package org.json;
/*
Copyright (c) 2002 JSON.org
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
The Software shall be used for Good, not Evil.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
*/
import java.io.IOException;
import java.io.StringWriter;
import java.io.Writer;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.util.Collection;
import java.util.Enumeration;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Locale;
import java.util.Map;
import java.util.ResourceBundle;
/**
* A JSONObject is an unordered collection of name/value pairs. Its external
* form is a string wrapped in curly braces with colons between the names and
* values, and commas between the values and names. The internal form is an
* object having <code>get</code> and <code>opt</code> methods for accessing the
* values by name, and <code>put</code> methods for adding or replacing values
* by name. The values can be any of these types: <code>Boolean</code>,
* <code>JSONArray</code>, <code>JSONObject</code>, <code>Number</code>,
* <code>String</code>, or the <code>JSONObject.NULL</code> object. A JSONObject
* constructor can be used to convert an external form JSON text into an
* internal form whose values can be retrieved with the <code>get</code> and
* <code>opt</code> methods, or to convert values into a JSON text using the
* <code>put</code> and <code>toString</code> methods. A <code>get</code> method
* returns a value if one can be found, and throws an exception if one cannot be
* found. An <code>opt</code> method returns a default value instead of throwing
* an exception, and so is useful for obtaining optional values.
* <p>
* The generic <code>get()</code> and <code>opt()</code> methods return an
* object, which you can cast or query for type. There are also typed
* <code>get</code> and <code>opt</code> methods that do type checking and type
* coercion for you. The opt methods differ from the get methods in that they do
* not throw. Instead, they return a specified value, such as null.
* <p>
* The <code>put</code> methods add or replace values in an object. For example,
*
* <pre>
* myString = new JSONObject().put("JSON", "Hello, World!").toString();
* </pre>
*
* produces the string <code>{"JSON": "Hello, World"}</code>.
* <p>
* The texts produced by the <code>toString</code> methods strictly conform to
* the JSON syntax rules. The constructors are more forgiving in the texts they
* will accept:
* <ul>
* <li>An extra <code>,</code> <small>(comma)</small> may appear just
* before the closing brace.</li>
* <li>Strings may be quoted with <code>'</code> <small>(single
* quote)</small>.</li>
* <li>Strings do not need to be quoted at all if they do not begin with a quote
* or single quote, and if they do not contain leading or trailing spaces, and
* if they do not contain any of these characters:
* <code>{ } [ ] / \ : , = ; #</code> and if they do not look like numbers and
* if they are not the reserved words <code>true</code>, <code>false</code>, or
* <code>null</code>.</li>
* <li>Keys can be followed by <code>=</code> or <code>=></code> as well as by
* <code>:</code>.</li>
* <li>Values can be followed by <code>;</code> <small>(semicolon)</small> as
* well as by <code>,</code> <small>(comma)</small>.</li>
* </ul>
*
* @author JSON.org
* @version 2012-07-02
*/
public class JSONObject {
/**
* JSONObject.NULL is equivalent to the value that JavaScript calls null,
* whilst Java's null is equivalent to the value that JavaScript calls
* undefined.
*/
private static final class Null {
/**
* There is only intended to be a single instance of the NULL object,
* so the clone method returns itself.
* @return NULL.
*/
protected final Object clone() {
return this;
}
/**
* A Null object is equal to the null value and to itself.
* @param object An object to test for nullness.
* @return true if the object parameter is the JSONObject.NULL object
* or null.
*/
public boolean equals(Object object) {
return object == null || object == this;
}
/**
* Get the "null" string value.
* @return The string "null".
*/
public String toString() {
return "null";
}
}
/**
* The map where the JSONObject's properties are kept.
*/
private final Map map;
/**
* It is sometimes more convenient and less ambiguous to have a
* <code>NULL</code> object than to use Java's <code>null</code> value.
* <code>JSONObject.NULL.equals(null)</code> returns <code>true</code>.
* <code>JSONObject.NULL.toString()</code> returns <code>"null"</code>.
*/
public static final Object NULL = new Null();
/**
* Construct an empty JSONObject.
*/
public JSONObject() {
this.map = new HashMap();
}
/**
* Construct a JSONObject from a subset of another JSONObject.
* An array of strings is used to identify the keys that should be copied.
* Missing keys are ignored.
* @param jo A JSONObject.
* @param names An array of strings.
* @throws JSONException
* @exception JSONException If a value is a non-finite number or if a name is duplicated.
*/
public JSONObject(JSONObject jo, String[] names) {
this();
for (int i = 0; i < names.length; i += 1) {
try {
this.putOnce(names[i], jo.opt(names[i]));
} catch (Exception ignore) {
}
}
}
/**
* Construct a JSONObject from a JSONTokener.
* @param x A JSONTokener object containing the source string.
* @throws JSONException If there is a syntax error in the source string
* or a duplicated key.
*/
public JSONObject(JSONTokener x) throws JSONException {
this();
char c;
String key;
if (x.nextClean() != '{') {
throw x.syntaxError("A JSONObject text must begin with '{'");
}
for (;;) {
c = x.nextClean();
switch (c) {
case 0:
throw x.syntaxError("A JSONObject text must end with '}'");
case '}':
return;
default:
x.back();
key = x.nextValue().toString();
}
// The key is followed by ':'. We will also tolerate '=' or '=>'.
c = x.nextClean();
if (c == '=') {
if (x.next() != '>') {
x.back();
}
} else if (c != ':') {
throw x.syntaxError("Expected a ':' after a key");
}
this.putOnce(key, x.nextValue());
// Pairs are separated by ','. We will also tolerate ';'.
switch (x.nextClean()) {
case ';':
ca
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论












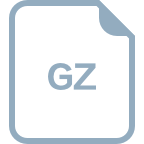












收起资源包目录



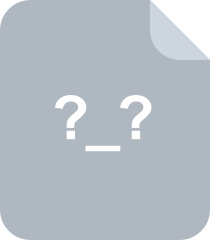
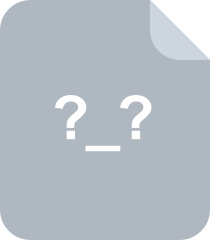
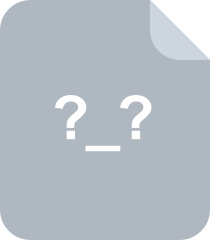
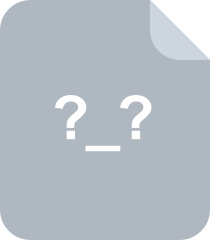

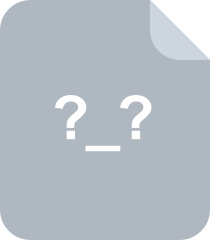


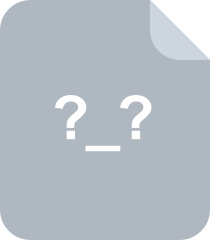
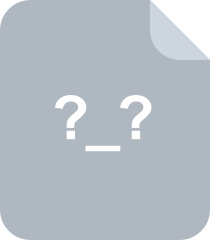
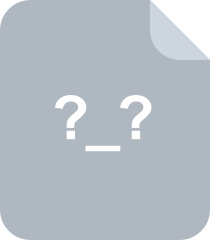
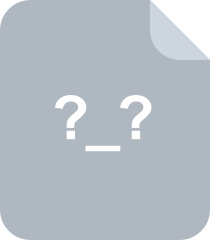
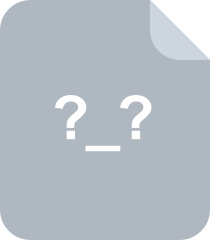
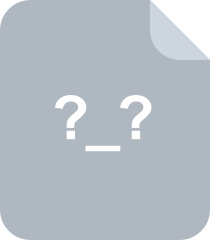
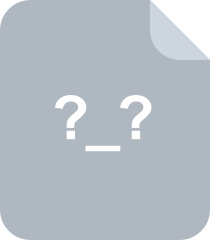
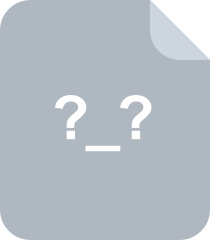
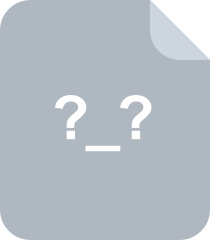
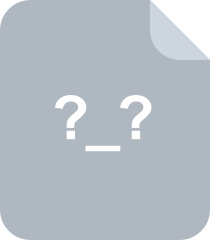
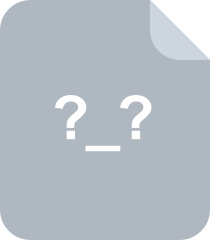
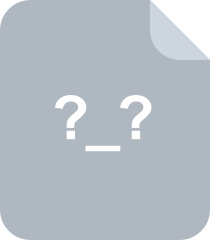
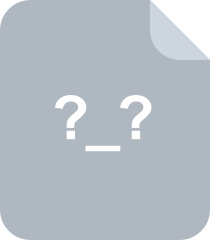
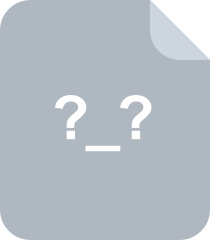
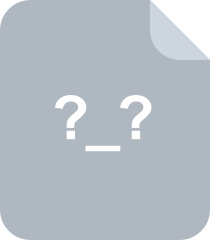
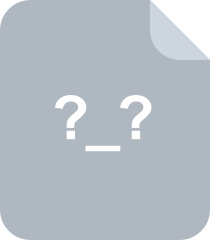


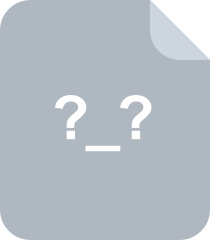
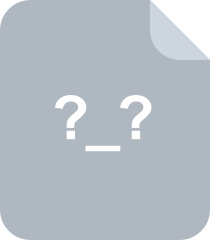

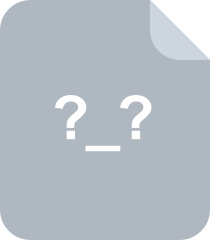
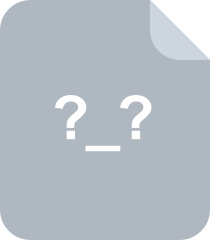
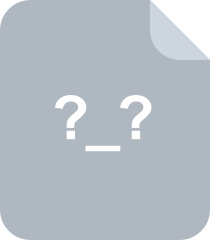
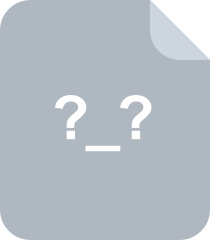
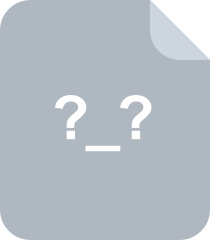

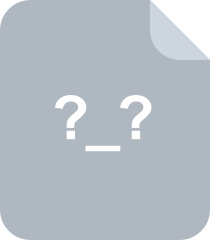

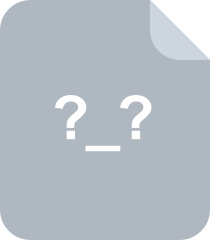

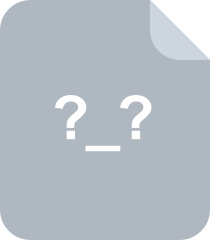
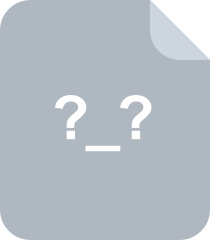
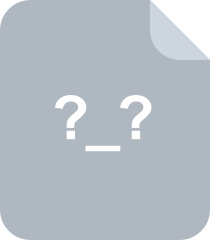


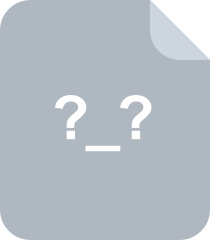

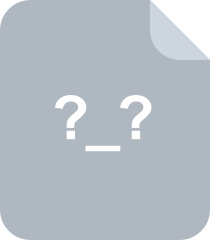

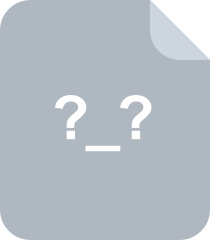


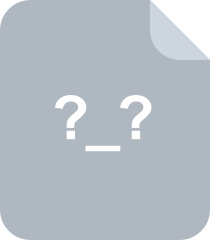
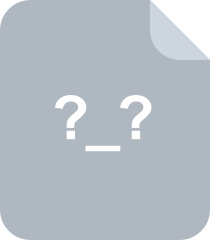
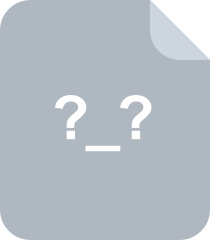
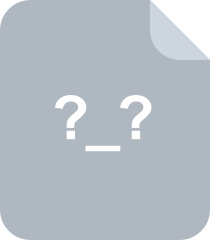
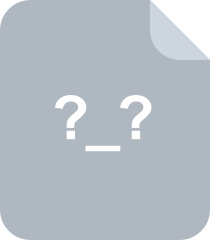
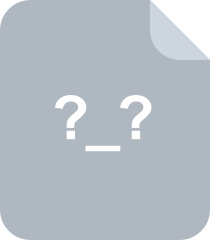
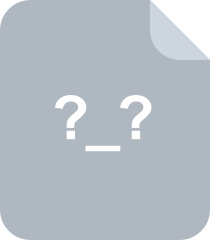
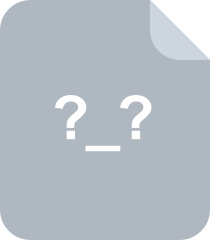
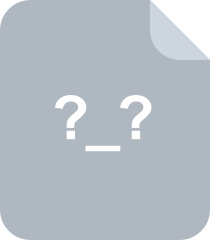
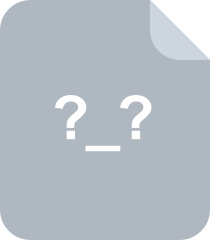
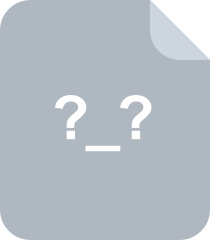
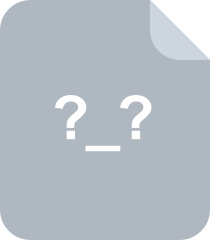
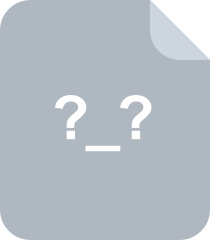
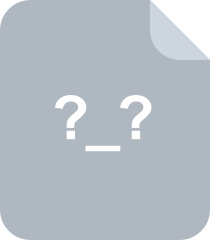
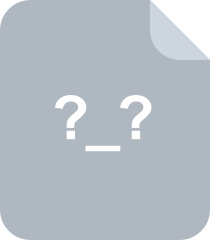
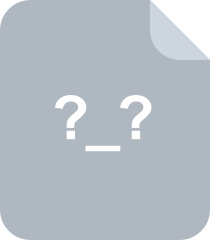
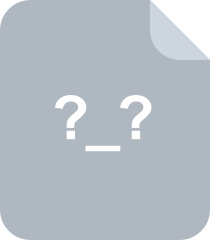


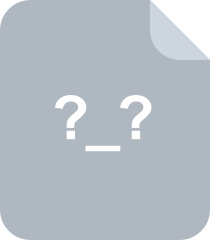
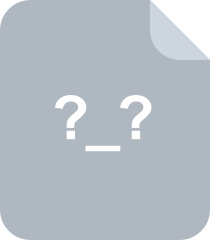

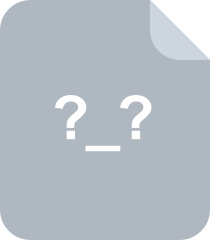
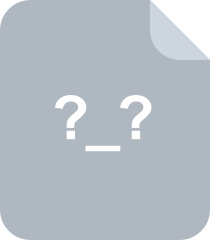
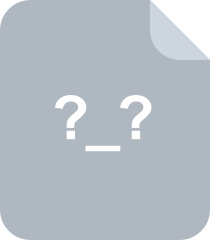
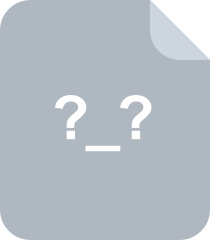
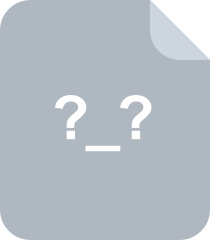

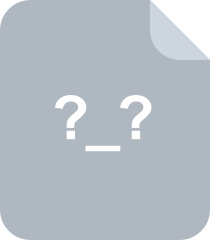

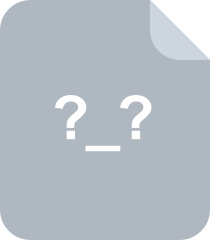

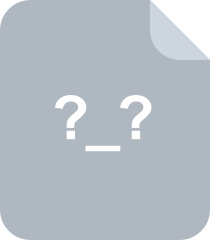
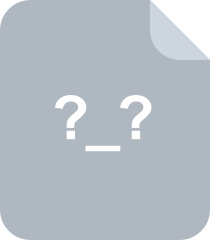

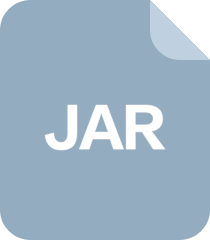
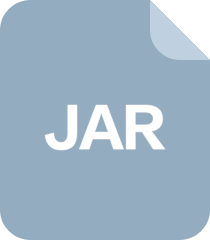
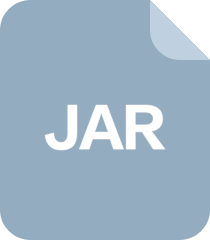
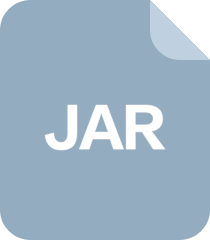
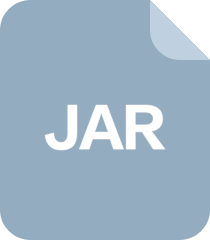
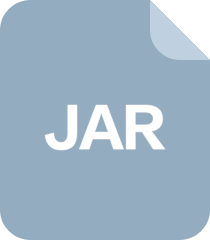
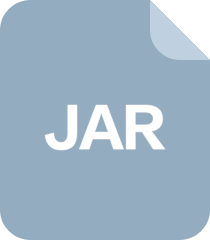
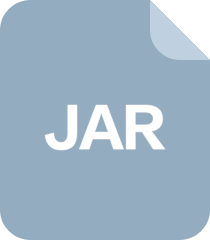
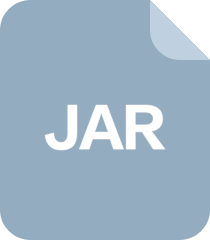
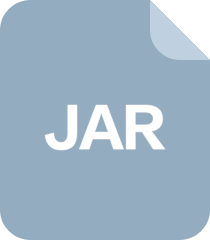
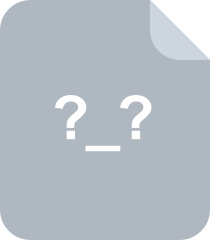

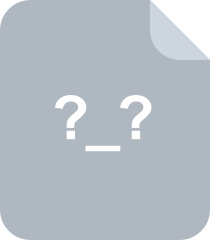
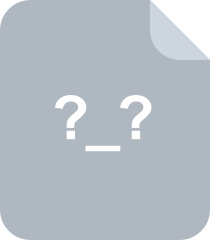
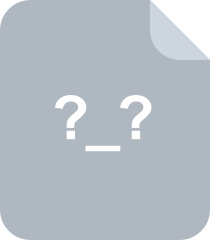

共 78 条
- 1
资源评论
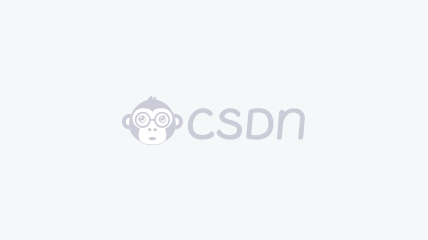
- #完美解决问题
- #运行顺畅
- #内容详尽
- #全网独家
- #注释完整

Qsword555
- 粉丝: 1
- 资源: 7
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

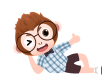
最新资源
- 基于Opencv和Filterpy实现YOLOV3-SORT车辆跟踪与车流统计算法.zip
- 基于Vue的微信小程序设计源码
- 基于opencv设计的人脸识别考勤系统.zip
- 使用SSM设计的在线视频播放项目(包括前后台).zip
- 基于C++和qt的Graphics框架编写.zip
- 基于vue.js设计的摄影师社交平台网站.zip
- 基于 SSM 框架 JavaEE实现分布式爬虫新闻聚合网站.zip
- 基于微信小程序的游泳馆在线预约与管理设计源码
- 基于SSM框架的预约挂号系统设计与实现源码
- 利用svm进行对银杏树的分类,机器学习作业.zip
- 基于SSM设计的外卖系统.zip
- 基于JSP+Servlet+Tomcat的电子图书管理系统设计源码
- 自己写的MVC框架 搭建的GMS系统 包括权限,模块,统计,接口等等...zip
- KTV系统,C#前后台,Android客户端。播放器使用迅雷开源APlayer播放引擎。.zip
- 安卓程序分析系统.zip
- 基于Html和Vue的抖音微信APP短剧系统设计源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


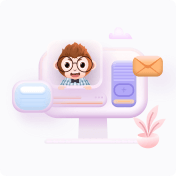
安全验证
文档复制为VIP权益,开通VIP直接复制
