package com.queue;
import java.util.Scanner;
public class ArrayQueueDemo {
public static void main(String[] args) {
//创建队列
ArrayQueue arrayQueue = new ArrayQueue(3);
char key = ' '; //接收用户输入
Scanner scanner = new Scanner(System.in);
boolean loop = true;
//输出一个菜单
while(loop){
System.out.println("s(show) : 显示队列");
System.out.println("e(exit) : 退出程序");
System.out.println("a(add) : 添加数据");
System.out.println("g(get)) : 取出数据");
System.out.println("h(head) : 查看队列头数据");
key = scanner.next().charAt(0);
switch (key){
case 's':
arrayQueue.showQueue();
break;
case 'a':
System.out.println("请输入一个数字:");
int value = scanner.nextInt();
arrayQueue.addQueue(value);
break;
case 'g':
try{
int res = arrayQueue.getQueue();
System.out.printf("取出的数据是%d\n",res);
}catch (Exception e){
System.out.println(e.getMessage());
}
break;
case 'h':
try{
int res = arrayQueue.headQueue();
System.out.printf("队列头的数据是%d\n",res);
}catch (Exception e){
System.out.println(e.getMessage());
}
break;
case 'e':
scanner.close();
loop = false;
break;
default:
break;
}
}
System.out.println("程序退出");
}
}
//使用数组模拟队列
class ArrayQueue{
private int maxSize; //数组最大容量
private int front; //队列头
private int rear; //队列尾
private int[] arr; //存放数据,模拟队列
//创建队列的构造齐全
public ArrayQueue(int arrMaxsize){
maxSize = arrMaxsize;
arr = new int[maxSize];
front = -1; //指向队列头部,队列头的前一个位置
rear = -1; //指向队列尾,包含队列尾的数据
}
//判断队列是否满
public boolean isFull(){
return rear == maxSize - 1;
}
//判断队列是否为空
public boolean isEmpty(){
return rear == front;
}
//添加数据到队列
public void addQueue(int n){
if(isFull()){
System.out.println("队列满,不能加入");
return;
}
rear++; //rear后移
arr[rear] = n;
}
//获取队列的数据,出队列
public int getQueue(){
//判断队列是否为空
if(isEmpty()){
//抛异常
throw new RuntimeException("队列空,不能取数据");
}
front++; //front后移
return arr[front];
}
//显示队列的所有数据
public void showQueue(){
if(isEmpty()){
System.out.println("队列空,无法读取数据");
return;
}
//遍历
for(int i=0;i<arr.length;i++){
System.out.printf("arr[%d] = %d\n",i,arr[i]);
}
}
//显示队列的头数据
public int headQueue(){
if(isEmpty()){
System.out.println("队列为空");
throw new RuntimeException("队列空");
}
return arr[front + 1];
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
尚硅谷Java数据结构与java算法(Java数据结构与算法).zip
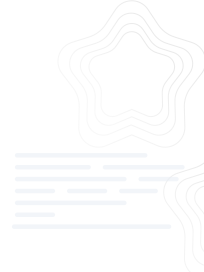
共5个文件
java:2个
xml:2个
iml:1个

需积分: 2 1 下载量 63 浏览量
2024-01-14
12:42:16
上传
评论
收藏 4KB ZIP 举报
温馨提示
算法与数据结构它们分别涵盖了以下主要内容: 数据结构(Data Structures): 逻辑结构:描述数据元素之间的逻辑关系,如线性结构(如数组、链表)、树形结构(如二叉树、堆、B树)、图结构(有向图、无向图等)以及集合和队列等抽象数据类型。 存储结构(物理结构):描述数据在计算机中如何具体存储。例如,数组的连续存储,链表的动态分配节点,树和图的邻接矩阵或邻接表表示等。 基本操作:针对每种数据结构,定义了一系列基本的操作,包括但不限于插入、删除、查找、更新、遍历等,并分析这些操作的时间复杂度和空间复杂度。 算法: 算法设计:研究如何将解决问题的步骤形式化为一系列指令,使得计算机可以执行以求解问题。 算法特性:包括输入、输出、有穷性、确定性和可行性。即一个有效的算法必须能在有限步骤内结束,并且对于给定的输入产生唯一的确定输出。 算法分类:排序算法(如冒泡排序、快速排序、归并排序),查找算法(如顺序查找、二分查找、哈希查找),图论算法(如Dijkstra最短路径算法、Floyd-Warshall算法、Prim最小生成树算法),动态规划,贪心算法,回溯法,分支限界法等。 算法分析:通过数学方法分析算法的时间复杂度(运行时间随数据规模增长的速度)和空间复杂度(所需内存大小)来评估其效率。 学习算法与数据结构不仅有助于理解程序的内部工作原理,更能帮助开发人员编写出高效、稳定和易于维护的软件系统。
资源推荐
资源详情
资源评论
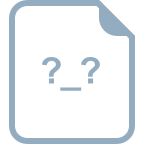
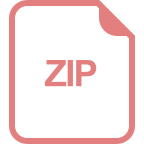
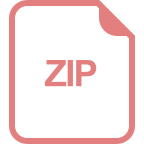
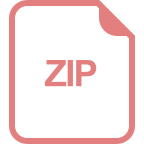
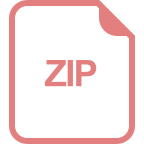
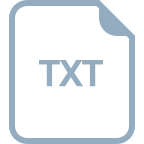
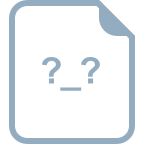
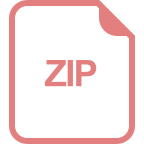
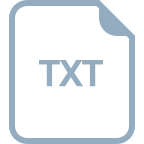
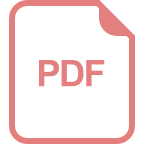
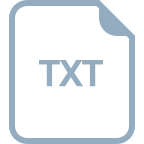
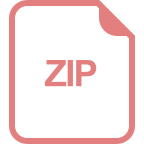
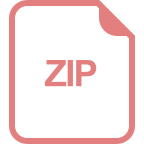
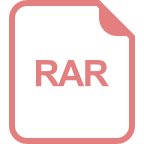
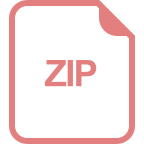
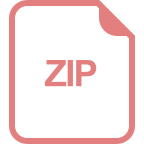
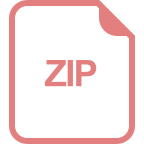
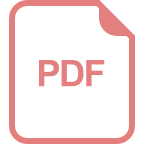
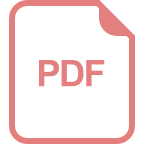
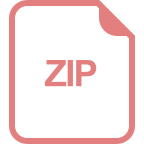
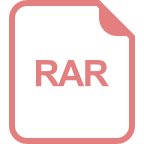
收起资源包目录





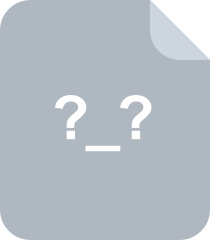

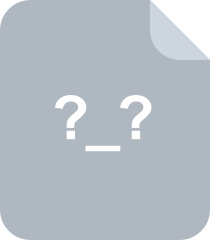

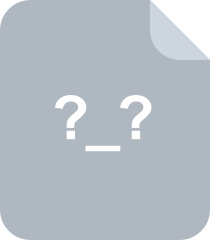
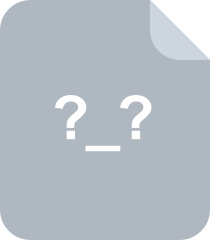
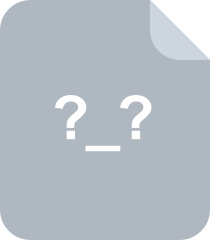
共 5 条
- 1
资源评论
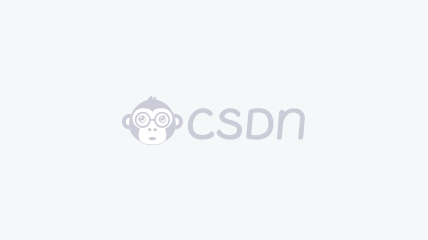

极致人生-010
- 粉丝: 2908
- 资源: 2826
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

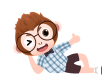
安全验证
文档复制为VIP权益,开通VIP直接复制
