#include"linux_serial.h"
#include <stdio.h> /*标准输入输出定义*/
#include <stdlib.h> /*标准函数库定义*/
#include <unistd.h> /*Unix标准函数定义*/
#include <math.h>
#include <sys/types.h> /**/
#include <sys/stat.h> /**/
#include <fcntl.h> /*文件控制定义*/
#include <termios.h> /*PPSIX终端控制定义*/
#include <errno.h> /*错误号定义*/
#include "opencv/highgui.h"
#include "opencv/cv.h"
#define FALSE -1
#define TRUE 0
/***@brief 设置串口通信速率
*@param fd 类型 int 打开串口的文件句柄
*@param speed 类型 int 串口速度
*@return void*/
int speed_arr[] = { B38400, B19200, B9600, B4800, B2400, B1200, B300,
B38400, B19200, B9600, B4800, B2400, B1200, B300, };
int name_arr[] = {38400, 19200, 9600, 4800, 2400, 1200, 300,
38400, 19200, 9600, 4800, 2400, 1200, 300, };
/*
unsigned char head[2];
unsigned char datax[2];
unsigned char datay[2];
unsigned char dataf[2];
*/
unsigned char data[8];
void set_speed(int fd, int speed)
{
int i;
int status;
struct termios Opt;
tcgetattr(fd, &Opt);
for ( i= 0; i < sizeof(speed_arr) / sizeof(int); i++)
{
if (speed == name_arr[i])
{
tcflush(fd, TCIOFLUSH);
cfsetispeed(&Opt, speed_arr[i]);
cfsetospeed(&Opt, speed_arr[i]);
status = tcsetattr(fd, TCSANOW, &Opt);
if (status != 0)
perror("tcsetattr fd1");
return;
}
tcflush(fd,TCIOFLUSH);
}
}
/**
*@brief 设置串口数据位,停止位和效验位
*@param fd 类型 int 打开的串口文件句柄*
*@param databits 类型 int 数据位 取值 为 7 或者8*
*@param stopbits 类型 int 停止位 取值为 1 或者2*
*@param parity 类型 int 效验类型 取值为N,E,O,,S
*/
int set_Parity(int fd,int databits,int stopbits,int parity)
{
struct termios options;
if ( tcgetattr( fd,&options) != 0)
{
perror("SetupSerial 1");
return(FALSE);
}
options.c_cflag &= ~CSIZE;
switch (databits) /*设置数据位数*/
{
case 7:
options.c_cflag |= CS7;
break;
case 8:
options.c_cflag |= CS8;
break;
default:
fprintf(stderr,"Unsupported data size\n");
return (FALSE);
}
switch (parity)
{
case 'n':
case 'N':
options.c_cflag &= ~PARENB; /* Clear parity enable */
options.c_iflag &= ~INPCK; /* Enable parity checking */
break;
case 'o':
case 'O':
options.c_cflag |= (PARODD | PARENB); /* 设置为奇效验*/
options.c_iflag |= INPCK; /* Disnable parity checking */
break;
case 'e':
case 'E':
options.c_cflag |= PARENB; /* Enable parity */
options.c_cflag &= ~PARODD; /* 转换为偶效验*/
options.c_iflag |= INPCK; /* Disnable parity checking */
break;
case 'S':
case 's': /*as no parity*/
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
break;
default:
fprintf(stderr,"Unsupported parity\n");
return (FALSE);
}
/* 设置停止位*/
switch (stopbits)
{
case 1:
options.c_cflag &= ~CSTOPB;
break;
case 2:
options.c_cflag |= CSTOPB;
break;
default:
fprintf(stderr,"Unsupported stop bits\n");
return (FALSE);
}
/* Set input parity option */
if (parity != 'n')
options.c_iflag |= INPCK;
options.c_cc[VTIME] = 250; // 15 seconds
options.c_cc[VMIN] = 1;
//设置串口的raw方式
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG); /*Input*/
options.c_oflag &= ~OPOST; /*Output*/
options.c_oflag &= ~OPOST;
options.c_oflag &= ~(ONLCR | OCRNL); //添加的
options.c_iflag &= ~(ICRNL | INLCR);
options.c_iflag &= ~(IXON | IXOFF | IXANY); //添加的
tcflush(fd,TCIFLUSH); /* Update the options and do it NOW */
if (tcsetattr(fd,TCSANOW,&options) != 0)
{
perror("SetupSerial 3");
return (FALSE);
}
return (TRUE);
}
/**
*@breif 打开串口
*/
int OpenDev(char *Dev)
{
int fd = open( Dev, O_RDWR| O_NOCTTY); //| O_NOCTTY | O_NDELAY
if (-1 == fd)
{ /*设置数据位数*/
perror("Can't Open Serial Port");
return -1;
}
else
return fd;
}
//以下是串口发送程序
int send_serial(CvPoint objectPos, int flag, int fd)
{
int nwrite;//写入数据数目
/*
head[0] = 0xaa;
head[1] = 0x55;
datax[0] = objectPos.x;
datax[1] = objectPos.x/256;
datay[0] = objectPos.y;
datay[1] = objectPos.y/256;
dataf[0] =0x01; (unsigned char)flag;
dataf[1] = 0x0a;
*/
data[0] = 0xaa;
data[1] = 0x55;
data[2] = objectPos.x;
data[3] = objectPos.x/256;
data[4] = objectPos.y;
data[5] = objectPos.y/256;
data[6] = (unsigned char)flag;
data[7] = 0x0a;
nwrite = write(fd, data, 8);
/*
nwrite = write(fd, (head), 1);
sleep(0.01);
nwrite = write(fd, (head+1), 1);
sleep(0.01);
nwrite = write(fd, datax, 1);
sleep(0.01);
nwrite = write(fd, (datax+1), 1);
sleep(0.01);
nwrite = write(fd, (datay), 1);
sleep(0.01);
nwrite = write(fd, (datay+1), 1);
sleep(0.01);
nwrite = write(fd, (dataf), 1);
sleep(0.01);
nwrite = write(fd, (dataf + 1), 1);
if (nwrite < 1)
printf("write serial error\n");
*/
printf("send right %x %x %d\n",data[0] ,data[1], nwrite);
return nwrite;
}
int read_serial(int fd)
{
unsigned char data[5];
int nwrite = 0;
nwrite = read(fd, data, 1);
if (nwrite != 1)
printf("read wrong\n");
if (data[0] == 0x0c)
return 0;
else
return -1;
}
/**
*@breif main()
*/
/*
int main(int argc, char **argv)
{
int fd;
int nread;
char buff[512];
char *dev ="/dev/ttyUSB0";
fd = OpenDev(dev);
if (fd>0)
set_speed(fd,4800);
else
{
printf("Can't Open Serial Port!\n");
exit(0);
}
if (set_Parity(fd,8,1,'N')== FALSE)
{
printf("Set Parity Error\n");
exit(1);
}
while(1)
{
nread = read(fd,buff,512);
//buff[nread+1]='\0';
printf("%s",buff);
}
close(fd);
exit(0);
}
*/
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
基于颜色的图像识别与轨迹跟踪。作品名为“基于图像识别的粉笔书写装置”,主要原理为:通过摄像头装置实时读取人手的书写信息,并反馈给上位机,上位机对得到的图像信息进行图像识别相关处理之后,得到相应轨迹的点位信息,对这些点位进行插补得到连续的轨迹,再通过执行装置将轨迹复现在黑板上,完成书写。该作品由四部分构成:图像读取装置(摄像头或工业相机),上位机(个人电脑或嵌入式系统),控制执行装置的 51 单片机系统,执行装置(包括两个步进电机以及丝杠螺母机构等)。上位机为运行 linux 系统的桌面计算机,相应软件功能包括图像处理、音频处理、多进程协同编程、串口通讯等。
资源推荐
资源详情
资源评论
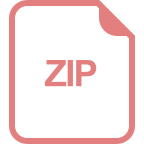
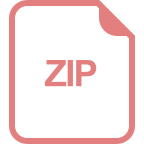
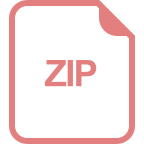
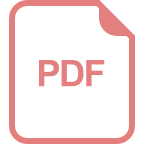
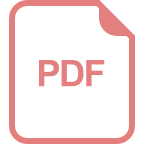
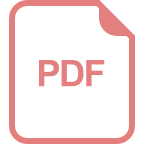
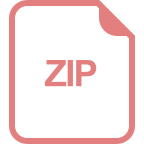
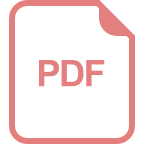
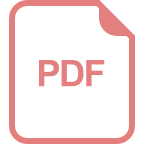
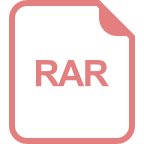
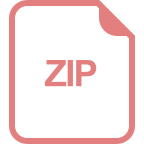
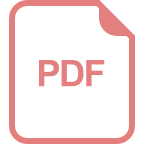
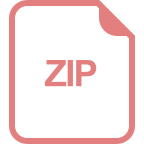
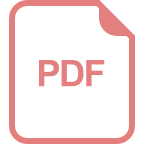
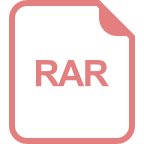
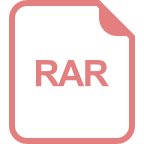
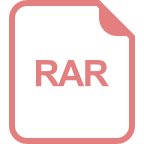
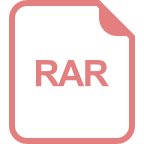
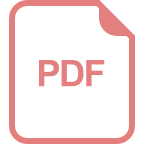
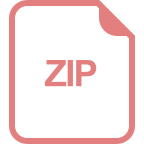
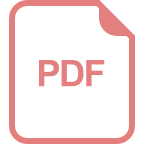
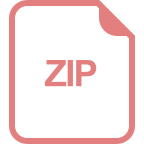
收起资源包目录


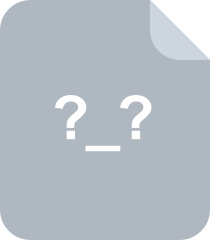
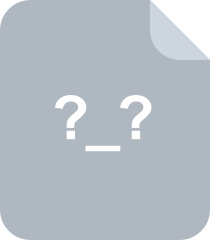
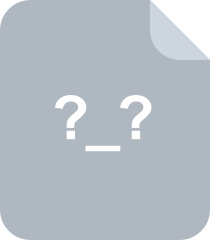
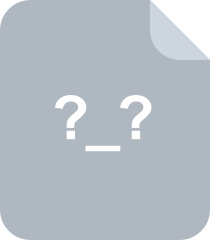
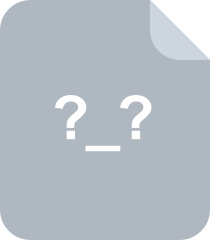
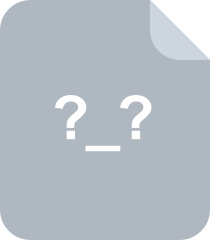
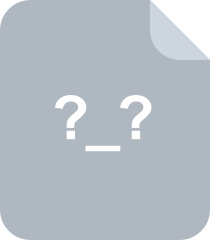
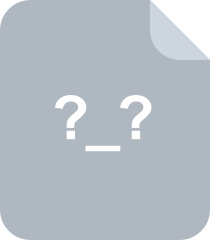
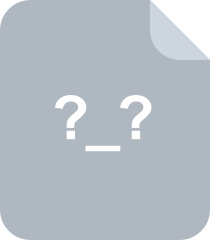
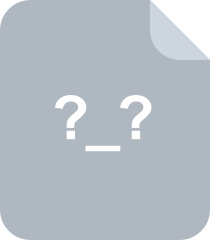
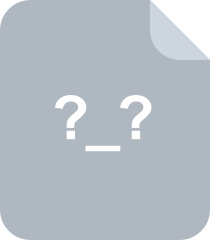
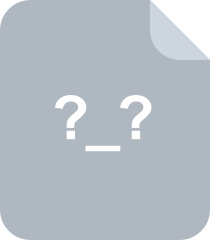
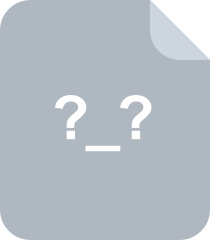
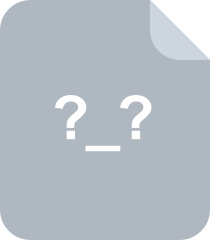
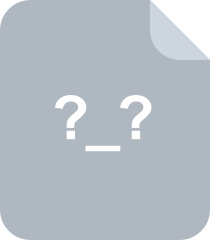
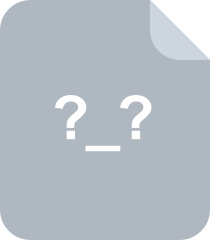
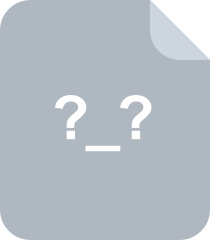
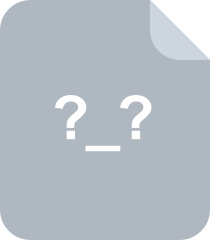
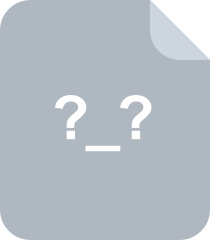
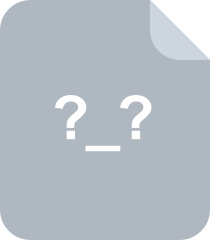
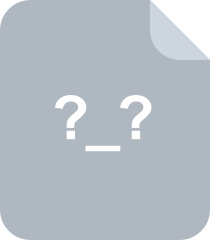
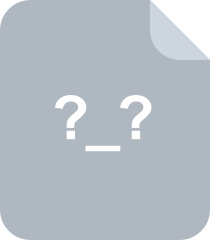
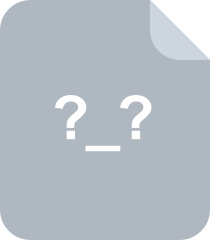
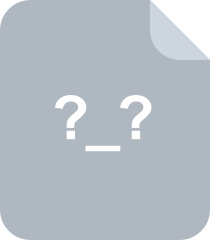
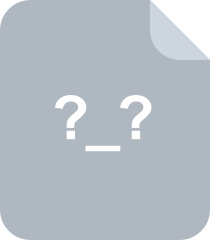
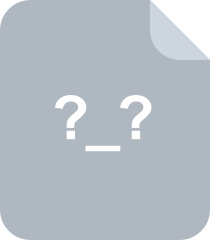
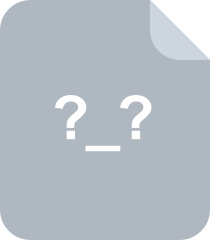
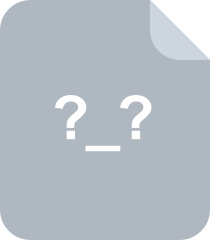
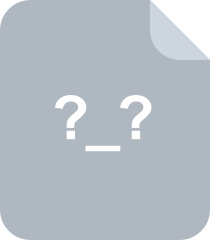
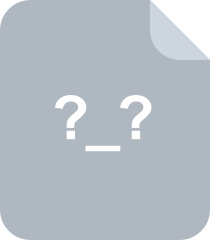
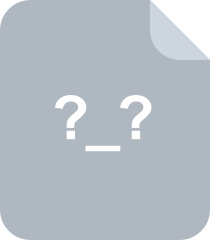
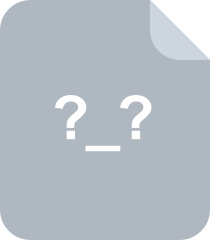
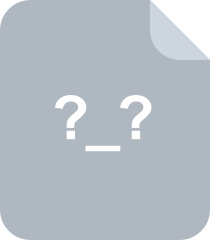
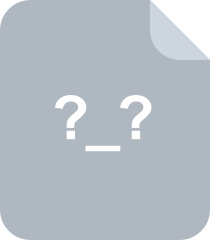
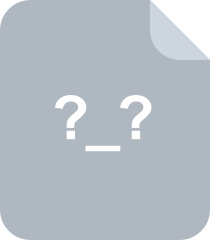
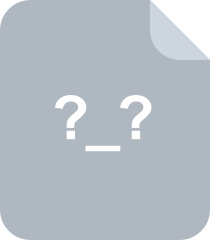
共 36 条
- 1
资源评论
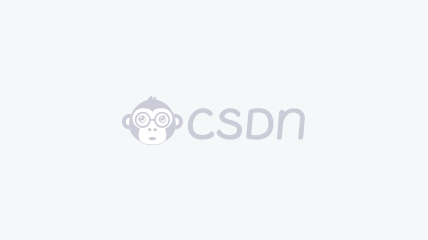
- qq_585721342024-03-06感谢资源主的分享,很值得参考学习,资源价值较高,支持!

Java程序员-张凯
- 粉丝: 1w+
- 资源: 7366

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

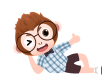
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


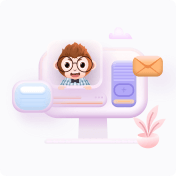
安全验证
文档复制为VIP权益,开通VIP直接复制
