package com.islizx.controller.admin;
import cn.hutool.core.lang.Validator;
import cn.hutool.extra.servlet.ServletUtil;
import com.github.pagehelper.PageInfo;
import com.islizx.config.annotation.SystemLog;
import com.islizx.entity.*;
import com.islizx.model.dto.LizxConst;
import com.islizx.model.dto.Msg;
import com.islizx.model.enums.*;
import com.islizx.service.BlogService;
import com.islizx.service.CommentService;
import com.islizx.service.MailService;
import com.islizx.vo.CommentQuery;
import com.sun.org.apache.xpath.internal.operations.Bool;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import java.util.*;
/**
* @author lizx
* @date 2020-02-14 - 15:26
*/
@Controller
@Slf4j
@RequestMapping("/admin")
public class CommentController {
@Autowired
private CommentService commentService;
@Autowired
private BlogService blogService;
@Autowired
private MailService mailService;
/**
* 评论人相关信息
*/
public static final String COMMENT_AUTHOR_IP = "ip";
public static final String COMMENT_AUTHOR = "nickname";
public static final String COMMENT_EMAIL = "email";
public static final String COMMENT_CONTENT = "content";
@RequestMapping("/comments/getpublishedsize")
@ResponseBody
public Msg getpublishedsize() {
// 三种评论类型个数
Integer publish = commentService.countCommentByPass(CommentStatusEnum.PUBLISHED.getCode());
Integer draft = commentService.countCommentByPass(CommentStatusEnum.CHECKING.getCode());
Integer trash = commentService.countCommentByPass(CommentStatusEnum.RECYCLE.getCode());
return Msg.success().add("publish", publish).add("draft", draft).add("trash", trash);
}
/**
* 后台评论列表显示
*
* @return modelAndView
*/
@RequestMapping(value = "/comments", method = RequestMethod.GET)
public String index(@RequestParam(required = false, defaultValue = "1") Integer pageIndex,
@RequestParam(required = false, defaultValue = "15") Integer pageSize,
@RequestParam(required = false, defaultValue = "1") Integer pass,
HttpSession session, Model model) {
// 三种文章类型个数
Integer publish = commentService.countCommentByPass(CommentStatusEnum.PUBLISHED.getCode());
Integer draft = commentService.countCommentByPass(CommentStatusEnum.CHECKING.getCode());
Integer trash = commentService.countCommentByPass(CommentStatusEnum.RECYCLE.getCode());
HashMap<String, Object> criteria = new HashMap<>(1);
criteria.put("pass", pass);
PageInfo<Comment> commentPageInfo = commentService.pageComment(pageIndex, pageSize, criteria);
model.addAttribute("pageInfo", commentPageInfo);
model.addAttribute("publish", publish);
model.addAttribute("draft", draft);
model.addAttribute("trash", trash);
model.addAttribute("pass", pass);
String msg = (String) session.getAttribute("msg");
if (msg != null && !msg.equals("")) {
model.addAttribute("msg", msg);
session.removeAttribute("msg");
}
return "admin/comment/comments";
}
/**
* 分页条件查询
*
* @param pageIndex 页数
* @param pageSize
* @param commentQuery
* @param model
* @return
*/
@RequestMapping("/comments/search")
public String search(@RequestParam(required = false, defaultValue = "1") Integer pageIndex,
@RequestParam(required = false, defaultValue = "15") Integer pageSize, CommentQuery commentQuery, Model model) {
HashMap<String, Object> criteria = new HashMap<>();
String keywords = commentQuery.getKeywords();
Integer pass = commentQuery.getPass();
String sort = commentQuery.getSort();
String order = commentQuery.getOrder();
String searchType = commentQuery.getSearchType();
if (!StringUtils.isBlank(keywords)) {
if (COMMENT_CONTENT.equals(searchType)) {
criteria.put("content", keywords);
} else if (COMMENT_AUTHOR.equals(searchType)) {
criteria.put("nickname", keywords);
} else if (COMMENT_EMAIL.equals(searchType)) {
criteria.put("email", keywords);
} else if (COMMENT_AUTHOR_IP.equals(searchType)) {
criteria.put("ip", keywords);
}
}
if (pass != null) {
criteria.put("pass", pass);
} else {
criteria.put("pass", 1);
}
if (sort != null && !StringUtils.isBlank(sort)) criteria.put("sort", sort);
if (order != null && !StringUtils.isBlank(order)) criteria.put("order", order);
PageInfo<Comment> commentPageInfo = commentService.pageComment(pageIndex, pageSize, criteria);
model.addAttribute("pageInfo", commentPageInfo);
return "admin/comment/comments :: commentList";
}
/**
* 将评论改变为发布状态
* 评论状态有两种:待审核1,回收站2
* 对待审核转发布的,发邮件
*
* @param commentId 评论编号
* @return 重定向到/admin/comment
*/
@ResponseBody
@RequestMapping(value = "/comments/revert", method = RequestMethod.PUT)
@SystemLog(description = "回滚评论", type = LogTypeEnum.OPERATION)
public Msg moveToPublish(@RequestParam("id") Integer commentId, HttpSession session) {
User loginUser = (User) session.getAttribute("user");
//评论
Comment comment = commentService.getById(null,null,null,commentId);
Blog blog = blogService.getBlogByPublishedAndId(null, null,comment.getBlogId());
Comment result = commentService.updateCommentPass(commentId, CommentStatusEnum.PUBLISHED.getCode());
// 判断是不是子评论,如果是,也对被回复人发邮件
if(result.getParentCommentId() != null && result.getParentCommentId() > 0){
//被回复的评论
Comment lastComment = commentService.getById(null,null, null,comment.getParentCommentId());
//邮件通知
new EmailToAuthor(result, lastComment, blog).start();
}
//判断是否启用邮件服务
new NoticeToAuthor(result, blog, result.getPass()).start();
return Msg.success().add("msg", "评论已发布!");
}
/**
* 删除评论
*
* @param commentId commentId
* @return string 重定向到/admin/comment
*/
@RequestMapping(value = "/comments/delete", method = RequestMethod.DELETE)
@ResponseBody
@SystemLog(description = "删除评论", type = LogTypeEnum.OPERATION)
public Msg moveToAway(@RequestParam("id") Integer commentId) {
//评论
Comment comment = commentService.getById(null,null, null, commentId);
if (Objects.equals(comment.getPass(), CommentStatusEnum.RECYCLE.getCode())) {
commentService.deleteComment(commentId);
return Msg.success().add("msg", "评论已彻底删除");
} else {
commentService.updateCommentPass(commentId, CommentStatusEnum.RECYCLE.getCode());
return Msg.success().add("msg", "评论仍入回收站");
}
}
/**
* 管理员回复评论,并通过评论
*
* @param commentId 被回复的评论
* @param commentContent 回复的内容
没有合适的资源?快使用搜索试试~ 我知道了~
基于SpringMVC+Spring+MyBatis开发的个人博客网站,使用IDEA工具开发,毕业设计.zip
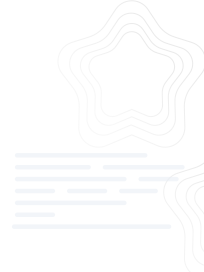
共1534个文件
js:537个
html:298个
css:184个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 30 浏览量
2023-08-04
12:31:10
上传
评论
收藏 17.16MB ZIP 举报
温馨提示
基于SpringMVC+Spring+MyBatis开发的个人博客网站,使用IDEA工具开发,毕业设计.zip
资源推荐
资源详情
资源评论
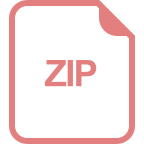
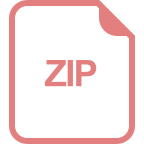
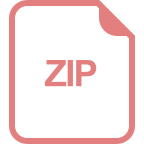
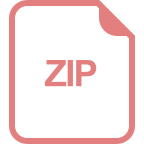
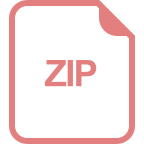
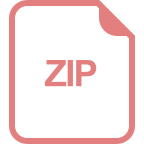
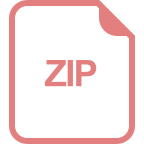
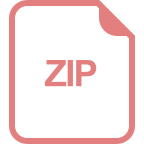
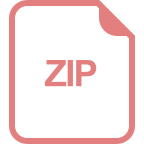
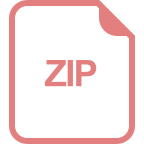
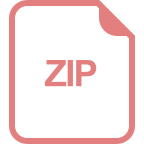
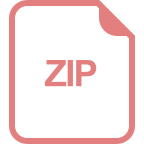
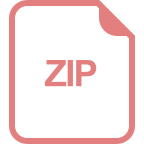
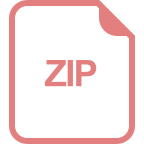
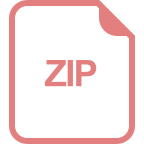
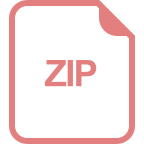
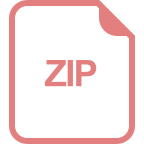
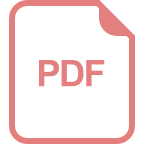
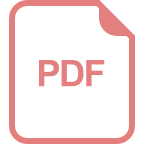
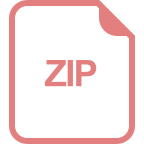
收起资源包目录

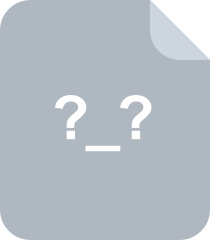
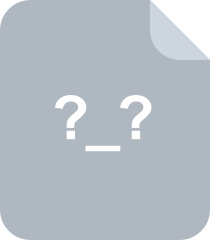
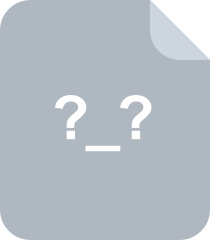
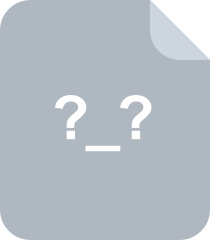
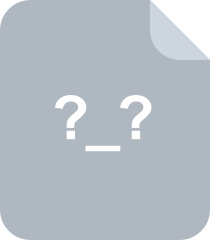
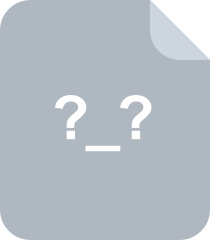
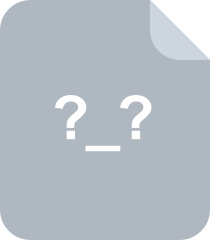
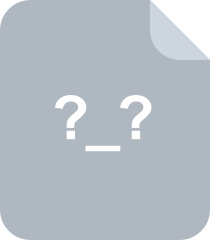
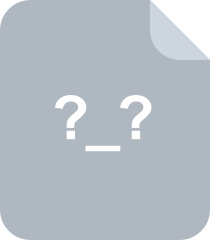
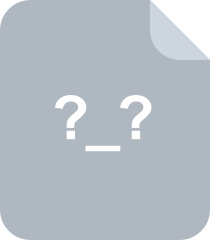
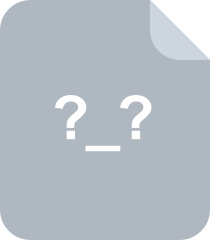
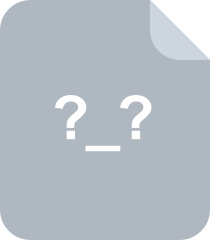
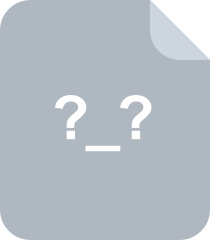
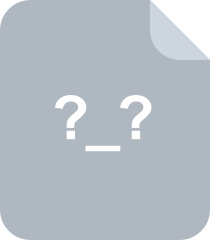
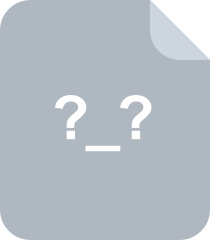
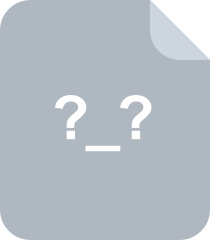
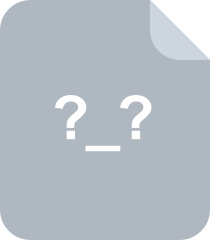
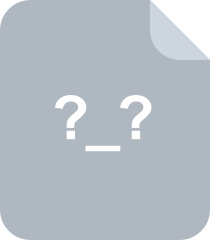
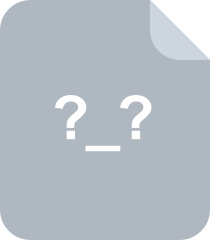
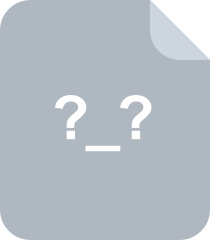
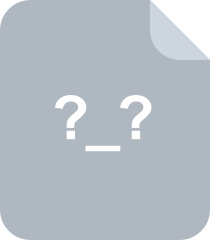
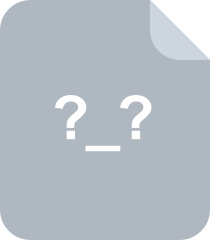
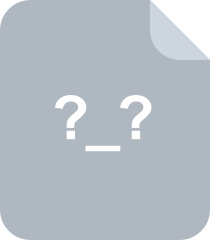
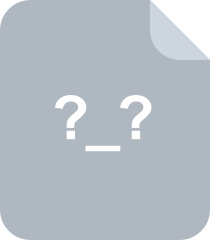
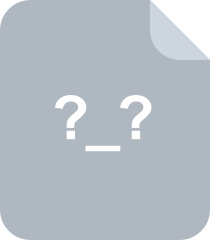
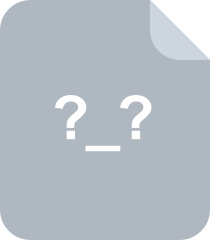
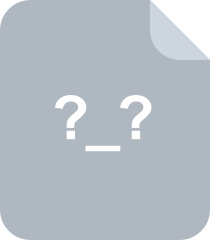
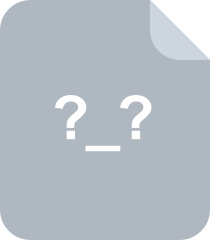
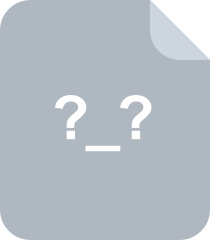
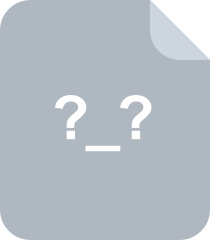
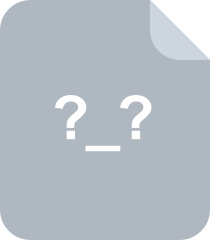
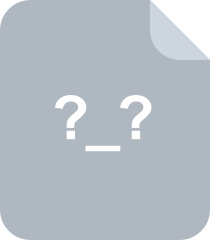
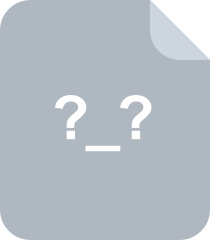
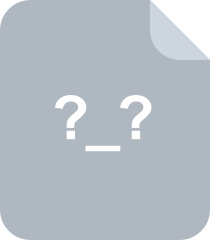
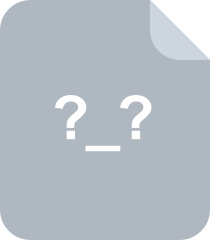
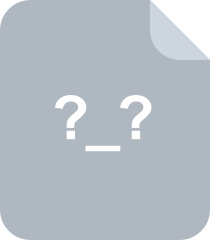
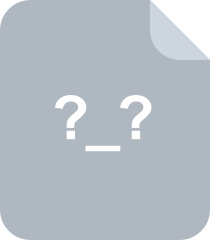
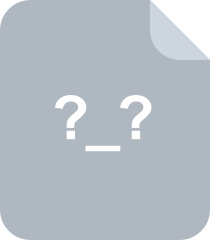
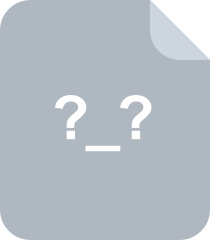
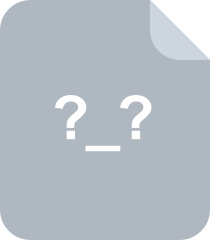
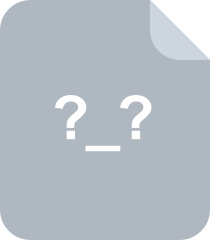
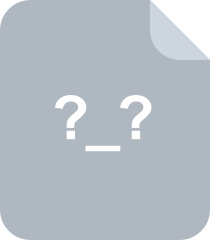
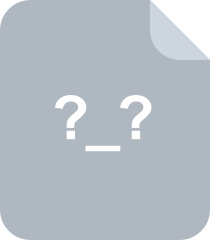
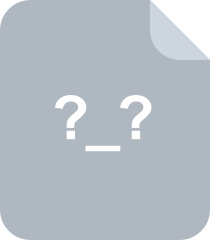
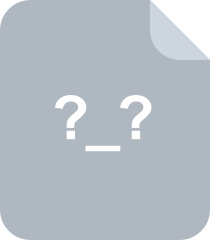
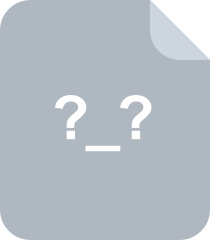
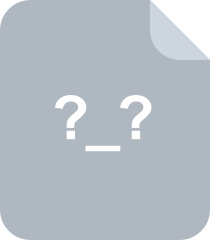
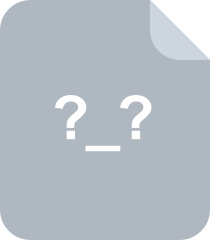
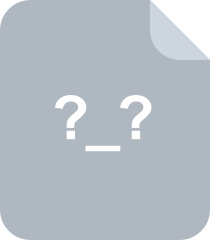
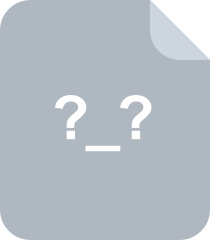
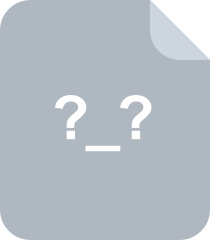
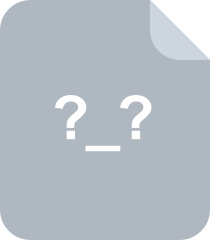
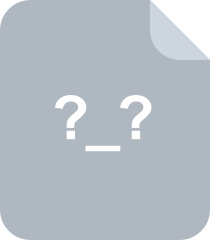
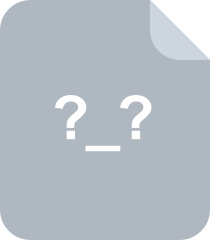
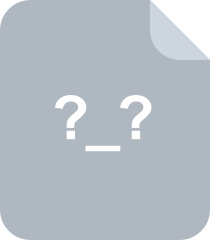
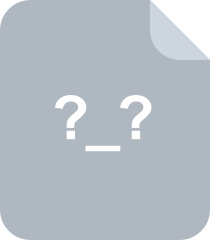
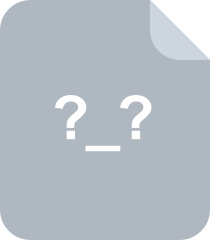
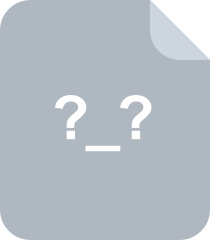
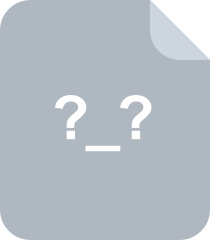
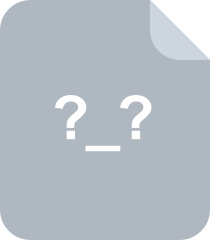
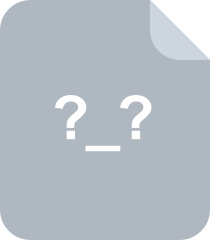
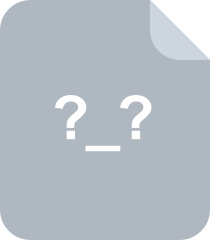
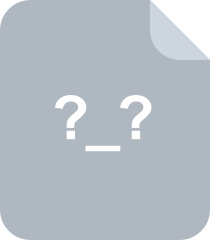
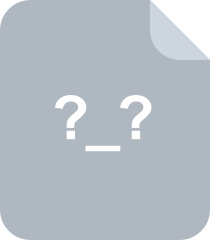
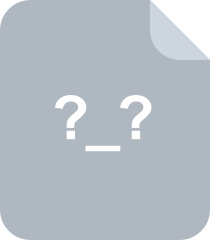
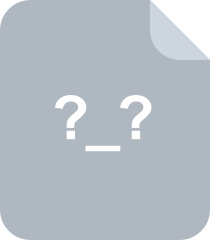
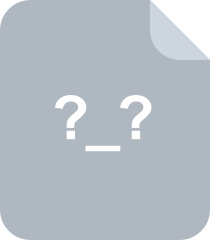
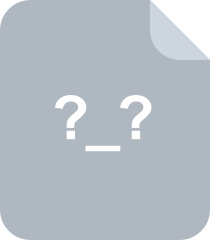
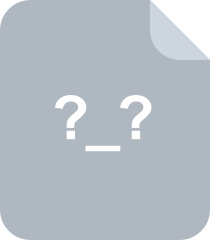
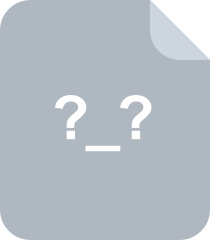
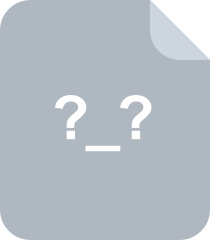
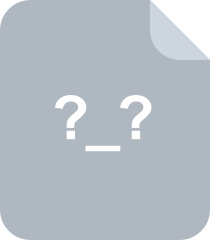
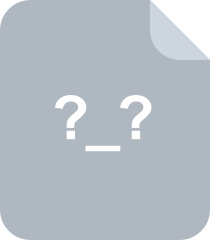
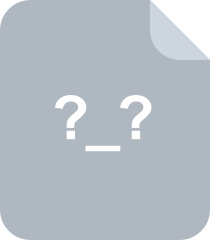
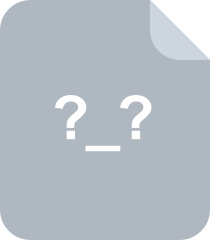
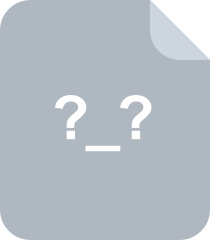
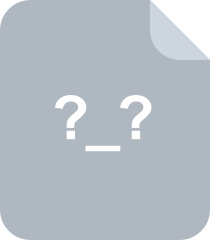
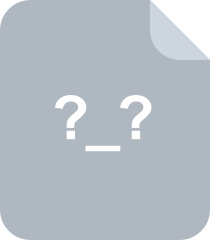
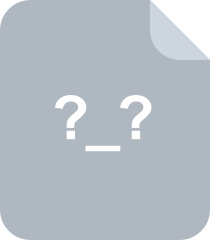
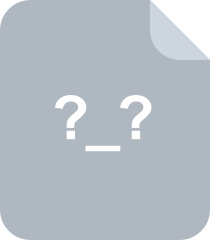
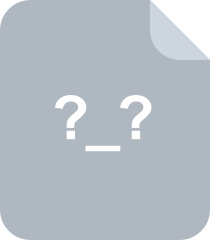
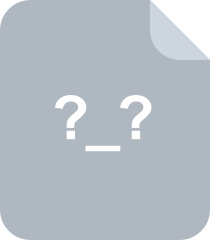
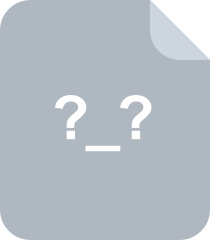
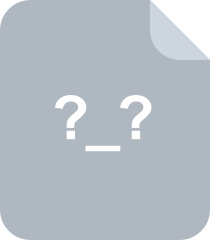
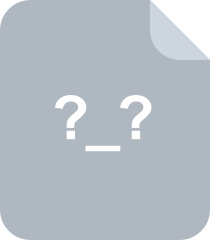
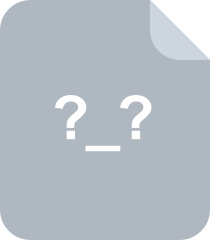
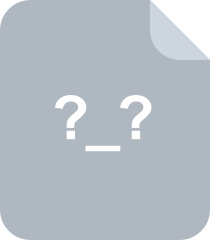
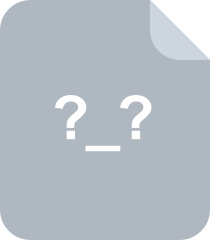
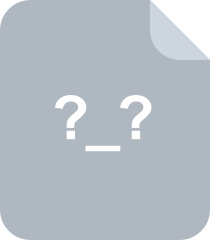
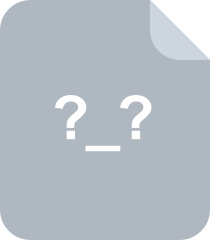
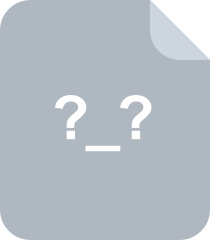
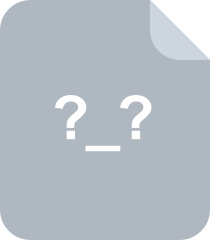
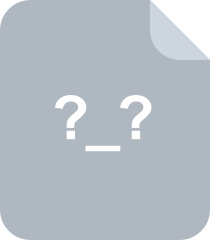
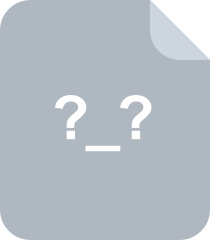
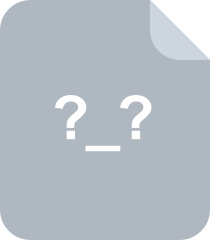
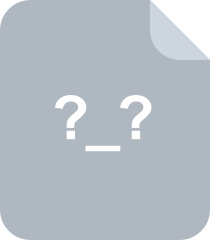
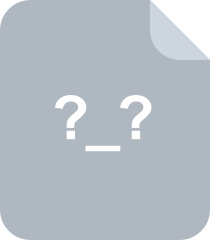
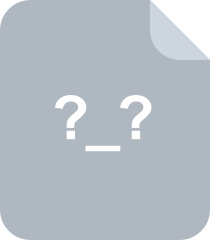
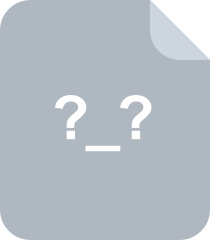
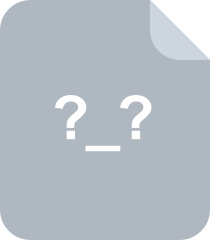
共 1534 条
- 1
- 2
- 3
- 4
- 5
- 6
- 16
资源评论
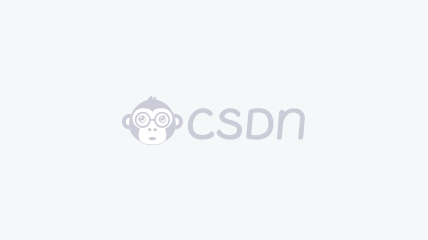

AbelZ_01
- 粉丝: 894
- 资源: 5441
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

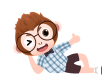
安全验证
文档复制为VIP权益,开通VIP直接复制
