package com.ivo.mas.system.utils;
import org.springframework.util.Base64Utils;
import java.io.BufferedReader;
import java.io.DataOutputStream;
import java.io.InputStreamReader;
import java.io.UnsupportedEncodingException;
import java.net.HttpURLConnection;
import java.net.URL;
import java.security.MessageDigest;
import java.util.*;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class StringUtils {
public static String ENCODING = "UTF-8";
public static boolean isNotEmpty(String s) {
return s != null && !s.trim().equals("");
}
public static boolean isEmpty(String s) {
return s == null || s.trim().equals("");
}
public static boolean isNotNull(String s) {
return s != null;
}
public static boolean isEqual(String s, Object o) {
return s != null && s.equals(o);
}
public static String ArraytoString(String s[]) {
String result = "";
if (null != s && s.length > 0) {
for (int i = 0; i < s.length; i++) {
result += s[i] + ",";
}
}
if (result.length() > 1) {
return result.substring(0, result.length() - 1);
} else {
return "";
}
}
public static String convert(String s) {
if (s == null)
return "";
return s.replaceAll("&", "&").replace("'", "'").replace("\"",
""").replace("<", "<").replace(">", ">");
}
public static String string2MD5(String inStr) {
MessageDigest md5 = null;
try {
md5 = MessageDigest.getInstance("MD5");
} catch (Exception e) {
System.out.println(e.toString());
e.printStackTrace();
return "";
}
char[] charArray = inStr.toCharArray();
byte[] byteArray = new byte[charArray.length];
for (int i = 0; i < charArray.length; i++)
byteArray[i] = (byte) charArray[i];
byte[] md5Bytes = md5.digest(byteArray);
StringBuffer hexValue = new StringBuffer();
for (int i = 0; i < md5Bytes.length; i++) {
int val = ((int) md5Bytes[i]) & 0xff;
if (val < 16)
hexValue.append("0");
hexValue.append(Integer.toHexString(val));
}
return hexValue.toString();
}
/**
* Decode a string using Base64 encoding.
*
* @param str
* @return String
*/
public static String decodeString(String str) {
if (str == null)
return null;
try {
byte[] b = Base64Utils.decode(str.getBytes(ENCODING));
return new String(b, ENCODING);
} catch (Exception e) {
return null;
}
}
// 全角转半角
public static String ToDBC(String input) {
char c[] = input.toCharArray();
for (int i = 0; i < c.length; i++) {
if (c[i] == '\u3000') {
c[i] = ' ';
} else if (c[i] > '\uFF00' && c[i] < '\uFF5F') {
c[i] = (char) (c[i] - 65248);
}
}
String returnString = new String(c);
return returnString;
}
//去除空格、回车、换行符、制表符
public static String replaceBlank(String str) {
String dest = "";
if (str != null) {
Pattern p = Pattern.compile("\\s*|\t|\r|\n");
Matcher m = p.matcher(str);
dest = m.replaceAll("");
}
return dest;
}
/**
* @param string
* @return
*/
public static String swapFirstLetterCase(String string) {
StringBuffer sbuf = new StringBuffer(string);
sbuf.deleteCharAt(0);
if (Character.isLowerCase(string.substring(0, 1).toCharArray()[0])) {
sbuf.insert(0, string.substring(0, 1).toUpperCase());
} else {
sbuf.insert(0, string.substring(0, 1).toLowerCase());
}
return sbuf.toString();
}
static public String createGUID() {
// -----------------------------------------------------------------------------------------------------------------
Random objRandom = new Random();
String strEncode = "01234567890ABCDEFGH";
String strGUID = "";
int nPos = 0;
char c = '\0';
// -----------------------------------------------------------------------------------------------------------------
for (int i = 0; i < 36; i++) {
if (i == 8 || i == 13 || i == 18 || i == 23) {
c = '-';
} else {
nPos = objRandom.nextInt();
if (nPos < 0)
nPos *= -1;
nPos = nPos % strEncode.length();
c = strEncode.charAt(nPos);
} // if( i
strGUID += c;
} // for i
// -----------------------------------------------------------------------------------------------------------------
return strGUID;
}
/**
* 将字符串数组转化为in条件用的字符串
*
* @param str
* @return
*/
public static String getInClause(String[] str) {
StringBuffer result = new StringBuffer();
for (int i = 0; i < str.length; i++) {
if (i > 0) {
result.append(",");
}
result.append("'" + str[i] + "'");
}
return result.toString();
}
public static String getInClause(List<String> list) {
String[] arr = (String[]) list.toArray(new String[list.size()]);
return getInClause(arr);
}
/**
* <p>
* Checks if a CharSequence is whitespace, empty ("") or null.
* </p>
*
* <pre>
* StringUtils.isBlank(null) = true
* StringUtils.isBlank("") = true
* StringUtils.isBlank(" ") = true
* StringUtils.isBlank("bob") = false
* StringUtils.isBlank(" bob ") = false
* </pre>
*
* @param cs the CharSequence to check, may be null
* @return {@code true} if the CharSequence is null, empty or whitespace
* @since 2.0
* @since 3.0 Changed signature from isBlank(String) to
* isBlank(CharSequence)
*/
public static boolean isBlank(CharSequence cs) {
int strLen;
if (cs == null || (strLen = cs.length()) == 0) {
return true;
}
for (int i = 0; i < strLen; i++) {
if (Character.isWhitespace(cs.charAt(i)) == false) {
return false;
}
}
return true;
}
/**
* <p>
* Checks if a CharSequence is not empty (""), not null and not whitespace
* only.
* </p>
*
* <pre>
* StringUtils.isNotBlank(null) = false
* StringUtils.isNotBlank("") = false
* StringUtils.isNotBlank(" ") = false
* StringUtils.isNotBlank("bob") = true
* StringUtils.isNotBlank(" bob ") = true
* </pre>
*
* @param cs the CharSequence to check, may be null
* @return {@code true} if the CharSequence is not empty and not null and
* not whitespace
* @since 2.0
* @since 3.0 Changed signature from isNotBlank(String) to
* isNotBlank(CharSequence)
*/
public static boolean isNotBlank(CharSequence cs) {
return !StringUtils.isBlank(cs);
}
/**
*
*/
public static String extract(String regex, String base) {
Pattern p = Pattern.compile(regex);
Matcher m = p.matcher(base);
if (m.find()) {
return m.group();
}
return "";
}
/**
* <p>
* Removes a substring only if it is at the end of a source string,
* otherwise returns the source string.
* </p>
*
* <p>
* A {@code null} source string will return {@code null}. An empty ("")
* source string will return the empty string. A {@code null} search string
* will re
没有合适的资源?快使用搜索试试~ 我知道了~
毕设 基于JAVASpringBoot和Vue小说在线听书网站设计.zip
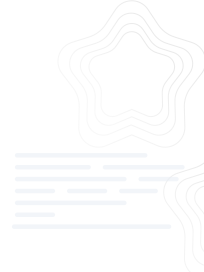
共866个文件
png:453个
js:166个
css:82个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 174 浏览量
2023-10-24
10:27:52
上传
评论
收藏 5.06MB ZIP 举报
温馨提示
matlab算法,毕设、课设程序,全部源码均已进行严格测试,可以直接运行! matlab算法,毕设、课设程序,全部源码均已进行严格测试,可以直接运行! matlab算法,毕设、课设程序,全部源码均已进行严格测试,可以直接运行! matlab算法,毕设、课设程序,全部源码均已进行严格测试,可以直接运行! matlab算法,毕设、课设程序,全部源码均已进行严格测试,可以直接运行! matlab算法,毕设、课设程序,全部源码均已进行严格测试,可以直接运行! matlab算法,毕设、课设程序,全部源码均已进行严格测试,可以直接运行! matlab算法,毕设、课设程序,全部源码均已进行严格测试,可以直接运行! matlab算法,毕设、课设程序,全部源码均已进行严格测试,可以直接运行! matlab算法,毕设、课设程序,全部源码均已进行严格测试,可以直接运行! matlab算法,毕设、课设程序,全部源码均已进行严格测试,可以直接运行! matlab算法,毕设、课设程序,全部源码均已进行严格测试,可以直接运行! matlab算法,毕设、课设程序,全部源码均已进行严格测试,可以直接运行! matlab算法,毕设、课设程序,全部源码均已进行严格测试,可以直接运行!
资源推荐
资源详情
资源评论
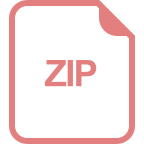
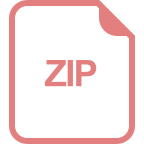
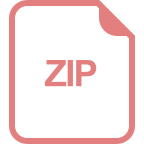
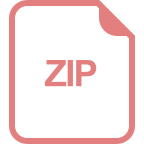
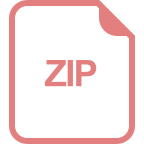
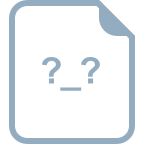
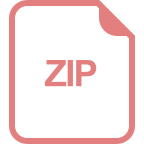
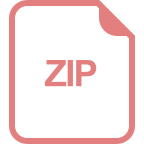
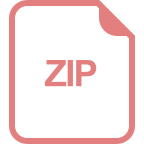
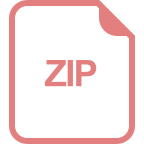
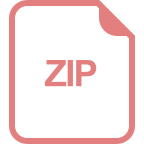
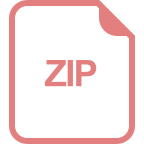
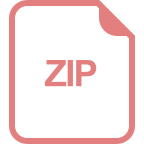
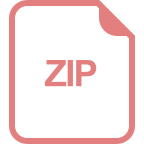
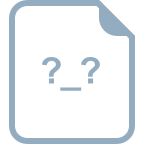
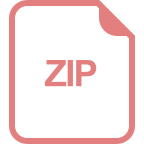
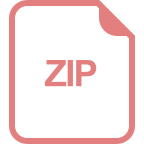
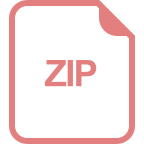
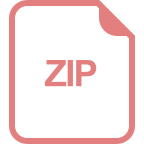
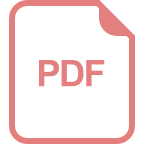
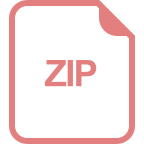
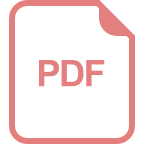
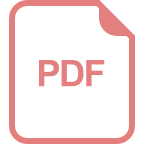
收起资源包目录

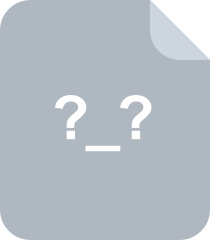
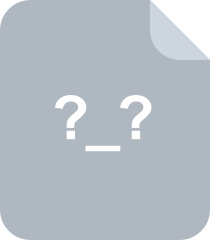
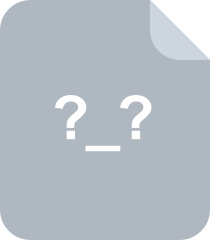
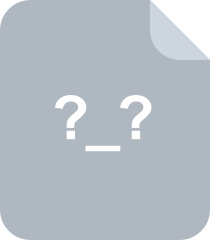
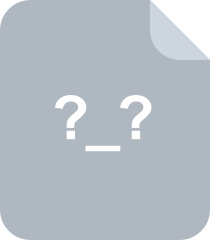
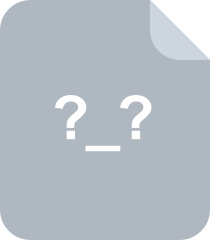
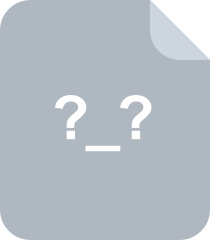
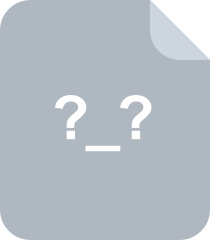
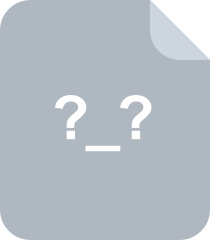
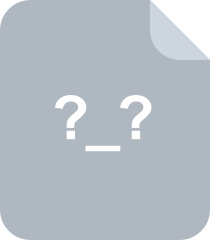
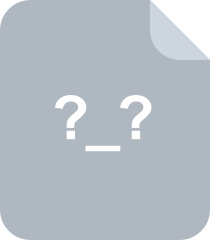
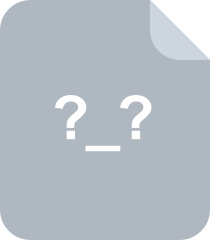
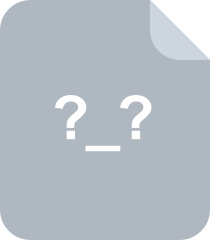
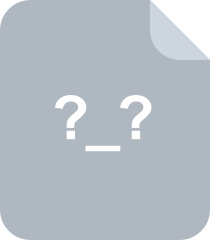
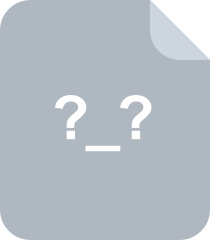
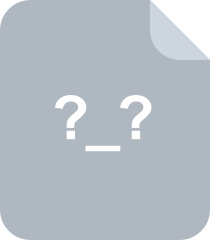
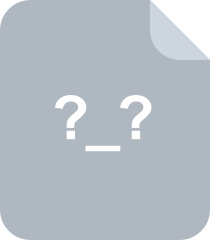
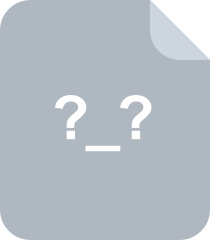
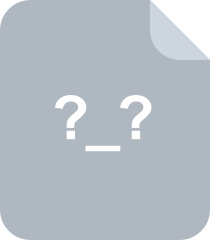
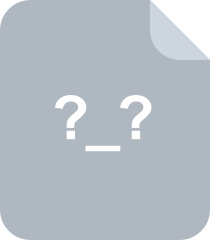
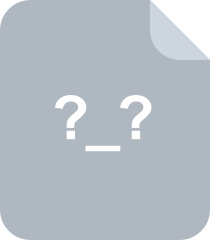
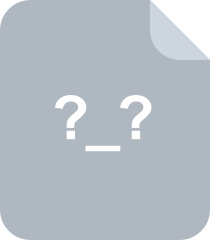
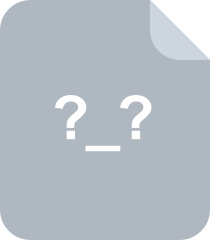
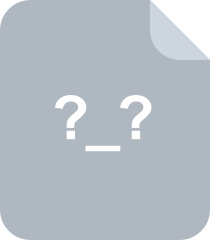
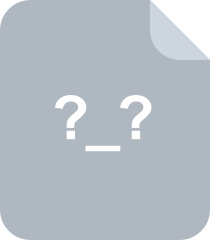
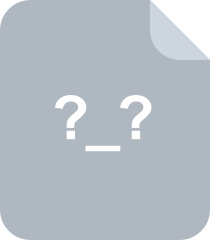
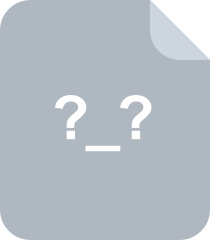
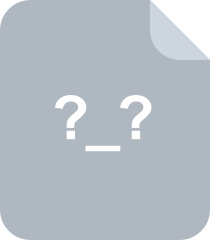
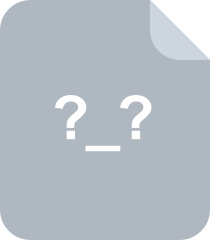
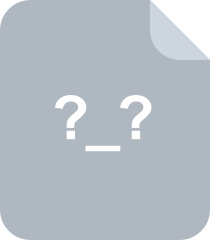
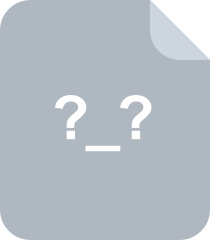
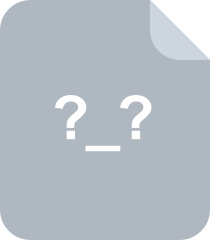
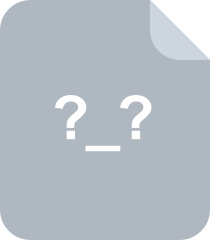
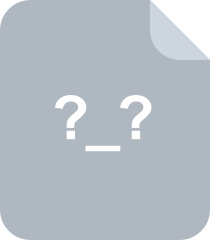
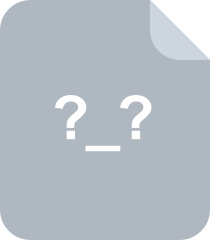
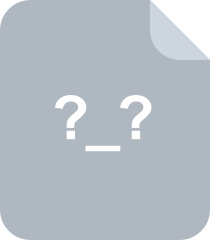
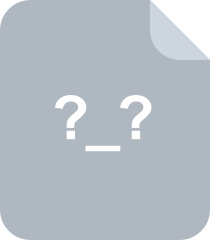
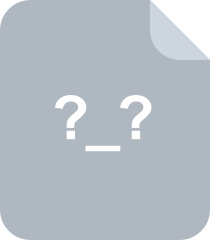
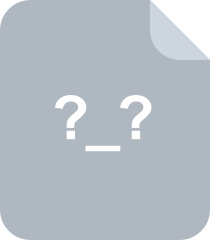
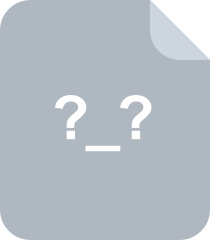
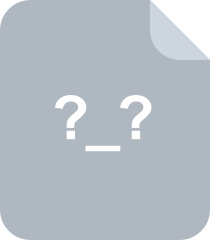
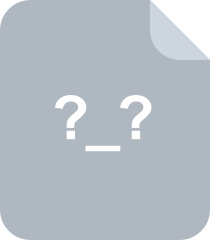
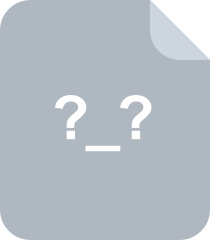
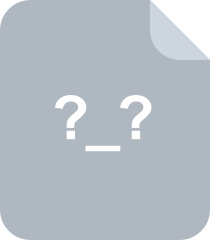
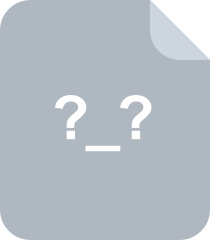
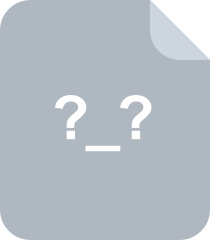
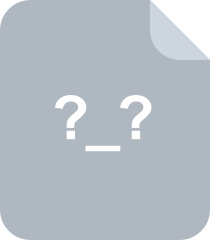
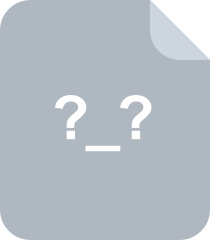
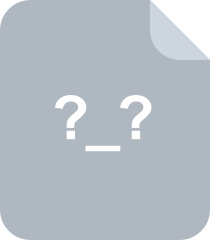
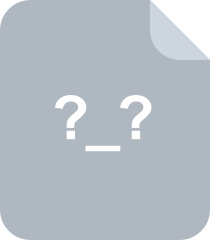
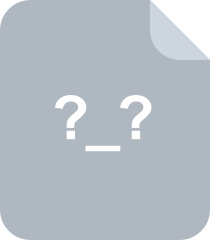
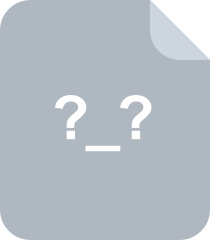
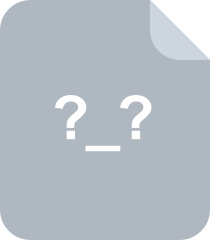
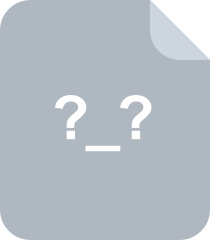
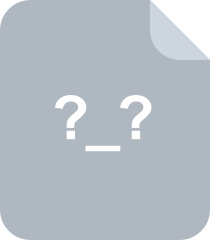
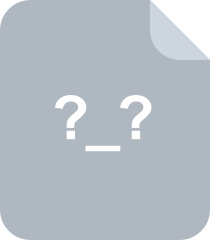
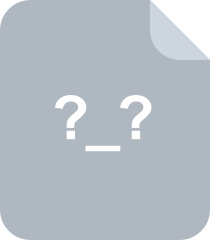
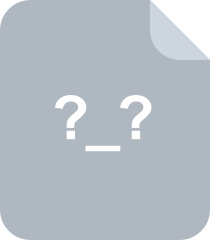
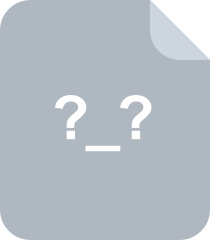
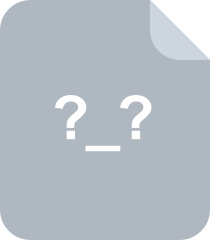
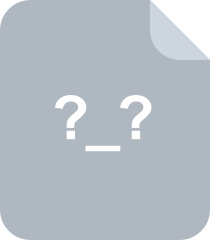
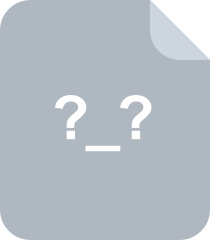
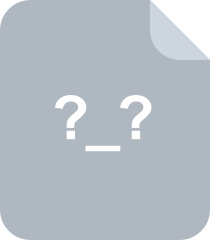
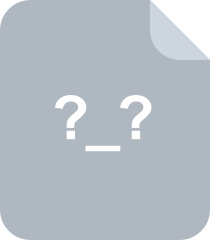
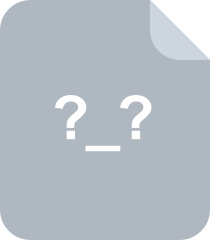
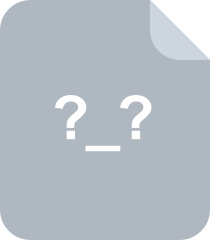
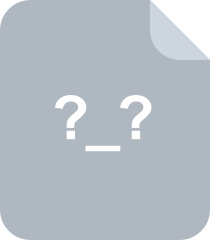
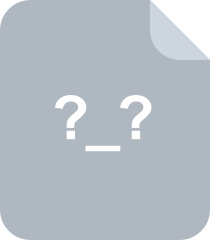
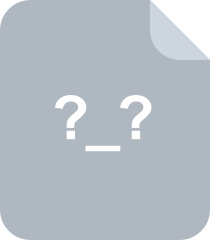
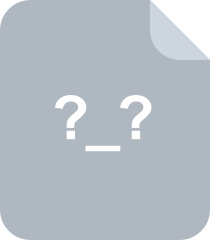
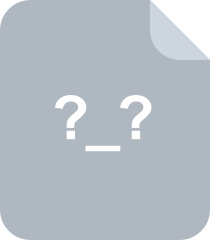
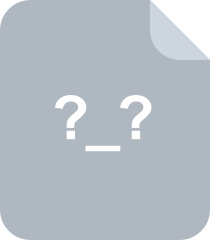
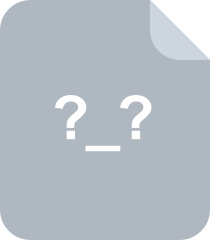
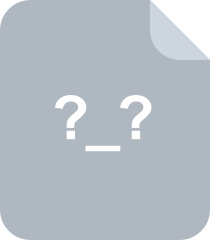
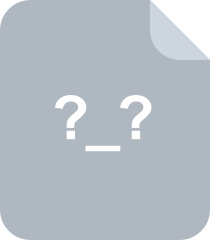
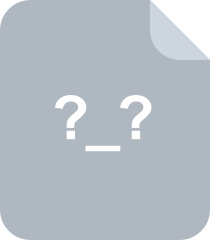
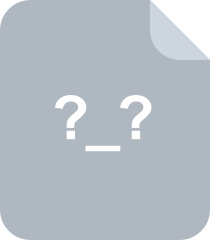
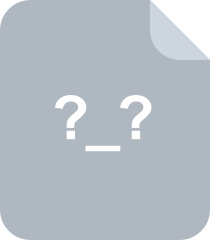
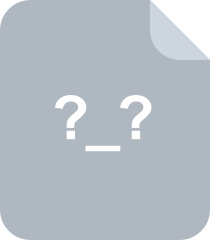
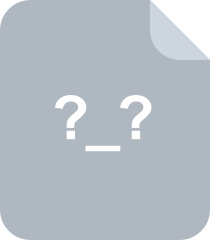
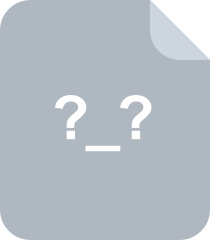
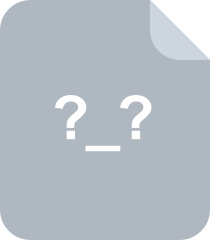
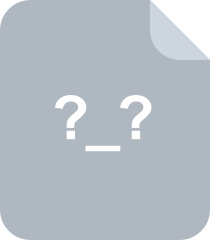
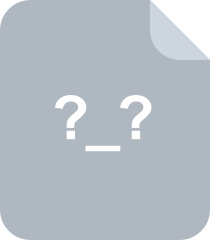
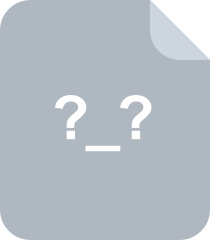
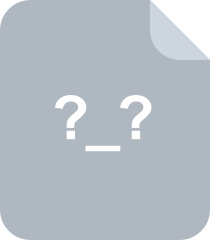
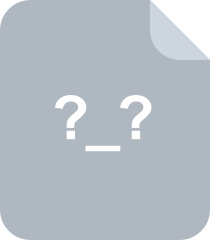
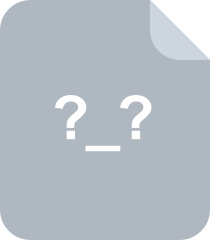
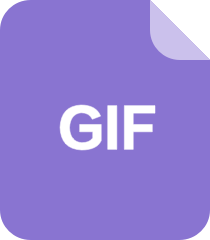
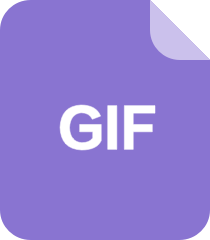
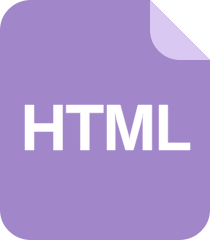
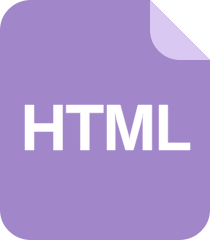
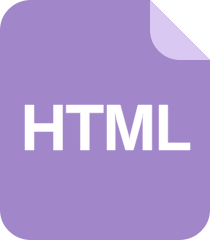
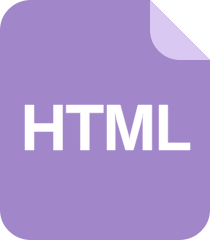
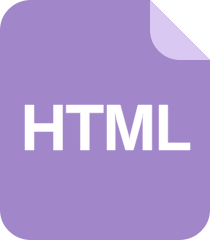
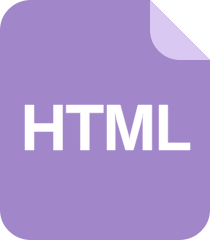
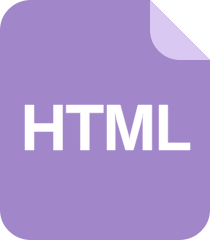
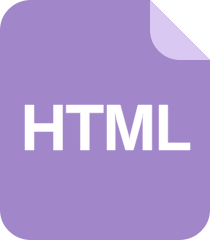
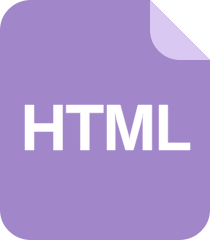
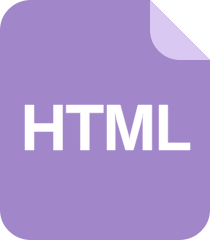
共 866 条
- 1
- 2
- 3
- 4
- 5
- 6
- 9
资源评论
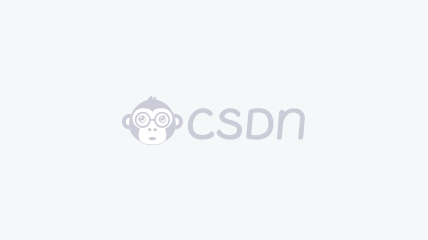

天天501
- 粉丝: 626
- 资源: 5904
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

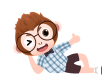
最新资源
- 计算机专业前端React入门开发一个小应用教程
- 新能源汽车车载双向OBC,PFC,LLC,V2G 双向 充电桩 电动汽车 车载充电机 充放电机 MATLAB仿真模型 (1)基于V2G技术的双向AC DC、DC DC充放电机MATLAB仿真模型; (
- 图像分割数据集:房屋建筑图像语义分割数据集(21类分割,约400张数据和标签)
- LED恒流驱动器Hi600X系列高精度调光及应用详解
- 安全隐患自查表.docx
- “私车公养”问题自查自纠表.docx
- 变压器损耗对照表.docx
- 部门年度绩效自评工作报告(参考提纲).docx
- 创客人才基础人才购房补贴申请表.doc
- 创客人才基础人才购房优惠资格认定表.doc
- 创新型领军和拔尖人才购房安家补助申请表.doc
- 大型医院巡查工作方案(2025-2026年度).docx
- 单位内设机构领导职数情况对照表.doc
- 电力变压器损耗及损失电量速算表.docx
- 独生子女户、农村纯二女结扎户子女审核确认花名册.wps
- 发放劳务费明细表.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


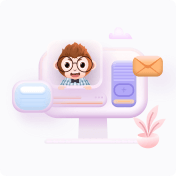
安全验证
文档复制为VIP权益,开通VIP直接复制
