package com.hsk.utils;
import cn.hutool.core.collection.CollUtil;
import cn.hutool.core.io.FileUtil;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.hsk.entity.CoCo.*;
import com.hsk.entity.voc.VocImage;
import com.hsk.entity.voc.VocItem;
import io.micrometer.common.util.StringUtils;
import lombok.extern.slf4j.Slf4j;
import javax.imageio.ImageIO;
import java.awt.image.BufferedImage;
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.*;
import java.util.concurrent.atomic.AtomicInteger;
import java.util.stream.Collectors;
/**
* @author: huangshikai
* @Date: 2024/5/2210:20
* @Description:数据集工具类
*/
@Slf4j
public class DataSetUtil {
// 图片路径
private static final String IMAGE_PATH = "images";
// 标注信息路径
private static final String ANNOTATION_PATH = "annotations";
/*
* @return void
* @Author HuangShiKai
* @Description ocr数据集转coco数据集
* @Date 2024/5/22 10:25
* @Param [labelTxt(标注信息文件), targetPath(输出路径)]
**/
public static void ocrDataSetToCoco(String labelTxtPath, String targetPath) {
Path labelPath = Path.of(labelTxtPath);
if (!Files.exists(labelPath) || !Files.isRegularFile(labelPath)) {
log.error("Label file does not exist or is not a regular file: {}", labelTxtPath);
return;
}
DataSet dataSet = new DataSet();
try {
// 获取Voc数据集
List<VocImage> vocImages = getVocDataSet(labelPath.toFile());
if (CollUtil.isNotEmpty(vocImages)) {
// 获取分类
Map<String, Integer> vocKeyCls = getVocKeyCls(vocImages);
// 解析Image
String imageParentPath = labelPath.getParent().toString();
dataSet.setImages(analysisImages(vocImages, imageParentPath));
dataSet.setAnnotations(analysisAnnotations(vocImages, vocKeyCls));
dataSet.setCategories(analysisCategories(vocKeyCls));
//转为json
ObjectMapper objectMapper = new ObjectMapper();
String json = objectMapper.writeValueAsString(dataSet);
// 创建目录
createDir(targetPath);
//创建json文件
String jsonDir = targetPath + File.separator + "annotations";
Path jsonPath = Path.of(jsonDir);
Files.write(jsonPath.resolve("coco.json"), json.getBytes());
// 复制图片
copyImage(imageParentPath, targetPath, dataSet.getImages());
}
} catch (Exception e) {
log.error("Error parsing label file: {}", labelTxtPath, e);
return;
}
}
/*
* @return java.util.List<com.hsk.entity.voc.VocImage>
* @Author HuangShiKai
* @Description 将Voc数据集转为对象
* @Date 2024/5/22 13:56
* @Param [labelTxtPath(labelTxt文件路径)]
**/
public static List<VocImage> getVocDataSet(File labelTxtPath) {
List<VocImage> vocImages = new ArrayList<>();
ObjectMapper objectMapper = new ObjectMapper();
// 读取label文件
try (BufferedReader bufferedReader = new BufferedReader(new FileReader(labelTxtPath));) {
String line;
AtomicInteger id = new AtomicInteger(1);
while ((line = bufferedReader.readLine()) != null) {
String fileName = line.split("\t")[0];
String itemsJson = line.split("\t")[1];
if (StringUtils.isNotBlank(fileName) && StringUtils.isNotBlank(itemsJson)) {
// 处理文件名,只保留文件名部分
fileName = fileName.substring(fileName.lastIndexOf("/") + 1);
VocImage vocImage = new VocImage();
vocImage.setFileName(fileName);
vocImage.setId(id.getAndIncrement());
// 将标签信息反序列化为对象
vocImage.setItems(objectMapper.readValue(itemsJson, new TypeReference<>() {
}));
vocImages.add(vocImage);
}
}
} catch (Exception e) {
log.error("读取Voc数据集失败");
}
return vocImages;
}
/*
* @return java.util.Map<java.lang.String,java.lang.Integer>
* @Author HuangShiKai
* @Description //把分类放入内存
* @Date 2024/5/22 16:29
* @Param [vocImages]
**/
public static Map<String, Integer> getVocKeyCls(List<VocImage> vocImages) {
Map<String, Integer> vocKeyCls = new HashMap<>();
// 用于生成唯一索引,从1开始
AtomicInteger index = new AtomicInteger(1);
vocImages.parallelStream()
.flatMap(vocImage -> vocImage.getItems().parallelStream())
.forEach(vocItem -> {
// 获取分类
vocKeyCls.computeIfAbsent(vocItem.getKeyCls(), k -> index.getAndIncrement());
});
return vocKeyCls;
}
/*
* @return java.util.List<com.hsk.entity.CoCo.Image>
* @Author HuangShiKai
* @Description 解析Images
* @Date 2024/5/22 16:49
* @Param [vocImages]
**/
public static List<Image> analysisImages(List<VocImage> vocImages, String imageParentPath) {
if (vocImages == null || vocImages.isEmpty()) {
return Collections.emptyList();
}
List<Image> images = new ArrayList<>();
try {
// 获取图片名及id
images = vocImages.parallelStream()
.map(vocImage -> {
Image image = new Image();
// 从map中获取id
image.setId(vocImage.getId());
image.setFileName(vocImage.getFileName());
BufferedImage bufferedImage = null;
try {
String imagePath = imageParentPath + File.separator + vocImage.getFileName();
bufferedImage = ImageIO.read(new File(imagePath));
image.setHeight(bufferedImage.getHeight());
image.setWidth(bufferedImage.getWidth());
} catch (IOException e) {
log.error("读取图片信息失败: {}", vocImage.getFileName(), e);
}
return image;
}).collect(Collectors.toList());
} catch (Exception e) {
log.error("解析Images失败:", e);
}
return images;
}
/*
* @return java.util.List<com.hsk.entity.CoCo.Annotation>
* @Author HuangShiKai
* @Description 解析Annotations
* @Date 2024/5/22 18:42
* @Param [vocImages]
**/
private static List<Annotation> analysisAnnotations(List<VocImage> vocImages, Map<String, Integer> vocKeyCls) {
List<Annotation> annotations = new ArrayList<>();
try {
AtomicInteger id = new AtomicInteger(1);
vocImages.forEach(vocImage -> {
// 根据文件名分组,获取vocimage对象
Map<String, List<VocItem>> vocItemMap = vocImage.getItems().parallelStream().collect(Collectors.groupingBy(VocItem::getKeyCls));
vocItemMap.forEach((keyCls, vocItems) -> {
Annotation annotation = new Annotation();
annotation.setId(id.getAndIncrement());
// 图片id
annotation.setImageId(vocImage.getId());
// 分类id
annotation.setCategoryId(vocKeyCls.get(keyCls));
ExtraInfo extraInfo = new ExtraInfo();
extraInfo.setLab

Java程序员-张凯
- 粉丝: 1w+
- 资源: 7528
最新资源
- 纤维混凝土,纤维骨料细观尺度混凝土模型,可控制骨料,纤维的尺寸和体积率 四面体网格划分及六面体网格投影 模型可用于abaqus Ansys ls-dyna flac3d等有限元软件
- 户外储能电源方案双向逆变器板资料,原理文件,PCB文件,源代码,电感与变压器规格参数,户外储能电源2KW(最大3KW)双向逆变电源生产资料,本生产资料含有前级DCDC源程序,后级的SPWM 本户外储能
- maxwell外转子电机设计,外转子电机电磁仿真
- Shizuku_.apk
- 光储交直流微电网离并网变 仿真模型由光伏PV及其DC DC变器、储能及其双向DC DC变器、直流负载、逆变器、交流负载、断路器以及交流主网组成的光储交直流微电网 光储交直流电网 运行目标: 储能控制
- prescan,carsim,simulink三软件联合仿真,实现弯道超车,避撞前方机动车,使用frent坐标系下五次多项式规划加模型预测控制,有横向轨迹跟踪对比图,仿真图 可包调试运行 需要安装
- 模拟IC设计,Sigma-delta ADC,三阶单环Sigma-Delta 调制器,实际电路和仿真状态,enob为16;并有文档相关说明;用好需要有一定的模拟IC基础,适合sd adc入门用
- 双向Buck-Boost电路仿真,闭环控制算法
- Comsol等离子体仿真,Ar细通道棒板流注放电 电子密度,电子温度等
- Comsol等离子体仿真,Ar棒板粗通道流注放电 电子密度,电子温度,电场强度等 5.5,6.0版本
- 2408统计表 ,2408统计表
- COMSOL电磁超声仿真: Crack detection in L-shaped aluminum plate via electromagnetic ultrasonic measurements
- Comsol波导BIC
- 光伏储能基于VSG同步发电机控制的并网仿真模型 基于Matlab Simulink仿真平台 储能为buck-boost电路(双向DC DC变) 光伏为boost电路 主电路采用三相全桥PWM逆变器 1
- 斜碰,侧碰,偏置碰撞有限元模型,ls dyna
- 两极式单相光伏并网仿真 前极:Boost电路+电导增量法 后极:桥式逆变+L型滤波+电压外环电流内环控制 并网电流和电网电压同频同相,单位功率因数并网,谐波失真率0.39%,并网效率高 有配套vid
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


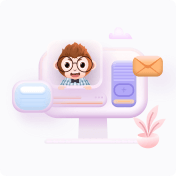