#include "openglmathmode.h"
#include "ui_openglmathmode.h"
#include <gl/GLU.h>
#include<sstream>
#include <cmath>
//gl_Position = matrixModelViewProjection * vec4(vertexPosition, 1.0);\n\
// gl_Position = vec4(aPos, 1.0);\n\
//
//顶点着色器
QString vertexShaderSource =
R"(#version 330 core
layout (location = 0) in vec3 aPos;
// varyings (output) 输出给片段着色器
varying vec3 esVertex, esNormal;
varying vec2 uv;
varying vec4 color;
varying vec4 v_position;
attribute vec3 vertexPosition;
attribute vec3 vertexNormal;
attribute vec2 uvVertex;
attribute vec4 vertexColor;
uniform mat4 matrixNormal;
uniform mat4 matrixModelView;
uniform mat4 matrixModelViewProjection;
void main()
{
esVertex = vec3(matrixModelView * vec4(aPos, 1.0));
esNormal = vec3(matrixNormal * vec4(vertexNormal, 1.0));
//color = vertexColor;
color = vec4(0.0f, 1.0f, 0.0f, 1.0f);
v_position = -matrixModelView * vec4(aPos, 1.0);
gl_Position = matrixModelViewProjection * vec4(aPos, 1.0);
uv = uvVertex;
})";
//vec4(r, g, b, a), 前三个参数表示片元像素颜色值RGB,第四个参数是片元像素透明度A,1.0表示不透明, 0.0表示完全透明。
//片段着色器只需要一个输出变量,这个变量是一个4分量向量,它表示的是最终的输出颜色,
// 我们应该自己将其计算出来。我们可以用out关键字声明输出变量,这里我们命名为FragColor。
// 下面,我们将一个alpha值为1.0(1.0代表完全不透明)的橘黄色的vec4赋值给颜色输出。
// gl_FragColor = vec4(1.0f, 1.0f, 0.0f, 1.0f);\n\ (也可使用内置变量修改颜色)
//片段着色器
QString fragmentShaderSource =
R"(#version 330 core
out vec4 FragColor;
#ifdef GL_ES
precision mediump float;
precision mediump int;
#endif
// uniforms
uniform vec4 backColor;
uniform vec4 lightPosition;
uniform vec4 lightAmbient;
uniform vec4 lightDiffuse;
uniform vec4 lightSpecular;
uniform int hsvactive;
uniform int thereisRGBA;
uniform float shininess;
uniform int glFrontFacing_1;
// varyings
varying vec3 esVertex, esNormal;
varying vec2 uv;
varying vec4 color;
varying vec4 v_position;
// All components are in the range [0…1], including hue.
uniform sampler2D imgTexture;
vec3 hsv2rgb(vec3 c)
{
vec4 K = vec4(1.0, 2.0 / 3.0, 1.0 / 3.0, 3.0);
vec3 p = abs(fract(c.xxx + K.xyz) * 6.0 - K.www);
return c.z * mix(K.xxx, clamp(p - K.xxx, 0.0, 1.0), c.y);
}
void main()
{
vec4 color1=color;
vec3 normal = normalize(esNormal);
vec3 light;
if (lightPosition.w == 0.0)
{
light = normalize(-lightPosition.xyz);
}
else
{
light = normalize(lightPosition.xyz - esVertex);
}
if (hsvactive == 1)
color1 = vec4(hsv2rgb(color1.xyz), color1.w);
vec3 view = normalize(-esVertex);
vec3 halfv = normalize(light + view);
vec4 fragColor = lightAmbient * color1;
float dotNL = max(dot(normal, light), 0.0f);
fragColor += lightDiffuse * dotNL * color1; // add diffuse
float dotNH = max(dot(normal, halfv), 0.0);
vec3 LightReflect = normalize(reflect(halfv, normal));
float SpecularFactor = dot(vec3(0.0f, 0.0f, 1.0f), -LightReflect);
if (SpecularFactor > 0) {
SpecularFactor = pow(SpecularFactor, shininess);
fragColor += lightSpecular * 1.0f * SpecularFactor;
}
if (glFrontFacing_1 == 1){
FragColor = vec4(1.0, 0.0f, 0.0f, 1.0f);
}
else if (glFrontFacing_1 == 2) {
FragColor = vec4(0.6f, 0.6f, 0.6f, 1.0f);
}
else if (glFrontFacing_1 == 3) {
FragColor = vec4(1.0f, 0.0f, 0.0f, 1.0f);
}
else if (glFrontFacing_1 == 4) {
FragColor = vec4(0.0f, 1.0f, 0.0f, 1.0f);
}
else if (glFrontFacing_1 == 5) {
FragColor = vec4(0.0f, 0.0f, 1.0f, 1.0f);
}
else if (glFrontFacing_1 == 6) {
FragColor = texture(imgTexture, uv);
}
else {
float back = dot(vec3(0.0f, 0.0f, 1.0f), normal);
if (back < 0.0f) {
FragColor = vec4(1.0f, 1.0f, 0.0f, 1.0f);
}
else {
if (glFrontFacing_1 == 6) {
FragColor = texture(imgTexture, uv);
}
else {
FragColor = fragColor;
}
}
}
})";
OpenglMathMode::OpenglMathMode(QWidget* parent)
: QGLWidget(parent)
, viewPortWidth(1600.0)
, viewPortHeight(1000.0)
{
m_texture = nullptr;
}
OpenglMathMode::~OpenglMathMode()
{
}
/*
QOpenGLFunctions::initializeOpenGLFunctions(): 为当前上下文初始化OpenGL函数解析。调用此函数后,QOpenGLFunctions对象只能与当前上下文以及与其共享的其他上下文一起使用。再次调用initializeOpenGLFunctions()以更改对象的上下文关联。
QOpenGLContext::makeCurrent(): 在给定的surface上使当前线程中的上下文成为当前上下文。成功返回true, 否则返回false。如果表面未暴露,或者由于例如应用程序被挂起而导致图形硬件不可用,则后者可能发生。
QOpenGLContext::swapBuffers(): 交换渲染表面的前后缓冲区。调用此命令以完成OpenGL渲染的框架,并确保在发出任何其他OpenGL命令(例如,作为新框架的一部分)之前再次调用makeCurrent()。
*/
void OpenglMathMode::initializeGL()
{
//设置裁剪面
glViewport(0, 0, viewPortWidth, viewPortHeight);
//开启深度测试
glEnable(GL_DEPTH_TEST);
m_texture = new QOpenGLTexture(QImage("D:/5.png")); //5.jpg
//计算投影矩阵
proj();
//为当前上下文初始化OpenGL函数解析
initializeGLFunctions();
//初始化着色器
InitShader();
//初始化缓冲区
InitBuffer();
}
void OpenglMathMode::resizeGL(int w, int h)
{
if (w > 300) {
viewPortWidth = w;
viewPortHeight = h;
}
//计算投影矩阵
proj();
}
/*
//视图矩阵
QMatrix4x4 view;
view.setToIdentity();//对矩阵进行单位化,保证所有矩阵运算都会在一个单位矩阵上进行
view.lookAt(QVector3D(2, 2, 2), //眼睛的位置
QVector3D(0, 0, 0), //眼睛看的位置
QVector3D(0, 1, 0)); //此参数表示方向,相对于眼睛向上的方向。
//模型矩阵
QMatrix4x4 model;
model.setToIdentity();
model.rotate(QTime::currentTime().msec(), 1.0f, 5.0f, 0.5f);//旋转 该矩阵将坐标绕矢量 (x, y, z) 旋转angle度
model.scale(0.6f);//缩放
*/
void OpenglMathMode::paintGL()
{
resize(viewPortWidth, viewPortHeight);
glViewport(0, 0, viewPortWidth, viewPortHeight);
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
int i = 1000;
glLoadName(i++);
glPushMatrix();
// set modelview matrix
matrixViewx.setToIdentity();
matrixViewx.translate(camera_pos);
matrixViewx.rotate(rotation);
matrixViewx.translate(translate.x(), translate.y(), translate.z());
matrixModelViewProjectionx = matrixProjectionx * matrixViewx;
matrixNormalx = matrixViewx;
matrixNormalx.setColumn(3, QVector4D(0, 0, 0, 1));
indexBuffer->bind();
uvBuffer->bind();
m_texture->bind();
shader.bind();
glUniformMatrix4fv(uniformMatrixModelView, 1, false, matrixViewx.data());
glUniformMatrix4fv(uniformMatrixModelViewProjection, 1, false, matrixModelViewProjectionx.data());
glUniformMatrix4fv(uniformMatrixNormal, 1, false, matrixNormalx.data());
shader.enableAttributeArray("uvVertex");
shader.enableAttributeArray("vertexNormal");
shader.enableAttributeArray("aPos");
shader.setUniformValue("glFrontFacing_1", 0);
// 抗锯齿
//glEnable(GL_LINE_SMOOTH);
//glHint(GL_LINE_SMOOTH, GL_NICEST);
// 多边形偏移
glEnable(GL_POLYGON_OFFSET_FILL);
glPolygonOffset(1.0f, 1.0f);
// 模型主体绘制
glDrawElements(GL_TRIANGLES, indices.size(), GL_UNSIGNED_INT, 0);
glDisable(GL_POLYGON_OFFSET_FILL);
glPopMatrix();
shader.setUniformValue("glFrontFacing_1", 1);
// 设置网格线的宽度
glLineWidth(0.4f);
// 模型网格绘制
glDrawElements(GL_LINE_LOOP, indices.size(), GL_UNSIGNED_INT, 0);
//世界网格绘制
shader.setUniformValue("glFrontFacing_1", 2);
glLineWidth(0.3);
glDrawArrays(GL_LINES, PlanStartIndex, 80);
//X轴绘制
shader.setUniformValue("glFrontFacing_1", 3);
glLineWidth(3.0);
glDrawArrays(GL_LINES, PlanStartIndex + 80, 2);
//Y轴绘制
shader.setUniformValue("glFrontFacing_1", 4);
glDrawArrays(GL_LINES, PlanStartIndex + 82, 2);
//Z轴绘制
shader.setUniformValue("glFrontFacing_1", 5);
glDrawArrays(GL_LINES, PlanStartIndex + 84, 2);
glLoadName(i++);
glPushMatrix();
// set modelview matrix
matrixViewx.setToIdentity();
matrixViewx.translate(QVector3D(-14.0, 0.0, -14.0));
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
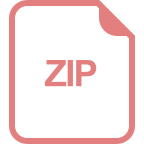
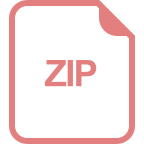
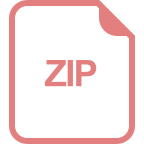
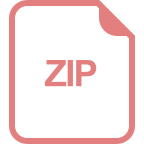
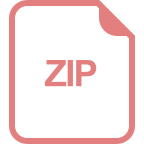
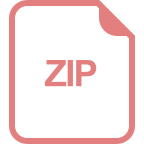
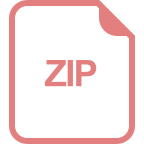
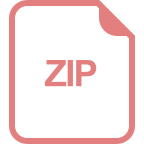
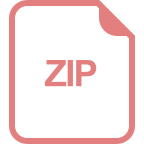
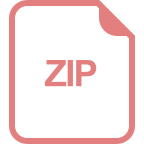
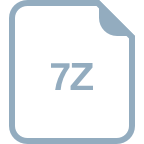
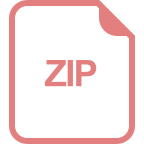
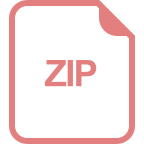
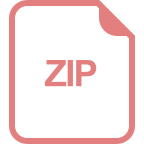
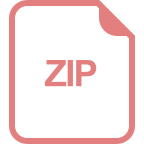
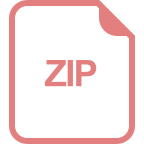
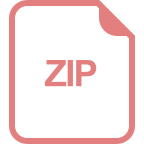
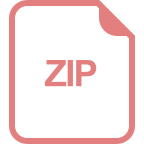
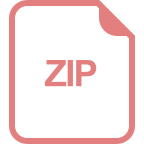
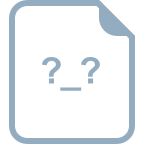
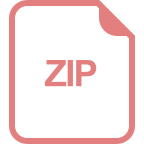
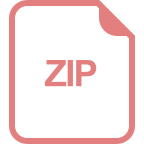
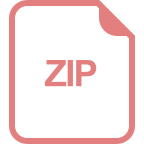
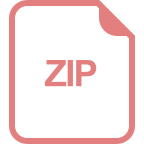
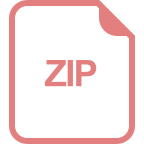
收起资源包目录


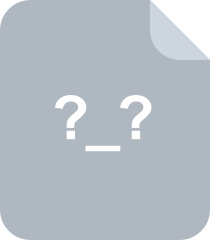
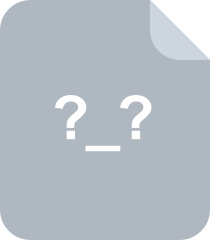
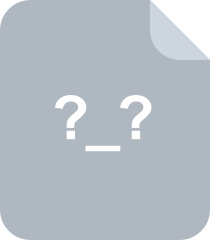
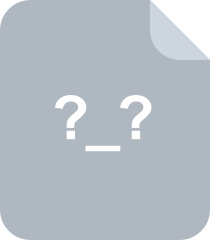
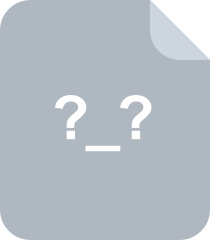
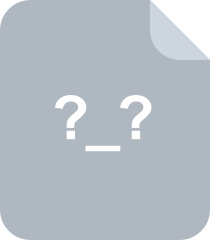
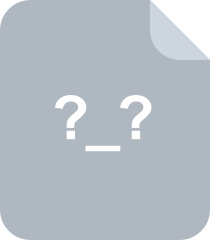
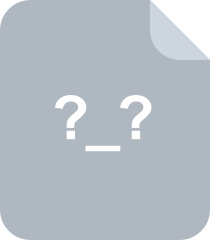
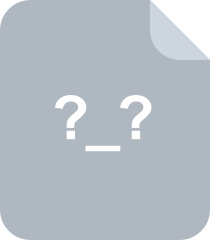
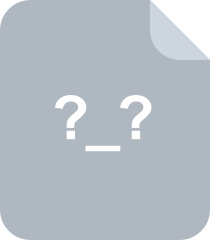
共 10 条
- 1
资源评论
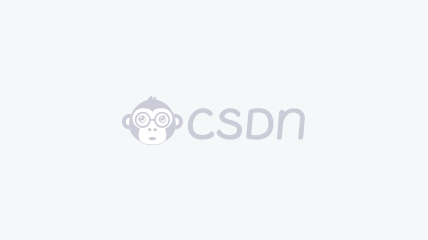

好家伙VCC
- 粉丝: 2041
- 资源: 9145

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

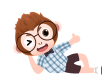
最新资源
- 2010-2020年31省第三产业占GDP比重(%)
- elasticsearch-analysis-ik 8.16.0
- HTML5实现剪刀石头布小游戏源码
- (源码)基于ArduinoIDE的ESP32CAMQR码识别系统.zip
- elasticsearch-analysis-hanlp
- 人工智能-增量学习基于密度峰值聚类和共享最近邻的IIOT机械半监督自训练故障诊断(源码+数据+说明文档).zip
- C#ASP.NET人力资源HR系统源码数据库 SQL2008源码类型 WebForm
- 基于滴滴平台211个城市数据测算的城市发展环境指数【重磅,更新!】
- (源码)基于NodeMCU和MQTT的温湿度监测系统.zip
- 一周入门FPGA源代码资料
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


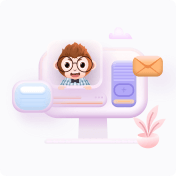
安全验证
文档复制为VIP权益,开通VIP直接复制
