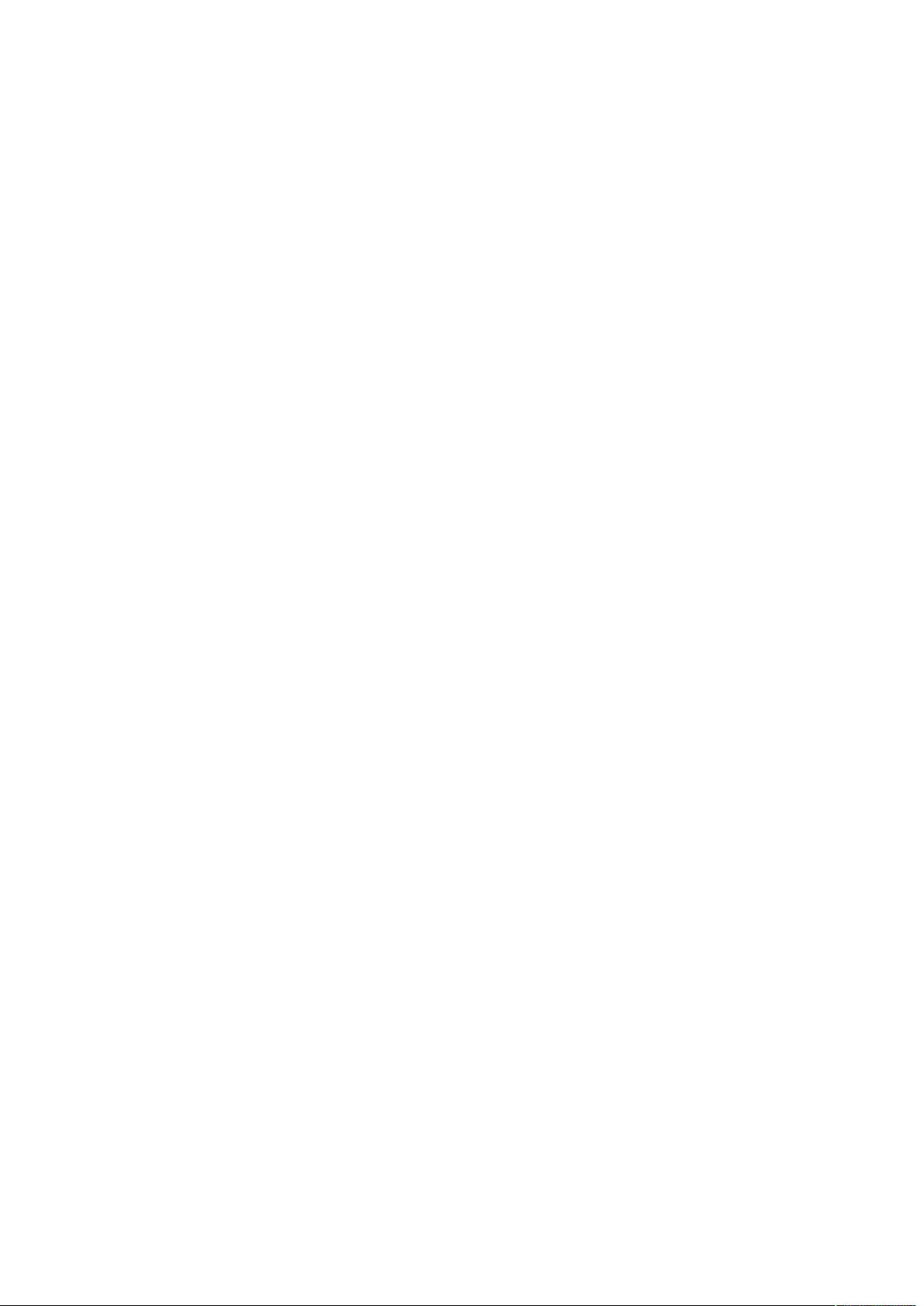
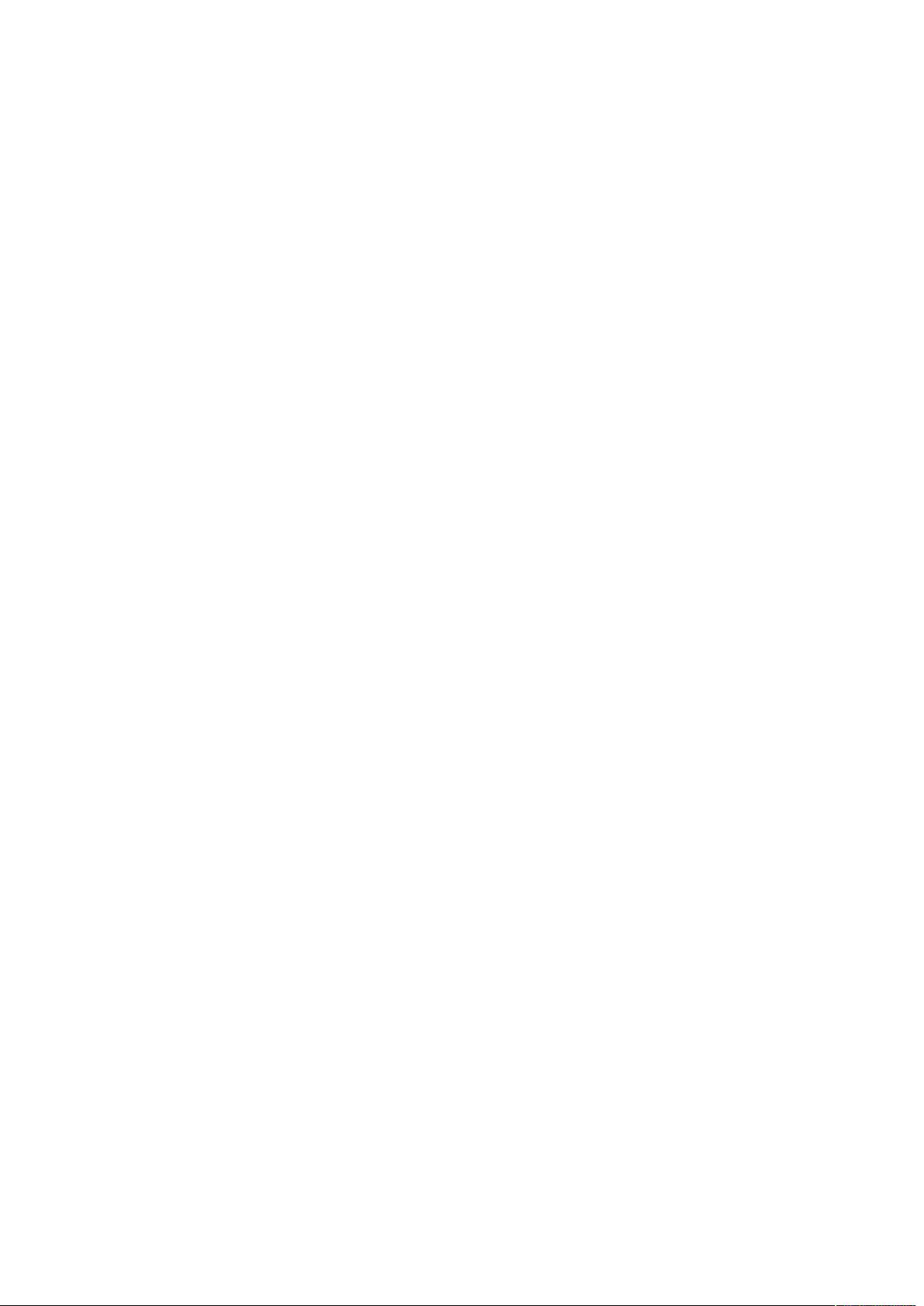
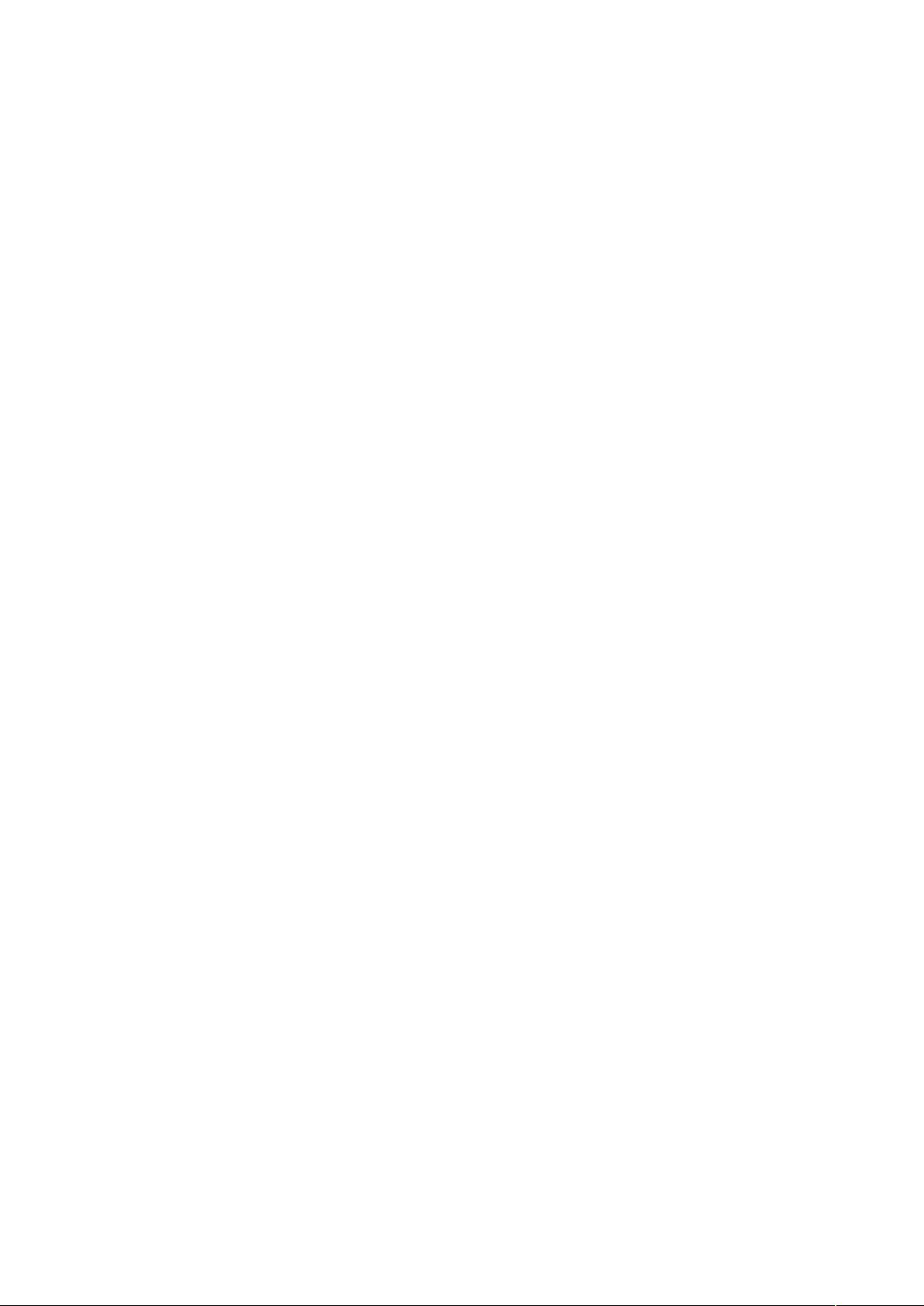
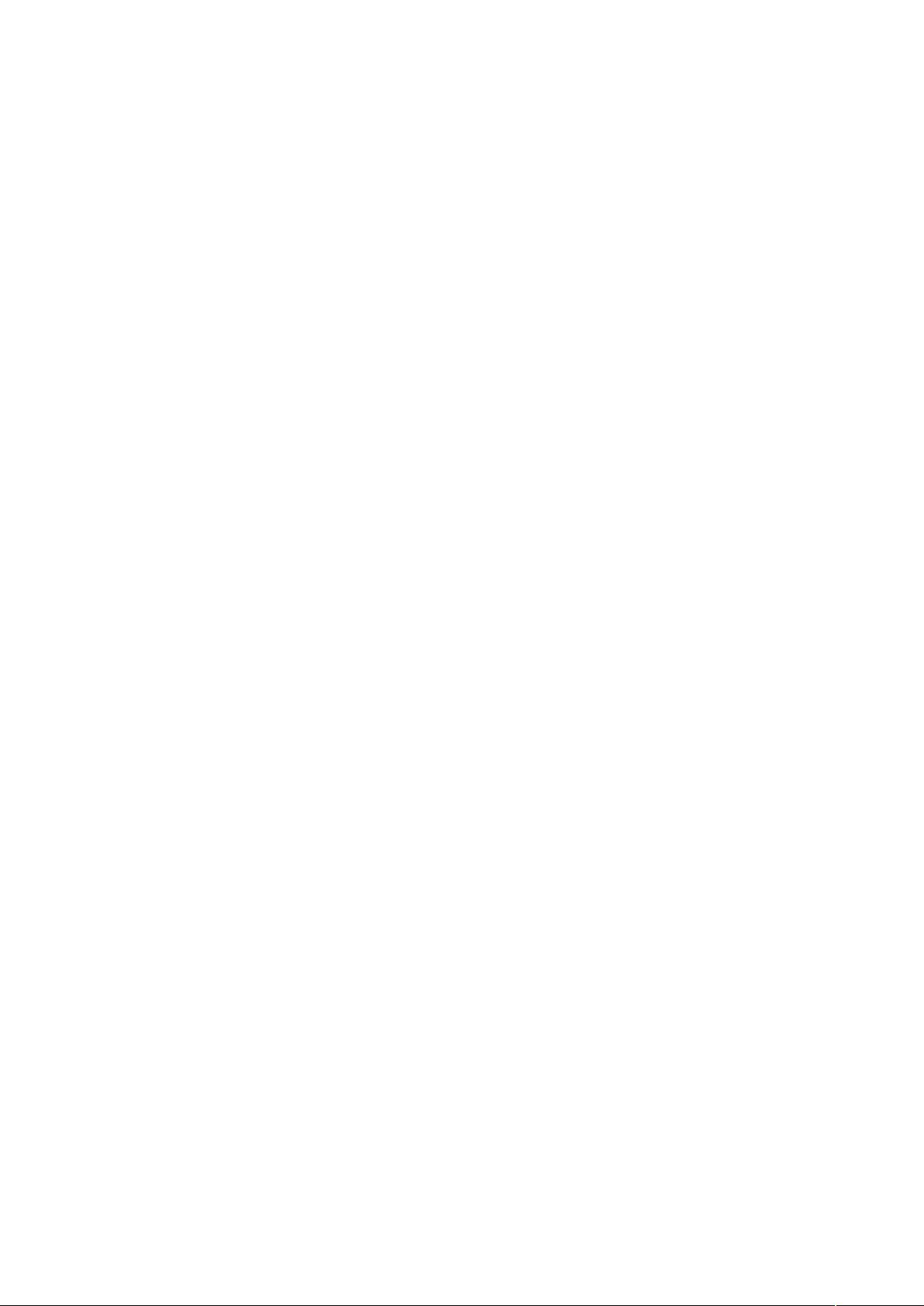
剩余63页未读,继续阅读
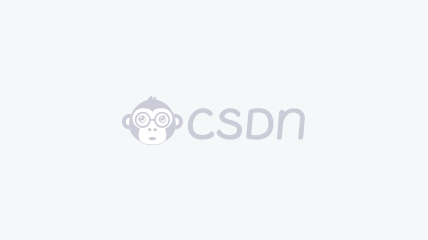
- mxj09262019-12-07资源还是不错的,就是解析的还不够清楚

- 粉丝: 8
- 资源: 6
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

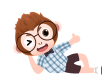
最新资源

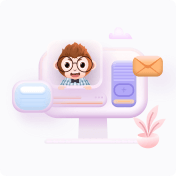
