<div align='center'>
<h1>TypeBox</h1>
<p>JSON Schema Type Builder with Static Type Resolution for TypeScript</p>
<img src="https://github.com/sinclairzx81/typebox/blob/master/typebox.png?raw=true" />
<br />
<br />
[](https://badge.fury.io/js/%40sinclair%2Ftypebox)
[](https://www.npmjs.com/package/%40sinclair%2Ftypebox)
[](https://github.com/sinclairzx81/typebox/actions)
</div>
<a name="Install"></a>
## Install
#### Npm
```bash
$ npm install @sinclair/typebox --save
```
#### Deno
```typescript
import { Static, Type } from 'npm:@sinclair/typebox'
```
#### Esm
```typescript
import { Static, Type } from 'https://esm.sh/@sinclair/typebox'
```
## Example
```typescript
import { Static, Type } from '@sinclair/typebox'
const T = Type.Object({ // const T = {
x: Type.Number(), // type: 'object',
y: Type.Number(), // required: ['x', 'y', 'z'],
z: Type.Number() // properties: {
}) // x: { type: 'number' },
// y: { type: 'number' },
// z: { type: 'number' }
// }
// }
type T = Static<typeof T> // type T = {
// x: number,
// y: number,
// z: number
// }
```
<a name="Overview"></a>
## Overview
TypeBox is a runtime type builder that creates in-memory JSON Schema objects that can be statically inferred as TypeScript types. The schemas produced by this library are designed to match the static type assertion rules of the TypeScript compiler. TypeBox enables one to create a unified type that can be statically checked by TypeScript and runtime asserted using standard JSON Schema validation.
This library is designed to enable JSON schema to compose with the same flexibility as TypeScript's type system. It can be used as a simple tool to build up complex schemas or integrated into REST or RPC services to help validate data received over the wire.
License MIT
## Contents
- [Install](#install)
- [Overview](#overview)
- [Usage](#usage)
- [Types](#types)
- [Standard](#types-standard)
- [Extended](#types-extended)
- [Modifiers](#types-modifiers)
- [Options](#types-options)
- [Generics](#types-generics)
- [References](#types-references)
- [Recursive](#types-recursive)
- [Conditional](#types-conditional)
- [Template Literal](#types-template-literal)
- [Guards](#types-guards)
- [Unsafe](#types-unsafe)
- [Strict](#types-strict)
- [Values](#values)
- [Create](#values-create)
- [Clone](#values-clone)
- [Check](#values-check)
- [Convert](#values-convert)
- [Cast](#values-cast)
- [Equal](#values-equal)
- [Hash](#values-hash)
- [Diff](#values-diff)
- [Patch](#values-patch)
- [Errors](#values-errors)
- [Mutate](#values-mutate)
- [Pointer](#values-pointer)
- [TypeCheck](#typecheck)
- [Ajv](#typecheck-ajv)
- [TypeCompiler](#typecheck-typecompiler)
- [TypeSystem](#typesystem)
- [Types](#typesystem-types)
- [Formats](#typesystem-formats)
- [Policies](#typesystem-policies)
- [Benchmark](#benchmark)
- [Compile](#benchmark-compile)
- [Validate](#benchmark-validate)
- [Compression](#benchmark-compression)
- [Contribute](#contribute)
<a name="usage"></a>
## Usage
The following shows general usage.
```typescript
import { Static, Type } from '@sinclair/typebox'
//--------------------------------------------------------------------------------------------
//
// Let's say you have the following type ...
//
//--------------------------------------------------------------------------------------------
type T = {
id: string,
name: string,
timestamp: number
}
//--------------------------------------------------------------------------------------------
//
// ... you can express this type in the following way.
//
//--------------------------------------------------------------------------------------------
const T = Type.Object({ // const T = {
id: Type.String(), // type: 'object',
name: Type.String(), // properties: {
timestamp: Type.Integer() // id: {
}) // type: 'string'
// },
// name: {
// type: 'string'
// },
// timestamp: {
// type: 'integer'
// }
// },
// required: [
// 'id',
// 'name',
// 'timestamp'
// ]
// }
//--------------------------------------------------------------------------------------------
//
// ... then infer back to the original static type this way.
//
//--------------------------------------------------------------------------------------------
type T = Static<typeof T> // type T = {
// id: string,
// name: string,
// timestamp: number
// }
//--------------------------------------------------------------------------------------------
//
// ... then use the type both as JSON schema and as a TypeScript type.
//
//--------------------------------------------------------------------------------------------
import { Value } from '@sinclair/typebox/value'
function receive(value: T) { // ... as a Static Type
if(Value.Check(T, value)) { // ... as a JSON Schema
// ok...
}
}
```
<a name='types'></a>
## Types
TypeBox types are JSON schema fragments that can be composed into more complex types. Each fragment is structured such that a JSON schema compliant validator can runtime assert a value the same way TypeScript will statically assert a type. TypeBox provides a set of Standard types which are used create JSON schema compliant schematics as well as an Extended type set used to create schematics for constructs native to JavaScript.
<a name='types-standard'></a>
### Standard Types
The following table lists the Standard TypeBox types. These types are fully compatible with the JSON Schema Draft 6 specification.
```typescript
┌────────────────────────────────┬─────────
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
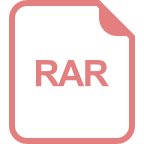
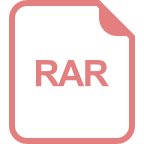
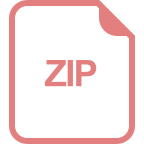
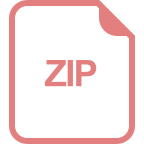
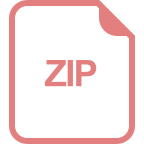
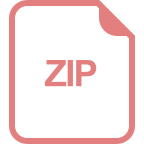
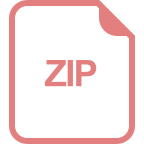
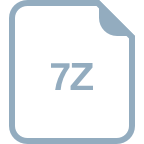
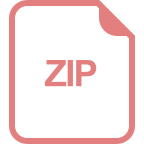
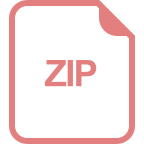
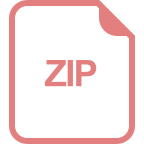
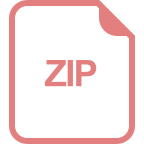
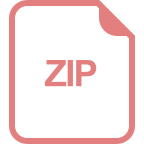
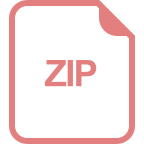
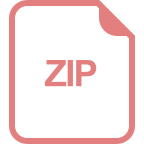
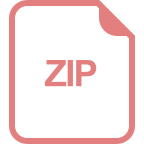
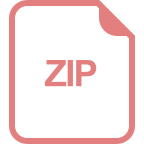
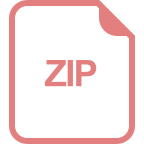
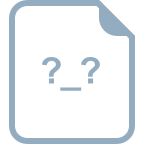
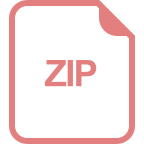
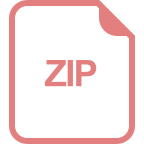
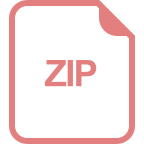
收起资源包目录

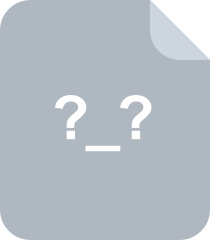
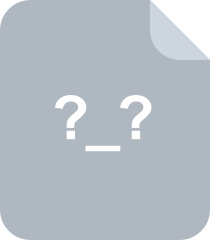
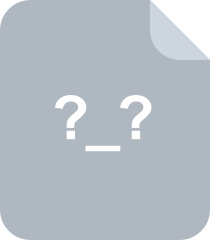
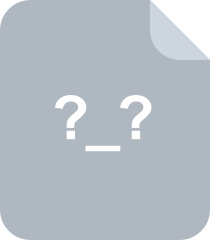
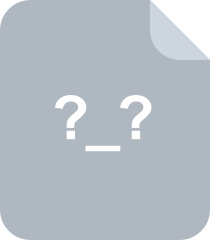
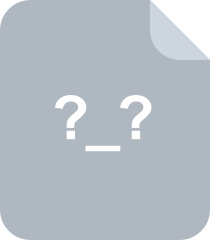
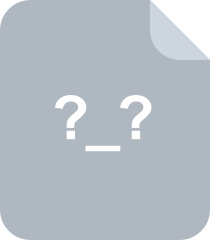
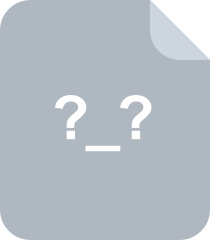
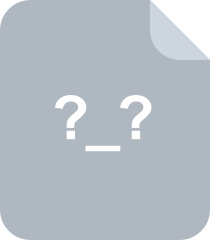
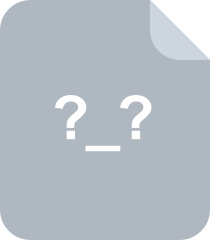
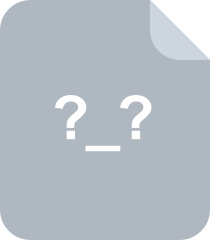
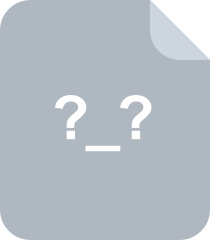
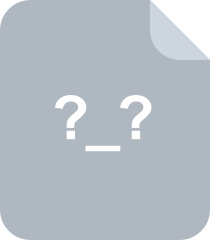
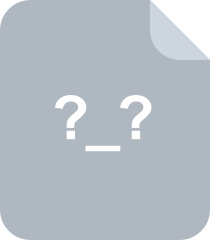
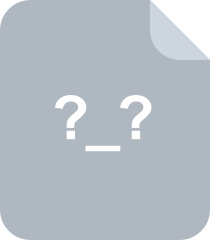
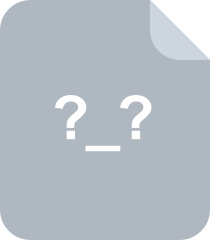
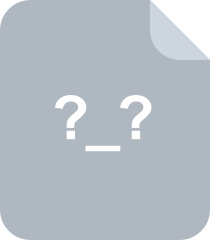
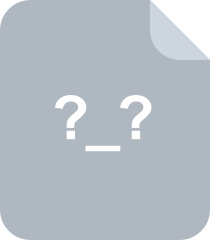
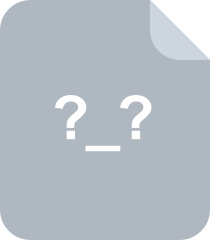
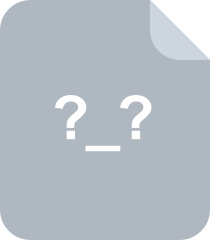
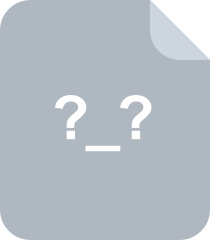
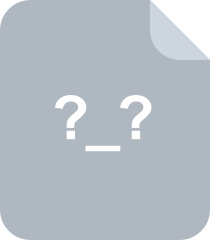
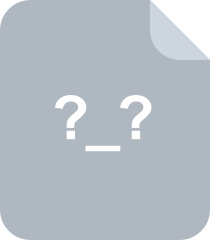
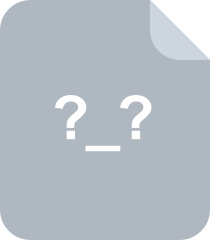
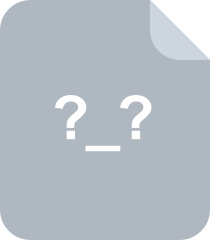
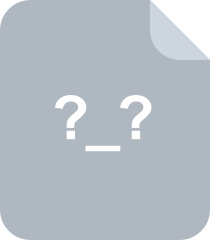
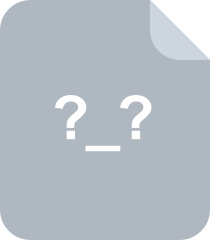
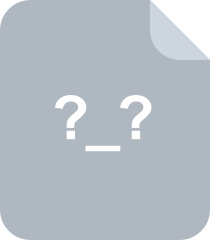
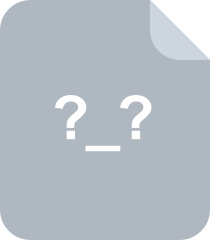
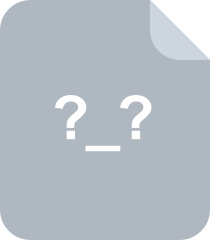
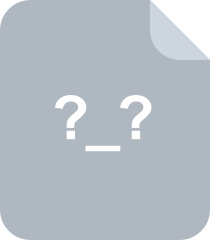
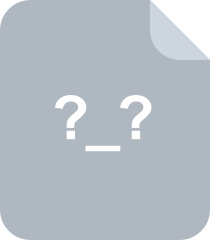
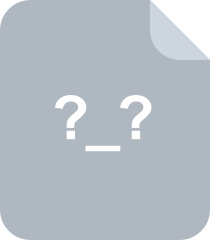
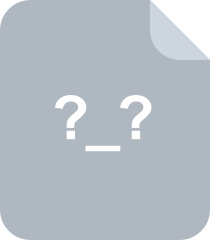
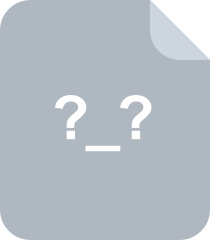
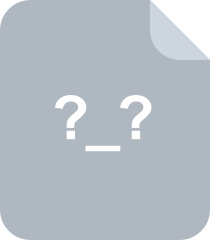
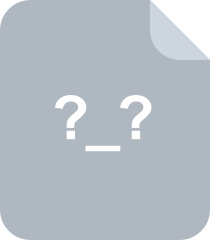
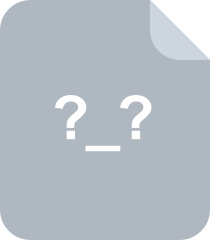
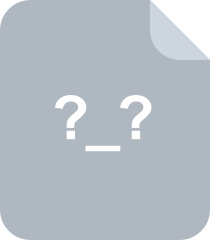
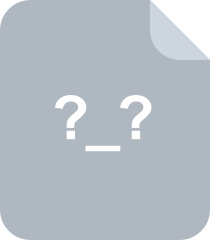
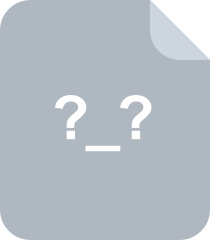
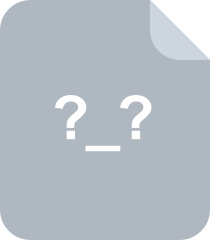
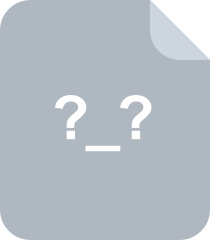
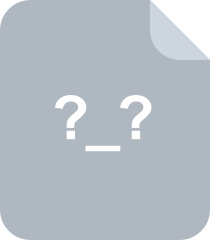
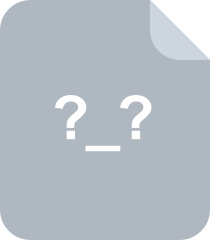
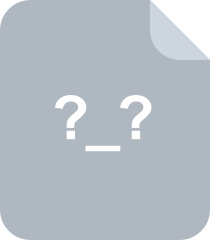
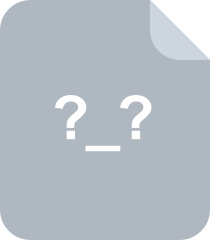
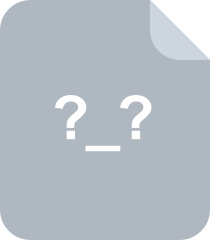
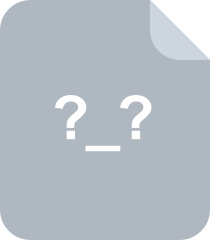
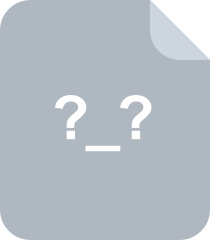
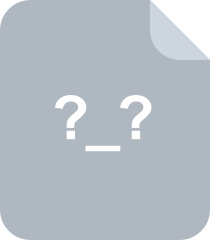
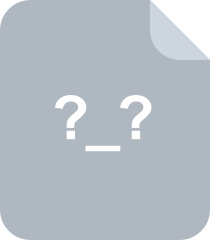
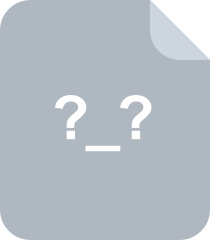
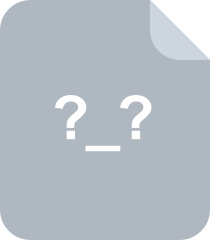
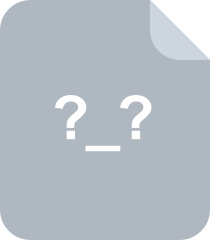
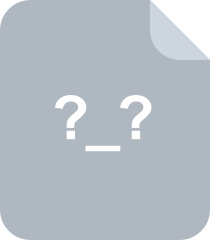
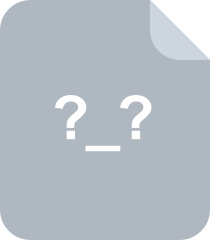
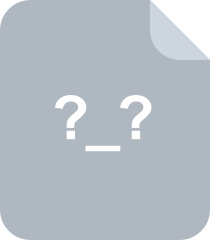
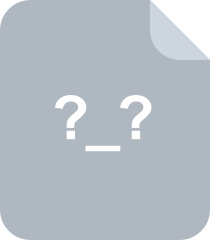
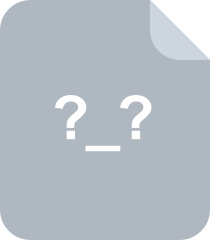
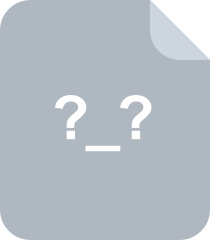
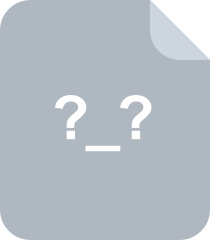
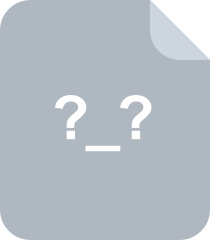
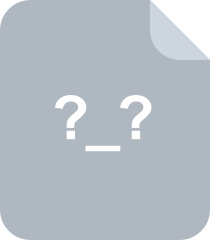
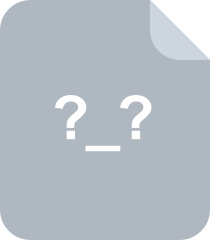
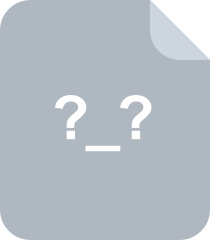
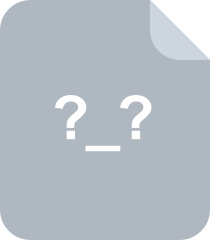
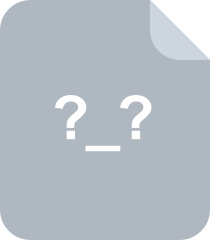
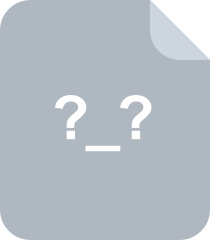
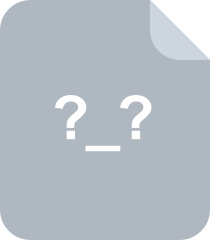
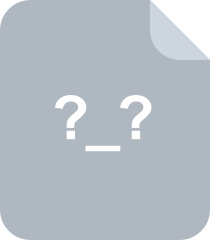
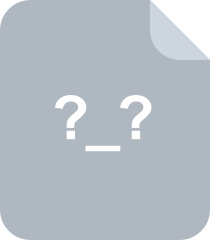
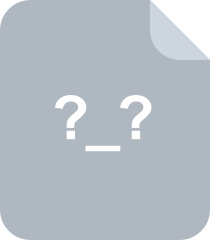
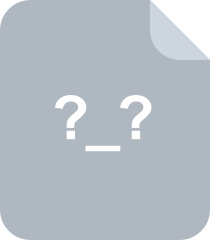
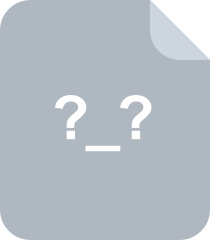
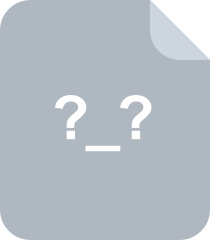
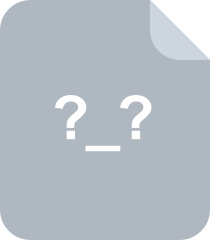
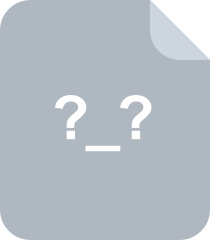
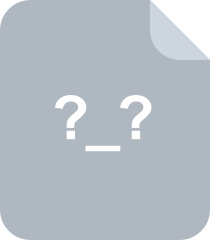
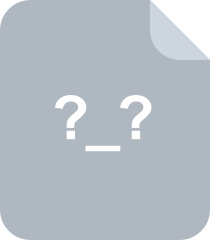
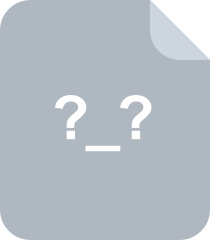
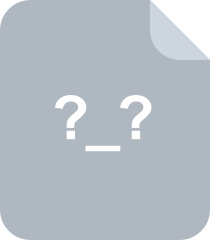
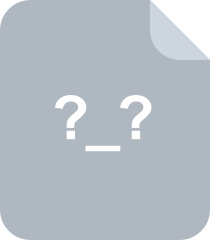
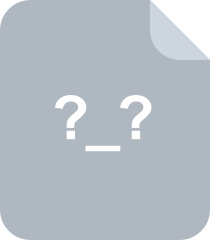
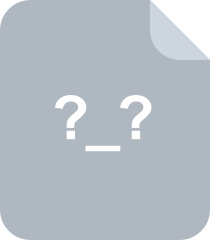
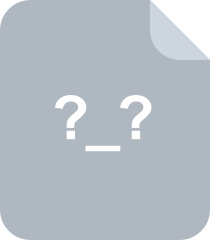
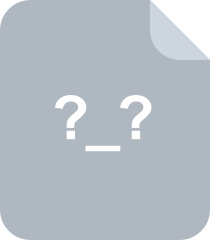
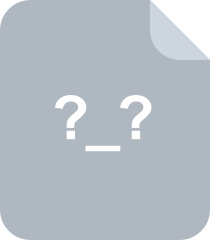
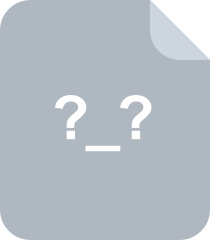
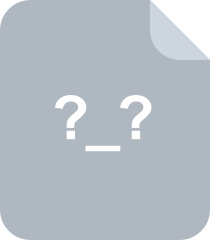
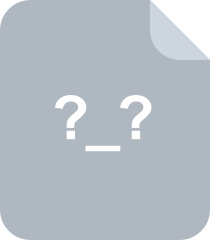
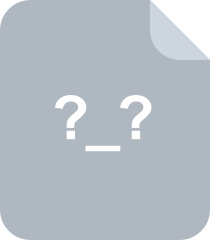
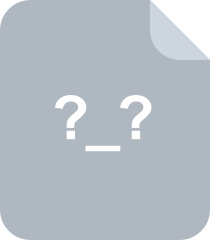
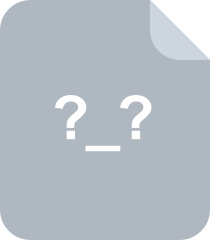
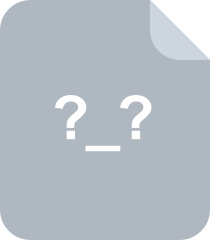
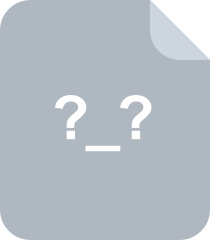
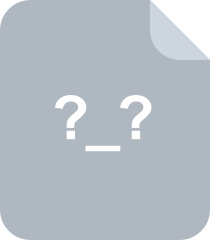
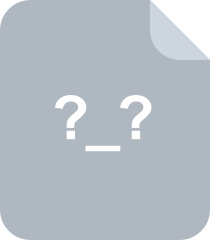
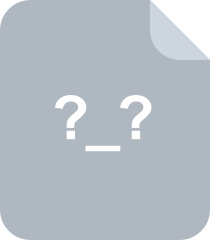
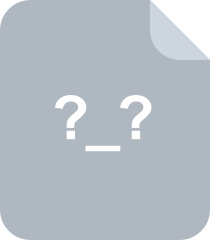
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
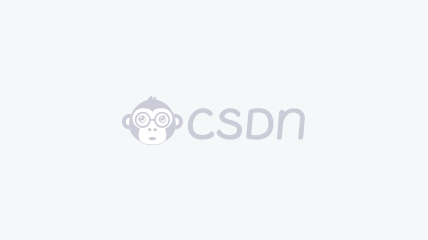

吾名招财
- 粉丝: 1813
- 资源: 36
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

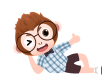
最新资源
- GoDS(Go 数据结构)-集合、列表、堆栈、映射、树、队列等等.zip
- C#asp.net电子会签系统源码带操作手册数据库 SQL2008源码类型 WebForm
- GoCD-持续交付服务器主存储库.zip
- 基于JavaScript+CSS的地图插件-文章中插入交互式地图(支持GoogleMap、高德地图、百度地图、Geoq地图和 OpenStreetMap).zip
- mt管理器base.apk
- 图书信息的收缩与展开.zip
- pIYBAFwi3cCAaDf7AAWvXOZOHlA452 (1).pdf
- 【java毕业设计】基于Java的菜匣子优选系统设计与实现源码(ssm+jsp+mysql+说明文档+LW).zip
- go-fastdfs 是一个简单的转型文件系统(树木云存储),具有无中心、高性能,高可靠,免维护等优点,支持断点续传,分块上传,小文件合并,自动同步,自动修复 Go-fastdfs是一个简单的.zip
- C++五子棋源码,一个简单的例子,可以学习一下
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


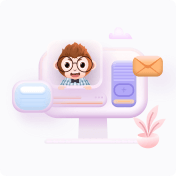
安全验证
文档复制为VIP权益,开通VIP直接复制
