package com.yiidian.ssm.blog.service.impl;
import com.github.pagehelper.PageHelper;
import com.github.pagehelper.PageInfo;
import com.yiidian.ssm.blog.enums.ArticleCommentStatus;
import com.yiidian.ssm.blog.service.ArticleService;
import com.yiidian.ssm.blog.entity.*;
import com.yiidian.ssm.blog.mapper.ArticleCategoryRefMapper;
import com.yiidian.ssm.blog.mapper.ArticleMapper;
import com.yiidian.ssm.blog.mapper.ArticleTagRefMapper;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import java.util.ArrayList;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
/**
* 文章Servie实现
*
* @author 言曌
* @date 2017/8/24
*/
@Service
@Slf4j
public class ArticleServiceImpl implements ArticleService {
@Autowired(required = false)
private ArticleMapper articleMapper;
@Autowired(required = false)
private ArticleCategoryRefMapper articleCategoryRefMapper;
@Autowired(required = false)
private ArticleTagRefMapper articleTagRefMapper;
@Override
public Integer countArticle(Integer status) {
Integer count = 0;
try {
count = articleMapper.countArticle(status);
} catch (Exception e) {
e.printStackTrace();
log.error("根据状态统计文章数, status:{}, cause:{}", status, e);
}
return count;
}
@Override
public Integer countArticleComment() {
Integer count = 0;
try {
count = articleMapper.countArticleComment();
} catch (Exception e) {
e.printStackTrace();
log.error("统计文章评论数失败, cause:{}", e);
}
return count;
}
@Override
public Integer countArticleView() {
Integer count = 0;
try {
count = articleMapper.countArticleView();
} catch (Exception e) {
e.printStackTrace();
log.error("统计文章访问量失败, cause:{}", e);
}
return count;
}
@Override
public Integer countArticleByCategoryId(Integer categoryId) {
Integer count = 0;
try {
count = articleCategoryRefMapper.countArticleByCategoryId(categoryId);
} catch (Exception e) {
e.printStackTrace();
log.error("根据分类统计文章数量失败, categoryId:{}, cause:{}", categoryId, e);
}
return count;
}
@Override
public Integer countArticleByTagId(Integer tagId) {
return articleTagRefMapper.countArticleByTagId(tagId);
}
@Override
public List<Article> listArticle(HashMap<String, Object> criteria) {
return articleMapper.findAll(criteria);
}
@Override
public List<Article> listRecentArticle(Integer limit) {
return articleMapper.listArticleByLimit(limit);
}
@Override
@Transactional(rollbackFor = Exception.class)
public void updateArticleDetail(Article article) {
article.setArticleUpdateTime(new Date());
articleMapper.update(article);
if (article.getTagList() != null) {
//删除标签和文章关联
articleTagRefMapper.deleteByArticleId(article.getArticleId());
//添加标签和文章关联
for (int i = 0; i < article.getTagList().size(); i++) {
ArticleTagRef articleTagRef = new ArticleTagRef(article.getArticleId(), article.getTagList().get(i).getTagId());
articleTagRefMapper.insert(articleTagRef);
}
}
if (article.getCategoryList() != null) {
//添加分类和文章关联
articleCategoryRefMapper.deleteByArticleId(article.getArticleId());
//删除分类和文章关联
for (int i = 0; i < article.getCategoryList().size(); i++) {
ArticleCategoryRef articleCategoryRef = new ArticleCategoryRef(article.getArticleId(), article.getCategoryList().get(i).getCategoryId());
articleCategoryRefMapper.insert(articleCategoryRef);
}
}
}
@Override
public void updateArticle(Article article) {
articleMapper.update(article);
}
@Override
public void deleteArticleBatch(List<Integer> ids) {
articleMapper.deleteBatch(ids);
}
@Override
public void deleteArticle(Integer id) {
articleMapper.deleteById(id);
}
@Override
public PageInfo<Article> pageArticle(Integer pageIndex,
Integer pageSize,
HashMap<String, Object> criteria) {
PageHelper.startPage(pageIndex, pageSize);
List<Article> articleList = articleMapper.findAll(criteria);
for (int i = 0; i < articleList.size(); i++) {
//封装CategoryList
List<Category> categoryList = articleCategoryRefMapper.listCategoryByArticleId(articleList.get(i).getArticleId());
if (categoryList == null || categoryList.size() == 0) {
categoryList = new ArrayList<>();
categoryList.add(Category.Default());
}
articleList.get(i).setCategoryList(categoryList);
// //封装TagList
// List<Tag> tagList = articleTagRefMapper.listTagByArticleId(articleList.get(i).getArticleId());
// articleList.get(i).setTagList(tagList);
}
return new PageInfo<>(articleList);
}
@Override
public Article getArticleByStatusAndId(Integer status, Integer id) {
Article article = articleMapper.getArticleByStatusAndId(status, id);
if (article != null) {
List<Category> categoryList = articleCategoryRefMapper.listCategoryByArticleId(article.getArticleId());
List<Tag> tagList = articleTagRefMapper.listTagByArticleId(article.getArticleId());
article.setCategoryList(categoryList);
article.setTagList(tagList);
}
return article;
}
@Override
public List<Article> listArticleByViewCount(Integer limit) {
return articleMapper.listArticleByViewCount(limit);
}
@Override
public Article getAfterArticle(Integer id) {
return articleMapper.getAfterArticle(id);
}
@Override
public Article getPreArticle(Integer id) {
return articleMapper.getPreArticle(id);
}
@Override
public List<Article> listRandomArticle(Integer limit) {
return articleMapper.listRandomArticle(limit);
}
@Override
public List<Article> listArticleByCommentCount(Integer limit) {
return articleMapper.listArticleByCommentCount(limit);
}
@Override
@Transactional(rollbackFor = Exception.class)
public void insertArticle(Article article) {
//添加文章
article.setArticleCreateTime(new Date());
article.setArticleUpdateTime(new Date());
article.setArticleIsComment(ArticleCommentStatus.ALLOW.getValue());
article.setArticleViewCount(0);
article.setArticleLikeCount(0);
article.setArticleCommentCount(0);
article.setArticleOrder(1);
articleMapper.insert(article);
//添加分类和文章关联
for (int i = 0; i < article.getCategoryList().size(); i++) {
ArticleCategoryRef articleCategoryRef = new ArticleCategoryRef(article.getArticleId(), article.getCategoryList().get(i).getCategoryId());
articleCategoryRefMapper.insert(articleCategoryRef);
}
//添加标签和文章关联
for (int i = 0; i < article.getTagList().size(); i++) {
ArticleTagRef articleTagRef = new ArticleTagRef(article.getArticleId(), article.getTagList().get(i).getTagId());
articleTagRefMapper.insert(articleTagRef);
}
}
@Override
public void updateCommentCount(Integ
没有合适的资源?快使用搜索试试~ 我知道了~
基于SpringMVC+Spring+MyBatis个人技术博客系统源码.zip
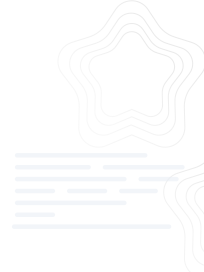
共929个文件
class:160个
gif:156个
xml:128个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 90 浏览量
2022-04-24
19:37:38
上传
评论 3
收藏 37.15MB ZIP 举报
温馨提示
基于SpringMVC+Spring+MyBatis个人技术博客系统源码.zip 完整代码,可运行 项目描述 基于SSM实现的一个个人博客系统,适合初学SSM和个人博客制作的同学学习。有了这个源码,直接买了阿里云或腾讯服务器,就可以部署独立个人技术博客啦。博客源码主要涉及技术包括的包括 Maven、Spring、SpringMVC、MyBatis、Redis、JSP等。后台地址:/admin 或者 /login 技术栈 SpringMVC + Spring + MyBatis 运行环境 IDEA + Tomcat8以上 + MySQL5.5以上 注意事项 「1. 开发工具的选择」 请使用 IntelliJ IDEA, 尽量不要用 Eclipse/MyEclipse 「2. 确保你安装了 Maven」 没有用过 Maven 的童鞋,感觉去学一下,安装一下,可以使用 3.3.9 或 3.5.0的版本 「3. 请安装 Lombok 插件」 代码中多次使用 @Data 注解,请确保你的 IDE
资源推荐
资源详情
资源评论
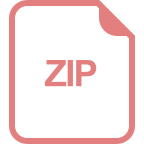
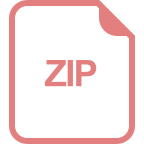
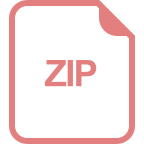
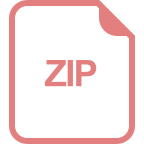
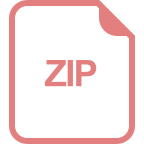
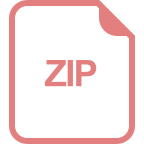
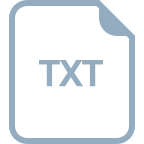
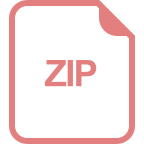
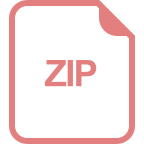
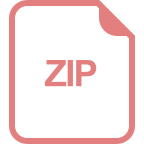
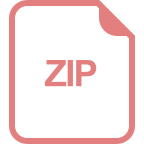
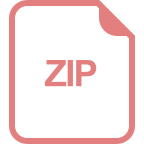
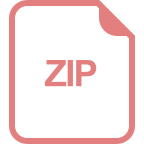
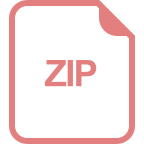
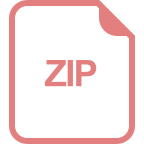
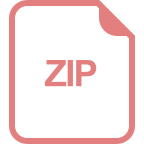
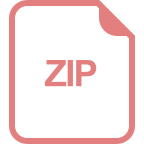
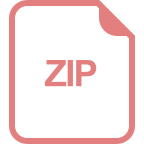
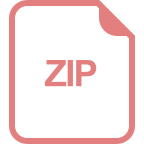
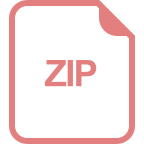
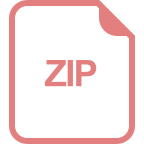
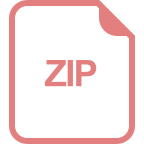
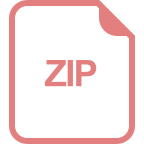
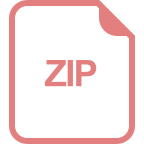
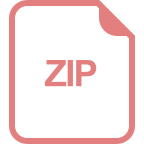
收起资源包目录

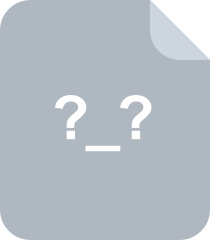
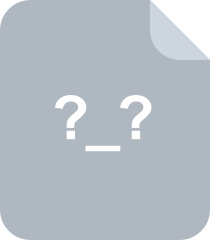
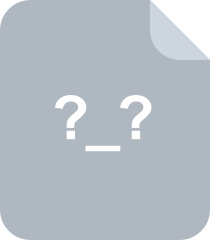
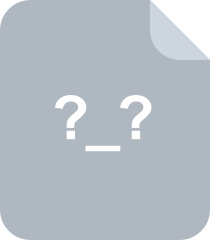
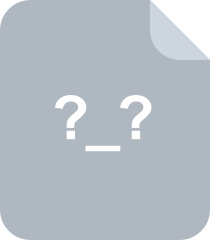
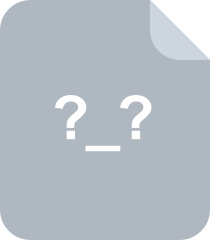
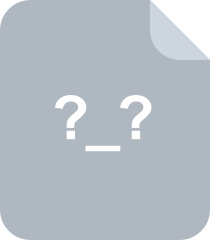
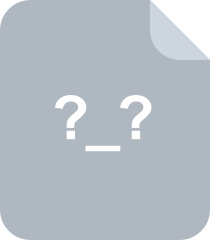
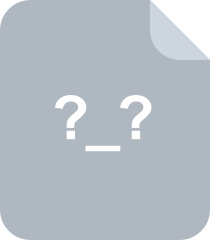
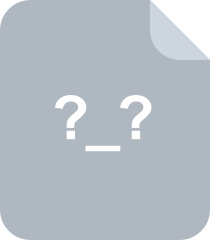
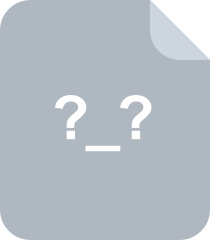
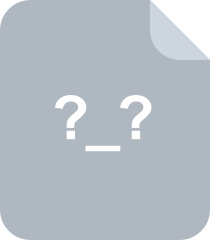
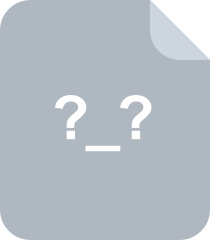
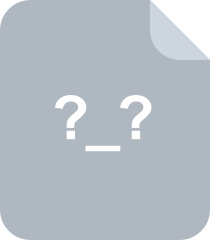
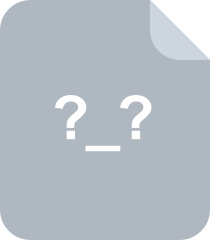
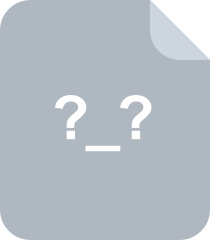
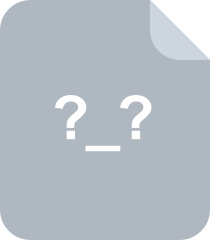
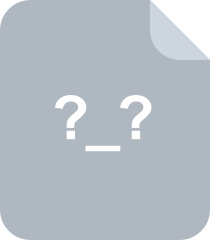
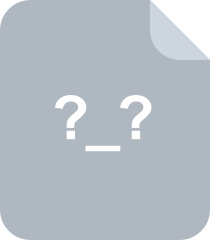
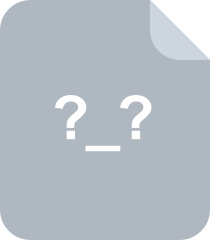
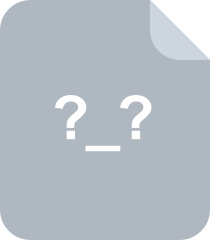
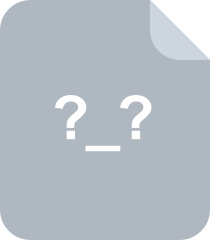
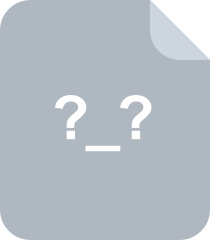
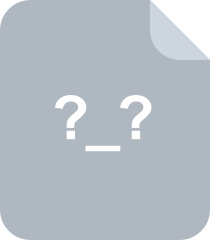
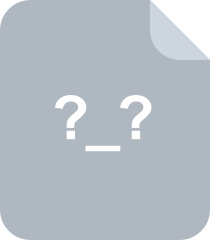
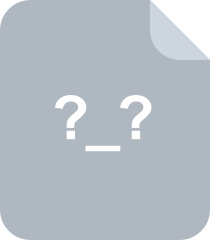
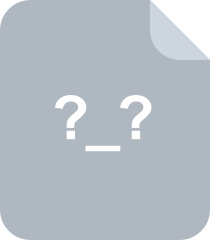
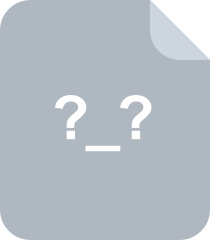
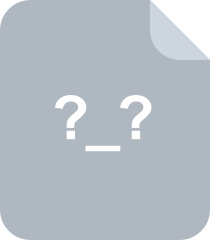
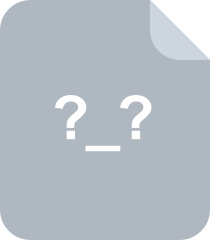
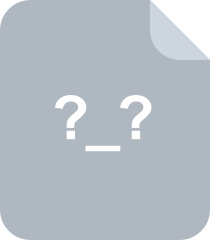
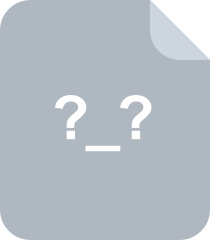
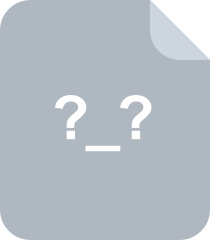
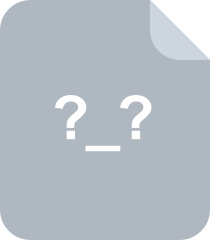
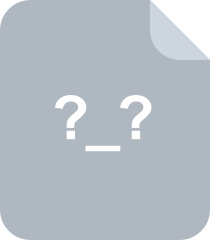
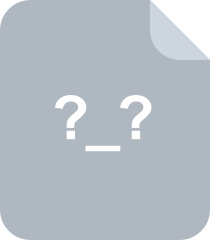
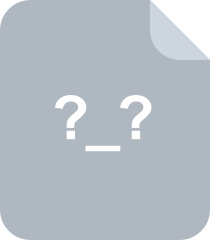
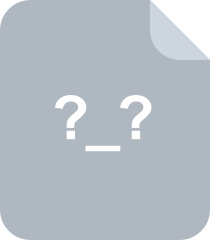
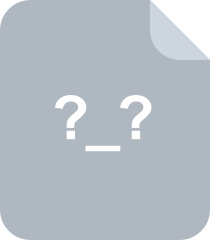
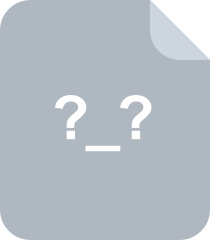
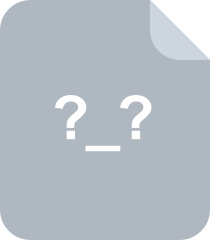
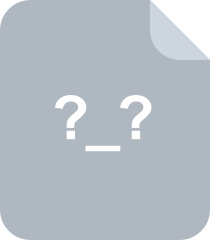
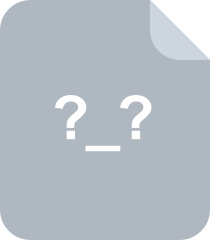
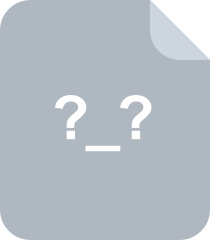
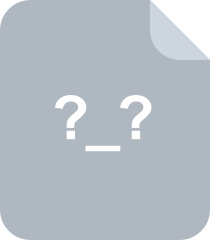
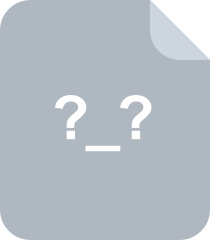
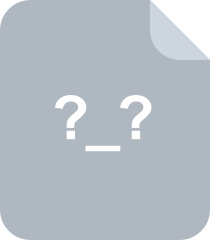
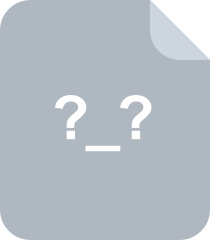
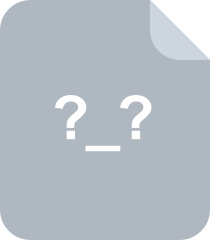
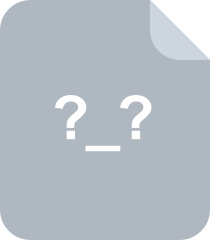
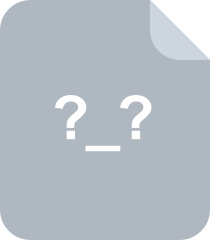
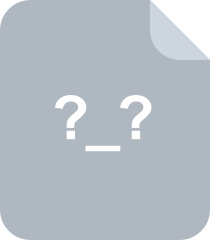
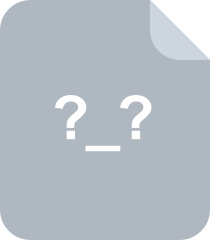
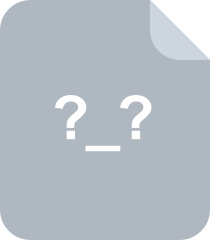
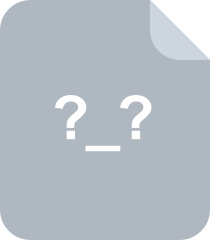
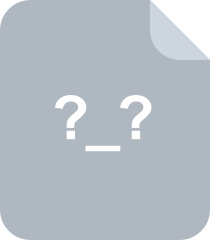
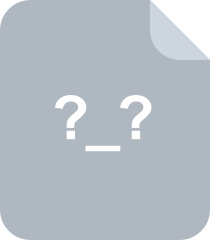
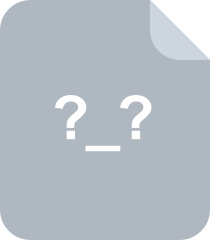
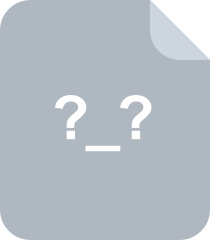
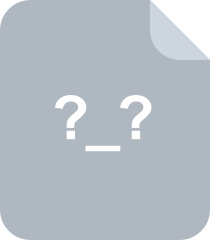
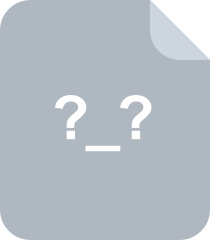
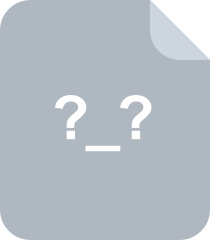
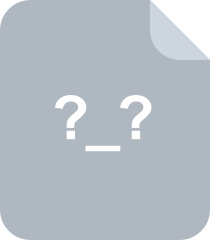
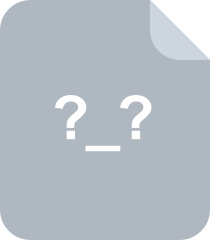
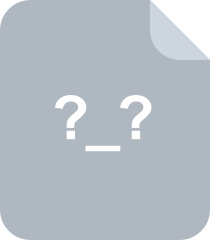
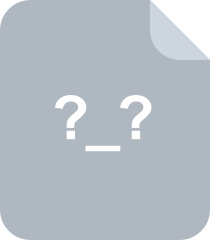
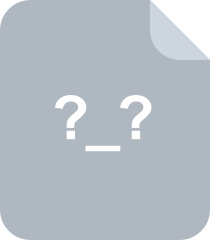
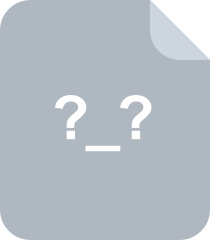
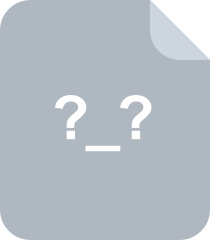
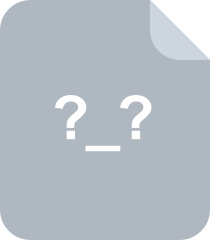
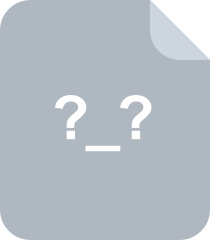
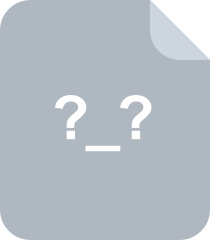
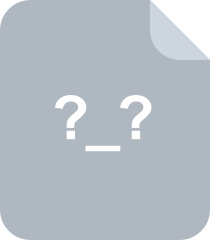
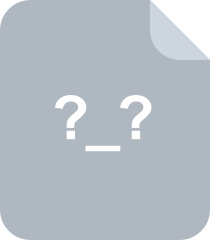
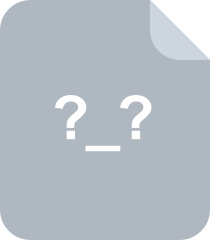
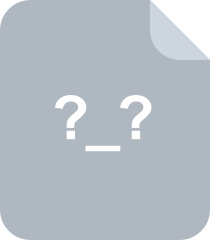
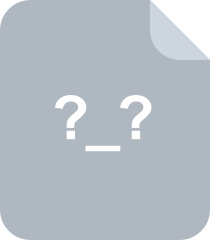
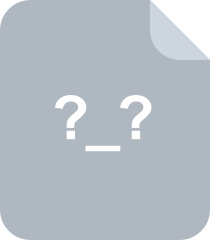
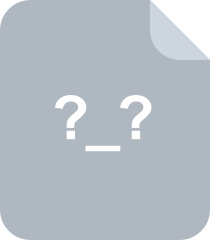
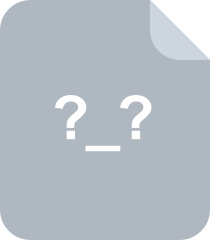
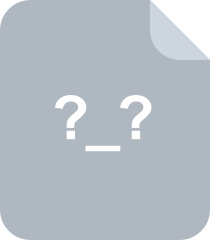
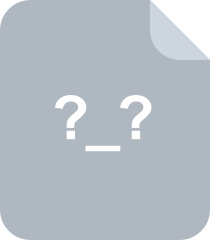
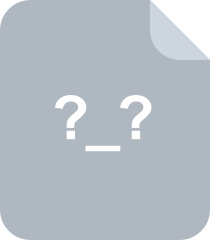
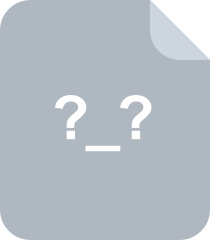
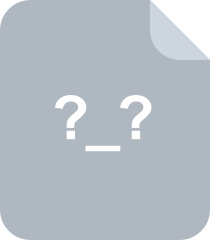
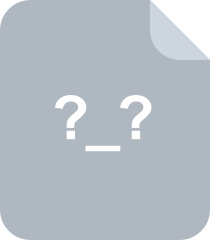
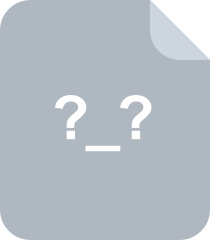
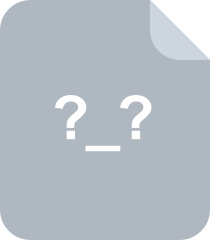
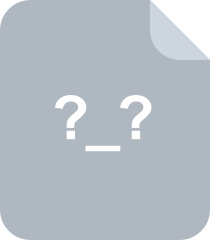
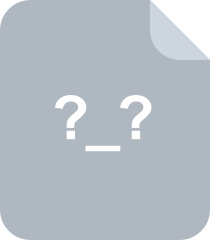
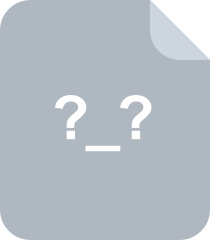
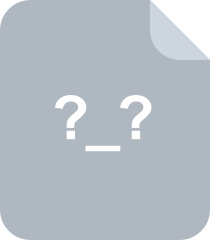
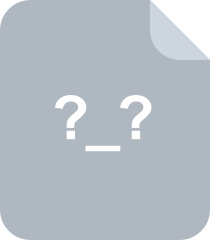
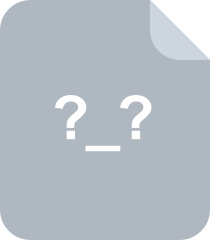
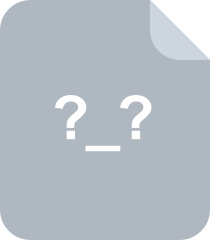
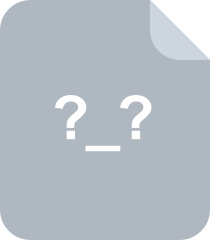
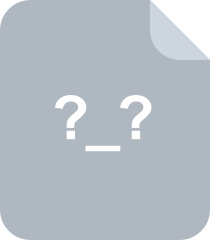
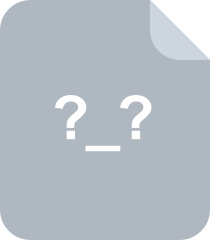
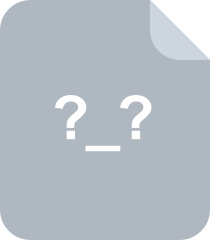
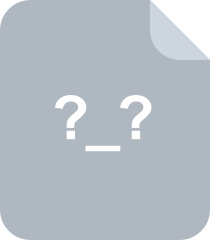
共 929 条
- 1
- 2
- 3
- 4
- 5
- 6
- 10
资源评论
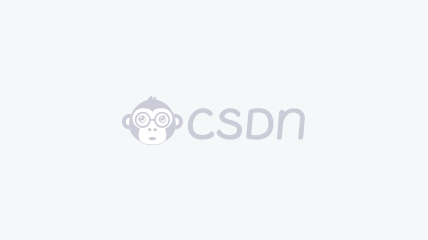

智慧安全方案
- 粉丝: 3814
- 资源: 59万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

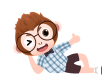
最新资源
- HengCe-18900-2024-2030全球与中国eMMC和UFS市场现状及未来发展趋势-样本.docx
- 2024第十四届APMCM亚太地区-C题完整论文.pdf
- HengCe-18900-2024-2030中国硬碳负极材料市场现状研究分析与发展前景预测报告-样本.docx
- PHP面向对象与设计模式
- HengCe-2024-2030全球与中国掩模基板市场现状及未来发展趋势-样本
- CSS3制作的聚光灯下倒影文字选装动画特效代码.zip
- mongodb笔记和资料
- 工具变量2022-2004年中国省级市场分割指数数据.xlsx
- stm32f1 编写MPU6050程序代码
- js+jquery实现经典推箱子游戏
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


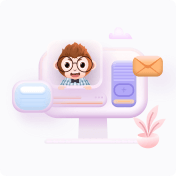
安全验证
文档复制为VIP权益,开通VIP直接复制
