
[OpenGL Mathematics](http://glm.g-truc.net/) (*GLM*) is a header only C++ mathematics library for graphics software based on the [OpenGL Shading Language (GLSL) specifications](https://www.opengl.org/registry/doc/GLSLangSpec.4.50.diff.pdf).
*GLM* provides classes and functions designed and implemented with the same naming conventions and functionality than *GLSL* so that anyone who knows *GLSL*, can use *GLM* as well in C++.
This project isn't limited to *GLSL* features. An extension system, based on the *GLSL* extension conventions, provides extended capabilities: matrix transformations, quaternions, data packing, random numbers, noise, etc...
This library works perfectly with *[OpenGL](https://www.opengl.org)* but it also ensures interoperability with other third party libraries and SDK. It is a good candidate for software rendering (raytracing / rasterisation), image processing, physics simulations and any development context that requires a simple and convenient mathematics library.
*GLM* is written in C++98 but can take advantage of C++11 when supported by the compiler. It is a platform independent library with no dependence and it officially supports the following compilers:
- [*GCC*](http://gcc.gnu.org/) 4.7 and higher
- [*Intel C++ Compose*](https://software.intel.com/en-us/intel-compilers) XE 2013 and higher
- [*Clang*](http://llvm.org/) 3.4 and higher
- [*Apple Clang 6.0*](https://developer.apple.com/library/mac/documentation/CompilerTools/Conceptual/LLVMCompilerOverview/index.html) and higher
- [*Visual C++*](http://www.visualstudio.com/) 2013 and higher
- [*CUDA*](https://developer.nvidia.com/about-cuda) 9.0 and higher (experimental)
- [*SYCL*](https://www.khronos.org/sycl/) (experimental: only [ComputeCpp](https://codeplay.com/products/computesuite/computecpp) implementation has been tested).
- Any C++11 compiler
For more information about *GLM*, please have a look at the [manual](manual.md) and the [API reference documentation](http://glm.g-truc.net/0.9.8/api/index.html).
The source code and the documentation are licensed under either the [Happy Bunny License (Modified MIT) or the MIT License](manual.md#section0).
Thanks for contributing to the project by [submitting pull requests](https://github.com/g-truc/glm/pulls).
```cpp
#include <glm/vec3.hpp> // glm::vec3
#include <glm/vec4.hpp> // glm::vec4
#include <glm/mat4x4.hpp> // glm::mat4
#include <glm/ext/matrix_transform.hpp> // glm::translate, glm::rotate, glm::scale
#include <glm/ext/matrix_clip_space.hpp> // glm::perspective
#include <glm/ext/scalar_constants.hpp> // glm::pi
glm::mat4 camera(float Translate, glm::vec2 const& Rotate)
{
glm::mat4 Projection = glm::perspective(glm::pi<float>() * 0.25f, 4.0f / 3.0f, 0.1f, 100.f);
glm::mat4 View = glm::translate(glm::mat4(1.0f), glm::vec3(0.0f, 0.0f, -Translate));
View = glm::rotate(View, Rotate.y, glm::vec3(-1.0f, 0.0f, 0.0f));
View = glm::rotate(View, Rotate.x, glm::vec3(0.0f, 1.0f, 0.0f));
glm::mat4 Model = glm::scale(glm::mat4(1.0f), glm::vec3(0.5f));
return Projection * View * Model;
}
```
## [Lastest release](https://github.com/g-truc/glm/releases/latest)
## Project Health
| Service | System | Compiler | Status |
| ------- | ------ | -------- | ------ |
| [Travis CI](https://travis-ci.org/g-truc/glm)| MacOSX, Linux 64 bits | Clang 3.6, Clang 5.0, GCC 4.9, GCC 7.3 | [](https://travis-ci.org/g-truc/glm)
| [AppVeyor](https://ci.appveyor.com/project/Groovounet/glm)| Windows 32 and 64 | Visual Studio 2013, Visual Studio 2015, Visual Studio 2017 | [](https://ci.appveyor.com/project/Groovounet/glm)
## Release notes
### [GLM 0.9.9.9](https://github.com/g-truc/glm/releases/tag/0.9.9.9) - 2020-XX-XX
#### Features:
- Added *GLM_EXT_scalar_reciprocal* with tests
- Added *GLM_EXT_vector_reciprocal* with tests
- Added `glm::iround` and `glm::uround` to *GLM_EXT_scalar_common* and *GLM_EXT_vector_common*
- Added *GLM_EXT_matrix_integer* with tests
#### Improvements:
- Added `constexpr` qualifier for `cross` product #1040
- Added `constexpr` qualifier for `dot` product #1040
#### Fixes:
- Fixed incorrect assertion for `glm::min` and `glm::max` #1009
- Fixed quaternion orientation in `glm::decompose` #1012
- Fixed singularity in quaternion to euler angle roll conversion #1019
- Fixed `quat` `glm::pow` handling of small magnitude quaternions #1022
- Fixed `glm::fastNormalize` build error #1033
- Fixed `glm::isMultiple` build error #1034
- Fixed `glm::adjugate` calculation #1035
- Fixed `glm::angle` discards the sign of result for angles in range (2*pi-1, 2*pi) #1038
- Removed ban on using `glm::string_cast` with *CUDA* host code #1041
### [GLM 0.9.9.8](https://github.com/g-truc/glm/releases/tag/0.9.9.8) - 2020-04-13
#### Features:
- Added *GLM_EXT_vector_intX* and *GLM_EXT_vector_uintX* extensions
- Added *GLM_EXT_matrix_intX* and *GLM_EXT_matrix_uintX* extensions
#### Improvements:
- Added `glm::clamp`, `glm::repeat`, `glm::mirrorClamp` and `glm::mirrorRepeat` function to `GLM_EXT_scalar_commond` and `GLM_EXT_vector_commond` extensions with tests
#### Fixes:
- Fixed unnecessary warnings from `matrix_projection.inl` #995
- Fixed quaternion `glm::slerp` overload which interpolates with extra spins #996
- Fixed for `glm::length` using arch64 #992
- Fixed singularity check for `glm::quatLookAt` #770
### [GLM 0.9.9.7](https://github.com/g-truc/glm/releases/tag/0.9.9.7) - 2020-01-05
#### Improvements:
- Improved *Neon* support with more functions optimized #950
- Added *CMake* *GLM* interface #963
- Added `glm::fma` implementation based on `std::fma` #969
- Added missing quat constexpr #955
- Added `GLM_FORCE_QUAT_DATA_WXYZ` to store quat data as w,x,y,z instead of x,y,z,w #983
#### Fixes:
- Fixed equal *ULP* variation when using negative sign #965
- Fixed for intersection ray/plane and added related tests #953
- Fixed ARM 64bit detection #949
- Fixed *GLM_EXT_matrix_clip_space* warnings #980
- Fixed Wimplicit-int-float-conversion warnings with clang 10+ #986
- Fixed *GLM_EXT_matrix_clip_space* `perspectiveFov`
### [GLM 0.9.9.6](https://github.com/g-truc/glm/releases/tag/0.9.9.6) - 2019-09-08
#### Features:
- Added *Neon* support #945
- Added *SYCL* support #914
- Added *GLM_EXT_scalar_integer* extension with power of two and multiple scalar functions
- Added *GLM_EXT_vector_integer* extension with power of two and multiple vector functions
#### Improvements:
- Added *Visual C++ 2019* detection
- Added *Visual C++ 2017* 15.8 and 15.9 detection
- Added missing genType check for `glm::bitCount` and `glm::bitfieldReverse` #893
#### Fixes:
- Fixed for g++6 where -std=c++1z sets __cplusplus to 201500 instead of 201402 #921
- Fixed hash hashes qua instead of tquat #919
- Fixed `.natvis` as structs renamed #915
- Fixed `glm::ldexp` and `glm::frexp` declaration #895
- Fixed missing const to quaternion conversion operators #890
- Fixed *GLM_EXT_scalar_ulp* and *GLM_EXT_vector_ulp* API coding style
- Fixed quaternion componant order: `w, {x, y, z}` #916
- Fixed `GLM_HAS_CXX11_STL` broken on Clang with Linux #926
- Fixed *Clang* or *GCC* build due to wrong `GLM_HAS_IF_CONSTEXPR` definition #907
- Fixed *CUDA* 9 build #910
#### Deprecation:
- Removed CMake install and uninstall scripts
### [GLM 0.9.9.5](https://github.com/g-truc/glm/releases/tag/0.9.9.5) - 2019-04-01
#### Fixes:
- Fixed build errors when defining `GLM_ENABLE_EXPERIMENTAL` #884 #883
- Fixed `if constexpr` warning #887
- Fixed missing declarations for `glm::frexp` and `glm::ldexp` #886
### [GLM 0.9.9.4](https://github.com/g-truc/glm/releases/tag/0.9.9.4) - 2019-03-19
#### Features:
- Added `glm::mix` implementation for matrices in *GLM_EXT_matrix_common/ #842
- Added *CMake* `BUILD_SHARED_LIBS` and `BUILD_STATIC_LIBS` build options #871
#### Improvements:
- Added GLM_FORCE_INTRINSICS to ena

吾名招财
- 粉丝: 1979
- 资源: 40
最新资源
- 基于C语言的嵌入式软件定时器详细文档+全部资料+高分项目+源码.zip
- 基于ffmpeg的直播推流器,超级稳定,经过长时间稳定性测试,超低延时,可用于手机,电视,嵌入式等直播App及设备。详细文档+全部资料+高分项目+源码.zip
- 基于DCT算法的水印嵌入和提取的移动智能终端数字图像证据系统详细文档+全部资料+高分项目+源码.zip
- 基于FPGA的DDR1控制器,为低端FPGA嵌入式系统提供廉价、大容量的存储详细文档+全部资料+高分项目+源码.zip
- 基于FreeRTOS开发的嵌入式开发框架详细文档+全部资料+高分项目+源码.zip
- 基于FMCW雷达的多天线定位系统详细文档+全部资料+高分项目+源码.zip
- 基于FriendlyARM6410平台的嵌入式Qt程序:实时天气信息,远程vnc控制,远程监视摄像头,语音控制,语音输出TTS详细文档+全部资料+高分项目+源码.zip
- 基于FSMPSTem32的嵌入式音乐播放器、实训作业详细文档+全部资料+高分项目+源码.zip
- 基于GEC6818嵌入式大作业详细文档+全部资料+高分项目+源码.zip
- 基于jetty嵌入式容器的java性能分析工具,内嵌H2 database,以图表形式直观展现应用当前性能数据详细文档+全部资料+高分项目+源码.zip
- 基于jq开发的数学公式插件,可随意嵌入web中详细文档+全部资料+高分项目+源码.zip
- 基于Linux系统的应用程序,旨在搭建一套完整的多进程多线程通讯的消息框架. 支持多SOC的嵌入式APP详细文档+全部资料+高分项目+源码.zip
- 基于mplayer的嵌入式音视频播放器详细文档+全部资料+高分项目+源码.zip
- 基于LSM-Tree的嵌入式数据库详细文档+全部资料+高分项目+源码.zip
- 基于liunx下的一个QT程序,KTV点歌系统嵌入式设备详细文档+全部资料+高分项目+源码.zip
- 基于MySQL的嵌入式Linux智慧农业采集控制系统详细文档+全部资料+高分项目+源码.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


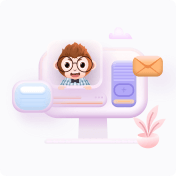