"""
#!/usr/bin/env python
# -*- coding:utf-8 -*-
@Project : project1
@File : main_interface.py
@Author : 19082
@Time : 2023/9/17 21:01
"""
import datetime
import time
from tkinter import *
from tkinter.messagebox import *
from tkinter import filedialog
global question_image
global question_photo
global question_path
global answer_photo
global answer_image
global question_new_path
global question_new_name
global first_global
global easy_question_list
class main_interface(object):
def __init__(self, master=None):
self.root = master # 定义内部变量root
self.root.geometry('%dx%d' % (1000, 800)) # 设置窗口大小
self.username = StringVar()
self.password = StringVar()
self.createPage()
def createPage(self):
self.page0 = Frame(self.root) # 创建Frame
self.page0.pack()
Label(self.page0, text='杜晓扬考研错题本').grid(row=0, stick=W, pady=30)
Button(self.page0, text='添加错题', command=self.add_all_question).grid(row=1, pady=5)
Button(self.page0, text='错题回看', command=self.review_all_question).grid(row=2, pady=5)
def add_all_question(self):
self.page0.destroy()
self.page = Frame(self.root)
self.page.pack()
Label(self.page, text='杜晓扬考研错题本').grid(row=0, stick=W, pady=30)
Button(self.page, text='高数', command=self.higher_mathematics_page).grid(row=1, pady=5)
Button(self.page, text='现代', command=self.linear_algebra_page).grid(row=2, pady=5)
Button(self.page, text='概率论', command=self.probabilism_page).grid(row=3, pady=5)
Button(self.page, text='专业课', command=self.special_disciplines_page).grid(row=4, pady=5)
Button(self.page, text='英语阅读', command=self.read_page).grid(row=5, pady=5)
Button(self.page, text='英语翻译', command=self.translation_page).grid(row=6, pady=5)
Button(self.page, text='英语作文', command=self.composition_page).grid(row=7, pady=5)
def higher_mathematics_page(self):
import tkinter
import tkinter as tk
from tkinter import ttk
global question_photo
global answer_photo
global question_type
global question_new_path
global answer_new_path
global question_label
global question_grade
question_photo = None
answer_photo = None
self.page.destroy()
self.page1 = Frame(self.root)
self.page1.pack()
question_type = "高数"
question_new_path = "F:\study\question\higher_mathematics"
answer_new_path = "F:\study\snswer\higher_mathematics"
Label(self.page1, text='考研高数').grid(row=0, stick=W, pady=30)
Button(self.page1, text='导入题目', command=self.add_question).grid(row=1, pady=0)
self.question_path = StringVar()
Entry(self.page1, state='readonly', text=self.question_path, width=100).grid(row=2, pady=0)
Button(self.page1, text='导入答案', command=self.add_answer).grid(row=3, pady=3)
self.answer_path = StringVar()
Entry(self.page1, state='readonly', text=self.answer_path, width=100).grid(row=4, pady=0)
Button(self.page1, text='加入题库', command=self.add_question_bank).grid(row=5, pady=3)
self.question_grade = StringVar()
Entry(self.page1, textvariable=self.question_grade).grid(row=6, column=1, stick=E)
Button(self.page1, text='难度等级', command=self.add_question_grade).grid(row=6, pady=3)
self.question_image_show = Label(self.page1, image=question_photo)
self.question_image_show.grid(column=7, row=1)
self.answer_image_show = Label(self.page1, image=answer_photo)
self.answer_image_show.grid(column=7, row=2)
def add_question_grade(self):
global question_grade
question_grade = self.question_grade.get()
print(question_grade)
def add_question(self):
import tkinter as tk
import tkinter.filedialog
from PIL import Image, ImageTk
global question_image
global question_photo
global question_path
global question_new_path
global question_new_name
global new_name
global question_label
global question_grade
question_path = filedialog.askopenfilename(title='选择文件', filetypes=[(('JPG', '*.jpg')), ('All Files', '*')])
question_image = Image.open(question_path)
question_image.show()
new_size = (200, 200)
question_image = question_image.resize(new_size)
question_photo = ImageTk.PhotoImage(question_image) # 实际上是把这个变成全局变量
self.question_image_show.configure(image=question_photo)
self.question_image_show.image = question_photo
print(question_path)
import datetime
import time
now_time = datetime.datetime.now()
question_year = str(now_time.year)
question_month = str(now_time.month)
question_day = str(now_time.day)
question_hour = str(now_time.hour)
question_minute = str(now_time.minute)
question_second = str(now_time.second)
question_first = str("")
new_name = question_year + "" + question_month + "" + question_day + "" + question_hour + "" + question_minute + "" + question_second
question_new_name = question_first + "" + new_name
print(question_new_name)
def add_answer(self):
import tkinter as tk
import tkinter.filedialog
global answer_path
from PIL import Image, ImageTk
global answer_new_name
global answer_new_path
global answer_image
global new_name
global answer_photo
answer_path = filedialog.askopenfilename(title='选择文件', filetypes=[(('JPG', '*.jpg')), ('All Files', '*')])
answer_image = Image.open(answer_path)
answer_image.show()
answer_new_name = question_new_name
new_size = (200, 200)
answer_image = answer_image.resize(new_size)
answer_photo = ImageTk.PhotoImage(answer_image) # 实际上是把这个变成全局变量
self.answer_image_show.configure(image=answer_photo)
self.answer_image_show.image = answer_photo
print(answer_path)
def add_question_bank(self):
global answer_new_path
global question_new_path
global question_type
global question_new_name
global answer_new_name
global question_label
import mysql.connector
global answer_path
global question_path
global question_grade
global new_size
new_size = (200, 200)
# 人员表1
add_question_bank = mysql.connector.connect(
host="localhost",
user="root",
passwd="dxy123",
db='study'
)
mycursor = add_question_bank.cursor()
mysql = "insert into 高数 (题目编号,题目类型,题目地址,答案编号,答案地址,标记) values (%s,%s,%s,%s,%s,%s)"
try:
# 执行sql
mycursor.execute(mysql, (
question_new_name, question_type, question_new_path, answer_new_name, answer_new_path, question_grade))
add_question_bank.commit()
print("插入数据成功")
except Exception as e:
print(e)
add_question_bank.rollback()
print("插入数据失败")
finally:
# 关闭游标连接
mycursor.close()
# 关闭数据库连接
add_question_bank.close()
from PIL import Image
import os
question_new_name = question_new_name + "" + ".jpg"
answer_n


江湖妖兔
- 粉丝: 3
- 资源: 1
最新资源
- 中风风险预测数据集(70K记录,18特征)CSV
- 2024 年电子游戏销售数据集( 64,000 款游戏,14特征)CSV
- 龙珠数据集(用于问答项目,13K+文件)TXT
- 美国住房数据集(300 行 10 列房地产相关数据)CSV
- 每日食品与营养数据集( 1000 个独特用户的 10000 条食物摄入量数据记录,14特征)CSV
- 基于MATLAB的智能抠图系统:GUI界面与两万字详解文档,基于MATLAB的智能抠图系统:GUI界面与两万字详解文档,基于MATLAB的抠图系统+GUI界面+两万字文档 本程序利用基于学习的抠图方法
- 视觉识别windows程序
- FactoryIO液位PID仿真程序入门指南:使用TIA Portal V15与FactoryIO 2.4.0的梯形图编程实践,FactoryIO液位PID仿真程序:西门子TIA Portal V15
- filezilla服务器和客户端安装包,用于做文件传输测试等
- 内外网DeepSeek部署实战:基于Ollama的多客户端集成与安全强化方案
- PPTFancyScrollView
- Matlab遗传算法实现无人机协同任务分配方案优化:最小代价下的高效路径选择与时间分配策略,基于遗传算法优化无人机任务分配方案:航程与耗时双重考量,matlab:基于遗传算法的多无人机协同任务分配
- 基于西门子PLC与组态王技术,设计八层电梯电气控制系统:智能控制界面与组态画面动画实现,基于西门子PLC与组态王画面的八层电梯电气控制系统设计与动画展示,76#基于西门子plc和组态王八层电梯控制8层
- 内网AI知识库构建:DeepSeek离线部署全攻略,涵盖国产化适配与安全加固
- 基于MATLAB的模拟退火算法优化车辆路径问题的研究:位置定位下的最短路径选择及运输成本最低方案,基于Matlab的模拟退火算法优化VRP路径规划系统:最短路径与成本最低的车辆调度方案,基于matla
- 《互联网时代的项目管理》读书分享PPT
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


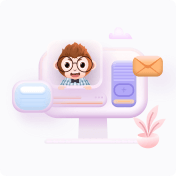