argcheck
========
A powerful function argument checker and function overloading system for
Lua or LuaJIT.
`argcheck` generates specific code for checking arguments of a function. This
allows complex argument checking (possibly with optional values), with
little overhead (with [LuaJIT](http://luajit.org)). `argcheck` computes a
tree of all possible variants of arguments, allowing efficient overloading
and default argument management.
Installation
------------
The easiest is to use [luarocks](http://www.luarocks.org).
If you use Torch, simply do
```sh
luarocks install argcheck
```
else
```sh
luarocks build https://raw.github.com/torch/argcheck/master/rocks/argcheck-scm-1.rockspec
```
You can also copy the `argcheck` directory where `luajit` (or `lua`) will
find it.
Changelog
---------
- Version 2.0 (git)
- Rewrote completely the code generation.
- Now creates a tree of possible argument paths (much more efficient).
- Thanks to the tree approach, many bugs have been fixed.
- `argcheck` will produce an error if there are ambiguous argument rules.
- The feature `chain` is still deprecated (but available). Use `overload` instead.
- True overloading is happening. Contrary to `chain`, `overload` functions must be overwritten.
- Same functionalities than in 1.0, plus
- Handles named method calls
- Can generate a dot graphviz file of the argument paths for debugging purposes.
- Version 1.0
- Simplified the way of calling `argcheck`.
- Same functionalities than before, except named method call were not handled anymore.
- Added the `call` option to call a function if the arguments match given rules.
- Added the `chain` option to chain several `argcheck` function calls (a cheap version of overloading).
- Simplified the way of adding a type.
- Version 0.5
- Handling of default arguments (including defaulting to another argument), optional arguments (which might be `nil`), named arguments, named only option.
- Complicated way to handle method and functions.
- Calling function is mandatory.
Documentation
------------
To use `argcheck`, you have to first `require` it:
```lua
local argcheck = require 'argcheck'
```
In the following, we assume this has been done in your script.
Note that `argcheck` does not import anything globally, to avoid cluttering
the global namespace. The value returned by the require is a function: for
most usages, it will be the only thing you need.
_Note that in the following examples we do not use local variables for
check functions or example functions. This is bad practice, but helpful if
you want to cut-and-paste the code in your interactive lua to see how
this is running._
The `argcheck()` function creates a fast pre-compiled function for checking
arguments, according to rules provided by the user. Assume you have a
function which requires a unique number argument:
```lua
function addfive(x)
print(string.format('%f + 5 = %f', x, x + 5))
end
```
You can make sure everything goes fine by creating the rule:
```lua
check = argcheck{
{name="x", type="number"}
}
function addfive(...)
local x = check(...)
print(string.format('%f + 5 = %f', x, x + 5))
end
```
If a user try to pass a wrong argument, too many arguments, or no arguments
at all, `argcheck` will complain:
```lua
arguments:
{
x = number --
}
stdin:2: invalid arguments
```
A rule must at least take a `name` field. The `type` field is optional
(even though it is highly recommended!). If `type` is not provided, `argcheck` will make
sure the given argument is not `nil`. If you want also to accept `nil` arguments, see the
[`opt` option](#argcheck.opt).
### Default arguments
Arguments can have defaults:
```lua
check = argcheck{
{name="x", type="number", default=0}
}
```
In which case, if the argument is missing, `argcheck` will pass the default
one to your function:
```lua
> addfive()
0.000000 + 5 = 5.000000
```
### Help (or doc)
`argcheck` encourages you to add help to your function. You can document each argument:
```lua
check = argcheck{
{name="x", type="number", default=0, help="the age of the captain"}
}
```
Or even document the function:
```lua
check = argcheck{
help=[[
This function is going to do a simple addition.
Give a number, it adds 5. Amazing.
]],
{name="x", type="number", default=0, help="the age of the captain"}
}
```
Then, if the user makes a mistake in the arguments, the error message
becomes more clear:
```lua
> addfive('')
stdin:2: invalid arguments
This function is going to do a simple addition.
Give a number, it adds 5. Amazing.
arguments:
{
[x = number] -- the age of the captain [default=0]
}
```
Note that is (equivalently) possible to use the key `doc=` instead of `help=`.
### Multiple arguments
Until now, our function had only one argument. Obviously, `argcheck` can
handle as many as you wish:
```lua
check = argcheck{
help=[[
This function is going to do a simple addition.
Give a number, it adds 5. Amazing.
]],
{name="x", type="number", default=0, help="the age of the captain"},
{name="msg", type="string", help="a message"}
}
function addfive(...)
local x, msg = check(...)
print(string.format('%f + 5 = %f', x, x + 5))
print(msg)
end
```
`argcheck` handles well various cases, including those where some arguments
with defaults values might be missing:
```lua
> addfive(4, 'hello world')
4.000000 + 5 = 9.000000
hello world
>
> addfive('hello world')
0.000000 + 5 = 5.000000
hello world
>
> addfive(4)
stdin:2: invalid arguments
This function is going to do a simple addition.
Give a number, it adds 5. Amazing.
arguments:
{
[x = number] -- the age of the captain [default=0]
msg = string -- a message
}
```
### Default argument defaulting to another argument
Arguments can have a default value coming from another argument, with the
`defaulta` option. In the following
```lua
check = argcheck{
{name="x", type="number"},
{name="y", type="number", defaulta="x"}
}
function mul(...)
local x, y = check(...)
print(string.format('%f x %f = %f', x, y, x * y))
end
```
argument `y` will take the value of `x` if it is not passed during the function call:
```lua
> mul(3, 4)
3.000000 x 4.000000 = 12.000000
> mul(3)
3.000000 x 3.000000 = 9.000000
```
### Default arguments function
In some more complex cases, sometimes one needs to run a particular function when
the given argument is not provided. The option `defaultf` is here to help.
```lua
idx = 0
check = argcheck{
{name="x", type="number"},
{name="y", type="number", defaultf=function() idx = idx + 1 return idx end}
}
function mul(...)
local x, y = check(...)
print(string.format('%f x %f = %f', x, y, x * y))
end
```
This will output the following:
```lua
> mul(3)
3.000000 x 1.000000 = 3.000000
> mul(3)
3.000000 x 2.000000 = 6.000000
> mul(3)
3.000000 x 3.000000 = 9.000000
```
<a name="argcheck.opt"/>
### Optional arguments
Arguments with a default value can be seen as optional. However, as they
do have a default value, the underlying function will never receive a `nil`
value. In some situations, one might need to declare an optional argument
with no default value. You can do this with the `opt` option.
```lua
check = argcheck{
{name="x", type="number", default=0, help="the age of the captain"},
{name="msg", type="string", help="a message", opt=true}
}
function addfive(...)
local x, msg = check(...)
print(string.format('%f + 5 = %f', x, x + 5))
print(msg)
end
```
In this example, one might call `addfive()` without the `msg` argument. Of
course, the underlying function must be able to handle `nil` values:
```lua
> addfive('hello world')
0.000000 + 5 = 5.000000
hello world
> addfive()
0.000000 + 5 = 5.000000
nil
```
### Torch Tensors
`argcheck` supports Torch `Tensors` type checks.
Specific tensor types like `Int`, `Float`, or `Double` can be
checked with `torch.<Type>Tensor`.
Any tensor type can be checked with `tor
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
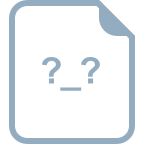
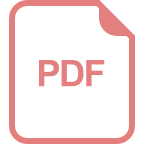
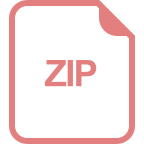
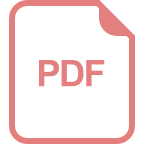
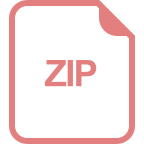
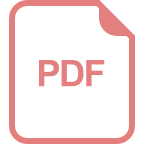
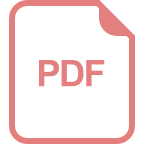
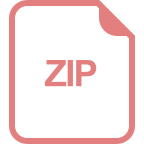
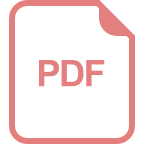
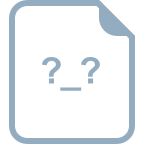
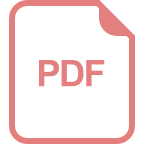
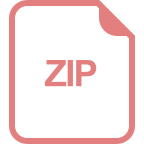
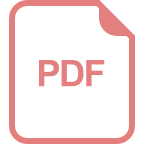
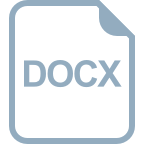
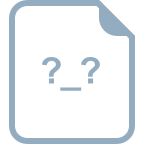
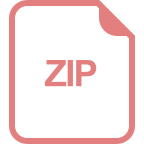
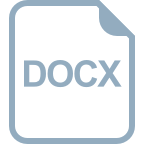
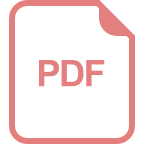
收起资源包目录

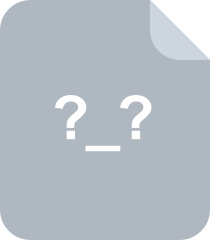
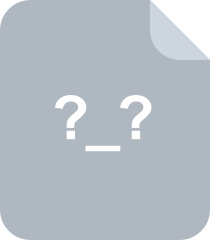
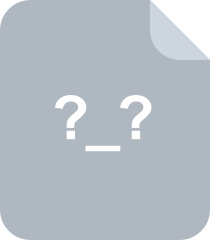
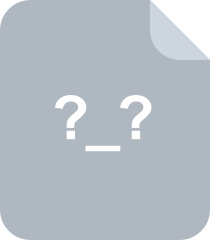
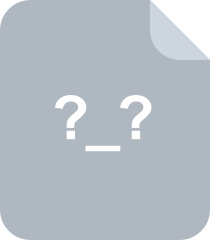
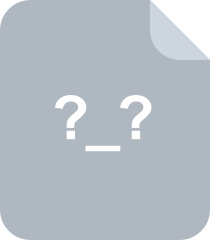
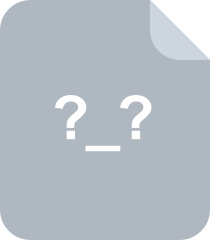
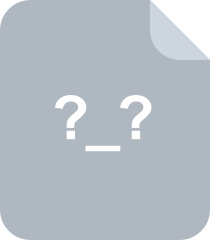
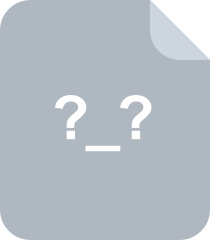
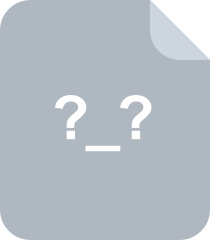
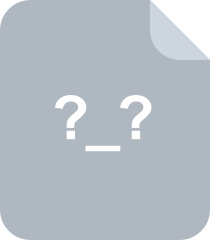
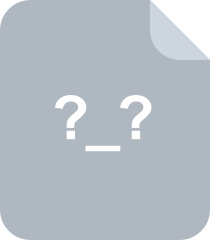
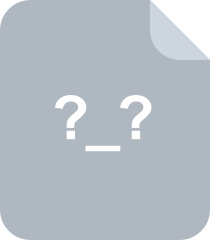
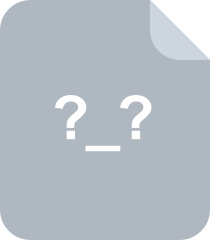
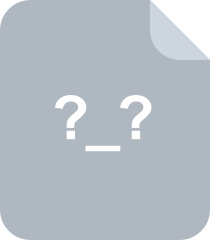
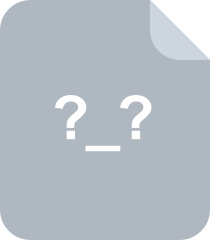
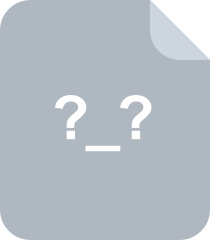
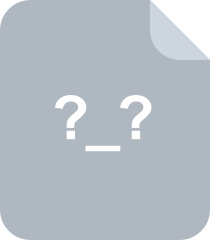
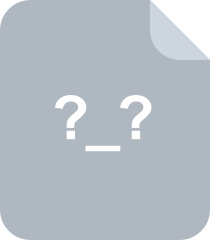
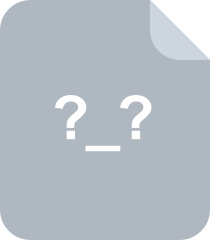
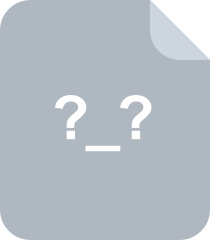
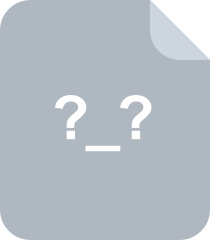
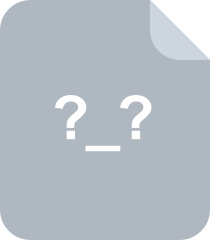
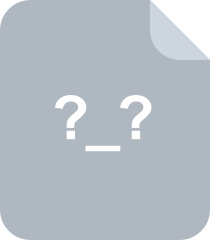
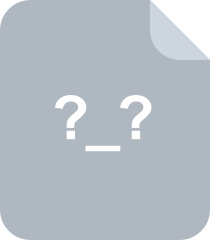
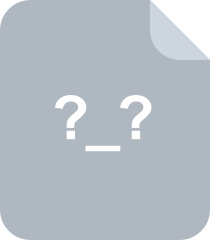
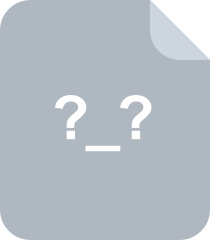
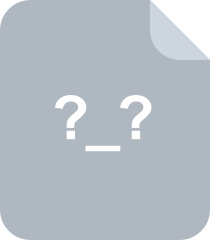
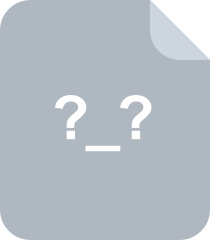
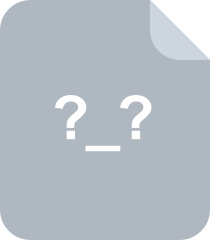
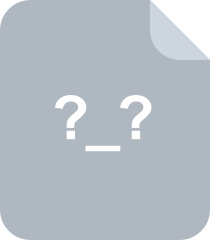
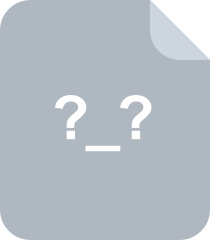
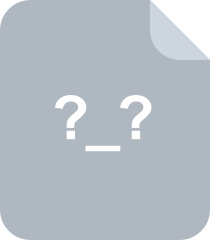
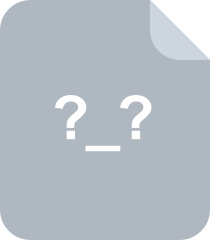
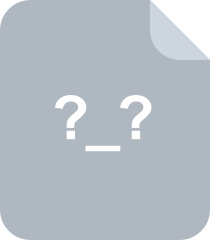
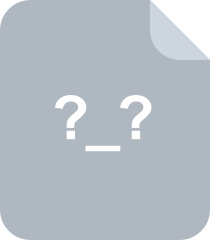
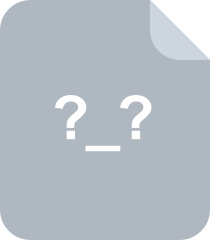
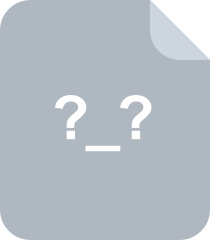
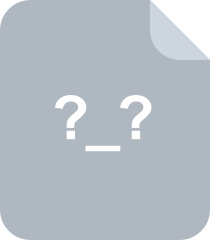
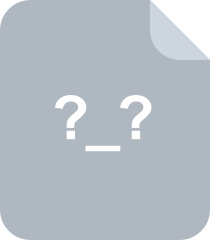
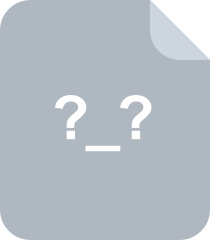
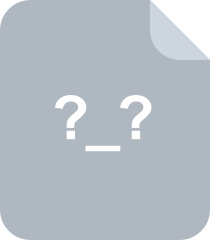
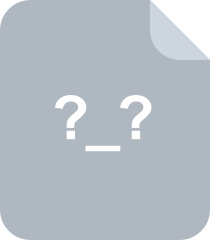
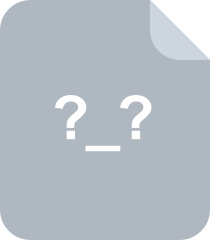
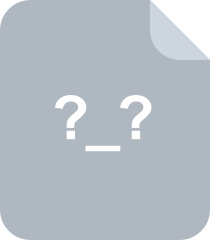
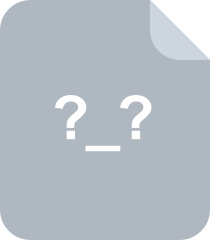
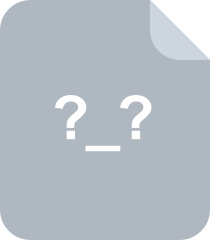
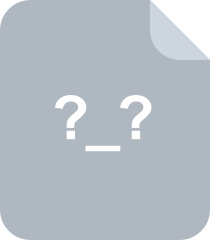
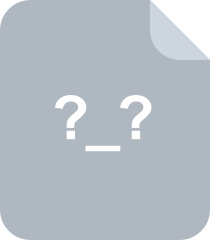
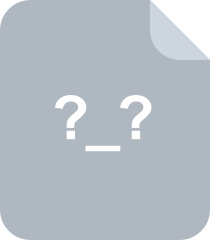
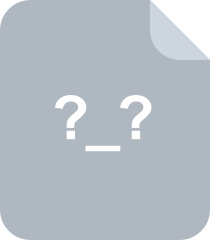
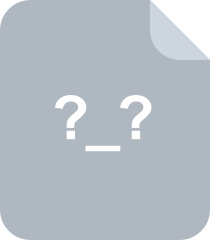
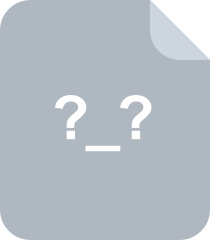
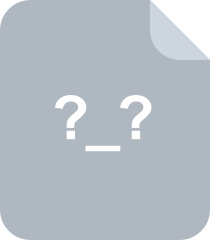
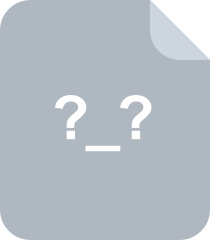
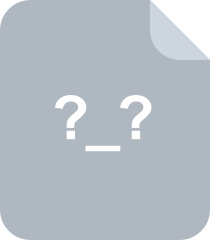
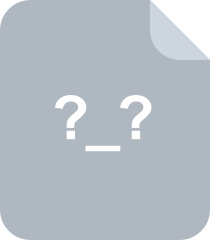
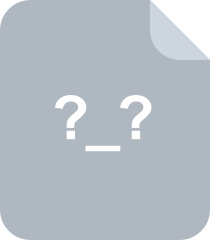
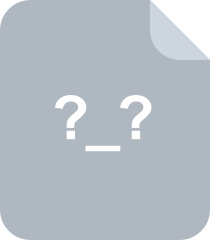
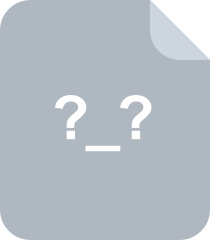
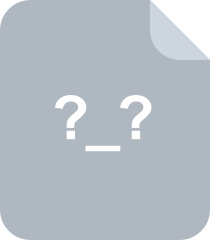
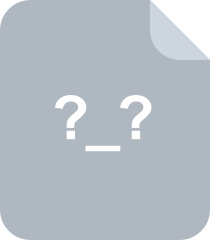
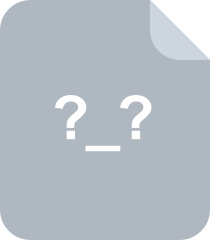
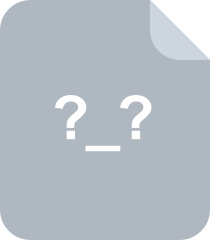
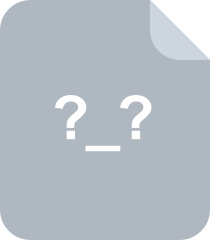
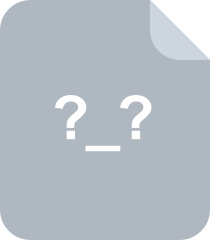
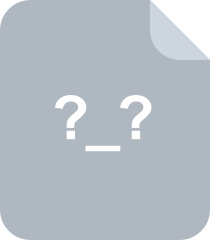
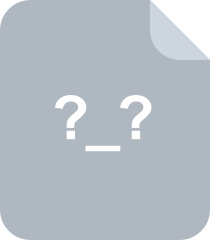
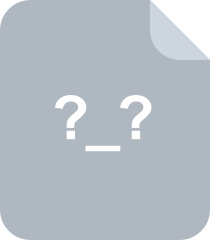
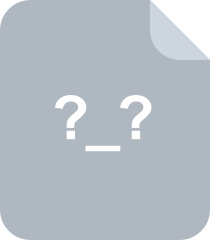
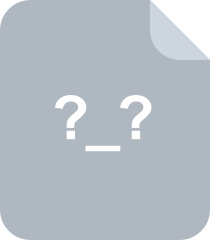
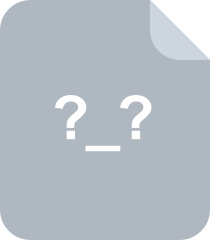
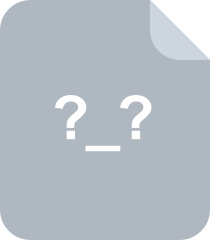
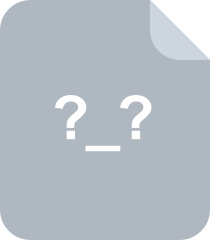
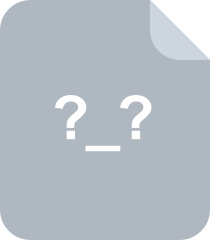
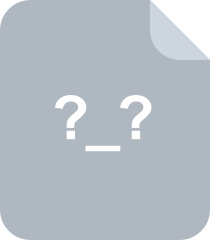
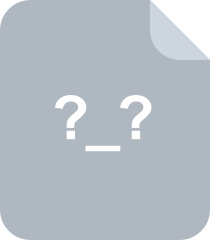
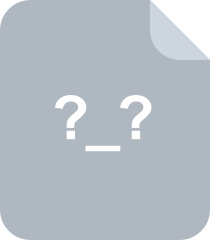
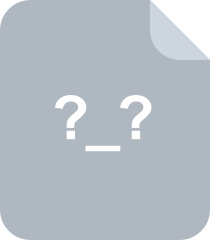
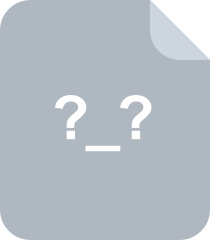
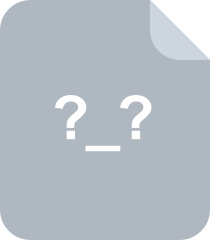
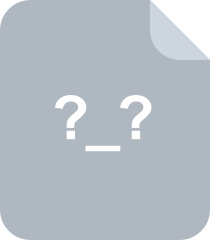
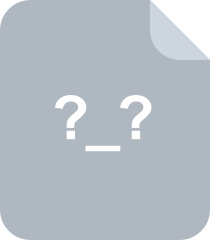
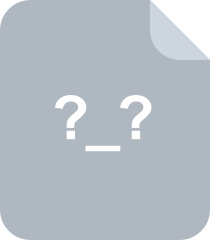
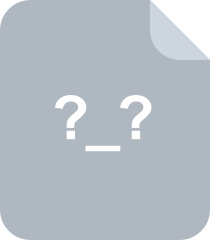
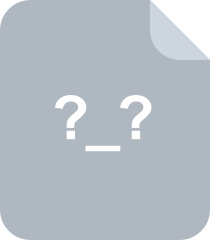
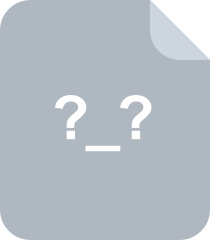
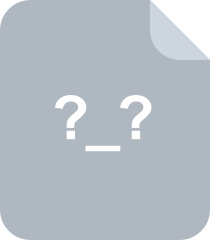
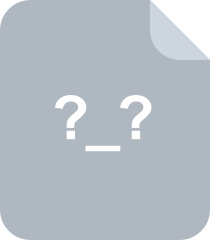
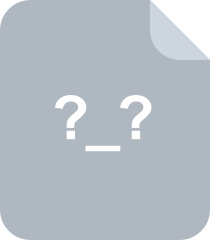
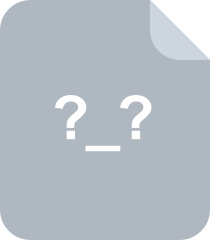
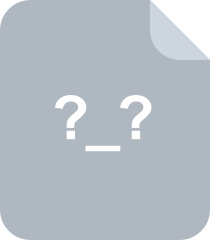
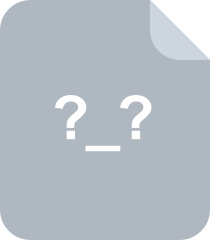
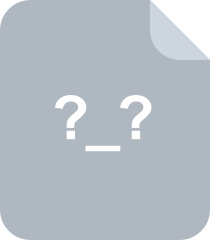
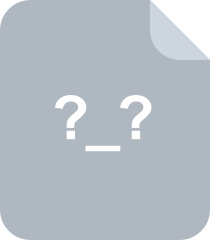
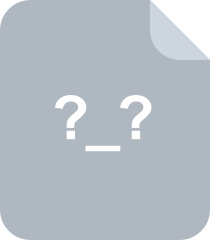
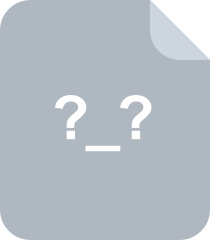
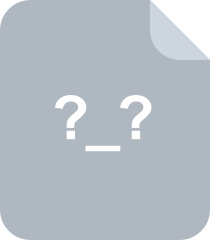
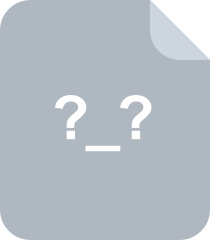
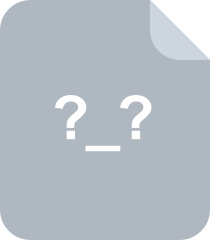
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
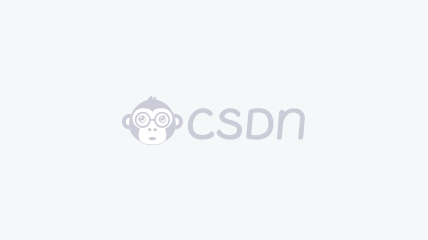

AI布道
- 粉丝: 184
- 资源: 40
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

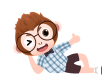
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


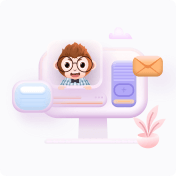
安全验证
文档复制为VIP权益,开通VIP直接复制
