function [Metrics]=polygonareametric(ActualLabel, PredictedLabel,isPlot)
%%%%% WARNING %%%%%%%
%NUMERICALLY LARGER CLASS WILL BE AUTOMATICALLY ASSIGNED AS PositiveClass
%THIS IS BECAUSE OF THE FUNCTION OF perfcurve. IT REQUIRES LARGER CLASS AS PositiveClass.
% INTRODUCTION:
% This study proposes a stable and profound knowledge criterion that allows the performance of a classifier
% to be evaluated with only a single metric called as polygon area metric (PAM). This function is not only
% calculates PAM value, but also gives Classification Accuracy (CA), Sensitivity (SE), Specificity (SP),
% Kappa (K) and F measure metrics.
%
% CITATION INFORMATION:
% Please cite the following paper for the usage of PAM value:
% Aydemir O., A New Performance Evaluation Metric for Classifiers: Polygon Area Metric, Journal of Classification, (2020). https://doi.org/10.1007/s00357-020-09362-5
%
% USAGE OF THE FUNCTION:
% INPUTS;
% -ActualLabel: Actual label of the trials (samples), 1xN dimension binary labels
% -PredictedLabel: Predicted (estimated) label of the trials (samples), 1xN dimension binary labels
% -isPlot: A logical value indicating whether the resultant figure will be drawn. Default is true
%
% OUTPUT;
% -Metrics: This struct gives 7 evaluation metrics which are Polygon Area...
% (PA), Classification_Accuracy (CA), Sensitivity (SE), Specificity...
% (SP), AUC (AUC), Kappa (K), F_measure (F_M), respectively.
% AUC: Area under curve value, which should be obtained by Receiver operating characteristic (ROC), 0<AUC<1
%
% EXAMPLE;
% -ActualLabel=[1 1 1 1 1 0 0 0 0];
% -PredictedLabel=[1 1 1 0 0 0 0 0 1];
% -[Metrics]=polygonareametric(ActualLabel,PredictedLabel)
%Code introduction
if nargin<2
error('You have to supply all required input paremeters, which are ActualLabel, PredictedLabel')
end
if nargin < 3
isPlot = true;
end
%plotting the widest polygon
A1=1;
A2=1;
A3=1;
A4=1;
A5=1;
A6=1;
a=[-A1 -A2/2 A3/2 A4 A5/2 -A6/2 -A1];
b=[0 -(A2*sqrt(3))/2 -(A3*sqrt(3))/2 0 (A5*sqrt(3))/2 (A6*sqrt(3))/2 0];
if isPlot
figure
plot(a, b, '--bo','LineWidth',1.3)
axis([-1.5 1.5 -1.5 1.5]);
set(gca,'FontName','Times New Roman','FontSize',12);
hold on
%grid
end
% Calculating the True positive (TP), False Negative (FN), False Positive...
% (FP),True Negative (TN), Classification Accuracy (CA), Sensitivity (SE), Specificity (SP),...
% Kappa (K) and F measure (F_M) metrics
PositiveClass=max(ActualLabel);
NegativeClass=min(ActualLabel);
cp=classperf(ActualLabel,PredictedLabel,'Positive',PositiveClass,'Negative',NegativeClass);
CM=cp.DiagnosticTable;
TP=CM(1,1);
FN=CM(2,1);
FP=CM(1,2);
TN=CM(2,2);
CA=cp.CorrectRate;
SE=cp.Sensitivity; %TP/(TP+FN)
SP=cp.Specificity; %TN/(TN+FP)
Pr=TP/(TP+FP);
Re=TP/(TP+FN);
F_M=2*Pr*Re/(Pr+Re);
FPR=FP/(TN+FP);
TPR=TP/(TP+FN);
K=TP/(TP+FP+FN);
[X1,Y1,T1,AUC] = perfcurve(ActualLabel,PredictedLabel,PositiveClass);
%ActualLabel(1) means that the first class is assigned as positive class
%plotting the calculated CA, SE, SP, AUC, K and F_M on polygon
x=[-CA -SE/2 SP/2 AUC K/2 -F_M/2 -CA];
y=[0 -(SE*sqrt(3))/2 -(SP*sqrt(3))/2 0 (K*sqrt(3))/2 (F_M*sqrt(3))/2 0];
if isPlot
plot(x, y, '-ko','LineWidth',1)
set(gca,'FontName','Times New Roman','FontSize',12);
% shadowFill(x,y,pi/4,80)
fill(x, y,[0.8706 0.9216 0.9804])
end
%calculating the PAM value
% Get the number of vertices
n = length(x);
% Initialize the area
p_area = 0;
% Apply the formula
for i = 1 : n-1
p_area = p_area + (x(i) + x(i+1)) * (y(i) - y(i+1));
end
p_area = abs(p_area)/2;
%Normalization of the polygon area to one.
PA=p_area/2.59807;
if isPlot
%Plotting the Polygon
plot(0,0,'r+')
plot([0 -A1],[0 0] ,'--ko')
text(-A1-0.3, 0,'CA','FontWeight','bold','FontName','Times New Roman')
plot([0 -A2/2],[0 -(A2*sqrt(3))/2] ,'--ko')
text(-0.59,-1.05,'SE','FontWeight','bold','FontName','Times New Roman')
plot([0 A3/2],[0 -(A3*sqrt(3))/2] ,'--ko')
text(0.5, -1.05,'SP','FontWeight','bold','FontName','Times New Roman')
plot([0 A4],[0 0] ,'--ko')
text(A4+0.08, 0,'AUC','FontWeight','bold','FontName','Times New Roman')
plot([0 A5/2],[0 (A5*sqrt(3))/2] ,'--ko')
text(0.5, 1.05,'J','FontWeight','bold','FontName','Times New Roman')
plot([0 -A6/2],[0 (A6*sqrt(3))/2] ,'--ko')
text(-0.65, 1.05,'FM','FontWeight','bold','FontName','Times New Roman')
set(gca,'FontName','Times New Roman','FontSize',12);
grid
daspect([1 1 1])
end
Metrics.PA=PA;
Metrics.CA=CA;
Metrics.SE=SE;
Metrics.SP=SP;
Metrics.AUC=AUC;
Metrics.K=K;
Metrics.F_M=F_M;
categories = {'多边形面积PAM';'分类准确率';'灵敏度';'特异性';'曲线下面积AUC';'Kappa系数'; 'F_measure'};
printVar = cell(7,2);
printVar(:,1)=categories;
printVar(:,2)={PA, CA, SE, SP, AUC, K, F_M};
disp('预测结果打印:')
for i=1:length(categories)
fprintf('%23s: %.2f \n', printVar{i,1}, printVar{i,2})
end
没有合适的资源?快使用搜索试试~ 我知道了~
遗传粒子群混合算法优化BP神经网络回归预测(GAPSO-BP),融合遗传算法的粒子群算法优化BP神经网络回归预测,多变量输入单输
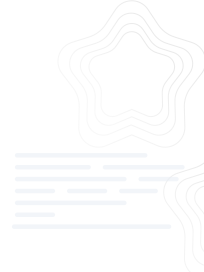
共7个文件
m:6个
xlsx:1个


温馨提示
遗传粒子群混合算法优化BP神经网络回归预测(GAPSO-BP),融合遗传算法的粒子群算法优化BP神经网络回归预测,多变量输入单输出模型。 评价指标包括:R2、MAE、MSE、RMSE和MAPE等,代码质量极高,方便学习和替换数据。
资源推荐
资源详情
资源评论
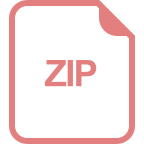
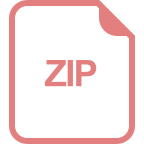
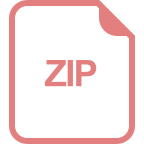
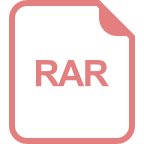
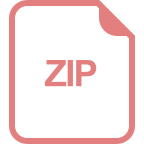
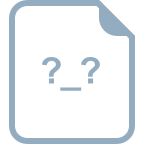
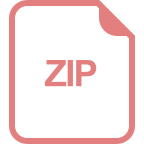
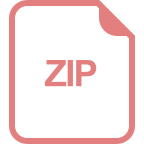
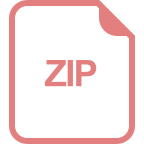
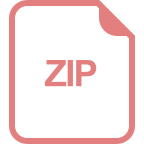
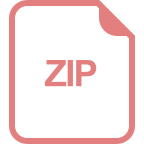
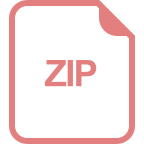
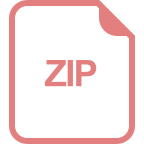
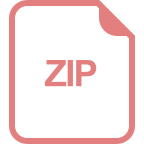
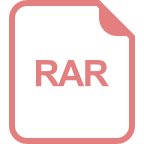
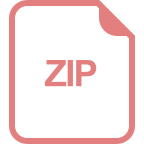
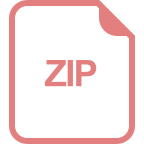
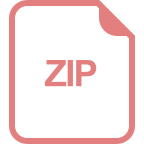
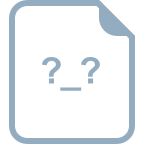
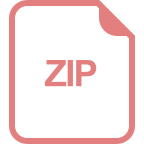
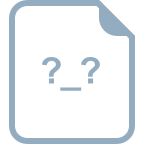
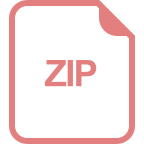
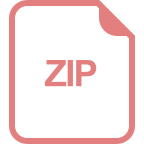
收起资源包目录

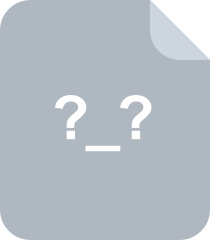
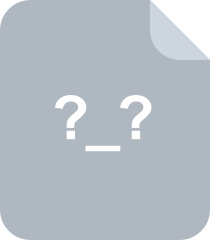
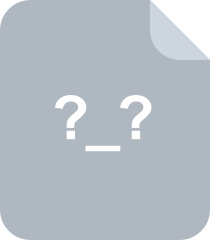
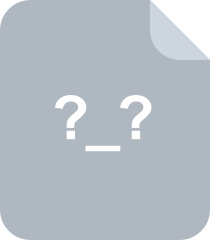
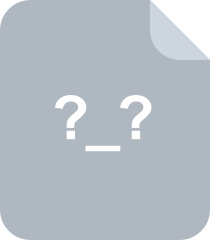
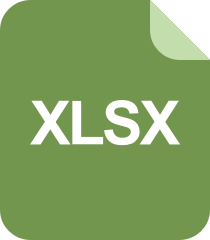
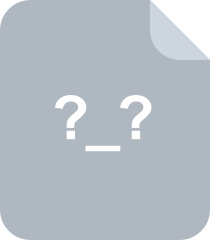
共 7 条
- 1
资源评论
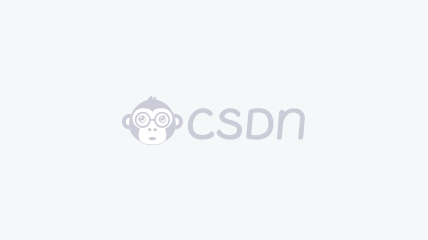
- 普通网友2023-10-12请问有没有一套完整的代码?主函数运行出错

智能算法及其模型预测
- 粉丝: 2438
- 资源: 871
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

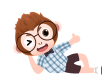
最新资源
- 学校课程软件工程常见10道题目以及答案demo
- javaweb新手开发中常见的目录结构讲解
- 新手小白的git使用的手册入门学习demo
- 基于Java观察者模式的info-express多对多广播通信框架设计源码
- 利用python爬取豆瓣电影评分简单案例demo
- 机器人开发中常见的几道问题以及答案demo
- 基于SpringBoot和layuimini的简洁美观后台权限管理系统设计源码
- 实验报告五六代码.zip
- hdw-dubbo-ui基于vue、element-ui构建开发,实现后台管理前端功能.zip
- (Grafana + Zabbix + ASP.NET Core 2.1 + ECharts + Dapper + Swagger + layuiAdmin)基于角色授权的权限体系.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


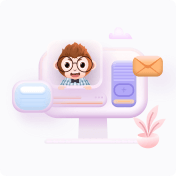
安全验证
文档复制为VIP权益,开通VIP直接复制
