//ov8825 the same with ov14825
/******************************************************************************
*
* Copyright 2010, Dream Chip Technologies GmbH. All rights reserved.
* No part of this work may be reproduced, modified, distributed, transmitted,
* transcribed, or translated into any language or computer format, in any form
* or by any means without written permission of:
* Dream Chip Technologies GmbH, Steinriede 10, 30827 Garbsen / Berenbostel,
* Germany
*
*****************************************************************************/
/**
* @file OV2685.c
*
* @brief
* ADD_DESCRIPTION_HERE
*
*****************************************************************************/
#include <ebase/types.h>
#include <ebase/trace.h>
#include <ebase/builtins.h>
#include <common/return_codes.h>
#include <common/misc.h>
#include "isi.h"
#include "isi_iss.h"
#include "isi_priv.h"
#include "OV2685_priv.h"
#define CC_OFFSET_SCALING 2.0f
#define I2C_COMPLIANT_STARTBIT 1U
/******************************************************************************
* local macro definitions
*****************************************************************************/
CREATE_TRACER( OV2685_INFO , "OV2685: ", INFO, 1U );
CREATE_TRACER( OV2685_WARN , "OV2685: ", WARNING, 1U );
CREATE_TRACER( OV2685_ERROR, "OV2685: ", ERROR, 1U );
CREATE_TRACER( OV2685_DEBUG, "OV2685: ", INFO, 1U );
CREATE_TRACER( OV2685_REG_INFO , "OV2685: ", INFO, 1);
CREATE_TRACER( OV2685_REG_DEBUG, "OV2685: ", INFO, 0U );
#define OV2685_SLAVE_ADDR 0x78U //0x20 /**< i2c slave address of the OV2685 camera sensor */
#define OV2685_SLAVE_ADDR2 0x20
#define OV2685_SLAVE_AF_ADDR 0x00U /**< i2c slave address of the OV2685 integrated AD5820 */
/******************************************************************************
* local variable declarations
*****************************************************************************/
const char OV2685_g_acName[] = "OV2685_ SOC_PARREL";
extern const IsiRegDescription_t OV2685_g_aRegDescription[];
extern const IsiRegDescription_t OV2685_g_svga[];
extern const IsiRegDescription_t OV2685_g_1600x1200[];
extern const IsiRegDescription_t OV2685_g_1920x1080[];
const IsiSensorCaps_t OV2685_g_IsiSensorDefaultConfig;
#define OV2685_I2C_START_BIT (I2C_COMPLIANT_STARTBIT) // I2C bus start condition
#define OV2685_I2C_NR_ADR_BYTES (2U) // 1 byte base address and 2 bytes sub address
#define OV2685_I2C_NR_DAT_BYTES (1U) // 8 bit registers
static uint16_t g_suppoted_mipi_lanenum_type = SUPPORT_MIPI_FOUR_LANE;
#define DEFAULT_NUM_LANES SUPPORT_MIPI_FOUR_LANE
/******************************************************************************
* local function prototypes
*****************************************************************************/
static RESULT OV2685_IsiCreateSensorIss( IsiSensorInstanceConfig_t *pConfig );
static RESULT OV2685_IsiReleaseSensorIss( IsiSensorHandle_t handle );
static RESULT OV2685_IsiGetCapsIss( IsiSensorHandle_t handle, IsiSensorCaps_t *pIsiSensorCaps );
static RESULT OV2685_IsiSetupSensorIss( IsiSensorHandle_t handle, const IsiSensorConfig_t *pConfig );
static RESULT OV2685_IsiSensorSetStreamingIss( IsiSensorHandle_t handle, bool_t on );
static RESULT OV2685_IsiSensorSetPowerIss( IsiSensorHandle_t handle, bool_t on );
static RESULT OV2685_IsiCheckSensorConnectionIss( IsiSensorHandle_t handle );
static RESULT OV2685_IsiGetSensorRevisionIss( IsiSensorHandle_t handle, uint32_t *p_value);
static RESULT OV2685_IsiGetGainLimitsIss( IsiSensorHandle_t handle, float *pMinGain, float *pMaxGain);
static RESULT OV2685_IsiGetIntegrationTimeLimitsIss( IsiSensorHandle_t handle, float *pMinIntegrationTime, float *pMaxIntegrationTime );
static RESULT OV2685_IsiExposureControlIss( IsiSensorHandle_t handle, float NewGain, float NewIntegrationTime, uint8_t *pNumberOfFramesToSkip, float *pSetGain, float *pSetIntegrationTime );
static RESULT OV2685_IsiGetCurrentExposureIss( IsiSensorHandle_t handle, float *pSetGain, float *pSetIntegrationTime );
static RESULT OV2685_IsiGetAfpsInfoIss ( IsiSensorHandle_t handle, uint32_t Resolution, IsiAfpsInfo_t* pAfpsInfo);
static RESULT OV2685_IsiGetGainIss( IsiSensorHandle_t handle, float *pSetGain );
static RESULT OV2685_IsiGetGainIncrementIss( IsiSensorHandle_t handle, float *pIncr );
static RESULT OV2685_IsiSetGainIss( IsiSensorHandle_t handle, float NewGain, float *pSetGain );
static RESULT OV2685_IsiGetIntegrationTimeIss( IsiSensorHandle_t handle, float *pSetIntegrationTime );
static RESULT OV2685_IsiGetIntegrationTimeIncrementIss( IsiSensorHandle_t handle, float *pIncr );
static RESULT OV2685_IsiSetIntegrationTimeIss( IsiSensorHandle_t handle, float NewIntegrationTime, float *pSetIntegrationTime, uint8_t *pNumberOfFramesToSkip );
static RESULT OV2685_IsiGetResolutionIss( IsiSensorHandle_t handle, uint32_t *pSetResolution );
static RESULT OV2685_IsiRegReadIss( IsiSensorHandle_t handle, const uint32_t address, uint32_t *p_value );
static RESULT OV2685_IsiRegWriteIss( IsiSensorHandle_t handle, const uint32_t address, const uint32_t value );
static RESULT OV2685_IsiGetCalibKFactor( IsiSensorHandle_t handle, Isi1x1FloatMatrix_t **pIsiKFactor );
static RESULT OV2685_IsiGetCalibPcaMatrix( IsiSensorHandle_t handle, Isi3x2FloatMatrix_t **pIsiPcaMatrix );
static RESULT OV2685_IsiGetCalibSvdMeanValue( IsiSensorHandle_t handle, Isi3x1FloatMatrix_t **pIsiSvdMeanValue );
static RESULT OV2685_IsiGetCalibCenterLine( IsiSensorHandle_t handle, IsiLine_t **ptIsiCenterLine);
static RESULT OV2685_IsiGetCalibClipParam( IsiSensorHandle_t handle, IsiAwbClipParm_t **pIsiClipParam );
static RESULT OV2685_IsiGetCalibGlobalFadeParam( IsiSensorHandle_t handle, IsiAwbGlobalFadeParm_t **ptIsiGlobalFadeParam);
static RESULT OV2685_IsiGetCalibFadeParam( IsiSensorHandle_t handle, IsiAwbFade2Parm_t **ptIsiFadeParam);
static RESULT OV2685_IsiGetIlluProfile( IsiSensorHandle_t handle, const uint32_t CieProfile, IsiIlluProfile_t **ptIsiIlluProfile );
static RESULT OV2685_IsiMdiInitMotoDriveMds( IsiSensorHandle_t handle );
static RESULT OV2685_IsiMdiSetupMotoDrive( IsiSensorHandle_t handle, uint32_t *pMaxStep );
static RESULT OV2685_IsiMdiFocusSet( IsiSensorHandle_t handle, const uint32_t Position );
static RESULT OV2685_IsiMdiFocusGet( IsiSensorHandle_t handle, uint32_t *pAbsStep );
static RESULT OV2685_IsiMdiFocusCalibrate( IsiSensorHandle_t handle );
static RESULT OV2685_IsiGetSensorMipiInfoIss( IsiSensorHandle_t handle, IsiSensorMipiInfo *ptIsiSensorMipiInfo);
static RESULT OV2685_IsiGetSensorIsiVersion( IsiSensorHandle_t handle, unsigned int* pVersion);
/*****************************************************************************/
/**
* OV2685_IsiCreateSensorIss
*
* @brief This function creates a new OV2685 sensor instance handle.
*
* @param pConfig configuration structure to create the instance
*
* @return Return the result of the function call.
* @retval RET_SUCCESS
* @retval RET_NULL_POINTER
* @retval RET_OUTOFMEM
*
*****************************************************************************/
static RESULT OV2685_IsiCreateSensorIss
(
IsiSensorInstanceConfig_t *pConfig
)
{
RESULT result = RET_SUCCESS;
OV2685_Context_t *pOV2685Ctx;
TRACE( OV2685_INFO, "%s (enter)\n", __FUNCTION__);
if ( (pConfig == NULL) || (pConfig->pSensor ==NULL) )
{
return ( RET_NULL_POINTER );
}
pOV2685Ctx = ( OV2685_Context_t * )malloc ( sizeof (OV2685_Context_t) );
if ( pOV2685Ctx == NULL )
{
TRACE( OV2685_ERROR, "%s: Can't allocate ov14825 context\n", __FUNCTION__ );
return ( RET_OUTOFMEM );
}
MEMSET( pOV2685Ctx, 0, sizeof( OV2685_Context_t ) );
result = HalAddRef( pConfig->HalHandle );
if ( result != RET_SUCCESS )
{
free ( pOV2685Ctx );
r
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
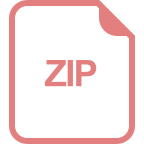
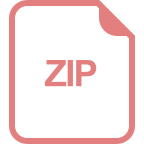
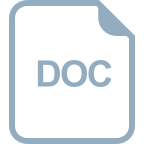
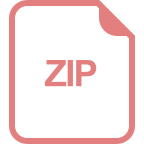
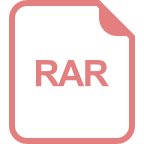
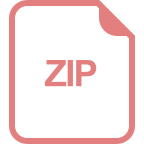
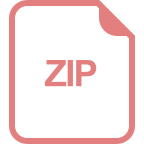
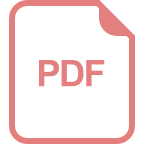
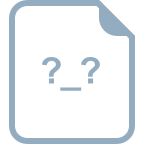
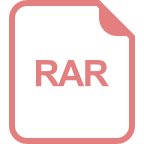
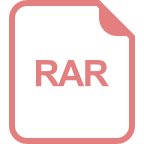
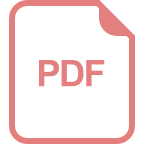
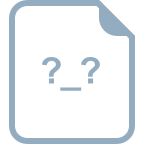
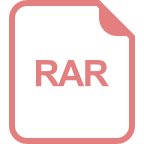
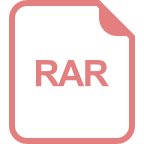
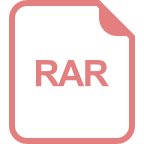
收起资源包目录


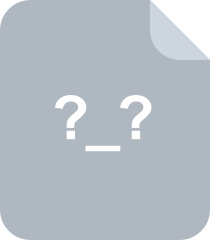
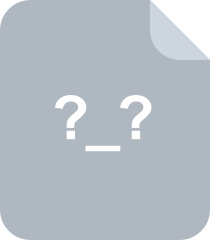
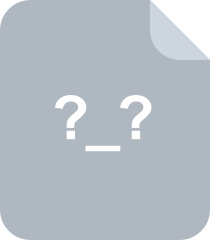
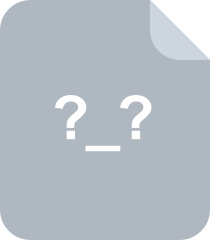

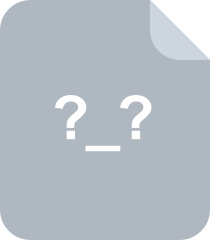
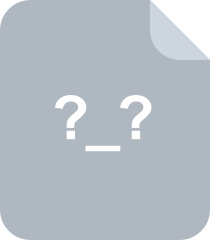
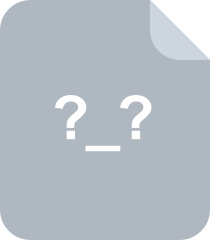
共 7 条
- 1
资源评论
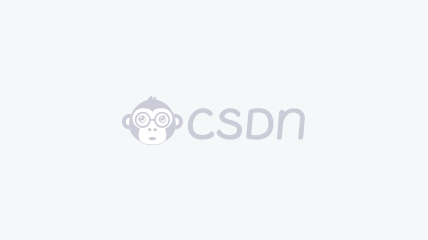
- 2543271732020-11-25作用不大,没有8918的驱动,仅仅是3288的mipi采集调试,且有没有出图还说不准
- binnary_012022-10-12垃圾 #毫无价值 #标题与内容不符
- HelloBirthday2019-07-22可以参考一下
- DYyanfa2023-09-26没看评论直接下载了,吃亏上当只有一次

空~。
- 粉丝: 9
- 资源: 13
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

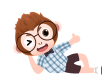
安全验证
文档复制为VIP权益,开通VIP直接复制
