!function(e){if("object"==typeof exports&&"undefined"!=typeof module)module.exports=e();else if("function"==typeof define&&define.amd)define([],e);else{("undefined"!=typeof window?window:"undefined"!=typeof global?global:"undefined"!=typeof self?self:this).mqtt=e()}}(function(){return function(){return function e(t,r,n){function i(s,a){if(!r[s]){if(!t[s]){var u="function"==typeof require&&require;if(!a&&u)return u(s,!0);if(o)return o(s,!0);var c=new Error("Cannot find module '"+s+"'");throw c.code="MODULE_NOT_FOUND",c}var l=r[s]={exports:{}};t[s][0].call(l.exports,function(e){return i(t[s][1][e]||e)},l,l.exports,e,t,r,n)}return r[s].exports}for(var o="function"==typeof require&&require,s=0;s<n.length;s++)i(n[s]);return i}}()({1:[function(e,t,r){(function(r,n){"use strict";var i=e("events").EventEmitter,o=e("./store"),s=e("mqtt-packet"),a=e("readable-stream").Writable,u=e("inherits"),c=e("reinterval"),l=e("./validations"),f=e("xtend"),p=e("debug")("mqttjs:client"),h=n.setImmediate||function(e){r.nextTick(e)},d={keepalive:60,reschedulePings:!0,protocolId:"MQTT",protocolVersion:4,reconnectPeriod:1e3,connectTimeout:3e4,clean:!0,resubscribe:!0},g=["ECONNREFUSED","EADDRINUSE","ECONNRESET","ENOTFOUND"],b={0:"",1:"Unacceptable protocol version",2:"Identifier rejected",3:"Server unavailable",4:"Bad username or password",5:"Not authorized",16:"No matching subscribers",17:"No subscription existed",128:"Unspecified error",129:"Malformed Packet",130:"Protocol Error",131:"Implementation specific error",132:"Unsupported Protocol Version",133:"Client Identifier not valid",134:"Bad User Name or Password",135:"Not authorized",136:"Server unavailable",137:"Server busy",138:"Banned",139:"Server shutting down",140:"Bad authentication method",141:"Keep Alive timeout",142:"Session taken over",143:"Topic Filter invalid",144:"Topic Name invalid",145:"Packet identifier in use",146:"Packet Identifier not found",147:"Receive Maximum exceeded",148:"Topic Alias invalid",149:"Packet too large",150:"Message rate too high",151:"Quota exceeded",152:"Administrative action",153:"Payload format invalid",154:"Retain not supported",155:"QoS not supported",156:"Use another server",157:"Server moved",158:"Shared Subscriptions not supported",159:"Connection rate exceeded",160:"Maximum connect time",161:"Subscription Identifiers not supported",162:"Wildcard Subscriptions not supported"};function y(e,t,r){p("sendPacket :: packet: %O",t),p("sendPacket :: emitting `packetsend`"),e.emit("packetsend",t),p("sendPacket :: writing to stream");var n=s.writeToStream(t,e.stream,e.options);p("sendPacket :: writeToStream result %s",n),!n&&r?(p("sendPacket :: handle events on `drain` once through callback."),e.stream.once("drain",r)):r&&(p("sendPacket :: invoking cb"),r())}function m(e,t,r,n){p("storeAndSend :: store packet with cmd %s to outgoingStore",t.cmd),e.outgoingStore.put(t,function(i){if(i)return r&&r(i);n(),y(e,t,r)})}function _(e){p("nop ::",e)}function v(e,t){var r,n=this;if(!(this instanceof v))return new v(e,t);for(r in this.options=t||{},d)void 0===this.options[r]?this.options[r]=d[r]:this.options[r]=t[r];p("MqttClient :: options.protocol",t.protocol),p("MqttClient :: options.protocolVersion",t.protocolVersion),p("MqttClient :: options.username",t.username),p("MqttClient :: options.keepalive",t.keepalive),p("MqttClient :: options.reconnectPeriod",t.reconnectPeriod),p("MqttClient :: options.rejectUnauthorized",t.rejectUnauthorized),this.options.clientId="string"==typeof t.clientId?t.clientId:"mqttjs_"+Math.random().toString(16).substr(2,8),p("MqttClient :: clientId",this.options.clientId),this.options.customHandleAcks=5===t.protocolVersion&&t.customHandleAcks?t.customHandleAcks:function(){arguments[3](0)},this.streamBuilder=e,this.outgoingStore=t.outgoingStore||new o,this.incomingStore=t.incomingStore||new o,this.queueQoSZero=void 0===t.queueQoSZero||t.queueQoSZero,this._resubscribeTopics={},this.messageIdToTopic={},this.pingTimer=null,this.connected=!1,this.disconnecting=!1,this.queue=[],this.connackTimer=null,this.reconnectTimer=null,this._storeProcessing=!1,this._packetIdsDuringStoreProcessing={},this.nextId=Math.max(1,Math.floor(65535*Math.random())),this.outgoing={},this._firstConnection=!0,this.on("connect",function(){var e=this.queue;p("connect :: sending queued packets"),function t(){var r=e.shift();p("deliver :: entry %o",r);var i;r&&(i=r.packet,p("deliver :: call _sendPacket for %o",i),n._sendPacket(i,function(e){r.cb&&r.cb(e),t()}))}()}),this.on("close",function(){p("close :: connected set to `false`"),this.connected=!1,p("close :: clearing connackTimer"),clearTimeout(this.connackTimer),p("close :: clearing ping timer"),null!==n.pingTimer&&(n.pingTimer.clear(),n.pingTimer=null),p("close :: calling _setupReconnect"),this._setupReconnect()}),i.call(this),p("MqttClient :: setting up stream"),this._setupStream()}u(v,i),v.prototype._setupStream=function(){var e,t=this,n=new a,i=s.parser(this.options),o=null,u=[];function c(){if(u.length)r.nextTick(l);else{var e=o;o=null,e()}}function l(){p("work :: getting next packet in queue");var e=u.shift();if(e)p("work :: packet pulled from queue"),t._handlePacket(e,c);else{p("work :: no packets in queue");var r=o;o=null,p("work :: done flag is %s",!!r),r&&r()}}if(p("_setupStream :: calling method to clear reconnect"),this._clearReconnect(),p("_setupStream :: using streamBuilder provided to client to create stream"),this.stream=this.streamBuilder(this),i.on("packet",function(e){p("parser :: on packet push to packets array."),u.push(e)}),n._write=function(e,t,r){o=r,p("writable stream :: parsing buffer"),i.parse(e),l()},p("_setupStream :: pipe stream to writable stream"),this.stream.pipe(n),this.stream.on("error",function(e){p("streamErrorHandler :: error",e.message),g.includes(e.code)?(p("streamErrorHandler :: emitting error"),t.emit("error",e)):_(e)}),this.stream.on("close",function(){var e;p("(%s)stream :: on close",t.options.clientId),(e=t.outgoing)&&(p("flushVolatile :: deleting volatile messages from the queue and setting their callbacks as error function"),Object.keys(e).forEach(function(t){e[t].volatile&&"function"==typeof e[t].cb&&(e[t].cb(new Error("Connection closed")),delete e[t])})),p("stream: emit close to MqttClient"),t.emit("close")}),p("_setupStream: sending packet `connect`"),(e=Object.create(this.options)).cmd="connect",y(this,e),i.on("error",this.emit.bind(this,"error")),this.options.properties){if(!this.options.properties.authenticationMethod&&this.options.properties.authenticationData)return t.end(()=>this.emit("error",new Error("Packet has no Authentication Method"))),this;if(this.options.properties.authenticationMethod&&this.options.authPacket&&"object"==typeof this.options.authPacket)y(this,f({cmd:"auth",reasonCode:0},this.options.authPacket))}this.stream.setMaxListeners(1e3),clearTimeout(this.connackTimer),this.connackTimer=setTimeout(function(){p("!!connectTimeout hit!! Calling _cleanUp with force `true`"),t._cleanUp(!0)},this.options.connectTimeout)},v.prototype._handlePacket=function(e,t){var r=this.options;if(5===r.protocolVersion&&r.properties&&r.properties.maximumPacketSize&&r.properties.maximumPacketSize<e.length)return this.emit("error",new Error("exceeding packets size "+e.cmd)),this.end({reasonCode:149,properties:{reasonString:"Maximum packet size was exceeded"}}),this;switch(p("_handlePacket :: emitting packetreceive"),this.emit("packetreceive",e),e.cmd){case"publish":this._handlePublish(e,t);break;case"puback":case"pubrec":case"pubcomp":case"suback":case"unsuback":this._handleAck(e),t();break;case"pubrel":this._handlePubrel(e,t);break;case"connack":this._handleConnack(e),t();break;case"pingresp":this._handlePingresp(e),t();break;case"disconnect":this._handleDisconnect(e),t()}},v.prototype._checkDisconnecting=function(e){return this.disconnecting&&(e?e(new Error("client disconnecting")):this.emit("error",new Error("client disconnecting"))),this.disconnecting},v.prototype.publish=function(e,t,r,n){var i;p("publis
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
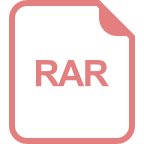
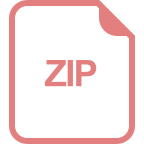
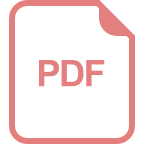
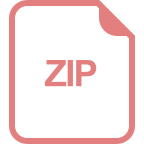
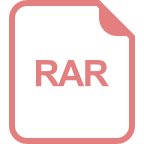
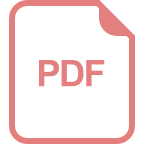
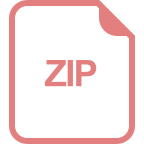
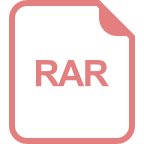
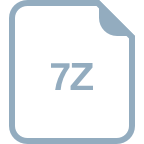
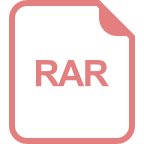
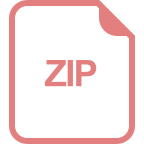
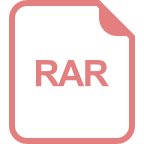
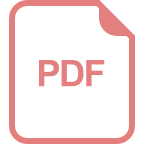
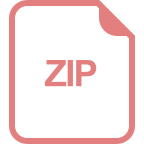
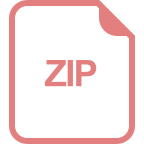
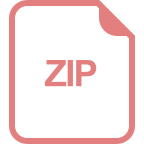
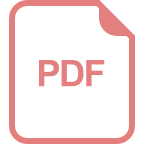
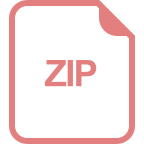
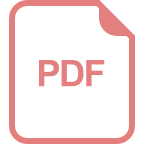
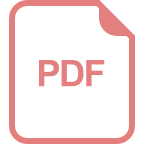
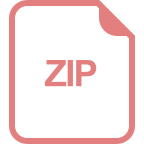
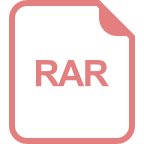
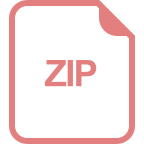
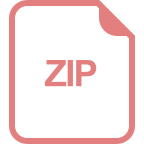
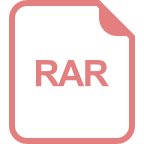
收起资源包目录


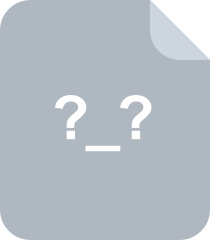
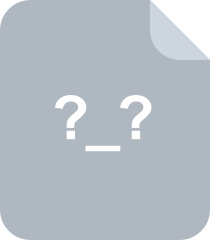


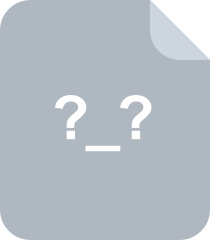
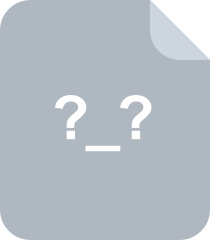
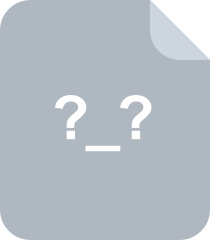
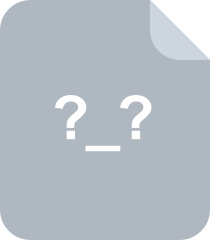

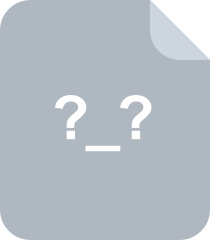
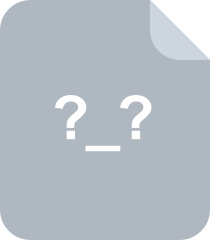
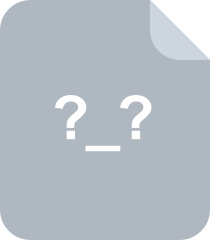
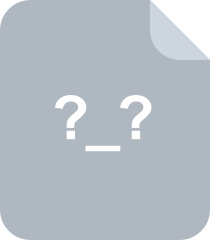
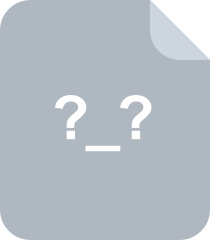
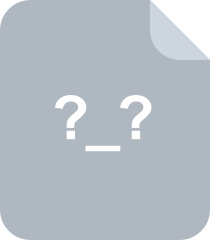
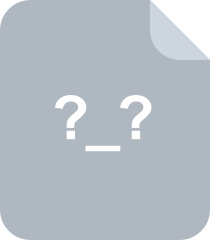

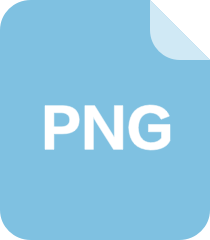
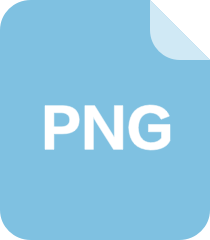
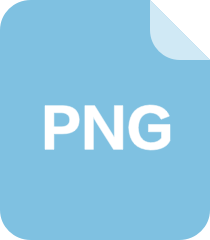
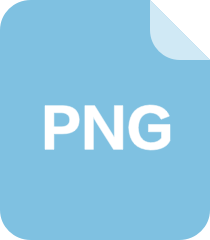
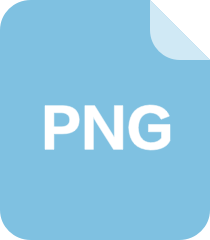
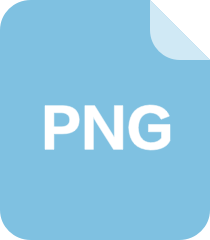
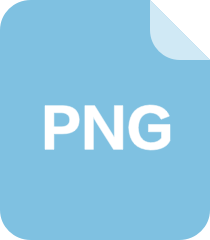
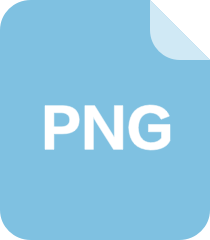
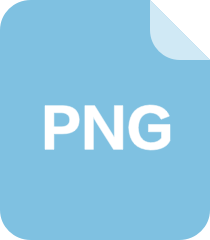
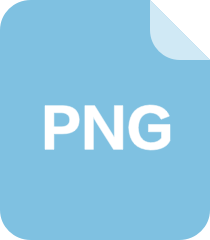
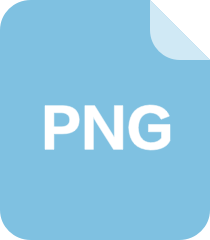
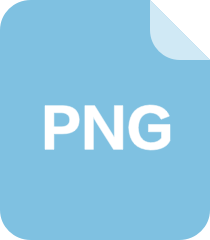
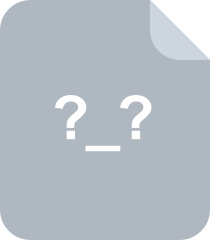

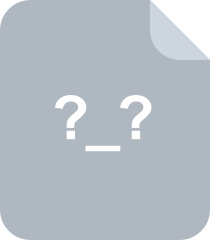
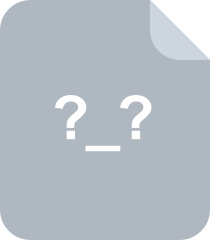

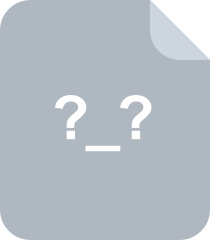
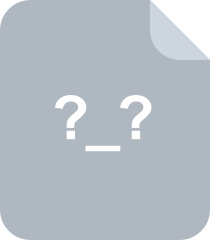


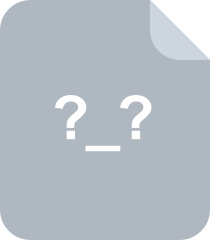



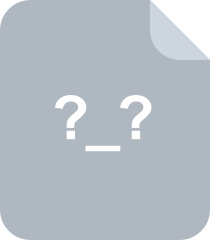

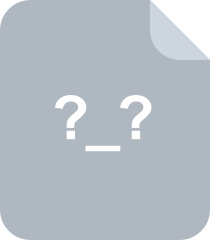

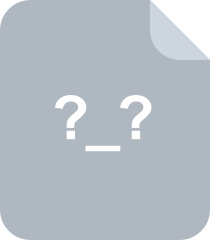

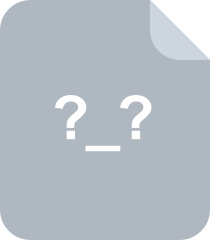

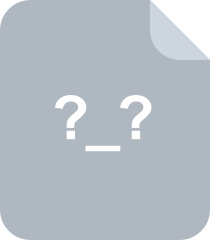

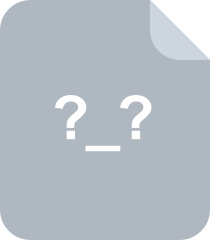

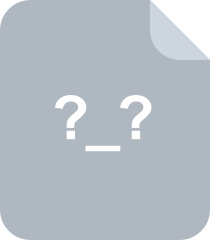

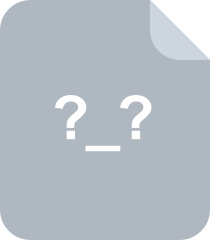

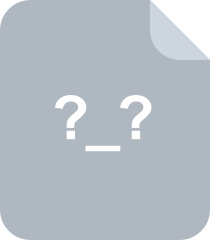

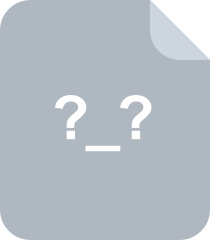

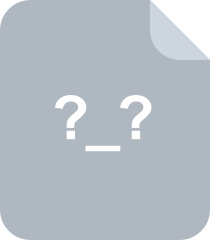

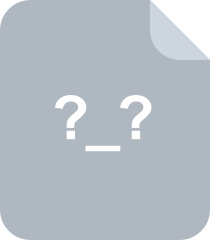

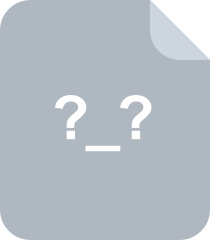

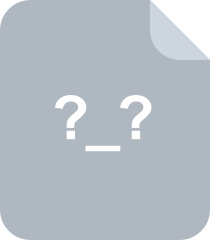

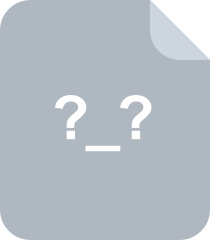
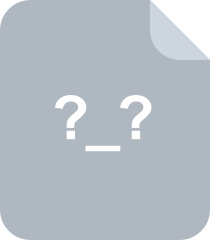


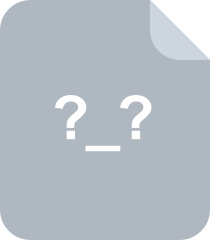

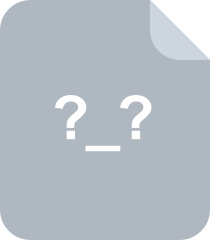

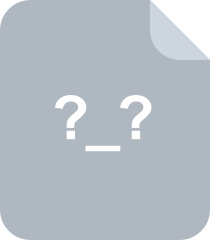

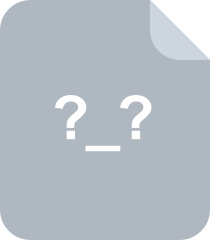


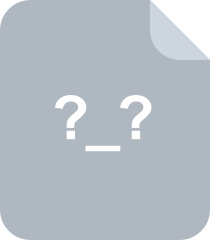

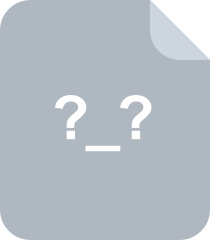

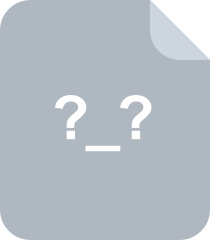

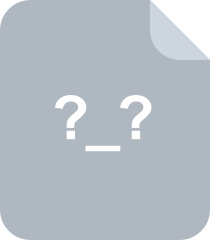

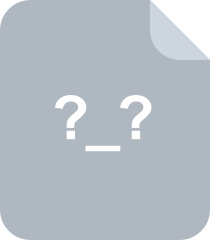

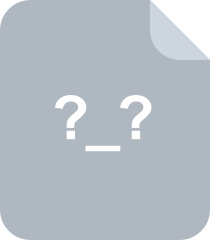

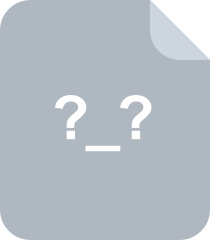

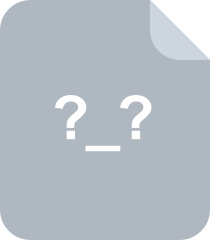

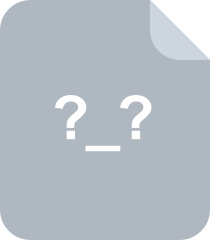

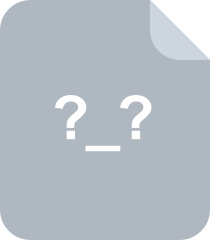

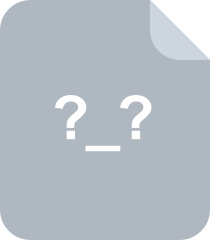

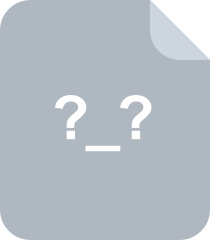

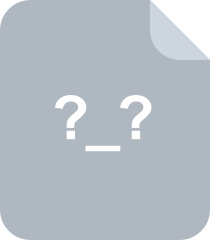

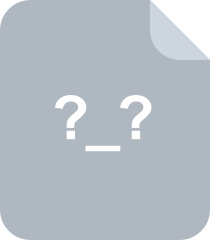

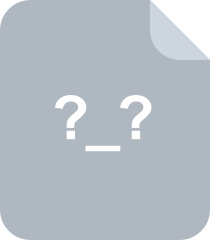

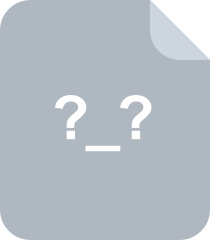

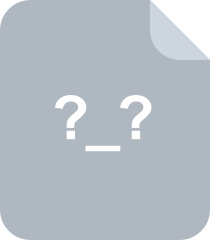
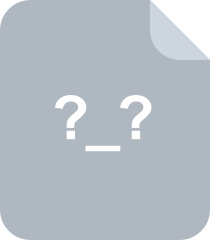

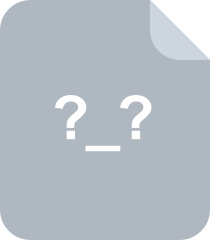

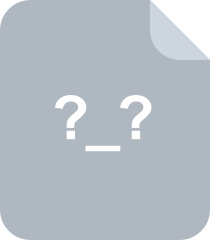


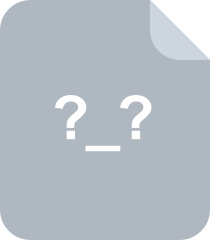

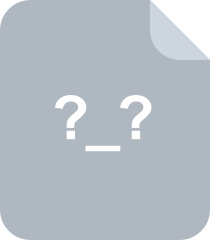
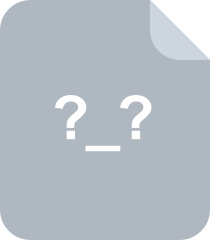
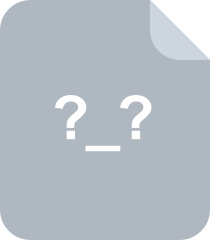
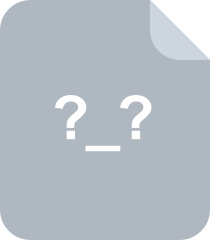
共 76 条
- 1
资源评论
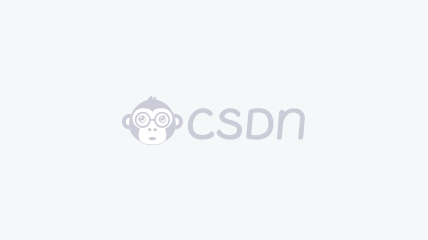

GE567
- 粉丝: 0
- 资源: 4
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

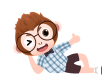
最新资源
- F1:帮助/help F2:切换相对/坐标值 F3: 显示全部 F4:参考点“自From” F5:切换当前坐标 F6:导航/栅格/智能 F7: 三视图导航开关/guide F8:正交/ortho F9
- 基于Cisco Packet Tracer 6.2的校园网仿真实验.pkt
- A051-基于Spring Boot的网络海鲜市场系统的设计与实现
- 使用WindowsAPI写的一些渗透小工具.zip
- 不要过审 ,不要过审 ,不要过审
- 神经网络的概要介绍与分析
- C#实现下拉列表显示datagridview
- A049-基于Java的实习管理系统的设计与实现
- A048-基于SpringBoot的在线考试系统的设计与实现
- 搜索引擎的概要介绍与分析
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


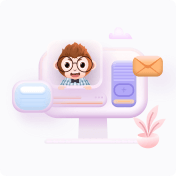
安全验证
文档复制为VIP权益,开通VIP直接复制
