/*
* Copyright 2015 The WebRTC project authors. All Rights Reserved.
*
* Use of this source code is governed by a BSD-style license
* that can be found in the LICENSE file in the root of the source
* tree. An additional intellectual property rights grant can be found
* in the file PATENTS. All contributing project authors may
* be found in the AUTHORS file in the root of the source tree.
*/
#import <Foundation/Foundation.h>
#import <WebRTC/RTCMacros.h>
@class RTC_OBJC_TYPE(RTCConfiguration);
@class RTC_OBJC_TYPE(RTCDataChannel);
@class RTC_OBJC_TYPE(RTCDataChannelConfiguration);
@class RTC_OBJC_TYPE(RTCIceCandidate);
@class RTC_OBJC_TYPE(RTCIceCandidateErrorEvent);
@class RTC_OBJC_TYPE(RTCMediaConstraints);
@class RTC_OBJC_TYPE(RTCMediaStream);
@class RTC_OBJC_TYPE(RTCMediaStreamTrack);
@class RTC_OBJC_TYPE(RTCPeerConnectionFactory);
@class RTC_OBJC_TYPE(RTCRtpReceiver);
@class RTC_OBJC_TYPE(RTCRtpSender);
@class RTC_OBJC_TYPE(RTCRtpTransceiver);
@class RTC_OBJC_TYPE(RTCRtpTransceiverInit);
@class RTC_OBJC_TYPE(RTCSessionDescription);
@class RTC_OBJC_TYPE(RTCStatisticsReport);
@class RTC_OBJC_TYPE(RTCLegacyStatsReport);
typedef NS_ENUM(NSInteger, RTCRtpMediaType);
NS_ASSUME_NONNULL_BEGIN
extern NSString *const kRTCPeerConnectionErrorDomain;
extern int const kRTCSessionDescriptionErrorCode;
/** Represents the signaling state of the peer connection. */
typedef NS_ENUM(NSInteger, RTCSignalingState) {
RTCSignalingStateStable,
RTCSignalingStateHaveLocalOffer,
RTCSignalingStateHaveLocalPrAnswer,
RTCSignalingStateHaveRemoteOffer,
RTCSignalingStateHaveRemotePrAnswer,
// Not an actual state, represents the total number of states.
RTCSignalingStateClosed,
};
/** Represents the ice connection state of the peer connection. */
typedef NS_ENUM(NSInteger, RTCIceConnectionState) {
RTCIceConnectionStateNew,
RTCIceConnectionStateChecking,
RTCIceConnectionStateConnected,
RTCIceConnectionStateCompleted,
RTCIceConnectionStateFailed,
RTCIceConnectionStateDisconnected,
RTCIceConnectionStateClosed,
RTCIceConnectionStateCount,
};
/** Represents the combined ice+dtls connection state of the peer connection. */
typedef NS_ENUM(NSInteger, RTCPeerConnectionState) {
RTCPeerConnectionStateNew,
RTCPeerConnectionStateConnecting,
RTCPeerConnectionStateConnected,
RTCPeerConnectionStateDisconnected,
RTCPeerConnectionStateFailed,
RTCPeerConnectionStateClosed,
};
/** Represents the ice gathering state of the peer connection. */
typedef NS_ENUM(NSInteger, RTCIceGatheringState) {
RTCIceGatheringStateNew,
RTCIceGatheringStateGathering,
RTCIceGatheringStateComplete,
};
/** Represents the stats output level. */
typedef NS_ENUM(NSInteger, RTCStatsOutputLevel) {
RTCStatsOutputLevelStandard,
RTCStatsOutputLevelDebug,
};
typedef void (^RTCCreateSessionDescriptionCompletionHandler)(
RTC_OBJC_TYPE(RTCSessionDescription) *_Nullable sdp, NSError *_Nullable error);
typedef void (^RTCSetSessionDescriptionCompletionHandler)(NSError *_Nullable error);
@class RTC_OBJC_TYPE(RTCPeerConnection);
RTC_OBJC_EXPORT
@protocol RTC_OBJC_TYPE
(RTCPeerConnectionDelegate)<NSObject>
/** Called when the SignalingState changed. */
- (void)peerConnection
: (RTC_OBJC_TYPE(RTCPeerConnection) *)peerConnection didChangeSignalingState
: (RTCSignalingState)stateChanged;
/** Called when media is received on a new stream from remote peer. */
- (void)peerConnection:(RTC_OBJC_TYPE(RTCPeerConnection) *)peerConnection
didAddStream:(RTC_OBJC_TYPE(RTCMediaStream) *)stream;
/** Called when a remote peer closes a stream.
* This is not called when RTCSdpSemanticsUnifiedPlan is specified.
*/
- (void)peerConnection:(RTC_OBJC_TYPE(RTCPeerConnection) *)peerConnection
didRemoveStream:(RTC_OBJC_TYPE(RTCMediaStream) *)stream;
/** Called when negotiation is needed, for example ICE has restarted. */
- (void)peerConnectionShouldNegotiate:(RTC_OBJC_TYPE(RTCPeerConnection) *)peerConnection;
/** Called any time the IceConnectionState changes. */
- (void)peerConnection:(RTC_OBJC_TYPE(RTCPeerConnection) *)peerConnection
didChangeIceConnectionState:(RTCIceConnectionState)newState;
/** Called any time the IceGatheringState changes. */
- (void)peerConnection:(RTC_OBJC_TYPE(RTCPeerConnection) *)peerConnection
didChangeIceGatheringState:(RTCIceGatheringState)newState;
/** New ice candidate has been found. */
- (void)peerConnection:(RTC_OBJC_TYPE(RTCPeerConnection) *)peerConnection
didGenerateIceCandidate:(RTC_OBJC_TYPE(RTCIceCandidate) *)candidate;
/** Called when a group of local Ice candidates have been removed. */
- (void)peerConnection:(RTC_OBJC_TYPE(RTCPeerConnection) *)peerConnection
didRemoveIceCandidates:(NSArray<RTC_OBJC_TYPE(RTCIceCandidate) *> *)candidates;
/** New data channel has been opened. */
- (void)peerConnection:(RTC_OBJC_TYPE(RTCPeerConnection) *)peerConnection
didOpenDataChannel:(RTC_OBJC_TYPE(RTCDataChannel) *)dataChannel;
/** Called when signaling indicates a transceiver will be receiving media from
* the remote endpoint.
* This is only called with RTCSdpSemanticsUnifiedPlan specified.
*/
@optional
/** Called any time the IceConnectionState changes following standardized
* transition. */
- (void)peerConnection:(RTC_OBJC_TYPE(RTCPeerConnection) *)peerConnection
didChangeStandardizedIceConnectionState:(RTCIceConnectionState)newState;
/** Called any time the PeerConnectionState changes. */
- (void)peerConnection:(RTC_OBJC_TYPE(RTCPeerConnection) *)peerConnection
didChangeConnectionState:(RTCPeerConnectionState)newState;
- (void)peerConnection:(RTC_OBJC_TYPE(RTCPeerConnection) *)peerConnection
didStartReceivingOnTransceiver:(RTC_OBJC_TYPE(RTCRtpTransceiver) *)transceiver;
/** Called when a receiver and its track are created. */
- (void)peerConnection:(RTC_OBJC_TYPE(RTCPeerConnection) *)peerConnection
didAddReceiver:(RTC_OBJC_TYPE(RTCRtpReceiver) *)rtpReceiver
streams:(NSArray<RTC_OBJC_TYPE(RTCMediaStream) *> *)mediaStreams;
/** Called when the receiver and its track are removed. */
- (void)peerConnection:(RTC_OBJC_TYPE(RTCPeerConnection) *)peerConnection
didRemoveReceiver:(RTC_OBJC_TYPE(RTCRtpReceiver) *)rtpReceiver;
/** Called when the selected ICE candidate pair is changed. */
- (void)peerConnection:(RTC_OBJC_TYPE(RTCPeerConnection) *)peerConnection
didChangeLocalCandidate:(RTC_OBJC_TYPE(RTCIceCandidate) *)local
remoteCandidate:(RTC_OBJC_TYPE(RTCIceCandidate) *)remote
lastReceivedMs:(int)lastDataReceivedMs
changeReason:(NSString *)reason;
/** Called when gathering of an ICE candidate failed. */
- (void)peerConnection:(RTC_OBJC_TYPE(RTCPeerConnection) *)peerConnection
didFailToGatherIceCandidate:(RTC_OBJC_TYPE(RTCIceCandidateErrorEvent) *)event;
@end
RTC_OBJC_EXPORT
@interface RTC_OBJC_TYPE (RTCPeerConnection) : NSObject
/** The object that will be notifed about events such as state changes and
* streams being added or removed.
*/
@property(nonatomic, weak, nullable) id<RTC_OBJC_TYPE(RTCPeerConnectionDelegate)> delegate;
/** This property is not available with RTCSdpSemanticsUnifiedPlan. Please use
* `senders` instead.
*/
@property(nonatomic, readonly) NSArray<RTC_OBJC_TYPE(RTCMediaStream) *> *localStreams;
@property(nonatomic, readonly, nullable) RTC_OBJC_TYPE(RTCSessionDescription) * localDescription;
@property(nonatomic, readonly, nullable) RTC_OBJC_TYPE(RTCSessionDescription) * remoteDescription;
@property(nonatomic, readonly) RTCSignalingState signalingState;
@property(nonatomic, readonly) RTCIceConnectionState iceConnectionState;
@property(nonatomic, readonly) RTCPeerConnectionState connectionState;
@property(nonatomic, readonly) RTCIceGatheringState iceGatheringState;
@property(nonatomic, readonly, copy) RTC_OBJC_TYPE(RTCConfiguration) * configuration;
/** Gets all RTCRtpSenders associated with this peer connection.
* Note: reading this property returns different instance
没有合适的资源?快使用搜索试试~ 我知道了~
WebRTC.framework iOS Release环境 Arm64与x86_64架构
Mach-O universal binary with 2 architectures: [x86_64:Mach-O 64-bit dynamically linked shared library x86_64] [arm64]
Mach-O 64-bit dynamically linked shared library x86_64
Mach-O 64-bit dynamically linked shared library arm64
收起资源包目录


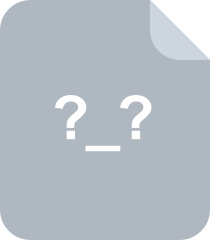

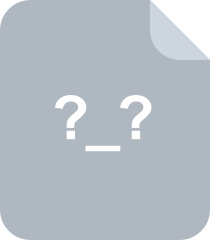

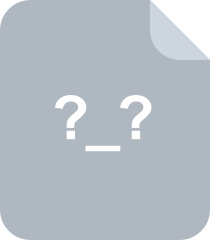
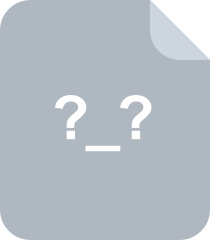
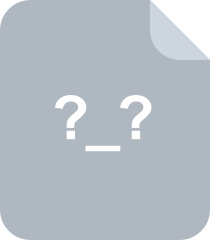
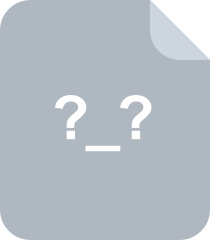
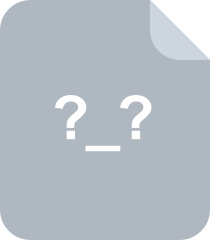
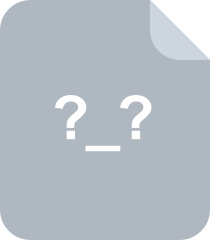
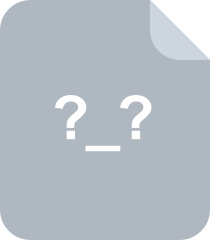
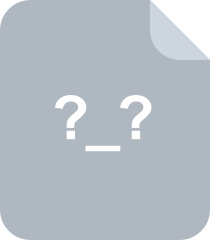
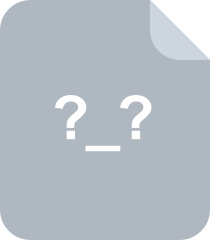
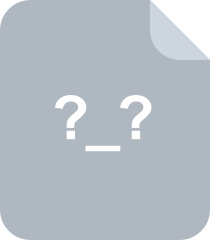
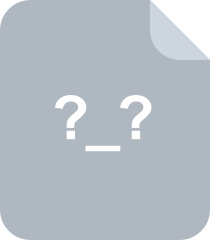
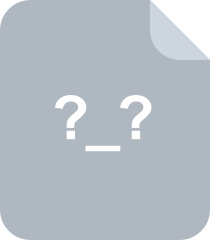
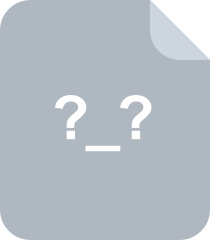
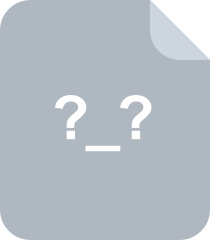
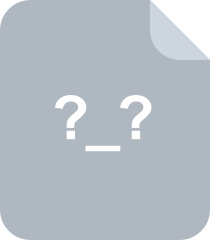
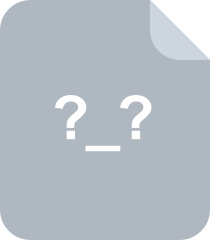
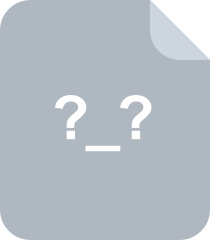
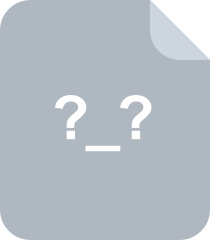
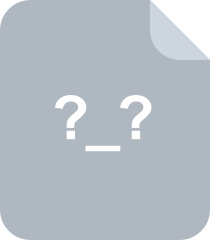
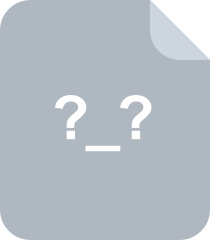
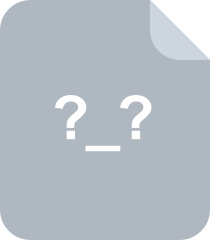
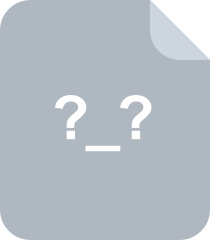
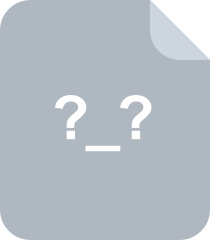
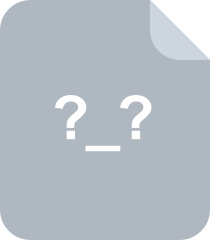
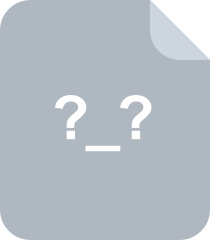
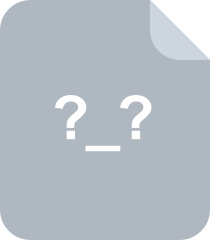
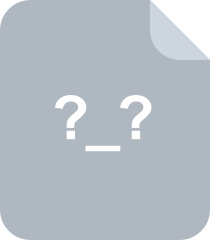
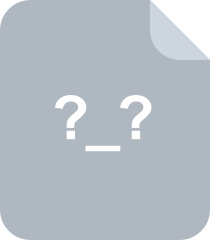
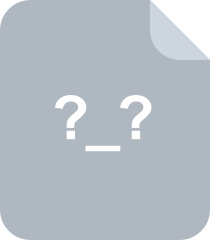
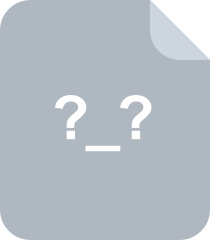
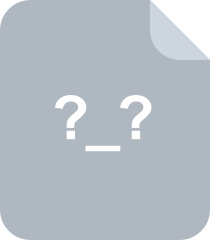
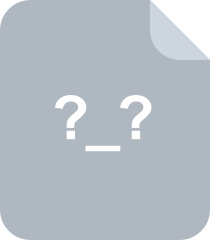
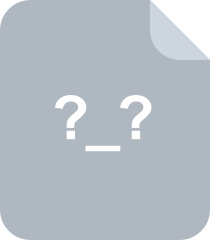
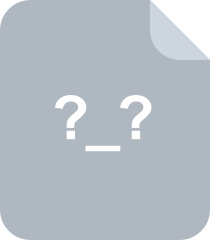
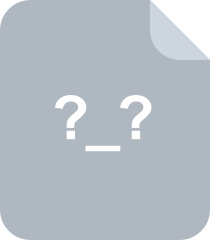
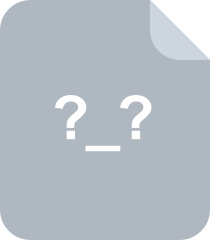
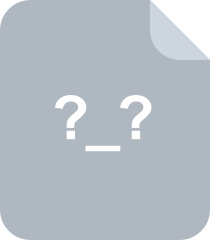
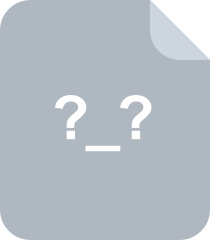
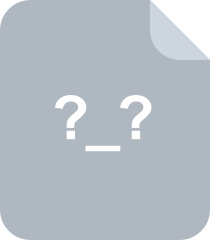
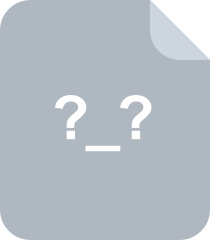
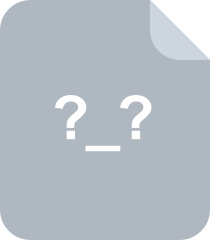
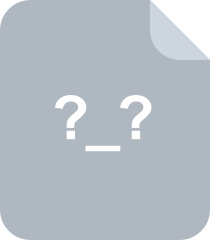
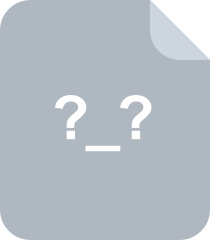
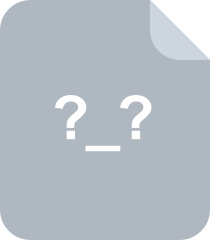
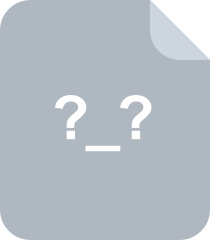
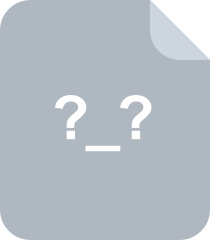
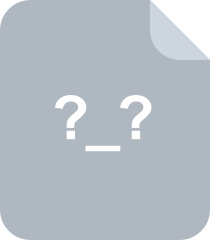
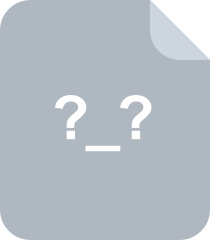
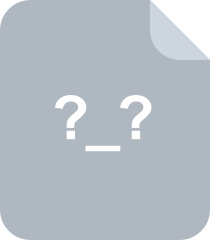
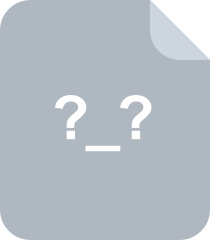
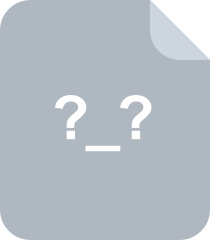
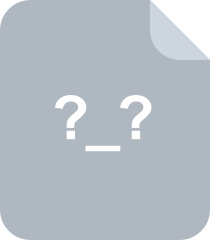
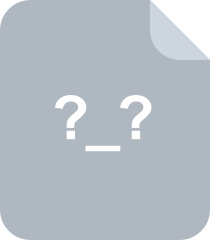
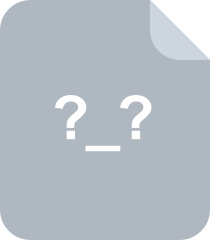
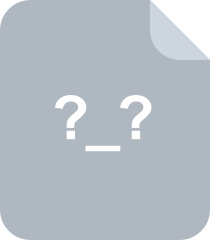
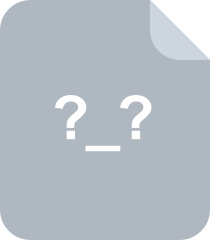
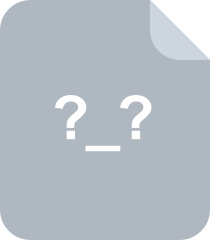
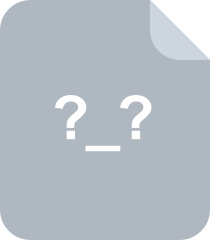
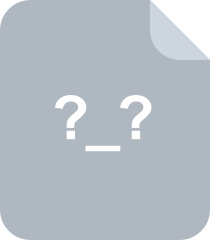
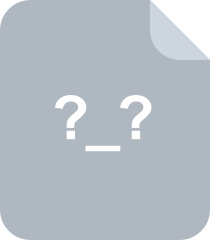
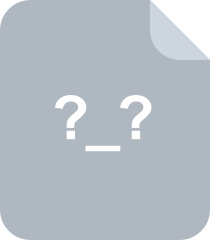
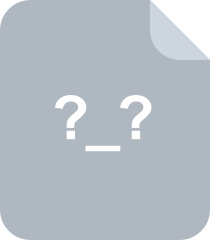
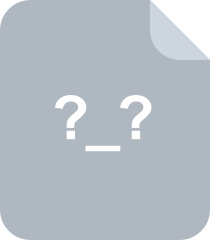
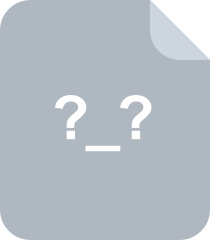
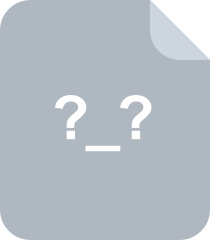
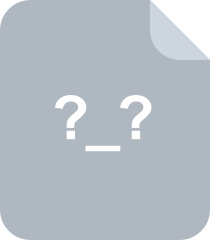
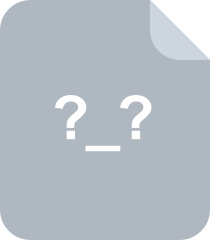
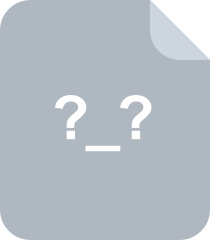
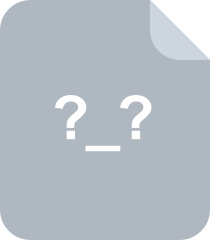
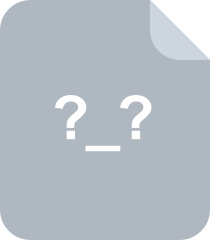
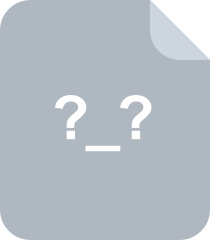
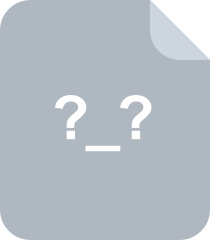
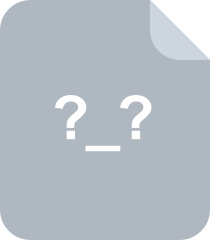
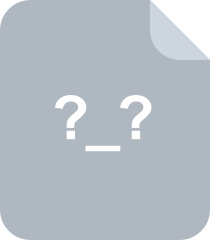
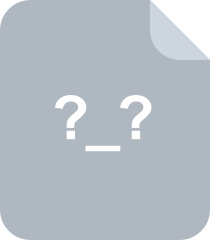
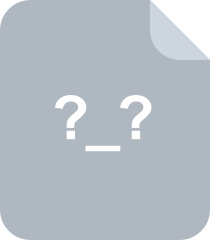
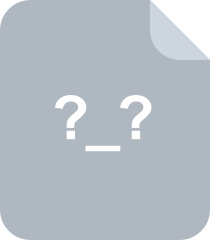
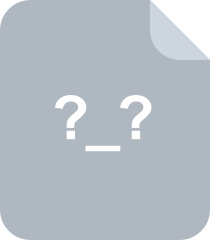
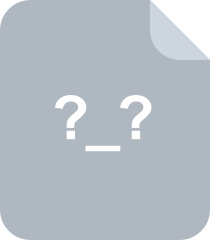
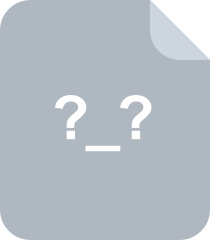
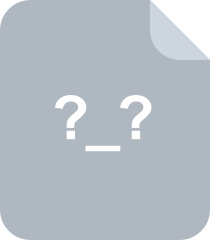
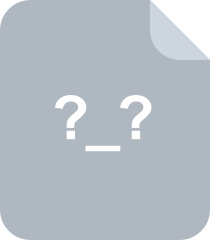
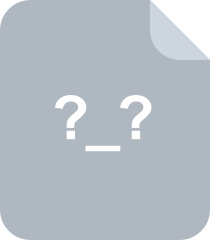
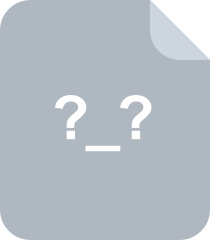
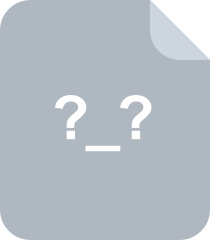
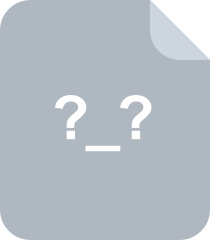
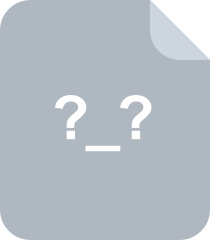
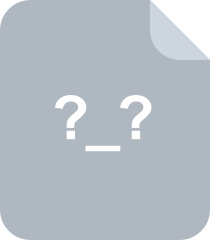
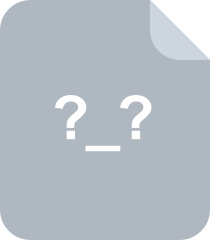
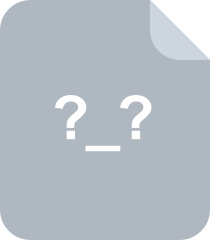
共 92 条
- 1
资源推荐
资源预览
资源评论
163 浏览量
2021-07-20 上传
2020-06-03 上传
118 浏览量
148 浏览量
113 浏览量
177 浏览量
128 浏览量
2017-12-27 上传

2017-10-25 上传
188 浏览量
2014-03-07 上传

2023-08-23 上传
2023-11-16 上传
2020-05-01 上传
资源评论
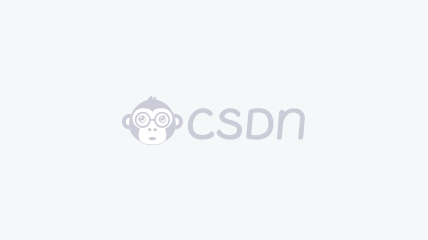

谷云龙GGBond
- 粉丝: 81
- 资源: 2
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

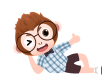
安全验证
文档复制为VIP权益,开通VIP直接复制
