VC调用ffmpeg步骤及示例代码
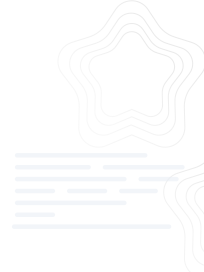


FFmpeg是一个开源项目,提供了音频、视频处理的强大工具和库,包括编码、解码、转换、流媒体等功能。在VC++环境下,通过调用FFmpeg的API,我们可以实现各种音视频处理任务。以下是对"VC调用ffmpeg步骤及示例代码"的详细解释: 一、调用FFmpeg的步骤 1. **下载与编译FFmpeg**: 你需要从FFmpeg的官方网站(https://ffmpeg.org/download.html)下载源码,并根据你的VC++版本选择合适的构建选项进行编译。确保在编译时包含动态库(.dll)和静态库(.lib)。 2. **配置项目设置**: 在VC++项目中,添加编译好的FFmpeg库路径到“附加包含目录”中,同时将对应的.lib文件添加到“附加依赖项”。 3. **引入头文件**: 在需要调用FFmpeg的源文件中,引入必要的头文件,例如`#include <libavformat/avformat.h>`、`#include <libavcodec/avcodec.h>`等。 4. **初始化FFmpeg**: 在程序开始处,需要调用`av_register_all()`和`avformat_network_init()`来注册所有编解码器和初始化网络功能。 5. **打开输入文件**: 使用`avformat_open_input()`函数打开一个视频文件,然后使用`avformat_find_stream_info()`获取文件中的流信息。 6. **解析流信息**: 通过`av_find_best_stream()`找到最合适的视频或音频流,然后获取相应的解码器上下文`AVCodecContext`。 7. **解码数据**: 加载解码器,如`avcodec_find_decoder()`,然后使用`avcodec_open2()`打开解码器。接着,可以使用`av_read_frame()`读取一帧数据,然后`avcodec_decode_video2()`或`avcodec_decode_audio4()`进行解码。 8. **处理解码后的数据**: 对于视频,你可以通过`av_frame_get_buffer()`创建一个`AVFrame`对象,然后使用`avcodec_decode_video2()`解码后将结果保存到`AVFrame`中。对于音频,可以处理解码后的PCM数据。 9. **渲染或保存结果**: 对于视频,可以将`AVFrame`的数据转换成BMP或其他图像格式。对于音频,可以播放或保存到文件。 10. **清理资源**: 程序结束时,记得释放所有分配的资源,包括`AVFormatContext`、`AVCodecContext`、`AVFrame`等,并调用`avformat_close_input()`关闭输入文件。 二、示例代码 以下是一个简单的将视频文件转为BMP图片的VC++代码片段: ```cpp #include <libavformat/avformat.h> #include <libavcodec/avcodec.h> #include <libswscale/swscale.h> // ... 初始化FFmpeg部分 ... int main() { AVFormatContext* pFormatCtx = NULL; avformat_open_input(&pFormatCtx, "input.mp4", NULL, NULL); avformat_find_stream_info(pFormatCtx, NULL); AVStream* videoStream = av_find_best_stream(pFormatCtx, AVMEDIA_TYPE_VIDEO, -1, -1, NULL, 0); AVCodecContext* pCodecCtx = videoStream->codec; AVCodec* pCodec = avcodec_find_decoder(pCodecCtx->codec_id); avcodec_open2(pCodecCtx, pCodec, NULL); AVFrame* pFrame = av_frame_alloc(); AVPacket packet; while (av_read_frame(pFormatCtx, &packet) >= 0) { if (packet.stream_index == videoStream->index) { avcodec_decode_video2(pCodecCtx, pFrame, NULL, &packet); if (pFrame->data[0]) { // 创建色彩空间转换上下文 SwsContext* swsCtx = sws_getCachedContext(NULL, pCodecCtx->width, pCodecCtx->height, pCodecCtx->pix_fmt, pCodecCtx->width, pCodecCtx->height, AV_PIX_FMT_BGR24, SWS_BICUBIC, NULL, NULL, NULL); // 转换颜色空间 uint8_t* outBuffer = new uint8_t[pCodecCtx->width * pCodecCtx->height * 3]; AVFrame* pOutFrame = av_frame_alloc(); avpicture_fill((AVPicture*)pOutFrame, outBuffer, AV_PIX_FMT_BGR24, pCodecCtx->width, pCodecCtx->height); sws_scale(swsCtx, pFrame->data, pFrame->linesize, 0, pCodecCtx->height, pOutFrame->data, pOutFrame->linesize); // 保存为BMP文件 SaveAsBMP("output.bmp", pOutFrame->data[0], pCodecCtx->width, pCodecCtx->height, pOutFrame->linesize[0]); // 清理 sws_freeContext(swsCtx); av_frame_free(&pOutFrame); delete[] outBuffer; } } av_free_packet(&packet); } // ... 清理部分 ... return 0; } // 自定义的保存BMP函数 void SaveAsBMP(char* filename, uint8_t* data, int width, int height, int stride) { // 实现代码... } ``` 以上代码展示了如何在VC++中使用FFmpeg读取视频文件,解码每一帧为BMP图片并保存。请注意,`SaveAsBMP`函数需要根据实际需求实现,以将BGR24格式的数据保存为BMP文件。 这个例子只是FFmpeg调用的一个基础示例,实际上FFmpeg可以进行更复杂的操作,如音频处理、视频编码、流媒体传输等。通过深入学习FFmpeg的API和文档,你可以实现更多自定义的功能。
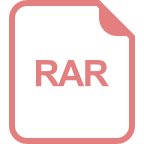
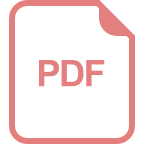
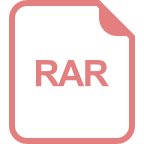
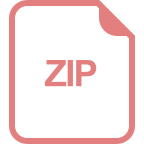
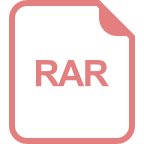
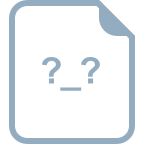
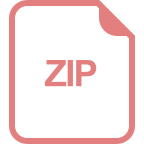
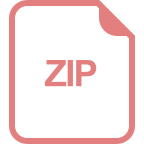
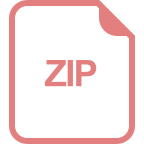
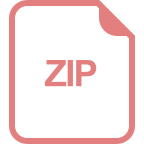
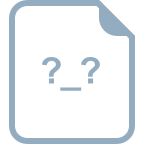
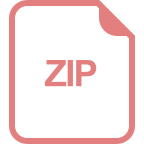
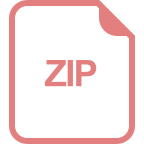
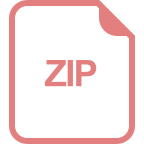
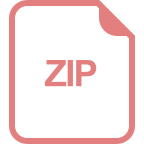
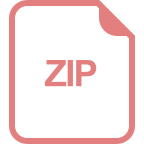
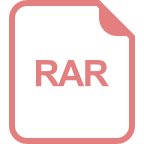
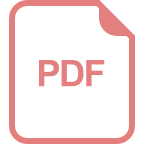
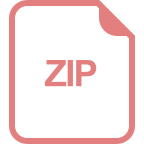
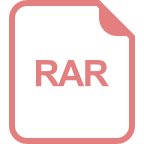

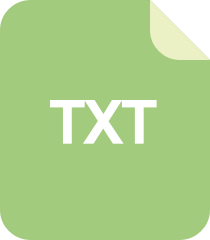
- 1

- 粉丝: 11
- 资源: 13
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

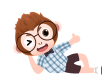
最新资源

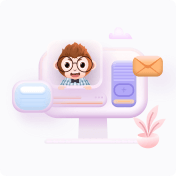

- 1
- 2
- 3
- 4
- 5
前往页