# 一、实验名称:
超市商品管理系统链表实现
# 二、实验学时:4学时
# 三、实验目的:
1. 掌握单链表的定义和使用方法
2. 掌握单链表的建立方法
3. 掌握单链表中节点的查找与删除
4. 掌握输出单链表节点的方法
5. 掌握链表节点排序的一种方法
6. 掌握C语言创建菜单的方法
7. 掌握结构体的定义和使用方法
# 四、实验原理:
1、 单链表的数据操作
2、 基本语法的使用
3、 C语言文件操作函数的理解和使用
4、 Visual Studio开发环境
# 五、实验内容:
1、 创建单链表并将其定义为新类型 goodlist ,同时定义inventory为链表头节点。
2、 使用c语言文件操作函数fopen , fclose , fprintf , fscanf与fprintf_s,fscanf_s等打开指定txt文件,并读取txt文件内容。
3、 将读取到的内容录入到链表。
4、 输出超市系统封面(main函数)。

5、 完善链表操作函数
void insert(void);查重,确认无重复后,将新商品插入。

void search(void);查找所需函数,若没有则打印错误信息。
void update(void);寻找目标节点,提示用户输入新信息,若没有,打印错误信息


void deletes(void);提示用户输入信息,寻找目标信息,若存在,则删除,若不在则打印错误信息。


void print(void);将商品信息从头开始打印。

void bubblesort(void);冒泡排序。按照价格或者序号进行排序。


# 六、实验器材(设备、元器件):
个人电脑一台
# 七、实验步骤:
1、 阅读实验要求,明确实验目的
2、 查阅相关资料,为编写程序做好准备
3、 编写程序
4、 运行测试
5、 提交老师审查
# 八、实验结果与分析(含重要数据结果分析或核心代码流程分析)
### 代码1-1创建单链表并定义头节点
```cpp
typedef struct goods {
int number;
int price;
char name[MAX_NAME_LEN + 1];
char goods_discount[MAX_DISCOUNT_LEN + 1];
int on_hand;
struct goods *next;
}goodslist;
goodslist *inventory;
```
创建单链表并将其定义为新声明goodslist方便以后的使用,然后定义inventory为头节点。
### 代码1-2定义系统需要调用的函数
```cpp
//系统主体功能(单链表的操作)
void insert(void);
void search(void);
void update(void);
void deletes(void);
void print(void);
void bubblesort(void);
//文件操作函数及退出函数
void goodslist_init(void);
void save_to_file_exit(void);
void DestroyGoods(goodslist* inventory);
```
将系统分割为几大功能块,并将其定义为各个函数,精简main函数的同时,明确目标及操作流程。
### 代码1-3输出系统封面(即完善main函数)
```cpp
int main(void)
{
int choice;
goodslist_init();
while(1)
{
printf("Supermarket Goods Manage System\n");-
printf("********************************************\n");
printf("1. show all the goods:\n");
printf("2. update one good:\n");
printf("3. insert one good:\n");
printf("4. delete one good:\n");
printf("5. search one good:\n");
printf("6. save and exit:\n");
printf("7. bubble sort by price(optional question):\n");
printf("********************************************\n");
printf("enter your choice: ");
scanf_s("%d", &choice,20);
switch (choice)
{
case 1:print(); break;
case 2:update(); break;
case 3:insert(); break;
case 4:deletes(); break;
case 5:search(); break;
case 6:save_to_file_exit(); break;
case 7:bubblesort(); break;
default:printf("Please enter a right choise!"); break;
}
printf("\n");
}
}
```
使用根据系统特点,选择使用while(1)进行外圈大循环,swich函数进行内圈小循环,选择需要执行的函数。
### 代码2-1链表初始化
```cpp
void goodslist_init(void)
{
FILE *fp;
int count = 0;
goodslist *cur, *prev;
inventory = NULL;
prev = inventory;
cur = NULL;
if ((err = fopen_s(&fp,"goodsinfo.txt", "r")) != 0)
{
if ((err = fopen_s(&fp, "goodsinfo.txt", "w")) != 0)
{
printf("TIPS:CAN NOT CREAT GOODS DATABASE FILE \n");
return;
}
else
{
printf("TIPS: now there are 0 items in list\n");
printf("Please insert new one!\n");
}//若文件不存在,新建空文件并且让用户输入新的信息
}
else {
inventory = (goodslist*)malloc(sizeof(goodslist));
fscanf_s(fp, "%d", &inventory->number,20);
fscanf_s(fp, " %s", inventory->name,20);
fscanf_s(fp, " %d", &inventory->price,20);
fscanf_s(fp, " %s", inventory->goods_discount,20);
fscanf_s(fp, " %d\n", &inventory->on_hand,20);
inventory->next=NULL;
while (!feof(fp))
{
cur = (goodslist*)malloc(sizeof(goodslist));
for (prev = inventory;
prev->next != NULL;
prev = prev->next
);
if (cur == NULL) {
printf("Database is full; can't add more goods.\n");
return;
}
prev->next = cur;
cur->next = NULL;
fscanf_s(fp, "%d", &cur->number,20);
fscanf_s(fp, " %s",cur->name,20);
fscanf_s(fp, " %d", &cur->price,20);
fscanf_s(fp, " %s", cur->goods_discount,20);
fscanf_s(fp, " %d\n", &cur->on_hand,20);
prev = cur;
count++;
}
printf("TIPS: now there are %d items in list\n", count+1);
}
cur = NULL;
prev = NULL;
fclose(fp);
fp = NULL;
}
```
打开同目录下指定文件,先初始化首节点inventory,之后逐行读取文本内容并依次存入cur节点,使用prev指针寻找到链表尾,然后将cur节点接入到链表尾,每接入一次,count+1,最后打印时加上头节点
#### 2-2-1保存链表内容到文件并清空链表内存
```cpp
void save_to_file_exit(void)
{
goodslist *cur, *prev;
int savecount = 0;//计数
FILE *fp;
if ((err = fopen_s(&fp, "newgoodsinfo.txt", "w")) != 0)
{
printf("TIPS:can not open goods file database!\n");
return;
}
for (cur = inventory, prev = NULL;
cur != NULL;
prev = cur, cur = cur->next)
{
fprintf(fp, "%d", cur->number);
fprintf(fp, " %s", cur->name);
fprintf(fp, " %d",cur->price);
fprintf(fp, " %s", cur->goods_discount);
fprintf(fp, " %d\n",cur->on_hand);
savecount++;
}
fclose(fp);
fp = NULL;
DestroyGoods(inventory);
cur = NULL;
prev = NULL;
printf("TIPS:%d items save into newgoodsinfo.txt \n", savecount );
}
```
在相对目录下创建空txt文件,使用for循环,将链表内容依次打印到txt文件中,打印结束后使用DestroyGoods函数回收内存。
#### 2-2-2清空链表所占用内存
```cpp
void DestroyGoods(goodslist* inventory) {
if (!inventory) return;
goodslist *current, *prev;
current = inventory;
while (current->next!=NULL)
{
prev = current;
current = current->next;
free(prev);
}
prev = NULL;
free(current);
current = NULL;
}
```
依次清空链表占用的内存空间
### 代码2-3链表插入新节点
```cpp
void insert(void)
{
goodslist *current, *pre,*check;
pre = inventory;
current = (goodslist*)malloc(sizeof(goodsl
没有合适的资源?快使用搜索试试~ 我知道了~
基于C++实现的超市商品管理系统链表【100013042】
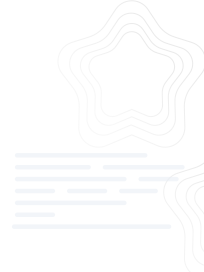
共17个文件
jpg:9个
bmp:2个
txt:1个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
1、 创建单链表并将其定义为新类型 goodlist ,同时定义inventory为链表头节点。 2、 使用c语言文件操作函数fopen , fclose , fprintf , fscanf与fprintf_s,fscanf_s等打开指定txt文件,并读取txt文件内容。 3、 将读取到的内容录入到链表。 4、 输出超市系统封面(main函数)。 5、 完善链表操作函数 void insert(void);查重,确认无重复后,将新商品插入。
资源推荐
资源详情
资源评论
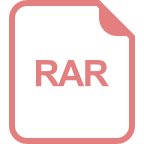
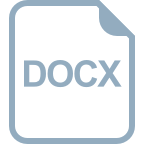
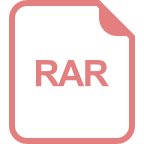
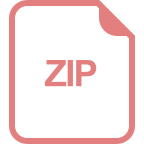
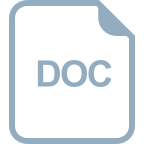
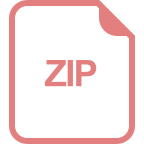
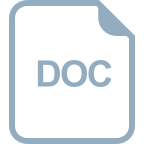
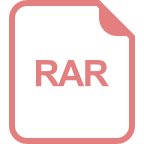
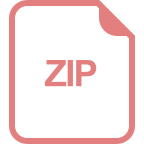
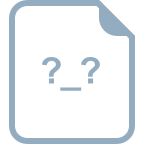
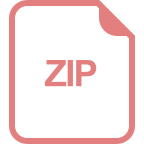
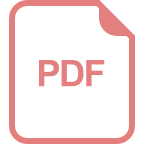
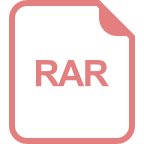
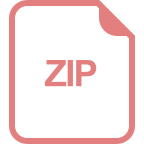
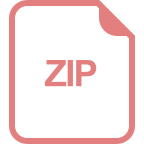
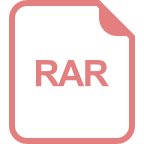
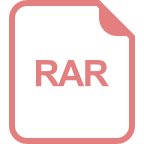
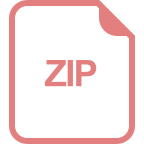
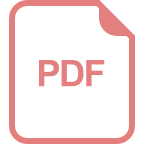
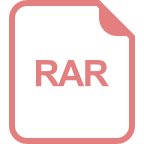
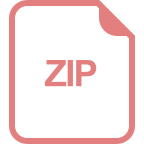
收起资源包目录


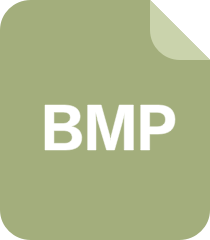
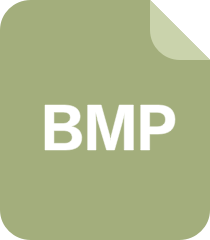
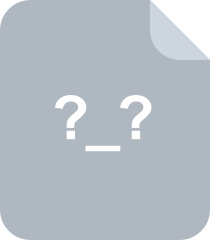
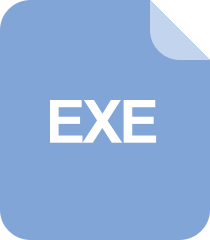
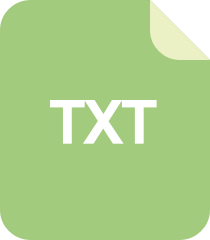
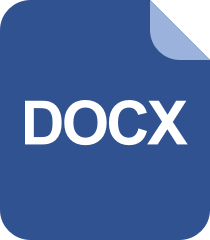

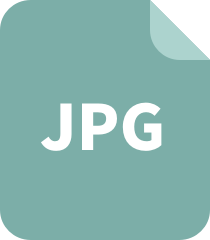
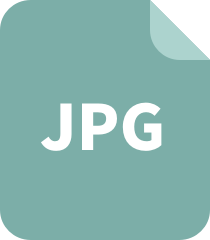
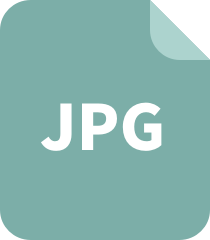
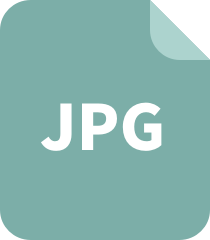
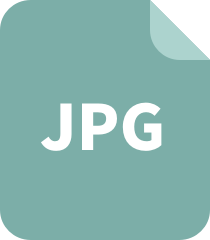
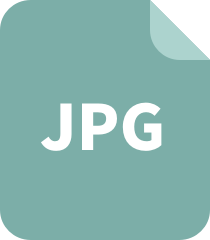
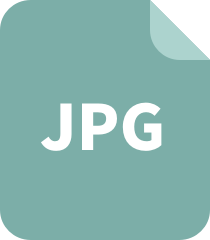
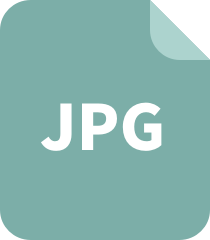
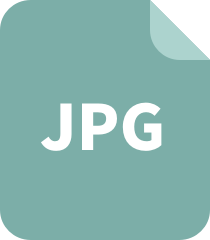
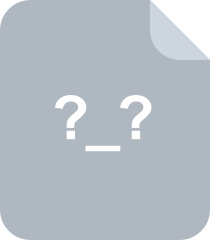
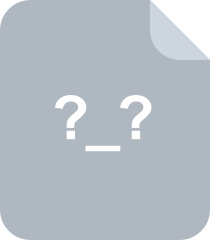
共 17 条
- 1
资源评论
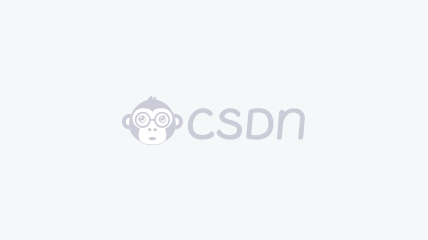
- 菜洛洛2023-10-25这个资源值得下载,资源内容详细全面,与描述一致,受益匪浅。
- azzz2023-10-31资源很实用,内容详细,值得借鉴的内容很多,感谢分享。
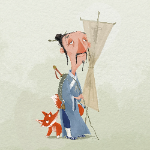
神仙别闹
- 粉丝: 3691
- 资源: 7461
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

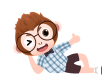
最新资源
- 云平台VPC.vsdx
- PIPE物理层接口规范:PCIe SATA USB3.1 DisplayPort 和 Converged IO 架构
- SparkSQL进阶操作相关数据
- java制作的小游戏,作为巩固java知识之用.zip
- Java语言写的围棋小游戏 半成品A Go game written in golang(Semi-finished).zip
- 基于Java-swing的俄罗斯方块游戏:源码+答辩文档+PPT.zip
- florr map详细版
- shiahdifhiahfiqefiwhfi weifwijfiwqufiqweefijeq0jfe
- registry-2.8.3<arm/amd>二进制文件
- Kotlin接口与抽象类详解及其应用
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


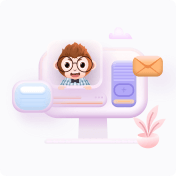
安全验证
文档复制为VIP权益,开通VIP直接复制
