package automobileLeasingManagementSystem;
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.FlowLayout;
import java.awt.Font;
import java.awt.Toolkit;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.FocusEvent;
import java.awt.event.FocusListener;
import java.io.UnsupportedEncodingException;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import java.util.zip.DataFormatException;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.JTextField;
import javax.swing.ScrollPaneConstants;
class AutomobileMessage implements ActionListener {
JFrame jf, jf1, jf2, jf3;
Dimension d = Toolkit.getDefaultToolkit().getScreenSize();
JButton insert, delete, alter, search, showAll,backMenu;
boolean flag = false;
AutomobileMessageInsert ami = null;
AutomobileMessageManagement amm = null;
AutomobileMessageShown ams = null;
JButton sure, back;
JTextArea area;
automobileLeasingManagementSystem.AutomobileMessage.AutomobileMessageInsert.FocusHandler fh;
private String dbUrl = "jdbc:mysql://localhost:3306/automobile?serverTimezone=UTC&useUnicode=true&characterEncoding=utf8&useSSL=false&allowPublicKeyRetrieval=true";
private String dbUser = "Alan";
private String password = "1234";
public AutomobileMessage() {
jf = new JFrame("汽车基本信息管理");
jf.setLayout(new FlowLayout());
JPanel p1 = new JPanel();
JPanel p2 = new JPanel();
JPanel p3 = new JPanel();
JPanel p4 = new JPanel();
JPanel p5 = new JPanel();
JPanel p6 = new JPanel();
insert = new JButton("增添信息");
delete = new JButton("删除信息");
alter = new JButton("修改信息");
search = new JButton("查询信息");
showAll = new JButton("查看所有汽车信息");
backMenu = new JButton("返回主菜单");
insert.addActionListener(this);
delete.addActionListener(this);
alter.addActionListener(this);
search.addActionListener(this);
showAll.addActionListener(this);
backMenu.addActionListener(this);
p1.setBackground(Color.BLACK);
p2.setBackground(Color.BLACK);
p3.setBackground(Color.BLACK);
p4.setBackground(Color.BLACK);
p5.setBackground(Color.BLACK);
p6.setBackground(Color.BLACK);
p1.add(insert);
p2.add(delete);
p3.add(alter);
p4.add(search);
p5.add(showAll);
p6.add(backMenu);
jf.add(p1);
jf.add(p2);
jf.add(p3);
jf.add(p4);
jf.add(p5);
jf.add(p6);
jf.getContentPane().setBackground(Color.BLACK);
int width = 520;
int height = 140;
jf.setBounds((d.width - width) / 2, (d.height - height) / 2, width, height);
jf.setVisible(true);
}
public void actionPerformed(ActionEvent e) {
try {
if (e.getSource() == insert) {
ami = new AutomobileMessageInsert("插入",0);
} else if (e.getSource() == delete) {
amm = new AutomobileMessageManagement("删除");
} else if (e.getSource() == alter) {
amm = new AutomobileMessageManagement("修改");
} else if (e.getSource() == search) {
amm = new AutomobileMessageManagement("查询");
} else if (e.getSource() == showAll) {
ams = new AutomobileMessageShown("所有汽车信息");
ams.showAll();
} else if (e.getSource() == backMenu) {
jf.dispose();
}
} catch (SQLException e1) {
e1.printStackTrace();
} catch (UnsupportedEncodingException e1) {
e1.printStackTrace();
}
}
class AutomobileMessageInsert {
JLabel jl1, jl2, jl3, jl4, jl5, jl6, jl7;
JTextField jt1, jt2, jt3, jt4, jt5, jt6, jt7;
Pattern pattern = Pattern.compile("^[1-9]\\d*$");
Pattern pattern1 = Pattern.compile("^[+]{0,1}(\\d+)$");
Pattern pattern2 = Pattern.compile("^((0{1}\\.\\d+)|([1-9]\\d*\\.{1}\\d+)|([1-9]+\\d*)|0)$");
Matcher matcher;
private final String conditions="正常待租";
Connection con;
Statement stmt;
void clearJTextField() {
jt1.setText("");
jt2.setText("");
jt3.setText("");
jt4.setText("");
jt5.setText("");
jt6.setText("");
jt7.setText("");
}
public AutomobileMessageInsert(String s,long Cnum) {
jf1 = new JFrame(s+"汽车信息");
jf1.setLayout(new FlowLayout());
jl1 = new JLabel("车号");
jl2 = new JLabel("车型");
jl3 = new JLabel("颜色");
jl4 = new JLabel("购置年份");
jl5 = new JLabel("保险情况(有/无)");
jl6 = new JLabel("现存油量(单位:mL)");
jl7 = new JLabel("一天租金(单位:元)");
sure = new JButton("确定");
back = new JButton("返回");
jt1 = new JTextField(15);
jt2 = new JTextField(15);
jt3 = new JTextField(15);
jt4 = new JTextField(15);
jt5 = new JTextField(10);
jt6 = new JTextField(10);
jt7 = new JTextField(15);
fh = new FocusHandler();
jt1.addFocusListener(fh);
jt4.addFocusListener(fh);
jt6.addFocusListener(fh);
jt7.addFocusListener(fh);
JPanel p1 = new JPanel();
JPanel p2 = new JPanel();
JPanel p3 = new JPanel();
JPanel p4 = new JPanel();
JPanel p5 = new JPanel();
JPanel p6 = new JPanel();
JPanel p7 = new JPanel();
JPanel p8 = new JPanel();
p1.add(jl1);
p1.add(jt1);
p2.add(jl2);
p2.add(jt2);
p3.add(jl3);
p3.add(jt3);
p4.add(jl4);
p4.add(jt4);
p5.add(jl5);
p5.add(jt5);
p6.add(jl6);
p6.add(jt6);
p7.add(jl7);
p7.add(jt7);
p7.add(sure);
p7.add(back);
area = new JTextArea(8, 45);
area.setEditable(false);
Font f = new Font("仿宋",Font.PLAIN,20);
area.setFont(f);
jf1.add(p1);
jf1.add(p2);
jf1.add(p3);
jf1.add(p4);
jf1.add(p5);
jf1.add(p6);
jf1.add(p7);
jf1.add(p8);
JScrollPane js = new JScrollPane(area);
js.setVerticalScrollBarPolicy(ScrollPaneConstants.VERTICAL_SCROLLBAR_ALWAYS);
js.setHorizontalScrollBarPolicy(ScrollPaneConstants.HORIZONTAL_SCROLLBAR_ALWAYS);
jf1.add(js);
int width = 550;
int height = 420;
jf1.setBounds((d.width - width) / 2, (d.height - height) / 2, width, height);
jf1.setVisible(true);
sure.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(s.equals("插入")) {
try {
Class.forName("com.mysql.cj.jdbc.Driver");
con = DriverManager.getConnection(dbUrl, dbUser, password);
PreparedStatement addStmt = con.prepareStatement(
"insert into AutomobileMessage(Cnumber,Cmodel,Ccolor,Cdate,Cinsurance,Coil,Crent,conditions)values(?,?,?,?,?,?,?,?)");
long Cnumber = Long.parseLong(jt1.getText().trim());
String Cmodel = jt2.getText().trim();
String Ccolor = jt3.getText().trim();
String Cdate = jt4.getText().trim();
String Cinsurance = jt5.getText().trim();
int Coil = Integer.parseInt(jt6.getText().trim());
matcher = pattern2.matcher(jt7.getText().trim());
if (!matcher.matches()) {
JOptionPane.showMessageDialog(jt7, "请您输入一个大于等于0的数值型数据!");
jt7.setText("");
jt7.requestFocus();
return;
}
if(jt1.getText().equals("")||jt2.getText().equals("")||jt4.getText().equals("")||jt7.getText().equals("")) {
JOptionPane.showMessageDialog(null, "请您将信息补充完整!");
return;
}
double Crent = Double.parseDouble(jt7.getText().trim());
addStmt.setLong(1, Cnumber);
addStmt.setString(2, Cmodel);
addStmt.setString(3, Ccolor);
addStmt.setString(4, Cdate);
addStmt.setString(5, Cinsurance);
addStmt.setInt(6, Coil);
addStmt.setDouble(7, Crent);
addStmt.setStri
没有合适的资源?快使用搜索试试~ 我知道了~
资源详情
资源评论
资源推荐
收起资源包目录


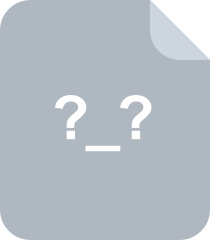


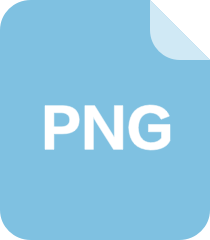
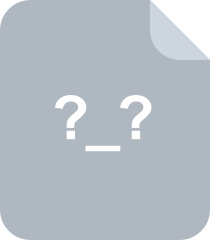
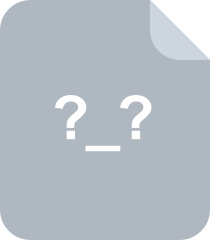
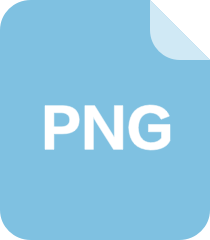
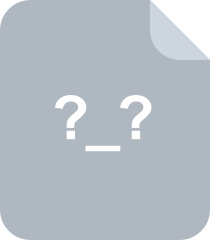
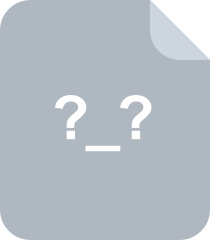
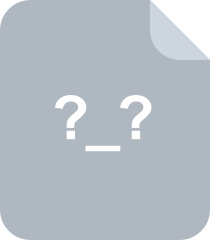
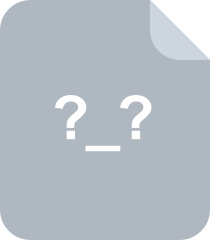
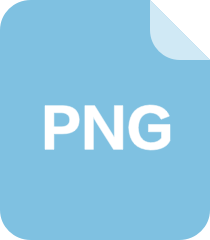
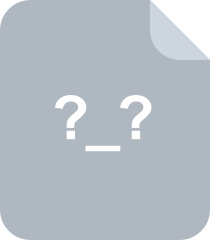
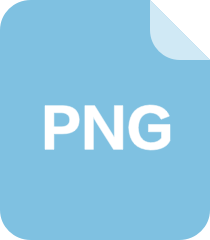
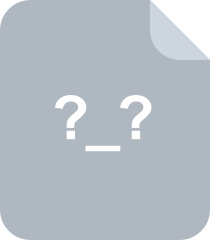
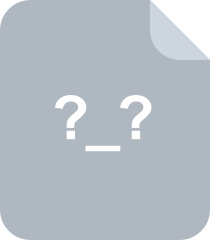
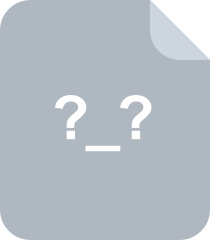
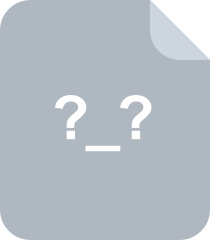
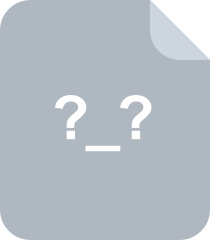
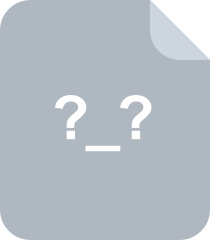
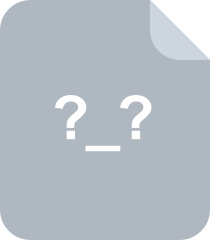
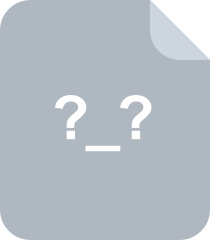
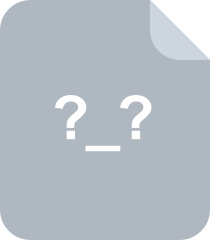
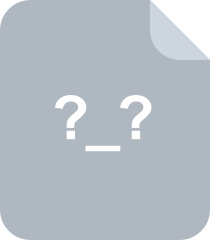
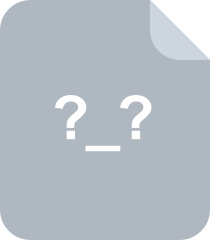
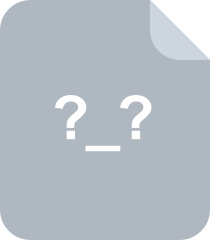
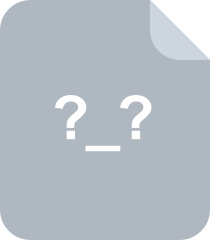
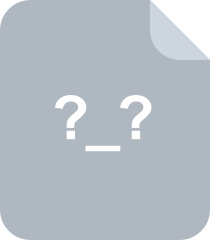
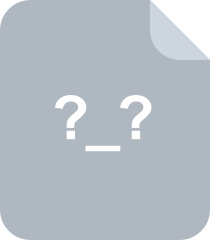
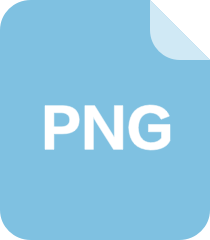
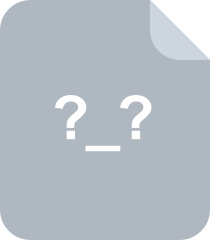
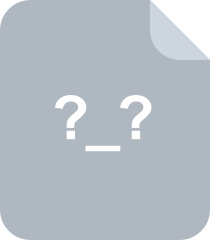
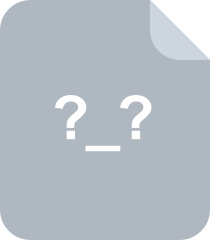
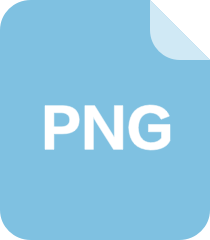
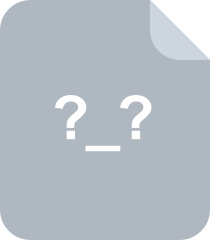
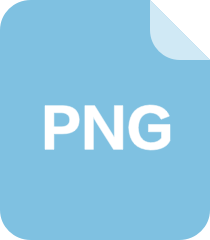
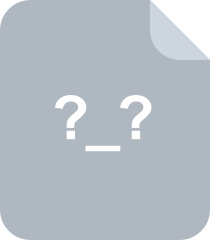

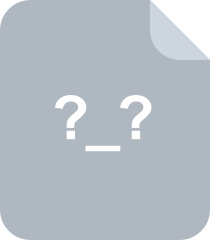


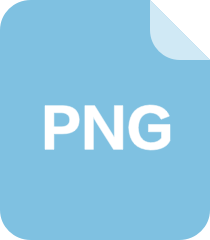
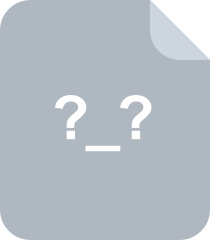
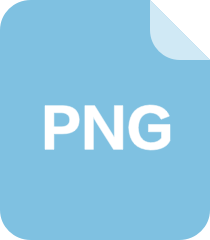
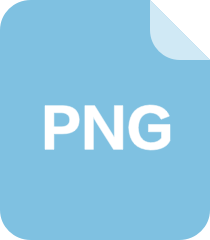
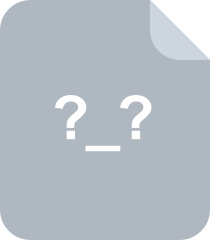
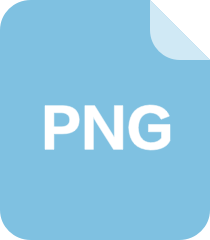
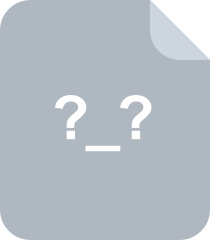
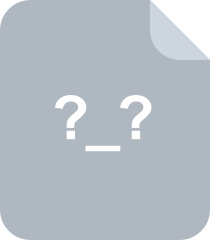
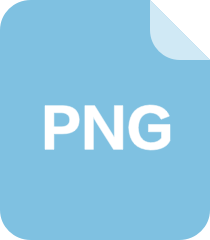
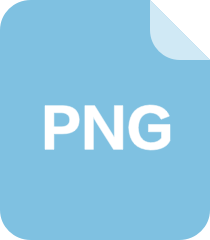
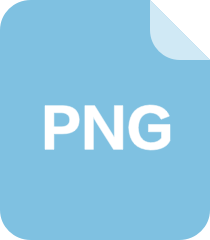
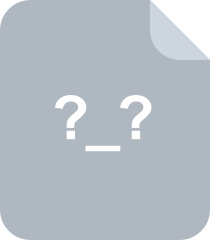

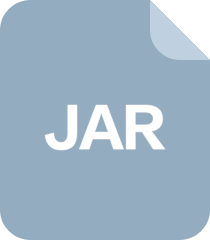
共 49 条
- 1
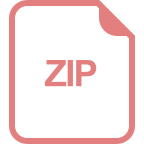
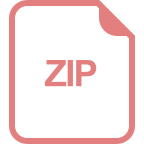
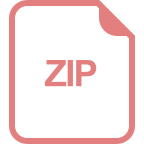
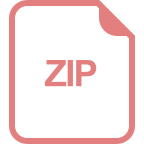
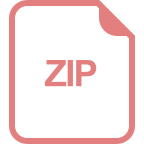
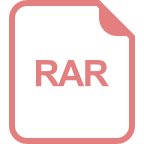
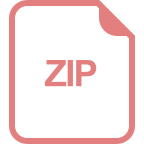
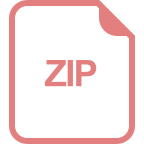
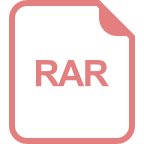
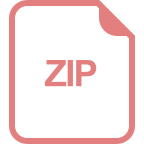
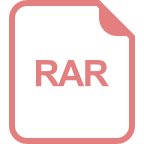
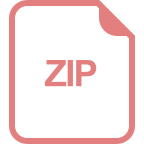
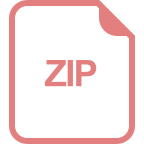
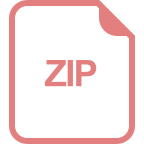
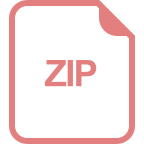
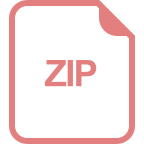
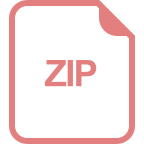
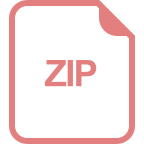
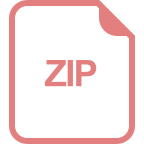
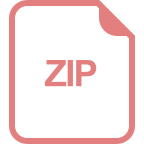
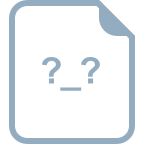
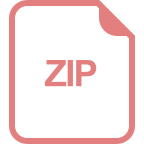
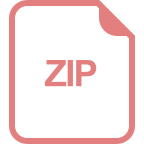
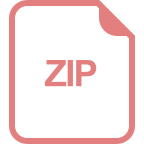
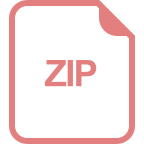
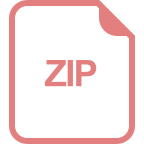

Dijkstra'sMonk-ey
- 粉丝: 150
- 资源: 17
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

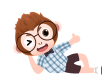
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


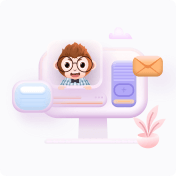
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0