import tkinter as tk
from tkinter import ttk
from Mysql import MysqlHelper
class SortFrame(tk.Frame): # 排序
def __init__(self, root):
super().__init__(root)
# tk.Label(self, text="录入页面").pack()
self.id = tk.StringVar()
self.name = tk.StringVar()
self.english = tk.StringVar()
self.python = tk.StringVar()
self.c = tk.StringVar()
self.status = tk.StringVar()
self.create_page()
def create_page(self):
columns = ("id", "name", "english", "python", "c", "sum")
columns_values = ("编号", "姓名", "英语", "python", "c语言", "总分") # 中文
self.tree_view = ttk.Treeview(self, show='headings', columns=columns) # columns=columns显示的列,show='headings'隐藏首列
for strCo in columns:
self.tree_view.column(strCo, width=80, anchor='center') # 定义列
for (strCo, strView) in zip(columns, columns_values):
self.tree_view.heading(strCo, text=strView) # 定义表头
self.tree_view.pack(fill=tk.BOTH, expand=True)
self.show_data_by_english()
tk.Label(self, text='按成绩升序排序').pack(side='left')
tk.Button(self, text='英语', command=self.show_data_by_english).pack(side='left', padx=35, pady=10, ipadx=10)
tk.Button(self, text='python', command=self.show_data_by_python).pack(side='left', padx=35, pady=10, ipadx=10)
tk.Button(self, text='c', command=self.show_data_by_c).pack(side='left', padx=35, pady=10, ipadx=10)
tk.Button(self, text='总分', command=self.show_data_by_sum).pack(side='left', padx=35, pady=10, ipadx=10)
# tk.Label(self, text='柱形图').pack(anchor=tk.SW)
# tk.Button(self, text='英语', command=self.show_data_by_english).pack(padx=35, pady=10, ipadx=10)
# tk.Button(self, text='python', command=self.show_data_by_python).pack(side='left', padx=35, pady=10, ipadx=3)
# tk.Button(self, text='c', command=self.show_data_by_c).pack(side='left', padx=35, pady=10, ipadx=20)
def show_data_by_english(self):
for _ in map(self.tree_view.delete, self.tree_view.get_children()): # 删除旧的节点(取得所有root下的列)
pass
# 从数据库中获取信息
students = MysqlHelper().sort("english")
for stu in students:
# print(stu)
self.tree_view.insert('', 'end', values=( # 插入新的一列
stu[0], stu[1], stu[2], stu[3], stu[4], stu[5]
))
def show_data_by_python(self):
for _ in map(self.tree_view.delete, self.tree_view.get_children()): # 删除旧的节点(取得所有root下的列)
pass
# 从数据库中获取信息
students = MysqlHelper().sort("python")
for stu in students:
# print(stu)
self.tree_view.insert('', 'end', values=( # 插入新的一列
stu[0], stu[1], stu[2], stu[3], stu[4], stu[5]
))
def show_data_by_c(self):
for _ in map(self.tree_view.delete, self.tree_view.get_children()): # 删除旧的节点(取得所有root下的列)
pass
# 从数据库中获取信息
students = MysqlHelper().sort("c")
for stu in students:
# print(stu)
self.tree_view.insert('', 'end', values=( # 插入新的一列
stu[0], stu[1], stu[2], stu[3], stu[4], stu[5]
))
def show_data_by_sum(self):
for _ in map(self.tree_view.delete, self.tree_view.get_children()): # 删除旧的节点(取得所有root下的列)
pass
# 从数据库中获取信息
students = MysqlHelper().sort("sum")
for stu in students:
# print(stu)
self.tree_view.insert('', 'end', values=( # 插入新的一列
stu[0], stu[1], stu[2], stu[3], stu[4], stu[5]
))
没有合适的资源?快使用搜索试试~ 我知道了~
学生信息管理系统-python-大作业
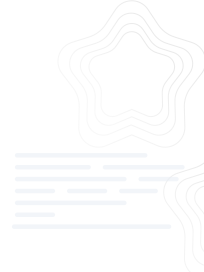
共28个文件
py:11个
pyc:9个
xml:4个

需积分: 0 2 下载量 55 浏览量
2023-07-04
17:59:10
上传
评论
收藏 25KB ZIP 举报
温馨提示
学生信息管理系统-python-大作业
资源推荐
资源详情
资源评论
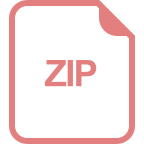
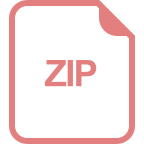
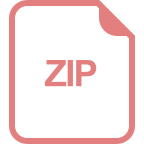
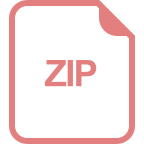
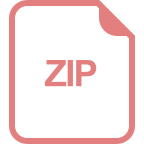
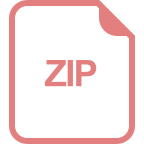
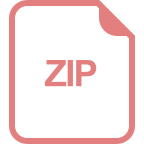
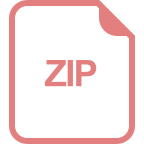
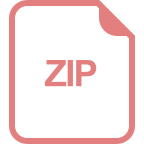
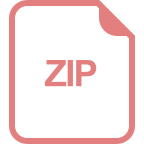
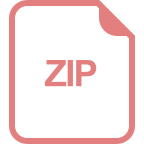
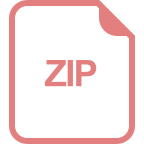
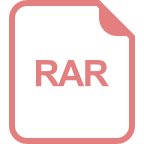
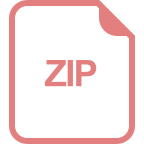
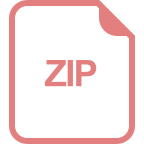
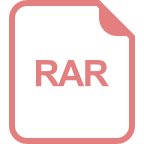
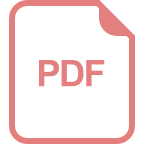
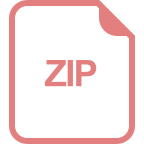
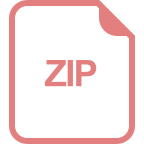
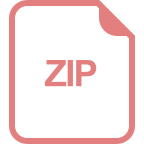
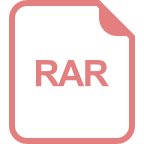
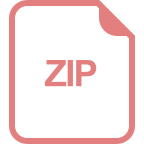
收起资源包目录




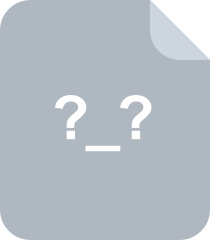
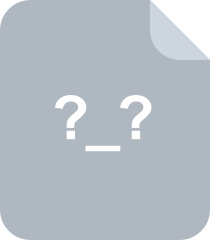
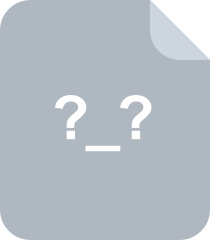
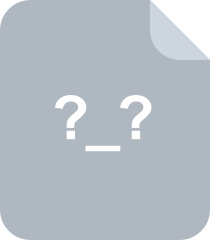

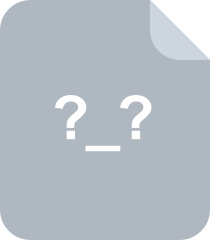
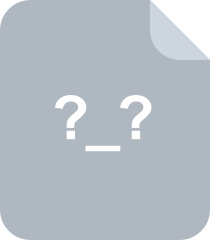
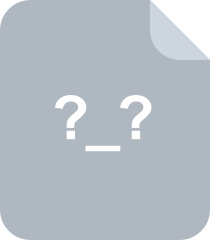
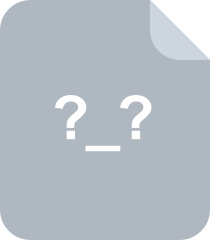

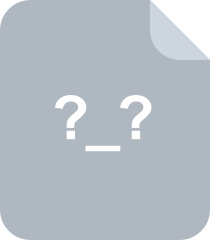
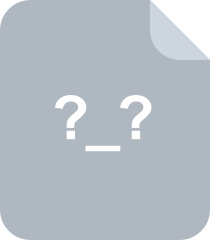
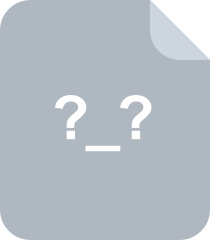
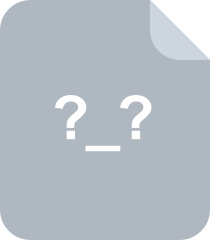
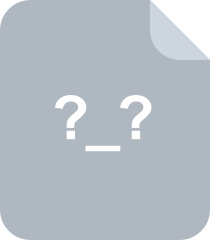

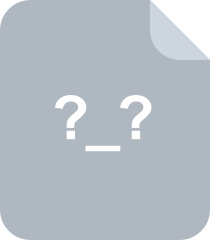
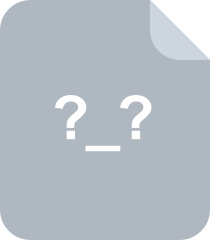
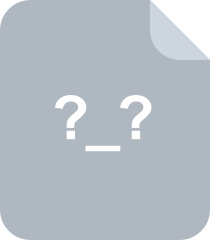
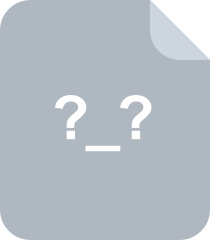
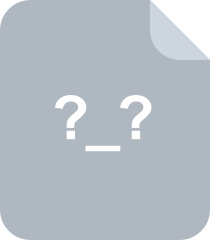
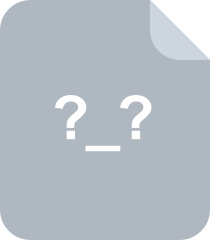
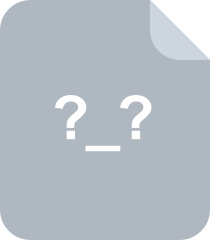
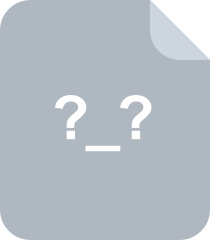
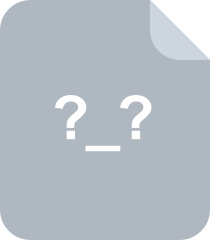

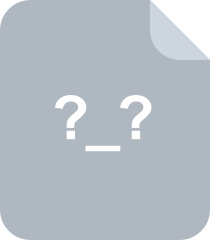
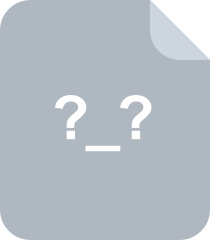
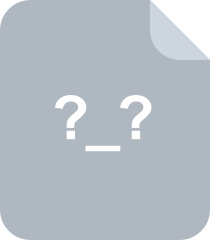
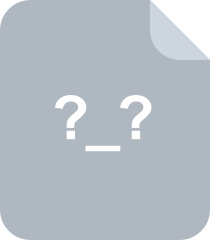
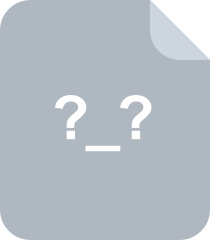
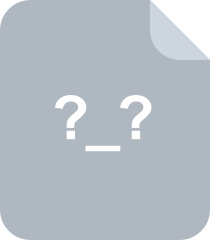
共 28 条
- 1
资源评论
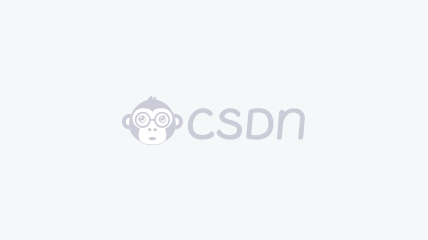

qq_45880857
- 粉丝: 1
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

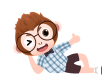
安全验证
文档复制为VIP权益,开通VIP直接复制
