1. Are there any memory errors in the following programs? If so, list all of them. Assume that the user enters in correct input, and that the sizes entered are at least one. Write your solution in a text or Word file and submit it below. void main() { char *str, *input; int *ilist; int i, size1, size2; printf("Number of letters in word: "); scanf("%d", &size1;); /* user inputs an integer */ printf("Number of integers: "); scanf("%d", &size2;); /* user inputs an integer */ str = (char *) malloc(size1); ilist = (int *) malloc(size2); printf("Word: "); scanf("%s", str); /* user inputs a string */ for(i = 0; i < size2; i++) { printf("Number %d of %d: ", i + 1, size2); scanf("%d", ilist + i); /* user inputs an integer */ } } 2. Are there any memory errors in the following program? If so, list all of them. Write your solution in a text or Word file and submit it below. /* return 1 if str is "1", 0 otherwise */ int checkIf1(char *str) { char *newstr = malloc(strlen(str) + 1); strcpy(newstr, str); /* set newstr to str */ if (strcmp(newstr, "1") == 0) { /* newstr is "1" */ return 1; } free(newstr); return 0; } void main() { char *strArr[4] = {"1", "2", "3", "4"}; int i; for(i = 0; i < 4; i++) { printf("%d\n", checkIf1(strArr[i])); } } 3. Are there any memory errors in the following program? If so, list all of them. Write your solution in a text or Word file and submit it below. struct data { char *str1, *str2; }; /* returns two strings concatenated if they are not the same, NULL otherwise */ char *mergeSingleIfDifferent(char *s1, char *s2) { char *str = (char *) malloc(strlen(s1) + strlen(s2) + 1); if (strcmp(s1, s2) == 0) { /* strings are equal */ str = NULL; } else { strcpy(str, s1); strcat(str, s2); } return str; } /* copies merged strings (or NULL) into array of strings passed in (results) */ void mergeArrayIfDifferent(char *results[], char *strA1[], char *strA2[], int size) { int i; for(i = 0; i < size; i++) { results[i] = mergeSingleIfDifferent(strA1[i], strA2[i]); } } void printAndFree(int c, char *str) { if (str != NULL) { printf("%d: %s\n", c, str); free(str); } } void main() { char *strArr1[8] = {"1", "2", "3", "4", "5", "6", "7", "8"}; char *strArr2[8] = {"a", "2", "c", "4", "e", "6", "g", "8"}; char *results[8]; int i; mergeArrayIfDifferent(results, strArr1, strArr2, 8); for(i = 0; i < 8; i++) { printAndFree(i, results); } } 内存错误是编程中常见的问题,特别是在C语言中,由于手动管理内存,很容易出现内存泄漏、内存越界等错误。在给定的三个程序段中,我们来逐一分析并解决这些问题。 1. 第一个程序中,存在内存泄漏的问题。在分配内存后没有进行释放。为避免内存泄漏,我们需要在不再使用分配的内存时调用`free()`函数。因此,对`main()`函数进行如下修改: ```c void main() { char *str, *input; int *ilist; int i, size1, size2; printf("Number of letters in word: "); scanf("%d", &size1); // 注意这里应该添加分号 printf("Number of integers: "); scanf("%d", &size2); // 同上 str = (char *) malloc(sizeof(char) * size1); ilist = (int *) malloc(sizeof(int) * size2); printf("Word: "); scanf("%s", str); for (i = 0; i < size2; i++) { printf("Number %d of %d: ", i + 1, size2); scanf("%d", ilist + i); } // 添加内存释放部分 free(str); str = NULL; free(ilist); ilist = NULL; } ``` 2. 第二个程序中,`checkIf1()`函数在字符串等于"1"时,没有释放`newstr`。这也会导致内存泄漏。修正后的代码如下: ```c int checkIf1(char *str) { char *newstr = malloc(strlen(str) + 1); strcpy(newstr, str); if (strcmp(newstr, "1") == 0) { free(newstr); // 添加释放内存的语句 return 1; } free(newstr); // 这里仍然需要释放,即使不等于"1" return 0; } void main() { char *strArr[4] = {"1", "2", "3", "4"}; int i; for (i = 0; i < 4; i++) { printf("%d\n", checkIf1(strArr[i])); } } ``` 3. 第三个程序中,存在两个问题。`printAndFree()`函数的参数应该是指向字符串的指针,而不是数组本身。在`mergeSingleIfDifferent()`函数中,当字符串相等时,直接将`str`设为`NULL`,导致无法释放内存。修正后的代码如下: ```c struct data { char *str1, *str2; }; char *mergeSingleIfDifferent(char *s1, char *s2) { char *str = (char *) malloc(strlen(s1) + strlen(s2) + 1); if (strcmp(s1, s2) == 0) { free(str); return NULL; } strcpy(str, s1); strcat(str, s2); return str; } // 修改参数类型为指针数组 void mergeArrayIfDifferent(char **results, char *strA1[], char *strA2[], int size) { int i; for (i = 0; i < size; i++) { results[i] = mergeSingleIfDifferent(strA1[i], strA2[i]); } } void printAndFree(int c, char *str) { if (str != NULL) { printf("%d: %s\n", c, str); free(str); } } void main() { char *strArr1[8] = {"1", "2", "3", "4", "5", "6", "7", "8"}; char *strArr2[8] = {"a", "2", "c", "4", "e", "6", "g", "8"}; char **results = malloc(sizeof(char *) * 8); int i; mergeArrayIfDifferent(results, strArr1, strArr2, 8); for (i = 0; i < 8; i++) { printAndFree(i, results[i]); } // 不要忘记释放results数组 for (i = 0; i < 8; i++) { free(results[i]); } free(results); results = NULL; } ``` 以上是针对三个程序段中内存错误的详细分析和修正方案。在编程时,尤其是使用C语言,要时刻注意内存管理,确保在使用完内存分配的区域后及时释放,以避免内存泄漏和其他相关的内存问题。
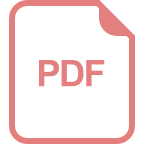
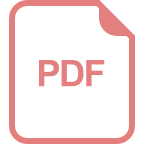
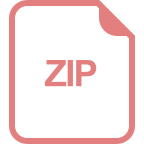
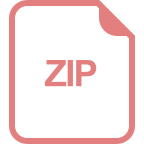
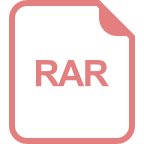
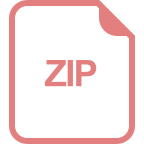
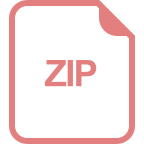
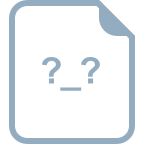
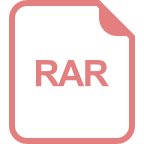
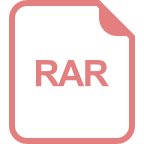
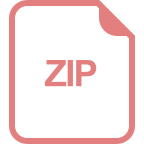
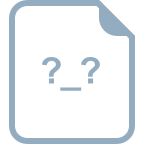
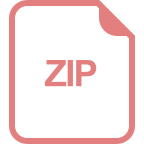
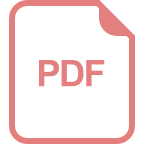
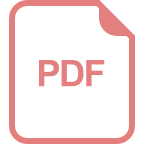
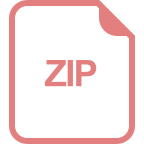
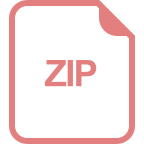
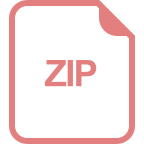
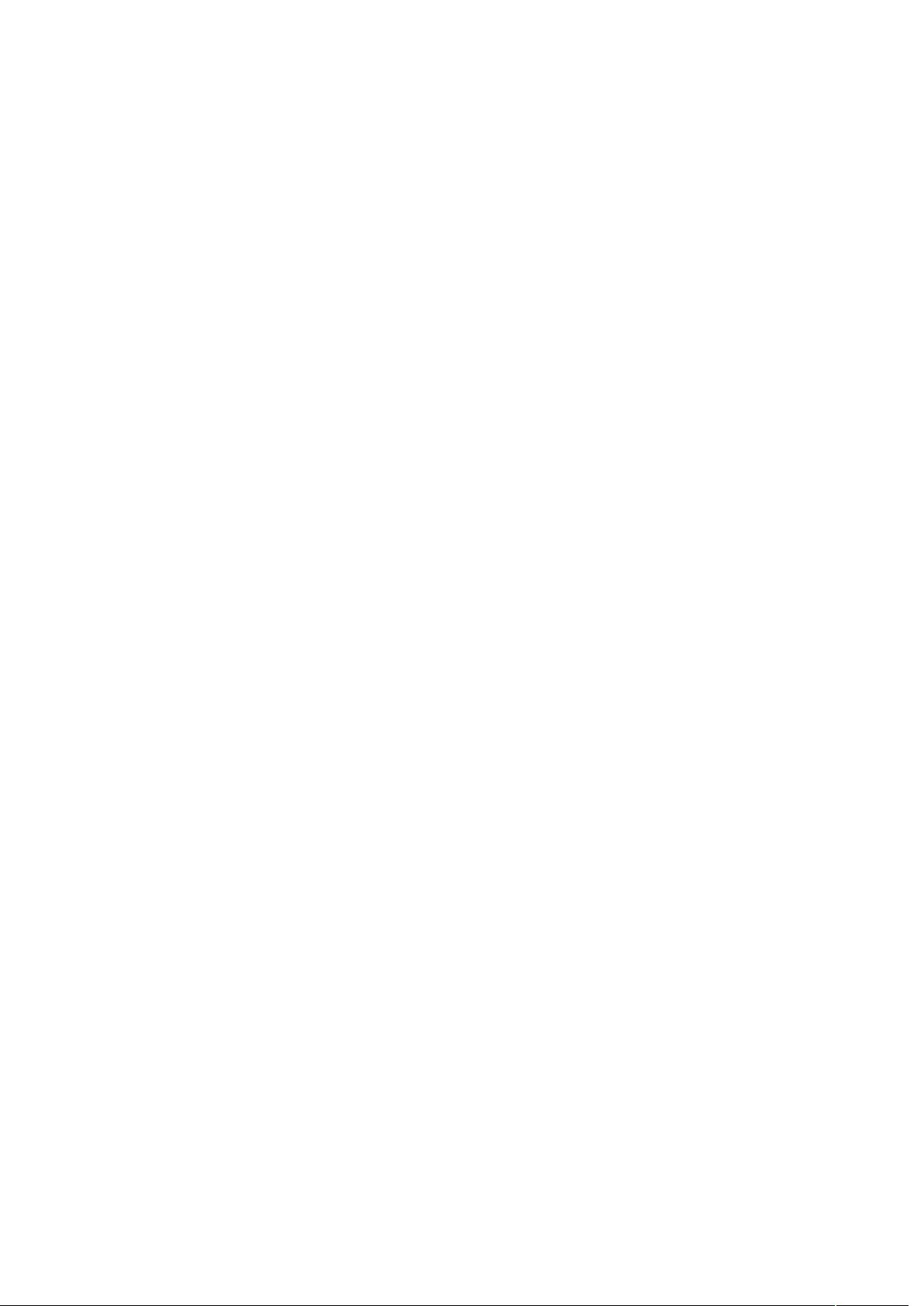
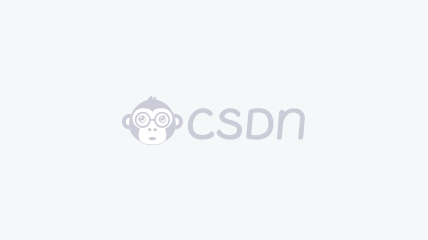
- yp_xiaoliu2013-11-26还行,可以用,不过没好全面
- xuying1234567892013-11-19还行吧。能用。
- wuzhixi49352013-11-21不大全面,但还好吧
- legend00662014-11-02有很大的帮助。

- 粉丝: 1
- 资源: 3
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

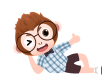
最新资源
- 【java毕业设计】springboot医学电子技术线上课堂系统(springboot+vue+mysql+说明文档).zip
- java 输入任意字符串找回文
- NewModel_3.2.2(1).zip
- 上海交通大学版 asp.NET第152页-运用ADO.NET访问数据库(注册账号并在网站中查询)
- 【源码+数据库】利用Java Swing框架与Socket技术开发的即时通讯系统,系统分为客户端和服务端,类似于qq聊天
- 计算机科学与技术数据结构实践考核要求.ppt
- 【java毕业设计】springboot中医院问诊系统的设计与实现(springboot+vue+mysql+说明文档).zip
- MATLAB大数计算工具箱及其用法
- 基于 python 实现的微博的数据挖掘与社交舆情分析
- Screenshot_20241105_140450.jpg

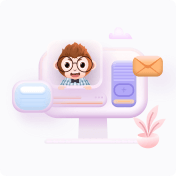
