package com.light.hexo.core.admin.service.impl;
import com.github.pagehelper.PageHelper;
import com.github.pagehelper.PageInfo;
import com.light.hexo.common.base.BaseServiceImpl;
import com.light.hexo.core.admin.component.BaiDuPushService;
import com.light.hexo.common.constant.ConfigEnum;
import com.light.hexo.common.constant.HexoExceptionEnum;
import com.light.hexo.mapper.mapper.PostMapper;
import com.light.hexo.mapper.base.BaseMapper;
import com.light.hexo.mapper.model.*;
import com.light.hexo.common.event.PostEvent;
import com.light.hexo.core.admin.service.*;
import com.light.hexo.core.portal.constant.PageConstant;
import com.light.hexo.core.portal.model.HexoPageInfo;
import com.light.hexo.common.base.BaseRequest;
import com.light.hexo.common.component.event.BaseEvent;
import com.light.hexo.common.component.event.EventEnum;
import com.light.hexo.common.component.event.EventPublisher;
import com.light.hexo.common.constant.HexoConstant;
import com.light.hexo.common.exception.GlobalException;
import com.light.hexo.common.request.PostRequest;
import com.light.hexo.common.util.*;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.io.FileUtils;
import org.apache.commons.lang3.StringUtils;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.cache.annotation.CacheConfig;
import org.springframework.cache.annotation.Cacheable;
import org.springframework.context.annotation.Lazy;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.util.CollectionUtils;
import org.springframework.web.context.WebApplicationContext;
import tk.mybatis.mapper.entity.Example;
import tk.mybatis.mapper.util.Sqls;
import javax.servlet.ServletContext;
import java.io.*;
import java.nio.charset.StandardCharsets;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.util.*;
import java.util.concurrent.TimeUnit;
import java.util.function.Function;
import java.util.stream.Collectors;
/**
* @Author MoonlightL
* @ClassName: PostServiceImpl
* @ProjectName hexo-boot
* @Description: 文章 Service 实现
* @DateTime 2020/7/31 17:28
*/
@CacheConfig(cacheNames = "postCache")
@Service
@Slf4j
public class PostServiceImpl extends BaseServiceImpl<Post> implements PostService {
@Autowired
private PostMapper postMapper;
@Autowired
private CategoryService categoryService;
@Autowired
private TagService tagService;
@Autowired
private PostTagService postTagService;
@Autowired
private ConfigService configService;
@Autowired
private BaiDuPushService baiDuPushService;
@Autowired
private PostTaskService postTaskService;
@Autowired
@Lazy
private EventPublisher eventPublisher;
private static final Integer COVER_NUM = 20;
@Override
public BaseMapper<Post> getBaseMapper() {
return this.postMapper;
}
@Override
protected Example getExample(BaseRequest request) {
// 获取查询参数
PostRequest postRequest = (PostRequest) request;
Example example = Example.builder(Post.class)
.notSelect("content", "contentHtml").orderByDesc("createTime").build();
Example.Criteria criteria = example.createCriteria();
Boolean publish = postRequest.getPublish();
if (publish != null) {
criteria.andEqualTo("publish", publish);
}
Boolean top = postRequest.getTop();
if (top != null) {
criteria.andEqualTo("top", top);
}
String title = postRequest.getTitle();
if (StringUtils.isNotBlank(title)) {
criteria.andLike("title", "%" + title.trim() + "%");
}
Integer categoryId = postRequest.getCategoryId();
if (categoryId != null) {
criteria.andEqualTo("categoryId", categoryId);
}
String publishDate = postRequest.getPublishDate();
if (StringUtils.isNotBlank(publishDate)) {
criteria.andEqualTo("publishDate", publishDate.trim());
}
String authCode = postRequest.getAuthCode();
if (StringUtils.isNotBlank(authCode)) {
if ("1".equals(authCode)) {
criteria.andNotEqualTo("authCode", "");
} else {
criteria.andEqualTo("authCode", "");
}
}
return example;
}
@Override
public PageInfo<Post> findPage(BaseRequest<Post> request) throws GlobalException {
PageInfo<Post> pageInfo = super.findPage(request);
List<Post> list = pageInfo.getList();
if (CollectionUtils.isEmpty(list)) {
return new PageInfo<>();
}
List<Category> categoryList = this.categoryService.findAll();
Map<Integer, Category> categoryMap = categoryList.stream().collect(Collectors.toMap(Category::getId, Function.identity(), (k1, k2)->k1));
for (Post post : list) {
Category category = categoryMap.get(post.getCategoryId());
post.setCategoryName(category == null ? "默认" : category.getName());
if (StringUtils.isNotBlank(post.getCustomLink())) {
post.setLink(post.getCustomLink() + ".html");
}
}
return pageInfo;
}
@Override
public void removePostBatch(List<String> idStrList) throws GlobalException {
List<Long> idList = idStrList.stream().map(Long::valueOf).collect(Collectors.toList());
Example example = new Example(Post.class);
example.createCriteria().andIn("id", idList);
int num = this.getBaseMapper().deleteByExample(example);
if (num > 0) {
this.eventPublisher.emit(new PostEvent(this,null, PostEvent.Type.POST_NUM));
}
}
@Override
@Transactional(rollbackFor = Exception.class)
public void savePost(Post post) throws GlobalException {
Category category = this.categoryService.findById(post.getCategoryId());
if (category == null || !category.getState()) {
ExceptionUtil.throwEx(HexoExceptionEnum.ERROR_CATEGORY_NOT_EXIST);
}
// 前端上传的参数可能带空格,影响条件查询
post.setTitle(post.getTitle().trim());
Example example = new Example(Post.class);
example.createCriteria().andEqualTo("title", post.getTitle());
int titleCount = this.getBaseMapper().selectCountByExample(example);
if (titleCount > 0) {
ExceptionUtil.throwEx(HexoExceptionEnum.ERROR_POST_TITLE_REPEAT);
}
if (StringUtils.isBlank(post.getAuthor())) {
post.setAuthor(this.configService.getConfigValue(ConfigEnum.BLOG_AUTHOR.getName()));
}
String coverUrl = post.getCoverUrl();
if(StringUtils.isBlank(coverUrl)) {
int num = new Random().nextInt(COVER_NUM);
coverUrl = HexoConstant.DEFAULT_IMG_DIR + "/post/post_cover_" + num + ".jpg";
post.setCoverUrl(coverUrl);
}
boolean isMarkdown = this.configService.getConfigValue(ConfigEnum.EDITOR_TYPE.getName()).equals("markdown");
String content = post.getContent();
// 摘要
post.setSummary(this.interceptContent(content, isMarkdown))
.setSummaryHtml(this.interceptContentHtml(content, isMarkdown));
if (isMarkdown) {
post.setContentHtml(MarkdownUtil.md2html(post.getContent()));
} else {
post.setContentHtml(this.transformHtml(post.getContent()));
}
LocalDateTime now = LocalDateTime.now();
LocalDateTime jobTime = post.getJ
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
idea连接mysql数据库,基于 Spring Boot + MySQL 的博客系统,个人经导师指导并认可通过的98分大作业设计项目,适用人群:计算机,人工智能、电子信息,软件工程等专业的大学生课程设计、期末大作业或毕业设计,作为“参考资料”使用。 idea连接mysql数据库,基于 Spring Boot + MySQL 的博客系统,个人经导师指导并认可通过的98分大作业设计项目,适用人群:计算机,人工智能、电子信息,软件工程等专业的大学生课程设计、期末大作业或毕业设计,作为“参考资料”使用。 idea连接mysql数据库,基于 Spring Boot + MySQL 的博客系统,个人经导师指导并认可通过的98分大作业设计项目,适用人群:计算机,人工智能、电子信息,软件工程等专业的大学生课程设计、期末大作业或毕业设计,作为“参考资料”使用。
资源推荐
资源详情
资源评论


























收起资源包目录

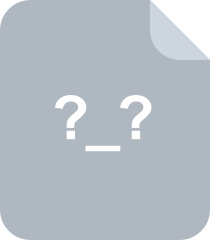
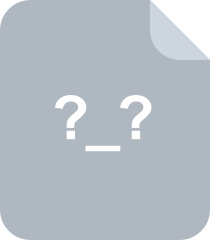
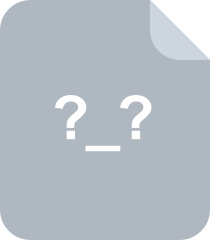
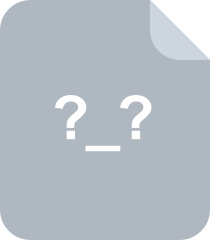
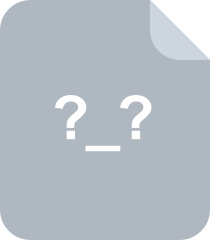
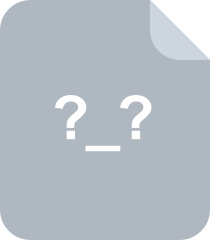
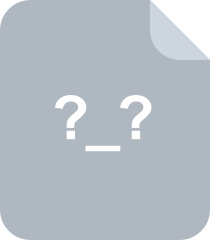
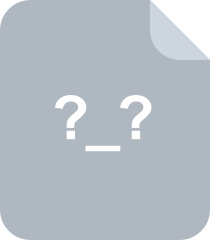
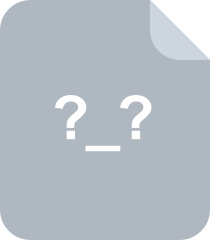
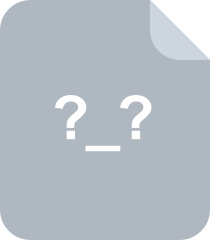
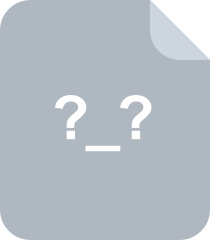
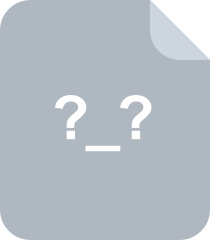
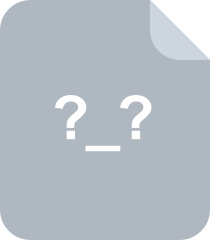
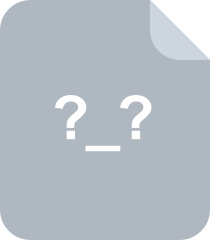
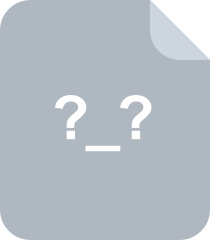
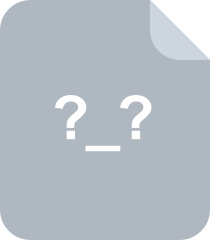
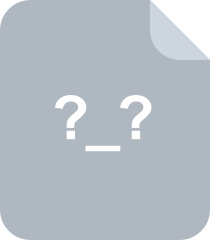
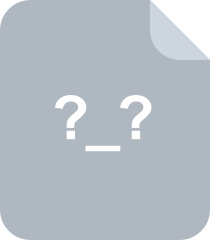
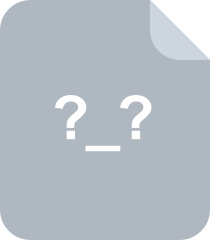
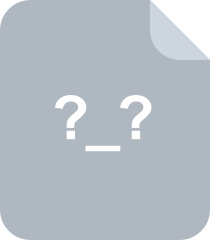
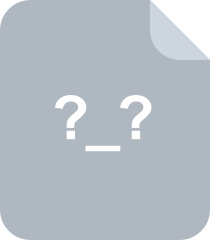
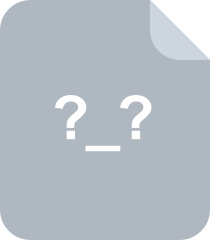
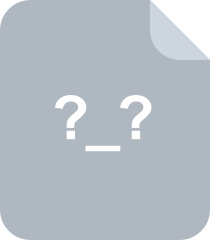
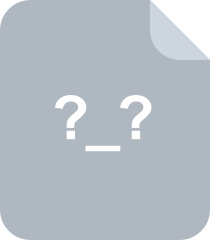
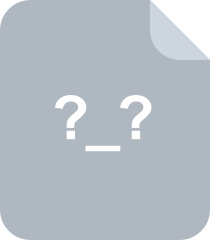
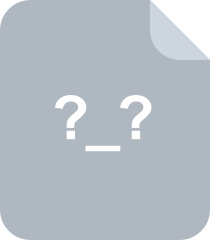
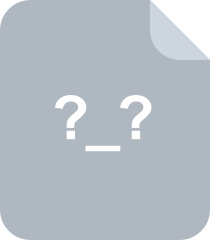
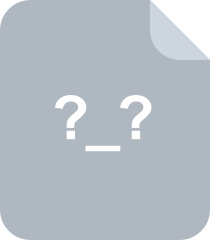
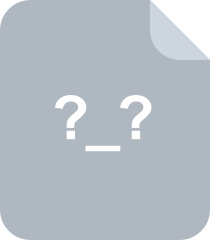
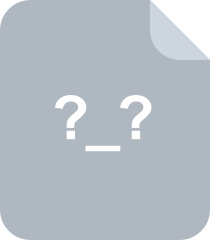
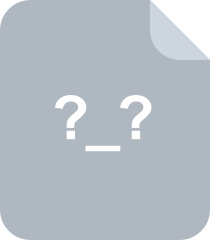
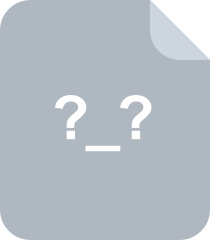
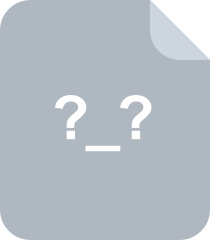
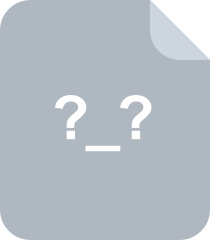
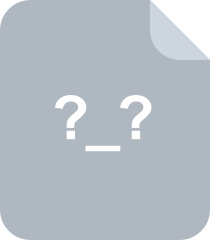
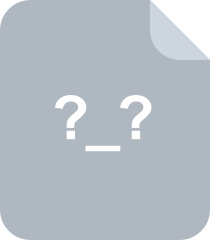
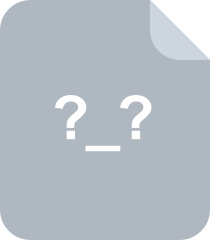
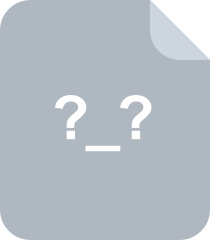
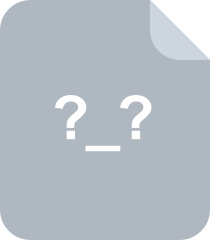
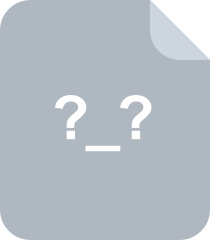
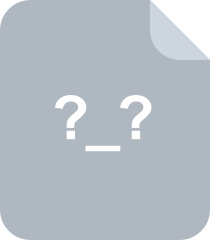
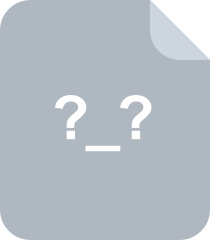
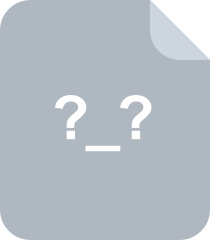
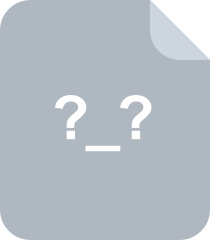
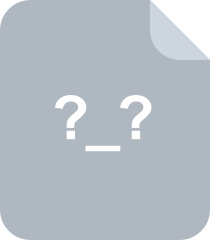
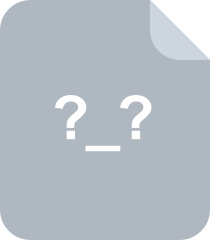
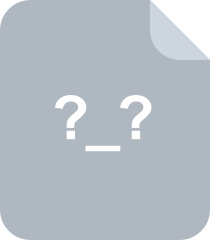
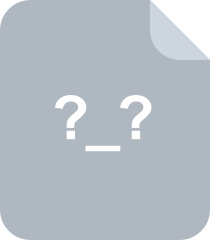
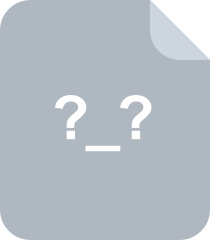
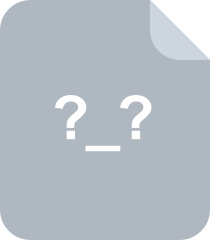
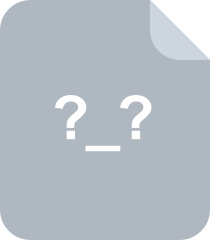
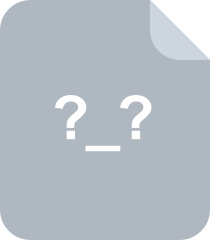
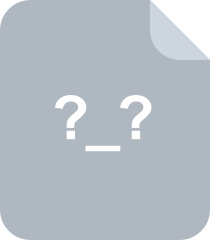
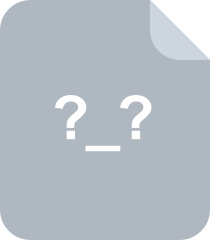
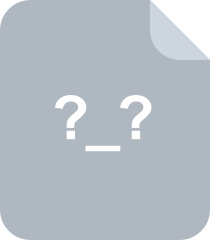
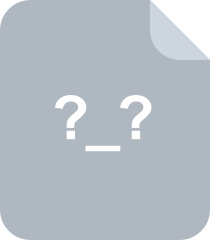
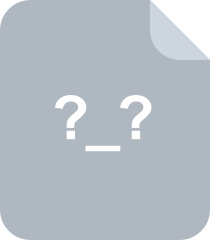
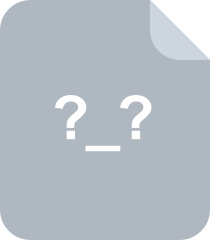
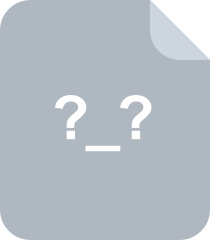
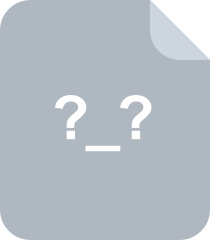
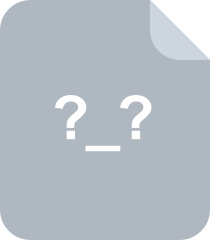
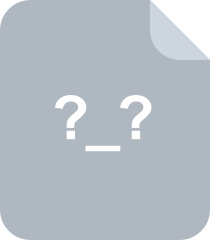
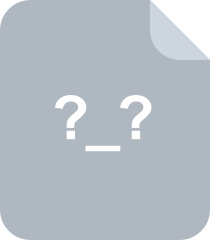
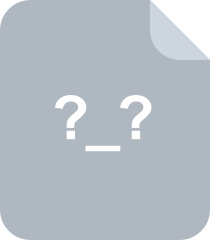
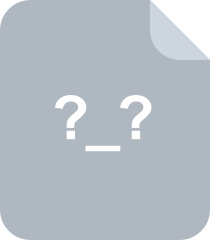
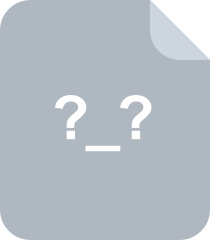
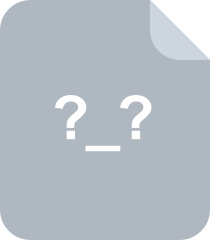
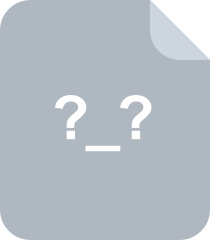
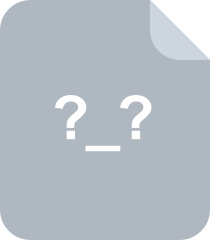
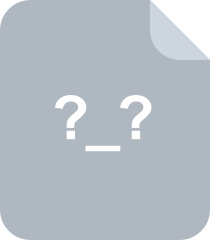
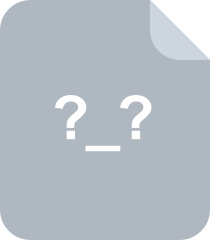
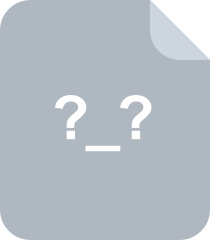
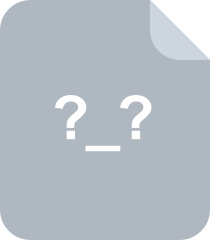
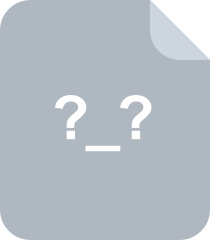
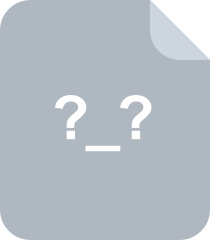
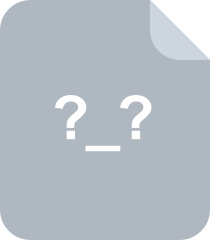
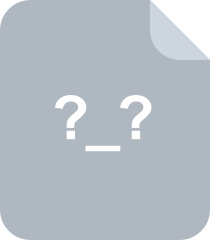
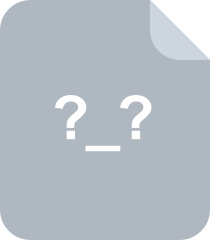
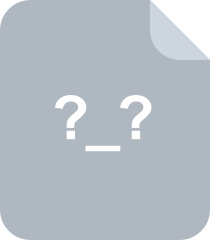
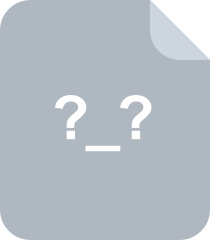
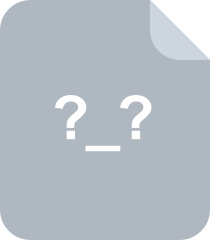
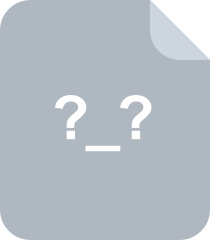
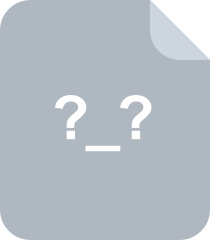
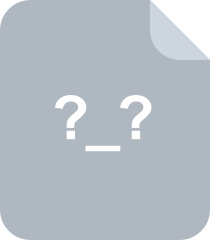
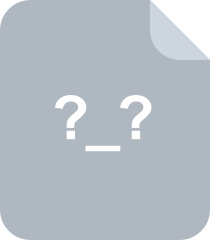
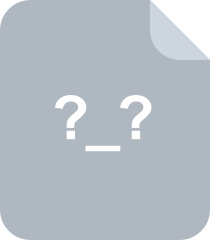
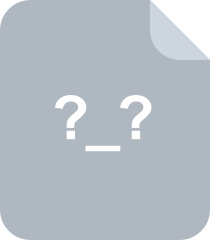
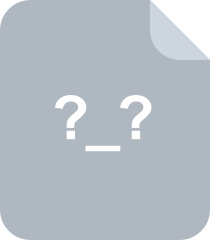
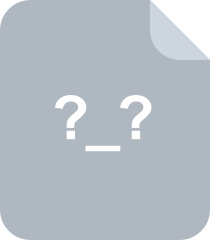
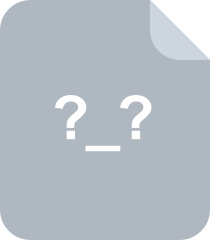
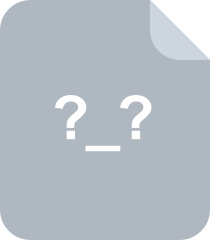
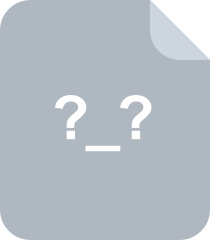
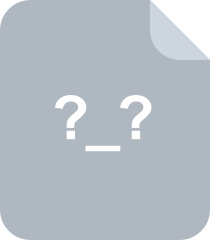
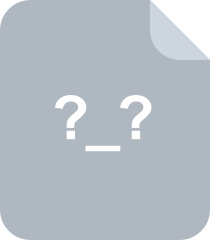
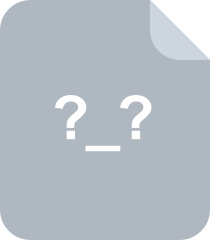
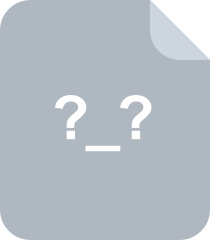
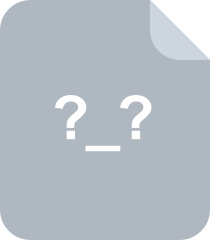
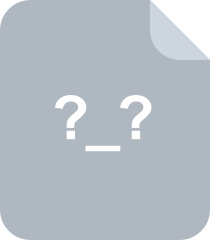
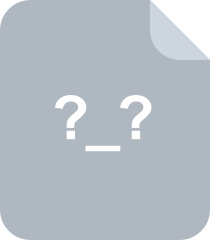
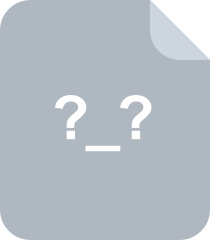
共 1923 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
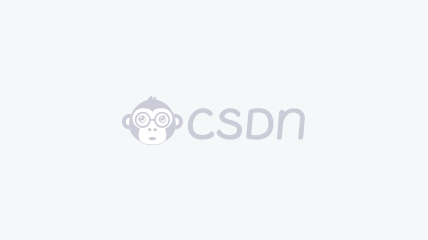

byg_qlh
- 粉丝: 1088
- 资源: 144
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

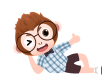
最新资源
- 域名交易管理系统新版源码+说明-高校毕设
- 农产品电子商务运营职业技能初级考试系统设计源码
- 共享农庄平台(公共版)源码+数据库-课程设计
- 农产品溯源系统新版源码+数据库+说明-毕业设计
- Cadance LDO带隙基准电路输出电压设计:基于TSMC 18rf工艺,模拟电路设计文件,工程文件齐全可直接导入模拟电路设计应用 ,Cadance LDO带隙基准电路输出电压1.2V,基于TSMC
- 基于COMSOL多物理场耦合仿真的变压器流固耦合与振动噪声分析:几何构造划分及仿真实践,基于COMSOL多物理场耦合仿真的变压器流固耦合与振动噪声分析:几何构造划分及仿真实践,基于COMSOL多物理场
- 运维监控-prometheus-linux
- windows平台-java使用jpcat使用网卡
- flaskProject1.rar
- 深度学习中VGG网络集成GAM注意力机制的PyTorch实现用于图像分类
- 农业病虫害命名实体识别数据集
- 逆合成孔径雷达稀疏成像技术:短孔径高分辨率压缩感知方法,逆合成孔径雷达稀疏成像与短孔径成像技术:结合压缩感知的探讨,逆合成孔径雷达稀疏成像,短孔径成像,压缩感知 ,核心关键词:逆合成孔径雷达; 稀疏
- 运维监控prometheus-node-exporter-1.8.2.linux-amd64-2025
- 农业病虫害智能识别系统新版源码
- 苏苏源码-springboot245-springboot项目评审系统(论文+PPT).zip
- 基于python的农业机器人后台监测系统新版源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


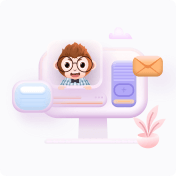
安全验证
文档复制为VIP权益,开通VIP直接复制
