/*Title: mjbWorld
Copyright (c) 1998-2005 Martin John Baker
This program is free software; you can redistribute it and/or
modify it under the terms of the GNU General Public License
as published by the Free Software Foundation; either version 2
of the License, or (at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
For information about the GNU General Public License see http://www.gnu.org/
To discuss this program http://sourceforge.net/forum/forum.php?forum_id=122133
also see website http://www.euclideanspace.com/
translated to C++ by Ed Sinjiashvili (slorcim@sf.net)
*/
#include <qobjectlist.h>
#include "transformGroupBean.h"
#include "vetoPropertyChange.h"
#include "d_button.h"
#include "sfvec3f.h"
#include "mfint32.h"
#include "mfvec3f.h"
#include "sfrotation.h"
#include "mfparam.h"
#include "sfvec3fEditor.h"
#include "sfrotationEditor.h"
#include "sftransform.h"
#include "sceneBean.h"
#include "sftransformEditor.h"
#include "behaviorBean.h"
namespace mjbModel
{
int transformGroupBean::TOOL_CONVERTTOJOINT = 1;
QString transformGroupBean::PAR_ROTATION = "rotation";
QString transformGroupBean::PAR_CENTRE = "center";
QString transformGroupBean::PAR_SCALE = "scale";
QString transformGroupBean::PAR_SCALEORIENTATION = "scaleOrientation";
QString transformGroupBean::PAR_TRANSLATION = "translation";
QString transformGroupBean::PAR_TRANSFORM = "transform";
QString transformGroupBean::PAR_MATRIX = "matrix";
sfvec3f& transformGroupBean::defaultCenter()
{
static sfvec3f d(0.0, 0.0, 0.0);
return d;
}
sfrotation& transformGroupBean::defaultRotation()
{
static sfrotation d(0,0,1,0.0);
return d;
}
sfvec3f& transformGroupBean::defaultScale()
{
static sfvec3f d(1.0,1.0,1.0);
return d;
}
sfrotation& transformGroupBean::defaultScaleOrientation()
{
static sfrotation d(0,0,1,0.0);
return d;
}
sfvec3f& transformGroupBean::defaultTranslation()
{
static sfvec3f d(0.0,0.0,0.0);
return d;
}
transformGroupBean::~transformGroupBean ()
{
delete vetos;
}
transformGroupBean::transformGroupBean ()
: groupBean (),
matrix (0), matrix1 (0), matrix2 (0),
matrix3 (0), matrix4 (0),
top (0), bottom (0), mult (0)
{
vetos = new vetoPropertyChange;
// sets up children params
params_->insert(new sfparam(sfparam::EXPOSEDFIELD,
sfvec3f::Factory (),
PAR_CENTRE, &defaultCenter()));
params_->insert(new sfparam(sfparam::EXPOSEDFIELD,
sfrotation::Factory (),
PAR_ROTATION,&defaultRotation()));
params_->insert(new sfparam(sfparam::EXPOSEDFIELD,
sfvec3f::Factory (),
PAR_SCALE, &defaultScale()));
params_->insert(new sfparam(sfparam::EXPOSEDFIELD,
sfrotation::Factory (),
PAR_SCALEORIENTATION, &defaultScaleOrientation()));
params_->insert(new sfparam(sfparam::EXPOSEDFIELD,
sfvec3f::Factory (),
PAR_TRANSLATION, &defaultTranslation()));
applyTemp = false;
}
void transformGroupBean::setDefaultTranslation(sfvec3f* /*d*/)
{
sfparam* par = params_->findSFParam(PAR_TRANSLATION);
par->setDefault(new sfvec3f(1000.0,1000.0,1000.0));
}
void transformGroupBean::setTrans(sfvec3f* shift, mfint32* instance)
{
set(PAR_TRANSLATION,shift,instance);
//Console.WriteLine("transformGroupBean::setTranslation("+shift+","+instance+")");
int inst =0;
if (instance!=0)
if(instance->howmany()>0) inst=instance->getLastValue();
setMatrix(calcTransform(inst, 0, 0, 0, 0, 0),inst);
// Console.WriteLine("tranformGroupBean::setTranslation("+instance+") veto " + pve->getPropertyChangeEvent());
}
void transformGroupBean::setTranslationAndRotation(sfparam* shift, sfparam* rot,
nodeBean* /*callback*/)
{
//Console.WriteLine("transformgroupBean.setTranslationAndRotation("+shift+","+callback.generateUniqueName()+") this="+generateUniqueName());
if (shift!=0)
{
sfparam* par = params_->findSFParam(PAR_TRANSLATION);
vector<mjbModel::Property*>* vecs = shift->getArray();
par->setArray(vecs);
if (vecs==0)
{
// if array is 0
// FIXME: try/catch
// try {
//Console.WriteLine("transformgroupBean.setTranslation("+shift+") vecs==0");
vetos->fireVetoableChange(PAR_TRANSLATION,0,shift); // if translation==shift veto is not fired
/*
} catch (ConstraintException pve){
if (callback is kinematicsBean) {
((kinematicsBean)callback).veto(0,pve.Message);
}
}
*/
}
else
{
// if array is not 0
//Console.WriteLine("transformgroupBean.setTranslation("+shift+") vecs!=0 this="+generateUniqueName());
// int[] instance = new int[1];
for (int i=0;i < (int)vecs->size ();i++)
{
// instance[0]=i;
//Console.WriteLine(" >transformgroupBean.setTranslation("+shift+") i="+i+" this="+generateUniqueName());
setMatrix(calcTransform(i,0,0,0,0,0),i); // setMatrix updates Java3D scenegraph
if ( (*vecs)[i] !=0)
{
// FIXME: try/catch
// try {
//Console.WriteLine("transformgroupBean.setTranslation vecs["+i+"]"+vecs[i]);
vetos->fireVetoableChange(PAR_TRANSLATION,0, (*vecs)[i]); // if translation==shift veto is not fired
//Console.WriteLine("transformgroupBean.setTranslation veto not fired");
/*
} catch (ConstraintException pve){
//Console.WriteLine("transformgroupBean.setTranslation veto fired");
if (callback is kinematicsBean) {
((kinematicsBean)callback).veto(i,pve.Message);
}
}
*/
}
}
}
}
if (rot!=0)
{
vector<mjbModel::Property*>* vecs = rot->getArray();
sfparam* par2 = params_->findSFParam(PAR_ROTATION);
par2->setArray(vecs);
if (vecs==0)
{
// FIXME: try/catch
// try {
//Console.WriteLine("transformgroupBean.setRotation("+shift+") vecs==0");
vetos->fireVetoableChange(PAR_ROTATION,0,rot); // if translation==shift veto is not fired
/*
} catch (ConstraintException pve){
if (callback is kinematicsBean) {
((kinematicsBean)callback).veto(0,pve.Message);
}
}
*/
}
没有合适的资源?快使用搜索试试~ 我知道了~
mjbWorld_cpp_qt_opengl_source_525a.zip
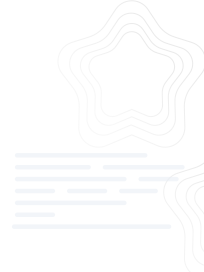
共416个文件
h:196个
cpp:188个
root:4个

需积分: 5 0 下载量 16 浏览量
2024-09-21
12:18:09
上传
评论
收藏 777KB ZIP 举报
温馨提示
C++三维程序
资源推荐
资源详情
资源评论














收起资源包目录

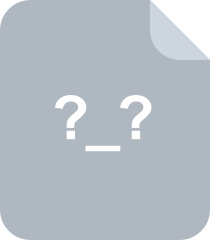
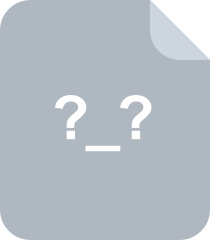
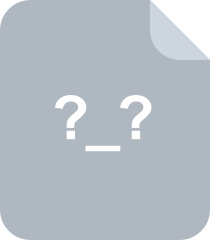
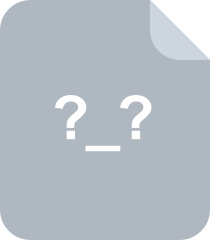
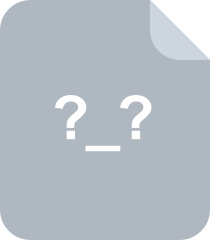
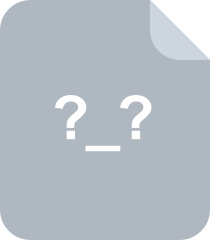
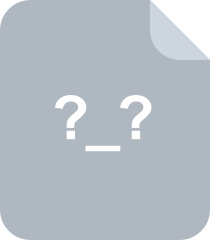
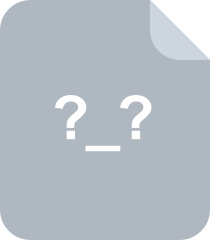
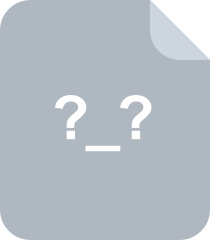
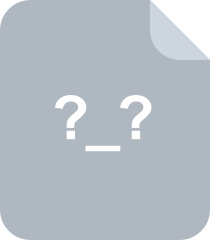
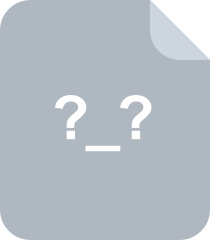
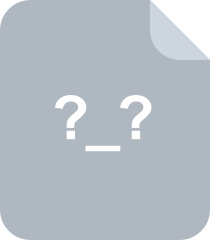
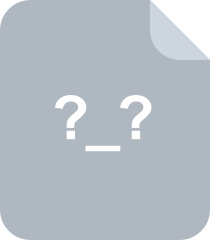
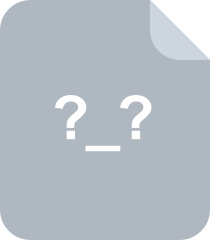
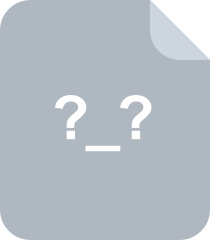
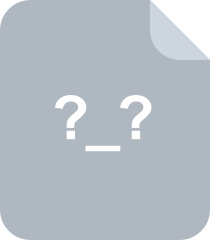
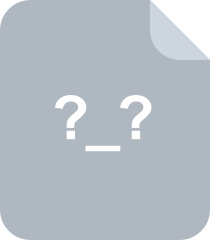
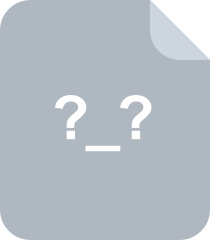
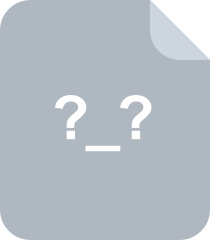
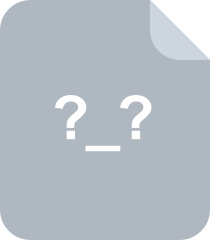
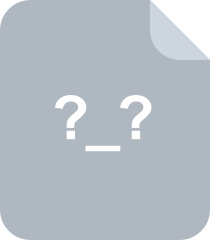
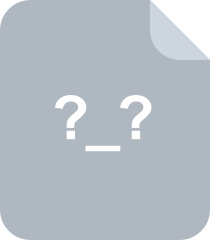
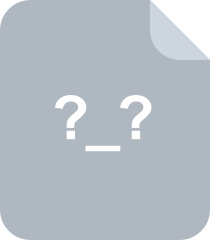
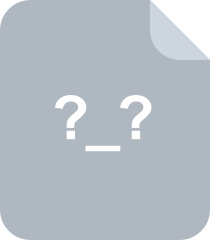
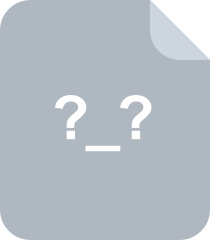
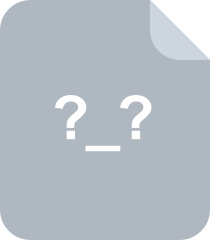
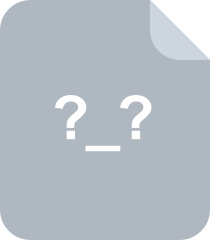
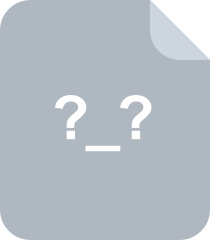
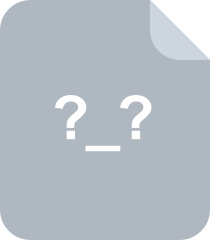
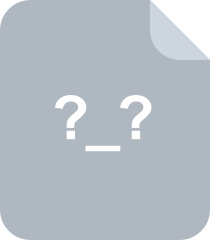
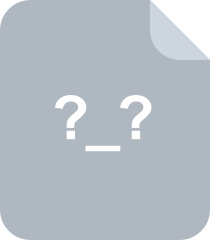
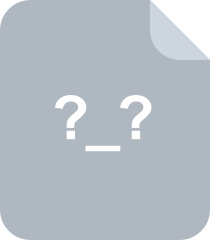
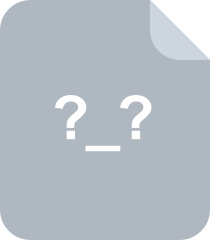
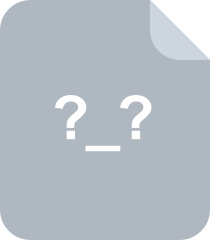
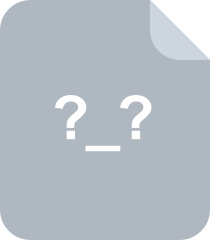
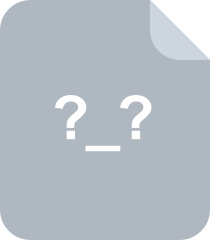
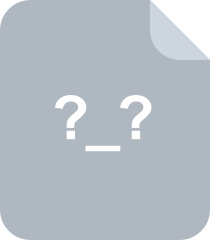
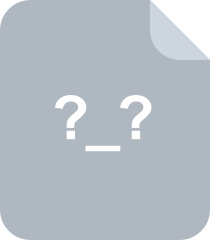
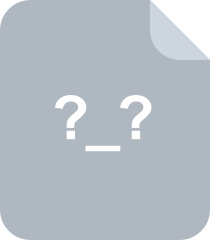
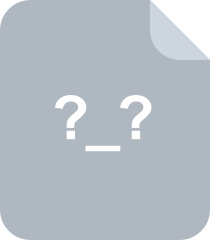
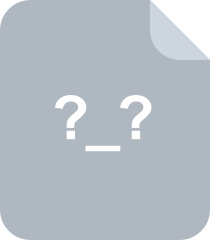
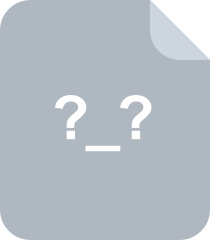
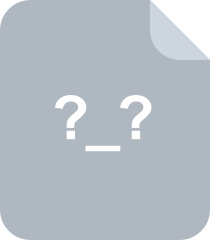
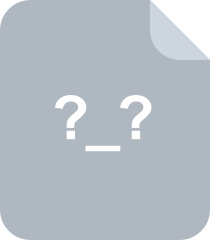
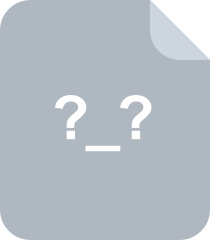
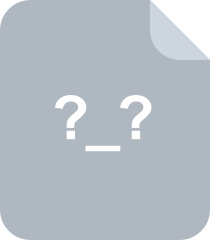
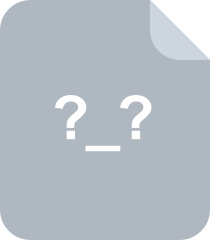
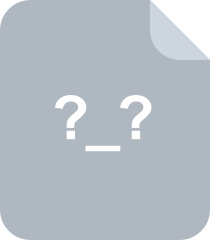
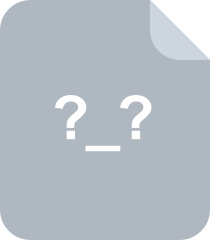
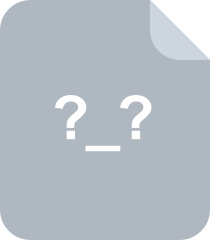
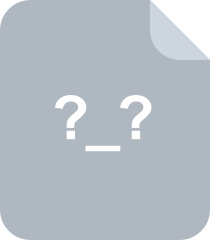
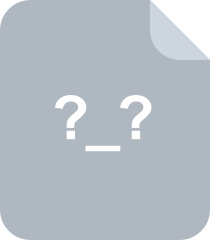
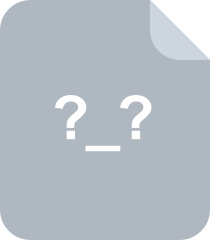
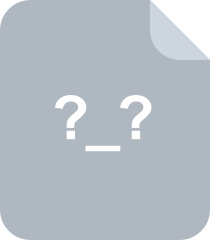
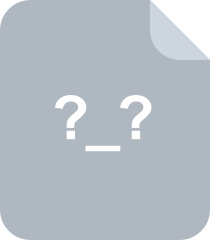
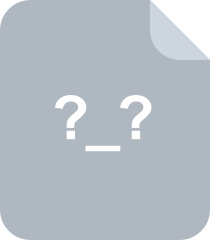
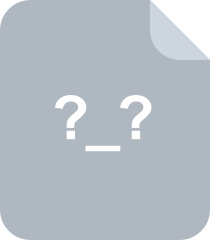
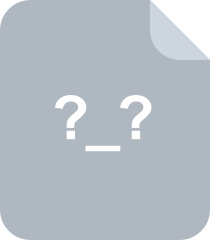
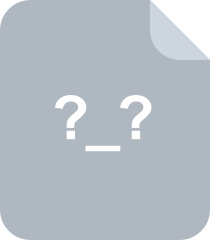
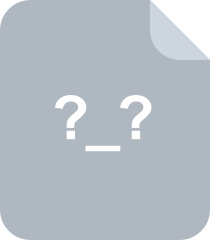
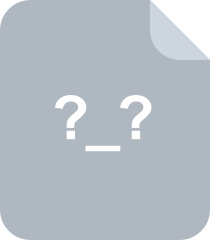
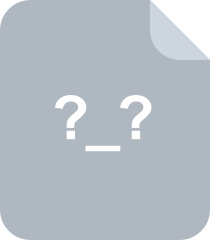
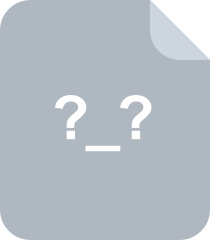
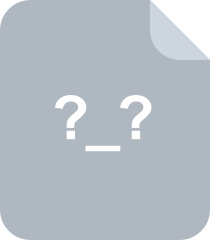
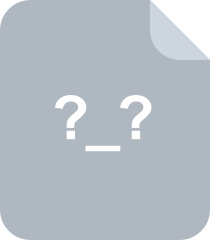
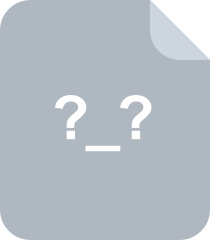
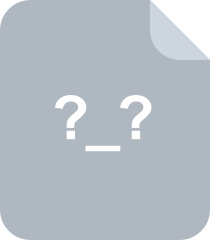
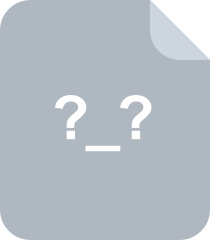
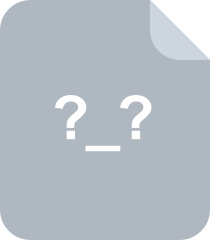
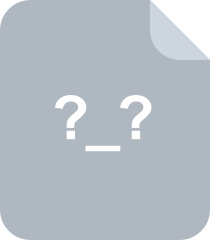
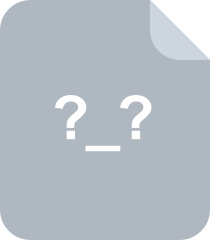
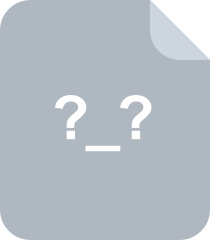
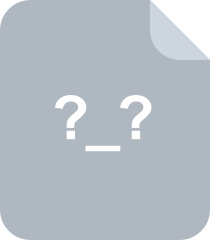
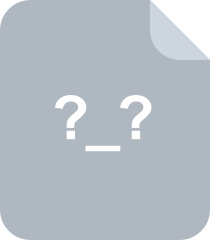
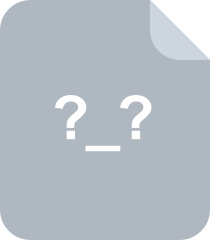
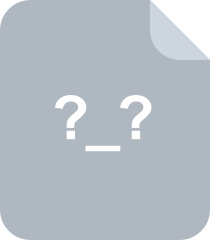
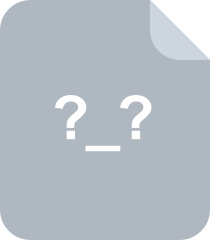
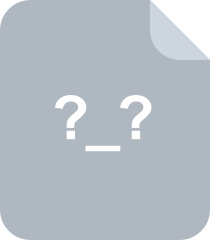
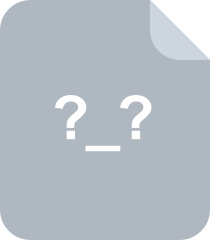
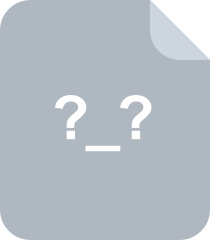
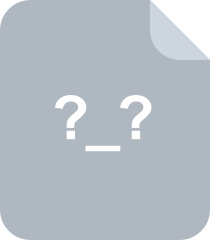
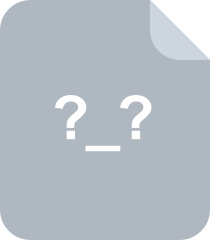
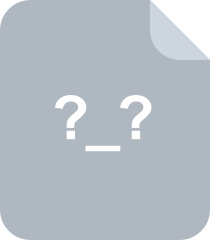
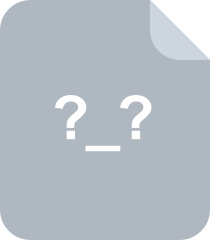
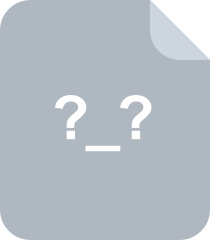
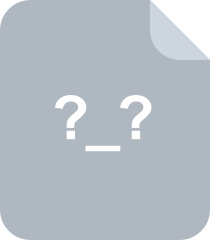
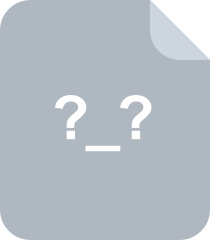
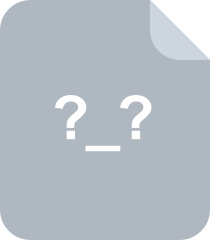
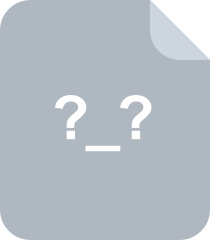
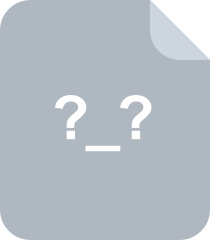
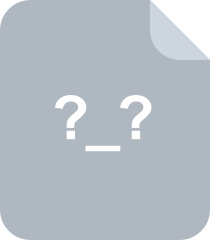
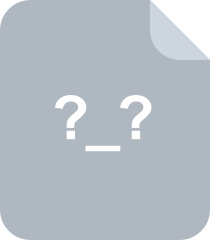
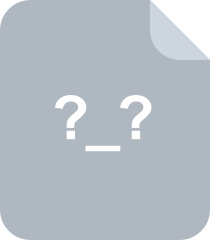
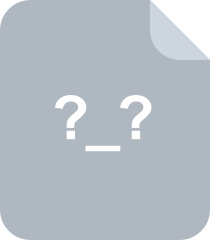
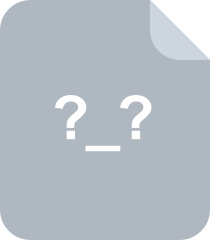
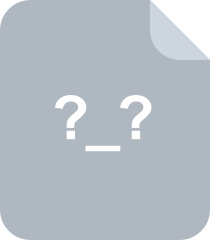
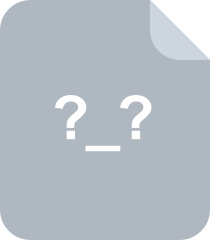
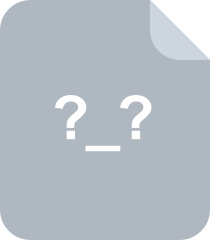
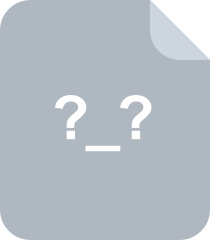
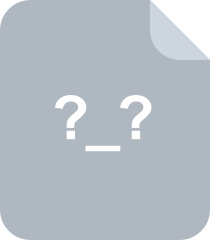
共 416 条
- 1
- 2
- 3
- 4
- 5
资源评论
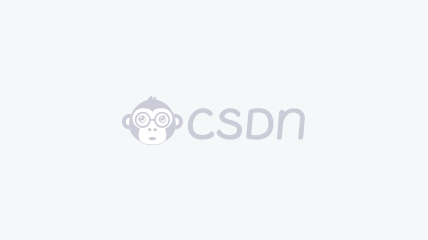

fanxbl957
- 粉丝: 4156
- 资源: 5655
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

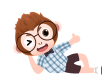
最新资源
- 管家婆普及版TOP9.16.zip
- ObjectARX 2025
- 电动汽车动力系统匹配与整车动力经济性计算模型:参数输入一键生成,仿真模型助力项目实践,电动汽车动力系统匹配与整车动力经济性计算模型:一键生成参数,助力高效研发仿真设计,电动汽车动力系统匹配计算模型:输
- 管家婆普及版TOP15.0.zip
- JellySprites
- chap1threading1.py
- 管家婆普及版TOP12.6.zip
- 一个随机随林的演示代码
- Deepseek使用提问公式-全是技巧
- A02114237余瑶开题报告.docx
- GESP 2024年12月认证 Python 1-6级真题和答案.rar
- 计算机软考备战指南-备考攻略详解与成功秘籍
- 管家婆普普版TOP 12.9.zip
- 管家婆普普版TOP 12.71.zip
- 管家婆普普版TOP 12.6.zip
- 【matlab代码】四个模型的IMM(交互式多模型)例程,四模型分别为:CV(匀速)、CA(匀加速)、CS(匀加加速度)、CT(匀速转弯),滤波使用EKF
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


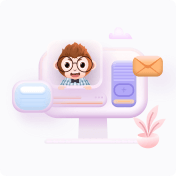
安全验证
文档复制为VIP权益,开通VIP直接复制
